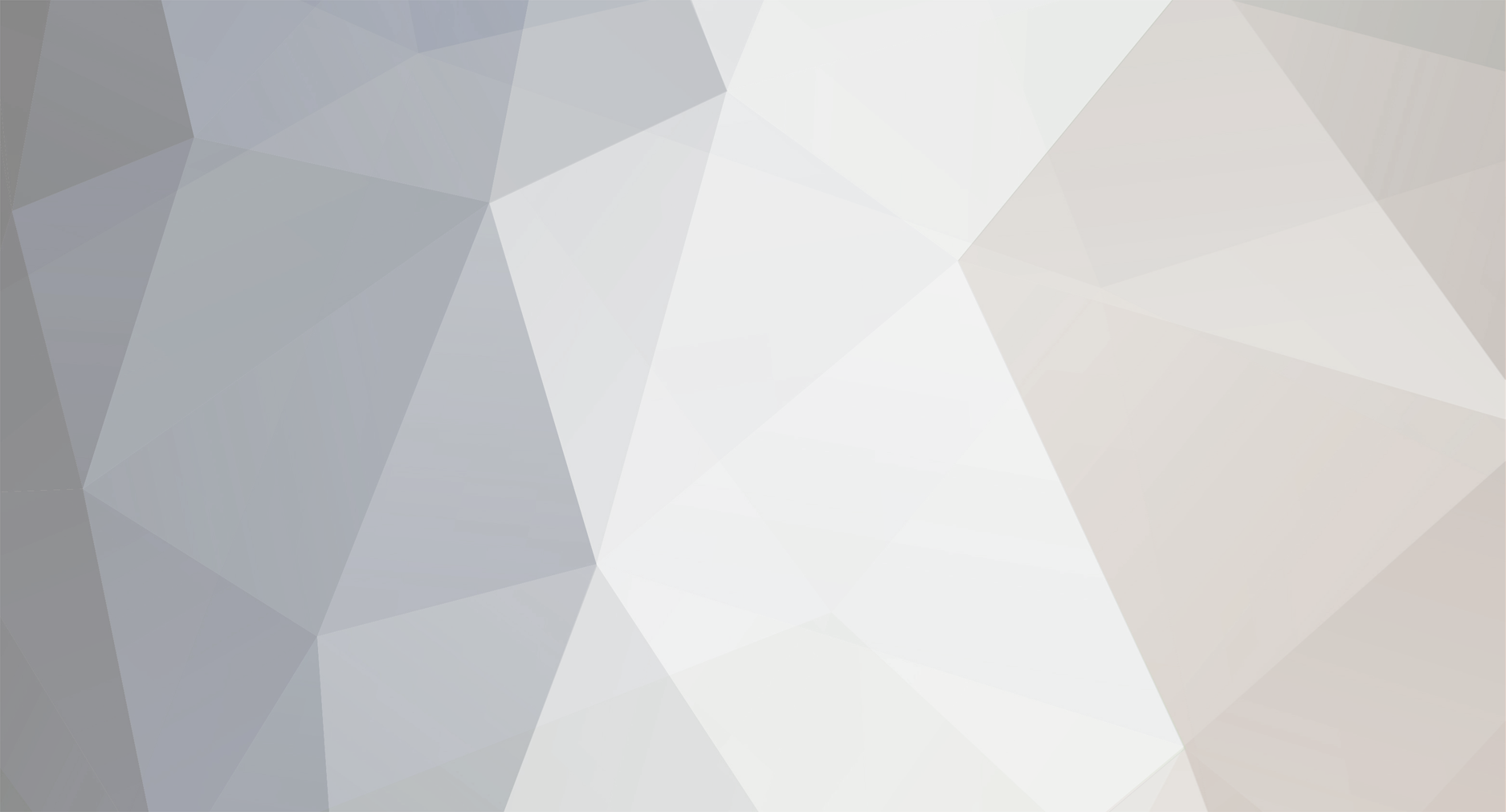
roopurt18
Staff Alumni-
Posts
3,746 -
Joined
-
Last visited
Never
Everything posted by roopurt18
-
help with sequential values within an array
roopurt18 replied to norbertk's topic in PHP Coding Help
How does a unique appointment ID show up more than once in the array? Something tells me you haven't modeled your data in the best manner possible. -
Two while loops on the same page using mysql_fetch_assoc not working
roopurt18 replied to Presto-X's topic in PHP Coding Help
I don't understand why you're using two loops. Why not use just one? Is your page XHTML? If yes, then why aren't you closing your br tags? -
Just remember that Bob and be friends with Sally while at the same time Sally is blocking Bob. You will probably want to honor "block" requests over "friend" requests when displaying information. You can accomplish this with UNION EXCEPT or sub-queries.
-
I find try...catch blocks to be result in cleaner code than the typical nested-if syndrome. You just wrap an operation in try...catch and keep calling your objects until one throws an exception. Of course at a deeper level in your code you still have the nested-if syndrome, but at least its not exposed to code at the more "abstract" or outer level of logic. As ignace has already suggested, you should run your program in a top-level exception handler. I use mod_rewrite in all of my programs to send all necessary requests through index.php and then my index.php tends to look like this: <?php define( 'APP_DIR_HOME', dirname( __FILE__ ) ); define( 'APP_DIR_APP', APP_DIR_HOME . '/app' ); define( 'APP_DIR_CLASSES', APP_DIR_APP . '/classes' ); // more defines... require_once( APP_DIR_CLASSES . '/Site.php' ); try { $site = Site::getInstance(); $site->run(); }catch( Exception $ex ) { Site::TopLevelException( $ex ); } ?> My Site::TopLevelException usually does the following: 1) Generate a unique ID, call it UniqueID 2) Log the exception message and UniqueID to APP_DIR_LOGS . '/errors.txt' 3) Display a message to the user of the form: Your most recent action has resulted in an unexpected condition; please try again or call support with ID# <UniqueID-goes-here> A) I can use exception-based error-handling throughout my project, which means code at a higher level of logic is usually cleaner. B) Users are not exposed to nasty error and exception messages. C) If an exception I have not planned for is thrown, my program displays a more friendly message to the user in addition to an ID I can use to find the exact exception in my log file.
-
There's nothing wrong with echo. However you will find that your life as a program maintainer will be made significantly easier when files are mostly PHP or mostly markup. It's not always the case but I tend to keep most of my PHP at the top of a file. Using some sort of view and controller mechanism helps as well; basically MVC without the M.
-
No. You don't store multiple values in a single "cell" as delimited strings. Table: member Columns: id | name | ... Table: member_friends Columns: id | member_id | friend_id Table member_blocked_members Columns: id | member_id | blocked_member_id EXAMPLE: Table: member Columns: id | name | ... 3, Jerry 4, Bob 5, Larry 6, Sally Table: member_friends Columns: id | member_id | friend_id 1, 3, 4 -- Jerry is friends with Bob 2, 3, 6 -- Jerry is friends with Sally 3, 5, 6 -- Larry is friends with Sally Table member_blocked_members Columns: id | member_id | blocked_member_id 1, 4, 5 -- Bob has blocked Larry 2, 6, 5 -- Sally has blocked Larry
-
You should still type out the fields you are using explicitly. If the table has more columns added to it later, then they will also be returned by the *. If one of those column names collides with another table in your query, then your page that was working will now be broken with MySQL errors complaining about ambiguous column names. Stop being lazy and type the column names you expect.
-
SELECT u.`id`, ... FROM `users` AS u ... If you have that in your query, then the id field returned is that from `users`. This is one reason we use aliases. When a query contains multiple tables and the tables have columns with the same name, we want to identify which table the column we want comes from. As for your ORDER BY question, why not consult the MySQL manual? http://dev.mysql.com/doc/refman/5.0/en/select.html It clearly shows ORDER BY coming after WHERE.
-
SELECT u.* -- select these rows from these tables FROM `users` AS u --use table users, alias it as u JOIN `user_status` AS us ON u.id = us.user_id --use table user_status, alias it as us, include only rows where u.id=us.user_id JOIN `friends` AS f ON us.user_id = f.friend_id --use table friends, alias it as f, include only rows where us.user_id=f.friend_id -- I left out your WHERE, not because you don't need it, but because I don't feel like interpreting it
-
Prevent a form from processing if required fields aren't completed?
roopurt18 replied to JoeAM's topic in PHP Coding Help
Check the form links in my signature if you're still having trouble. And when the user is using NoScript, what then? -
I don't see any reason why storing PHP code in MySQL is not secure. If you're storing the programming code in MySQL, then make sure you call mysql_real_escape_string() on the code before inserting it into the database. When you pull the code to display in a browser, just make sure you call htmlentities() before echo'ing it. To fix the spacing, place it within < pre> and </ pre> tags (without spaces of course).
-
Aliases: FROM <table_name> [AS <alias>] The square brackets mean the AS <alias> is optional. If you provide it, then you can give the table an alias, or alternative name. FROM `users` AS u Means that you don't have to repeatedly type `users` in your query. Now you can just use u. SELECT u.`id`, u.`name`, u.`email` FROM `users` AS u WHERE u.`active`=1 Aliases are barely useful when a query runs on just one table. They are incredibly useful when multiple tables are involved in a query or when the same table is involved multiple times, such as during joins or sub-queries.
-
Where is best to store user info for a secure area
roopurt18 replied to gibbo1715's topic in PHP Coding Help
I typically only store the users UserID in the session. If UserID is not in the session, then the user is not logged in. If UserID is stored in the session then it is the primary key of the user's data in the database and I can pull it in with a query on each page load. I would not concern myself with the overhead of running a similar query on each page load. The database has a query cache in memory that it will return results from if the query is frequently run. Consider that sessions are [by default] stored as files on the server. Anything you store in the session is therefore stored in a file. If you're on a shared hosting account then you don't know who or what has access to these files. You also don't know when a backup might kick off and anything in those files might even be backed up and sent to who knows where. Just be careful about what you store in sessions. -
One last thing. All of that free-floating code in the global namespace, it gives me bad feelings. I like to really, really make sure my pages and their variables are completely self-contained. Therefore I usually define a base Page class with common functions like: redirect( $url, $msg = null ) // redirect to $url, optionally save a message in $_SESSION to redirect page to display / use get_session_message() // returns saved session message post_val( $name, $default ); // retrieves from $_POST with optional default query_val( $name, $default ); // retrieves from $_GET with optional default is_post() // returns true if post page... i.e: return count( $_POST ) > 0 get_db() // returns handle to database db_escape( $val ) // escapes database value so I don't have to markup_escape( $val, $entities = true, $striptags = true ) // will strip tags and htmlentities a value abstract function run(); // derived pages must provide this method Now your page can look like this: <?php //TODO // Page should be in a different file class Page { protected function post_val( $name, $default = false ) { return array_key_exists( $name, $_POST ) ? $_POST[ $name ] : $default; } protected function get_db() { static $db = null; if( $db === null ) { // TODO Change path to correct one include( dirname( __FILE__ ) . '/db.config.php' ); $db = new mysqli( HOST, USERNAME, PASSWORD, DATABASE ); } return $db; } protected function insert_query( $table, $values ) { $db = $this->get_db(); $cols = array_keys( $values ); foreach( $cols as $key => $col ) { $cols[ $key ] = "`{$col}`"; } foreach( $values as $col => $value ) { $values[ $col ] = $db->real_escape_string( $value ); } return " insert into `{$table}` ( " . implode( ', ', $cols ) . " ) values ( " . implode( ', ', $values ) . " ) "; } protected function log( $msg ) { // TODO Write $msg to a log file of your choosing. } } class CreateLinkPage extends Page { public function run() { if( $this->is_post() ) { $vals = array(); $vals[ 'link_name' ] = $this->post_val( 'link_name' ); $vals[ 'color' ] = $this->post_val( 'color' ); $vals[ 'parent_link' ] = $this->post_val( 'parent_link' ); $vals[ 'article' ] = $this->post_val( 'article' ); $vals[ 'url' ] = $this->post_val( 'url' ); $vals[ 'admin' ] = $this->post_val( 'admin' ); if( $vals[ 'url' ] != '' ) { $vals[ 'artucle' ] = ''; } $insert_qry = $this->insert_query( 'links', $values ); $db = $this->get_db(); if( $db->query( $insert_qry ) ) { $this->redirect( 'new_url.php', "Link added successfully." ); }else{ $this->log( $db->error ); $this->redirect( 'new_url.php', "Unable to add link at this time." ); } } $html = ''; // TODO rest of your code for non-post echo $html; } } $pg = new CreateLinkPage(); $pg->run(); ?>
-
// Form was submitted, we need to create the link if(isset($_POST['submit'])) { See this thread: http://www.phpfreaks.com/forums/index.php/topic,167898.15.html error_reporting(E_ALL); ini_set('display_errors', '1'); session_start(); define('ROOT', "../"); require_once(ROOT . "includes/config.php"); $mysqli = new mysqli(HOST,USERNAME,PASSWORD,DATABASE); I imagine the head of all your files looks like that. Seems like a lot of redundant typing to me. Why not use mod_rewrite and send all requests through index.php. Then you can perform those steps in index.php and later include() the necessary page based on $_SERVER['REQUEST_URI']. $ln = $mysqli->real_escape_string($_POST['link_name']); You're not checking that 'link_name' is present in post and opening your script up for errors. You should do something like: $link_name = post_val( 'link_name' ); // And if link_name has a default you can use then you can do: $link_name = post_val( 'link_name', 'Untitled Link' ); function post_val( $name, $default = false ) { return array_key_exists( $name, $_POST ) ? $_POST[ $name ] : $default; } $_SESSION['msg'] = "Link was not added: " . $mysqli->error; I imagine this message is displayed to the user. Unless you're the only one using this, there's never a good reason to display database message errors to users. Instead you should log the database error so you [the developer] can see what happened. The user should get something more generic like Unable to add link at this time. $result = $mysqli->query("SELECT * FROM articles WHERE nolink = 0"); You should avoid select * and instead list the columns you are expecting by name. This prevents the database from passing unnecessary data over the socket, which speeds things up. And if this table grows to have more columns and many, many rows select * can negate the benefit of any indexes you place on the table. $articlebox .= "<option value=''>None"; You're not closing any of your option tags. If this page is supposed to be XHTML strict [which is ideal in most circumstances], then not closing tags makes it invalid markup. Invalid markup will adversely affect any CSS or JavaScript you write. Often times JavaScript (dealing with the DOM) or CSS rules don't work as expected and it's often because of bad markup. But people don't realize this so they write 5 times more CSS or JavaScript to work around the problem instead of just closing the necessary tags. $articlebox .= "<option value='{$row[0]}'>{$row[1]}"; What's $row[0]? What's $row[1]? That's not very descriptive. Oh sure, today they're name and description. But what happens if you add a column before description? Then $row[1] is no longer description and your code breaks. Instead why not use: while( $row = $result->fetch_object() ) { echo $row->id . ' ' . $row->name . PHP_EOL; // assuming columns are id and name } fetch_object() is the best! "<option value='{$row[0]}'>{$row[1]}"; Why are you not using htmlentities()? Should be: $row[0] = htmlentities( $row[0], ENT_QUOTES ); $row[1] = htmlentities( $row[1], ENT_QUOTES ); "<option value='{$row[0]}'>{$row[1]}"; <form name='form' action='create.php' method='POST'> I'm pretty sure attributes are supposed to be lower-case nowadays. $footer = getFooter(); echo $footer; Could be echo getFooter();
-
Probably due to historical and backwards-compatibility reasons. Almost every C-based programming language has globals and many other languages as well. I've had many programming courses taught by people with years of programming experience and I've read many programming books. When it comes to globals both resources pretty much say, "This is a global. Now it can be used anywhere. That said, don't use globals." And they're right.
-
There's probably more reasons than I can think of off the top of my head why not to use globals, but first and foremost is they break the encapsulation of a function. Functions are intended to receive values as arguments and return values via a return statement. When you write a function without globals, you know a lot more about the variables within that function. Consider this: function foo( $a, $b, $c ) { $total = $a * $b + $c; // Let's say $total is now: 34 magical_function(); // Does $total still equal 34? Yes it does! // We know for a FACT it is 34 because it is not global and we did not assign to it. echo $total; // Still 34! } Now consider this: function foo( $a, $b, $c ) { global $total; $total = $a * $b + $c; // Let's say $total is now: 34 magical_function(); // Does $total still equal 34? We DONT KNOW! // It's global so magical_function might have altered it without our knowing. echo $total; // Might be 34! Might be something else! } Now you say, "So what?" Well when your programs are small you can easily navigate to magical_function() and see if it modifies the global $total. If your program is 10 lines or less, then maybe this is practical. As your programs increase in size, however, it becomes more and more difficult to track down the true value of the variable. Then your program will inevitably have a bug in it and because you've used globals you're going to have a difficult time tracking down the source of the problem. Not to mention you'll be very, very likely to fix A but break B. Then you'll fix B and both A and C will break. Lastly, consider that programmers are lazy and don't like typing; therefore variable names are recycled. For example I commonly use $name as a variable name. When you start introducing globals you have a hard time keeping track of the variable $name. Is this the global name? Is this a local $name? Use globals at your own peril and don't expect to be hired by any intelligent managers you submit code to with global in it. The very best reasons to not use globals are: 1) They cause problems (as I explained) 2) They're completely unnecessary. I've written some pretty complicated, functional programs that span thousands of lines of code. I've not used global once.
-
Automatically re-sending the user to an https link
roopurt18 replied to svgmx5's topic in Apache HTTP Server
Nope. I do it all the time. Maybe something else in the syntax is wrong. Try turning on RewriteLog and RewriteLogLevel. -
Automatically re-sending the user to an https link
roopurt18 replied to svgmx5's topic in Apache HTTP Server
The rule you've provided says "If HTTPS protocol is ON, then redirect to HTTPS." You want a rule that says "If HTTPS is OFF, then redirect to HTTPS." Such a rule is: RewriteCond %{HTTPS} !on RewriteRule .* https://%{HTTP_HOST}%{REQUEST_URI} -
global $mail; echo $mail['footers']; Also, you're a bad person for using globals.
-
You don't need to know anything about XML to use AJAX. Most common JavaScript frameworks / tools transfer data as JSON and PHP has JSON encode / decode functions. So really all the OP needs to know is PHP, JavaScript, and the appropriate way of invoking an AJAX call with a popular framework like Dojo or jquery or whatever else exists out there.
-
This reminds me of the many times on [back when I played] WoW or more recently xbox when someone desperate asks: R U GURL?!?!?! It never works.
-
You might try looking at ActiveMQ. It's essentially a pub / sub mechanism. My coworker has been looking at it intently since HTML5 supports web sockets.
-
I meant the SVN client not the server version. I forgot to mention that SVN (server binaries) is hosted on a separate server. Well, of course an SVN client needs to be installed on the development server; otherwise how would you check code in / out? I guess it could be confusing to someone unfamiliar to version control reading this thread.
-
I admit it; that made me lol.