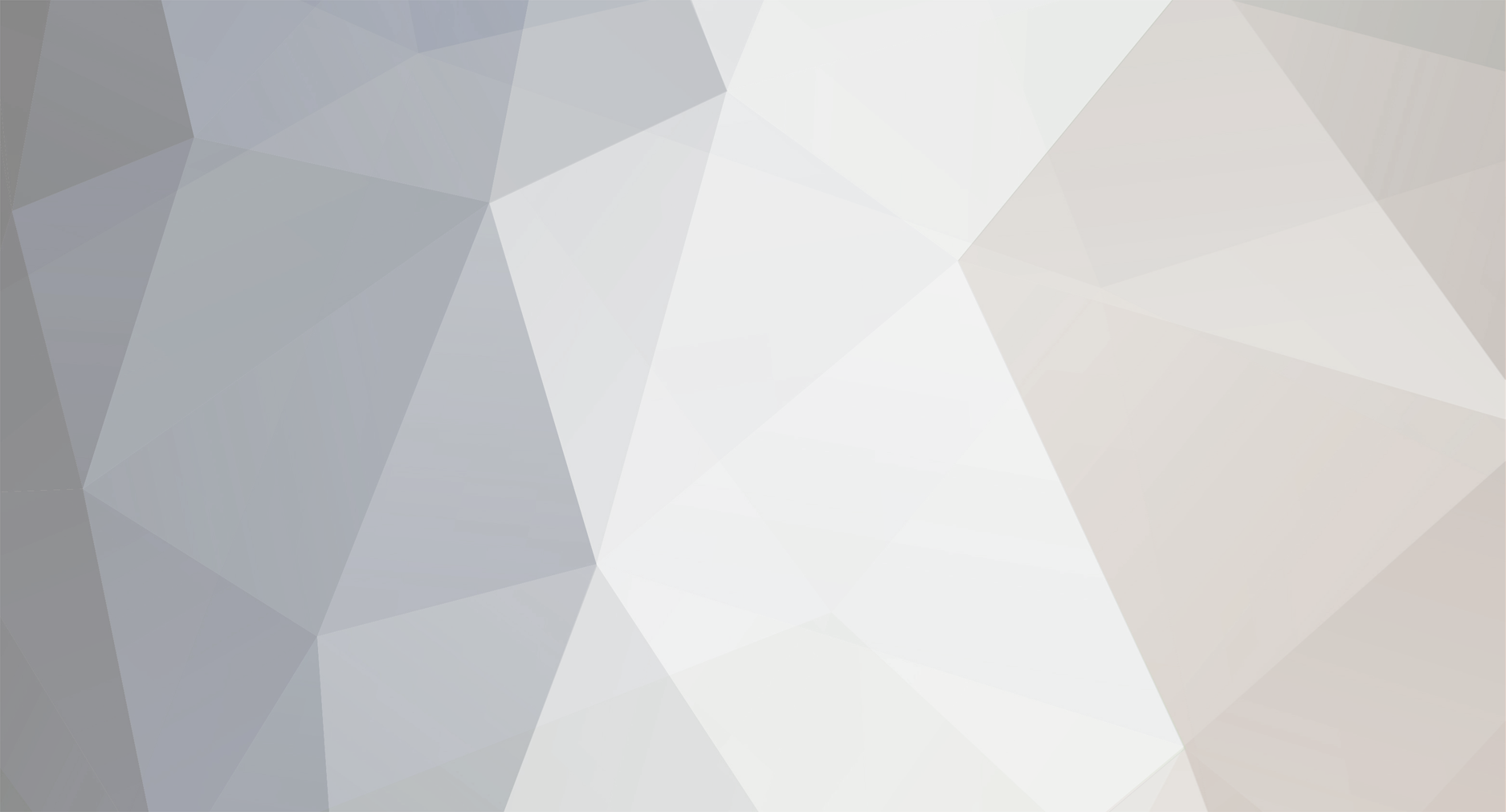
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
If you did [code]if (isset($variable))[/code] Then you would be checking if $variable is set. [code]if (!isset($variable))[/code] checks if $variable is NOT set. Basically, it gives you the opposite result to what you would normally get. Another example: [code]if ($var > 5) { print "$var is greater than 5\n";} if (!($var > 5)) { print "$var is NOT greater than 5\n";}[/code] The second is the exact opposite of the first.
-
I don't have any ".so" file for my extension either. I think it is ok. The extension still works properly. I have these files only (my extension is named c_array): c_array.c c_array.o config.m4 CREDITS php_c_array.h c_array.lo c_array.php config.w32 EXPERIMENTAL tests I am using php-5.1.6 source.
-
It does have an operator (well, a function), called parse_url(). I agree though that it can be hard to find php functions sometimes. Try checking $_SERVER['SCRIPT_URI'] for the the full URL of your script (that's URI, not URL). So, something like [code]$url_parts = parse_url($_SERVER['SERVER_URI']); if ($url_parts['scheme']) == 'http') { $link_to = 'secure'; $address = preg_replace('|^http|', 'https', $_SERVER['SERVER_URI']); } else { $link_to = 'insecure'; $address = preg_replace('|^https|', 'http', $_SERVER['SERVER_URI']); } echo "<a href=\"$address\">$link_to</a>";[/code] The meaning of that preg_replace() is to replace "http" with "https" (or the other way around for the second one), but ONLY at the start (that's why the "^" is there, it means "at the start). The "|" is just a marker for the start and end of the expression, it has no special meaning.
-
Maybe your query failed? Add this line [code]if ($query_auto === false) die("Query $sql_auto failed\n");[/code] My first guess would be that POSTS_TABLE is not defined, or not correctly defined (or not properly escaped). But there are many other reasons for query failure. The die() call above will show you the query contents, which should help you diagnose the problem.
-
Can you give a bit more detail about your situation? You can certainly say [code]if (some test) { require_once('file.php'); }[/code] But it sounds like you want something not so straightforward? Are you trying to detect the name of the script which is including a particular file, from within that included file?
-
That code is sufficiently complex that I can't spend the time to analyze it.. BUT, there are two good testing techniques you can use. The first is to create a test directory structure, complete with test files inside, and see if it handles that correctly. The second is to have it print out everything it will do, but not actually DO anything. Essentially, you will comment out all the parts where files are created or deleted. Then you can read through the list of changes and look for anything odd. The only problem there is that it will fail when a directory should have been renamed. So for directory renaming, you will have to rely on the first testing technique only. I have one concern - I don't see any test to see if $newPath already exists, such as "if (file_exists($newPath)) { ... }"
-
I get a 404 error on that URL. Does your code work? I'm unclear on what your question is. It looks to me like your code will work, as long as there is only one title, category, description and link in each item. There is no need to set $searchfile = eregi(...) if you aren't going to reference that variable later though. You can just call eregi() the same way you call ereg() later in your code.
-
Hi! Your array probably looks like this: [code]$_POST['description'] = array( 'part1', 'part2', '', '', '', '', '', );[/code] So, all you need to do in that foreach loop is to check "if ($description == '') continue;" The "continue" will skip to the next element in the array used by the foreach. Alternatively, you can use "break" if you want to end the foreach immediately. To verify this, try adding the code "print_r($_POST['description'])" or "var_dump($_POST['description'])". Then you can see what the array contents are, and work out how to deal with those empty items. Good luck :)
-
can anyone get me started on adding in better oop support?
btherl replied to drkstr's topic in PHP Coding Help
I think the mailing lists would be the best place to start, to get an idea of what people have been discussing. Looking at the code only can often leave you behind the times. In the source tree in the Zend directory there are a few files, OBJECT2_HOWTO, and zend_object*.c -
To avoid problems like this in future, I recommend you split such code over multiple lines. For example: [code]foreach($_POST['description'] as $key=>$description) { $query = "INSERT INTO table (description,number) VALUES ('" . mysql_real_escape_string($description) . "', " . intval($_POST['number'][$key] . " )"; mysql_query($query) or die(mysql_error().$query); }[/code] This makes it easier to see errors. Another nice convention is: [code]foreach($_POST['description'] as $key=>$description) { $description_esc = mysql_real_escape_string($description); $number = intval($_POST['number'][$key]); $query = "INSERT INTO table (description,number) VALUES (" . "'{$description_esc}', " . "{$number} )"; mysql_query($query) or die(mysql_error().$query); }[/code] This code is much easier to read. And "easy to read" means "fewer bugs" :)
-
Lansing, do you want entries which are in both Table A and Table B, or entries which are in Table A and NOT in Table B? If you want entries in Table A and Table B, then inner join will work. If you want entries in Table A but NOT in Table B, then you need to use this: [code]SELECT a.* FROM $order_table a LEFT OUTER JOIN $commisions_table b ON (a.user_id = b.user_id) WHERE b.user_id IS NULL[/code] This will give you all rows from Table A which does NOT match a row from Table B. This is because an outer join puts NULL in the b.user_id (and all columns from b) if no row from b was found.
-
Hi, You should not be running "./config.m4" directly. Instead, you should edit config.m4, and then run "./buildconf" These are the instructions you should follow (from extskel): "To use your new extension, you will have to execute the following steps: 1. $ cd .. 2. $ vi ext/hello/config.m4 3. $ ./buildconf 4. $ ./configure --[with|enable]-hello 5. $ make 6. $ ./php -f ext/hello/hello.php 7. $ vi ext/hello/hello.c 8. $ make Repeat steps 3-6 until you are satisfied with ext/hello/config.m4 and step 6 confirms that your module is compiled into PHP. Then, start writing code and repeat the last two steps as often as necessary." Good luck, and have fun :)
-
It might be a path issue. Try: [code]setcookie("WFS2U", $userName, time()+60*60*24, '/')[/code] That time()+60*60*24 sets it to expire in 1 day. You have to include an expiry time in order to set the cookie path (yes, it doesn't make sense, it's because of the argument order). Also, remember that $_COOKIE[] only contains cookies received from the browser. You will only see these in the NEXT request, and not in the same request that you set the cookie.
-
Welcome back moon-man :) I think your problem is with variable names. At the top you have $dir = '/tmp/TESTING', and at the bottom you call remove_bar_char($dir). So far so good. But inside remove_bar_char(), your variable names are all mixed up. I will have a go at fixing it: [code] #!/usr/bin/php <?PHP $start_dir = '/tmp/TESTING'; $cnt=0; # The input for remove_bar_char() is always a directory, so I will call it $dir. function remove_bar_char($dir){ global $cnt; # So we can keep a global count $handle = opendir($dir); while(($file = readdir($handle)) !== false){ echo "File found: " .$file ."\n"; if (preg_match('/[' .preg_quote('\/ : * ? " < > |', '/') .']/', $file)){ echo "Bad file found: " .$file ." " ; $cnt++ ; $path = $dir . DIRECTORY_SEPARATOR . $file; if (!in_array($file, array('..', '.')) && is_file($path)){ $contents = file_get_contents($path); $newPath = $dir . DIRECTORY_SEPARATOR . preg_replace('/[' .preg_quote('\/ : * ? " < > |', '/') .']/', '', $file); echo "Replacing with " .$newPath ."\n" ; if (file_put_contents($newPath, $contents) !== false){ unlink($path); //delete old file only if able to create new } } }elseif(is_dir($file)){ remove_bar_char($file) ; } } } echo "<------------Starting Replace!------------>\n" ; echo "\n"; remove_bar_char($start_dir); echo "\n"; echo "\n"; echo "<------------Replace Finnished------------>\n" ; echo "There were " .$cnt ." Replacements Made\n" ; ?>[/code] With functions, you can use a different variable name when you call it. That variable will be renamed within the function. For example: $other_dir = '/tmp/OTHER'; remove_bar_char($other_dir); Even though you called it with $other_dir, you must use just "$dir" within the function, because the function is declared as "function remove_bar_char($dir)". Hope that helps :)
-
Hi, I would like to run this idea by all of you and see if it makes sense. I would like to create an extension that simulates an array of structs in C. What I am replacing is this PHP array: $arr = array( array( 'name' => 'Brian', 'expires' => '2006-12-31', 'active' => true, ), array( ... ), ... ) The replacement will look like this: $c_arr = c_array_create(); c_array_add_elem($c_arr, 'name', 'string'); c_array_add_elem($c_arr, 'expires', 'date'); c_array_add_elem($c_arr, 'active', 'bool'); So much for initialization. The above is the declaration of a structure like this: typedef struct { char *name; date_t expires; bool active; } s_person; The extension will have a pre-defined list of types that it understands. It needs to know types both for efficient storage, and to enable sorting. Then, array entries can be added (to the end only) c_array_insert($c_arr, 'Brian', '2006-12-31', true); And they can be fetched from any location $brian = c_array_fetch($c_arr, 0); Additionally, I would like to implement sorting in C for these arrays (can use qsort with appropriate callbacks) c_array_sort($c_arr, 'name=asc,expires=desc'); I will most likely implement a 'c_array_fetch_serialized()' function too, which will be quite simple due to the limited data types. I have a need for it in my application. Q: Why bother? A: C Structs do not use any space for the names of elements of the structure. C arrays do not use any space for the names of elements of the array. This can lead to considerable saved space, at the expense of reduced flexibility. Q: Why not use C for everything? A: I want it all! I want PHP's high-level power combined with C's efficient storage. Q: How is the data stored and accessed? A: The same as a C struct. Data will be accessed as (array_offset * struct size + struct element offset), and all data is stored in a single (very large) char*. Strings within the array will be a char* pointing to the string itself, as is usual in C. Q: How do you delete data from the middle of an array? A: You can't. But you can filter the data in php before adding it to the array. Any comments? Is it practical? I've never written a PHP extension before, but I am expert in both C and PHP programming, so I am interested in comments from people with experience in writing PHP extensions.
-
You might also need to call session_start() at the top of your page too (before ANY html at all, even blank lines. If you have trouble, check the thread on header errors). You might not need to call it, it depends on your php configuration. Once sessions are active (triggered by session_start()), then any data you place into the $_SESSION[] array will be available between requests.
-
The function is part of an extension which may not be installed in your copy of PHP. In this case it's the GD extension. The main page for a group of functions will tell you if you need a particular extension to use that set of functions. At http://sg.php.net/manual/en/ref.image.php you will see: [quote]To enable GD-support configure PHP --with-gd[=DIR], where DIR is the GD base install directory. To use the recommended bundled version of the GD library (which was first bundled in PHP 4.3.0), use the configure option --with-gd. GD library requires libpng and libjpeg to compile.[/quote] If you are using a binary distribution, then how you enable this extension will depend on your distribution.
-
Thanks for the comments! The reason it's a concern is that I want to process large amounts of data, and I want to sort the entire data set by various keys. The larger data sets are 250k rows, and possibly larger. The data would fit in memory, but the overhead of storing the data is currently much larger than the data itself. Jenk, I'm not sure what you mean by "PHP uses C directly for primitives". As I understand, php uses hash tables for arrays. Zanus, there looks to be a small memory benefit to using integers instead of strings, based on small tests. I will try it and see if it measurably improves memory usage, thanks :) I don't think the underlying structure is different for numerically indexed arrays however. It would be nice if it was :) But memory usage is the same for numerically indexed arrays and arrays indexed by short strings. I would expect lower memory usage if a simple array was being used.
-
The problem here is that you are setting the $friend variable from the result of your "SELECT * ..." query above. Then you are deleting THAT friend. You need to check the $_POST data to see which friend should be deleted. This means that you need to submit the friend to be deleted along with the form. For example [code]<input type=hidden name=friend value="$to"> (this must go inside each form, inside the while loop. It will ensure that $_POST['friend'] is set when the form is submitted) ... if($_POST['delete']){ $friend_to_delete = $_POST['friend'] mysql_query("DELETE FROM friends WHERE username = '$user' AND friend = '$friend_to_delete' LIMIT 1"); mysql_query("DELETE FROM friends WHERE username = '$friend_to_delete' AND friend = '$user' LIMIT 1"); echo "<font color=red> The User has been removed from your friends list, please refresh to view changes.</font>"; } [/code]
-
PHP's arrays have high overhead, particularly for complex structures such as [code]$a[0] = array( 'host' => 'www.phpfreaks.com', 'path' => 'forums', 'file' => 'index.php', 'args' => 'action=post', 'count' => 5, 'potatoes' = true, ... etc etc );[/code] Are there any extensions for php which allow for more efficient data storage, at the expense of reduced flexibility? I am looking for something similar to a C structure, where data is tightly packed, and names for the elements do not need to be stored. The structure needs to support: [list] [*]Inserting at the end of the array [*]Fetching data from any location in the array (indexed by integers, like a C array) [*]Sorting (this could be complex) [/list] My main concern is that the labels for each data item (such as 'host', 'path') are repeates for EVERY element in my array $a. This is a painful waste of space. My secondary concern is that I do not want to use an entire zval to store a simple boolean, or a simple integer. I would like to pack these values more tightly, even if accessing them becomes more costly. Thanks for any advice :)
-
[code]function replace_bad_files($dir) { $handle = opendir($dir); ... while (($file = readdir($handle)) !== false) { # If we found a directory, call ourselves again! Simple $path = $dir . DIRECTORY_SEPARATOR . $file; if (is_dir($path)) { replace_bad_files($path); continue; # This will skip to the next file } ... } }[/code] The important steps here are [list] [*]Make a function [*]Call the function recursively when you find a directory (as indicated by is_dir()) [/list] To count the global number of replacements, you can either use a global variable, or you can have your function return the count, by adding "return $cnt;" at the end. In that case, the recursive call should be "$cnt += replace_bad_files($path)".
-
I don't think preg_split() is useful. I need to split on commas, but commas can also be present within the fields, enclosed by quotes, for example: [code]"first, second",third[/code] This is two comma seperated values: [code]first,second third[/code] I can't think of any regexp that will do that for me! Also, it needs to handle backslash-escaped double quotes within values.
-
It sounds like you want username to be converted like "234" => "s234" ? If your username field is a varchar, you can do [code]UPDATE users SET username = CONCAT('s', username)[/code] First, you should try [code]SELECT username, CONCAT('s', username) FROM users[/code] to make sure that it is doing what you want it to.
-
[a href=\"http://smarty.php.net/\" target=\"_blank\"]Smarty[/a] is a common solution to this problem. With it, you can write design code such as: [code]<table> {foreach from=$my_array item=$row} <tr> <td>{$row.item1}</td> <td>{$row.item2}</td> </tr> {/foreach} </table>[/code]
-
I have written a function which acts like explode(), but honours quotes around elements. Here it is: [code]############################################################################## # The PHP explode() function, but honouring and stripping double quotes. # Also recognizes backslash escaped double quotes. # '"hello, there",fred' => array("hello, there", "fred") function explode_quote($sep, $instr) { $out = array(); $i = 0; $inquotes = false; $cur = ""; while (($c = $instr{$i}) != "") { if ($c == '"') { $inquotes = !$inquotes; } elseif ($c == $sep) { if ($inquotes) { $cur .= $c; } else { $out[] = $cur; $cur = ""; } } elseif ($c == '\\') { /* Single backslash */ $i++; $cur .= $instr{$i}; } else { $cur .= $c; } $i++; } $out[] = $cur; return $out; }[/code] However, it is not very fast. Is there a way I can write this which will make it faster?