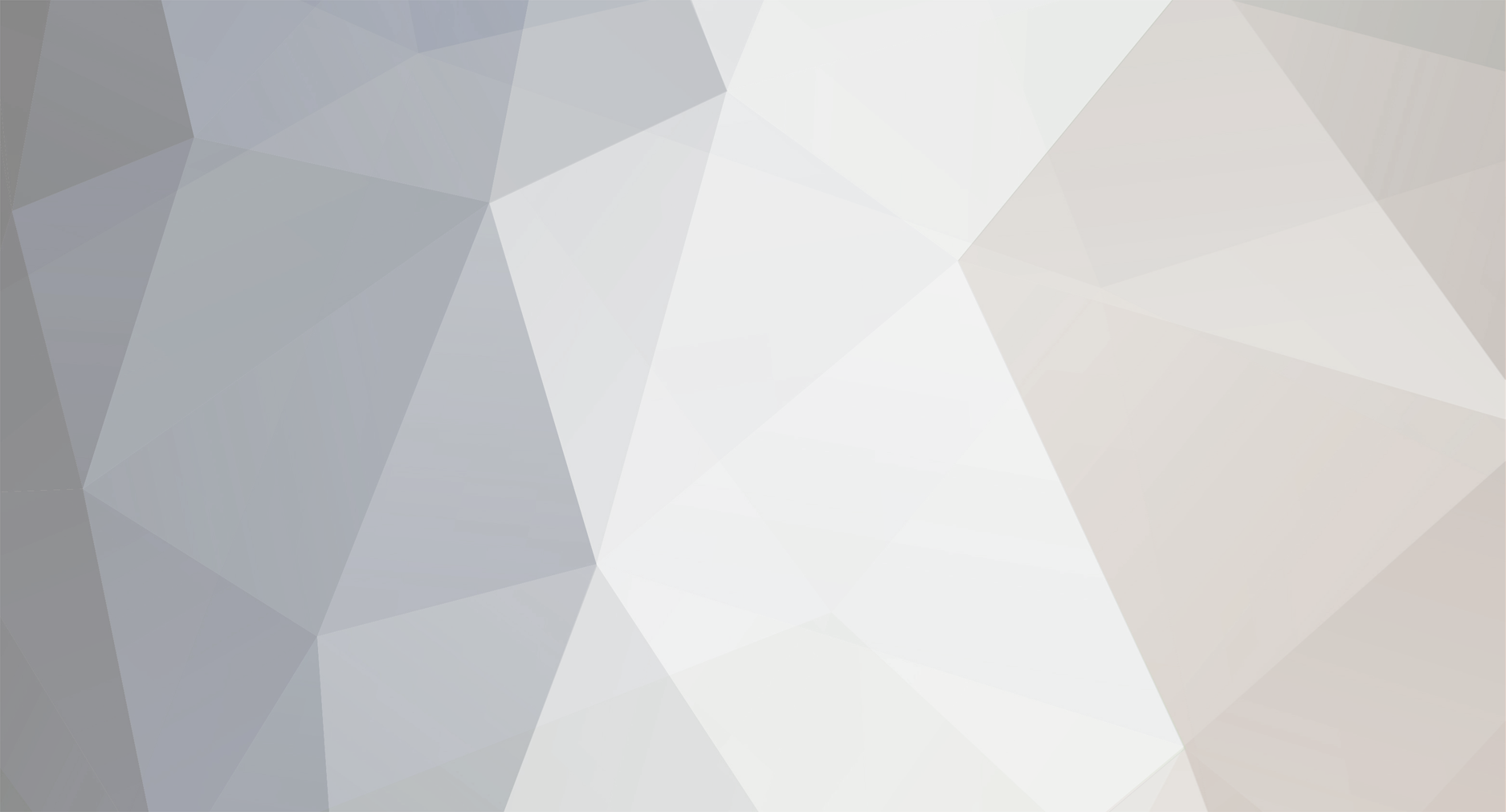
nogray
-
Posts
930 -
Joined
-
Last visited
Posts posted by nogray
-
-
try this
var h = Math.max(document.body.clientHeight, document.documentElement.clientHeight); alert(h);
-
Add an id to your file input field
<input name='photo_filename[]' type='file' id='my_photo' />
and use document.getElementById()
if (document.getElementById('my_photo').value == "") { ...
-
Use a pre tag and replace all the < with < and > with >
If you have dreamweaver, copy the code you want to display, go to design mode, click on where you want it and paste it. Dreamweaver will take care of the rest.
-
tables, tr, td can't have focus since the user doens't interact with them directly. Depending on what are you trying to do with the focus event, there is another approach.
You can add an anchor tag inside your td and once that anchor tag receive focus, you would simulate a focus on the td visually. Here is a quick sample.
<input type="text" value="tab out of here" /> <table id="my_table" style="cursor:pointer;" onclick="do_focus(); do_click(); document.getElementById('my_anchor').focus();"> <tr> <td> <a href="#" id="my_anchor" onclick="do_click(); return false;" onfocus="do_focus();" onblur="do_blur();"></a> This is some text </td> </tr> </table> <input type="text" value="tab out of here" /> <script type="text/javascript"> function do_click(){ alert('clicked'); }; function do_focus(){ document.getElementById('my_table').style.background = "#336699"; document.getElementById('my_table').style.color = "#ffffff"; document.getElementById('my_table').style.border = "dotted 1px #000000"; }; function do_blur(){ document.getElementById('my_table').style.background = "#ffffff"; document.getElementById('my_table').style.color = "#000000"; document.getElementById('my_table').style.border = "0px"; }; </script>
-
Well, he'll have to fix it in IE9 because it's all broken up. No fliker though
-
When you set the style you need to add "px" after the top or left.
e.g.
cropFrame.style.left = ((cropPicWidth / 2) - (cropFrameWidth / 2))+"px"; cropFrame.style.top = ((cropPicHeight / 2) - (cropPicHeight / 2))+"px";
-
This might not be a loading problem, but an execution time problem. The browser might load the JS file and continue on the page and load the next file, but there might be a delay in executing the first file and the second file start executing which causes the error. This can happen specilly if you are loading locally or from cache.
To avoid this problem, make sure all your functions are in the ready function or add a defer="defer" to your additional script tags (after the jq).
-
whenever you alter the innerHTML of an object, the browser will reset the entier object html (and you would lose and dom refrence as well). You should add the tr, td using document.createElement(tag) and object.appendChild(child)
-
Could be a load issue, make sure your script is placed in the bottom of the body and executes once the page is loaded.
-
You might have a line break here
<br /> <textarea
JavaScript doesn't support multi line string, either put them in one line or split the string.
-
You should make the pop-up close itself (e.g. window.close()) after sending the value.
Parent window cant' close the pop up unless you have specific security settings or a trusted html application.
-
Your .moncalimari is too far to the left, so the width will be as wide as the longest word. Setting the width property will fix the issue.
-
You are forgetting the quotes around the id string (since it's a string in the setimeout), this will fix it
window.setTimeout("fnHide2('" + oToHide.id + "')", 3000);
-
Just for security reasons, place your site in the trusted sites and only enable that option for trusted sites zone. Browsing the internet with ActiveX on might compromise your computer security.
-
.hta is an HTML application and need to run on a desktop (not on a server). I tested on IE 9 as an html file on my desktop, I did something similar long time ago with IE7.
There are a few zones for IE, make sure activex is allowed on your security zone (either local intranet or internet). ActiveX is not recommended for browsing the internet in general.
.hta files is an HTML file that runs like a desktop application without any security restrictions.
If you need this to run as a server app, you might have to add the your site (or localhost) to the trusted sites security zone and allow activex to run on trusted sites. Also, make sure the file bath is correct and accessible from your page.
-
First your editable content area should be iframed to avoid security issues and conflicts. I assume you have an "Edit HTML" button, whenever the user change the HTML code, you would need to scan it for any scripts (including events) or flash objects and remove them.
-
Could you be a security setting that is blocking the activex, try to rename your .html file to .hta and try again.
-
Basiclly,
If the user computer doesn't have the lucida grande, it will try tahome. If not avaliable, verdana, etc...
-
hummm, don't think there is a book that will show this trick. You'll just have to search for different techniques (even in different programming languages) and try to apply the same principles to your code.
-
Technically, you cannot print directly using javascript without user interaction. The reason your script doesn't work is because the print dialog will block any script exection until the user either clicked yes or no.
However, you can run around this issue by creating a text file and opening in with a print command. The sample code below will only print the text of your page (will get the innerText of your print div).
<div id="my_print_div"> <strong>This is a test</strong><br /><br />Hello World </div> <script type="text/javascript"> // the text file name (same as the html file + .txt) var txt_file = "C:\\Users\\Desktop\\test.txt"; // you will have to change this, use double \ // creating a file and printing it function create_file(){ var fso = new ActiveXObject("Scripting.FileSystemObject"); var f = fso.CreateTextFile(txt_file, true); f.WriteLine(document.getElementById('my_print_div').innerText); f.Close(); print_file(); }; function print_file(){ var shell = new ActiveXObject("WScript.Shell"); shell.Run("notepad.exe /p "+txt_file); }; create_file(); </script>
Notes: Use a <br /> for line breaks and the output is only text. Use double \ in the file path to avoid wrong path issues.
If you can get the printable content in a RTF format (for text format), you can replace the .txt in the file name to .rtf and change NotePad.exe to wordpad.exe
-
To set the height in CSS to a percentage, you would need to set the height of all the parent elements including the html document. Otherwise, the browser doesn't have a refernace for the percentage. Last is to add overflow:auto to the content div.
e.g.
html, body {height: 100%}
This might not work the same in all browsers, not tested
-
If you use the NoGray Calendar http://www.nogray.com/calendar.php you can set the date_format option to "jS F Y" and leave the server_date_format as the default "Y-n-j" you would get exactly what you need.
Disclaimer: I am the developer of the NoGray Calendar
-
You would need to add a value="" to the Select a Category and add an ID to the select menu
<select name="dropdown" id="dropdown"><option selected="selected" value="">Select A Category</option>
and test for value == ""
// I don't use jQuery if (document.getElementById('dropdown').value == "") { // do something }
-
Try my calendar =)
Recognizing if a specific select option was chosen?
in Javascript Help
Posted
try this