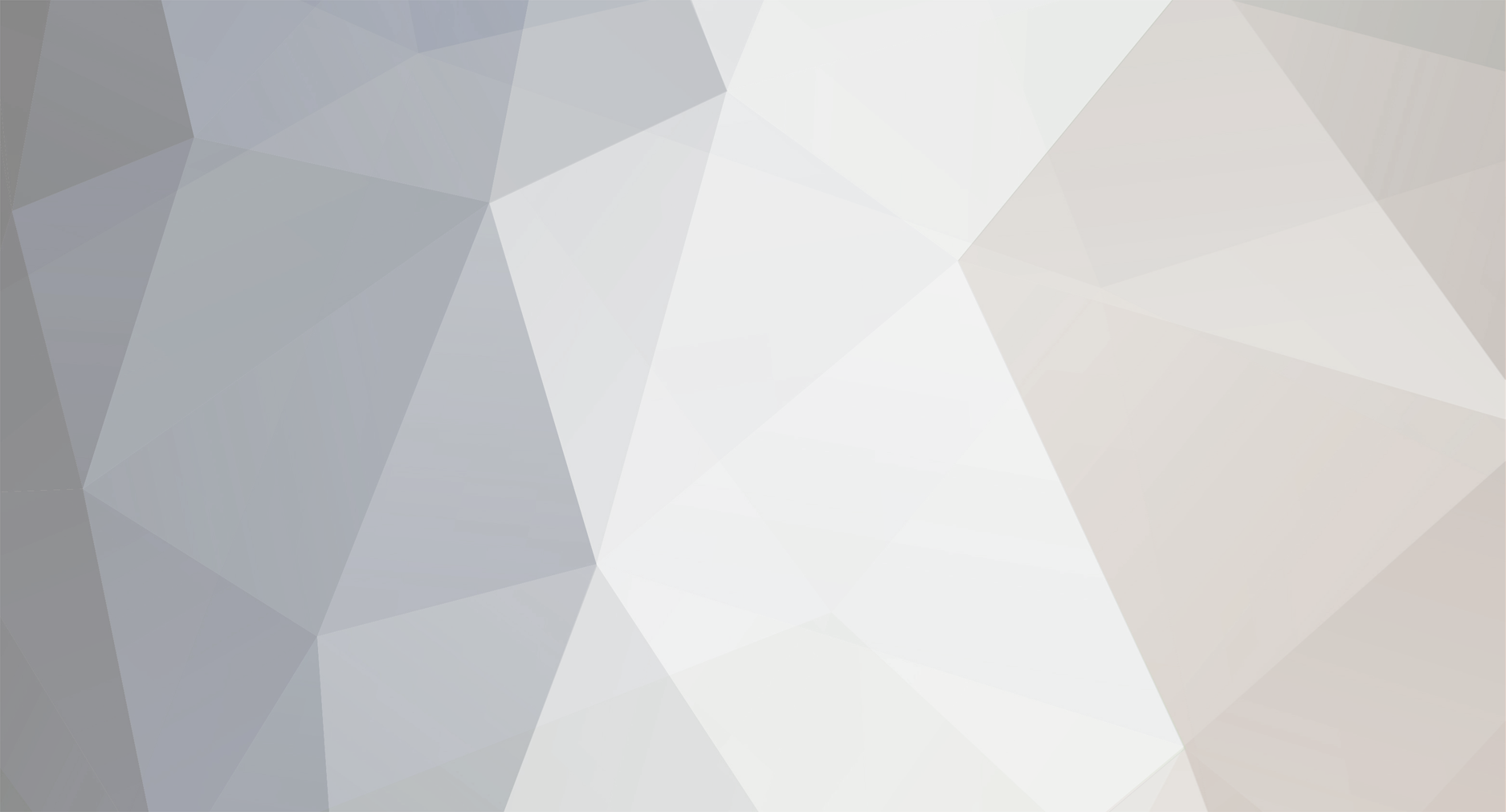
nogray
-
Posts
930 -
Joined
-
Last visited
Posts posted by nogray
-
-
You can use Javascript to change the form action as the following
<select onchange="document.getElementById('people').action = this.value">
Put include phpmady solution in case the user javascript is turned off.
-
You can try and see if it works. I find it more reliable to create a function in the main page to alter the values.
-
Since you are in an iframe within an iframe, you would need to create a few functions
in the main page (outside the iframes), create a function that update the value
ie function update_val(val) { document.getElementById('my_id').value = val; }
in the first iframe window, you'll need to create a function that call the parnet update_val function. (function something(val) {parent.update_val(val);})
In the last iframe, you'll need to make a function that call that function. You also try to call parent.parent.update_val(val) from the inside iframe.
-
Try to change it to the following and see if that's solve the problem
<html> <head>CSS</head> <body> html code JS source files script tag $(document).ready(function(){ script code that require dom }); </body> </html>
-
Is all your scripts in the bottom of the page (right above the </body> tag) and are you using the jquery ready function?
-
you can use document.getElementsByTagName('input') which will return an array with all the input elements. Loop through it and check if the type is "checkbox"
-
depending on what is "this" and if it has a child or not.
-
This could be because of loading from cache and execution time. If javascript runs before the dom is created, you will get errors. This happens even if you put the code below the dom element because new browsers execute cached or local javascript very fast.
You would need ot make sure everything is loaded and use the ready function.
-
Maybe try my calendar that will put all the events automatically,
-
Put your code in an onload function or in the bottom of the body (above the </body> tag). JavaScript in the head section loads before the body so the images are undefined.
-
You would need UTF-8 or another encoding for your website.
<meta http-equiv="Content-Type" content="text/html; charset=utf-8" />
-
Yes, the function pass the entire query into a php via post and some scripts out there actually do some dumb stuff like that. A few years ago someone asked me to check why his video sharing website database was wiped clean. After reviewing the code, I found that the script was creating the mysql query in javascript and passing them into php via post. With so many scripts out there, there is always someone who contributes unsecure code without knowing the problems it can cause.
-
Just a quick note, you might want to read up on mysql injection and security issues. It might sound like a lot, but it's pretty simple and will save you a lot of headaches in the future.
-
Start here on how to get the page with PHP curl http://php.net/manual/en/book.curl.php
Once you managed to get the page text, you would have to a lot of work to get the text you need out (by searching, spliting, replaceing, etc)
-
Here is a very simple ajax request. If you are building a game and don't know how to do this, you might want to consider hiring someone.
// call the ajax request by using update_xy(New X, New Y); function update_xy(x, y){ // make sure to validate the x and y values in your php page var url = 'my_page.php?X='+x+'&Y='+y; var xhr = new XMLHttpRequest(); this.privates.xhr.open("GET", url, true); xhr.send(null); }
Not tested
-
Simple, something like this will work
<div id="my_cal_div"></div> .... later after adding the script files ..... <script type="text/javascript"> ng.ready(function(){ // review the link in my previous post for all the possible options var my_cal = new ng.Calendar({object:'my_cal_div', visible:true}); }); </script>
-
Databases are queried via user input all the time. What do you think a user registration form ultimately does? Or when data is retrieved based on a GET value? The key is in sanitizing the input.
Yes, but the data is validated and filtered before you store them in the database and the actual query happens in the backend. I've seen open source project where they use something like this
ajax.query("update `sometable` set `something` = 'something'");
on the front end javascript code. As you can see, that's a huge security risk. All I ment is to never run an actual sql query from a user input. Collect the parts you need for the query, validate, filter and run it in the backend.
-
Search for javascript tooltip and you'll find planty of scripts out there (some are small). The only way to show it instantly is by using a div to display the title content.
-
You connect to the database using PHP. Never run a query on your database from a user input or your website will be hacked right away. To change the session variable, simple call a php page that will change the session variable to whatever you want (directly or via ajax).
-
What url do you want? is the class="bump" the class name of the link or will it be a part of the URL
Maybe this
function golink() {window.location.href('bumpbox.php?id=<?php echo $pageinfo['id'];?>&class=bump&rel=800-500');}
-
check out my calendar at http://www.nogray.com/calendar.php
-
I am not a jquery expret, but try to change
$('#my_form').submit();
with
document.getElementById('my_form').submit();
If still no luck, try to comment out the code inside the suggest function and comment out the 2 lines above the submit code to make sure nothing is causing an error in your code.
-
Without the html, we can't debug this problem. What's the action of your form? the .submit() will not fire the onsubmit event if you are using ajax.
-
Are you using ie9 64 bit? If so, flash does not have a distrubtion ready version for ie9 64 bit. You can download their beta (which doesn't play everything) or use the normal 32 bit.
Javascript do something only once
in Javascript Help
Posted
If you remove the function or only allow it to work one time, you would need to create a function for each input field (which defeats the purpose of a function). You can do something like this instead (notice the title is the same as the default value)