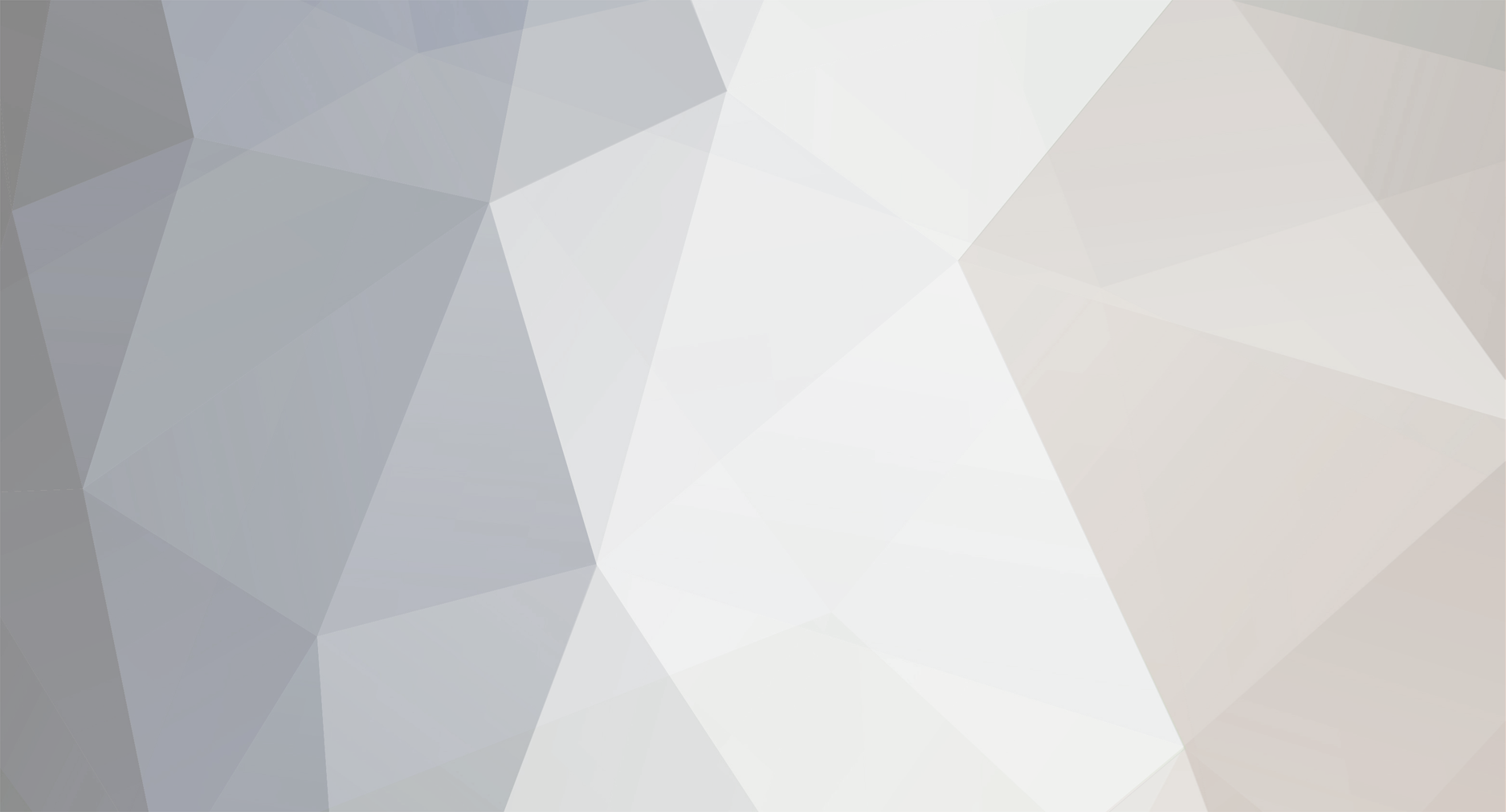
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
Use PDO. It's better anyways. Or just wrap it in your own alias as daniel suggested. No reason you can't name it mres or sql_escape or z if you want to be lazy and non-descriptive.
-
As Ron White would say, "You can't cure stupid."
-
<?php ini_set( 'output_buffering', false ); ?>
-
You can try using an optional character class: RewriteCond %{HTTPS} off RewriteCond %{REQUEST_URI} securepage[23]?\.php RewriteRule securepage([23]?)\.php https://sharedssl.com/mydomain.com/user/securepage$1.php That should mean "securepage; followed by an optional 2 or 3; followed by .php"
-
RewriteCond %{HTTPS} off RewriteCond %{REQUEST_URI} securepage\.php RewriteRule .* https://sharedssl.com/mydomain.com/user/securepage.php
-
PHP runs wherever you tell it to. You'd want to read the Apache documentation to see how to configure it to run from elsewhere or how to set up each virtual host to come from a different document_root.
-
Oh I see now.
-
And then how will you know you've reached the expiration date?
-
Does that mean this is solved? If so please mark it.
-
The salary of a web developer is irrelevant. Why not instead do a PowerPoint presentation on how the central banks have taken over all the major governments and converted our entire system of obtaining goods into a debt-based system. Then you can go on to show that no matter which career path you choose, the path is always ladened with debt-based institutions you must pass through. The final slide could then say, "Yah, you're !@#$ed." On topic, depending on locale, experience, and some other factors, you'll see numbers between $40k and $80k on average for web development; that's in U.S. dollars.
-
So I'm waiting for a server maintenance to finish and stumbled across this thread and then this gem: http://mingle2.com/dating/phases I have tears in my eyes.
-
Which Windows machine are you worried about in this case? Yours or the school's computer?
-
While you're at it you should purchase more books on language and grammar of your native language. One of the signs of a good programmer is solid communication skills. More and more frequently people (or maybe kids) visiting tech forums are speaking some language I call jibberish. I have nightmares that my great, great grandchildren will live in a world where the most common sounds are: "beep blip beep blop bloop," but it will be humans speaking rather than robots and androids. By the way this wasn't targeted at the OP.
-
We use nu-coder and phpexpress where I develop. Why? Because a year and a half a go the only PHP developer that worked here tried Zend and ZendGuard and didn't like it.
-
I'm no guru in either linux or apache, but my understanding of bash is that when you run a bash script it creates a sub-shell that inherits environment settings of the current shell. My initial guess would be the environment when the script is run by apache is not the same as that when run from bash. I would assume things like stdin, stdout, and pipes would work the same, but like I said I'm no guru. As a step by step process to figure out what is happening, I would recreate the shell script as a new file with commands up to the point that it stops working. In other words, copy the script and remove all the commands that make it go nuts. Then try invoking those commands from the PHP script (accessed by apache). I guess what you really want to know is, Can cinelerra be invoked by PHP under Apache without problems just by itself?
-
Where you write the file I only see $ID; I don't see $ID2. I applaud you for attempting error-checking in your file handing code, but you've gone a bit overkill with the is_readable() and is_writable() IMO. In addition your code is calling fclose() only if it couldn't write to the file. You need to call fclose() when the file handle is valid and you are done with it. A typical file writing block might look like: <?php $fp = fopen( 'the_file.txt', 'a' ); // or use mode 'w' if( $fp ) { fwrite( $fp, 'line 1' ); fwrite( $fp, 'line 2' ); // or write in a loop for( $i = 0; $i < 5; $i += 1 ) { fwrite( $fp, 'loop: ' . $i ); } fclose( $fp ); unset( $fp ); // finally close and lose the file handle } ?> You'll notice I didn't do much error checking during the fwrite() calls. If you want to be more robust and check for errors but don't want a bunch of nested if...else, then you can use a nifty trick with do...while. You create a nifty loop like so: <?php $success = false; do { // some stuff $success = true; } while( false ); ?> The loop only executes one time, but it has the advantage of supporting the break statement. This means we can very easily drop out of the loop at any point in time. By setting $success to false at the beginning of the loop, if we drop out early, $success will still be false at the end of the loop. However if the code makes it to the very end of the loop (the line above the while( false )), then we assume all operations within the loop were successful so we set $success to true. So here's a better example: <?php $fp = fopen( 'the_file.txt', 'a' ); // or use mode 'w' if( $fp ) { $success = false; // Assume failure do { if( ! fwrite( $fp, 'line 1' ) ) break; // if one write fails, end the loop if( ! fwrite( $fp, 'line 2' ) ) break; // if one write fails, end the loop // or write in a loop $success = true; // Now we assume success for( $i = 0; $success && $i < 5; $i += 1 ) { if( ! fwrite( $fp, 'loop: ' . $i ) ) $success = false; } // Since we assumed success=true right before the loop, if success is // now false we know a write failed, so we end the loop again if( ! $success ) break; $success = true; // if the code made it this far in the do...while, // then we know all of the writes were successful } while( false ); if( $success ) echo "All file writes were made correctly "; else echo "Not all file writes were done correctly "; // finally we close the handle if( fclose( $fp ) ) echo "and the file handle was closed."; else echo "and there was a problem closing the file handle."; unset( $fp ); // it's a good idea to "lose" the file handle, an old C habit } ?> (edit) I almost forgot, you can also forgo the fopen(), fwrite(), fclose() nonsense and do it all with file_put_contents(). The availability of that function depends on your version of PHP and it may not support file appending, although you could do something like: <?php $file = '/some/file.txt'; $append = 'some content' . PHP_EOL; file_put_contents( $file, (is_file( $file ) ? file_get_contents( $file ) : '') . $append ); ?>
-
You say this site is old. Has it always done this or is this a new development? If it's a new development, has there been any upgrades or modifications in server software? I would perform searches on the following: REMOTE_ADDR REMOTE_ client_ip ip _SERVER HTTP_ If you're sure that $_SERVER is always populated correctly, then you could also attempt changing the offending line: $client_ip = $_SERVER['REMOTE_ADDR'];
-
Just specify the full or relative path to the file. <?php // assume this script is in: c:\myproj\myscrypt.php // we want to delete: c:\myproj\mydata\junkfile.dat unlink( 'mydata/junkfile.dat' ); // relative unlink( 'c:/myproj/mydata/junkfile.dat' ); // absolute // I prefer to provide absolute paths based on a path I'm certain about unlink( dirname( __FILE__ ) . '/mydata/junkfile.dat' ); ?>
-
How check if JS script exists in some website
roopurt18 replied to rellect's topic in PHP Coding Help
You can try to pull it down with wget, curl, file_get_contents, httprequest, etc. -
@fantomel, kickstart isn't trying to type PHP code above. He's saying that users are being kicked out of the application because it uses those PHP variables and those are examples of weird data being put into them. @kickstart, Sorry but I got nothing for you. (edit) I changed my mind. kickstart you might try doing a full text search on all of the source files for those patterns and seeing what comes up. It could be that phpBB, under some circumstances, is replacing the actual values with those values.
-
I don't use ZF, but does it implement some sort of a cache? Maybe you have to tell it to look for new actions or something.
-
I'm still confused what the joke is. /shrug
-
I understand English may not be your first language, but what the !@#$ are you talking about?