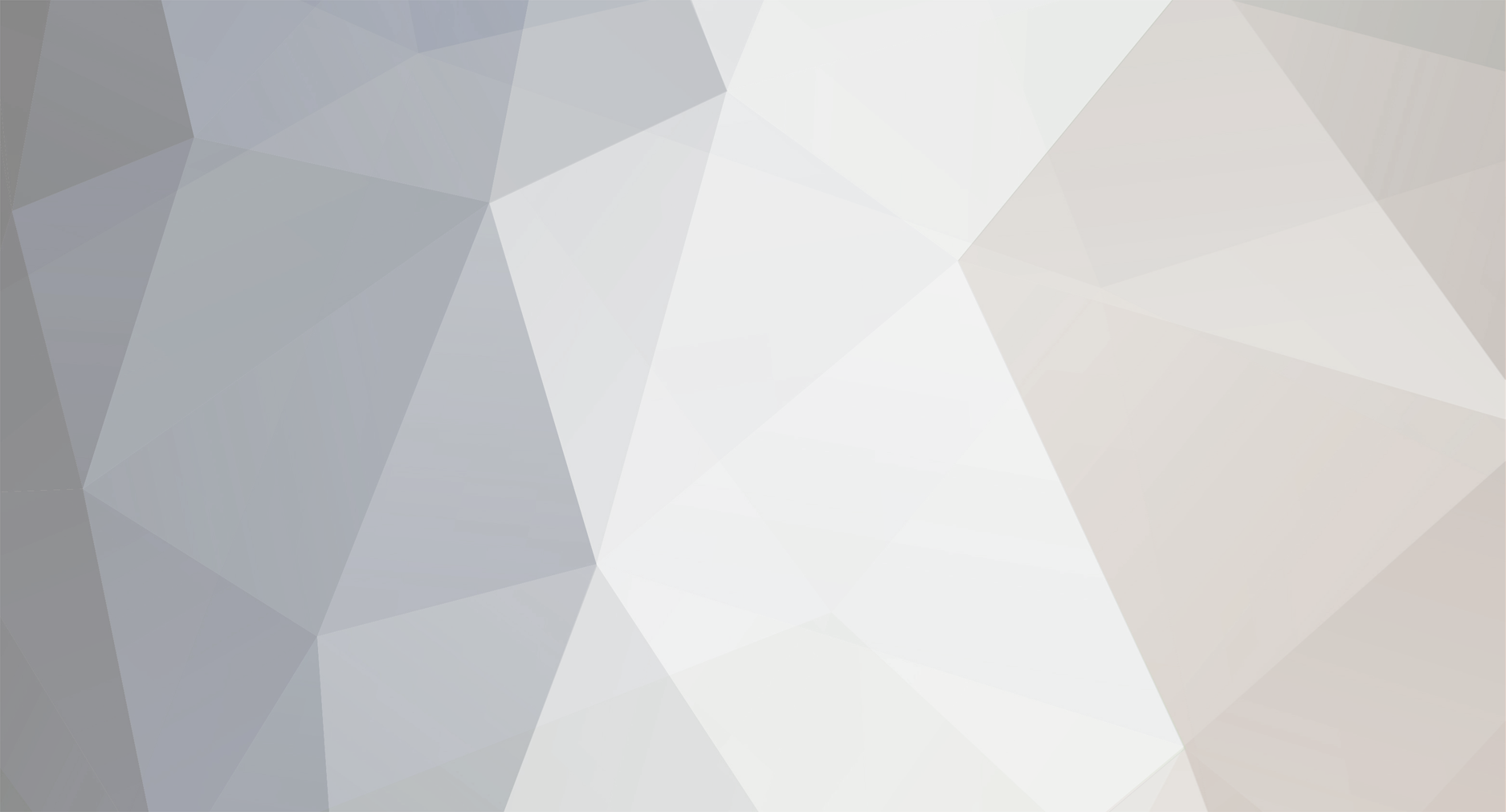
Ninjakreborn
Members-
Posts
3,922 -
Joined
-
Last visited
Everything posted by Ninjakreborn
-
I have been working on a site in Zend Framework. Basically there was another developer long ago who set up the basic inner workings of this code. I have just been building onto it. I was almost done and just getting ready to do some file handling and I ran into what appears to be a simple issue..I can't get the last mysql ID. Generally I would just call the mysql function to get that. Or I would run a Mysql Query to get it directly. Neither one of those methods work here. It seems it's not connected to the database unless actually using the class. So after failing to do that, I started doing some more research into the way you can handle databases in Zend Framework..obviously I found it in the documentation. Here is my code. <?php public function getTable() { if(null === $this->_table){ require_once APPLICATION_PATH . '/models/DbTable/Projects.php'; $this->_table = new Model_DbTable_Projects; } return $this->_table; } // Save a new record public function save(array $data) { $table = $this->getTable(); $fields = $table->info(Zend_Db_Table_Abstract::COLS); foreach($data as $field => $value){ if(!in_array($field, $fields)){ unset($data[$field]); } } $table->insert($data); $table->lastInsertId(); return $last_id; } ?> Here is the link were I found the documentation: http://framework.zend.com/manual/en/zend.db.html Here is what it says: I followed that. Obviously I am not using DB class because he set it into Table. When I do what this recommends I get the following error via Ajax: I have tried using the original Mysql method, I have tried writing a query for it, and I tried it like the documentation say's. That is all the documentation says about it. Anyone have any advice?
-
For the spider that is a good price. A very good price. I would probably have charged about the same. I am building SEO tools for a company. Been working on quite a few and a lot of them deal with spiders..some are really advanced (3k or so) while others are simpler (1500 or so). But you should fluctuate what you charge. Most of the time I charge a flat 30.00 per hour. Sometimes I get paid as much as 50. There is a current client that "offered" me 50.00 per hour off the start with a chance of around 200 hours per month. So a lot of time it depends on the skill level. But you never stop learning. I took on a few projects recently that I knew how to do but new elements came up during development and I had to learn some new stuff. That happens..generally I don't charge for the hours "learning". What you need is good time tracking software (good luck with that I am still trying to find the right tools for that). SO as you can see..I have worked for as little as 30 to as much as 50 per hour. It depends on the client, their budget, and their generosity a lot of times. Depends on what they feel is a fair amount. Don't go too low..set a marker. For your range I would set a flat range of NO LESS than 20.00 per clocked hour. Track your hours. When your "working" clock those, when your learning clock those separate. The best way is to get a timer with a stopwatch (as I said I haven't found the right tool for me yet). Go through and clock the hours as your working..if you stop to look something up on Google or learn something you don't know, stop the timer. Learn it, then start clocking again when your making viable progress on the system again. Overall your on the right track, just keep knocking stuff out.
-
Looking through those now. A lot of those I have tried. There are 2-3 that I haven't tried. I will take a look at them.
-
Quickbooks - It was almost what I wanted...except the time tracking was a bit off. If the time tracking had of been a little better. I want to be able to add Client/Projects for that client/Tasks for that project. Then anytime I want to go to a task I want to be able to click on a task then choose start/stop/pause timer. Then it would add on the hours. Say if I work 3 hours this morning on a specific task then stop. I go and do some things and want to come back and work more on that task. I put another 2 hours into that same task...after I am done I can move onto whatever other tasks I want. That kind of setup. It's timer software just wasn't what I needed. Beyond that I have tried a lot...let's see. Rescue Time, Kronos, replicon, http://en.wikipedia.org/wiki/Comparison_of_time_tracking_software <- Almost everything there (looking for desktop but I tried to web based one's as well). I also tried easytimetracking, my timeforce, acti-time (web based), complete-time-tracking, dovico, ace project. I tried many many more. Almost everything I could find on google, as well as everything I was able to find on top lists and wikipedia. I have used quite a lot that I could find. I have also found other's that were harder to find..like listed under comments under some of the top lists I found. Some of those I didn't find on google but once I saw someone mentioning them I tried them. I have tried a lot. Just hoping maybe there was one I missed or something.
-
I have been trying to use almost everything on the market. I have tried all time tracking software I can find. I have tried different types of account software. So after digging around myself for months..trying multiple different things I wanted to ask the PHPFreaks community if they know of anything. Questions: Looking for the right type of software. Timetracker/invoicing and hopefully accounting? I was looking for a specific type of software. I wanted to find something that did three main things. Time tracking (for my projects), invoicing, and accounting (light). The two primary features I am looking for is something that would allow me to setup clients, projects, and tasks. Then those tasks (or it could be dates) could be timed. I work by the hour so I would need to be able to utilize a timer. I mean start/stop/pause. I would also like something that has invoicing built in..atleast something halfway decent. I would also like to try to find something that did quite a bit of accounting so I can track and deal with all of my finances as well..I wanted to be able to enter what bills I owe and what dates, which ones are recurring and keep track of practically all of my financial information. Is there anything like that? The timetracking is something like they have at GetHarvest. I used it for awhile and it's a web based system that is almost what I wanted.I just wanted to find something for desktop..and hopefully something that has the accounting built in.
-
Yes, I have other issues as well. That's because I am trying to use the stupid site_id as both a primary key and everything else. I am going to create me another field which is an Auto-Incing normal ID and use it as my identifier. That'll make editing the site_id a lot easier. I should have done that in the first place but this was an issue because I was taking over someone else's database which was quite an issue.
-
Out of on error and into another. I went through and did some more reading, as well as based off of what you said. I went ahead and set them all to cascade. Since it was editing the site_id and that was something that was set as a foreign key to 4-5 tables, I had to go through and set them all to cascade. Once I did that, it started letting me edit. However after editing more than once it returned another error. In this situation it's the same thing. It's a simple edit statement (which I showed earlier).
-
I am not trying to delete the record. I am trying to edit the record. I need to be able to delete as well...but right now I would be happy just getting the editing/adding working for the time being.
-
Below is some additional clarification. First off I have my function which gets the fields from the form: <?php // The grid calls this function when a site is edited public function editsitefieldsAction() { // Disable layout $this->_helper->layout()->disableLayout(); // Setup request and get the needed parameters $request = $this->getRequest(); $site_id = $request->getParam('site_id'); $fields['site_id'] = $request->getParam['site_id']; $fields['site_name'] = $request->getParam('site_name'); $fields['site_description'] = $request->getParam['site_description']; $fields['api_url'] = $request->getParam['api_url']; $fields['api_site_id'] = $request->getParam['site_id']; $fields['api_username'] = $request->getParam['api_username']; $fields['api_password'] = $request->getParam['api_password']; $fields['api_generation_id']= $request->getParam['api_generation_id']; $fields['api_usage_id'] = $request->getParam['api_usage_id']; $fields['api_weather_id'] = $request->getParam['api_weather_id']; $fields['is_active'] = $request->getParam['is_active']; $fields['timezone_offset'] = $request->getParam['timezone_offset']; // Now let's database the results $model = $this->_getSitesModel(); $model->update($fields, $site_id); } ?> Then I have my model save function which deals with saving the data: <?php public function update(array $data, $id) { // Get table information $table = $this->gettable(); // Form where clause $where = $table->getAdapter()->quoteInto('site_id = ?', $id); // Remove fields that we aren't editing $fields = $table->info(Zend_Db_Table_Abstract::COLS); foreach($data as $field => $value) { if (!in_array($field, $fields)) { unset($data[$field]); } } // Perform edit return $table->update($data, $where); } ?> The strange thing..it's also giving me the same error when I am trying to add a new field, which of course uses a different function but I just don't understand how this same issue can affect me adding a new field as well. Edit: Also one other note. The table I am trying to do this with is sites. The Sites_photo's should have nothing to do with this table, I don't even know why that error is coming up.
-
That is the situation with this specific table. The Site_ID is the same as another field called Site_Api_ID. They are suppose to be the same. Site_id is not auto-increment as it is something that can be changed by the user. They are allowed to change the site_id and when they change that ID it also automatically sets the api_site_id to the site_id as well. I have no choice but to make that field editable. Since the api id is something that they have to be able to update since it is based off of what API they are connecting to. So yes, in this situation it is updating the site_id. Edit: But my query should not be editing anything with the photo table. I am using Zend Framework built in: return $table->update($data, $where);
-
I really wanted to avoid asking for help, but I had no choice. I tried everything I can think of and this just isn't working. I am working on an internal system. It's basically a CMS for an administrator of a site. I am building one area of CMS for each table. Projects, Sites, Energy, and Meters. I finished up projects. I finished it up within a Jquery Jgrid as well as added add/edit/delete functionality. The system is built using Zend Framework. All of that was no problem at all. Then I went to do the sites. I basically reused the same code since I already had a lot of the stuff for Jgrid done that allowed me all the ajax add/edit/delete functionality. I hooked up all the backend and went to test it. Ran into a few issues which I easily fixed. Then ran into a new problem I have never dealt with before. It came back with some error about a constraint. The error was something along the lines of: That basically was the error I got. So I did some searching on the error. I have never dealt with or used Constraints before so I was unfamiliar with the concept. After a few minutes I went through and started setting the constraints to "No Action" because I thought it would allow me to edit/delete the rows. We'll it didn't. I did some more reading and found out the constraint no action doesn't allow you to process an update or a delete. All I am trying to do is the person goes in and a form comes up with the data prepopulated into the form for that record. They edit some fields and submit it back to the database. It always returns that error. Even if I try to submit the form with no fields change...so even without changing any fields it still returns that error. I was thinking about going through a deleting all the foreign ID's, but I am somewhat familiar with them. I have never had this type of issue. Does anyone have any advice?
-
JavaScript Minification what are your opinions on it?
Ninjakreborn replied to Ninjakreborn's topic in Miscellaneous
I agree. I minify when the client requests it. When I have a choice I end up using the original JS files. The reason I don't like minifying is because I like being able to look through the code. I only do it when the client requests it. I also sometimes modify the base javascript file or extend them so I need to see them to be able to do that. -
I "normally" create one controller for each "type" of things then put different functions to handle the actions. So Users would have it's own controller. Then inside the users controller there would be a function: login, logout, forgotpassword, and everything else. Those deal with the login page, logout page and so forth. This is generally how I set up my controller. There are even times when there are a lot of misc things I need but there so small they may not need a controller I use on called misc controller. That's what I do. I use the same naming convention in Codeingitor, cakephp, zend, and the others. Almost anything that deals in MVC, that's how I name them.
-
I do it most of the time. What are your thoughts on minification?
-
Ok...strange issue. I have a multi-adding system. It allows a user to input data then add similiar items. It is suppose to create the new form on a slider and moves the values from the old form to the new form. Nothing is submitted yet, it's all just in xhtml/css/javascript. They can add as many as they want then submit them all at once. The only field I can't seem to pass is the file. I want to be able to pass the photo from one form to the next. var inputs = document.forms["itemAddMultipleForm"].elements; for(var n = 0; n < inputs.length; n++) { if(inputs[n].id.replace('item['+assetType+']['+fromItem+']', '') == inputs[n].id) continue; var fromElem = $(inputs[n].id); var toElem = inputs[n].id.replaceAll('item['+assetType+']['+fromItem+']', 'item['+assetType+']['+toItem+']'); toElem = $(toElem); //for (x in fromElem) { // alert(x); //} switch(fromElem.type) { case "hidden": case "textarea": case "text": toElem.value = fromElem.value; break; case "select-one": toElem.selectedIndex = fromElem.selectedIndex; break; case "checkbox": toElem.checked = fromElem.checked; break; case "file": toElem.value = fromElem.value; break; } You can see were I added case "file" to try and do it..I tried moving value from the other to the new..and it's not working. Any advice.
-
[SOLVED] Strange array issue, never happened before.
Ninjakreborn replied to Ninjakreborn's topic in PHP Coding Help
This is a good idea. Something to think about. Another good idea, something else to think about. A good idea, but not a valid solution in my situation. I don't really have authority to adjust the ini settings. Thanks again this should get me going. -
[SOLVED] Strange array issue, never happened before.
Ninjakreborn replied to Ninjakreborn's topic in PHP Coding Help
I don't really have a choice. I need the results for a variety of different export types. If I was doing straight Export to xlS then it would be fine building the excel output straight away (which would work). What I need is have access to excel, filemaker, doc, pdf, csv, and a few other different types of exports. -
[SOLVED] Strange array issue, never happened before.
Ninjakreborn replied to Ninjakreborn's topic in PHP Coding Help
It's right there just as I posted it. The first one is there for testing...as I stated before. The second one is there for testing. The purpose of this is to find out why the first one is showing up and hte second one isn't. As I mentioned in the first post. I KNOW it is suppose to be, but it's not. No there is not more to it than is shown. As far as the issue I think I found out what it is, but why I don't know. When I run a full query (32,000 something results) it's not working in this location. When I limit it down to roughly 200 it works fine. I need to find a way to allow it to return all of the results. -
[SOLVED] Strange array issue, never happened before.
Ninjakreborn replied to Ninjakreborn's topic in PHP Coding Help
Just to simplify things here is the new code. <?php $sql = 'SELECT * FROM assets_resources'; #### Run Query #### $assets = array(); //This is the master array that will contain all results. $query = mysql_query($sql); while ($row = mysql_fetch_array($query, MYSQL_ASSOC)) { $assets[] = $row; echo '<pre>'; print_r($assets); echo '</pre>'; } echo '<pre>'; print_r($assets); echo '</pre>'; ?> The first print_r is shown correctly, the second one is not. -
[SOLVED] Strange array issue, never happened before.
Ninjakreborn replied to Ninjakreborn's topic in PHP Coding Help
That was the first thing I thought, but no. So far all the code is bits and pieces of a larger export system I am putting together. Now, the whole code your looking at now is under one action. I am using "action" parameters to decide what is going to happen on the page. All of this code however is under the same action Export_Data so that shouldn't have an effect. Beyond that there is nothing. I have a lot of parameters that are "going" to affect the query later on down the road (when the code is more developed) but for the time being this is the basic application. -
[SOLVED] Strange array issue, never happened before.
Ninjakreborn replied to Ninjakreborn's topic in PHP Coding Help
Flag all is something else. It does not affect the code I am currently running. I have verified the code is running with the flag. It's not meant to run without. This is part of a much larger system, this is just one piece that is being built. For the time being I am trying to use the data array immediately after. I have an echo '<pre>'; print_r($data); echo '</pre>'; right after the while loop. If I do that inside the while loop it shows the array as it's being built..the first time through has $data[0] the second time through had $data[0] and $data[1] the third time is $data[0], $data[1], and $data[2] and so forth. However...when I do it right outside of the while loop however it's like the $data array isn't even set at this point for some reason? At the very least it should be detected the empty array at the top..strange situation. -
mysql_fetch_array returns 1 array per call. Generally that's why it is inserted into a while statement. I generally loop through them in a while statement and put them all in a master array so I have all the results in one place instead of having to use them on the spot in the while loop. So here is what I generally do: // If the flag all is set then run an "all" query. else we need to do some specifics on the query instead. <?php if ($flag['all'] == 1) { $sql = 'SELECT * FROM data_resources'; }else { $sql = ''; } #### Run Query #### $data = array(); //This is the master array that will contain all results. $query = mysql_query($sql); while ($row = mysql_fetch_array($query, MYSQL_ASSOC)) { $data[] = $row; } ?> If I echo out the data array inside the while statement it works properly, but when I try to access it outside of the while it doesn't work. What is going on? Normally this works just fine.
-
Force reload after form-launched download
Ninjakreborn replied to geminidomino's topic in PHP Coding Help
I gave up for the time being. Nothing I tried worked. Everything I found on Google states it's not possible. I have tried: 1) JavaScript refresh 2) PHP Redirect 3) Iframe with form..then refresh Iframe. I have tried another variety of little tricks, work-around, and hacks and nothing is working. This seems to be a lost cause. The only thing I haven't tried is actually building the file and save it to a location then prompt for download..but I don't feel like going to all that trouble..and in-theory it sounds like it would cause the same issue. Naturally..this would be nice for the "user-friendly" department..but it's not coming together. It's standard functionality..but I don't know if there "is" a workaround that can be implemented for it to function the way we're wanting. Then the only other solution would be providing a download link..but that just defeats the purpose. I was going to download http://www.formtofile.com/ and see how that does it and see if it has a workaround..but I don't really feel like going to the trouble after everything else I tried. -
Force reload after form-launched download
Ninjakreborn replied to geminidomino's topic in PHP Coding Help
I have something I am trying now that might work, give me a minute. -
Force reload after form-launched download
Ninjakreborn replied to geminidomino's topic in PHP Coding Help
This has me stumped. After reviewing your issue and my own...I am running out of ideas. Header doesn't work because it's already being passed. window.location doesn't work, opening it a new window doesn't work. The first 50 pages worth of google for 3-4 different terms doesn't work. Still looking, if anyone has solutions to either of these issues feel free to jump in.