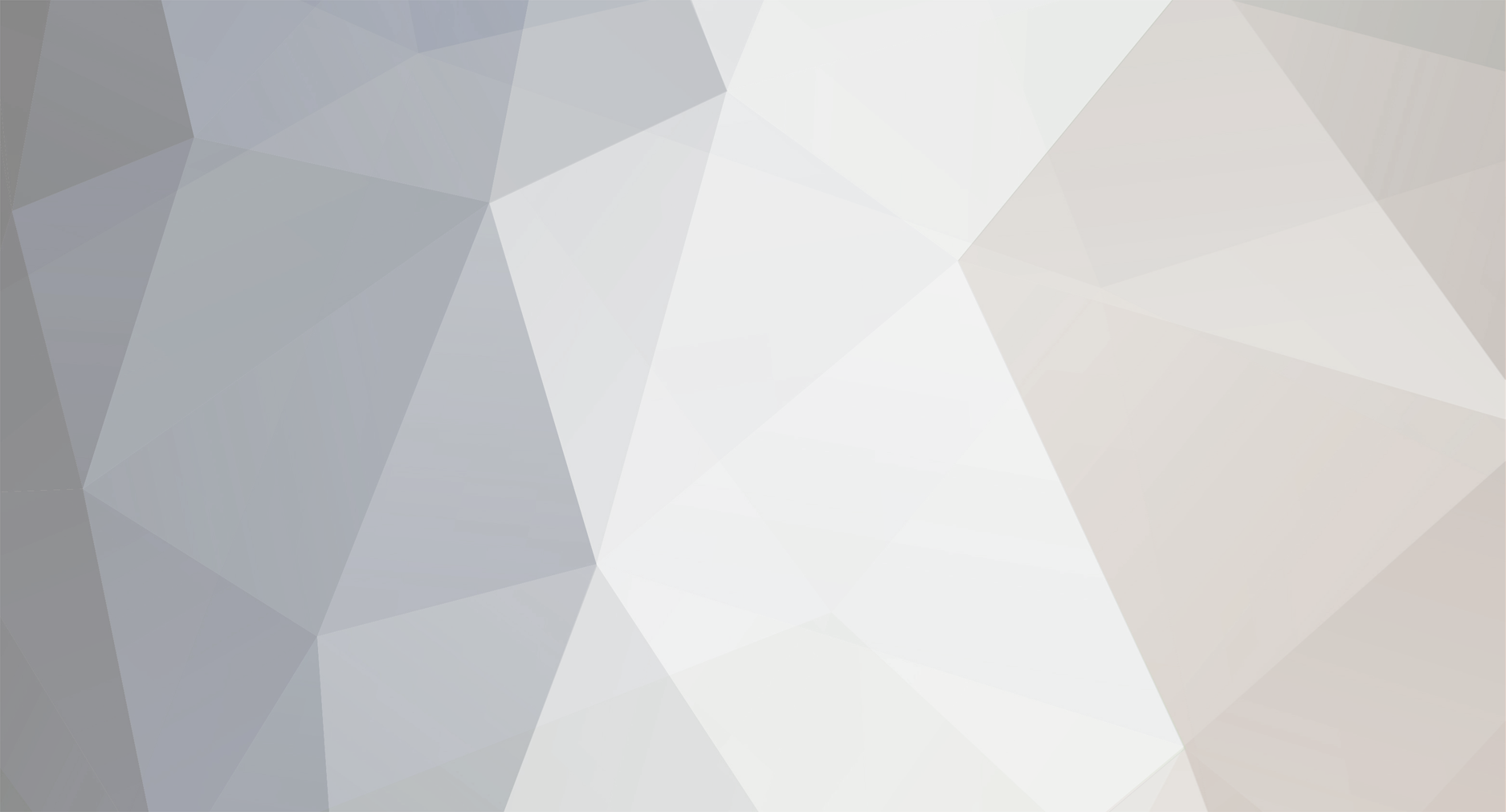
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Savings Coumpound Interest (With Monthly Contribultion)
Psycho replied to Skylight_lady's topic in PHP Coding Help
Is the monthly contribution supposed to be included with the interest calculation or not? If yes $IntTotal = ( ($balance + $contribution) * (pow(1 + $Rate, $terms) - 1) ); If no $IntTotal = ($balance * (pow(1 + $Rate, $terms) - 1) ) + $contribution; -
Aside from your question I would add the following advice: Instead of copying the files into a subfolder I would advise creating a new top level directory, copy all your files there, and then create a sub-domain for your development environment, e.g. dev.mydomain.com. If you have any file paths in the PHP or HTML that is relative to the root of the web root or the URL you will run into errors if just running from a sub-folder. But, then again, if your intent is to modify the site to work from sub-folders then you would want to do that.
-
Are you even sure that the query is being run? You have the query in a foreach() loop (which is very poor practice: see updated code below that will get ALL the records with one query) but what if there are no items in the array? You also have if() conditions in your code. Have you validated that what you think the variables contain they actually contain? Add debugging code into your scripts so you can see exactly what is happening rather than running code and scratching your head when you don't get the output you expect! Also, why are you storing your cart info as a comma separated string? It looks like you are repeating each item id based upon the quantity. That's way too complicated. You are storing it in the session array, just make a sub array using the id as the index and the value as the quantity (which is what you are trying to convert it into anyway). Give the following a try - not tested so there may be some minor typos function showCart() { global $db; //Prepare cart data from session variable $itemsAry = array(); if (isset($_SESSION['cart'])) { //Convert cart data to an array $itemsAry = explode(',', $_SESSION['cart']); //Convert items to integers $itemsAry = array_map('mysql_real_escape_string', $items); //Remove false/empty values $itemsAry = array_filter($items); //Create array using IDs as key and qty as value $cartAry = array_count_values($itemsAry); } //Create comma separated string of just the item IDs (enclosed in quotes) $cartIDs = "'" . implode("', '", array_keys($cartAry)) . "'"; //Create and run query $query = "SELECT BikeCode, Model, Price FROM Bike WHERE BikeCode IN ({$cartIDs})"; $result = $db->query($query); if(!$result) { //Query failed $output = "<p>There was a problem getting your cart.</p>"; //Uncomment next line for debugging // $output .= "<br>Query: {$query}<br>Error: " . mysql_error(); } elseif(!mysql_num_rows($result)) { //Cart was empty $output = "<p>Your shopping cart is empty.</p>"; } else { //Start form $output = "<form action=\"cart.php?action=update\" method=\"post\" id=\"cart\">\n"; $output .= "<table>\n"; $grand_total = 0; while($row = $result->fetch()) { $quantity = $cartAry[$row['BikeCode']]; $sub_total = $quantity * $row['Price']; $grand_total += $sub_total; $output .= "<tr>\n"; $output .= "<td><a href=\"cart.php?action=delete&id={$id}\" class=\"r\">Remove</a></td>\n"; $output .= "<td>{$row['BikeCode']}</td>\n"; $output .= "<td>£ {$row['Price']}</td>\n"; $output .= "<td><input type=\"text\" name=\"qty[{$row['BikeCode']}] value=\"{$quantity}\" size=\"3\" maxlength=\"3\" /></td>\n"; $output .= "<td>£{$sub_total}</td>\n"; $output .= "</tr>\n"; } //Close form $output .= "</table>\n"; $output .= "<p>Grand total: <strong>£{$total}</strong></p>\n"; $output .= "<div><button type=\"submit\">Update cart</button></div>\n"; $output .= "</form>\n"; } return $output; }
-
It looks like you are trying to compare the values of each number combination in the arrays multiplied by different values. So, there isn't 140,515,219,945,627,518,837,736,801 possible combinations - the possible combinations is infinite. And, knowing how many combinations would need to be tested before finding a match would require some math that makes my head hurt. The difficult part, from my perspective, would be in trying to develop the logic to know what the next highest combination would be since the numbers are dynamic. But, here is the logic I would use: 1. Create an index variable to count the number of iterations through the loop. Then use the variable to exit the loop at a set number of iterations when a match is not found. This will prevent you from getting stuck in an infinite loop or one that will cause a timeout issue. 2. Create a process to get the next highest combination from an array. This is the hard part. 3. In the loop, have the process continue as long as no match is found and the loop counter has not reached the maximum value. 4. Do a test between the current calculated value between array 1 and array 2. If they are equal, then set the value in a variable and exit the loop. If they are not equal then increment the value of the one that is less than the other. Repeat
-
Mysql_Num_Rows($Result) Not Working As Expected, Need Advise.
Psycho replied to equivalents's topic in PHP Coding Help
So, you are getting a result from mysql_num_rows() of 0 and no error is reported? That would mean the query is executing successfully, but that there were no matching records. In the above script I see where you connect to the DB server, but I don't see where you select what database to use. But, you code makes no sense. You run a query to get ALL records from that table then check if there was exactly 1 result.IF there was one result then you echo a success message, else you have an error message for wrong username/password. So, if there were 0 records or more than 1 records in that table you would always get a failure. I think you meant for your query to find any records with the specific username and password. -
No need to guess. You can simply read the manual for that statement and figure out exactly how it works: http://dev.mysql.com/doc/refman/5.5/en/select.html The part on LIMIT is about 1/10 the way down the page.
-
iNko, the previous posters are trying to help you do "proper" JOINS. The process you have been using is a normal join that JOIN every record from both tables together then excludes the ones you don't want. There is a ton of functionality using the different JOINs and if you don't learn them, you will be stuck in how much you can do.
-
Write And Erase Contents Of A File After A Length Of Time Has Expired
Psycho replied to rad1964's topic in PHP Coding Help
Please see kicken's post above that should help you get started with coding it in a better way. We understand that you may be new, but the process you are trying to implement is a very poor one. It would reflect poorly on us if we were to encourage bad behaviors in someone because they have some code they think they understand. Let me break down they basics of what we are proposing so it will, hopefully, make sense to you. I think it would be best to break it down into three distinct processes. But, one thing to understand is that there will be a "master" alerts file that contains all of the current alerts. 1. There should be a process that checks for new alert files. based upon your statements above I assume the external web page writes files to a certain directory. So, there should be logic to check for any new alert files. If any are found, the script will read the contents of those files and update the "master" alerts file (see more on this below). Then delete the file that created the new alert so you don't process it again next time. 2. There needs to be a process to allow you to manually insert/remove alerts. This would be accomplished by providing a page to view current alerts and edit/remove them or to add new alerts. When this page is posted you would check what the user chose to do and then update the "master" alerts file (see more on this below). 3. Updating the master alerts file will be done by reading the file into memory and converting to an array then making updates as follows: a. If the user chose to make a manual change then perform the appropriate action: remove/edit the selected alert or add a new alert. b. If new alerts were processed from files, then add them to the array and delete the file (so you don't add them again.) Once all changes have been made you will then encode the array back to JSON and write the contents to the "master" alerts file. c. Also, you can iterate over the alerts in the array and remove any that are expired. Now, once all of the above is complete you will have all the current alerts as an array in memory. If there were any changes from the above them convert to a JSON encoded string and write the result back to the master alerts file. Then iterate over the array and show the current alerts to the user -
Mysql Help Unknown Column 'name' In 'where Clause'
Psycho replied to Hypersource's topic in PHP Coding Help
Well, if the error is what you have put into the title of the post then the error is just as it says - there is no column with the name of "name". When debugging such errors it is always helpful to echo the actual query to the page. Otherwise you can spend hours trying to figure out a problem with a query that is actually a problem with a variable: $query = "UPDATE potions SET color='$color', pet='$pet' WHERE owner='$tik' AND name=' $name '"; $result = mysql_query($query) or die("Query: $query<br>Error: " . mysql_error()); -
That's probably because you aren't checking for those errors! if(!isset($_POST['name']) || !isset($_POST['password']) || !isset($_POST['email_address'])) { echo "Error: Required fields are missing."; } else { $name = mysql_real_escape_string(trim($_POST['name'])); $email_address = mysql_real_escape_string(trim($_POST['email_address'])); $password = mysql_real_escape_string(trim($_POST['password'])); if(!empty($name) && !empty($email_address) && !empty($password)) { $query= "INSERT INTO `a_database`.`email` (`name`, `email_address`, `password`) VALUES ('$name', '$email_address', '$password')"; $result = mysql_query($query); if($result) { echo "Account Created"; } else { echo "Error running script<br><br>Query: {$query}<br>Error: " . mysql_error(); } } }
-
Write And Erase Contents Of A File After A Length Of Time Has Expired
Psycho replied to rad1964's topic in PHP Coding Help
You are over-thinking/over-engineering your problem. Requinix provided a the solution for a basic process that would give you the results you are after. I think you aren't seeing the forest through the trees. His process would allow you to manage the alerts coming from the external web process as well as ones that are manually added - either singular or stacked. You are asking the wrong question because there is no need to run a count-down timer. When someone access the page where alerts will be shown you simply need to see if there are any new alerts to process. If so, update the "master" file with the new alerts. If no new updates - then don't update the master file. Then, show any current alerts from that master file. -
PHP has nothing to do with whether the form will submit or not. The form is built in HTML. So, the problem is either with the HTML source (including Javascript) of the form OR with the processing page. Trying to debug HTML errors from the PHP source to create it is difficult. Since you have many 'conditions' in your code there is no way for us to determine what the source of your HTML form even is. Plus, the above code would not generate a full HTML page so there is obviously other code to build that page not displayed.
-
I like the ternary operator as much as the next guy, but that code is going to be a bear to maintain. I think you would do yourself a great favor by writing out the logic if full and adding comments. I've read your question a few times and, to be honest, I don't understand what you want or, more importantly, where the logic should go. BUt, I will give it a shot. //Set the default winner value $winner = '?'; if (array_key_exists(0, $this->bracket[$fr]) && $s1 != -1 && $s2 != -1) { if ($s1>$s2 && $this->bracket[$fr][0]['c2'] = 'BY') { $winner = $this->bracket[$fr][0]['c1']; } elseif ($s1>$s2 && $this->bracket[$fr][0]['c1'] = 'BY') { $winner = $this->bracket[$fr][0]['c2']; } }
-
3 Days Of Head Scraching And Still Cant Get This?
Psycho replied to hopbop's topic in PHP Coding Help
I have o clue which array index is the age and you didn't tell us that. But, my understanding is that you want the records grouped by age ranges. You also didn't state what those ranges are - which could change the best logic to use. Instead of putting them in different arrays, put them into separate keys in the same array. Example: $query = "SELECT * FROM form_data WHERE last_update >= 2012-10-01 AND form_template_id >= 14;"; $result = mysql_query($query) or die( mysql_error() ); while($row = mysql_fetch_array( $result, MYSQL_ASSOC )) { $jsonIterator = new RecursiveArrayIterator(json_decode(trim(mcrypt_decrypt(MCRYPT_RIJNDAEL_256, $KEY, $row{'data'}, MCRYPT_MODE_ECB)), true)); foreach ($jsonIterator as $key => $val) { //Set AGE_KEY to the appropriate key for the age $age = $jsonIterator[AGE_KEY]; if($age<10) { $primaryKey = '0-9'; } elseif($age<=20) { $primaryKey = '10-20'; } elseif($age<=30) { $primaryKey = '21-30'; } elseif($age<=40) { $primaryKey = '31-40'; } elseif($age<=50) { $primaryKey = '41-50'; } else { $primaryKey = '51+'; } $items[$primaryKey][] = $val; } } -
If you were to take the time to properly explain your request you would have already had a solution by now. Based upon mine and the other responder's first posts you obviously did a poor job of it. I don't mean to berate you, just point out that it is beneficial to you (and considerate of those providing help) to take the time to effectively write a query. Your last response is much different than your original question and contains a significant change - there are two variables in the strings. You also haven't stated where $keywords_id is coming from. Is it a static variable that will be the same for each $keywords value? What you want to achieve can't be achieved through implode(), but based upon your last response and some of my assumptions for what you have NOT provided, you can try this foreach(array_keys($keywords) as $keyword) { echo "<a href='{$keywords_id}'>{$keyword}</a>."; }
-
Huh? Is this what you mean? echo "<a href=\"\">" . implode("<br />\n",array_keys($comments)) . "</a>"
-
That's beyond my skillset. But, in testing that code in the hopes of breaking it down it doesn't seem to be working for nested content. Using this as the content: $content = '<h1>heading</h1><p>page content</p> <p>outer content 1 <p>Nested Content</p> outer content 2 </p>'; The regex is succeeding, but with 0 matches. I'm actually quite interested in this possible solution as I had to implement a workaround to a similar problem in some previous code and I'd like to go back and refactor if there is a simpler solution.
-
You are making it harder than it needs to be. Just do the one query and process the records in a while() loop. Then if you have certain fields that can be duplicated that you want printed one time, create a flag variable in the loop to check when it changes. Here is a basic example based upon what you have now $keyword_id = false; while($row = mysql_fetch_assoc($domains_sql)) { //Check if this keyword ID is different from the last if($keyword_id !== $row['keywords_id']) { //Display keyword as header echo "<br><b>Keyword: {$row['keywords_id']}</b><br>\n"; } //Display domain $domains_name = preg_replace('#^https?://www.#', '', $row['domains_url']); echo "$domains_name<br>\n"; }
-
As the others have stated we need to know exactly how the content can vary. But, IF the variable will only contain 1 pair of P tags then a simple regex will suffice. In fact, you can use regex if there are multiple P tag pairs as long as they are not nested and properly paired.. //If only one P tag pair in content if(preg_match("#<p>[^<]+</p>#i", $content, $match)) { //Assign the paragraph to a variable $para = array_shift($match); } else { $para = false; } //If multiple P tag pairs in content if(preg_match_all("#<p>[^<]+</p>#i", $content, $matches)) { //Assign the paragraphs to an array $paraAry = array_shift($matches); } else { $paraAry = false; }
-
Retaining Drop Down Select Values After Submitting A Form
Psycho replied to kdigital's topic in PHP Coding Help
I would highly suggest creating a function to create and auto-select the options instead of putting the code for each and every option. I typically define my options as an array and then pass the array and the currently select value (if there is one) to a function. Also isset($_POST) is worthless since it will always be set even if there was no form posted. Lastly, I would also consider changing the values of the options to something that won't cause problems with HTML markup. The displayed label can still be with the quote marks though. In my example below, I used what 'appears' to be a decimal value, but is just the feet/inches separated by a period. This is just a rough example <?php $height_options = array( '5.0' => "5'0\""; '5.2' => "5'2\""; '5.4' => "5'4\""; '5.6' => "5'6\""; '5.8' => "5'8\""; '5.10' => "5'10\""; '5.0' => "6'0\""; ); function createOptions($optionsAry, $selectValue=false) { $optionsHTML = ''; foreach($optionsAry as $value => $label) { $value = htmlspecialchars($value); $label = htmlspecialchars($label); $selected = ($selectValue===$value) ? ' selected="selected"' : ''; $optionsHTML .= "<option value='{$value}'>{$label}</option>\n"; } return ; } ?> <select name="height"> <>php echo createOptions($height_options, $_POST['height']); ?> </select> -
Sorry, I thought it was only numbers. That's easy enough to fix: just use the period character (to match against any character) for the additional characters to pad the code out to 16 characters. This also simplifies the process a bit since you can just use str_pad() since you will be padding with just a single character '.' vs. '\d' //Array of alarm codes and their descriptions $alarm_codes = array( '7694181110' => 'Fire Alarm', '7694183110' => 'Fire Alarm Clear', '7694181111' => 'Smoke Alarm', '7694183111' => 'Smoke Alarm Clear', '7694181302' => 'Low System Battery', '769418130101000' => 'AC Power Failure', '769418330101000' => 'AC Power Restore', ); //Specify the file $file = 'latest.txt'; //Get contents of file $contents = file_get_contents($file); //Make replacements in content for each alarm code foreach($alarm_codes as $code => $description) { $pattern = str_pad($code, 16, '.'); $replacement = "{$code} {$description}"; $contents = preg_replace("#{$pattern}#", $replacement, $contents); } //Write new content to file file_put_contents($file, $contents);
-
This seems to work per your requirements and only requires you to add a new alarm code and description to the array //Array of alarm codes and their descriptions $alarm_codes = array( '7694181110' => 'Fire Alarm', '7694183110' => 'Fire Alarm Clear', '7694181111' => 'Smoke Alarm', '7694183111' => 'Smoke Alarm Clear', '7694181302' => 'Low System Battery', '769418130101000' => 'AC Power Failure', '769418330101000' => 'AC Power Restore', ); //Specify the file $file = 'latest.txt'; //Get contents of file $contents = file_get_contents($file); //Make replacements in content for each alarm code foreach($alarm_codes as $code => $description) { $addlDigits = str_repeat('\d', 16 - strlen($code)); $pattern = "#{$code}{$addlDigits}#"; $replacement = "{$code} {$description}"; $contents = preg_replace($pattern, $replacement, $contents); } //Write new content to file file_put_contents($file, $contents);
-
Although regular expressions are slower than string functions, I think that is the best solution for you in this situation. But, file_put_contents() is probably not a good choice at it will likely encounter a performance hit as well since each call is effectively opening, writing and closing the file. It would probably be better to read the file into a variable, modify the contents of the variable, then write the file one time. //Specify the file $file = '/etc/asterisk/alarm/latest.txt'; //Get contents of file $contents = file_get_contents($file); //Make replacements in file $contents = preg_replace('#7694181110\d{6}#', '7694181110 Fire Alarm', $contents); $contents = preg_replace('#7694183110\d{6}#','7694183110 Fire Alarm Clear ', $contents); $contents = preg_replace('#7694181111\d{6}#','7694181111 Smoke Alarm ', $contents); $contents = preg_replace('#7694183111\d{6}#','7694183111 Smoke Alarm Clear ', $contents); $contents = preg_replace('#7694181302\d{6}#','7694181302 Low System Battery ', $contents); $contents = preg_replace('#769418130101000\d#','769418130101000 AC Power Failure ', $contents); $contents = preg_replace('#769418330101000\d#','769418330101000 AC Power Restore ', $contents); file_put_contents($file, $contents); Note, the \d represent any digit and the {6} means to repeat the previous character 6 times. So, the first 5 replacements are looking for the specific number followed by any 6 additional digits. The last two are looking for the specific number followed by any one digit. But, if all of the codes in the source file are always 16 digits, I'd suggest creating an array of all your alarm codes and creating a function to dynamically do the replacements.
-
Search engines cannot view any information you do not make available to them. If being logged in is a requirement to see some information, then that would not be available to the search engine.
-
As the other two already stated, using a JOIN to create ONE query to get all the records you need is the correct solution. Having queries in loops is one of the worst things you can do with respect to performance. If you provide the first query we can help with how to create the query and how to process it.