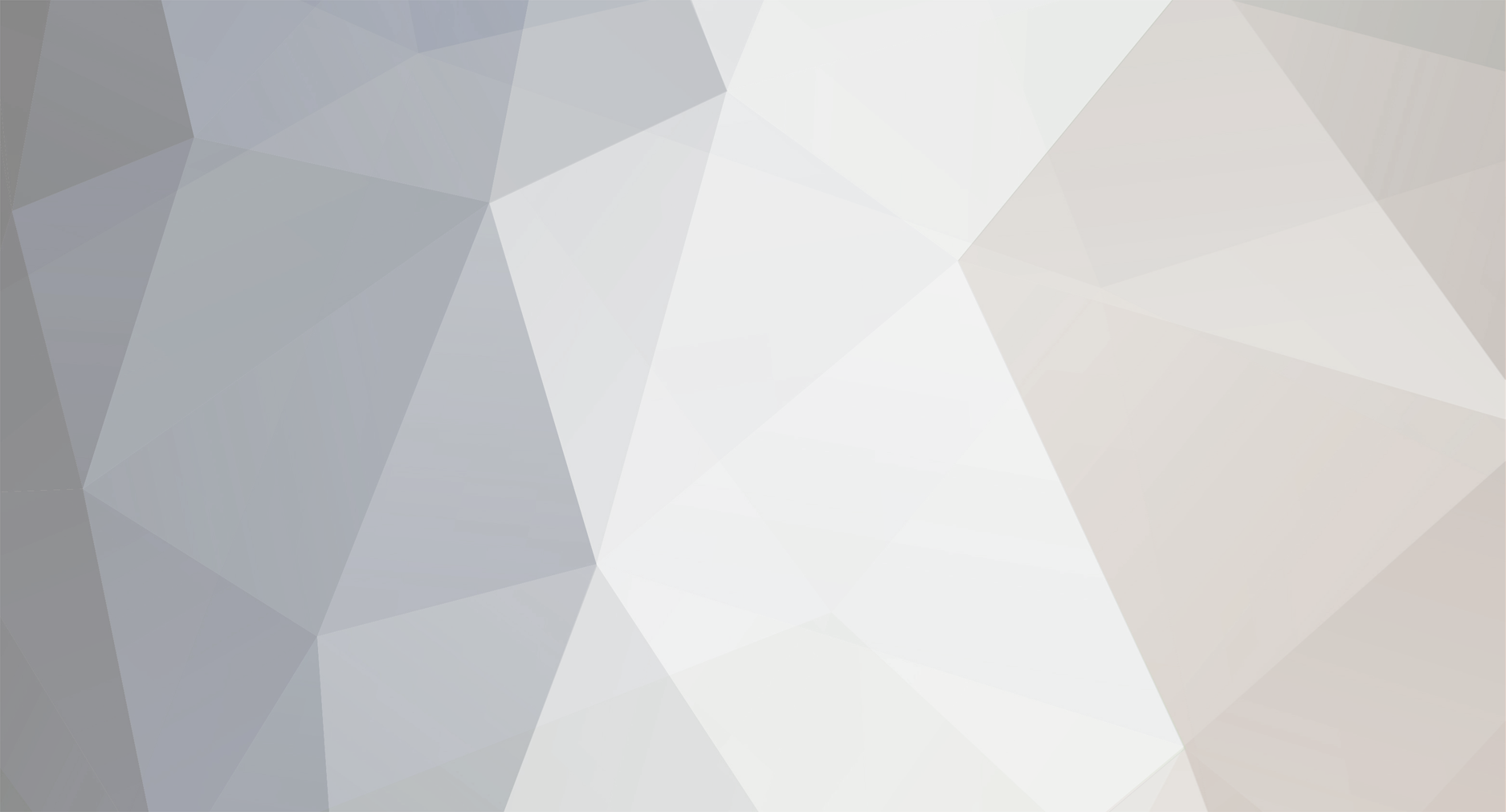
sKunKbad
Members-
Posts
1,832 -
Joined
-
Last visited
-
Days Won
3
Everything posted by sKunKbad
-
Let's say that I have a table for vegetables, and the vegetables are normally sorted by the date they arrived at the store. There is also a status, where the vegetable can be acceptable, good, great, special, rotten, etc. So if I were to query for the vegetables, sorted by the arrival time, it would be a simple sort by time. What if I always want to ensure that vegetables of a certain status are at the end of the returned set of rows? Expected result: Carrot, 2015-7-10 08:00:00, rotten Broccoli, 2015-7-10 07:00:00, great Radish, 2015-7-10 06:00:00, good Lettuce, 2015-7-10 05:00:00, acceptable Pepper, 2015-7-10 05:00:00, good Potato, 2015-7-10 09:00:00, expired Tomato, 2015-7-10 08:00:00, expired Turnip, 2015-7-10 07:00:00, expired I just used vegetables as an example, but what I wanted to show was that although sorted by date, the vegetables with a status of expired are at the end of the list. I can't sort by status, because status is not guaranteed to be alpha or numeric or anything else. Also, I need to be able to paginate this, so how do I get those with special status (expired in this case) at the end? Sorry, I'm not especially good with MySQL.
-
[CodeIgniter] Ternary Operator as array element causing white screen
sKunKbad replied to sKunKbad's topic in Frameworks
I tried: error_reporting(E_ALL); ini_set("display_errors", 1); Still nothing, just white screen. Nothing logged. -
[CodeIgniter] Ternary Operator as array element causing white screen
sKunKbad replied to sKunKbad's topic in Frameworks
Cool, thanks for the answer. It would be nice if PHP would have at least spit out some kind of an error. -
[CodeIgniter] Ternary Operator as array element causing white screen
sKunKbad replied to sKunKbad's topic in Frameworks
5.4.41 -
[CodeIgniter] Ternary Operator as array element causing white screen
sKunKbad replied to sKunKbad's topic in Frameworks
Error logs don't show anything. I can replicate this by running the following script: <?php function set_value($x) { return FALSE; } $arr = [ 'a' => ( ! empty( set_value('a') ) ? date('Y-m-d', strtotime( set_value('a') ) ) : NULL ), 'b' => ( ! empty( set_value('b') ) ? date('Y-m-d', strtotime( set_value('b') ) ) : NULL ), 'c' => ( ! empty( set_value('c') ) ? date('Y-m-d', strtotime( set_value('c') ) ) : NULL ), 'd' => ( ! empty( set_value('d') ) ? date('Y-m-d', strtotime( set_value('d') ) ) : NULL ) ]; var_dump( $arr ); On production I get the white screen of death. On development I get: array(4) { ["a"]=> NULL ["b"]=> NULL ["c"]=> NULL ["d"]=> NULL } -
I've posted here because the set_value() function in the code below is exclusive to CodeIgniter. I've used ternary operators as array elements before, by simply wrapping them in parenthesis. What's confusing to me is that on my development environment, which is Ubuntu w/ PHP 5.5.X, everything works fine, but the production environment is some Linux hosting PHP 5.4.41, and it just gives a white screen. This happens without any error displayed, and happens even when the code is not executed, just simply loading the code (syntax error?). The code causing white screen: <?php $arr = [ 'a' => ( ! empty( set_value('a') ) ? date('Y-m-d', strtotime( set_value('a') ) ) : NULL ), 'b' => ( ! empty( set_value('b') ) ? date('Y-m-d', strtotime( set_value('b') ) ) : NULL ), 'c' => ( ! empty( set_value('c') ) ? date('Y-m-d', strtotime( set_value('c') ) ) : NULL ), 'd' => ( ! empty( set_value('d') ) ? date('Y-m-d', strtotime( set_value('d') ) ) : NULL ) ]; So, why would my development environment be OK with this code but production have a white screen of death?
-
How to catch all PHP errors in CodeIgniter Framework
sKunKbad replied to lilmer's topic in Frameworks
I've actually been using set_error_handler in a pre_system hook with CodeIgniter. If on development machine I just see the errors, however if on production the errors are logged to a file, then once every 15 minutes the file is emailed to me. Don't be tempted to email yourself with each error as it happens. I learned the hard way that if PHP loops through an array where a notice is generated it will send you as many emails as notices. I got 4000 emails one day! <?php function my_error_handler( $e_number, $e_message, $e_file, $e_line, $e_vars ) { // Debugging allows dev to log errors like production $debug = FALSE; // The amount of E_NOTICE + E_STRICT before we die() $error_limit = 25; // The number of errors counted on this request static $error_count = 1; // Set the text of type of error displayed switch( $e_number ) { case E_USER_ERROR: $error_type = 'E_USER_ERROR'; break; case E_USER_WARNING: $error_type = 'E_USER_WARNING'; break; case E_USER_NOTICE: $error_type = 'E_USER_NOTICE'; break; case E_WARNING: $error_type = 'E_WARNING'; break; case E_NOTICE: $error_type = 'E_NOTICE'; break; case E_STRICT: $error_type = 'E_STRICT'; break; default: $error_type = 'UNKNOWN ERROR TYPE'; break; } // Output for development or testing environments (unless debug === TRUE) if( ENVIRONMENT != 'production' && $debug !== TRUE ) { echo '<hr />PHP ' . $error_type . ' #' . $e_number . ' - Date/Time: ' . date('n/j/Y H:i:s') . PHP_EOL . '<br />File: <b>' . $e_file . '</b>' . PHP_EOL . '<br />Line: <b>' . $e_line . '</b>' . PHP_EOL . '<br /><b>' . $e_message . '</b><hr />' . PHP_EOL; } // Log file for production environment else { // MAIN MESSAGE $message = '#---' . PHP_EOL . 'PHP ' . $error_type . ' #' . $e_number . ' - Date/Time: ' . date('n/j/Y H:i:s') . PHP_EOL . 'File: ' . $e_file . PHP_EOL . 'Line: ' . $e_line . PHP_EOL . 'Message: ' . $e_message . PHP_EOL; // POST VARS if( isset( $_POST ) && ! empty( $_POST ) ) { $message .= 'POST vars:' . PHP_EOL; foreach( $_POST as $k => $v ) { $message .= '\t' . $k . ' = ' . $v . PHP_EOL; } } // REQUEST HEADERS if( $request_headers = apache_request_headers() ) { $message .= 'Request headers:' . PHP_EOL; foreach( $request_headers as $k => $v ) { $message .= '\t' . $k . ' = ' . $v . PHP_EOL; } } // REQUEST URI $message .= 'Request URI: ' . $_SERVER['REQUEST_URI'] . PHP_EOL; // ERROR COUNT $message .= 'Error Count: ' . $error_count . PHP_EOL; $message .= '#--' . PHP_EOL; // Second param (3) says to store error in specified log file error_log($message, 3, FCPATH . '/application/logs/php_errors/php_errors.log' ); // If not an E_NOTICE or E_STRICT, die() if( $e_number != E_NOTICE && $e_number < E_STRICT ) { die( '<br /><br /><span style="color:red;">A system error occurred. We apologize for the inconvenience.</span>'); } // If too many E_NOTICE or E_STRICT, die() $error_count++; if( $error_count > $error_limit ) { die( '<br /><br /><span style="color:red;">A system error occurred. We apologize for the inconvenience.</span><br /><span style="font-size:50%;">E_NOTICE + E_STRICT > ' . $error_limit . '</span>'); } } // Don't execute PHP internal error handler return TRUE; } function my_error_handling() { set_error_handler('my_error_handler', E_ALL); } -
1) The enctype attribute of the HTML form must allow for file uploads. You haven't done that. 2) The action attribute of the HTML form must point to the PHP processing script, not an email address. 3) The file input needs a name attribute. The processing script 1) The processing script will need to validate the form submission. 2) The processing script will need to make sure that the file that was uploaded is safe, and of an expected type or types. Email PHP offers a built-in way to send email, and since you are sending an attachment to yourself, you will need to learn how to add the attachment. Please use code tags when adding code to you posts.
-
parsing xml - with PHP-simpleXML - a headstart into this technique
sKunKbad replied to dil_bert's topic in PHP Coding Help
Are you trying to parse XML with SimpleXML? Yes, you can do it, but whatever it is you're showing us doesn't look like or ask anything about parsing XML. -
If you don't want to use Composer, you could use the Symfony PSR-4 class loader: https://github.com/symfony/ClassLoader/blob/master/Psr4ClassLoader.php It has no dependencies, and is super easy to use: http://symfony.com/doc/master/components/class_loader/psr4_class_loader.html
-
Sorry, i figured it out already. The relative location is relative to the link, not where the script is running.
-
I'm having trouble with creating symlinks using a shell script. They end up being broken (dangling) unless I use the full path, but I'd like to make this script semi-portable. I'm over-simplifying this: #!/bin/bash if [ ! -f ./lib/electro.php ]; then ln -s ./source/lib/electro.php ./lib/electro.php fi This script is called .install.sh, and is located at ./source/scripts/.install.sh So I go to my terminal, cd to where ./ would reference and run: skunkbad: /var/www/example/application$ ./source/scripts/.install.sh The script does end up creating the symlink in the proper location, but it's a broken link. If I list the directory, it will look something like this: lrwxrwxrwx 1 skunkbad www-data 51 Jun 26 17:40 electro.php -> ./source/lib/electro.php But it's colored red, which is broken / dangling and it doesn't work. My installer script will work as expected if I use an absolute path. What am I doing wrong? Should I somehow insert the absolute path as the script runs?
-
I understand the probing, and have seen that before, but why change the HTTP host too?
-
No, it wasn't a full URL, but the request has always been to a page that doesn't exist. For instance, the site is not a WordPress site, but one of the requests was pointed at /wp-config.php.
-
I've recently starting noticing that I am getting requests where the HTTP_HOST is being spoofed. I don't know how they are doing it, but the server sends me an error message, and it's kind of annoying. What I'd like to know is if something like this in .htaccess will have any negative side-effects: RewriteCond %{HTTP_HOST} !^example\.com$ [NC] RewriteRule .* - [F] I've tested this on my dev machine, and it seems fine. Anything that I should be concerned about? Would it be effective?
-
It's clean, but nothing to get excited about. I think once you add a logo and some real content (with pictures or videos or something) it will look nice. You should show an example page with some content.
-
It sounds like you need to use preventDefault in your javascript to keep the HTML form from submitting.
-
Having spent way too much time parsing and creating XML, I have to ask why XML would be anyone's first choice. I prefer to just serialize the data. If the goal is to store data so you can use it later, then not creating XML means not parsing it later. Serialization retains the data types, which is handy. If you don't need to retain data types, then json encoding is a good option.
-
If you don't see anything in the console, you may need to switch to the network activity tab. It depends what console you are using. How do you know that you are receiving the json response?
-
Accessing global in constructor or parent class
sKunKbad replied to jbonnett's topic in PHP Coding Help
While you're still in the design stage, you might want to rethink your "super object" strategy, but if you insist to proceed, the proper technique for allowing a class to use such an object would be to inject it through the constructor. So at some point you are creating a new Controller, and you would just pass the core variable as a parameter. Also, unless just a typo here in the forum, you'll want to make sure your constructor method is named correctly. __constructor not __construtor -
Ideas for coding a Library / Component / Class ?
sKunKbad replied to nik_jain's topic in Miscellaneous
It would be pretty cool to have a KML generator that allowed for drawing bezier type curves (like illustrator), and created the necessary points so that adding KML shapes to gmaps could included "rounded" objects. I've already got one that draws regular KML, but it doesn't do rounded objects. -
I nice way to do this might be to create a sermon management console with uploader where you can specify the date and time you want. As the upload is being saved, you would save the path to the file, and any other details (like the date and time, name of pastor, topic, etc) in a database table (sermons_data). Later, if you decide to change anything about the sermon, you would just go back to your console and make the edit. Although wretched as an application, WordPress as a CMS using custom post types would handle this well for you. Even if nobody ever sees the WordPress app, you could just use it to populate a database, and then pull in the content through custom queries. I do this a lot because WordPress is a bloated hog.
-
For instance, I was working with KML files that were downloaded from the US census bureau. These are basically just really big XML type files, but regardless of what they are, Sublime Text 2 and gedit just don't handle them very well. Another example would be a database dump (MySQL) file that I want to search and replace the domain name. Some of these files are holding 100 tables worth of data. In maybe both cases I can just use the command line and use sed, but it would be nice if these text editors just worked and worked fast. What do you suggest for a text editor that loads the file in chunks (for Linux)?
-
I found one that seems to work well with large files: http://www.scintilla.org/SciTE.html I was able to do a search and replace on a file that was 170MB, and it only took about 5 seconds. Still curious about upgrading the RAM.
-
I've got kind of a basic computer that I built. Intel i5 processor with 8GB RAM and 1TB HDD. The operating system is Ubuntu 14.04. The problem I'm having is that I use Sublime Text 2 or gedit for working with text files, and large files are constantly freezing up either program. As an example, I copy and pasted 17MB of text into a file, and just trying to save it made the window go dark. It came back to life a few seconds later, but on many occassions it just permanently freezes. Back when I used to use Windows, I used to use Sublime Text 2 and Notepad++, and Sublime was always slow, but Notepad++ was always awesome. Unfortunately, Notepad++ is not available for Linux, and I don't want to run it in a virtual environment just for big files. It seems like Linux should have something that is just as fast. So, do you think upgrading to 16GB of RAM would make a difference? Is there a text editor that I could use on Linux that is fast for working with big files? Thanks for your advice.