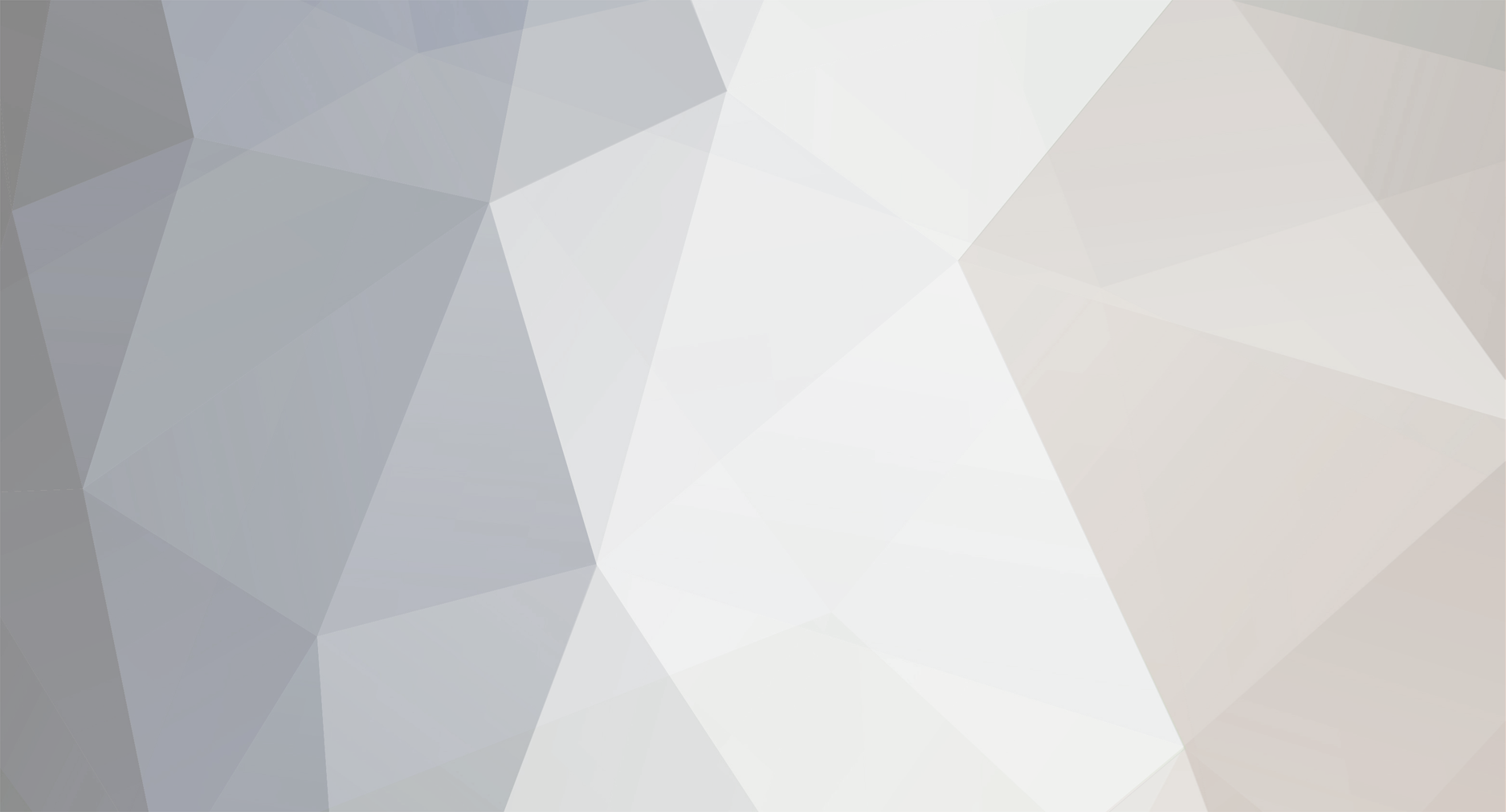
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
I completely agreed with ChristianF's original response. But, if you are required to send the data in three separate variables, then that is what you must do. But, if you are storing this data in the database you should absolutely be storing it as a valid date value and not separate values. Only separate into the components at the time that you need to. ChristianF's solution is the one I would go with if you are taking the submitted value directly and parsing it out. list($month, $day, $year) = explode('\', $_POST['CheckIn']);
-
Well, you never defined $pdf, so it's not going to include the URL parameter in that string. You said the $_GET global is working - so I'm confused as to why you are not using that.
-
It shouldn't take that long. Simply do a search for the + symbol in your code. If it is used for adding two number then replace with bcadd. Then do the same for the subtraction symbol and so on. I can't see that it would take that long. Also, since most of the BC function only work on two numbers, if you are adding, subtracting, multiplying multiple numbers in your code you might want to create some functions to handle multiple numbers: function addAll($arrayOfNumbers) { $total = array_shift($arrayOfNumbers); foreach($arrayOfNumbers as $number) { $total = bcadd($total, $number); } return $total; }
-
I wasn't disagreeing with anything you said, I was agreeing with a specific emphasis on queries - and he is running queries in loops as I stated above. He is running through an xml file and on each record he is calling the create() method which in turn calls the insert() method (which does a DB insert). I have to admit that I am not fully up to speed on the mysqli extension yet, but I'm pretty sure you can define a prepared statement and then run that prepared statement many times without the same performance issue as you would with running individual queries with mysql extension. What I also notice in the code is that for each line in the xml file the code is rebuilding the prepared statement. You should just be able to create the prepared statement on the first record and then just pass the new data to the prepared statement on each successive record. Rebuilding the prepared statement each time would destroy the benefit of creating a prepared statement in the first place. So, create a private property for the class to be the prepared statement (set default to false) then int the insert method check if the prepared statement exists - if not, create it. Then it would only be created once. I also see you are closing the db connection after each insert. I didn't really walk through all of the processes, but you should only open the DB connection when starting the processing of the XML file and close it when done.
-
Never, ever run queries in loops. It is one of the worst things you can do with regard to performance. In 80% of cases there is a way to accomplish the same thing without a loop, in another 19% of cases you have probably built your database schema incorrectly and in the remaining 1% of cases you are just doing something stupid. Of course, those percentages can change based upon the skillset of the programmer. In your code above you have a foreach() that calls the create method, which in turn calls the insert method that does a DB insert.. You need to change it so it is generating a single query with ALL the records to be added. Then execute the query one time.
-
Regular Expression is a great tool, but it should only be used when there are no alternatives. Forcing the value to a float would work, but it means you would potentially be changing the value from what the user entered. If the user accidentally entered "1.2.3" and really meant to enter "1.23", then forcing the value to a float would end up with "1.2". I would rather capture the error and send back to the user to correct.
-
Text input fields pass the data as "text". In fact, I think all POST data is passed as text except for maybe file uploads. So, when you use if (is_float($_POST['prod_price'])) $_POST['prod_price'] is always a string - even if the string is '1.23'. Although PHP is a loosely typed language and you could use the string '1.23' in a match function, the is_float() function is specifically checking if the element has the value of a float AND is of a numeric type. is_float() I think you want to be using is_numeric()
-
This topic has been moved to PHP Regex. http://forums.phpfreaks.com/index.php?topic=364308.0
-
Preformance of multiple designs in if...else statement
Psycho replied to MargateSteve's topic in PHP Coding Help
EDIT: ChristianF beat me too it with basically the same idea, but I had included some additional details that might be of value. What you are really talking about is different content not really styles (but styles would be one potential way to achieve that results). Without knowing how the multiple options will display the content it is really impossible if stylesheets would even work. It most likely can, but would require a LOT of pre-planning and detailed design. It would be very easy to create output into say a list that you can easily format for one output, but then find that it doesn't work for a different output. But, I have seen this done. allmusic.com is one that I know of. At the bottom of an artist or album page there is typically a list of albums, tracks, etc. The content is set up so you can switch from a condensed to list view and it all appears to be driven by styles that are hidden/unhidden. That works well for that type of application because they are both lists, just displayed a little different. But, if the way you will display some data will be functionally different that will be harder to do. Plus, if the mobile will be a stripped down version why make that user wait for all the content to download if it is only going to be hidden? A good approach would be to simply separate the logic into three parts 1. Create the templates that will contain the output. These would be just wire-frames with placeholders for the content where you will include() the necessary content file. So, the mobile template might only have one or two content placeholders while the high-def may have many. 2. Create the scripts to create the different contents you need. If you have multiple output modes that will use the same "basic" content for one of the sections just create that script to make the changes while it is generating the content. Ex: $query = "SELECT name, email, phone, address FROM user"; $result = mysql_query($query); while($row=mysql_fetch_assoc($result)) { echo "<div>\n"; //Show name and email for all versions echo "Name: {$row['name']}<br>\n"; echo "Email: {$row['email']}<br>\n"; if($display_mode == 'low_def' || $display_mode == 'high_def') { echo "Phone: {$row['phone']}<br>\n"; } if($display_mode == 'high_def') { echo "Address: {$row['address']}<br>\n"; } echo "</div>\n"; } That's probably an oversimplification. Of course you could just create three different scripts for each display option for the same data. But, this would be easier to maintain. If you were to ever change database schema you would only have to change the data access in one place. -
unlink to delete 1 or more files and not ALL files !!
Psycho replied to Skylight_lady's topic in PHP Coding Help
If you have a query that is selecting the files you want to delete, then just iterate over the results of the query and delete those files. //Query the file name for those to be deleted $query = "SELECT file_name FROM files_uploaded WHERE file_id = 'delete'"; $result = mysql_query($query); //Set path to the files if not included in the DB results $path = '/home/www/mydir/files-uploaded/'; //Loop through the results and delete teh files while($row = mysql_fetch_assoc($result)) { $file = $address . $row['file_name']; if (is_file($file)) {unlink($file); } } Just replace "file_name" in the two places above with the appropriate field name in your table. -
preventing PHP scripts from being tiggered from outside websites
Psycho replied to cbassett03's topic in PHP Coding Help
If you are talking about files that are only include(ed) in other files and not the ones that the user accesses via the browser the best solution, IMO, is to put those files outside/above the web root. The it is impossible for anyone to access the file directly. -
Preformance of multiple designs in if...else statement
Psycho replied to MargateSteve's topic in PHP Coding Help
@ialsoagree, No one here is going to agree with you. I think I can speak for everyone that what you are suggesting is a hack solution. Just because you can do something doesn't mean you should. The primary purpose of PHP is the ability to build dynamically driven websites. A typical page can perform hundreds, even thousands, of operations to display a single page. Having a simple piece of logic to include a link for the style sheet as per the HTML standards is trivial. When someone points out a potential problem you go off and find some workaround that would solve it. That's great. But here's the thing. The PHP solution is simple and does not require any workarounds or added complexity to work. Simply set the style sheet link in the header using an if/else or switch statement. No need to preload files and it will have vastly wider support on all devices and browsers. Maybe there is a break in how we are all understanding the issue. For me, giving the user the ability to switch the stylesheet is something the user will do one time and then should propagate to all subsequent page loads. Having that change happen instantaneously on the page which they select it is not something that adds a lot of value in my opinion. But, if you still feel your proposal is best I would post the following challenge to you. Find an existing site that uses JavaScript for such a thing. A real site mind you, not some proof of concept that shows it can be done. I know I can find many that set the style sheet on the server-side. -
Preformance of multiple designs in if...else statement
Psycho replied to MargateSteve's topic in PHP Coding Help
Not even close. JavaScript is a programming language. It's primary purpose is to enhance the user experience with features/functionality that a static page cannot. Sometimes that involves changing HTML content or Styles. If JavaScript is the main method of setting the styles for a page then CSS wouldn't exist. CSS is the primary/best method of setting the styles for a site. What you are suggesting is replacing stylesheets with JS. It takes about 1 billionth of a second to change the stylesheet on the server. if($platform=='mobile') { echo '<link rel="stylesheet" type="text/css" href="http://domain.com/styles/mobile.css" />' } else { echo '<link rel="stylesheet" type="text/css" href="http://domain.com/styles/desktop.css" />' } Then there's the problem with maintainability. When you need to make a change to the style you can just open the stylesheet(s) and make your changes the the appropriate classes. If you do that in JavaScript it would be much harder. It also goes against the philosophy of separating business logic from the content. -
Preformance of multiple designs in if...else statement
Psycho replied to MargateSteve's topic in PHP Coding Help
JavaScript? Seems like a less than optimal solution. Just have the PHP code define the appropriate stylesheet/images to be used and let the browser handle it normally when it renders the HTML. You should rethink that approach. When generating for mobile devices you want to keep the size of the content to a bare minimum. Mobile devices do not have as high a bandwidth as a desktop would and people have caps on their data limits. Here is the approach I would take. 1. Create "templates" for each display mode. The template is just the basic layout with placeholders for the various content. 2. Create code for each individual block of content. 3. Once you've determined the correct display, use the correct template that will then call the appropriate code to create the content. If you have two blocks of content that are only slightly different between platforms, you can create one set of logic and have it create the content differently based upon the platform. -
Um, either you stated what you meant backwards or that statement is incorrect. You can reference an index of a string or a constant in curly braces and you can use a constant outside of a string. So, not sure what you meant to state echo $foo['bar']; //Use string index only echo $foo[bar]; //Try constant, if not use string - will issue Notice echo "$foo[bar]"; //Use string index only echo "$foo['bar']"; //Invalid echo "{$foo[bar]}"; //Try constant, if not use string - will issue Notice echo "{$foo['bar']}"; //Use string index only
-
Then how would you expect fgets() to help you? Per the manual it gets 1 line of data. If you have no line breaks then it would, by default, get the entire file content since there are no line breaks. You can specify a length parameter to read the line/file in chunks, but then you're back to the problem of the scenario where the text you are searching for could be split between chunks. I can think of some elaborate means to overcome that, but I'm sure there is a more efficient method. if you were to describe the format of the file (and why you must compress the data) and what the search value would typically look like we might be able to provide some actual help. As of right now this is all hypothetical.
-
http://www.php.net/manual/en/language.types.array.php Scroll down to the section entitled appropriately enough Array do's and don'ts EDIT: Ok, I guess I missed the part further down where it does state that using an unquoted string for the index is OK only when it is inside a double quoted string (I assume for heredoc too). I'll still use the quotes around the index and put the entire array within curly braces. echo "This is {$foo['bar']}"; That way, when moving variables around that may or may not be ins strings I know that are still proper.
-
@ialsoagree, You are correct about being able to parse variables within double quoted strings, but the code you provided above is specifically discouraged. If the array key is a string, it should always be defined within quotes such as $row['leave_status'] and not $row[leave_status] When you leave out the quotes, PHP tries to parse that as a constant value. When none is found it then uses that value as a string. Although ti works, it is poor coding practice and it could very easily be disabled in future versions since it is really incorrect. From the PHP manual: The correct method to include an array with a string index in a double quoted string is to use curly braces: echo "<td><a href='admin_decline.php?status={$row['leave_status']}' onClick='return confirm('Are you sure to delete this news?')'>Decline</a> <a href='admin_approve.php?status={$row['leave_status']}' onClick='return confirm('Are you sure to delete this news?')'>Approve</a></td>"; @haruya, Please read my first post and follow those directions. Your problem has nothing to do with PHP except for the fact that you are using PHP to generate output. The problem is that you are generating output that is invalid HTML. I'm not going to tell you why because the problem could be found with the simplest of debugging, so I want you to learn how to do it. As for whether you should perform the validation within the onclick trigger or not is not the problem. But, most would agree that you should write that logic outside the actual trigger. QUESTION: Why do you have a confirmation for the approve link asking the user if they want to Delete?
-
Take a look at the actual HTML source code produced from that line and the problem will be obvious.
-
Yes it is, but I've been through a number of security audits with companies that use our software who are storing their customers' financial data. They would rather a user user be inconvenienced than expose the data. It's all a matter of how sensitive the data is and to what lengths you feel are necessary to safeguard it.
-
Retrieve field with highest value from a MySQL database using PHP
Psycho replied to twebman84's topic in PHP Coding Help
Although Brands suggestion is the right one for a properly normalized structure, if this is the only situation where you need to compare data across these fields, you could just do the logic in PHP. Just query all the columns and find the max for each record accordingly. EDIT: Just realized there might be a slightly more efficient method. When processing each record: Strip out the username using array_shift(), then get the max() value from the rest of the array, lastly get the position based upon that max value. $query = "SELECT * FROM appearances"; $result = mysql_query($query); while($row = mysql_fetch_assoc($result)) { $playerID = array_shift($row); //User ID must be 1st value in list $top_count = max($row); $top_position = array_seach($top_count); echo "Player ID: {$playerID}, Top Position: {$top_position}, Times Played: {$top_count}<br>\n"; } -
Reread my previous response - the code I provided would generate a query that does just that. Now, had I used OR instead of AND, the query would match any record where one or more of the words were included. But, I did use AND, so the query will only match records were every word in the search string appears in the record.
-
If you don't want FULL TEXT searching, then a better solution is to simply explode the search string into separate words and create multiple LIKE clauses. Since these are product names you can just look for results where all the words are included and not worry about the order at all. So, your query would look something like this SELECT * FROM table_name WHERE field_name LIKE '%word1%' AND field_name LIKE '%word2%' AND field_name LIKE '%word3%' AND field_name LIKE '%word4%' Here's one way to code that: $search_string = trim($_POST['search']); $search_words = array_filter(explode(' ', $search_string)); foreach($search_words as $idx => $word) { $search_words[$idx] = "field_name LIKE '" . mysql_real_escape_string($word) "'"; } $query = "SELECT * FROM table_name WHERE " . implode(' AND ', $search_words);
-
I prefer the throttling method. Keep track of the last failed log-in time, and don't allow another attempt until 1 second has elapsed. Rather than an attacker just sending 4 bad requests to get a user locked out for 15 minutes, the attacker has to constantly be sending bad requests to lock out the user. Combine this with your step 3, and it becomes very easy to weed out those kind of goofs. Only allowing 1 log-in attempt per second is enough to destroy most brute-force attacks, leaving you only open to a slow dictionary attack. These can be defeated by keeping a list of the most common 100,000 passwords or so, and not allowing them (http://download.openwall.net/pub/wordlists/). #2 Isn't primarily directed at scripted/automated attacks. It is designed to prevent a person (external or internal) from trying to get into someone else's account This is pretty standard in the industry. A coworker could try and get into a person's account using known information such as anniversary date, kids names, etc. After three attempts the account would be locked (while also logging the offending IP address).
-
If I am understanding you correctly, then what you really need is to implement FULL TEXT searching instead. What you are trying to do is inefficient and is basically trying to recreate functionality already built into MySQL.