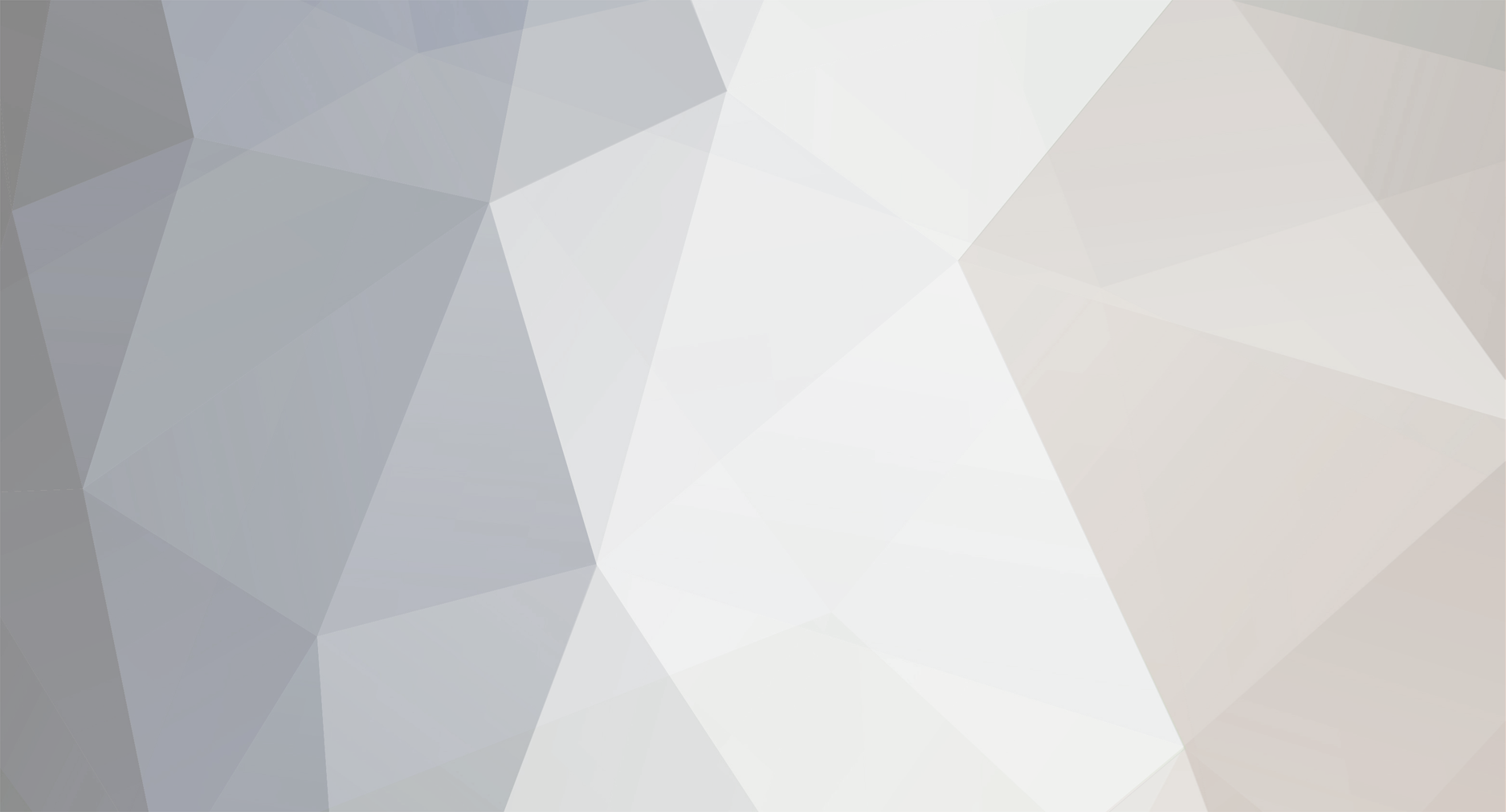
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
I don't know what I was thinking. I followed the post about using str_replace() and totally blew it on recognizing that preg_replace() was being used. BUt, now that I think about it, the square brackets should not have worked as delimiters. The delimiter is supposed to be the same character. A left and right bracket are different characters. Odd.
-
if you have the [] as part of the text to find it shouldn't retain those characters when it is replaced. Show the part of the text that contains '[date]' as well as what the value of $showDateS is.
-
How to populate a table elsewhere on the page.
Psycho replied to rantehoggen's topic in PHP Coding Help
No clue. The functions you describe are obviously custom functions so we can't really tell you how to modify them for your needs. But, this sounds like this may really be a JavaScript question. Can you provide some of the relevant code? -
Now, was that so hard to provide the data example? Here you go. The code at the bottom will extract the data you want into an array with named indexes. It is a fully functioning script. Go ahead and verify it does what you need,then incorporate the relevant parts into your existing script. Here is the output array generated using the sample data you provided: Array ( [sDX] => 6052 [TEL] => 5207426818 [OPSYS] => Windows 7 Home Premium x64 Edition 6.1 (build: 7601) Service Pack 1 [Physical Memory Size] => 3.47 GB [C:\] => 441.51 GB [Physical Address] => : XX-XX-XX-XX-XX-XX ) <?php if(isset($_POST['data'])) { $params = array('SDX', 'TEL', 'OPSYS', 'Physical Memory Size', 'C:\\', 'Physical Address'); $result = array(); //Ouput array $lines = explode("\n", $_POST['data']); foreach($lines as $line) { foreach($params as $param) { if(strpos($line, $param)===0) { //Get the value $value = trim(substr($line, strlen($param))); //Add special handling as needed for some values if($param=='C:\\') { $value = substr($value, 0, strpos($value, 'GB')+2); } //Add value to result array $result[$param] = $value; //Remove found key unset($params[array_search($param, $params)]); } } } } ?> <html> <head></head> <body> <pre><?php if(count($result)) { print_r($result); } ?></pre> <form action="" method="post"> Paste Data:<br> <textarea name="data" style="width:800px;height:400px"></textarea><br> <button type="submit">Submit</button> </form> </body> </html>
-
Regular expressions are typically slow compared to other options. String functions *could* be the best solution here. @refiking You need to provide an example of what the input looks like for us to even begin to provide a possible solution. Are there line breaks between each proposed variable? Can the values have spaces and/or line breaks? Too many possibilities to even begin to suggest something.
-
A quote of mine from the post in question If there was a built-in function for everything it would be impossible to find what you needed - even with a manual. Besides, what fun would programming be if all you were doing was looking up functions instead of really creating something that takes skill. Some people are simply not built to be programmers. But, that's OK, I suck at design.
-
//Function to return a string ordered alphanumericaly function orderByDigits($strValue) { $arrValue = str_split($strValue); sort($arrValue); return implode('', $arrValue); } //Check for exact or partial matches if(in_array($winning_number, $tickets)) { //One or more tickets matched EXACTLY the numbers AND positions } else { //Convert winning number and tickets into ordered values $ordered_winning_number = orderByDigits($strValue); $ordered_tickets = array_map('orderByDigits', $tickets); //Check if any tickets matched all the digits if(in_array($ordered_winning_number, $ordered_tickets)) { //One or more tickets matched all the numbers } else { //None of the tickets matched all the numbers } }
-
No, that will not work. Programming takes analytical thought. If you cannot or will not do that, then hire someone to write the code for you. Or, at least provide some context of what you are trying to achieve. Otherwise, you just piss off the people trying to help you because you come up with the solution you *think* you need and ask for that. But, then you end up getting that solution (which is incorrect) and just waste everyone's time. If the winning number is '1212' and a user's pick is '1234' the process - you asked for - will find that the user matched ALL the numbers! And, if you had simply stated what you were trying to achieve a real solution could have been provided.
-
And why would you expect a function to do something it was not designed to do because YOU have a particular need? There are functions to do "key" things and then it's up to the programmer to put on his thinking cap and write some logical code. As to my function, it works perfectly fine. I specifically stated that the function should work similar to in_array(). So, you should use it the same. The function will return the INDEX of the found value. That index could be a '0' which will resolve to FALSE if a loose comparison is done! If you do not do an IDENTICAL (comparison) and the returned index is 0 you will be running the wrong logic. Your responses in this thread and another one today are showing a lack of attention to detail that is making it frustrating to even provide help to you. if (str_in_array('1', $tickets) !== false) { echo "Yes I found a match"; }
-
Really?! You must be using some type of "new" math. Sure it does, but I could write an even more complicated solution that takes hundreds of lines to produce the same results. Kicken provided the solution you should be using.
-
Going in and out of PHP/HTML makes your code messy and difficult to debug. If you structure the code better these types of problems are easier to solve. Second, don't use an onclick in an option tag. Use an onchange for the select element. Because you don't have to "Click" an option to select it. You can use your keyboard to make a selection. If you only want the form to submit if a value other than the first option is selected, then have the onchange call a function that makes that determination. In fact, I would suggest you should always call a function instead of putting login into the trigger. Also, using MYSQL_NUM is kinda bad in my opinion. If you added a data abstraction layer you would have to potentially rewrite a lot of code. It could even expose sensitive information. Better to use the explicit field name in my opinion. Lastly, there is no "NULL" within HTML form values - use an empty string. As for your original problem I see no error handling for the query. So, logically either the query is failing or it might not be returning any results. But, I can see that it would be failing due to this "ORDERBY". I've cleaned up the code quite a bit, but have not tested it since I don't have your DB. <?php //Set selected make $selectedMake = (isset($_POST['make'])) ? $_POST['make'] : false; //Connect to DB $con = mysql_connect("localhost", "root", "letmein"); mysql_select_db("car", $con); //Create Make options $query = "SELECT makeID, car_make FROM make ORDER BY makeID ASC"; $result = mysql_query($query) or die(mysql_error()); $makeOptions = ''; while($row = mysql_fetch_assoc($result)) { $selected = ($selectedMake === $row['engineID']) ? ' selected="selected"' : ''; $makeOptions .= "<option value='{$row['makeID']}'{$selected}>{$row['car_make']}</option>\n" } //Create form for Engine selection (if make was selected) $engineForm = ''; if($selectedMake !== false) { $query = "SELECT engineID, engine FROM engine ORDER BY engineID ASC"; $result = mysql_query($query) or die(mysql_error()); $engineForm .= "<form name='form2' action='' method='post'>\n"; $engineForm .= "<select name=\"engine\" onchange='if(this.selectedIndex!=0) {this.form.submit();};'>\n"; $engineForm .= "<option value=''>Select Engine</option>\n"; while($row = mysql_fetch_assoc($result)) { $makeOptions .= "<option value='{$row['engineID']}' {$selected}>{$row['engine']}</option>\n" } $engineForm .= "</select>\n"; $engineForm .= "</form>\n"; } ?> <html> <head> </head> <body <!--I left the action blank to refresh itself--> <form name="form" method="post" action=""> <select name="make" style="background-color: #008000; color:#FFFFFF;" onchange="if(this.selectedIndex!=0) {this.form.submit();};"> <option value="">Select Make</option> <?php echo $makeOptions; ?> </select> </form> <?php echo $engineForm; ?> </body> </html> Edit: Updated onchange event to only trigger when NOT the first option is selected. But, I still think you shoudl call a function - I was just too lazy to do so.
-
Here you go. This function will work very similar to in_array() but it will work on a partial string match. function str_in_array($needle, $array, $caseSensitive=false) { $needle = (string) $needle; foreach($array as $index => $haystack) { $haystack = (string) $haystack; if($caseSensitive) { if(stripos($haystack, $needle)!==false) { return $index; } } else { if(strpos($haystack, $needle)!==false) { return $index; } } } return false; } EDIT: Revised function to typecast values as strings.
-
@monkuar You really aren't making any sense. You state and then state you will use which will result in values < 1000 I think kicken correctly deduced that what you REALLY want is a number between 0 and 9999 which is always padded to four digits. So, the number 41 will be 0041. If that is what you want, then the solution he provided is what you should probably use. The more time you take into specifying your requirements as accurately and completely as possible will greatly increase your chances of getting the correct answer the first time.
-
Assuming you set them up to have the same total min and the same total max there is no difference in "randomness" Using rand(0, 9999); there is a 1 in 10,000 chance of any possible option. Using rand(0,9).rand(0,9).rand(0,9).rand(0,9) there is a 1 in 10 chance for any specific digit. 10 x 10 x 10 x 10 = 10,000
-
No, they are not the same rand(1000 , 9999) will produce a number between 1,000 and 9,999. While rand(0,9).rand(0,9).rand(0,9).rand(0,9) will produce a number between 0 and 9,999. You could, of course, change the first call in that series to be rand(1, 9) to get the same results. but, that second example is just ridiculous IMO.
-
Why, is there any disadvantages. Additionaly this is not a real project I am doing this for just learning. And this, creating table issue is not going to be busy because it is an admin panel, I am just trying to make admin panel more user friendly. If this is for learning purposes,then you should use it as an opportunity to learn the right way. Creating different tables to store the same type of data is inefficient and does not scale. Instead you just need to add another column to the table to identify the country. Here are a few example of why what you are doing is a bad idea: 1. If you need to pull records from multiple countries if only for gathering metrics or summary data, you would have to query each table individually or build up a massive query using UNION. 2. If you need to JOIN data between multiple tables you would have to put in variables for that table name. It makes the code harder to read and, more importantly, debug when there are issues. So, instead of a query such as SELECT * FROM {$country_name}_ip WHERE foo = 'bar' You would instead write SELECT * FROM ip WHERE foo = 'bar' AND country = '{$country_name}'
-
This topic has been moved to PHP Freelancing. http://forums.phpfreaks.com/index.php?topic=365280.0
-
Showing multiple order/orderdetails on single page
Psycho replied to skinnyguy76's topic in PHP Coding Help
I had started to write some code, but your current output wouldn't work. If you are outputting this into a table then you need to have the same number of columns. You have 4 fields in the header rows and only two for the data. I had no clue how they should be structured so I didn't take any time to provide anything. -
Why are you posting in a thread that is over a year old? You could start a new thread and reference this one if applicable. Anyway, your problem is exactly the same as the OP in this thread. You stated you used the same solution, but I don't see that you have. The problem is that you are using a POST value to determine the criteria to use for filtering the content. But, when th euser selects to go to another page there will not be any POST value. So, you need to store the POSTed search criteria into a SESSION or in some manner. But, looking at your code there are other problems as well. Such as this $sql = "select date,mobno,city,state,type,telecaller FROM import WHERE time IN($option1)GROUP BY mobno,telecaller ORDER BY date DESC"; $pages_query= mysql_query($sql); $pages=ceil(mysql_result($pages_query, 0)/$per_page); You are running a query to return a set of records matching the criteria and using the first value from the first record in determining the number of pages?! You need to get a COUNT() of the records matching the criteria. I stopped looking at the code from that point on. You should find a tutorial on pagination and work through it to understand the process. Then use it to implement as needed for your use.
-
Very simple script randomly stopped working and won't work again...
Psycho replied to acidpunk's topic in PHP Coding Help
You seem to be contradicting yourself. If there was an error - what was it? We are not mind readers so please share with us any pertinent information. By the way I just noticed in your queries that you are trying to do math with strings timeleft=timeleft-'1' WHERE timeleft<='0' I know MySQL does do type conversion, but still, you should use numbers in mathematical statements. -
Very simple script randomly stopped working and won't work again...
Psycho replied to acidpunk's topic in PHP Coding Help
Have you tried even the most basic of debugging? There is no way for us to tell you why it is not running. You should run the same code directly and see what errors are returned $query = "UPDATE dead_mobs SET timeleft=timeleft-'1'"; echo "Running Query: {$query}<br>\n": $result = mysql_query($query); if(!$result) { echo "Error: " . mysql_error(); } else { echo "Affected Rows: " . mysql_affected_rows(); } $query = "DELETE FROM dead_mobs WHERE timeleft<='0'"; echo "<br><br>Running Query: {$query}<br>\n": $result = mysql_query($query); if(!$result) { echo "Error: " . mysql_error(); } else { echo "Affected Rows: " . mysql_affected_rows(); } However, looking at your queries, I think you are simply taking the wrong approach. You should not need to delete records based upon timeleft < 0. Your queries for extracting records you want should simply ignore records where timeleft < 0. And, reducing timeleft by -1 may also not be needed. -
Showing multiple order/orderdetails on single page
Psycho replied to skinnyguy76's topic in PHP Coding Help
I highly suggest you get into the habit of separating the logic (i.e. core PHP) and the presentation (i.e. the HTML output). It makes it much easier to maintain and enhance your code. In fact, I actually separate my PHP code and output code into separate files. So, what I suggest is in the loop for generating the order data, you add the output to a variable. Then the only PHP code you need in the presentation (i.e. HTML) are only simple echo statements. -
Check is the input value is a double (float) value
Psycho replied to haris244808's topic in PHP Coding Help
haris244808, Have you ever heard about the saying A big part of this forum, IMO, is not just providing an answer but providing information that helps users to understand how to think differently about problems they face to understand how to solve problems on their own in the future. You have been given the solution you need. It just wasn't spoon fed to you. Also, every integer is a float, so in addition to the first solution ManiacDan provided you need to pay attention to his last post as well. -
Showing multiple order/orderdetails on single page
Psycho replied to skinnyguy76's topic in PHP Coding Help
OK, I looked closer at the code so I could provide an example and am seeing something else that should be changed The logic on the WHERE clause is confusing. It looks like the logic is to select records where the OrderID = the order ID passed in the $_GET parameter OR if no ID is sent then use the orderID in the session. You made that logic way more complicated than it needs to be. You could have just had a single parameter in the where clause such as WHERE OrderID = $orderID and then define $orderID using //Set order ID if(isset($_GET['OrderID'])) { $orderID = intval($_GET['OrderID']); } elseif(isset($_SESSION['WADA_Insert_orders'])) { $orderID = intval($_SESSION['WADA_Insert_orders']); } So, if the order ID is set in the GET array it uses that value, else it uses the session value. But, that would only work if the session value is always set. Your current code doesn't account for the GET var and the SESSION var not being set. I've solved that below as well. <?php //Set order ID if(isset($_GET['OrderID'])) { $orderID = intval($_GET['OrderID']); } elseif(isset($_SESSION['WADA_Insert_orders'])) { $orderID = intval($_SESSION['WADA_Insert_orders']); } //Check that an Order ID was set from GET or SESSION if(!isset($orderID)) { die('Unable to get records. No Order ID available.'); } mysql_select_db($database_adventcms, $adventcms); //Create and run ONE query to get all the data needed $query = "SELECT o.OrderID, o.OrderBusiness, o.OrderUserID, o.OrderDate, o.OrderShipped, o.OrderAmount, o.OrderShipName, o.OrderShipAddress, o.OrderShipAddress2, o.OrderCity, o.OrderState, o.OrderZip, o.OrderCountry, o.OrderPhone, o.OrderFax, o.OrderShipping, o.OrderTax, o.OrderEmail, o.OrderTrackingNumber. od.* FROM orders AS o LEFT JOIN orderdetails as od ON od.orderdetails = o.OrderID WHERE o.OrderID = {$orderID} ORDER BY o.OrderID"; $result = mysql_query($query, $adventcms) or die(mysql_error()); if(!mysql_num_rows($result)) { echo "No records found"; } else { //Create flag to track change in Orders $lastOrderID = false; while($row = mysql_fetch_assoc($result)) { //Check if the order is different from last if($lastOrderID !== $row['OrderID']) { //This order is different from last. //Display the order info ##INSERT CODE HERE TO DISPLAY ORDER INFO //Set flag so additional details of this order //don't display the order info again $lastOrderID = $row['OrderID']; } //Display the order details ##INSERT CODE HERE TO DISPLAY ORDER DETAILS } } ?>