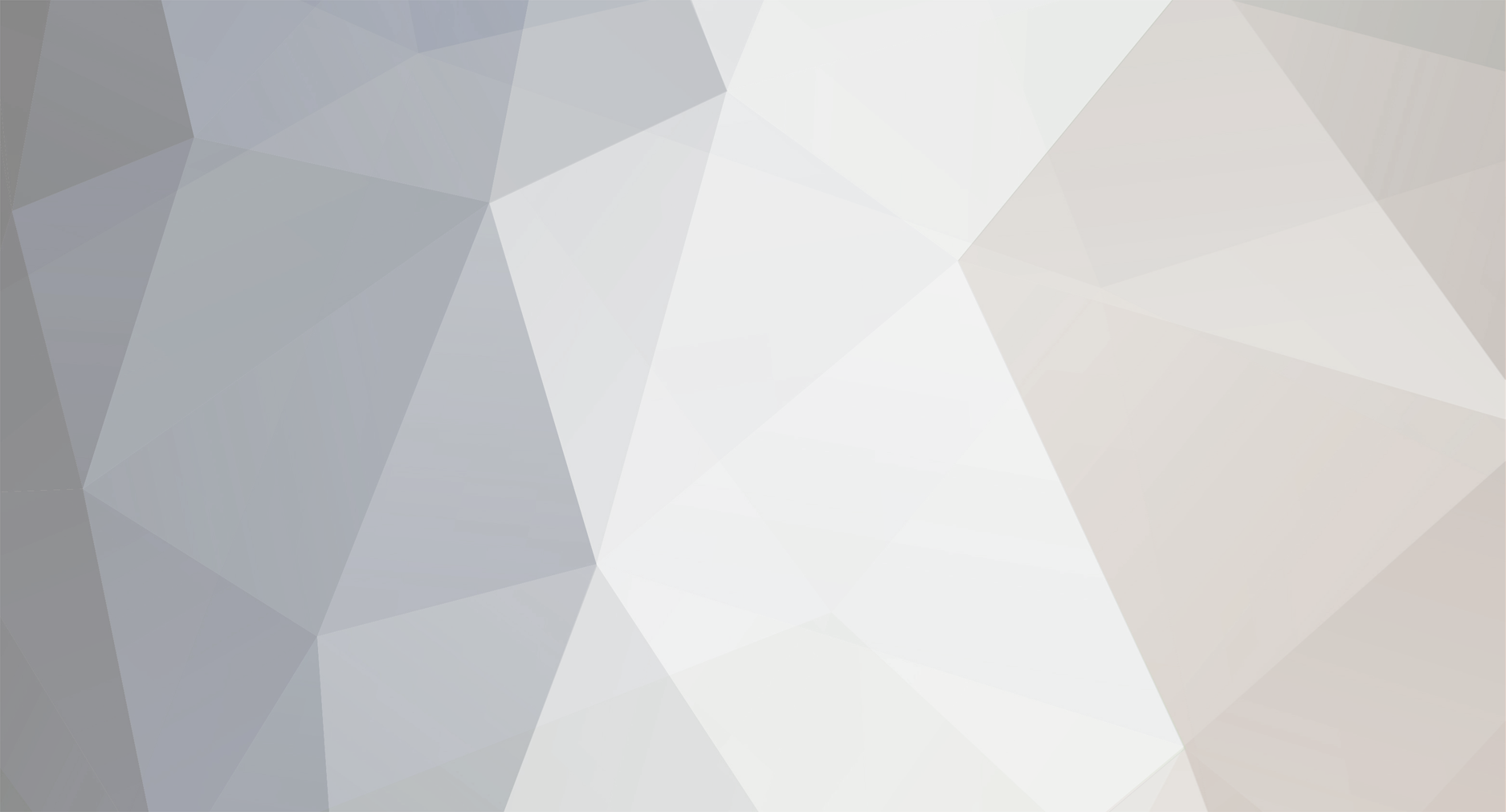
JasonLewis
-
Posts
3,347 -
Joined
-
Last visited
Posts posted by JasonLewis
-
-
Depending on how you do it, you could for instance have the function bound to the click event of the button. For example:
$('#button').click(function(){ $('#billing_address_setting').attr('href', $('#billing_address_setting').attr('href') + 'site_id' + data); // Now unbind the click event. $(this).unbind('click'); });
Or you could simply check to see if the URL already has ?a=100 at the end of it by using .indexOf()
if($('#billing_address_setting').attr('href').indexOf(data) == -1){
$('#billing_address_setting').attr('href', $('#billing_address_setting').attr('href') + 'site_id' + data);
}[/code]
-
I ran this on my local machine and it worked flawlessly.
Can you post your entire code? Something may be buggering it up...
-
If you're getting that, have you made sure that this link you are trying to append to has the correct ID.
<a href="http://www.somelinkhere.com/" id="billing_address_setting">Link text</a>
-
I did some reading on using overflow to clear floats just then, and it seems its still not foolproof but it's pretty bloody good. Apparently there are some instances with more complex layouts where it may break, but really. It's a wonderful idea, can't believe I'd never heard of that before.
As long as a width is defined for some browsers it's flawless.
-
Cool beans on the overflow: hidden cssfreakie.
-
Unfortunately I don't believe you can append that easily to a URL. Well, it's still easy but not like that.
$('#billing_address_setting').attr('href', $('#billing_address_setting').attr('href') + 'site_id' + data);
What is 'q'? You're using jQuery correct?
-
Just quickly looking at your site, you should probably reset your floats after those lists. Inside the wrapper div, however at the bottom right before the closing </div> put in another div like:
<div class="clear"></div>
Then create a CSS rule like this:
.clear { clear: both; }
It should reset the floats allowing the wrapper div to stretch to the full height of its children. This will then eliminate the need to supply a background-color to any of those child elements.
-
You're going to have to do it how PFMaBiSmAd has mentioned.
Set a single variable $active to 'active_1_20', or whatever the variable at the time will be. Then you can use the ternary operator to determine if the bar is active or not.
Unless you run the all the divs in the loop too, which will make it a lot easier. Something like:
for($i = 1; $i <= 501; $i += 20){ $active = ''; // for now, we'll set the active variable to nothing. if($i == $start){ $active = 'active'; // set the active variable to active } $from = $i - 1; $to = $i + 19; // Now you can construct each DIV echo '<div id="from_' . $from . '" class="rPosButton '' . $active . '" style="height: 185px;" onclick="getNextParticipants(this);this.blur();" onmouseover="Tip( get_value(\'' . $i . ' - ' . $to . '\'), TEXTALIGN, \'justify\', ABOVE,\'true\', TEXTALIGN, \'justify\', PADDING,10, TEXTALIGN, \'justify\', OPACITY,90, TEXTALIGN, \'justify\', FIX,[300,450], TEXTALIGN, \'justify\', DELAY,1, TEXTALIGN, \'justify\' );" onmouseout="UnTip();"> </div>'; }
Now you'll have to change your JS function getNextParticipants a bit, you can simply add in the from and to variables as parameters to that function. Otherwise you're going to have a crap load of JS functions. But I hope you get the idea...
-
Oh, how about setting $i to 0 again inside the if statement at the bottom.
$i = 0;
Is that what you mean?
-
Well, you don't actually increase the $loop_length in your if statement, so the loop will just end again. You'd need to reset the $loop_length, but also take note that this will make the loop go on for ever, well for a while anyway. So make sure you add in something to stop the loop at some stage. A simple boolean check somewhere to break out of the loop or something to that effect.
-
Where does $a come from first? You use it in your while() loop..
-
print_r($_SESSION) to see what sessions you have set.
-
I'd just like to point out that when your keys are strings, surround them with quotes to ensure PHP doesn't assume them to be constants.
echo $array[mother]; // this is bad, php first looks to see if a constant called mother is defined. echo $array['mother']; // this is good, always surround key with quotes. but only if it's an associative array, that is, the keys are named.
Now. What is the end result of these variables? If you are going to do something with them inside a loop, then something like this may suffice.
foreach($filters as $key => $value){ echo $key; // will echo 93 echo $value[0]; // will echo 96 }
Of course, if you have an array that is deeper you'll need to use a recursive function.
Hope this helps a bit.
-
I'm wondering the same as socratesone as to how you're using the $active_xx_xx variables. I offered a solution using arrays as well, but this isn't really an area you could use a loop. This is without using a loop:
if(!isset($_GET['start'])){ $start = '1'; }else{ $start = $_GET['start']; } $active = 'active_' . $start . '_' . ($start + 19); // Use variable variables to construct the new variable. // If you really need it done this way that's fine, but I suggest an array. $$active = 'active'; // Using an array. $active = array( $start . '_' . ($start + 19), 'active' ); // Limit $limit_by = 'LIMIT ' . ($start - 1) . ',' . ($start + 19); // Rank $rank = $start;
However if you are using a loop to ensure that no other values are set apart from the predefined ones, you could go for something like this:
if(!isset($_GET['start'])){ $start = '1'; }else{ $start = $_GET['start']; } for($i = 1; $i <= 501; $i += 20){ if($i == $start){ $active = 'active_' . $i . '_' . ($i + 19); $$active = 'active'; $rank = $i; $limit_by = 'LIMIT ' . ($i - 1) . ',' . ($i + 19); break; // finish the loop } }
-
if(isset($_POST['uloguj_se'])) <- wont work, its just the button's name.
Using the button name there is fine, upon pressing the submit button it sets the buttons name as a key and its value as a value in the $_POST array, thus using this allows you to determine if your form has been submitted.
hellboy012, you can change your code to how phppaper suggested, with the single query. However checking a variable against a mysql_query() returned value will achieve nothing. mysql_query() returns a MySQL resource, which is totally useless for what you want. You'll need to take it a bit further and determine if there are any rows, with mysql_num_rows.
$query = mysql_query("SELECT * FROM klijenti WHERE nick='$nick' AND password='$pass'"); if(mysql_num_rows($query) == 0){ echo '<center>Netacan username/pw.</center>'; }else{ echo '<center>Poz! </center>'; }
Good luck.
-
If I understand you correctly, and assuming that you're not putting in any line breaks manually before calling nl2br(), you can simply replace all line breaks prior to calling nl2br() since highlight_string() adds in the line breaks.
$content = preg_replace("/\<\?php.+\?\>/isUe", "highlight_string('$0', true);", $content); $content = preg_replace('/(<br\s*\/*>)/is', '', $content); $content = nl2br($content);
See if that does the trick.
-
I was under the impression that the default for these column types was not allowed to be null.
However I may be wrong.
-
Just to provide a few useful links using the jQuery library in paticular.
http://docs.jquery.com/Tutorials:Mouse_Position
http://jquery-howto.blogspot.com/2009/07/identifying-locating-mouse-position-in.html
Then of course, assuming you wrap the image in a div, just simple append the new image using .append() on the containing div. Ensure your containing div has a set position of relative, then set the position of the new image to absolute and position it according to the coords you received from the above techniques. You may also need to adjust the z-index of the new image.
Remember, Google is your friend.
Good luck.
-
You can actually use Flash to retrieve information from external files, such as PHP. See this thread for more information on that.
-
Right... and this must be done for every column which has a latin1 collation too? Crap, that's a lot of work...
-
So I've recently started looking into Character Encoding, after never really bothering with it, and I've got a few questions. After checking out my site it seems I've been using UTF-8 without really realising it, and I'm going to stick with UTF-8. However, when I jump into phpMyAdmin to check out my MySQL tables I run into a few problems.
Originally, for my database, the collation was set to latin1_swedish_ci. I have since changed this to utf8_general_ci. This however only affects newly created tables, not the 150 or so tables I currently have which are still latin1_swedish_ci. Do I need to convert the collation of these already existing tables to UTF-8, and if so what's the best method?
This takes me to my text editor, NetBeans 7. Do I need to alter anything here, I've not been successful with finding information relating to this?
Cheers guys.
-
Have you debugged it, checked your inputs and outputs. Make sure the query is running successfully, like Pikachu2000 has said, and confirm it's working by running it in phpMyAdmin.
Personally I wouldn't check for an identical match (with ===), I'd just check if they are equal (with ==).
-
Sorry mate, you can't do that. Refer to this page: http://codex.wordpress.org/Function_Reference/get_category_by_slug
It only takes a single string, so one slug, not multiple.
So re-arrange a bit of your code, and try it like this:
$slugs = array('homepage','blog'); foreach($slugs as $slug){ $id = get_category_by_slug($slug)->term_id; // store the id of the slug echo $id; }
-
My understanding of it is that you only really use them when you may need to roll back changes you make within MySQL if something goes wrong. From my point of view it just doesn't look like you really need to use transactions here, but then again, I'm still delving into PDO myself.
That said, you could simply use rollBack() in your catch if something does go wrong.
function inside function firing unexpectedly
in Javascript Help
Posted
event.stopPropagation seems to be what you're after. In the clickableCell click event add this: