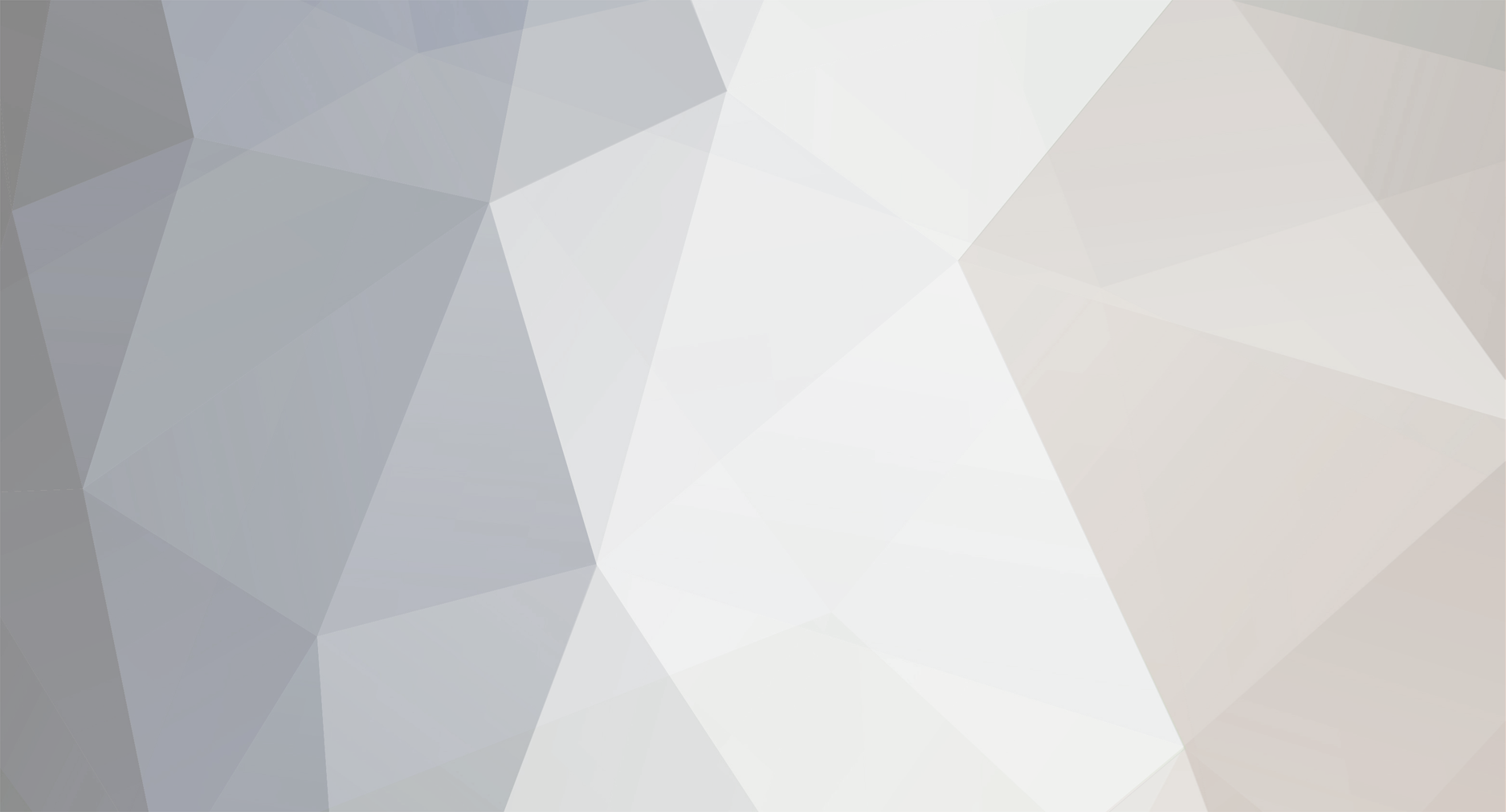
btherl
Staff Alumni-
Posts
3,893 -
Joined
-
Last visited
Everything posted by btherl
-
I'll offer another suggestion (sasa's idea will work, though there seems to be a syntax error). You can set class = 'temp' where class = 'pos1' Then class = 'pos1' where class = 'pos2' Then class = 'pos2' where class = 'temp' Three queries, when you could do it in one, but it will also work :) If you can get sasa's technique to work then use that, it is safer and faster than this one.
-
Oops.. I just copied and pasted. You need to say which table to select from too :) [code]$sql = "SELECT uname, SUM(points) AS total FROM table GROUP BY uname ORDER BY total DESC, uname ASC LIMIT 10"[/code] where 'table' is the name of your table containing the unames and points. Algorithmically, that will: 1. Fetch all rows from table 2. Find the sum of points for all matching unames, merging all matching unames into single rows. 3. Order the uname, total (which is sum of points) by total descending, then uname ascending as a tie-breaker. 4. Slice off the first 10 results 5. Return those results to you
-
can anyone help ( database connection issue )
btherl replied to the apprentice webmaster's topic in PHP Coding Help
A good start would be to check for errors when connecting or selecting the database. In place of [code]if ($$link) mysql_select_db($database);[/code] put this: [code]if (!$$link) die("Couldn't connect to database using server $server, username $username, database $database, link $link! " . mysql_error() . "\n"); if (!mysql_select_db($database)) die("Couldn't select database $database! " . mysql_error() . "\n");[/code] That should give you an idea of why it's failing (if it is failing in that function) -
It's your variable names! They are driving me crazy! And they are causing bugs due to that confusion. [code]$qCheck = "select * from TB_Users where penname = '$recip' limit 1"; $rsCheck = mysql_query($qCheck) or die(mysql_error()); $rsBlock = mysql_query("SELECT * FROM blocked_user WHERE username = '$recip'") or die(mysql_error()); $arrBlock = mysql_fetch_assoc( $rsBlock ); if ($arrBlock['blocked'] == $userpenname) { ...[/code] When your variable names are consistent, it's easy to see what's going on. Cheking for errors on every query is also good practice.
-
problem with php sessions/mysql - submitting NULL data to database!!
btherl replied to saturn's topic in PHP Coding Help
Then number 3 is very likely.. "3. The $query you are echoing IS sent to mysql, but another one is also sent." If you're getting a second mail, it's possible your script is being executed again (through some mechanism, maybe being included more than once?), and the second query blanks out your values. You might want to try making a log file, like this: [code]$log_fp = fopen("/tmp/mylog", "w"); if (!$log_fp) die("Couldn't open log file!<br>"); and then just before your mysql statement, add fwrite($log_fp, "About to execute $query\n");[/code] Then you'll get a record of all queries, including any which may not have the output sent to your browser. -
can anyone help ( database connection issue )
btherl replied to the apprentice webmaster's topic in PHP Coding Help
The code you pasted doesn't connect to the database, it just sets variables to be used when the connection is made. Is there any code looking like mysql_connect() or DB::connect() ? -
Call to undefined method DB_Error::fetchRow()
btherl replied to AncientSage's topic in PHP Coding Help
Single quotes vs double quotes is important. It should be: [code]$sql = "SELECT id, name FROM threads WHERE forum = '$id'";[/code] What you had would literally put "$id", not the contents of the variable named id, into the query. You should also check if your result is valid before calling fetchRow .. it's actually an error, which is why it has no fetchRow method. -
problem with php sessions/mysql - submitting NULL data to database!!
btherl replied to saturn's topic in PHP Coding Help
"Whenever you have eliminated the impossible, whatever remains, however improbable, must be true." :) If echo $query shows you a valid query, but the database is getting null values, then there are only so many explanations. I'm listing them in order of how likely I think they are. 1. The $query you are echoing is not the one being sent to mysql 2. The $query you are echoing isn't doing what you expect it to do (it looks good to me though) 3. The $query you are echoing IS sent to mysql, but another one is also sent. 4. The $query is corrupted after being sent to mysql_query() (very unlikely) The way I solve a problem like this is to add more and more debugging statements. In your case, I would add echo $query right before and after the mysql_query() call, just to be 100% sure. I would also see if I can get mysql to log all the queries executed. If you can do that, then you will have very valuable information. Since mysql_query() is the only thing that can change the database, if you focus on that function call, you will find the bug eventually. Good luck :) -
Here, you open two { but only close with one } .. this could be the problem: [code] if($user && $pass){ if ($logged_in_user == $user) { echo $user.", you are already logged in.<BR><BR>"; echo $links; exit; }[/code]
-
What do you mean by "hit back to the form"? And what does it look like when it desyncs?
-
[code]$files = array(); while ($file = readdir($dir)) { $start = substr($file, 0, 1); if ($start != ".") { $files[] = $file; } } sort($files) foreach ($files as $file) { $title = substr($file, 1); $title = preg_replace("/\..*?$/", "", $title); // removes the extension $title = preg_replace("/([A-Z0-9])/", " $1", $title); // places the space echo '<div class="thumb"><a href="image.php?folder=' . $folder . '&img=' . $file . '" target="view"><img src="' . $folder . "/" . $file . '"></a><br />' . $title . '</div>'; }[/code] That ought to work. Instead of reading the files and displaying them immediately, you will be reading them into an array, sorting the array, then displaying them. I've left the filtering of "." files in the first foreach, so you don't need to do it again in the second one..
-
A bit confusing, but i'll have a go :) [code]SELECT antispam.email_address FROM antispam LEFT JOIN users ON antispam.email_address = users.email_address WHERE newsletter = 0 OR users.email_address IS NULL[/code] That will give you all email addresses from antispam for which newsletter is 0, OR there is no matching row in users table. Is that what you mean by "... not match for that column then we assume its 0"?
-
I'm with roopurt18 here :) But I don't think you should include "DISTINCT". The group by will make usernames distinct already, and I don't think you want point scores to be distinct.. [code]$sql = "SELECT uname, SUM(points) AS total GROUP BY uname ORDER BY total DESC, uname ASC LIMIT 10"[/code] The "uname ASC" ordering is in case of ties.. you need to decide who gets in the top 10 if positions 9-11 have the same score, for example.
-
Can you add the following, just before the mkdir() and after "$user_id = $_POST['userid']" : [code]print "I am in directory " . getcwd() . "<br>"; print "I am about to make " . getcwd() . "/profiles/$userid<br>";[/code] And check if the output makes sense.
-
Can you tell us what you did, what you expected to happen and what actually happened? We need all those 3 things to tell you what's going wrong.
-
I don't do what you're doing, but I do select tables by namespace for calculating table size, in the following: [code]ci=# \d total_rel_size View "public.total_rel_size" Column | Type | Modifiers --------+--------+----------- name | name | tuples | real | gb | bigint | View definition: SELECT pg_class.relname AS name, pg_class.reltuples AS tuples, pg_total_relation_size(pg_class.relname::text) / (1024 * 1024) AS gb FROM pg_class WHERE pg_class.relpages > 1024 AND pg_class.relnamespace = 2200::oid AND pg_class.relkind = 'r'::"char" ORDER BY pg_class.relname;[/code] The part which may interest you is "pg_class.relnamespace = 2200::oid". That's the namespace which contains all my tables (it may be different on your system, and schemas may affect it). It doesn't include toast tables, but if you select all data from the main table then that will include toasted data. This whole thing would be much easier if you can use pg_dump (the command line program). That will take care of everything (though you might need to fix those permission problems).
-
Looks pretty good to me. As far as php is concerned, a number from a form is text which only has digits in it. Both is_numeric() and ctype_digit() will do this check for you. But is_int() will FAIL, because it's a string of digits, rather than an integer. PHP will automatically convert those strings into integers when necessary (which is why the calculations work), but is_int() will still return false.
-
You can't just put an image into the src attribute. The script that displays the picture needs to do nothing else but display that picture.. then another script needs to call that one. Script 1: [code]Get picture from database header('Content-type: image/gif'); or whatever the image is print $result_ar['Picture'];[/code] Script 2: [code]<img src="/script1?img=Image1" width="144" height="108" border="0" id="Image1" />[/url][/url]</td>[/code] Does that makes sense? The HTML in script 2 says to call script1 to get the image for display. It can't be done in a single script (not any way I know of)
-
I'm unclear on what you are asking. Can you give some more detail please?
-
There's a specific forum for regex questions.. I think your problem here is that "+" is a metacharacter. You need to use \+ if you want to match an actual + sign. "[" and "]" also need to be escaped, as \[ and \]. The rest looks ok to me
-
I think he's after this: [code]echo '<option ' . ($data1['maincat_id']==$maincat ? 'selected' : '') . ' value="'.$row['maincat_id'].'">'.$row['maincat_name'].'</option>';[/code] I prefer this style though.. less complexity in each line :) [code]$selected = $data1['maincat_id'] == $maincat ? 'selected' : ''; echo '<option ' . $selected . ' value="'.$row['maincat_id'].'">'.$row['maincat_name'].'</option>';[/code]
-
Typically people use meta redirect for this.. [code]<meta http-equiv="Refresh" content="0; http://site.com/path/file.php">[/code] That means "Act as if you got a header called Refresh with this value". The browser should redirect after 0 seconds (immediately). Display a link anyway in case it doesn't work. There's a number of javascript methods to redirect as well. Alternatively, you can do the email check before displaying any output. Then you can use a header redirect as usual. That sounds like it ought to work given your setup.
-
Try [a-zA-Z] instead of [a-Z].. or [[:alpha:]]
-
Newbie question: Extracting text from a string and storing in a variable
btherl replied to gregp's topic in PHP Coding Help
preg_match() will certainly work. You could also use substr(). Since the value you want is a fixed length at a fixed offset, you can use [code]$timestamp = substr($line, 1, 10);[/code] If you want to use preg_match(): [code]if (preg_match('/^\[([[:digit:]]*)\]/', $line, &$matches)) { $timestamp = $matches[1]; }[/code] might work, providing I haven't made any mistakes. PS, when using strpos() you should check "if (strpos($line, $aarnet_OK) !== false)" instead. For your case it will work, but you may have trouble later. See http://www.php.net/manual/en/function.strpos.php -
The example looks like it is doing that. The argument to extract() is telling it which directory to put the files in.