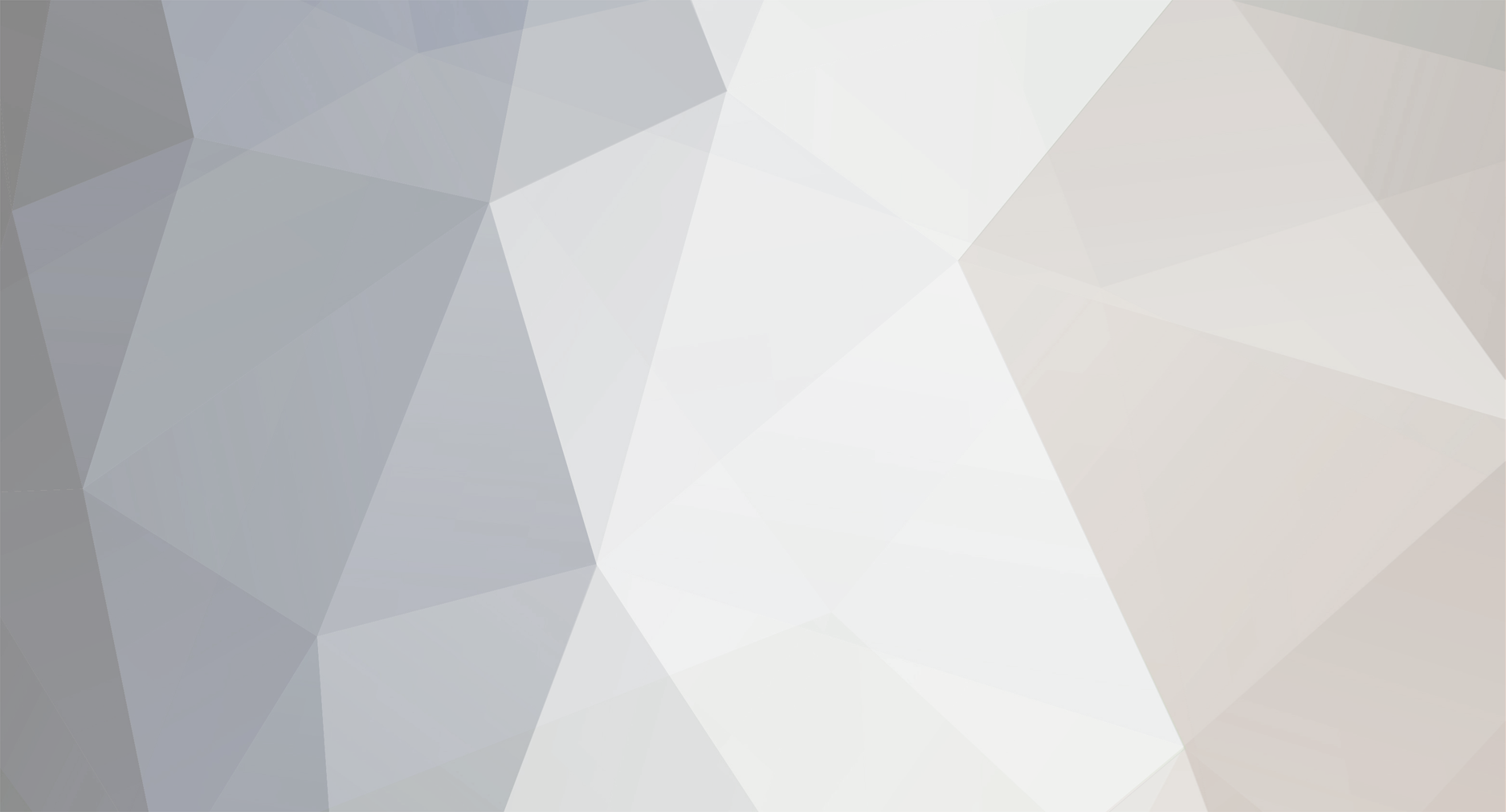
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
OK, I think you you need to slow down a moment. Let's take it one step at a time. You have a list of records that you want to update on a single form. Each record has (at least) 5 fields. So, you need to supply five input fields for each record. And, most important, you must have a way to logically associate those fields together so that when you POST the form you know which ones go together. In your code above you are giving the fields the exact same name. That will not work since only the last field will be sent in the post data. Each field MUST have a unique name. But, you can use arrays as your name. That is the key. In this case I would name each field according to the value it contains, i.e. the price field will be named price (for all of the price fields). But, you make it an array and use the record's unique ID as the index of the array. So, for example <input type="text" name="price[$rec_id]" value="$price"> Then, when the form is posted you can associate each "group" of fields using the record ID.
-
I find it extremely helpful to echo the query to the page when I encounter an error. Create your queries as a string variable THEN use that for your query. You will be amazed at how much easier it is find and fix these errors. Of course, you shouldn't be exposing any of these errors in a production environment. $query = "INSERT INTO tmp_price_compare (supplier_reference,quantity,price,wholesale_price) VALUES($supplier_reference,$quantity,$price,$wholesale_price"; mysql_query($query) or die("Query: $query<br>Error: " . mysql_error());
-
If you have already created a login process you are 80% there. As part of the login you should be storing some information to identify the user within session data - typically this could be the user ID. Also, if you have specific downloads for each user you *must* have that information stored somewhere such as a database. And that information should be tied to the user in some way (e.g. the user ID). So, on the page to display the downloads you would just use the user ID stored in the login session data to query the available downloads for that user. If you have some downloads that are available to more than 1 person, then you should at least have two tables: 1 to describe the downloads and another to specify which DLs belong to which users. So, the code could look something like this: if(!isset($_SESSION['user_id']) { //redirect to login page exit(); } $userID = intval($_SESSION['user_id']); $query = "SELECT name, path FROM downloads JOIN user_downloads USING(download_id) WHERE user_downloads.user_id = $userID"; //Execute query and output the results
-
I would suggest that instead of passing strings such as "2012-01-05" as the parameter on the URL - just send an offset from the current date (i.e. +5). It will make your code cleaner and validation simpler (which you are not doing now). It also helps in that you don't have to manipulate a lot of strings. Keep data in as simple/agnostic a format as possible until needed. <?php //Get offset from URL parameter, default is 0 $offset = isset($_GET['offset']) ? intval($_GET['offset']) : 0; //Define display date using current date and the offset $display_date = date('l F j, Y', strtotime("$offset days")); //Determine prev/next offsets based on current offset $prev_off = $offset - 1; $next_off = $offset + 1; ?> <h3 class="home-title"> Schedule for Today <span class="date"> <a href="test.php?offset=<?php echo $prev_off; ?>"><img src="assets/images/lftarrow.png" width="28" height="12"></a> <span class="datetxt"><?php echo $display_date;?></span> <a href="test.php?offset=<?php echo $next_off; ?>"><img src="assets/images/rtarrow.png" width="28" height="12"></a> </span> </h3>
-
Local vs. Global variable - Example from W3schools
Psycho replied to dmfgkdg's topic in PHP Coding Help
There is no echo inside the function. I'm not sure which echo you are referring to but the output is exactly as I would expect. When the script runs it will follow this logic: Define $a and $b as 5 and 10, respectively Define the function myTest() Echo the value of $b (which is currently 10 as defined at the beginning of the script) Call the function myTest() which . . . - Makes the scope of $a and $b global (so they have the values as defined outside the function) - Redefines $b as the sum of $a and $b (5 + 10) which is 15 - Function ends, execution picks up from where the function was called Echo the value of $b (which is now 15 since it was modified globally within the function)- 5 replies
-
- local variable
- global variable
-
(and 1 more)
Tagged with:
-
You almost have it. I'm guessing you are running that same block of code three times which is like picking a card out of a hat, putting the card back, and picking another card. You need to pick three words without starting over. After you shuffle all the words to randomize them you just need to pick the top three records. $filename="words.txt"; $words=file($filename); shuffle($words); $randomWords = array_slice($words, 0, 3); echo "<pre>" . print_r($randomWords, true) . "</pre>"; // Or echo $randomWords[0]; echo $randomWords[1]; echo $randomWords[2];
-
Additionally, checkbox elements are not sent in the POST data if they are not checked. Since your checkboxes have unique names (i.e. you are explicitly defining the array index) you can do a simple isset() check to determine whether they are checked or not. In fact, using $_POST["resid[0]"]=="is-on-benefits" Would produce a PHP warning message if you had not checked that option. The warning should be suppressed in a production environment, but it's best - IMHO - to not have them in the code.
-
This forum is for people to get help with code they have written. Are you looking for someone to do this for you? If so, we can move this to the freelance forum. If you are wanting to do this yourself, then we are willing to help. But, you have to at least make an effort. There are plenty of tutorials out there on this subject. And, there are plenty of posts on this forum where the same question/problem has been raised. In fact, there was one today! Have you read a tutorial or other forum post and having a problem understanding part of it? What have you done already?
-
loop through days 1 - 28 and then start again 1 - 28
Psycho replied to brown2005's topic in PHP Coding Help
I guess so. This will work - and again removes the problems with many calls to date() and strtotime() <?php //Determine the year $dYear = isset($_REQUEST['year']) ? trim($_REQUEST['year']) : false; if (strlen($dYear)!=4 || !ctype_digit($dYear)) { $dYear = date("Y"); } //Create vars for the code assignments $code = 16; //Start value $codeMax = 28; //Maximum value //Max number of cells for the days $maxCells = 37; //Create var to hold the output $calendarBodyHTML = ''; //Var to test for current day $todayStr = date('Y-n-j'); //Iterate through each month for($dMonth = 1; $dMonth <= 12; $dMonth++) { //Create needed date/time variables for the month $monthTimeStamp = strtotime("{$dYear}-{$dMonth}-01"); $monthName = date('F', $monthTimeStamp); $daysInMonth = date('t', $monthTimeStamp); $dowStart = date('N', $monthTimeStamp); $monthDaysHTML = ''; $dDay = 1; for($cellIdx=1; $cellIdx<=$maxCells; $cellIdx++) { //Show empty cell for days before/after days in month if($cellIdx<$dowStart || $cellIdx>$daysInMonth+$dowStart-1) { $monthDaysHTML .= "<td class=\"empty\"></td>\n"; } else { //Reset code, if needed if($code > $codeMax) { $code = 1; } //Determine appropriate class for the dow & day $dayClass = ($cellIdx%7==0 || $cellIdx%7==6) ? 'weekEnd' : 'weekDay'; if("{$dYear}-{$dMonth}-{$dDay}" == $todayStr) { $dayClass = 'currentDay'; } $monthDaysHTML .= "<td class=\"day {$dayClass}\"><b>{$dDay}</b><br>[{$code}]</td>\n"; //Increment code and day of week values $code++; $dDay++; } } //Create output for the current month $calendarBodyHTML .= "<tr>\n"; $calendarBodyHTML .= "<th class=\"monthName\">{$monthName}</th>\n"; $calendarBodyHTML .= $monthDaysHTML; $calendarBodyHTML .= "</tr>\n"; } //Create the calendar headers $dowNames = array('Mo', 'Tu', 'We', 'Th', 'Fr', 'Sa', 'Su'); $calendarHeadHTML = "<th>{$dYear}</th>\n"; for($cellIdx=0; $cellIdx<$maxCells; $cellIdx++) { $calendarHeadHTML .= "<th>{$dowNames[$cellIdx%7]}</th>\n"; } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Linear Calendar</title> <style type="text/css" media="all"> body { font-family: "Calibri"; color: #009; } table { border-collapse: collapse; border: 1px #009 solid; } td { height:60px; vertical-align: top; text-align: center; width: 30px; } .monthName { text-align: left; vertical-align: middle; padding-left: 10px; width: 10px; } .empty { background: #ccc; } .day:hover { background: #9F0; border-color: #000; } .currentDay { background: #FFC; color:red; } .weekDay { } .weekEnd { background:#ECECFF; } </style> </head> <body> <table width="100%" border="1" cellspacing="0" cellpadding="0"> <tr> <?php echo $calendarHeadHTML; ?> </tr> <?php echo $calendarBodyHTML; ?> </table> </body> </html> -
A couple tips: separate your logic (i.e. PHP) from your presentation (i.e. HTML). Put all of your PHP logic at the top of the script (or even in another file) and use the PHP to put the appropriate HTML content into variables. Then in the HTML just use simple echo statements to put that output where needed. Trying to read code that goes in and out between HTML/PHP can get confusing. It will make it easier for people to help you and it will make it easier for you to maintain. As to your problem, have you tried running that query in your database admin software (e.g. phpmyadmin for MySQL)? Are the records duplicated there? If so, the problem is your database construction, the data, or your query. I have no idea how your database tables are set up or what data is in them. Based upon what you have posted I don't see anything specific as to whay the records in your select list would be duplicated if there was only one instance of each value in your result set from the query.
-
loop through days 1 - 28 and then start again 1 - 28
Psycho replied to brown2005's topic in PHP Coding Help
Well, I'll help. But, here's the thing. If I really don't like something in someone's code I will change it. I'm not being paid to do this, so that's my perogative. And, I really don't like how you are outputting the calendar. It looks really odd how each month/row is staggered from the previous. I also moved the "logic" to the top of the page. Using functions such as date() and strtotime() are inefficient. So, I rewrote the logic from the ground up. Since we know there are 12 months in a year we only need to determine the number of days in each month to figure out how to build the calendar. So, we only need to use those functions once for each month - not each day. <?php //Determine the year $dYear = isset($_REQUEST['year']) ? trim($_REQUEST['year']) : false; if (strlen($dYear)!=4 || !ctype_digit($dYear)) { $dYear = date("Y"); } //Create vars for the code assignments $code = 16; //Start value $codeMax = 28; //Maximum value //Create array of weekday names for headers $dowNames = array('Sun', 'Mon', 'Tue', 'Wed', 'Thu', 'Fri', 'Fri'); //Create var to hold the output $calendarHTML = ''; //Var to test for current day $todayStr = date('Y-n-j'); //Iterate through each month for($dMonth = 1; $dMonth <= 12; $dMonth++) { //Create needed date/time variables for the month $monthTimeStamp = strtotime("{$dYear}-{$dMonth}-01"); $monthName = date('F', $monthTimeStamp); $daysInMonth = date('t', $monthTimeStamp); $dowInt = date('w', $monthTimeStamp); //Iterate through each day $monthHeadersHTML = ''; $monthDaysHTML = ''; for($dDay=1; $dDay<=31; $dDay++) { //Check if day exists if($dDay > $daysInMonth) { //Day does not exist in current month $monthDaysHTML .= "<td></td>\n"; $monthHeadersHTML .= "<th></th>\n"; } else { //Check if code has exceeded maximum if($code > $codeMax) { $code = 1; } //Determine appropriate class for the dow & day if($dowInt%7 != 0 && $dowInt%7 != 6) { $dowClass = " weekDay"; } else { $dowClass = " weekEnd"; } $dayClass = ("{$dYear}-{$dMonth}-{$dDay}" == $todayStr) ? 'currentDay' : $dowClass; //Create the output for the day & header $monthDaysHTML .= "<td class=\"day {$dayClass}\"><b>{$dDay}</b><br>[{$code}]</td>\n"; $monthHeadersHTML .= "<th class=\"dow {$dowClass}\">{$dowNames[$dowInt%7]}</th>\n"; //Increment code and day of week values $code++; $dowInt++; } } //Create output for the current month $calendarHTML .= "<tr>\n"; $calendarHTML .= "<th class=\"monthName\" rowspan=\"2\">{$monthName}</th>\n"; $calendarHTML .= $monthHeadersHTML; $calendarHTML .= "</tr>\n"; $calendarHTML .= "<tr>\n"; $calendarHTML .= $monthDaysHTML; $calendarHTML .= "</tr>\n"; } ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Linear Calendar</title> <style type="text/css" media="all"> body { font-family: "Calibri"; color: #009; } table { border-collapse: collapse; border: 1px #009 solid; } td { height:60px; vertical-align: top; text-align: center; width: 30px; } .monthName { text-align: left; vertical-align: middle; padding-left: 10px; width: 10px; border-top-width: 3px; border-bottom-width: 3px; } .dow { border-top-width: 3px; } .day { border-bottom-width: 3px; } .day:hover { background: #9F0; border-color: #000; } .currentDay { background: #FFC; color:red; border-color: #009 } .weekDay { } .weekEnd { background:#ECECFF; } </style> </head> <body> <table width="100%" border="1" cellspacing="0" cellpadding="0"> <tr> <th colspan="32"><?php echo $dYear; ?></th> </tr> <?php echo $calendarHTML; ?> </table> </body> </html> -
Plus, you can combine PFMaBiSmAd's suggestion with a function as you tried to do. That makes it reusable function createOptions($optionList, $selectedValue==false) { $optionsHTML = ''; foreach($optionList as $value) { $selected = ($value == $selectedValue) ? ' selected="selected"' : ''; $optionsHTML .= "<option value='{$value}'{$selected}>{$value}</option>\n"; } return $optionsHTML; } $statesList = array('AL','AK','AZ','AR', ... complete list of the states); $state = isset($state) ? $state : ''; // set to an empty default value if not set in the main code above due to the form submission $stateOptions = createOptions($statesList, $state); ?> <select name="state" class="textfield" id="select"> <?php echo $stateOptions; ?> </select>
-
when empty - prevent insert, but display a message
Psycho replied to wright67uk's topic in PHP Coding Help
Create a standard process for doing your form validations and error handling - then just use it. You *could* modify the logic if you only have one or two fields, but why? -
If the page is not reloading then this is a Javascript problem - not PHP. Moving to appropriate forum. EDIT: Looking closer at your code - it IS being posted. That is why the select list is being reset.
-
This tweak to Barand's code should work: $data = array(); $years = array(); /****************** * Store in array */ while($row = sqlsrv_fetch_array( $result,SQLSRV_FETCH_ASSOC)) { $data[$row['LISTCODE']][$row['YEAR']] = $row['COUNT']; if(!in_array($row['YEAR'], $years)) { $years[] = $row['YEAR'] } } /****************** * print the array */ foreach ($data as $listcode => $counts) { echo "<tr><th>$listcode</th>"; foreach ($years as $year) { $count = isset($counts[$year]) ? $counts[$year] : 0; echo "<td>$count</td>"; } echo "</tr>"; }
-
I was trying to JOIN the table on a subquery of the same table using a DISTINCT query on the years to try and get the data such that there would always be a record for all the applicable years for each listcode - but didn't have any success. That would be the easiest to code for. The other option is to pre-process the data into an array beforehand. If someone can figure out the query I would go that route.
-
Ok, looking at the code in more detail I think I understand what you are trying to do and it is way, way more complicated than it should be. Take this for example: if( ($IOCC->ioccLabor + $IOCC->ioccTravel + $IOCC->ioccMileage) > 0 ) { $rowtot = $IOCC->ioccLabor + $IOCC->ioccTravel + $IOCC->ioccMileage; echo money_format('$%i',$rowtot) . '<br>'; $rowstot += $rowtot; } else { echo "$" . number_format("0", 2) . '<br>'; $rowstot = ($rowstot + 0); } That basically says if the sum of those three values is > 0 then do something, else to the alternative. But, in the first condition you are taking the sum of those three values and outputting them in a money format. In the alternative you are basically doing the same thing, but with a hard coded value of 0. So, why the if() condition at all. If the sum is 0 just do the same thing. I believe this will do exactly the same thing - but without all the complexity and without the potential infinite loop. $grand_total = 0; foreach ($resultsIOCC['IOCC'] as $IOCC ) { if( $IOCC->ioccCostID == $Cost->CostID ) { $sub_total = $IOCC->ioccLabor + $IOCC->ioccTravel + $IOCC->ioccMileage; echo money_format('$%i', $sub_total) . "<br>\n"; $grand_total += $rowtot; } }
-
I don't think that while() loop is even needed. The while loop is supposed to continue as long as $rowtot is less than the number of records in the $resultsIOCC['IOCC']. But, inside the while loop there is a foreach() loop on the $resultsIOCC['IOCC'] array which increments $rowtot on each iteration. The net effect is that on the first iteration of the while loop the foreach loop will increase $rowtot such that there would never be a second iteration of the while loop. Perhaps you used the while loop to have the foreach() loop only run if the array contains records. If that's the case then you just need to do a simple if() condition check. But, maybe that is not the case since I see you modify $rowtot in two places in the loop. One is a simple (plus one) at the end of the loop and then there is this line: $rowtot = $IOCC->ioccLabor + $IOCC->ioccTravel + $IOCC->ioccMileage; I suspect that might be the cause of your problem. If I am reading it all correctly, the while loop will continue as long as $rowtot < count($resultsIOCC['IOCC']). In the foreach loop you increment $rowtot by one on each iteration. So, you would think that the $rowtot would be at least equal to count($resultsIOCC['IOCC']) after the completion of the first iteration. But, you have that other line that can modify $rowtot. So, if that line above is decreasing $rowtot in some scenarios the loop could be infinite.
-
execute PHP scripts stored in MySQL table row
Psycho replied to cbassett03's topic in PHP Coding Help
Really? That seems like a terrible waste to create flat files simply for the purpose of executing them as PHP. That is what the eval() function is for. But, I was specifically avoiding suggesting eval() function because it is highly advised to NOT use it. Even the manual states that: Note: I did not add the bold text - that is exactly as it is displayed in the manual. As stated above, it should only be used when there are no other alternatives. And, in this case, there is one which I provided previously. -
execute PHP scripts stored in MySQL table row
Psycho replied to cbassett03's topic in PHP Coding Help
You can, but you really shouldn't. It is a bad idea to try and execute code within a string. Your example is obviously not real - you would just put the static string "This is a test" in the output rather than making it an echo. What I assume you are wanting to do is put PHP variables in the line such as <html><head><title>Sample</title></head><?php echo $name; ?></html> So you can define the name and then create the output dynamically. Instead, what you should do is create or find a template system. So, the content you store in the DB would be more like this <html><head><title>Sample</title></head>[[name]]</html> Then you pass the template content and the variables needed to the templating process which replaces the placeholders in the template file with the appropriate variable. -
I reversed the $maxColumns and $count variables in the modulus operation. These two lines if($maxColumns % $count == 1) if($maxColumns % $count == 0) Should be changed to this if($count % $maxColumns == 1) if($count % $maxColumns == 0)
-
Give this a try. It has the correct logic to output the results as you want them. But, may not have data if you don't find the previous error <?php // CONNECT TO THE DATABASE $mysqli = new mysqli($DB_HOST, $DB_USER, $DB_PASS, $DB_NAME); if (mysqli_connect_errno()) { printf("Connect failed: %s\n", mysqli_connect_error()); exit(); } $query = "SELECT category_name FROM `gallery_category` WHERE `photo_owner`='NameHere'"; $result = $mysqli->query($query) or die($mysqli->error.__LINE__); //Define number of columns to use $maxColumns = 10; $count = 0; //Var to count records $categoryList = ''; //Var to hold HTML output while($row = $result->fetch_assoc()) { $count++; //Increment count //Open new row if first record in row if($maxColumns % $count == 1) { $categoryList .= "<tr>\n"; } //Output current record $categoryList .= "<td>{$row['category_name']}</td>\n"; //Close row if last record in row if($maxColumns % $count == 0) { $categoryList .= "</tr>\n"; } } //Close last row if needed if($maxColumns % $count != 0) { $categoryList .= "</tr>\n"; } ?> <table> <?php echo $categoryList; ?> </table>
-
Looking over your code, I think I see the problem. And, you could have done a couple of very simple things to identify the problem yourself. Let me explain the steps I would have taken to identify the problem. You stated that you could not get the data to display. My first instinct would be that the query failed. But, you have error handling in place to know if the query failed so that is probably not it. Although I might put a step in just to echo a "Query succeeded" just to verify. After I have confirmed that the query isn't failing, my next assumption would be that no records were returned. Maybe the value 'NameHere' isn't in that field or the wrong field was used. So, I would do a simple test of echoing the count of records to the page: echo "records returned: " . $result->num_rows; If that comes back as zero, then I know the problem is the query and/or the assumed values in the DB. If the count is greater than zero I would verify that the loop is actually running on those records. Sometimes there is logic in the loop do echo this or that. Maybe the logic is faulty and the loop is running but producing no output. In those cases you could put a simple echo at the beginning of the loop to ensure that it is in fact running. In this case, however, you don't have any such logic and it will always produce output. The problem is that (aside from the values in the result set) the only thing being output in the loop is HTML tags. If the values you are trying to display are empty you would see no "apparent" output in the page. I would bet that if you did a view source in your browser you are going to see those table HTML tags. The problem is that the values you are expecting are not included in the table. And why is that? Well, it is not apparent from the code if there is a field called 'category_name' or not - so that could be the cause. If you are only using one field from the table, then specify it in the field list of the SELECT statement - don't use '*'. But, even so, that code would not do what you think it should. If it worked it would be repeating the results of each record multiple times.
-
. . . and I think you are missing the bigger point of what Jessica has told you in the past. You need to learn to debug your code. When you encounter an error, just 'staring' at your code is going to be a hit or miss activity on being able to spot the problem. Many times the problem is that a variable or return value does not contain what you think it does. That is why it is important to echo things to the page so you can verify what the values are to determine if they make sense or not.