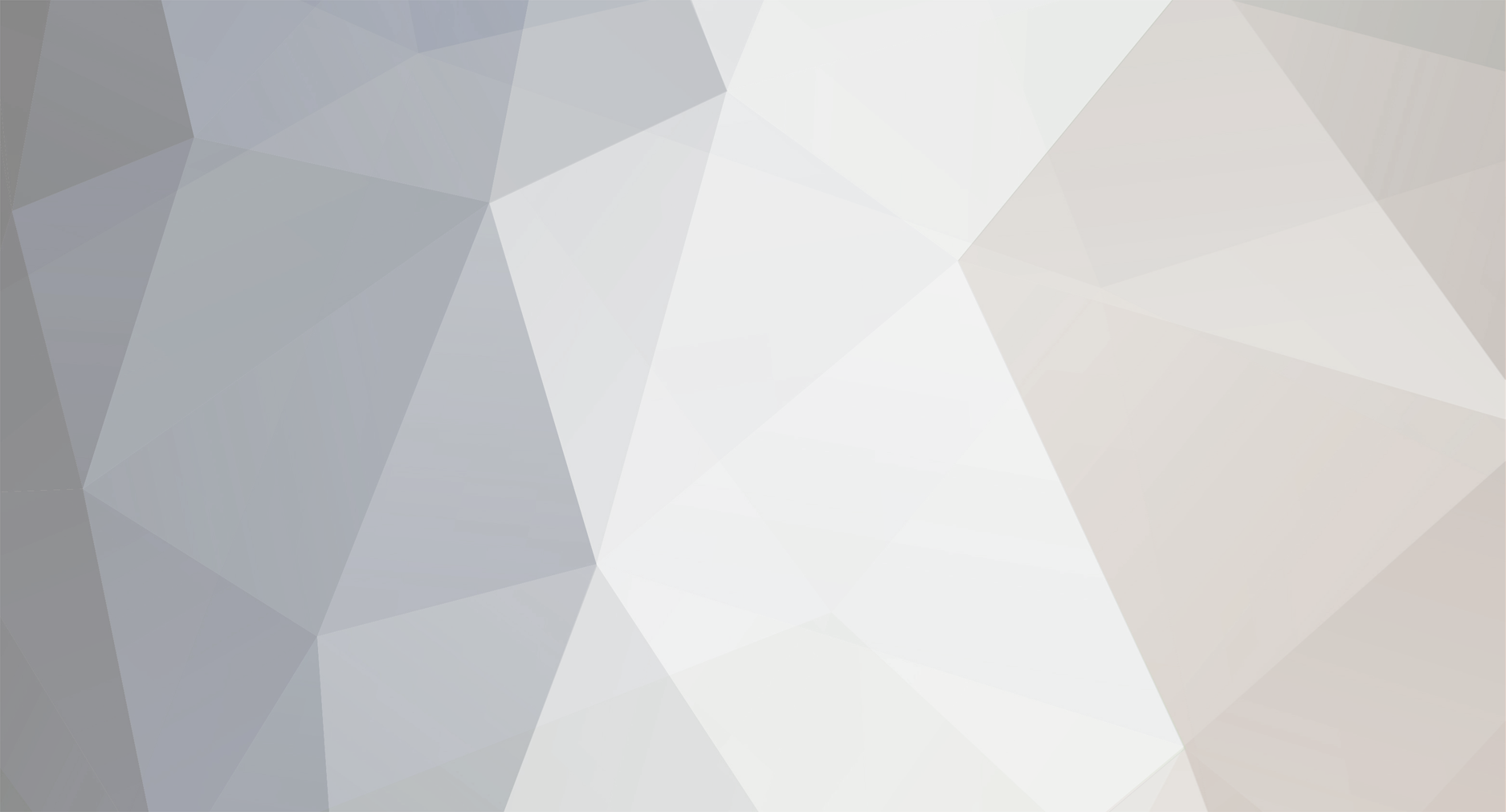
Ninjakreborn
Members-
Posts
3,922 -
Joined
-
Last visited
Everything posted by Ninjakreborn
-
OK finally got it. First off the class was for some reason recognizing these images as Jpg's instead of PNG files..so I manually passed the filetype 'IMAGETYPE_PNG' as the type. So now that it was taken care of I used the following code: <?php function output($image_type=IMAGETYPE_JPEG) { if( $image_type == IMAGETYPE_JPEG ) { imagejpeg($this->image); } elseif( $image_type == IMAGETYPE_GIF ) { imagegif($this->image); } elseif( $image_type == IMAGETYPE_PNG ) { imageAlphaBlending($this->image, true); imageSaveAlpha($this->image, true); imagepng($this->image); } } ?> All it took was the alphablending set to true and save it before doing the actually imagepng function. This finally works, and seems to work correctly in all browsers. Thanks for all the help.
-
If it helps the PHP version is 5.2.13. The GD information is: Could some of that data have anything to do with why this isn't working?
-
I just tried that and it didn't work. I also tried a few bits of other debugging work and nothing is working. I do not understand. This is a standard image. I can take any PNG file directly as is, with no resizing or any other changes and just output it. It's still distorted. As of right now I am doing no resizing at all. I have checked..even tried commenting out the resize code..then verified the parameters I am passing is (0, 0) is not causing a resize. I do not understand exactly what is happening here. Is it something to do with the headers I am used..I have verified it's using the one for PNG only. I am confused. Google doesn't seem to be causing any issues... it's not showing up anything related to the issue I am having and all of those issues I have tested for. Some stuff about IE only transparency issues which isn't the case here because it's happening in all browsers (although I tried it there as well). I tried the other changes you suggested and nothing else is seeming to work. The same code on a Jpg works perfectly but anytime I try to do a PNG file it just returns either a pure black image or an image with a lot of black around the edges. It can't be specifically the way those PNG's are made because I have gotten PNG files from quite a few sources to test this out thouroughly. If you think of anything else or have any more advice let me know. The strange thing is the Jpg files are working perfectly..and they ARE being resized. The PNG's aren't even being resized but their still causing this issue. I don't get it.
-
I tried this in a variety of ways as you mentioned. I did this to the resize function: <?php function resize($width,$height) { $new_image = imagecreatetruecolor($width, $height); $transparent = imagecolorallocatealpha($new_image, 255, 255, 255, 127); imagefilledrectangle($new_image, 0, 0, $width, $height, $transparent); imagecopyresampled($new_image, $this->image, 0, 0, 0, 0, $width, $height, $this->getWidth(), $this->getHeight()); $this->image = $new_image; } ?> I have also tried disabling resize so the image comes out as it normally would without any resizing..neither of those work. Also on upload I am doing nothing (no resizing or nothing) so the PNG's that are uploaded are uploaded unaltered (what I am doing here is on the fly on a page by page basis). So basically now I have tried all things with resizing...I even grabbed a test PNG off wikipedia and it actually shows up but still has a lot of black in the background. I am running out of ideas here. Not sure how to approach this issue. Any more advice?
-
I am having issues with PNG files showing strange black stuff in the background. I am using the following PHP image class: <?php if ( ! defined('BASEPATH')) exit('No direct script access allowed'); /* * File: SimpleImage.php * Author: Simon Jarvis * Copyright: 2006 Simon Jarvis * Date: 08/11/06 * Link: http://www.white-hat-web-design.co.uk/articles/php-image-resizing.php * * This program is free software; you can redistribute it and/or * modify it under the terms of the GNU General Public License * as published by the Free Software Foundation; either version 2 * of the License, or (at your option) any later version. * * This program is distributed in the hope that it will be useful, * but WITHOUT ANY WARRANTY; without even the implied warranty of * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the * GNU General Public License for more details: * http://www.gnu.org/licenses/gpl.html * */ /* Usage Examples http://www.white-hat-web-design.co.uk/articles/php-image-resizing.php Save the above file as SimpleImage.php and take a look at the following examples of how to use the script. The first example below will load a file named picture.jpg resize it to 250 pixels wide and 400 pixels high and resave it as picture2.jpg <?php include('SimpleImage.php'); $image = new SimpleImage(); $image->load('picture.jpg'); $image->resize(250,400); $image->save('picture2.jpg'); ?> If you want to resize to a specifed width but keep the dimensions ratio the same then the script can work out the required height for you, just use the resizeToWidth function. <?php include('SimpleImage.php'); $image = new SimpleImage(); $image->load('picture.jpg'); $image->resizeToWidth(250); $image->save('picture2.jpg'); ?> You may wish to scale an image to a specified percentage like the following which will resize the image to 50% of its original width and height <?php include('SimpleImage.php'); $image = new SimpleImage(); $image->load('picture.jpg'); $image->scale(50); $image->save('picture2.jpg'); ?> You can of course do more than one thing at once. The following example will create two new images with heights of 200 pixels and 500 pixels <?php include('SimpleImage.php'); $image = new SimpleImage(); $image->load('picture.jpg'); $image->resizeToHeight(500); $image->save('picture2.jpg'); $image->resizeToHeight(200); $image->save('picture3.jpg'); ?> The output function lets you output the image straight to the browser without having to save the file. Its useful for on the fly thumbnail generation <?php header('Content-Type: image/jpeg'); include('SimpleImage.php'); $image = new SimpleImage(); $image->load('picture.jpg'); $image->resizeToWidth(150); $image->output(); ?> The following example will resize and save an image which has been uploaded via a form <?php if( isset($_POST['submit']) ) { include('SimpleImage.php'); $image = new SimpleImage(); $image->load($_FILES['uploaded_image']['tmp_name']); $image->resizeToWidth(150); $image->output(); } else { ?> <form action="upload.php" method="post" enctype="multipart/form-data"> <input type="file" name="uploaded_image" /> <input type="submit" name="submit" value="Upload" /> </form> <?php } ?> */ class Simpleimage { var $image; var $image_type; function load($filename) { $image_info = getimagesize($filename); $this->image_type = $image_info[2]; if( $this->image_type == IMAGETYPE_JPEG ) { $this->image = imagecreatefromjpeg($filename); } elseif( $this->image_type == IMAGETYPE_GIF ) { $this->image = imagecreatefromgif($filename); } elseif( $this->image_type == IMAGETYPE_PNG ) { $this->image = imagecreatefrompng($filename); } } function save($filename, $image_type=IMAGETYPE_JPEG, $compression=75, $permissions=null) { if( $image_type == IMAGETYPE_JPEG ) { imagejpeg($this->image,$filename,$compression); } elseif( $image_type == IMAGETYPE_GIF ) { imagegif($this->image,$filename); } elseif( $image_type == IMAGETYPE_PNG ) { imagepng($this->image,$filename); } if( $permissions != null) { chmod($filename,$permissions); } } function output($image_type=IMAGETYPE_JPEG) { if( $image_type == IMAGETYPE_JPEG ) { imagejpeg($this->image); } elseif( $image_type == IMAGETYPE_GIF ) { imagegif($this->image); } elseif( $image_type == IMAGETYPE_PNG ) { imagepng($this->image); } } function getWidth() { return imagesx($this->image); } function getHeight() { return imagesy($this->image); } function resizeToHeight($height) { $ratio = $height / $this->getHeight(); $width = $this->getWidth() * $ratio; $this->resize($width,$height); } function resizeToWidth($width) { $ratio = $width / $this->getWidth(); $height = $this->getheight() * $ratio; $this->resize($width,$height); } function scale($scale) { $width = $this->getWidth() * $scale/100; $height = $this->getheight() * $scale/100; $this->resize($width,$height); } function resize($width,$height) { $new_image = imagecreatetruecolor($width, $height); imagecopyresampled($new_image, $this->image, 0, 0, 0, 0, $width, $height, $this->getWidth(), $this->getHeight()); $this->image = $new_image; } } ?> In order to call the file I am just calling it via an image tag..and using the following code (this is in a code ignitor framework) <?php function show_image($image_name, $height = 0, $width = 0, $dir = 'user_photos') { // Get extension $characters = 4; $length = strlen($image_name); $start = $length-$characters; $extension = substr($image_name, $start, $characters); // Show header based off extension switch($extension) { case '.jpg': header('Content-Type: image/jpeg'); break; case '.gif': header('Content-Type: image/gif'); break; case '.png': header('Content-Type: image/png'); break; default: header('Content-Type: image/jpeg'); break; } // Load image/resize/display $this->simpleimage->load(base_url() . 'public/assets/' . $dir . '/' . $image_name); // Resize based on passed parameters if ($height != 0 && $width == 0) { $this->simpleimage->resizeToHeight($height); }else if ($width != 0 && $height == 0) { $this->simpleimage->resizeToWidth($width); }else if ($height != 0 && $width != 0) { $this->simpleimage->resize($width,$height); } // Perform resizing based off parameters $this->simpleimage->output(); } ?> I have this same thing setup as you can see for jpeg's and gifs as well. The only file it's messing up is the PNG files...I have tried on multiple files for PNG type. Some of them show nothing but a black image while others show part of the original image surrounded by black background. I have been able to find out from google that it is something related to a Transparency issue...this is happening in all browsers...I even visit the image page directly and see it directly output and it's the same issue. It happens in every single browser. Any advice would be greatly appreciated, at this point I am out of ideas. I have tried a variety of image handling functions that I tried adding into the class to see if it started working and nothing is making that black background stuff go away. Thanks.
-
I have fought with image handling for a long time. I have done it manually, as well as used third party tools. I always seem to have issues with image handling of any type however. Anything dealing with resizing, cropping, or image work I end up having issues with. So I have a few questions. What is the best way for me to approach dealing with images. I mean the whole nine yards...validating filetype, validating size, performing resizing/cropping, and reducing filesize. Preferably I would rather use one of those image.php files that do it via a url call but I haven't been able to find one that does what I need. Currently I am needing to resize images down to 80 pixels height...then reducing the width down to scale based off the height without reducing scale quality. I also need to reduce the filesize as well so it's more acceptable for that size of an image. Is there an easy way to do this using one of those third party image.php files or is something like this really easy to do on upload?
-
I have a display class I have started using in Codeignitor to do all of my display stuff. I just threw it in Libraries and started using it as one of the built in Codeignitor libraries (by following their naming conventions. For the first time I have tried to use another library inside my display class and it throws an error that it's not a class. I am simply trying to call the $this->session->flashdata() inside the display class. Is there another way to do this, or am I doing it wrong?
-
I also just tried something along the lines of: <?php //>> GET DATA AND SET LOCAL DATA OBJECTS $objDataCollection = $objData->get_news(); function user_sort($a, $b) { $date1 = strtotime($a['news_date']); $date2 = strtotime($a['news_date']); if ($date1 == $date2) return 0; return ($date1 > $date2) ? -1 : 1; } uasort($objDataCollection, user_sort); ?> <?php //>> GET DATA AND SET LOCAL DATA OBJECTS $objDataCollection = $objData->get_news(); function user_sort($a, $b) { //$date1 = strtotime($a['news_date']); //$date2 = strtotime($a['news_date']); if ($a == $b) return 0; return ($a > $b) ? -1 : 1; } uasort($objDataCollection, user_sort); ?> This almost works but not entirely...for some reason some dates are off. Any advice?
-
I am probably using it wrong but this isn't working. function user_sort($a, $b) { if (strtotime($a['news_date']) > strtotime($a['news_date'])) { return 1; } } uasort($data, user_sort); The data object looks like this. Any one can help me figure out why this isn't working? I basically just want to short the array by the "news_date" value with the newest values showing up first.
-
I have dates in the DB set as varchar (not datetime) and the format '5/13/2009'. So I want to get results from the database that are sorted by this date...the newest ones would show first. I have tried an order by on the "news_date" field which is the date column but it makes no difference. Any advice?
-
My brain must have been fried. $logged = $user->check_login(); if ($_SERVER['PHP_SELF'] != '/gateway.php') { if ($logged == false) { header('Location: gateway.php'); } } That works..it was on the gateway page and causing this issue. Looks all worked out now, thanks.
-
With the header redirect it won't send over the form variables without you attaching them to the url String then you have to adjust how they are obtained by using get or request instead of post. I would get rid of header ridirect on a multi-page form and do it the old way. Also make sure you have session_start() at the top of each page or it could drop sessions off. Post your full code here if you need more help (all code) with sensitive data edited.
-
State saving: 1) Session/Cookie variables 2) Hidden field I would show the data on the same page that you are getting the data. You'll have to get the data again anyway if there is a large amount of data.
-
Post the entire code. It looks like your missing an ending tag but I can't tell until I see the full code.
-
class All Tested. // This handle login checking, gateway checking, and permission structuring. function check_login() { // Need to make sure they have a permission level. if (empty($_SESSION['territory']) && empty($_SESSION['area']) && empty($_SESSION['logged']) && empty($_SESSION['membership_level'])) { return false; } if ($_SESSION['logged'] == 1) { return true; }else { return false; } } Config File (Included in every file in the system..this is the file that causes the redirect issue. <?php /* Joyel Portable Framework */ session_start(); // Global session start // variables Edited // Connect to database EDITED // Core Libraries require_once('autoload_classes/functions.class.php'); require_once('autoload_classes/db.class.php'); require_once('autoload_classes/user.class.php'); require_once('autoload_classes/display.class.php'); // Optional Classes - These are optional. They are required here, but they are not instantiated until as needed. require_once('optional_classes/file.class.php'); require_once('optional_classes/math.class.php'); $logged = $user->check_login(); if ($logged == false) { header('Location: gateway.php'); } ?> Now when this script is called in gateway.php <?php require_once('framework/config.php'); .... ?> It throws this error: Any advice?
-
Basically I am wondering if it's possible to get Java Midlets installed "somehow" in the specific reality model? I have seen people do it on many other types of Samsung phones..but have no idea how to get it accomplished on the Reality. I mean like midlets at www.getjar.com (a site that was recommended to me) or other similar app sites.
-
Does anyone here use a Samsung Reality phone (one of the newer samsungs that came out? If so I had a quick question.
-
OK. I have a custom error handler function. I am setting that as a callback to the PHP error handling system: set_error_handler("user_error_handler"); user_error_handler is the custom function. It just databases the error data when PHP throws them. That all works fine. I am also using it as a custom function call sometimes. In one situation I have a "Code" parameter I am passing based off some authorization stuff from a third party system. I am doing a print_r on the code and for some reason it's printing EVERYTHING. The post, get, files, global, database objects, and every single thing in the entire system. I do not understand why it is doing this. I am passing it a code...when I try to print_r the code on the actual page it comes up empty, when I do it inside the function it prints out every single variable in the system including the DB configuration options and everything else. What reasons would cause print_r to do a system wide print?
-
By the way, I mis-told you on part of that. I can access it in those other files, just not inside of functions. This is related to scope. Is there a way I can make that class global. SO it'll be usable in and out of any functions. I may want to throw a few debug calls right inside of other functions that are dealing with loops and it's ignoring that..I don't want to have to pass my class by reference to every function or it'll just defeat the purpose of the time I am trying to save. IS this possible?
-
I have a debug class within portable_framework.php. <?php class Debug { function print_multiple($title, $array, $title2 = 'empty', $array2 = array(), $title3 = 'empty', $array3 = array(), $title4 = 'empty', $array4 = array()) { echo $title.'<br />'; echo '<pre>'; print_r($array); echo '</pre>'; echo '<br />'; if ($title2 != 'empty') { echo $title2.'<br />'; echo '<pre>'; print_r($array2); echo '</pre>'; echo '<br />'; } if ($title3 != 'empty') { echo $title3.'<br />'; echo '<pre>'; print_r($array3); echo '</pre>'; echo '<br />'; } if ($title4 != 'empty') { echo $title4.'<br />'; echo '<pre>'; print_r($array4); echo '</pre>'; echo '<br />'; } } } $debug = new Debug(); ?> This is a very simple test case to prove my point. I have this portable_framework.php included at the top of my master file..results.php. In results.php right after the require of that class file it let's me access that class. I have another file called "link_parser.php" that is included in the results.php further down. This is setup to allow me to cut my code up some so it's not all in one place. Here is hte issue. I can't access my Debug class inside hte link_parser.php at all. It's like the class being required into the results.php is accessible there, but not in other files that are included later on. Is that normal, and if so is there a way I can include a class file and have it work throughout the entire system..even if there are a few levels of includes?
-
echo <<<END This uses the "here document" syntax to output multiple lines with $variable interpolation. Note that the here document terminator must appear on a line with just a semicolon. no extra whitespace! END; What is this operator or setup called? I am trying to find the name, so I can look for an equivalent in C++, but I can't recall it's official name.
-
C++, How to manage the logic..
Ninjakreborn replied to Ninjakreborn's topic in Other Programming Languages
Perfect, thanks for the feedback. -
C++, How to manage the logic..
Ninjakreborn replied to Ninjakreborn's topic in Other Programming Languages
Wait..I am very, very confused now. I thought a C++ application was like a PHP application...Meaning it went line by line and ran the code as it went. Now based off this code...it works differently? I got a little lost here. However I implemented your code, and it runs it forever..like the while loop keeps going eternally and reshowing the choices over and over again very rapidly. Not to mention when I do it like this: bool validChoice = false; while(!validChoice) { switch(response) { case 1: std::cout<<"go to police station"; validChoice = true; break; case 2: std::cout<<"call the police station"; validChoice = true; break; case 3: std::cout<<"With a heavy heart you realize you just are not cut out to be a police officer. You crumble the letter up, roll it up into a" <<" ball and throw it into the garbage."; exit(1); break; default: std::cout<<"Invalid Choice"; std::cout<<choices; } std::cin.get(); } It does work to an extent...however it doesn't reflect the choices..so the first time if they select 6, which is an invalid choice.. then they select 1 it still goes as an invalid choices...it doesn't replay that switch statement. If that makes any sense. I was afraid of that, I know the while look will allow me to reshow the same choices but won't let me rerun the same switch statement. -
I am totally lost here... std::string choices = "You can:\n1: Go to the police station.\n2: Call the police station.\n3: Throw away the mail you got from the police station.\n\n"; std::cout<<choices; int response; std::cin>>response; std::cin.ignore(); switch(response) { case 1: std::cout<<"go to police station"; break; case 2: std::cout<<"call the police station"; break; case 3: std::cout<<"With a heavy heart you realize you just are not cut out to be a police officer. You crumble the letter up, roll it up into a" <<" ball and throw it into the garbage."; exit(1); break; default: std::cout<<"Invalid Choice"; std::cout<<choices; break; } Very straightforward. Prevent choice..based off their choices perform actions...now what happens if they perform an invalid choice. It reloads the choices..but then exits out of the system. From a logic standpoint..how would I present the list of choices again, as well as let the switch statement take affect again when a choice was selected the second time..In PHP for example your able to reshow a form if it's been entered incorrectly and still submit...do I have to put that switch statement into a function as well...but there are going to be tons of switch statements throughout this entire application..I am wondering if I have to have a separate one for each set of choices? Any advice on this issue?
-
Nevermind..it was me using the compare function wrong. if (std::strcmp(answer, "yes") == 0) { std::cout<<"Please stand by while the data is being gathered and the file is being generated...\n"; std::ofstream myfile; myfile.open("C:/Users/businessman332211/Desktop/example.txt"); myfile<<"This is data."; myfile.close(); return 0; } That is the right way.