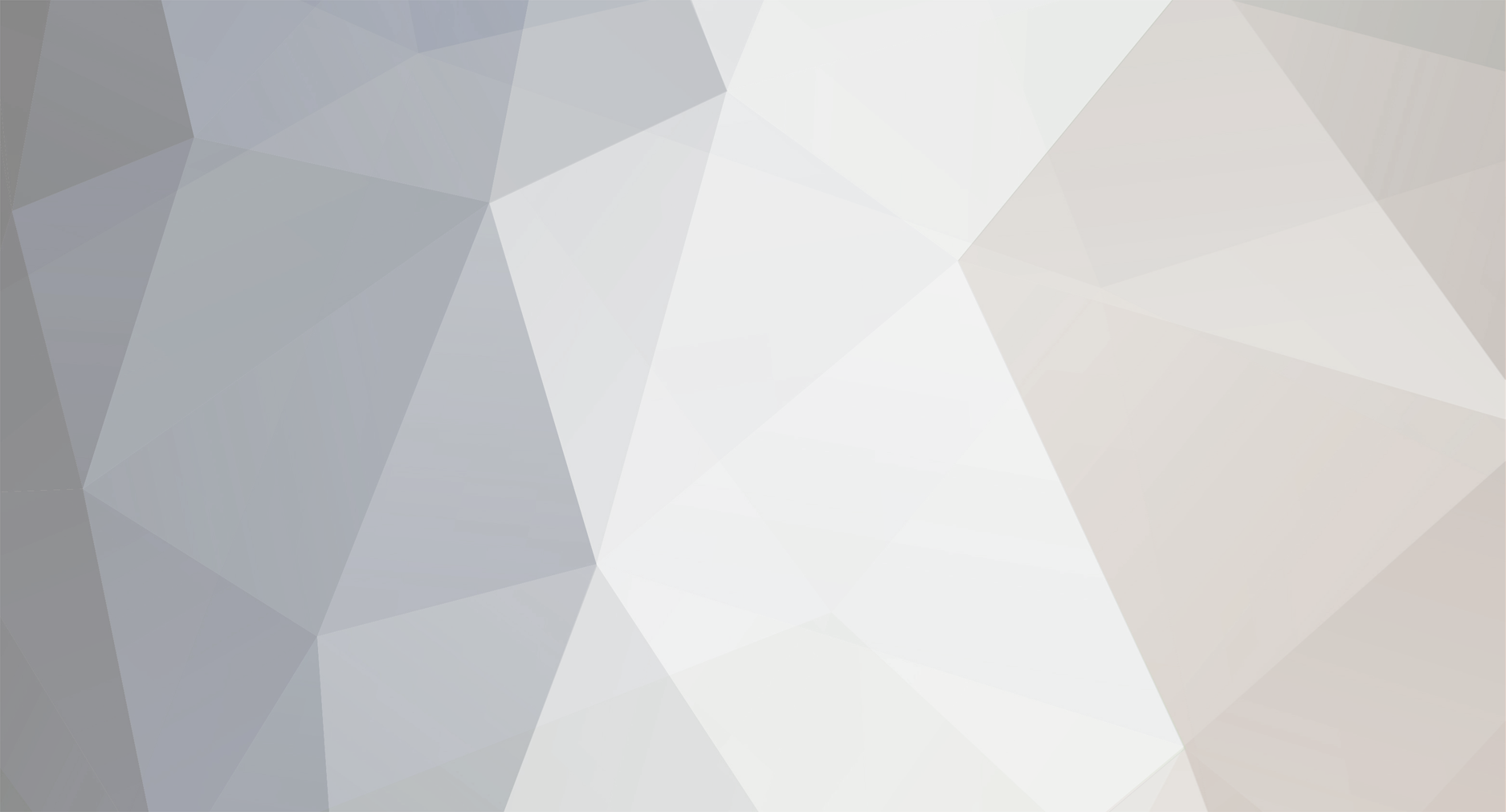
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Showing multiple order/orderdetails on single page
Psycho replied to skinnyguy76's topic in PHP Coding Help
@spiderwell: Not to be rude, but that is terrible advice. You should never run queries in loops, they are terribly inefficient. @skinnyguy76: This is a common pattern that is easily solved. The solution is actually very simple. But, almost everyone has to be shown the first time. What you want to do is query ALL the records you need. Typically this requires a JOIN query. That will result in some data being repeated in the results. In this case you will have the order info repeated for every item in the order details. But, that's OK. The trick is to simply set a flag variable to track the last parent record (order info). Then iterate through all the records to display the child records (order details). The trick is to check if the current parent (order info) is different from the last one - if so, show the parent info. -
Check is the input value is a double (float) value
Psycho replied to haris244808's topic in PHP Coding Help
Please reread ManiacDan's response. Don't just skim over it - really read it. And pay particular attention to the code examples. -
Why do you feel you need to use a hidden form field at all? You already have the user ID stored in a session variable. Just check that the user is logged in and use the session value. I see nothing gained from using a hidden form field. It only adds unnecessary overhead, IMO.
-
Your query is failing. You need to verify that the query passed before you try to use the results. I would advise always creating your queries as string variables. Then if there is an error you can echo the query to the page to verify the contents. If you had done that in this instance the error would have been obvious. In the query you had an opening single quote mark around the username, but no closing single quote mark. There were a lot of other potential problems $username = trim($_POST['username']); $password = trim($_POST['password']); if ($username!='' && $password!='') { $connect = mysql_connect("localhost","root","") or die("Couldn't connect!"); mysql_select_db("phplogin") or die("Couldn't find db"); $query = sprintf("SELECT * FROM users WHERE username='%s' AND password='%s'", mysql_real_escape_string($username), mysql_real_escape_string($password)); $result = mysql_query($query) or die(mysql_error()); if(!$result) { die("Query: $query<br>Error: " . mysql_error()); } else { $numrows = mysql_num_rows($query); echo $numrows; } } else { die("Please enter a username and password"); }
-
@jcbones: Although, you are correct that the source would be on one line and that is a HUGE pet peeve of mine. But, that is not the problem. I don't think the OP really understood the question from Katten @abjones116: It would have helped if you had posted some of the HTML source in question. But, looking at the code it appears you are opening the table before you start the loop. Then, in the loop, you are putting a closing table tag after each record. So, after the the first record , and the first closing table tag, the browser doesn't know how to interpret the subsequent TR and TD tags since they are not within a table. Try to be more organized in the logic and format of your code so these problems won't occur. Also, don't use PHP short tags (i.e. "<?") - use the full PHP tag - "<?php". I would also suggest putting the "logic" of your code (i.e. the core PHP code) at the top of your script. Use it to generate the output needed. Then put all the output (i.e. HTML) at the bottom of the script using echo's of the variables you created previously. The code below should work - not tested for minor syntax errors. <?php /* Get Transactions For Current User */ $query = "SELECT * FROM `transactions` WHERE `m_id`='{$sm_id}' ORDER BY time DESC"; $result = mysql_query($query); //Create variable to hold transaction output $txOutput ''; if(!$result) { //Use first line for debugging or 2nd line for production $txOutput = " <tr><td colspan='5'><b>Query failed!</b><br>Query: $query<br>Error: " . mysql_error() . "</td></tr>\n"; //$txOutput = " <tr><tdcolspan='5'>Unable to get transactions. If the problem continues contact the administrator.</td></tr>\n"; } if (!mysql_num_rows($result)) { $txOutput = " <tr><td colspan='5'>No Transactions Available.</td></tr>\n"; } else { while($row = mysql_fetch_array($result)) { $date = date('m/d/Y', strtotime($row['time'])); $txOutput .= " <tr>\n"; $txOutput .= " <td>{$date;}</td>\n"; $txOutput .= " <td>{$row['transaction_id']}</td>\n"; $txOutput .= " <td>{$row['method']}</td>\n"; $txOutput .= " <td>{$row['amount']}</td>\n"; $txOutput .= " <td>{$row['status']}</td>\n"; $txOutput .= " </tr>\n"; } } ?> <html> <body> <p><strong>Membership Transaction History:</strong></p> <p> </p> <table style="width: 100%" cellpadding="0" cellspacing="0" align="center"> <tr> <th><strong>Date</strong></th> <th><strong>Transaction ID</strong></th> <th><strong>Method</strong></th> <th><strong>Amount</strong></th> <th><strong>Status</strong></th> </tr> <?php echo $txOutput; ?> </body> </html> Edit: Note the spaces I have included in the output to pad the left side of the HTML code. Also, at the end of each line I used a \n (this will create a line break in the HTML source). Doing things like this will make it SIGNIFICANTLY easier to view the source of your rendered documents and find/fix problems. Note that the \n must be used within a double quoted string.
-
@Jesirose, you had the right solution, but I think you specified the wrong field. The OP is wanting to show a header whenever the "player" changes. @cdoyle, The solution is basically what jesirose stated, but change it to apply to the player name. Here is some mock code: $query = "SELECT spied_player, stat_1, stat_2, date FROM stat_table WHERE spying_player = '$playerID' ORDER BY spied_player, date"; $result = mysql_query($query); $last_spied_player = false; //Flag to track change in spied player while($row = mysql_fetch_assoc($result)) { //Display spied player name if different than last record if($last_spied_player !== $row['spied_player']) { //Add double line break if not first spied player if($last_spied_player !== false) { echo "<br><br>\n"; } //Set flag $last_spied_player = $row['spied_player']; //Display player header echo "<b>{$last_spied_player}</b><br>\n"; } //Display spied details echo "Date: {$row['date']}, {$row['stat_1']}, {$row['stat_2']}<br>" }
-
My results were pretty instantaneous. So, my only assumption would be that it's a connection issue. Remember, your server first has to request the file from the external server. Then your server sends the file to the user. So, every image displayed to the user has to go through two separate http requests. So, there could be a delay on either transmission or both that is causing a problem. Try adding some timing tests to see how long the file is taking to be read. That will at least tell you if the problem is on that end. Also, for testing purposes, take out the whole process altogether and just create an image tag with a direct link to the image and see what that performance looks like. If that also loads slow then the problem is due to the responsiveness of that external site and there's not much you could do. Since these images are generated via webcams I'm assuming they need to be current and caching them would be out of the question.
-
I don't know - I'm not going to try and figure out all the possibilities and try and benchmark them. But, the simplest thing I can think of would be this function displayImage($imageURL) { header ("Content-type: image/jpeg"); echo readfile($imageURL); } $imageURL = 'http://waternationlogbook.files.wordpress.com/2012/06/ocean-2-13887.jpg'; displayImage($imageURL);
-
Yes. I already have. I first rewrote the code into a function and then, for testing purposes, added a line between each step to capture the microseconds function createImageWithText($image_path, $text, $font) { $time['Step 1'] = microtime(); $width = imagefontwidth($font) * strlen($text); $time['Step 2'] = microtime(); $height = imagefontheight($font); $time['Step 3'] = microtime(); $image = imagecreatefromjpeg($image); $time['Step 4'] = microtime(); $x = imagesx($image) - $width -5; $time['Step 5'] = microtime(); $y = imagesy($image) - $height -5; $time['Step 6'] = microtime(); $backgroundColor = imagecolorallocate ($image, 255, 255, 255);\ $time['Step 7'] = microtime(); $textColor = imagecolorallocate ($image, 255, 255, 255); $time['Step 8'] = microtime(); imagestring ($image, $font, $x, $y, $text, $textColor); $time['Step 9'] = microtime(); //header ("Content-type: image/jpeg"); //imagejpeg($im); } Then I calculated the difference in milliseconds between each step. And here are the results from particular run: Step 1 - Step 2 : 0.00003500 Step 2 - Step 3 : 0.00001500 Step 3 - Step 4 : 0.05962100 Step 4 - Step 5 : 0.00003500 Step 5 - Step 6 : 0.00000800 Step 6 - Step 7 : 0.00001200 Step 7 - Step 8 : 0.00000700 Step 8 - Step 9 : 0.00002200 As you can see, the time to go from step 3 to step 4 took longer than all the other steps combined. The action performed on that step was $image = imagecreatefromjpeg($image_path); That was with a kinda big pic (800 x 600 I think). But, it would probably still be the bottleneck with smaller images. Also, I was doing this with a local image. If you are reading the files remotely you are going to have added overhead with that. But, that is what is solving your problem of not providing the images directly. Edit: I reran my tests with a remote image (from facebook) and the results were not changed much. but, you might want to test the time to read the images from the site you are working with.
-
Is it absolutely necessary to add the domain as text into the image? Could you just show the domain as text above/below the image? Then you don't need to process the image, just read it and spit back out to the user. In any event, I would add some code to time each step in that script and find where the most time is being used. Then see what you can do with that specific code: modify it, change to a different process, etc.
-
Grabbing HTML Table Rows That Are Sorted, But Results Aren't Sorted
Psycho replied to kinitex's topic in PHP Coding Help
It doesn't work like that! The dom class is reading the contents of the HTML - it does not try and render the content and then run JavaScript. What you view in your browser is the modified content that browser has changed due to the running of JavaScript. So, what you are suggesting is not an option. -
Grabbing HTML Table Rows That Are Sorted, But Results Aren't Sorted
Psycho replied to kinitex's topic in PHP Coding Help
For the love of all that is pure and good in this world, put some freaking line breaks in your output. All of your output in that page (starting from the table tag) is on a single line. How do you debug HTML issues? Do something like this: foreach($ret as $v) { echo "<tr>\n"; echo "<td>{$v['name']}</td>\n"; echo "<td>{$v['rating']}</td>\n"; echo "</tr>\n"; } So, as to your problem. You need to analyze the content you are getting and determine how you need to manipulate it. All you are doing is grabbing the raw HTML and trying to use it as data. You need to strip out the HTML and any unnecessary content. You are wanting to sort on rating, correct? Well, the cell that has the rating has a TON of code for the images of the stars including plenty of JavaScript events. You only need/want the rating so you can use that for ordering the results. So, first thing I would do is use strip_tags() on the values. Then see if the result is what you need to properly sort. The second problem is that asort() will not work on a milti-dimensional array. You can either use usort() as I suggested or an alternative that works for muti-dimensional arrays or you could use a different trick since you are only using two values. Put the name as the key and the rating as the value. Then you can and should use asort. This probably won't be perfect, but it will get you closer function scraping_table($url) { // create HTML DOM require_once('simple_html_dom.php'); $html = file_get_html($url); $result = array(); foreach($html->find('tr') as $row) { $name = strip_tags(trim($row->find('td',0)->plaintext)); $rating = strip_tags(trim($row->find('td',1)->innertext)); $result[$name] = $rating; } // clean up memory $html->clear(); unset($html); //Sort and return the results asort($result, SORT_NUMERIC); return $result; } $data = scraping_table('[url=http://www.forexfbi.com/best-forex-brokers-comparison/');]http://www.forexfbi.com/best-forex-brokers-comparison/');[/url] echo "<table>\n"; echo " <thead>\n" echo " <tr><th>Broker</th><th>Rating</th></tr>\n"; echo " </thead>\n"; echo " <tbody>\n"; foreach($data as $name => $rating) { echo "<tr>\n"; echo "<td>{$name}</td>\n"; echo "<td>{$rating}</td>\n"; echo "</tr>\n"; } echo " </tbody>\n"; echo "</table>\n"; -
Get the email body without the email footer
Psycho replied to son.of.the.morning's topic in PHP Coding Help
This is pretty worthless topic anyway. When replying/forwarding an email, the *way* in which previous messages in the thread will be formatted/segregated is completely up to the email application. So, you could write some logic to work for one scenario but would break for others. Of course, you could start adding more logic for other scenarios as they are identified, but you'd have no guarantee that they would not change at some point. In fact, some applications put the previous email thread at the top of the new message rather than the bottom. Plus, you would have to contend with the different formats and logic conflicting with each other. And, all of this assumes that the user won't add some text to the message that would be interpreted as a delimited for previous messages. -
Well, there's your problem. Why are you storing data in that manner? You should store each "record" separately. Otherwise you are creating bigger problems for yourself. Then, take the code you have to create the form - and rewrite it to create the form with the previously saved data. Do not copy the code and modify it. Instead you should rewrite that section of code to work with or without data to be used for populating the fields. Then you can include() that block of code when you need to create the form when there is existing data and when there is not.
-
Grabbing HTML Table Rows That Are Sorted, But Results Aren't Sorted
Psycho replied to kinitex's topic in PHP Coding Help
Are you expecting us to write the code for you? You already figured out how to get the first 6 records, so extend that to get all the records into an array. Then look into asort(), or another array sorting function, as to how to sort the result. So at least make an attempt - then if you have some specific problems post back with what specific step you are trying to achieve, what you have implemented and what is happening. -
Grabbing HTML Table Rows That Are Sorted, But Results Aren't Sorted
Psycho replied to kinitex's topic in PHP Coding Help
If the content, before JS run, is not sorted then you will need to grab ALL the rows, dump into a multi-dimensional array, then sort it yourself. I'd use a usort() to sort the records to my liking. -
I'm not really following you. If you stored the time in your timezone and want to know the difference then just calculate the difference between the time in the DB and your current time. How did you store the time based upon your timezone to begin with? You should be able to get what you want using DATEDIFF() to get the number of days difference. Or if, you want the difference in time use TIMEDIFF() SELECT DATEDIFF(NOW(), date_field) as difference FROM table But, if you want to work with times across timezones you should be storing it based upon GMT time - not your local time. Then translate the value to the appropriate offset when displaying it.
-
You will do yourself a huge favor by separating the logic from the presentation in your code. makes it much easier to find and fix errors. Having said that you should create a function to do this. Create a query to only get the fields you need and create a function to create the options list. You should be using an ID as the value of the options, but it's kind of hard to tell if that's the case above. Below is some sample code to point you in the right direction <?php //Function to create options dynamically function createOptions($optionsRS, $selectedValue) { $optionsHTML = ''; while($row = mysql_fetch_assoc($optionsRS)) { $selected = ($row['id']==$selectedValue) ? ' selected="selected"' : ''; $optionsHTML = "<option value='{$row['id']}'{$selected}>{$row['label']}</option>\n"; } return $optionsHTML; } //Run query to get current selected value $query = "SELECT obj_id as id FROM table_of_selections WHERE record_id = '{$_SESSION['record_id']}' LIMIT 1"; $result = mysql_query($query) or die*(mysql_error()); $selectedID = mysql_result($result, 0); //Run query to get list of options (set $query = "SELECT obj_id as id, obj_name as name FROM table_of_options WHERE foo = '{$_SESSION['bar']}' ORDER BY name DESC"; $optionResults = mysql_query($query) or die(mysql_error()); $fieldOptions = createOptions($optionResults, $selectedID ); ?> <select name="field_name"> <?php echo $fieldOptions; ?> </select>
-
OK, I like these types of problems, so I decided to write some code. This is by no means optimized code but it works. So, you will want to find where you can make it more efficient if you are going to run lost of products. The code will not create duplicates of the companies, types or ranges. But, I don't see anything that would be unique for the actual products. If there is a product ID that you are not sharing you could use that as a unique field in the DB. Or you could set (company_id + type_id + range_id + product) as a unique combination in the product table. I used a Excel sheet in the exact same format you you showed above. You must save the file as a CSV file before processing it with the code. I also provided the table structures as they require that you set up the company, type and range fields to be unique for this code to work. Code mysql_connect('localhost', 'root', '') or die(mysql_error()); mysql_select_db('products') or die(mysql_error()); //Open the file if (($handle = fopen("test.csv", "r")) !== FALSE) { //Get line 1 and do nothing with it $line1 = fgetcsv($handle, 1000, ","); //Create flag vars $current_company = false; $current_type = false; $current_range = false; //Create arrays to hold data $companies = array(); $types = array(); $ranges = array(); $products = array(); while (($data = fgetcsv($handle, 1000, ",")) !== FALSE) { //trim and escape the data $data = array_map('trim', $data); $data = array_map('mysql_real_escape_string', $data); //See if a new company exists if($data[0] != '') { $current_company = $data[0]; if(!in_array($current_company, $companies)) { $companies[] = $current_company; } } //See if a new type exists if($data[1] != '') { $current_type = $data[1]; if(!in_array($current_type, $types)) { $types[] = $data[1]; } } //See if a new range exists if($data[2] != '') { $current_range = $data[2]; if(!in_array($current_range, $ranges)) { $ranges[] = $current_range; } } //See if this is a product if($data[3] != '') { $products[] = array( 'product' => $data[3], 'details' => $data[4], 'company' => $current_company, 'type' => $current_type, 'range' => $current_range ); } } fclose($handle); } //Insert the new companies $query = "INSERT IGNORE INTO companies (company) VALUES ('" . implode("'), ('", $companies) . "')"; $result = mysql_query($query) or die(mysql_error()); //Get list of all companies with their IDs and put into array $query = "SELECT company_id, company FROM companies"; $result = mysql_query($query) or die(mysql_error()); $companies = array(); while($row = mysql_fetch_assoc($result)) { $companies[$row['company']] = $row['company_id']; } //Insert the new types then get list of all companies for the IDs $query = "INSERT IGNORE INTO types (type) VALUES ('" . implode("'), ('", $types) . "')"; $result = mysql_query($query) or die(mysql_error()); //Get list of all types with their IDs and put into array $query = "SELECT type_id, type FROM types"; $result = mysql_query($query) or die(mysql_error()); $types = array(); while($row = mysql_fetch_assoc($result)) { $types[$row['type']] = $row['type_id']; } //Insert the new companies then get list of all companies for the IDs $query = "INSERT IGNORE INTO ranges (range) VALUES ('" . implode("'), ('", $ranges) . "')"; $result = mysql_query($query) or die(mysql_error()); //Get list of all ranges with their IDs and put into array $query = "SELECT range_id, range FROM ranges"; $result = mysql_query($query) or die(mysql_error()); $ranges = array(); while($row = mysql_fetch_assoc($result)) { $ranges[$row['range']] = $row['range_id']; } //Process product data into insert values $prodValues = array(); foreach($products as $product) { //Set company, type and range to their respective IDs $companyID = $companies[$product['company']]; $typeID = $types[$product['type']]; $rangeID = $ranges[$product['range']]; //Add single insert record to array $prodValues[] = "('{$product['product']}', '{$product['details']}', '{$companyID}', '{$typeID}', '{$rangeID}')"; } //Create & run one insert query for all records $query = "INSERT INTO products (product, details, company_id, type_id, range_id) VALUES " . implode(', ', $prodValues); $result = mysql_query($query) or die(mysql_error()); Tables -- -- Table structure for table `companies` -- CREATE TABLE `companies` ( `company_id` int(11) NOT NULL auto_increment, `company` varchar(50) collate latin1_general_ci NOT NULL, PRIMARY KEY (`company_id`), UNIQUE KEY `company` (`company`) ) ENGINE=MyISAM AUTO_INCREMENT=19 DEFAULT CHARSET=latin1 COLLATE=latin1_general_ci AUTO_INCREMENT=19 ; -- -------------------------------------------------------- -- -- Table structure for table `products` -- CREATE TABLE `products` ( `prod_id` int(11) NOT NULL auto_increment, `product` varchar(50) collate latin1_general_ci NOT NULL, `details` varchar(50) collate latin1_general_ci NOT NULL, `company_id` int(11) NOT NULL, `type_id` int(11) NOT NULL, `range_id` int(11) NOT NULL, PRIMARY KEY (`prod_id`) ) ENGINE=MyISAM AUTO_INCREMENT=17 DEFAULT CHARSET=latin1 COLLATE=latin1_general_ci AUTO_INCREMENT=17 ; -- -------------------------------------------------------- -- -- Table structure for table `ranges` -- CREATE TABLE `ranges` ( `range_id` int(11) NOT NULL auto_increment, `range` varchar(50) collate latin1_general_ci NOT NULL, PRIMARY KEY (`range_id`), UNIQUE KEY `range` (`range`) ) ENGINE=MyISAM AUTO_INCREMENT=4 DEFAULT CHARSET=latin1 COLLATE=latin1_general_ci AUTO_INCREMENT=4 ; -- -------------------------------------------------------- -- -- Table structure for table `types` -- CREATE TABLE `types` ( `type_id` int(11) NOT NULL auto_increment, `type` varchar(50) collate latin1_general_ci NOT NULL, PRIMARY KEY (`type_id`), UNIQUE KEY `type` (`type`) ) ENGINE=MyISAM AUTO_INCREMENT=5 DEFAULT CHARSET=latin1 COLLATE=latin1_general_ci AUTO_INCREMENT=5 ;
-
EDIT: Requinix beat me to it, but including this anyway as there is additional info. I think you need to step back a moment. There is nothing wrong with a table with a million records in it. You should not break up data into separate tables because you think it might be "too much". You need to focus on creating a good database schema and properly indexing the tables to get appropriate performance. You will need to create a PHP script to read the data line by line and process the data appropriately. But, processing that many records could poise a timeout issue. Anyway, looking at the data, here is what I think you would need for the DB: 1. A table for companies. It should have two fields for "company_id" and "company_name" 2. A table for type. It should have two fields for type_id (primary) and type_name 3. A table for range. It should have two fields for range_id (primary) and "range_name" (not too sure about this one as I don't fully understand it) 4. Lastly a table for products which would have fields as follows: prod_id (primary), prod_name, prod_details, company_id, type_id, range_id Now, when you process the data you will need to take several steps to find and enter new companies, types and ranges before entering the products. because you need to get the ids of those values for the products table. So, map out the process on paper before you start coding anything. All of the primary keys in the tables should be auto-increment fields. The ID fields at the end of the products table will be foreign keys.
-
Uploaded files are stored as temporary files. You have to save the file to a permanent location if you want to keep them. So, you can name the file anything you want when you save it. So, the answer is yes.
-
I think is what is being missed here is that there are apparently multiple file input fields. I would assume that not all of them are required. But, the post/file data will include records for all the fields - even if they have no data. So, the logic needs to exclude fields where a file was not supplied. My take: $valid_types = array(IMAGETYPE_JPEG); //Check if any files were submitted if(isset($_FILES['photoname'])) { //Set flag variable $allFilesValid = true; //Iterate through all files submitted foreach($_FILES['photoname']['tmp_name'] as $tmp_file) { //If any "submitted" file is not valid set flag to false and exit loop if($tmp_file != '' && !in_array(exif_imagetype($tmp_file), $valid_types)) { $allFilesValid = false; break; } } }
-
Hmm, what constitutes a "valid" email address is a complicated issue. I've never seen one that was 100% correct. And, I doubt that any MySQL solution would be anywhere near as good as good as one in PHP could be. You need to START by creating a function/process for excluding invalid emails to begin with. Then you can use that same function to run a single process to remove any invalid emails from your table. But, you should prevent invalid entries being created before trying to fix the DB. Once you have a validation check in place and you have removed all the invalid entries you can just query the table for all the entries that have a value.
-
get value for variable by adding a counter as part of the name
Psycho replied to PeggyBuckham's topic in PHP Coding Help
Yes, it is complicated because you are trying to create variables where none are needed. This is a complete waste: foreach ($_POST as $key => $value) { $$key = $value; } So, if there is a field called 'foo' in your form you are creating a variable called $foo in your code. Why? Just reference $_POST['foo']. You are creating a whole extra level of complexity where none is needed. Also, I'm not sure from your code if the two arrays of values for the two options are used elsewhere or not. E.g. do you have those in the database? If so, you should query the database for the IDs and values. If not, then you should hard code the arrays and include them on the pages where they are needed. I wouldn't create them dynamically as you have done. Making changes could cause previous entries to report the wrong results. So, looking through your code it appears you have some groups of checkboxes and you want to auto-populate one in each group based upon the submitted values. You should create a function that takes two parameters: the array of options and the selected value. I'm not following everything you are doing, but the code below should get you started. To use it you only need to define the name/value pairs to use for each group of checkboxes and provide a "name" for the checkboxes. The name will be used to create an array of checkboxes. Also, the name is used to check for an existing POST values of the same name to use for the purposes of selecting the appropriate selected checkboxes. This is a complete working example <?php //These should be pulled from the DB $color_array = array( 1 => 'Blue', 2 => 'Green', 3 => 'Yellow', ); $example_array = array( 1 => 'example_1', 2 => 'example_2', 3 => 'example_3', ); function createCheckboxGroup($name, $valuesAry) { $checkboxesHTML = ''; //Check POST data to see if there was a selected value $selectedValues = (isset($_POST[$name])) ? $_POST[$name] : array(); //Create the checkbox group foreach($valuesAry as $value => $label) { $checked = (in_array($value, $selectedValues)) ? ' checked="checked"' : ''; $checkboxesHTML .= "<p><input style='width: 15px; margin:0' type='checkbox' name='{$name}[]' value='{$value}'{$checked} />{$label}</p>\n"; } return $checkboxesHTML; } ?> <html> <body> <form action="" method="post"> <b>Select your colors:</b><br> <?php echo createCheckboxGroup('color', $color_array); ?> <b>Select your examples:</b><br> <?php echo createCheckboxGroup('example', $example_array); ?> <br><button type="submit">Submit</submit> </form> </body> </html> -
No need to have an OR clause to pull records from today OR yesterday. Just create a value for the records starting yesterday and do a greater than comparison. However, your code is going TWO days back. So, you are getting everything from today, yesterday and the prior day. This should work SELECT title, date From From tbl_event WHERE `dateFrom` >= DATE_SUB(CURDATE(), INTERVAL 1 DAY) This will get all the values from today and yesterday