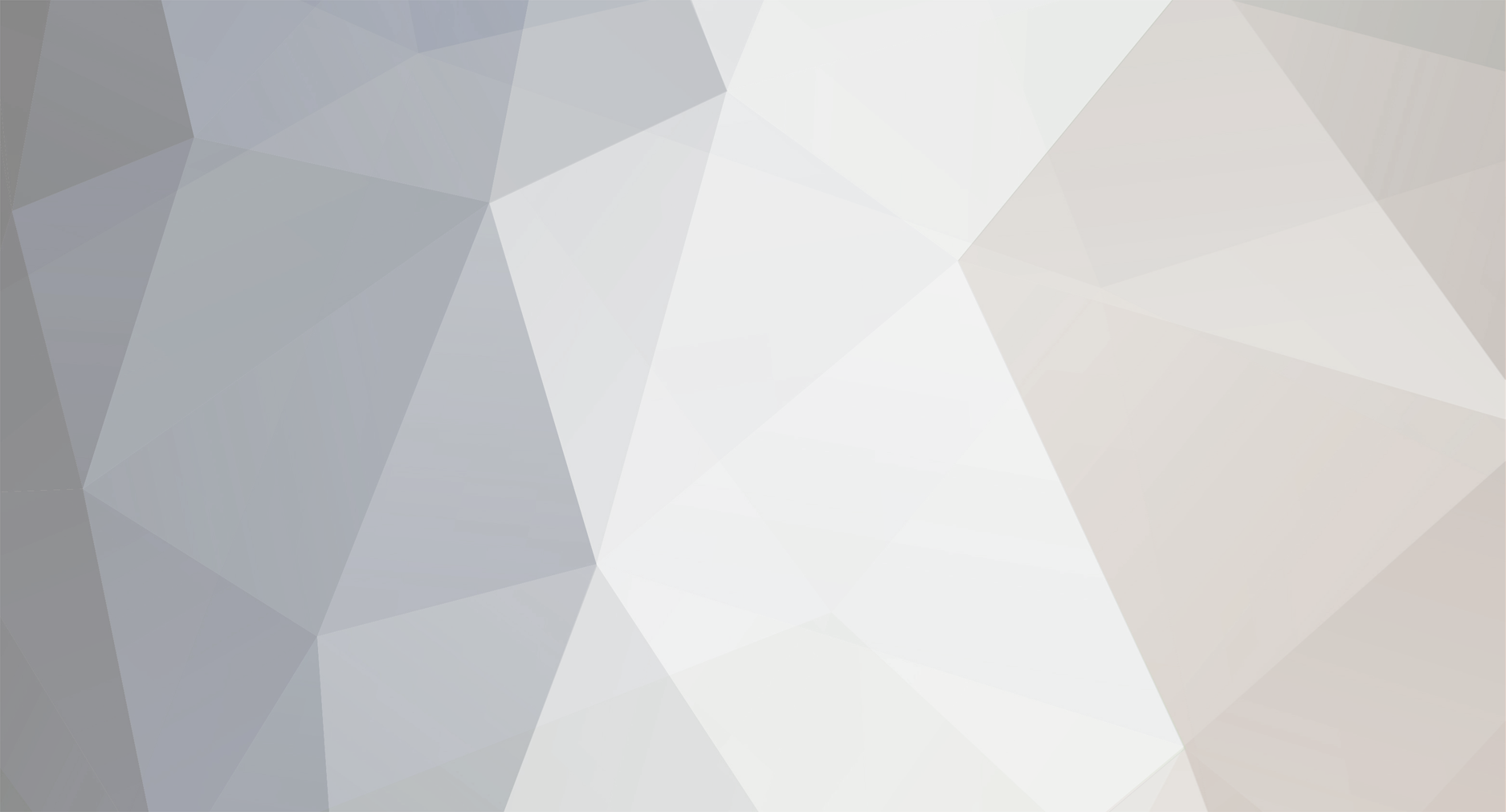
JasonLewis
-
Posts
3,347 -
Joined
-
Last visited
Posts posted by JasonLewis
-
-
I'm assuming you've loaded in the jQuery library or whichever framework you're using prior to calling the $.post() function?
-
Have you attempted to do this at all? I've never actually stored images in a database, unless it was the path to the location of the image, however you can fetch the data from the database and set the content-type header to image/jpeg or whatever the image is and output the image.
The best way to get it to appear in a lightbox would be to have an HTML img tag link to a PHP script with perhaps the ID of the image:
<img src="/path/to/image.php?id=1" alt="My Image!" />
Then in image.php:
<?php $id = $_GET['id']; $data = mysql_fetch_assoc(mysql_query(...)); // run query to fetch data, probably better to ensure your query executes properly first etc header('Content-type: image/jpeg'); echo $data['image']; ?>
Very basic, but you get the idea.
Good luck.
-
In reference to the "pretty URLs" part and not encoding your URLs, typically a hyphen is favored over an underscore. But that doesn't mean underscores aren't used. You check any Wiki and 99% of the time (if using MediaWiki) the page titles use underscores for separation. It's not common in most websites but I've seen it around. Personally I favor the hyphens, but you can use what you want.
-
No worries, just a question. Was it because of whitespace?
-
Ensure there is no whitespace before and after the opening/closing PHP tags, also try using image/jpeg as your content type. See what happens there... I've never stored images in a database before so I wish you luck.
-
It looks like you're getting muddled up. In your query you specify: SELECT img FROM pic
By that logic, the blob column is called img
So this line:
$image = $image['$image'];
Makes absolutely no sense because 1) you're referencing the $image variable inside single quotes which is making it into a string and 2) your column is titled img not $image
Change that line to:
$image = $image['img'];
-
You can't use the word 'desc' in your queries, unless you backtick it. 'desc' is a reserved word for MySQL, so you can order things.
SELECT * FROM table ORDER BY id DESC
I'd suggest choosing a different column name or surrounding it with backticks:
WHERE `Desc`='" . $id . "'
-
"It's not working" is not a good way to describe a problem. Some more details would be nice. Are you getting a corrupt image error or something?
-
Every time I hear the words "light years" I think of this Big Bang quote:
Penny: I'm guessing he's not looking for a fling. He's the kind of guy who gets into a relationship for, I don't know, like you would say lightyears.Sheldon: I would not say that. No one would say that. A lightyear is a unit of distance, not time. You see, people hear the word 'year' and they think of duration. Footpound has the same problem, that's a unit of work not of weight... It's common mistake.
I wish I could work at Google, only because their offices look simply amazing.
-
You want to split it by the space first, so that it's an array? I'm a bit confused by your question.
With the indexOf() method you can supply a second parameter as a start point, however the issue will come up when indexOf() actually finds nested words. So in your example it would find the "green" at the start of "greenish". The other way you can do it is by using regexp in JS, with match(). Of course match will only find the occurring strings and not actually return the location of them.
What exactly do you need this for, might be easier to provide a better and more suited solution.
-
Mad! Never knew they had competitions like this... Then again they have competitions for everything! Awesome stuff, good luck to you in the future!
-
I don't understand your reasoning. But if you're using a lot of JavaScript, use jQuery as it'll alleviate a lot of cross browser issues. Should be simple enough in jQuery.
$(document).ready(function(){ $('input[name=choice]').click(function(){ $('input[name=choice]').removeAttr('checked'); // uncheck all the checkboxes $(this).attr('checked', 'checked'); // check the clicked checkbox }); });
Something like that should suffice.
-
Why not just use radios instead of checkboxes, since the point of checkboxes is to allow multiple selections. Radios are used for a single selection item.
-
Have you used the developers tools for IE to see if the styling is being applied or not? Tried removing the number, although that shouldn't be a problem.
Otherwise wait for cssfreakie.
-
... separates us from the Clans that you younger gamers like to create.
You seem to be implying that everyone here is young.
I think "the" would've been a better choice of word.
Although I am a young, somewhat hardcore gamer I don't get into the whole clan business either. Gaming is primarily fun, but I love the competitive nature, as do most people that play games (mostly online).
Now, back on topic.
I really like the design of your website, however the navigation items when not active or hovered are slightly hard to read for me. The navigation items looks amazing, then the dropdown just looks boring. Why not make it slightly bigger and the same background color as the parent item so that it stands out a bit more.
I think the default text of "username" and "password" should be in the login boxes. Sure it's fairly obvious, but having that in there then onfocus removing it, and onblur if the contents is blank then adding it back in makes things a whole lot nicer for the end user.
The user icon that appears next to users in the online list is really quite repetitive. Every user has it. It looks ridiculous. I think doing something similar to Facebook would better suit this spot. You know how when you hover the link that says something like "2 people like this" and it shows the people that like it, something like that. A tooltip. Not to hard to implement but ensure that you're able to then move your mouse down the list and click a person if you need to. You could even make it a double column list. That would remove the ugly scroll bars.
The second search box further down the website seems a bit odd and ugly to me. Is it even really required to be there since you have a search at the top?
That's about all from me. It's good to see another SMF powered website.
Good luck with the future of the website.
-
It's also for webkit only browsers, Firefox is just shows icons. As for the mobile device, have you tested on any other devices? Different OS? Different browser for that phone perhaps?
-
When you say "above" do you mean it is literally above or there is a slight offset? I'm seeing a slight offset.
You might like to try the vertical-align property, setting it to middle might do the trick for you.
<input type="text" name="input" style="vertical-align: middle" />
Or use an external stylesheet and give the inputs a class name.
-
-
Does it matter that much that for a second or so the image may jitter when loading? This is pretty common in most web apps. I don't see the big deal, as next time the loading image appears it should appear straight away.
-
Here is a better answer then what I could ever come up with: http://stackoverflow.com/questions/3332074/what-are-the-disadvantages-of-using-persistent-connection-in-pdo
-
You'll need to run yourself through a few basic tutorials on selecting, updating and inserting records first. Familiarize yourself with how this is done and you'll have more of an idea on how to go about your task. It's hard to provide a straight out guide without writing a specific guide tailored to your needs which is essentially writing the code for you.
You may like to try here: http://www.codingforums.com/showthread.php?t=46916
Although that tutorial is a fair few years old and is quite out-dated but gives you the idea.
http://www.visualdesigncore.com/tutorials/PHP-MySQL/PHP-News-System/
Another older tutorial, again good for checking out some ideas.
-
Well, the purpose of binding variables by reference is so you don't need to run the entire query again to set the variables again. You can simply prepare your query, bind the parameters and set them, then execute the query, update the variables and execute the query again.
$sth = $db->prepare("SELECT foo FROM bar WHERE id=:id"); $sth->bindParam(':id', $id, PDO::PARAM_INT); $id = 2; $results[] = $sth->execute(); $id = 4; $results[] = $sth->execute();
Of course it's more commonly used when inserting or updating data, not selecting. But you get the idea. But again, I'm a bit rusty with PDO. Hopefully this is somewhat helpful though.
-
Like this?
fwrite($fp,"<div id=\"comments_box\"><div id=\"comment_name\"><p>$message <em>Says:</em></div><div id=\"comment_date\">Date: " . date("l, F d, Y h:i") . "</div><br />$message2</p></div>");
-
Not that I know of. It's usually up to the developer to ensure that code is cross browser compliant, or at least degrades gracefully in older browsers. The less hacks you use, the better. I personally avoid using hacks all together. If I have the option of making something work with a hack, I'll avoid it and just let it degrade gracefully. It might not look as flash as it should, but at least everything is valid and hack free.
BTW the HTML/CSS section would've been a more appropriate spot for this.
Whats my alternative to using a dropdown menu...
in HTML Help
Posted
Why do you not want to use a dropdown? I prefer dropdowns, it's easy to generate an array of days, months and years with PHP and loop over them to create the dropdowns. You can even add in some JavaScript to alter the number of days on specific months if you want to get technical, otherwise do the validation server side when they submit.
The easiest way for the coder though would be input boxes, although validation will still be required.