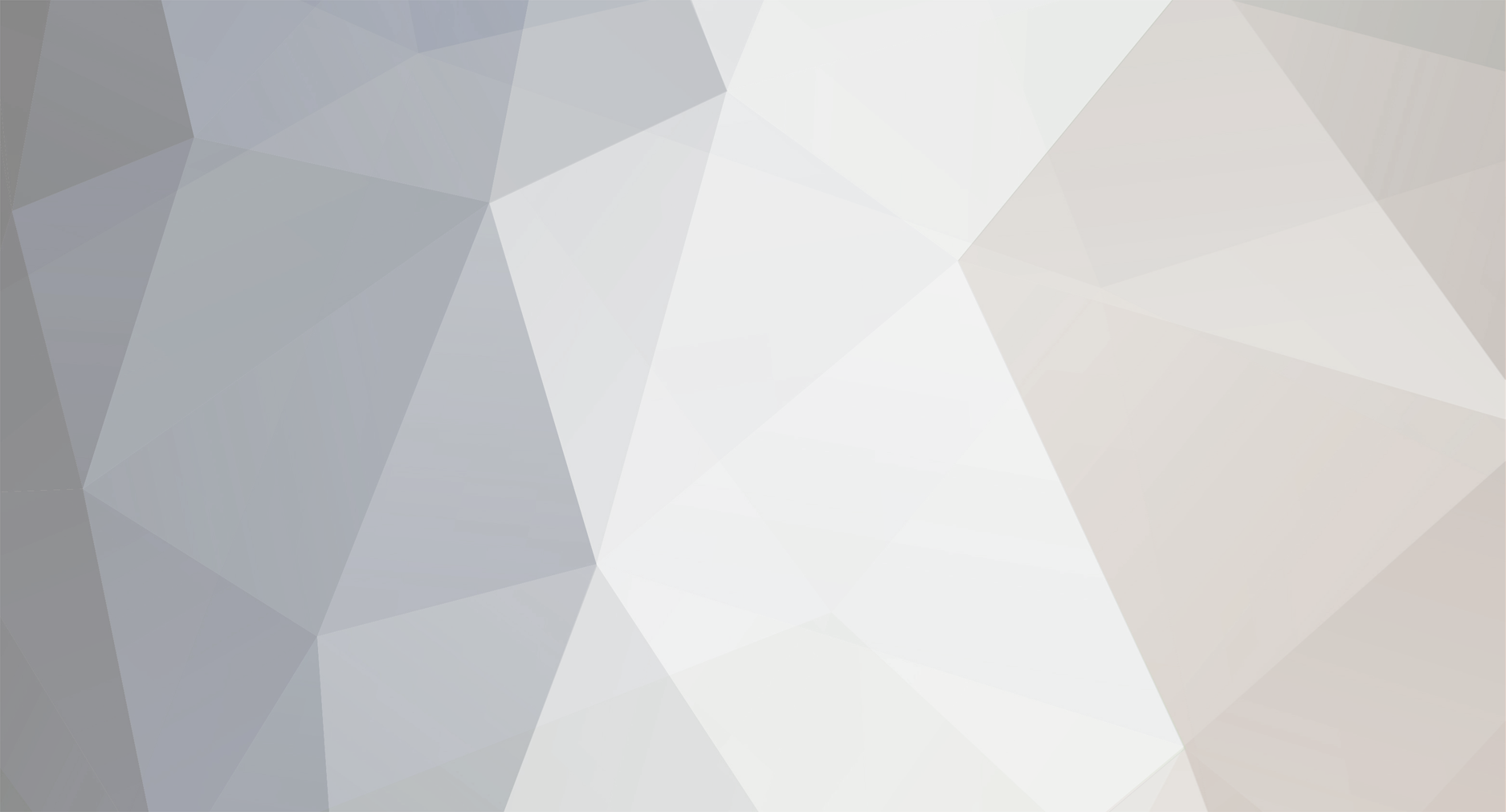
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
If you want your variables to be shared between all your files and functions, you can put "global $y" at the top of each function that uses $y. And global $x at the top of each that uses $x. Another way is to pass the variable in as function arguments and get them back as return values. Once your code gets larger you will probably want to do that.
-
You could make an array of all the tables you want to check and use a for loop.. is that the kind of solution you're looking for?
-
The most straightforward solution I can think of is to sort the trackids from longest to shortest. Then check them in that order. That guarantees finding the longest match first. Something like: [code]function length_cmp($a, $b) { $a_len = strlen($a); $b_len = strlen($b); if ($a_len < $b_len) return -1; if ($a_len > $b_len) return 1; return 0; } $trackids = array('string', 'stringed', 'stringest'); usort($trackids, 'length_cmp'); foreach ($trackids as $trackid) { # Check this one and replace if found }[/code] If the sort order is wrong, replace -1 with 1 and 1 with -1. I don't remember which way is correct :) Does that help?
-
Correct me if I'm wrong in my understanding here: - You have strings such as "stringerstringed". - You want to search for strings within these strings. For example, you want to search for "string" and "stringed". - You want to take the longest match in every case. That is, you prefer "stringed" over "string". Is this assumption correct? - Any character can appear immediately before and after trackids. Is this correct? Once that's clarified we can look at solutions.
-
You can have a hidden div that becomes visible when you click a link, using javascript. Or you can have a frame that is always there, but gets content only after you click a link (again using javascript). I can't help with details, but javascript is the way to go for that kind of dynamic stuff. PHP alone can't do what you're asking.
-
This isn't really a php question.. I'm guessing it's the "link:site.com" query syntax you want.
-
Hi, I Need Help with a function thanks im new in php.
btherl replied to zeppis's topic in PHP Coding Help
Try displaying your queries in the browser, to check that they are what you think they should be. Eg [code]$sql = "UPDATE ... "; echo "<pre> $sql </pre>"[/code] Also, check the return values on all your mysql queries. [code]$result = mysql_query($sql); if ($result === false) die("Query $sql failed with error " . mysql_error());[/code] And don't use @ .. that means you ignore errors instead of fixing them :) -
converting into 7 Bit ASCII format in PHP, Is this possible??
btherl replied to codeexploiter's topic in PHP Coding Help
Are you looking for the ord() function? -
'SERVER_NAME' The name of the server host under which the current script is executing. If the script is running on a virtual host, this will be the value defined for that virtual host. 'HTTP_HOST' Contents of the Host: header from the current request, if there is one. Going by that description, SERVER_NAME should be more stable, since it's defined by the web server. HTTP_HOST will probably have more variance. I haven't answered your question, but I hope this helps :)
-
What happens if you do "SELECT password("password")", and then do a query using the output of that? Are there any matching columns? And does the output of that first query make sense?
-
There's certainly something odd with the quotes. In general, you don't need to put any quotes around column names. [code]SELECT a.vis_type, c.countryName, count(a.u_id) AS a_u_id_count, ...[/code] This style should work.
-
SELECT countryName, COUNT(u_id) FROM _AEsurvey_rego WHERE countryName LIKE 'China' GROUP BY countryName; This ought to work.. I'm a bit confused as your query and error message do not match up. Or maybe they do match up, and mysql removes the second select. In any case, you only need to say "select" once :)
-
The sleep() function will wait a specific number of seconds. It is usually accurate to within a second, but it may wait longer, particularly if the system is under load. If you need EXACTLY 30 minutes, then that's more difficult. If you want approximately 30 minutes, then sleep(60 * 30) will work. Another option is to check time() regularly, and see when 30 minutes have passed. You can use sleep(1) in a loop to ensure that you only check the time once a second.
-
one to many update checkboxes - update syntax
btherl replied to john_6767's topic in PHP Coding Help
Can you explain what you did, what you expected to happen and what actually happened? Three things in total. Then it will be much easier for us to help you :) -
PHP is probably using (or at least looking for) php.ini in C:\Windows. It doesn't know that you wanted it to be in C:\ instead (if that's what you mean by "on the C drive"). I think the easiest solution is to check if there is a c:\Windows\php.ini, and if there is not, move your php.ini to there. Then edit it and see if the changes show up.
-
Like this.. when you use explode(), you are creating a new array. [code]$new_array = explode(';', $original_string); $i = 1; foreach ($new_array as $variable) { print "Exploded item $i is $variable\n"; $i++; }[/code] That first line means "Set $new_array to be the parts of $original_string, split by the ';' character".
-
Try including the sql statement in your die(), like this: [code]$sql = "SELECT * FROM table"; mysql_query($sql) or die( 'Error executing $sql ' . mysql_error());[/code]
-
The problem there is that you are setting $selected, then using the same value for both <option> tags. To keep the same style as you have, you can create an array $selected[], and set [code]$selected[0] = ' selected '; $selected[1] = '';[/code] for example, if you wanted 0 to be selected and 1 not selected. Then where you set $option, you can use $selected[0] and $selected[1], just as you use $sex[0] and $sex[1].
-
A shot in the dark here.. try giving your cookie a name with no spaces in it.
-
Yes, you can.. [code]SELECT JOB_ID,JOB_TITLE,JOB_DATEREMOVED FROM tbljobs WHERE (JOB_STAFF='$userData[USERID]' AND JOB_DELETE=1) OR (JOB_STAFF='$userData[USERID]' AND JOB_ARCHIVE=1) ORDER BY JOB_DATEREMOVED DESC SELECT COUNT(APP_ID) FROM tblapplications WHERE APP_JOBNUM='$jobsData[JOB_ID]' => SELECT JOB_ID, JOB_TITLE, JOB_DATEREMOVED, COUNT(*) AS APP_ID_COUNT FROM tbljobs JOIN tblapplications ON (tblapplications.APP_JOBNUM = tbljobs.JOB_ID) WHERE (JOB_STAFF='$userData[USERID]' AND JOB_DELETE=1) OR (JOB_STAFF='$userData[USERID]' AND JOB_ARCHIVE=1) GROUP BY JOB_ID, JOB_TITLE, JOB_DATEREMOVED ORDER BY JOB_DATEREMOVED DESC[/code] It won't matter if you use count(*) or count(app_id).. if you want the count of distinct app_ids, then you need count(distinct app_id) in there ( I think that works with mysql.. i'm more used to postgres actually). Anyway, the key thing there is you need not only a join, but also a group by. A simple join won't work because you want to count the results from the second table.
-
You said that you erase "CREATE DATABASE ...", but the error mentions that line. Are you sure you erased it?
-
Hmm.. I think it could be done with a join. But it could also be done in PHP. For a join, it would look like this: [code]$sql = "SELECT fb_color, fb_type, stock02 - stock01 as subbed FROM ( SELECT fb_color, fb_type, SUM(yards) as stock01 FROM my_inv WHERE ... ) JOIN ( SELECT fb_color, fb_type, SUM(yards) as stock02 FROM my_inv WHERE ... ) USING (fb_color, fb_type)"[/code] Those subqueries there where I put "..." will be the entire queries you already have. The idea is that before joining, you have output like Output 1: fb_color, fb_type, stock01 Output 2: fb_color, fb_type, stock02 When you join on fb_color and fb_type, you get: Joined output: fb_color, fb_type, stock01, stock02 Then you just need to specify that you want (stock02 - stock01) as an output column. You could simplify your initial queries like this: [code]$query01 = "SELECT fb_color, fb_type, SUM(yards) as stock01 FROM my_inv WHERE fb_type = \"{$fb_type}\" AND fb_color = \"{$fb_color}\" AND date_in <= \"{$end_date01}\" AND in_transit < 1 AND (date_out >= \"{$end_date01}\" OR date_out = \"0000-00-00\" ) GROUP BY fb_type, fb_color";[/code] That will work since only date_out varies between each OR branch. You can also combine the "greater" and "equal" into a single condition.
-
I would expect a query fetching fewer columns to be slightly more efficient. But it depends on a lot of factors. If all or most of those 1122 or 1467 rows must be fetched from disk, then both queries will be slow. Speed should be around the same. If that's the case, then you need to reduce the number of rows being fetched for each query. Right now your query has to fetch all 1000+ rows, sort them all, then chop off the top 10. If you can arrange your data so that fewer rows need fetching you can get better performance. One way could be to expire old threads, or move old threads into an "old threads" table. Then you can check the old threads table whenever you can't find 10 new threads in the "recent threads" table. I could be totally wrong :) So make sure you benchmark any changes and see if things have improved or gotten worse.
-
How can you grab a certain ammount of characters from a table?
btherl replied to Demonic's topic in PHP Coding Help
Assuming you are using mysql: [code]SELECT SUBSTRING(column FROM 1 FOR 50) FROM table[/code] That'll select the first 50 characters from column in table. -
The only solution for that is a timeout. If you record the last activity of each user, then you can have some code which checks if a user has not done anything for a certain amount of time. If they haven't, then you mark them offline. You don't have to log them out, just make them appear offline to others since they are inactive. You can either have it run as a regular job (like a cron script), or have it run each time a page is loaded. A common strategy is to have it run at random, say every 1 in 10 page loads. The right frequency depends on how often your pages get hit. Unfortunately there is no way to detect them closing the browser.