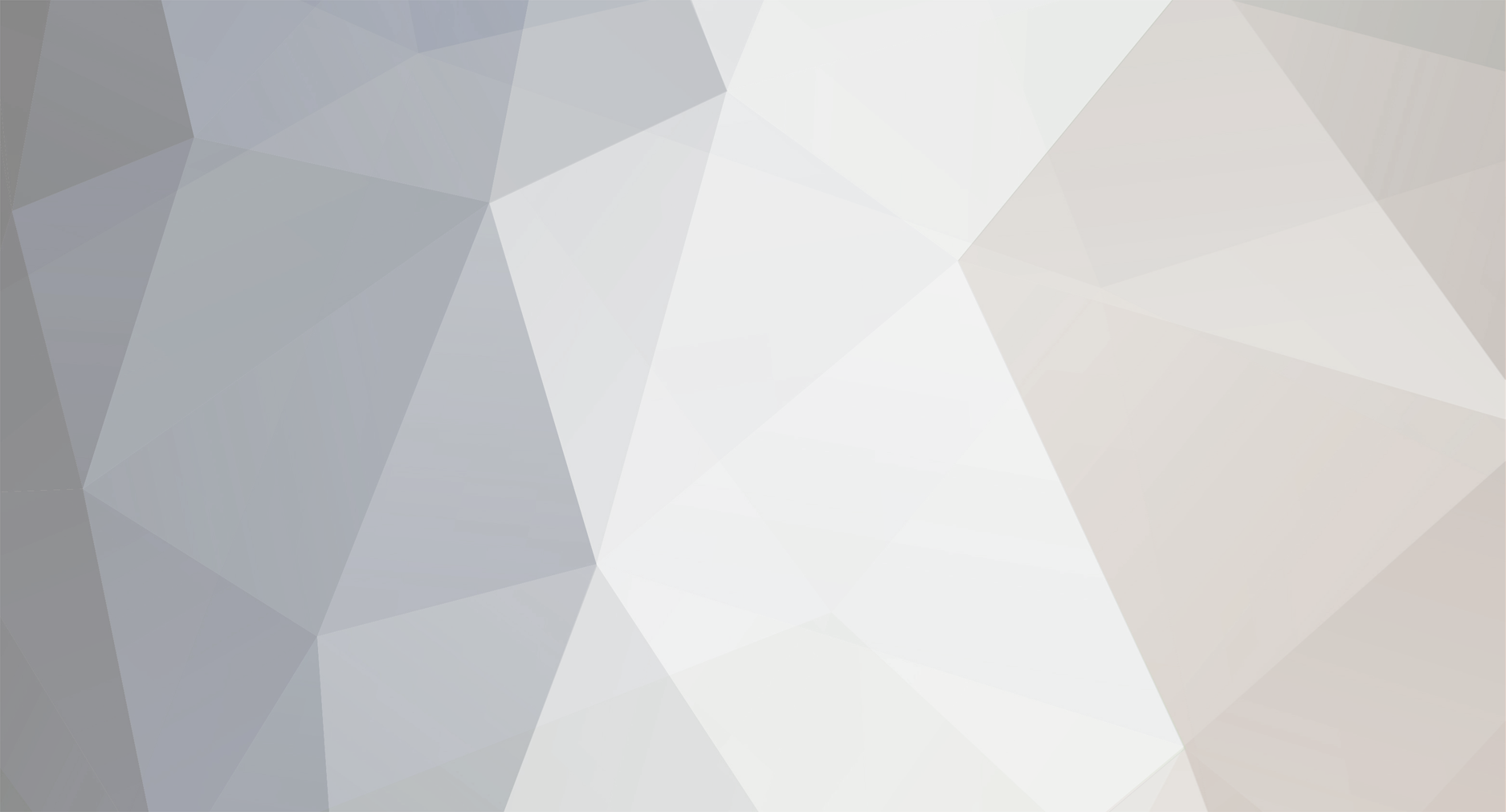
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
Yes. Let's say you had a `users` table and you wanted to sort by last name descending (z first, a last), first name ascending (a first, z last) and finally by age descending (100 before 1). ORDER BY last_name desc, first_name, age desc
-
insert/update functions for mysql, what do you think?
roopurt18 replied to Goat's topic in PHP Coding Help
You would want to see if there's a way to register a shared memory resource on your platform. I've never done such a thing with PHP, although I've certainly thought about it. Another thing you could do is create a PHP object to represent the table schemas and store it in the session. Then you will only need to query the schema once per user per session. You have to keep in mind though that MySQL is also optimized and will cache query results and serve result sets from its own internal cache. I would go with the static method since it's easiest to implement and just keep it as-is until you have a real need to optimize further with your own code and logic. -
ORDER BY is a key-phrase that MySQL sees and says, "Ah, I'm going to sort the records." MySQL then reads what comes next. In your case: id So MySQL says, "Ah! I'm going to order by the values in column `id`." This might be a profound thought to you, but you don't know what order the records are stored in the database. You might be inclined to think, "Well they're stored in the order I inserted them, silly!" You'd be wrong. You see, your table is represented by a file on disk. You might have 100 records in your table. All of the records combined might require 4kb of disk space. But if you were to look at the table's file on disk, it might be 2MB! That's a lot larger than 4kb. The reason is because MySQL assumes you will be storing lots of stuff in the table so it makes a file on disk larger than what it needs to actually store the data. As you insert more and more records, the actual space required by the records grows closer to the file's actual size on disk. When they approach close enough MySQL will make the file larger to accommodate many more records. Now each record has a starting and ending location within the file. When you delete a record, MySQL makes a note that the bytes in the file that correlate to that record are free to use for another record at a future time. More simply put each table has an associated disk file where the records are put. You have no idea where in that file a particular record might be. Therefore a SELECT statement without an ORDER BY will return the records in an arbitrary and unpredictable order. So even though your id is auto-incrementing. Depending on how you insert and delete records over time, they might be in the file in this order: 1, 2, 3, 127, 56, 78, 9, 10, 11, 12, 14, 37... So back to our ORDER BY. MySQL sees ORDER BY `id` and says, "Ah! I will take the records and sort them by `id`." That turns: 1, 2, 3, 127, 56, 78, 9, 10, 11, 12, 14, 37... into: 1, 2, 3, 9, 10, 11, 12, 14, 37, 56, 127 By default MySQL sorts in ASCending order. When MySQL sees the DESC it says, "Ah, I must reverse the sort order!" and the list becomes: 127, 56, 37, 14, 12, 11, 10, 9, 3, 2, 1 MySQL doesn't look at any timestamp that I know of when sorting values unless you were to specify a timestamp column in the order by: ORDER BY `my_column_that_has_a_timestamp_datatype`
-
Are you accessing the server with putty? If so you can just highlight the said lines; they will be automatically copied to the clipboard. At least that is the typical behavior. How did you install PHP on the server? Did you pre-select it as a package? Or did you run a command: apt-get php5 How did you install the PHP Postgres extension? Was it pre-installed? Or did you run a command: apt-get php5-pgsql If you are using apt-get or aptitude, the server might have created the following: /etc/php.ini # config file /etc/php.d # conf directory Once the extension is installed (with apt-get php5-pgsql or the equivalent), you want to make sure there is a file: /etc/php.d/pgsql.ini It should have the line that enables the extension: extension=php_pgsql.dso ; or whatever it's actually called Then from the command line you should be able to do the following: php -m | less Look that the pgsql extension is loaded. php -i | less Look at the configuration values for pgsql. Start and stop apache /etc/init.d/apache2 stop /etc/init.d/apache2 start Find your htdocs or webroot and create a file: <?php // i.php phpinfo(); ?> Load the file in your browser and check that PHP running under Apache has pgsql enabled. Lastly, I'll throw a little tidbit your way. If using PDO / Postgres there seems to be a bug when connecting to the database that causes seg faults. I use the following to get around them until a fix is available: <?php class LibPDO { /** * Creates a PDO object to be used with PostgreSQL and returns it. * * @param string $host * @param string $user * @param string $pass * @param string $dbname * @param [boolean] $throw_exceptions * @param [array] $addl_dns * @return PDO $pdo */ public static function CreateForPostgre( $host, $user, $pass, $dbname, $errors_as_exception = false, $addl_dns = null ) { if( strlen( $host ) === 0 ) { throw new Exception( "Host not provided." ); } if( strlen( $user ) === 0 ) { throw new Exception( "User not provided." ); } if( strlen( $pass ) === 0 ) { throw new Exception( "Password not provided." ); } if( strlen( $dbname ) === 0 ) { throw new Exception( "Database not provided." ); } $arg = "pgsql:host={$host} user={$user} password={$pass} dbname={$dbname}"; if( is_array( $addl_dns ) && count( $addl_dns ) ) { foreach( $addl_dns as $k => $v ) { $addl_dns[$k] = "{$k}={$v}"; } $arg .= " " . implode( ' ', $addl_dns ); } $driver_opts = array(); if( $errors_as_exception === true ) { $driver_opts[PDO::ATTR_ERRMODE] = PDO::ERRMODE_EXCEPTION; } if( empty( $driver_opts ) ) { return new PDO( $arg ); }else{ return new PDO( $arg, null, null, $driver_opts ); } } } ?> And I call it like so: <?php LibPDO::CreateForPostgre( $host, $user, $password, $database, true, /* causes pdo to throw exceptions */ array( 'sslmode' => 'disable' ) /* required to get around segfaults */ ); ?>
-
$example_single_quoted_string = 'hello there, my id is $id. cool, huh?'; // this does not work // so we end the string with a single quote, use concatenation with our variable, and then start the string again $example_single_quoted_string = 'hello there, my id is ' . $id . '. cool, huh?';
-
http://dev.mysql.com/doc/refman/5.0/en/sorting-rows.html
-
You're trying to use a variable inside of a single-quoted string, which won't work. The fact that the single-quoted string is a value in an array is irrelevant. You'll want to use string concatenation at the point where you want to use the variable if you want to stick with a single-quoted string.
-
insert/update functions for mysql, what do you think?
roopurt18 replied to Goat's topic in PHP Coding Help
When a variable in a function is declared static it will keep it's value between function calls. So on the first function call the variable is an empty array and it might retrieve info for table users. The second function call might be for table news so it retrieves info for news. The third function call might be for users again and since the variable is static it already has the table info. Essentially it ensures that your query to information_schema for the column info only occurs once per table per script execution. -
insert/update functions for mysql, what do you think?
roopurt18 replied to Goat's topic in PHP Coding Help
You might run into some issues if you want to insert a NULL or the current MySQL timestamp using NOW(). I'd make a suggestion though on improved type checking. Use a static array to keep track of the table schemas so you can cast to the proper types. $table = 'users'; $values = array( 'username' => 'larry', 'password' => 'asdlfjawfiwaoeijfalfjw2oifjo', 'active' => true ); function db_insert( $table, $values ) { if( ! is_string( $table ) ) throw new Exception( '$table is not a string: ' . var_export( $table, true ) ); if( ! strlen( $table ) ) throw new Exception( '$table can not be empty: ' . var_export( $table, true ) ); if( ! preg_match( '@[a-z][a-z0-9_]+@i', $table ) ) throw new Exception( '$table is improper format: ' . var_export( $table, true ) ); if( ! is_array( $values ) ) throw new Exception( '$values is not an array: ' . var_export( $values, true ) ); if( ! count( $values ) ) throw new Exception( '$values is empty: ' . var_export( $values, true ) ); $regexp_column = '[a-z][a-z0-9_]+'; $col_string = implode( ',', array_keys( $values ) ); if( ! preg_match( "@{$regexp_column}(,{$regexp_column})*@i", $col_string ) ) throw new Exception( 'column name in $values violates format: ' . var_export( array_keys( $values, true ) ) ); static $table_info = array(); if( ! array_key_exists( $table, $table_info ) ) { // we have not queried information_schema for the column info on this table // run a query such as: select * from information_schema.columns where table_name = $table // to get each columns type and length // if you fail, throw an exception } $cols = array(); $vals = array(); $info = $table_info[$table]; foreach( $values as $col => $val ) { // determine the columns type if( $info[$col]['type'] === 'tinyint' ) { if( $val != 0 && $val != 1 ) throw new Exception( "Attempting to set column {$col} to improper value: " . var_export( $val, true ) ); } // you could perform all sorts of various checks here for all sorts of data types / combinations $cols[] = "`{$col}`"; $vals[] = $val; // strings will already be enclosed in single quotes } $sql = "insert into `{$table}` ( " . implode( ', ', $cols ) . " ) values ( " . implode( ', ', $vals ) . " )"; // run sql } Just some thoughts. -
I managed to pick up on that. <?php $sort_status = array(); // want to init these values $sort_id = array(); // init $orders = array(); // init foreach($database_array as $x){ $db = "F:\location\\$x.mdb"; $conn = new COM('ADODB.Connection') or exit('Cannot start ADO.'); $conn->Open("Provider=Microsoft.Jet.OLEDB.4.0;Data Source=$db"); $sql = "SELECT * FROM MyTable ORDER By Status asc, ID desc"; $rs = $conn->Execute($sql); while(!$rs->Eof){ #bunch of results put into variables# $sort_status[] = $status; // the $sort_ARRAYS are for array_multisort $sort_id[] = $id; $orders[] = ( "$status||$id||$shop||$name||$orderdate" ); $rs->MoveNext(); } $rs->Close(); } array_multisort( $sort_status, SORT_ASC, $sort_id, SORT_DESC, $orders ); print_r( $orders ); ?> If you're later going to be breaking the strings apart into arrays, then you may want to assign to $orders like so: $orders[] = array( 'status' => $status, 'id' => $id, ... ); (edit 1) Looping over all the records to build the array, then sorting the array, and then ultimately looping over it again is computationally expensive. There might also be a way where you can create a joint dataset that allows you to select from all of the databases / tables at once and actually use the database to perform the sort. (edit 2) However if the record set is small OR this script is not run often OR you just are not generally concerned with the performance, then array_multisort() is fine.
-
I'm guessing his values are numerically indexed and that they are strings in the format he has provided. I'm also guessing there is a way he can do this while retrieving the values or he can retrieve the values in a modified manner that will make this sorting task easier. If he is unable to elaborate on his methods and formats, then there's only so much we can do to help.
-
Object doesn't support this property or method
roopurt18 replied to dlebowski's topic in Javascript Help
-
Weird problem with SELECT command..Help!
roopurt18 replied to patheticsam's topic in PHP Coding Help
$info = mysql_fetch_array( $data ); while($info = mysql_fetch_array( $data )) Each time you call mysql_fetch_array() you retrieve a row from the results. Notice how you have a call to mysql_fetch*() before the loop and you do nothing with info? Yah. That means you're throwing the first record away into the black void that is your computer's memory. -
I'd like to see the code, if possible, for:
-
Object doesn't support this property or method
roopurt18 replied to dlebowski's topic in Javascript Help
First off, do you understand the error message? If not, the error message means you have a reference to an object, call it O, and you are using a property P or method M that the object does not have. In other words, you are attempting: alert( O.P ); // use property P of object O, if P is not there you will receive this error alert( O.M() ); // call method M of object O, if M is not there you will receive this error You have a few places in your code where this might be occurring. document.getElementById() is pretty standard, so that's probably not it. I'm not familiar with setProperty() on DOM methods though. Are you sure the object returned by document.getElementById('yourmessage') has a set property method? Next you have an object called raw. Are you sure that raw has a data property? Are you sure that raw.data is an object? Are you sure that raw.data.yourmessage is a property? Can raw.data.yourmessage be converted to a string? -
Show me how you select from the databases please.
-
You need to make sure your installation of Apache, PHP, and MySQL are all at least the same version or higher as the server you are migrating off of. You also need to make sure Apache and PHP have enabled any modules that your software requires. For example if your PHP script uses mcrypt you'll need to enable it on the new server. You'll also want to check that certain ini directives are correctly configured. If I had seen this thread sooner my recommendation would have been to install VMWare Server, which is a free virtualization software. Once installed you could have created a guest machine running either Ubuntu or CentOS. Then I would have recommended fully configuring and installing your virtual server, performing such tasks as installing Apache, PHP, MySQL, configuring the firewall, testing each of your sites on it, creating a back-up routine, setting up e-mail, etc. Depending on how diligently you work on it, the entire process could take two to four weeks. But once finished you would be more comfortable with ordering and administering a server of your own. I know that they're helpful to novices, but software like WHM and plesk drives me nuts. They install shit you don't need and trying to find the proper check box for a setting can be a royal pain in the ass. (edit) Seeing as you've already ordered the server, I'd stay the course and get your stuff working on your new hosting. Then see how happy you are with how well things run (or don't run).
-
Is there a variable limit when performing many php calculations?
roopurt18 replied to fmesco's topic in PHP Coding Help
You could use variable variables. Or you can use arrays. Whatever you pick will cut down on the final number of lines of PHP you have to write, but you'll still probably have to maintain the list of variables being filtered. <?php // VARIABLE VARIABLES APPROACH $search = array( '$', ',' ); // Add any other possible punctuation $replace = ''; $tmp_other_servicefee = $node->field_other_servicefee[0]['value']; // values like this go into temp variables // just add any variables to filter to this array $vars = array( 'calc_template', 'calc_install', 'calc_tearout', 'calc_rasiect', 'calc_surcharge', 'tmp_other_servicefee' ); foreach( $vars as $var ) { if( is_string( ${$var} ) ) { ${$var} = str_replace( $search, $replace, ${$var} ); } } // note that since you are performing addition only, you can compute $svc_total // during the loop as i've done with the "ARRAY APPROACH" below // if you are doing mixed operations, i.e. division, addition, multiplication, etc // then you are best off performing the calculation in steps that clearly outline // the order of operations. $svc_total= $calc_template + $calc_install + $calc_tearout + $calc_rasiect + $calc_surcharge + $tmp_other_servicefee; $svc_total= number_format($svc_total, 2, '.', ','); print check_plain ($svc_total, 2); ?> <?php // ARRAY APPROACH // This approach will lend itself best when the operator is the same for all of them // (in your case: addition) $search = array( '$', ',' ); // Add any other possible punctuation $replace = ''; $values = array(); $values[] = $calc_template; $values[] = $calc_install; $values[] = $calc_tearout; $values[] = $calc_rasiect; $values[] = $calc_surcharge; $values[] = $node->field_other_servicefee[0]['value']; $svc_total = 0; foreach( $values as $key => $val ) { if( is_string( $val ) ) { $val = str_replace( $search, $replace, $val ); } $svc_total += $val; } $svc_total= number_format($svc_total, 2, '.', ','); print check_plain ($svc_total, 2); ?> -
This is not the proper place for this post. Anyways, the answer to your question is here: http://www.phpfreaks.com/forums/index.php/topic,37442.0.html
-
Is there a variable limit when performing many php calculations?
roopurt18 replied to fmesco's topic in PHP Coding Help
The problem is that your values are strings, which means they are casted to numeric types during the addition. In the case of "1,770.00", when you cast this to a float you get a one. 885 + 1 + 150 + 100 + 75 + 50 = 1261 Any punctuation in your values is going to throw off the automatic type-cast from string to float. Therefore you might consider the following: <?php $search = array( '$', ',' ); // Add any other possible punctuation $replace = ''; $calc_template = str_replace( $search, $replace, $calc_template ); $calc_install = str_replace( $search, $replace, $calc_install ); // repeat for each variable... $svc_total= $calc_template + $calc_install + $calc_tearout + $calc_rasiect + $calc_surcharge + $node->field_other_servicefee[0]['value']; $svc_total= number_format($svc_total, 2, '.', ','); print check_plain ($svc_total, 2); ?> -
Question/Help: Securely sending data to database
roopurt18 replied to shinichi_nguyen's topic in PHP Coding Help
Storing credit card numbers is a BIG deal. First you need to be PCI-compliant, which somebody mentioned already. PCI-compliant means you follow a set of best practices (or maybe even laws) in regards to how you store and serve this type of information. You need to find the official site and documentation on requirements, which is a lengthy read if I recall correctly. I don't recall if any of this is in the PCI compliance, but you'll also want to do all of the following: + Dedicated server with backups you control + HTTPS for web traffic + SSL connection to your database + Code encryption on your PHP code + A sys admin that can properly lock the box down In addition to all of the standard web attacks you might fall victim to, you also have to consider someone walking off with a backup or the machine itself. I'm not really sure what safeguards you can use to prevent someone walking off with a backup and running your code in a debugger. You also have to be really careful about where you store your source code for such applications. If any of the above is iffy or scary sounding to you, then you should be turning down such projects. -
If ClassA is a singleton with a private __construct(), then you can't create a factory ClassB to instantiate the singleton, unless PHP supports friend classes and I've somehow missed them. I'm still curious about the maintenance drawbacks you alluded to from your research. Do you have any links?
-
Is there a variable limit when performing many php calculations?
roopurt18 replied to fmesco's topic in PHP Coding Help
Try dumping each value and seeing if any are bad: <?php var_dump( $calc_template, $calc_install, $calc_tearout, $calc_rasiect, $calc_surcharge, $node->field_other_servicefee[0]['value'] ); $svc_total= $calc_template + $calc_install + $calc_tearout + $calc_rasiect + $calc_surcharge + $node->field_other_servicefee[0]['value']; var_dump( $svc_total ); $svc_total= number_format($svc_total, 2, '.', ','); var_dump( $svc_total ); print check_plain ($svc_total, 2); -
Eh. I guess one downside is that the classes are not true singletons and you leave your application with the ability to create multiple instances of them. However that can be an advantage as well. Most of the time database classes are implemented as singletons but I have applications that require multiple database objects to exist, due to dealing with multiple databases. I don't really see any maintenance problems with your approach. I'd be curious about what you read that hints at maintenance problems with such an approach. In the long run it's almost the same code you'd write if they were true singletons.