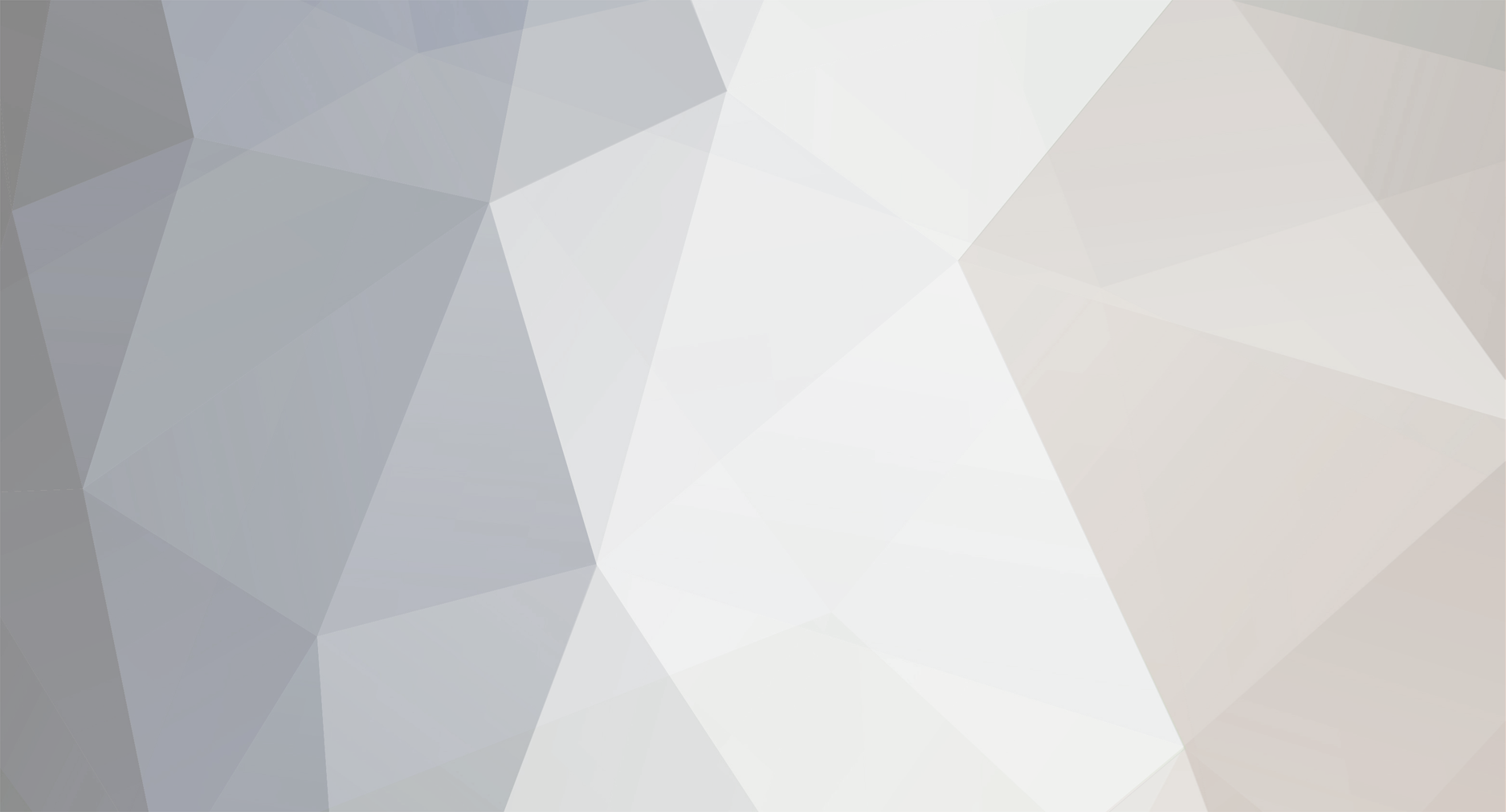
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
@scm22ri, You've provided no code from us to work with or clarified what you exact requirements are for the form validation. There are a lot of "gotchas" with respect to form validation. I would suggest you start by looking at each field and writing down what would be considered "valid" for that input. Here are some examples based upon the fields you have: Username: I would require that the field contains at least 6 characters. It would also not be unheard of to restrict that input to only certain characters, but is not required as long as you handle the display appropriately (e.g. htmlentities) and that goes for ANY input that could be used to display to the page. Email: There are many debates on validating email addresses and PHP even has a built in method nowadays. But, for this you should at least verify that the input is a properly formatted email address (I have my own function that I use) Password: At a minimum I would have a minimum length check on the password: see note below about using trim() When doing your validations you should do them in a specific order. You wouldn't check an email format before you check if it is empty. Also, always trim your input unless you have a valid reason not to. I've seen different positions on trimming password input. You also have to be careful about HOW you do the validations. You could, for example use empty() on a value, but if 0 is a valid value that function would return false. FYI: Your password fields should be of the type "password", i.e. not "text" So, here is sample fully working script based upon your fields. <?php //Create variables for submitted values (if posted) //We create tehse regardless if the form was posted to enable "sticky" values in the form $username = (isset($_POST['username'])) ? trim($_POST['username']) : ''; $email = (isset($_POST['email'])) ? trim($_POST['email']) : ''; $password = (isset($_POST['password'])) ? $_POST['password'] : ''; $confirmp = (isset($_POST['confirmp'])) ? $_POST['confirmp'] : ''; $errorMsg = ''; function validEmail($email) { $formatTest = '/^[\w!#$%&\'*+\-\/=?^`{|}~]+(\.[\w!#$%&\'*+\-\/=?^`{|}~]+)*@[a-z\d]([a-z\d-]{0,62}[a-z\d])?(\.[a-z\d]([a-z\d-]{0,62}[a-z\d])?)*\.[a-z]{2,6}$/i'; $lengthTest = '/^(.{1,64})@(.{4,255})$/'; return (preg_match($formatTest, $email) && preg_match($lengthTest, $email)); } //Only validate if form was POSTed if($_SERVER['REQUEST_METHOD'] == 'POST') { //Start validations $errors = array(); //Validate username if($username == '') { $errors[] = 'Username is required.'; } elseif(strlen($username) < 6) { $errors[] = 'Username must be at least 6 characters.'; } //Validate email if($email == '') { $errors[] = 'Email is required.'; } elseif(!validEmail($email)) { $errors[] = 'Email is not properly formatted.'; } //Validate passwords if($password == '' || $confirmp == '') { $errors[] = 'Password and confirmation are required'; } elseif(strlen($password) < 6) { $errors[] = 'Password must be at least 6 characters.'; } elseif($password != $confirmp) { $errors[] = "Password and password confirmation do not match."; } //Check if there were any validation errors if(count($errors)) { //Create error message $errorMsg .= "The following error(s) occured:\n"; $errorMsg .= "<ul>\n"; foreach($errors as $err) { $errorMsg .= "<li>{$err}</li>"; } $errorMsg .= "</ul>\n"; } else { //Form validation passed, include script to process the data echo "Validation passes. Include script to process the data.<br>"; //Redirect or load confirmation page echo "After processing the data, redirect or load confirmation page<br>"; echo "Click <a href=''>here</a> to reload form"; exit(); } } ?> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1" /> <title>Member Registration</title> </head> <body> <form action="" method="post" enctype="multipart/form-data"> <table width="600" align="center" cellpadding="4" border="0"> <tr> <th colspan="2">REGISTER AS A MEMBER</th> </tr> <tr> <td colspan="2"><font color="#FF0000"><?php echo $errorMsg; ?></font></td> </tr> <tr> <td width="163"><div align="right">User Name:</div></td> <td width="409"><input name="username" type="text" value="<?php echo $username; ?>" /></td> </tr> <tr> <td><div align="right">Email:</div></td> <td><input name="email" type="text" value="<?php echo $email; ?>"/></td> </tr> <tr> <td><div align="right">Password:</div></td> <td><input name="password" type="password" /> </tr> <tr> <td><div align="right">Confirm Password:</div></td> <td><input name="confirmp" type="password" /> </tr> <tr> <td><div align="right"></div></td> <td><input type="submit" name="Submit" value="Submit Form" /></td> </tr> </table> </form> </body> </html>
-
@Kicken, I would be very surprised if any editor looks into a function to see what the parameters are used for and then provides intellisense when using the function in code. The function could just as easily have several if() conditions for those parameters. It could be done, but since it would only be a hack solution I don't see why anyone would bother. But, maybe I'm wrong.
-
There are several applications out there that will convert those documents to HTML - but (any good ones) cost money and will require that you have rights to install the software on the server. I.e. if you are on a shared host you most likely won't be able to get it installed. You would likely needed to have a dedicated server.
-
OK, you can just modify the logic I already provided. Just create ONE array for the duplicates and use the concatenated addressid and personalnumber as the index. <?php //Test data $bestandAddrPers[] = array( "bestand" => 'file1', "addressid" => '1234', "personalnumber" => '1111'); $bestandAddrPers[] = array( "bestand" => 'file2', "addressid" => '4567', "personalnumber" => '2222'); $bestandAddrPers[] = array( "bestand" => 'file3', "addressid" => '8901', "personalnumber" => '3333'); $bestandAddrPers[] = array( "bestand" => 'file4', "addressid" => '1234', "personalnumber" => '1111'); $bestandAddrPers[] = array( "bestand" => 'file5', "addressid" => '4567', "personalnumber" => '4444'); $bestandAddrPers[] = array( "bestand" => 'file6', "addressid" => '4567', "personalnumber" => '2222'); $bestandAddrPers[] = array( "bestand" => 'file7', "addressid" => '8901', "personalnumber" => '1111'); $bestandAddrPers[] = array( "bestand" => 'file8', "addressid" => '9999', "personalnumber" => '5555'); //Create array to track duplicates $duplicateRecords = array(); //Function to remove non-duplicate values from duplicate arrays function removeNonDupes($subAry) { return count($subAry) > 1; } //Loop through all the records to add values to the duplicate arrays foreach($bestandAddrPers as $rec_idx => $record) { //Put all addr/per. numbers into duplicate arrays using value as key $duplicateRecords[$record['addressid'].'-'.$record['personalnumber']][] = $rec_idx; } //Remove all indexes in duplicate arrays that have only one value //i.e. the ones that really aren't duplicates $duplicateRecords = array_filter($duplicateRecords, 'removeNonDupes'); //The arrays above now only contain duplicates with the index being //the "values" that is duplicates and the value of the array is a //sub-array of all the record indexes that have that value duplicated // e.g. array('1234' => array('0', '2')) //Example Output echo "<h2>Files with duplicated address ID and Personal number:</h2>\n"; foreach($duplicateRecords as $dupeValues => $dupRecords) { list($addrID, $persNo) = explode('-', $dupeValues); echo "<h3>Address ID: {$addrID}, Personal No: {$persNo}</h3>\n"; echo "<ul>\n"; foreach($dupRecords as $recId) { echo "<li>" . $bestandAddrPers[$recId]['bestand'] . "</li>\n"; } echo "</ul>\n"; } ?>
-
OK, I decided to test it. there was typo. I also change $this->bestandAddrPers to just $bestandAddrPers because I was too lazy to create a class. Below is the original code with a couple minor fixes and some test data. <?php //Test data $bestandAddrPers[] = array( "bestand" => 'file1', "addressid" => '1234', "personalnumber" => '1111'); $bestandAddrPers[] = array( "bestand" => 'file2', "addressid" => '4567', "personalnumber" => '2222'); $bestandAddrPers[] = array( "bestand" => 'file3', "addressid" => '8901', "personalnumber" => '3333'); $bestandAddrPers[] = array( "bestand" => 'file4', "addressid" => '1234', "personalnumber" => '2222'); $bestandAddrPers[] = array( "bestand" => 'file5', "addressid" => '4567', "personalnumber" => '4444'); $bestandAddrPers[] = array( "bestand" => 'file6', "addressid" => '8888', "personalnumber" => '2222'); $bestandAddrPers[] = array( "bestand" => 'file7', "addressid" => '8901', "personalnumber" => '1111'); $bestandAddrPers[] = array( "bestand" => 'file8', "addressid" => '9999', "personalnumber" => '5555'); //Create arrays to track duplicates $duplicateAddressIDs = array(); $duplicatePersonalNos = array(); //Function to remove non-duplicate values from duplicate arrays function removeNonDupes($subAry) { return count($subAry) > 1; } //Loop through all the records to add values to the duplicate arrays foreach($bestandAddrPers as $rec_idx => $record) { //Put all addr/per. numbers into duplicate arrays using value as key $duplicateAddressIDs[$record['addressid']][] = $rec_idx; $duplicatePersonalNos[$record['personalnumber']][] = $rec_idx; } //Remove all indexes in duplicate arrays that have only one value //i.e. the ones that really aren't duplicates $duplicateAddressIDs = array_filter($duplicateAddressIDs, 'removeNonDupes'); $duplicatePersonalNos = array_filter($duplicatePersonalNos, 'removeNonDupes'); //The arrays above now only contain duplicates with the index being //the "values" that is duplicates and the value of the array is a //sub-array of all the record indexes that have that value duplicated // e.g. array('1234' => array('0', '2')) //Example Output echo "<h2>Duplicate Address IDs:</h2>\n"; foreach($duplicateAddressIDs as $addrValue => $dupRecords) { echo "<h3>Address: {$addrValue}</h3>\n"; echo "<ul>\n"; foreach($dupRecords as $recId) { echo "<li>" . $bestandAddrPers[$recId]['bestand'] . "</li>\n"; } echo "</ul>\n"; } echo "<h2>Duplicate Personal Numbers:\n"; foreach($duplicatePersonalNos as $noValue => $dupRecords) { echo "<h3>Personal Number: {$noValue}</h3>\n"; echo "<ul>\n"; foreach($dupRecords as $recId) { echo "<li>" . $bestandAddrPers[$recId]['bestand'] . "</li>\n"; } echo "</ul>\n"; } ?>
-
Might I first suggest giving your variables descriptive names rather than $key, $val, $key2, $val2. I think you will find it very helpful in the future. When you're in the thick of working on new code you know what those hold because you just created them. But, when you (or someone else) comes back later it is difficult to easily understand. Also, your double foreach() loop seems unnecessary since you are only referencing two values from the inner array. Or was that just an attempt? As to your request, it all depends on how you want to handle the duplicates. You might be able to do the processing in-line with what you have - or it might require you to preprocess the array first. Also, knowing how you are going to present the duplicates (i.e. the output) could affect the solution. But, if you only want to know what address Ids and personal Number have duplicates, that is easy enough. here is one possible solution (not tested, but logic should be correct) //Create arrays to track duplicates $duplicateAddressIDs = array(); $duplicatePersonalNos = array(); //Function to remove non-duplicate values from duplicate arrays function removeNonDupes($subAry) { return count($subAry) > 1; } //Loop through all the records to add values to the duplicate arrays foreach($this->bestandAddrPers as $rec_idx => $record) { //Put all addr/per. numbers into duplicate arrays using value as key $duplicateAddressIDs[$record['addressid']][] = $rec_idx; $duplicatePersonalNos[$record['personalnumber'][]] = $rec_idx; } //Remove all indexes in duplicate arrays that have only one value //i.e. the ones that really aren't duplicates $duplicateAddressIDs = array_filter($duplicateAddressIDs, 'removeNonDupes'); $duplicatePersonalNos = array_filter($duplicatePersonalNos, 'removeNonDupes'); //The arrays above now only contain duplicates with the index being //the "values" that is duplicates and the value of the array is a //sub-array of all the record indexes that have that value duplicated // e.g. array('1234' => array('0', '2')) //Example Output echo "<h1>Duplicate Address IDs:</h1><br>\n"; foreach($duplicateAddressIDs as $addrValue => $dupRecords) { echo "<h2>Address: {$addrValue}</h2><br>\n"; echo "<ul>\n"; foreach($dupRecords as $recId) { echo "<li>" . $this->bestandAddrPers[$recId]['bestand'] . "</li>\n"; } echo "</ul>\n"; } echo "<h1>Duplicate Personal Numbers:</h1><br>\n"; foreach($duplicatePersonalNos as $noValue => $dupRecords) { echo "<h2>Personal Number: {$noValue}</h2><br>\n"; echo "<ul>\n"; foreach($dupRecords as $recId) { echo "<li>" . $this->bestandAddrPers[$recId]['bestand'] . "</li>\n"; } echo "</ul>\n"; }
-
Your question is a bit confusing. If you are wanting the image to be a hyperlink to just the image then all you need to do is this. echo "<a href='{$image}'><img src='{$image}' height='200px' /></a>";
-
What? Your question really doesn't make sense the way you have stated it, but I am going to venture a guess as to what you mean. I *think* you are talking about the "intellisense" functionality that some editors have built into them. For example when I type "substr(" into my editor I get a flyout menu that shows me there are three parameters for the $string, $start index and the optional $length parameter. If that is what you are talking about, then the answer is no. There is nothing you can do to control that for others as that is controlled completely by the persons editor. Each editor is different. On some you might be able to add some configuration settings manually to make it work, while others can dynamically provide that functionality for custom functions - assuming that the function is "loaded" in the editor. And, some editors may provide the specific flags available as parameters for some built-in PHP functions, but I can't think of how they could dynamically determine that for a custom function. It would require the PHP editor to be able to read the code and 'understand' it as a human would,
-
There doesn't seem to be anything obviously wrong with that query from what I can tell, but as previously stated a good debugging tip is to echo out the query when there are problems to ensure it contains what you *think* it should. Especially when there are variables - it could be that $_POST['category'] has a typo between the field name and $category1 isn't set like you think it is. But, I'll take the debugging of queries a step further. 1. Start by running the queries directly in the DB (in this case you can use PHPMyAdmin). If you can't get them to run there with expected results, don't even think about trying to put them into code. 2. When implementing the query in your code to be created dynamically, always echo it out first to verify the contents. 3. Once you do implement the script add some logic to handle errors. Ideally you want a way to handle the errors differently in a test environment vs. a production environment. In a test environment you want the full error, but in production you don't want to expose information that could give someone information about your structure. But, for now just use an or die() clause to display the error if the query fails. $result=mysql_query($sql) or die("Query: $sql<br>Error: " . mysql_error()); If there is an error that will tell you what it is. But, what IF there wasn't an error and there were just no results? The query could be successfully run but legitimately have no results. I think that is probably the case here since you aren't getting errors from the mysql_fetch_array() function. I always like to check for empty results as well. if(!mysql_num_rows($result) { echo "There were no results found." //echo "Query: $sql"; //Uncomment this line to validate the query } else { while($row=mysql_fetch_array($result)) { //Display the results } }
-
Updating Image (With New Image) And Database Details In One Form
Psycho replied to peakymatt's topic in PHP Coding Help
Your code is extremely hard to follow, so I can't give specifics. But, one thing you stated that when no image is supplied a generic image is uploaded in it's place. Are you saying that you are creating multiple copies of that generic image for each item that does not have a custom image? If so, you can stop that. Instead do two checks: 1) Is there a defined image for the product and 2) Does that image exist. If the check for both is true, then use that image, ELSE default to a generic image - the same generic image used for any product that does not have an assigned image. As for allowing the user to change the image, I would do the following on the form. Either just above or below the image input field display the currently selected image (if there is one). That let's the user know if there is already an image or not. Then when the user submits the form do a check of the input field for the image. If the filed is empty then do nothing with that information (i.e. do not update/change the image value) - I think that is where you might have gotten hung up on. If there is a value, then use it to update the selected image for that item. The one trick you may want to implement is a means of allowing the user to REMOVE the current image without replacing it. So, instead of leaving the upload field empty (which will mean no change) you instead need to provide a different input/method to remove the image. Since you are only talking about one image per product, I would just add a "remove image" link next to the displayed current image (if there is one). That link can simply point to a page to remove the image (e.g. remove_image.php?id=id_of_item). So, when that page is called it will use the ID in the URL to remove the image assigned to that item then redirect the user back to the form. -
Then don't use the ID to apply the style properties! Every element must have a unique ID. But, you can give many elements that same class. So, modify the code to apply a class to the divs then define the class in your CSS file.
-
Is uploader_id supposed to be the user who uploaded the image or what 'object' it is associated with? If the latter, then you absolutely need a field for associating the image with the company or user. I'm not sure I understand how your "Tags" table is supposed to work. I'm *guessing* that your intent is that the type and uploader_id are the type and ID of what the image is shared with. I think that would work based upon your description. But, your field names are counter-intuitive to me based upon what I think they are supposed to mean. On second thought, you should make one change. In the images table don't use the i_type and uploader_id at all. Just put that information in the tags table - even for the original member/company the image was uploaded for. If you have a specific need to know what member/company the image was first uploaded for, then add a column in the tags table to identify that: [b]mage[/b] ------------ idimage name filename i_status [b]tags[/b] ------------ idtags idimage i_type uploader_id originator (set to 1 if this was the original entity the image was uploaded for, else 0) The way you originally had it would require two sets of JOINs - one on the uploader ID in the images table and another on the tags table
-
$api_params = array( 'api_key' => '1b6e67959f14d79c2c9c568b02c23f23', 'method' => 'flickr.photos.search', 'user_id' => '49466419@N05', 'format' => 'php_serial', ); Also, there is a comma after the last element - indicating that there should be another element in the array.
-
The issue you are having doesn't seem to have anything to do with your original question. Sounds like you are unable to get your Moodle installation working - then this is the wrong forum. This forum if for getting help with PHP code you have written. You should probably post in the Third Party Scripts forum: http://forums.phpfreaks.com/forum/26-third-party-php-scripts/
-
Then it sounds like you should be submitting the form to a different page - not the login page. You *may* have coded it to work that way, I don't really know. But, I would create a page called something like redeemVoucher.php to submit that form to. Have that page do the validation and if everything passes, set a session value and redirect to the login page. On the login page do a check for that session value, if not set redirect to the voucherForm page.
-
Don't know. We really don't know what the problem is. Could be that the cookie is being read on one page load before it is rewritten on the previous page load since you are doing this so quickly. But, I don't see why you would need to rewrite the cookie on each page load. I would only rewrite it when the user logs in - whether they logged in using a login form or using the hash. That should eliminate the problem completely. Rewriting the hash on each page load has no real benefit that I can see.
-
You want your form to POST to a login page? Are you trying to build a "pass-through" so someone can enter their login credentials on your form and be automatically logged in to that site? Could be that site is detecting that and refusing the form submission. EDIT: I just did a quick test of copying the form on that login page and submitting to it. I received the error "ADONewConnection: Unable to load database driver", so it is not detecting external form submissions. But, if the name of the form is any indication I don't think you are building a pass-through login form, so I don't see why a voucher form would be posting to a login page. It *could* be valid based upon the logic of that page, but I certainly wouldn't think that is the case if the logic of the site is separated into separate fiels as would be the norm
-
I'll add the following: First off, separating data into a separate "database" for the purposes of improving performance will likely have zero benefit (if not a degradation) if you just meant to put the data into a separate Database "instance". The term "database" is is vague because it can mean a physical database server, a database service/application, or a separate database instance on the same server/application. I doubt you are thinking about setting up a second physical (or virtual) database server and just creating another database instance on the same machine would still use the same processor, memory and other resources. Second, there are legitimate purposes for having separate database instances or even separate database servers. Separate database instances can be used to segregate information that has zero relational information. This makes it easy to manage the data in one database instance without worrying about the unrelated data. Or, the data may require specific handling that the other one doesn't. Putting data onto separate physical (or virtual) database server can be used for the purpose of creating scalability in the application. However, it would (probably) never be done with data that should relate between the two databases. But, more specific to your issue, why do you think putting the data related to your images into a separate database is needed to improve performance? Are you having a performance problem now? If so, I highly doubt that the data being in the same database is the issue. There could be many reasons for performance problems: bad DB design, bad coding practices, DB running on machine with too little resources, etc. Based upon prior experience on this board I would lean towards one (or both) of the first two problems.
- 7 replies
-
- database
- phpmyadmin
-
(and 2 more)
Tagged with:
-
That's impossible. That query is invalid. But, assuming you had the comma that is needed in there, it really is no different from the first code you posted.
-
Have you done some testing to figure out which one holds the newer value and which one holds the older value? My best guess is that the problem is with the cookie. Per the manual Perhaps the cookie is not done writing before the next page load and you are getting the old value? I wouldn't think so, but it's possible
-
Maybe it is just me, but those two scripts look exactly the same. In any event I don't see a form in the signup page. Take this ONE STEP at a time. Here is something to get you started.: 1. Create a form for the user to enter their data. 2. Create a page to receive that form input. Start by just doing a print_r($_POST) to verify that the data you expect was actually submitted. Then start building the functionality that it will use that data for. 3. Create a process to read the data file. Don't do anything with it, just output it to the page to ensure you are reading it correctly. 4. Add a process at the end of the file reading steps to add the new record to the file based upon the user data 5. Update the above process to look for matches based upon what the user entered and display those So, do one thing at a time. If you get stuck, show us what you have and explain what it IS doing and what it is SUPPOSED to be doing.
- 2 replies
-
- file write
- get
-
(and 2 more)
Tagged with:
-
I think you missed the point of what I was saying. All those checks are within an initial check to see if any required fields are missing. So, the check for comparing the email and comparing the password are within the condition that is run when required fields are empty. You would only run those checks if all the required fields have values! I would suggest not nesting separate if() conditions like that as it makes it difficult to "see" the logical flow. You need to think about all the validations that need to be performed and determine the correct order. Are there some validations that if they fail others should not be performed? Those answers will help determine the correct process. Here is one possible solution //Check for all required fields // Don't need to check email2 and password2 since those will be taken care of in the matching logic if((!$username) || (!$country) || (!$state) || (!$city) || (!$email) || (!$password) ) { //One or more required fields are missing $errorMsg = "You did not submit the following required information!<br /><br />"; if (!$username) { $errorMsg .= "--- User Name<br/>"; } if (!$country) { $errorMsg .= "--- Country<br/>"; } if (!$state) { $errorMsg .= "--- State<br/>"; } if (!$city) { $errorMsg .= "--- City<br/>"; } if (!$email) { $errorMsg .= "--- Email<br/>"; } if (!$password) { $errorMsg .= "--- Password<br/>"; } } //Check if email and passwords match if(!$errorMsg) { if ($email !== $email2) { $errorMsg .= 'ERROR: Your Email fields below do not match<br />'; } if ($password !== $password2) { $errorMsg .= 'ERROR: Your Password fields below do not match<br />'; } } //Perform username and email check if(!$errorMsg) { $sql_username_check = mysql_query("SELECT id FROM members WHERE username='$username' LIMIT 1"); $sql_email_check = mysql_query("SELECT id FROM members WHERE email='$email' LIMIT 1"); $username_check = mysql_num_rows($sql_username_check); $email_check = mysql_num_rows($sql_email_check); if ($username_check > 0) { $errorMsg .= "<u>ERROR:</u><br />Your User Name is already in use inside our system. Please try another."; } if ($email_check > 0) { $errorMsg .= "<u>ERROR:</u><br />Your Email address is already in use inside our system. Please try another."; } } if(!$errorMsg) { //All validations passed - insert the record }
-
I should have known that.
-
Not to be rude, but there is a lot a poor execution in that code. But, first let's look at the specific problem you have asked. I know that the new forums are not good about maintaining spacing in the code. So, it was hard to "see" the problem until I reformatted it. The condition to check if the passwords match is wrapped within a condition that only executes if there are missing fields (note the email check is also wrapped in that condition). So, the checks to see fi the passwords match or if the emails match will ONLY occur if there were missing fields int he post data. In other words, if all the fields have values, those check will NOT occur. If the code is properly indented based upon the structure it is pretty easy to see the problem. Some other things: 1. The ereg_ functions have been deprecated for years. You should definitely replace them with preg_ functions. 2. I would advise NEVER modifying the user input. Your regular expression could change "#password#" to the very insecure "password". Also, you are excluding certain characters such as the hyphen in data such as country, state, city, etc. That is a perfectly valid character for that type of data. Don't exclude characters without having a valid reason to do so, and when you do fail the validation and make the user fix it. Why are you restricting the input so much anyway? Even the letscapthis() function is a little overboard. Since you are apparently allowing input for other countries forcing the data to ucwords() is not appropriate. 3. You don't trim() anything. So, for some fields just spaces would be treated as valid input. There are other things as well, but that is what jumped out at me
-
$parsed = parse_url($image); $host_parts = explode('.', $parsed['host']); $domain = implode('.', array_slice($host_parts, count($host_parts)-2));