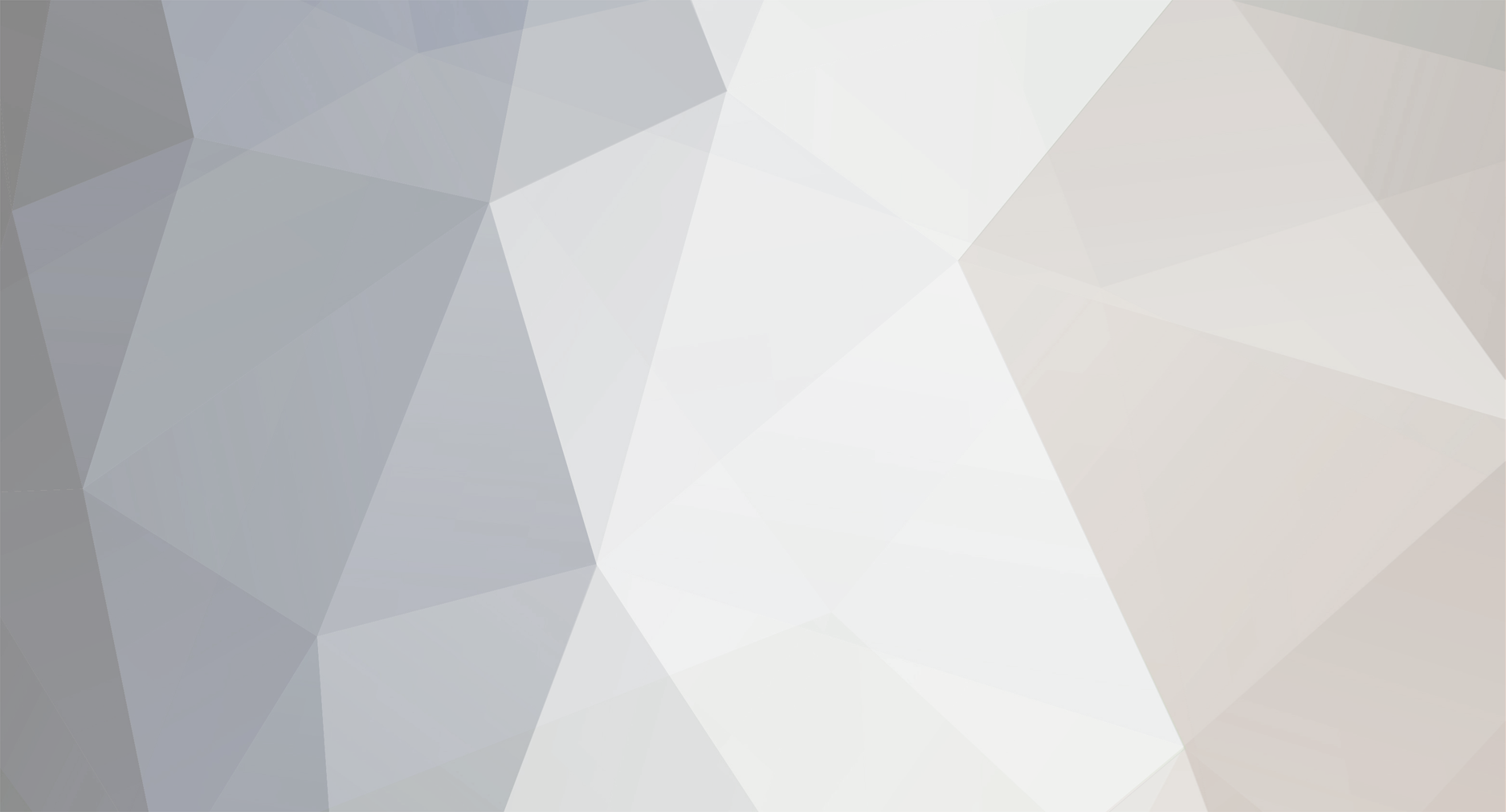
nogray
-
Posts
930 -
Joined
-
Last visited
Posts posted by nogray
-
-
You can simply add an onclick event
e.g.
<input type="radio" onclick="location.href='another_page.html';" />
-
You can do
window.onbeforeunload = function(){ return 'are you sure you want to leave?'; }
-
Your index will always starts at -1 because you defined it as var index = -1; in the begining than your function. Instead, you would need to define the index as a global variable
e.g
var index = -1; setInterval((function() { .... // do not use var index anywhere inside this funciton.
-
You would add an array to hold the error messages and in the end alert that array
e.g.
function validateForm(){ var err_arr = []; ...if (x=="") { document.getElementById("li_25").className = "error"; err_arr.push(' - field name'); } ... if (err_arr.length){ alert('Please complete the following:\n'+err_arr.join('\n')); return false; } return true; }
-
You can add a variable on top of your function to hold the valid status and check all the fields. If one is invalid, set the variable to false. In the end of the function, return that variable.
e.g.
function validateForm(){ var is_valid = true; ... if (x=="") { document.getElementById("li_25").className = "error"; is_valid = false; } ... return is_valid; }
-
The range of dates is approximately 285,616 years from either side of midnight, January 1, 1970. Negative numbers indicate dates prior to 1970.
If the age is before 1970, you would need to split it and calculate the time before 1970 and add it to the time after 1970.
Also, a date picker is not a good tool for birthdates, I don't want to click back 100 times to get back to my birthdate.
-
You can add a onresize event to the window and check if the browser is too small, than resize the window.
-
On the head of your page, add the following
<script>document.write('<style>._js_hide{display:none;}</style>');</script>
Use the class _js_hide in all the elements you want to hide
-
Create new date for each set (var st_dt = new Date(Year, Month, Date); // Month starts from 0 for Jan) and compare the times ( st_dt.getTime() < end_dt.getTime())
-
Now I see your html, you have a few issues. You can't have a div as a table element (outside the <td>), this will break your code. You can try to add the id into the tr, but I don't think you can write the tr html directly either. You would need to loop through the table cells inside that tr and update their content.
Sam
-
#user_rows represent an id and it should be unique in the page. If that's the case, you can just use $('#user_rows').html(); otherwise use a class.
-
You would need to add an html declartion on top of your page or you'll be working in quirk mode,
e.g. Instead of just html tag you'll have
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml">
-
try
<a href="#" onclick="location.href='my_url';">
-
SCRIPT5009: 'vBulletin_init' is undefined
bfgamerz.com, line 980 character 2
you'll need to make sure it's defined, if not, remove it from your code. the consule will give you the line to look at. This error could be blocking the rest of the script.
-
document.AddCReturn.product_id[x] should be document.AddCReturn['product_id'+x]
-
You actually need to edit your PHP file on the server to handle the files array. It seems your PHP script is only looking for one input file called data. If you want to do it with JavaScript, the form will have one file input. Add an onsubmit event to the form that create a new iframe, change the form target to the new iframe. After the form is submitted, you would reset it.
You can remove the iframe after you get your results.
-
in IE9, hit F12 to get the developers tools which should show you where is the error.
-
In IE9 hit the button "F12" and you'll get the developers tools. Click on "Consule" to find the errros on your code.
-
When you try to upload multible files, you need to use a array as the name for the input field
<input type="file" name="my_file[]" />
In your php, you $_FILES['my_file'] should be an array of files, so instead on uploading it once, you would loop through it using a foreach statment
foreach ($_FILES['my_file'] as $file){ // upload $file }
In some servers, using a array name for the input field doesn't work. You get a "string" value "Array" instead of arrays. In that cause, you can use a standard name (e.g. my_file_## where ## is a unique number) and do a foreach loop for the $_FILES and upload all that matches that name, or loop through the $_FILES and upload everything.
Sam
-
window.parent[ptarget]
-
It doesn't work because the syntax is incorrect. It should be
$("#time1000" + i +", #time1000" + x).timePicker();
-
You can't do that because the other site is not on the same domain. Browser Security.
-
You would first create a new element (e.g. new div) using document.createElement. Append the text in the new element and insert it where you need. You can use the insertBefore method
e.g.
var dv = document.createElement('div'); dv.innerHTML = 'my text'; var aftr = document.getElement.....; aftr.parentNode.insertBefore(dv, aftr.nextSibling);
-
replace
setStyle(p[i],"fontSize","font-size","58px");
with
p[i].style.fontSize = '58px';
and remove the entier function
Why the alert message is not appear
in Javascript Help
Posted
change && z='') to && z=='')