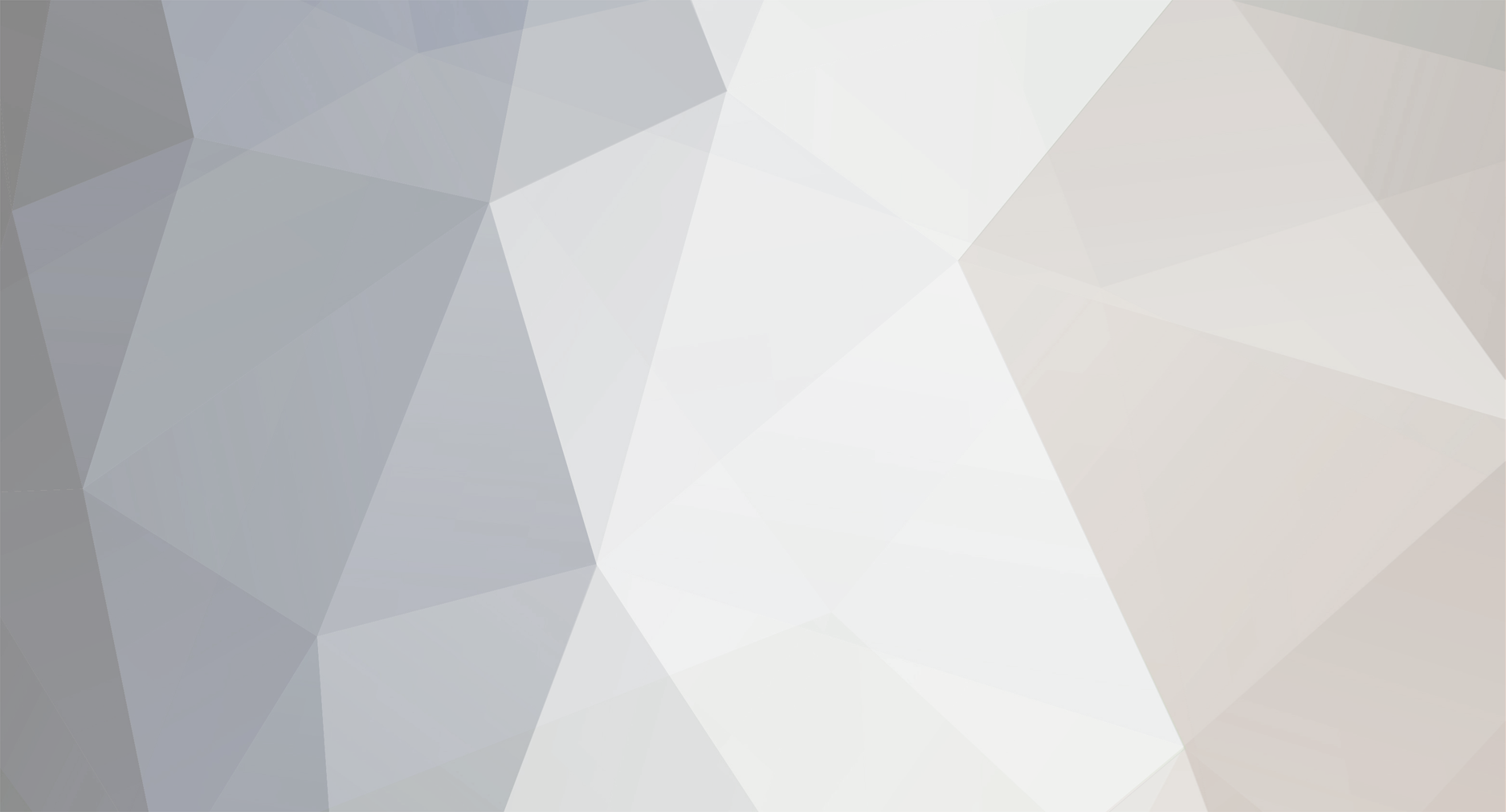
nogray
-
Posts
930 -
Joined
-
Last visited
Posts posted by nogray
-
-
You posted the part that works, but not the part that reset the class names. It's usually done with an if else statement (not sure what it didnt' work for you), but here is an example
if (document.registration_form.name.value == "") { document.getElementById("name_blank").style.display = "inline"; valid = false; document.registration_form.name.className = "invalid_form"; } else { document.getElementById("name_blank").style.display = "none"; document.registration_form.name.className = "valid"; }
-
anything that moves from the server to the client will consume bandwidth. You can try to gzip the text content to reduce the bandwidth but that's about it. Unless you are getting tons of clicks, I wouldn't worry about bandwidth too much. Most hosting companies offer a lot of bandwidth (if not unlimited) for a very low price.
-
Read CSSFREAKIE signture (reset, doctype) and if you are using margin:-25px, there might be an issue in your html that is causing the layout problems.
-
The reason why the author added two images is because the height issue with fluid layout. If you assign a background color to the news and side divs, and the content was too long. The news and side background won't extend down with the content. You can try to remove the background images and assign background colors to the news and side divs, add extra content and resize the window to see the effect.
If you want the same style layout (three color background to extend all the way for the three divs regardless of content and size of window) you would need to use the same images width. You can resize the height to 1px if that will save some kb.
P.S. I never read the book, but got the author intentions from your post and the sample you posted
-
value="408080" should have a hash mark, e.g. value="#408080" or the value will be invalid (for all the colors).
-
This could be a security zone conflict in your browser setting. For example, if you have a page on your PC, or a trusted security zone and you click on a link to a third part site or try to open a third party site that is in different security zone (e.g. default zone), IE will open it in a new window.
-
return will return a value from a function (just like php return). You can use break to stop the loop.
-
You can add an onchange event and set the div background color style to that value
e.g.
<input type="text" onchange="document.getElementById('my_div_id').style.backgroundColor = this.value;" /><div id="my_div_id"> </div>
This code won't validate the user input and an invalid color will cause an error.
-
you would need to loop through the array to test
e.g.
for (var i=0; i<array.length; i++){ if(search.test(array[i])){document.write('true');} }
p.s. document.write will overwrite the entier page.
-
Depends on the library you are using (from your sample, probably PrototypeJS or mooTools). It's about the same as document.getElementById() but adds extra methods to the objects.
-
-
Why is this in the middle of the buttons </form>, it looks like your code will not validate and the </form> will add a line break in IE. close the form where it should (not in the middle of the div between the buttons).
-
Try this,
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Snooker Brackets</title> <style type="text/css"> .base { background-color: #CF6; height: 50px; width: 400px; } .player1 { background-color: #000; height: 50px; width: 400px; color: #FFF; } .player2 { background-color: #fff; height: 50px; width: 400px; } .player1over { background-color: #009; height: 50px; width: 400px; color: #FFF; } .player2over { background-color: #C9C; height: 50px; width: 400px; } .class { background-color: #C9C; height: 50px; width: 400px; } </style> <script> function change_class(player, cls){ var my_ids = ['_div2', '_div3', '_div4', '_div5', '_div6']; for (var i=0; i<my_ids.length; i++){ document.getElementById(player+my_ids[i]).className = cls; } } </script> </head> <body style="margin: 0pt;"> <div class="base" id="player1_div1" onmouseover="change_class('player1', 'base player1over');" onmouseout="change_class('player1', 'base player1');">Player 1</div> <div class="base" id="player2_div1" onmouseover="change_class('player2', 'base player2over');" onmouseout="change_class('player2', 'base player2');">Player 2</div> <div class="base player1" id="player1_div2">Player 1</div> <div class="base player2" id="player2_div2">Player 2</div> <div class="base player1" id="player1_div3">Player 1</div> <div class="base player2" id="player2_div3">Player 2</div> <div class="base player1" id="player1_div4">Player 1</div> <div class="base player2" id="player2_div4">Player 2</div> <div class="base player1" id="player1_div5">Player 1</div> <div class="base player2" id="player2_div5">Player 2</div> <div class="base player1" id="player1_div6">Player 1</div> <div class="base player2" id="player2_div6">Player 2</div> </body> </html>
-
In that case you would need to submit the form and reproduce the form with the data (also update the form based on the make value)
e.g.
<select onchange="document.getElementById('my_form_id').submit();">
-
add type="button" to your buttons or change them into <input type="button" value="..." />
-
JavaScript strings can't have multible lines and probably your JS code looks something like this
sometextarea.value = "line 1 bla bla bla line2 bla bla bla line 3 bla bla bla "
You can replace the carriage returns with \n and it will show up as carriage returns in the textarea
-
Add an onchange event
<select name="make" onchange="location.href='calendar.php?page=booking&day=11&month=3&year=2011&make='+this.value;">
-
You need to change your onmouseover and onmouseout events to call the function, e.g.
onmouseover="change_class('player1', 'player1over');"
Also you would need to change this line
var my_ids = ['_div1', '_div2'];
to include the other divs (up to 6 from your code).
-
you can use document.getElementsByTagName('tag name here') to get an array of the elements. e.g.
HTML <ul id="my_list"><li>message 1</li><li>message 2</li></ul> Script var ul = document.getElementById('my_list'); var li_arr = ul.getElementsByTagName('li'); for (var i=0; i<li_arr.length; i++){ // do something }
If you are going to remove or add elements from the parent, you would need to use a while loop instead of for loop since the length property will change.
-
I don't think the font names are case senstive, I use arial all the time (but it's Arial in my system) and it works in every browser. try this code and see
<style> * {font-family:cOuRiEr} </style>
-
use http://www.xml-sitemaps.com/ and save yourself the headache
-
In your case, I'll make a function that will check the value and return the integer or a 0;
e.g.
function my_parse_int(val){ val = parseInt(val, 10); if (isNaN(val)) return 0; return val; }; ... change the onchange event to onchange="document.getElementById('total').value = (my_parse_int(document.getElementById('f1').value) ....) *1.5;
-
getElementsByName() will return an array of elements. If you only have 1 br in your page, you can access it by br[0]. Otherwise loop over the array and replace all the brs
-
First you would need to add a unique id to all your div tags (but they should all have a common part to match the player)
e.g.
<div class="..." id="player1_div1"></div><div class="..." id="player1_div2"></div>...
Than, create a javascript function that change the class name for all the ids listed,
e.g.
<script> function change_class(player, class){ var my_ids = ['_div1', '_div2']; for (var i=0; i<my_ids.length; i++){ document.getElementById(player+my_ids[i]).className = class; } } </script>
and your onmouseover and onmouse out will call the function with the appropriate variables.
Form Validation
in Javascript Help
Posted
Well, you would need to reset all the class names before you start validating the form, for example add "clear_errors()" in top of the validation to remove all the invalid classes. If you have a URL, I can help looking up the problem.