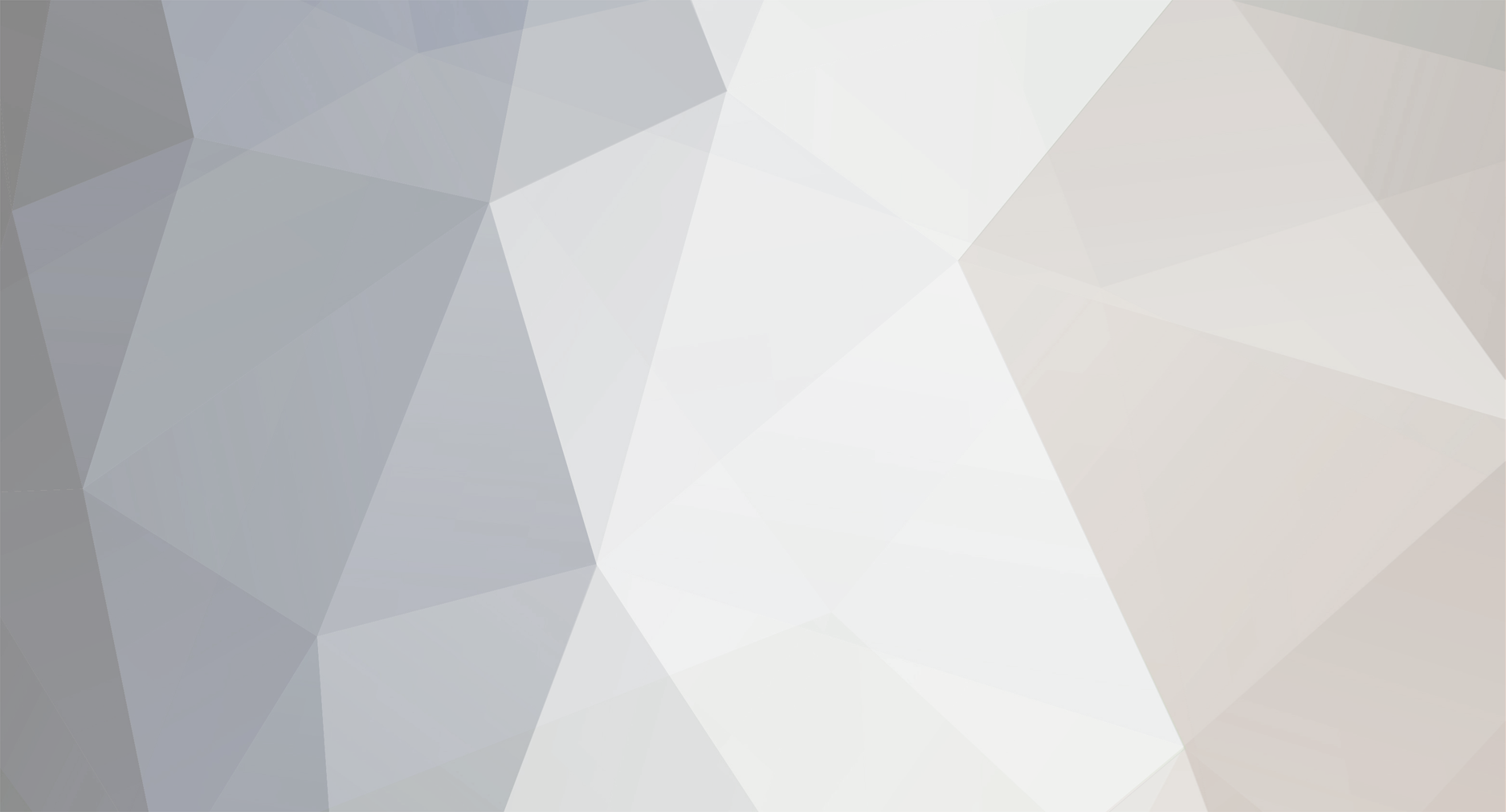
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
If the file is being created in unix and read in windows, you can probably fix it by using \r\n for linefeed instead of \n. If it's some other combination, the solution will be different. nl2br() is for displaying in html, so it doesn't help here.
-
I don't there is an automatic way to decode it. You can just do it manually: [code]$link = "<a href='http://www.whatever.com/a.php?args=$pro'>link</a>"; ... $dec = base64_decode($_GET['args']); $vals = split('&', $dec); foreach ($vals as $val) { list($varname, $varval) = split('=', $val); $_GET[$varname] = $varval; }[/code] Now $_GET['cmd'] and $_GET['id'] are set.
-
Ok I understand now. Instead of the account id, you want to display the account username. But you want to do this for both the owner and the post_owner? To do that in a single query, you'll need to join with the accounts table twice (assuming the accounts table has the username). [code]JOIN accounts AS a ON t.owner = a.id JOIN accounts AS ap ON p.post_owner = ap.id[/code] Then a.username will be the owner's username, and ap.username will be the post_owner's username. Is that what you're looking for? There's no need to specify "INNER", since that's the default.
-
Retrieve from MySQL a previously encripted string
btherl replied to ruano84's topic in PHP Coding Help
Are you sure that $row[1] is actually the password? Try printing out the values of $row[1], and also print out the result of that crypt() call. -
Retrieve from MySQL a previously encripted string
btherl replied to ruano84's topic in PHP Coding Help
Hmm, no I am wrong. Ignore that :) I must think before typing.. -
Debugging from the command line depends on your development environment.. I am developing in linux, so to test new code I do something like this: [code]$ret = foo($argv[1], $argv[2]); var_dump($ret); exit(0); function foo($a, $b) { ... }[/code] $ php code.php arg1 arg2 int(1) $ Which tells me that foo(arg1, arg2) returns the integer 1. If you are always accessing your script through a browser, you can do something similar like this: [code]$ret = foo($_GET['arg1'], $_GET['arg2']); var_dump($ret); exit(0);[/code] Then call your script as http://host.com/script.php?arg1=blah&arg2=bleh That shows you the result of just that function with those arguments. It's a good technique to use on new functions, and functions that you suspect might not be working properly.
-
This isn't really an answer to your question, but I use Smarty templates. Then the HTML and PHP are in separate files, and there is no question of how to indent the mixed code. The general rule I follow when deciding on how to indent is "Does it make the structure clear?" If it does, great. If it doesn't, try some other method of indenting. Eventually you'll hit on something which makes it look nice. You might need to change the indenting style for different pages because they have a different structure, rather than using one global rule for all pages.
-
Here, you need to use == instead of = [code]function checkType($type) { if($_REQUEST['type1'] == TRUE AND $_REQUEST['type2'] == FALSE) { $type = "1" } else if($_REQUEST['type1'] == FALSE and $REQUEST['type2'] == TRUE) { $type = "2" } else { $type= "3" } return $type; }[/code] Are you getting type1 and type2 from checkboxes? radio buttons?
-
Yes.. and for every other function too. It may seem like a lot of work, but it saves a lot of debugging work later. [code]Function create_greet() { $header = get_file_contents('header.txt'); if ($header === false) die("Couldn't read header.txt\n"); $body = get_file_contents('body.txt'); if ($body === false) die("Couldn't read body.txt\n"); $footer = get_file_contents('footer.txt'); if ($footer === false) die("Couldn't read footer.txt\n"); $greeting_fp = fopen('greeting.txt', 'w'); if ($greeting_fp === false) die("Couldn't open greeting.txt\n"); fwrite($greeting_fp, $header); fwrite($greeting_fp, $body); fwrite($greeting_fp, $footer); fclose($greeting_fp); } create_greet();[/code] It's not so essential to check fwrite() and fclose(), since they very rarely fail.
-
Echo variable before it is defined.. Follow-up question.. NEVERMIND!
btherl replied to a topic in PHP Coding Help
Smarty templates will solve this problem. It's a big step from standard php to Smarty though. Another option is to buffer your output using ob_start() and related functions. Then you can modify the buffer before displaying it, with str_replace() for example. [code]<? ob_start() ?> <title>{PAGE_TITLE}</title> ... $output = ob_get_clean(); $output = str_replace('{PAGE_TITLE}', $page_title_of_unique_content, $output); print $output;[/code] -
My standard php debugging technique is var_dump(), print_r() and print(). That's powerful enough to find 95% of my bugs. When that fails, I try commenting out parts of code. I also add sections like [code]$ret = some_function($argv[1,], $argv[2]); var_dump($ret); exit(0);[/code] to the start of files, and debug them from the command line.
-
At the top you say [code]$name = $_POST['fund'];[/code] But in the form you put [code]<? echo $fund; ?>[/code] Maybe that should be echo $name ? But that doesn't make so much sense, since you only display the form if $_POST['fund'] is not set. I am very confused now :)
-
This should work [code]$header = get_file_contents('header.txt'); $body = get_file_contents('body.txt'); $footer = get_file_contents('footer.txt'); $greeting_fp = fopen('greeting.txt', 'w'); fwrite($greeting_fp, $header); fwrite($greeting_fp, $body); fwrite($greeting_fp, $footer); fclose($greeting_fp);[/code] It's better if you check every function call for errors, like [code]if ($header === false) die("Couldn't read header.txt\n");[/code]
-
[code]SELECT t.id AS tid,t.*,p.id AS pid,p.*,a.id AS aid,a.* FROM topics AS t INNER JOIN posts AS p ON t.post_id=p.id INNER JOIN accounts AS a ON t.owner=a.id AND p.post_owner=a.id WHERE t.board_id=$board[bid] ORDER BY t.date DESC LIMIT $start,$limit[/code] I've reformatted the query a bit. I don't fully understand what you are trying to do. What do you want your results to look like?
-
Which version of PHP are you using? PHP4 uses the DOM XML extension, PHP5 uses the DOM extension, each which appear to have the same object names with different interfaces.
-
After each query, check for errors like this: [code]$query = "SELECT * FROM Gallery WHERE id=$id AND category=$category"; $result = mysql_query($query); if (!$result) die("Error in $query: " . mysql_error());[/code] I'm also not sure if you can call mysql_close() so soon. Maybe you should move it until after you have fetched the results. That added line will tell you two things - It will show you the query you are trying to execute. - It will show you the error message that caused the query to fail (if it did fail)
-
The logic in your script seems odd. At the end, with $query2, you say "If the user and password exist in the database, then insert them again". That doesn't make sense. If they are already in the database, then any attempt to insert them will fail. It should probably be [code]if ($count == 1) { echo "Username and password match!"; }[/code] Also, it's a good idea to check the result of your second mysql query, just in case. [code]if ($query2 === false) { die("$query2 failed: " . mysql_error()); }[/code] In this case, $query2 == 0 will give you the same result.
-
Would reloading the entire page, but reading the menu, header etc from a common file be good enough? That's the sort of thing that php is quite good at, especially using Smarty templates.
-
Try using $row['Name'], $row['Prodid'], $row['Price'] inside the paypal form. And delete any reference you have to $_POST. Those lines have no effect, because $_POST is never set, only $_GET.
-
Visitor logging script! problem HTTP_REFERRER
btherl replied to AbydosGater's topic in PHP Coding Help
It's $_SERVER["HTTP_REFERER"], with one R. While it can be forged, most people don't bother. It's perfectly fine for collecting statistics about visitors, but not useful for security. My experience is that most users leave it on. -
If I was debugging that, I would add var_dump($table) inside the loop where you create $table. That will show you what is happening. If that doesn't help, add var_dump($table[0]) before you try to print out $table[0]. The results should help you solve your problem :)
-
There's a misconception underlying your code which is probably causing much trouble. Any request to a PHP script will be either a GET or a POST. It can't be both. If it's a GET, then $_GET will be populated. If it's a POST, then $_POST will be populated. Never will you have variables in both $_GET and $_POST. If you want to set hidden fields in that post form, you should not be using $_POST at all. $_POST is for requests to your script, but that paypal form goes to someone else's script (it goes to paypal). So, $_POST should never be mentioned in your code (unless you have another post form somewhere). I think an essential thing to understand is that only one form is ever submitted in any request. Only data in that form is available to your script. The data in the post form is never available to you, because it goes to another script. If you want access to that data, you must add it to your other form as well. Good luck :)
-
I would guess that although it's the same phpmyadmin version, it's a different mysql version. Try deleting some things around where the error is. I would try deleting "default" first.. then try removing "charset = latin1". With enough mangling I'm sure it will start working :)
-
For scope, it doesn't matter if they are arrays. Here's an example for passing variables into functions and getting results back: [code]function increment($x) { $x = $x + 1; return $x; } $x = 1; # This is NOT the same $x as above, it is outside the function scope $x = increment($x); print "x is $x\n";[/code] There's no connection between the $x inside the function and the $x outside. You can check this by doing [code]$x = 1; print "Incremented x is " . increment($x) . "\n"; print "But x's value is still $x\n";[/code] Good luck :)
-
http://sg.php.net/manual/en/function.htmlspecialchars.php will probably do it. That will escape the HTML so it doesn't escape the textarea. Edit: Er.. I mean, so it doesn't "get out of" the textarea. Bad choice of words :)