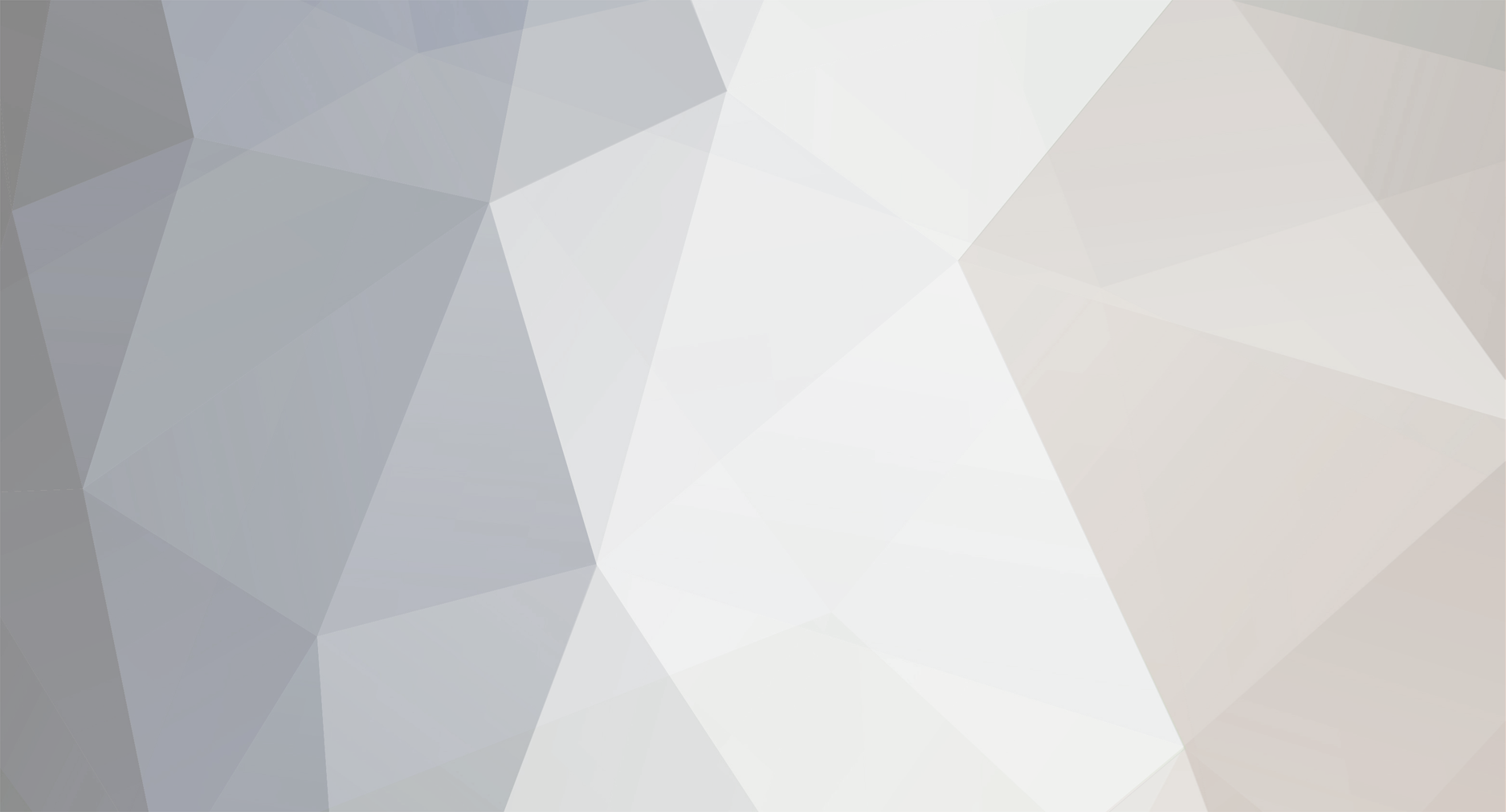
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Really? I did a simple google search for "MySQL Update tutorial" and the very first result provided a pretty simple example. And, how is this a PHP question? (moving to MySQL forum). But, to answer your question, you simply put a WHERE clause on the update statement. That WHERE clause can be broad (WHERE gender = 'female') or very specific (WHERE id = '1').
-
PHP cannot open windows (or do anything on the client-side). But, you are probably misunderstanding some thing. The JavaScript should open the new window - but it would be calling a PHP script to generate the output in that page.
-
I see two places where you are updating the value of 'stat_bonus' and in both the value is set as $player->stat_bonus - $amount I assume $player->stat_bonus is the users current value. So, just implement a check as follows before running those queries if($player->stat_bonus - $amount < 0) { //The amount would create a negative balance - implement error condition } else { //Allow the update }
-
yes, another way of writing it, except that you want to keep the dash immediately following the opening character class bracket so it is not treated as a meta-character. Also, the OP was using eregi(), which performs a case-insensitive search, thus the reason for the appended "i" modifier. Right, but doesn't the use of \w remove the need to use 'i' for case insensitivity?
-
No, YOU need to take a step back and understand what is being said. You have a select list of 10 options where the only difference is that the value/display is going from 0.00/0 to 10.00/10. Hard coding those options is just plain stupid. Drumming provided some code that would dynamically create those options AND auto-select the appropriate option based upon the previously selected value. Which you were told to do back on page 1 of this thread. You OBVIOUSLY didn't even try it. However, there was one difference in what his code would produce and what you currently have. The values would be 0, 1, 2 ... instead of 0.00, 1.00, 2.00, if that is really necessary that can be easily fixed. Here is my suggestion //Get the ID of the record being displayed //Variable name is just an example, replace with whatever you are using $recID = (int) $_GET['id']; //Get the currently selected value from the DB based upon the record //-- This assumes you need all the data for the record to display on the page //-- otherwise you would change the query for only the fields you need //-- The value of 'roastturkey' for the record will be used to auto-select the correct option $query = "SELECT * FROM pass WHERE id = '4'"; $result = mysql_query($query); $record = mysql_fetch_assoc($result); //Create a loop to generate ALL the select options using the value of //$recod['roastturkey'] to auto-select the record's current value $optionValues = range(0, 10); //Create an array of values from 0 to 10 echo "<select name=\"my_field\">\n"; foreach($optionValues as $val) { //Create a selected variable to set for each option $selected = ($val==$record['roastturkey']) ? ' selected="selected"' : ''; $decVal = number_format($val, 2); //Format value with decimals if required echo "<option value='{$decVal}'{$selected}>{$val}</option>\n"; } echo "</select>\n";
-
If I am understanding that expression correctly you could simplify that as preg_match( "~^[\w-/]+$~", $value )
-
Simple If statement wont work (MS Excel)
Psycho replied to Help!php's topic in Other Programming Languages
That works fine for me in Excel. Are you sure the value you are referencing is really in cell F29 and that is a number type? -
Output the page to an HTML file and debug the code to at least narrow the cause down to a particular process. If you can't find the problem then post the the JavaScript forum.
-
There's another option, but it may or may not fit your needs. If your scenario really is about producing units in a time period you probably want to have an inventory of them to do other things with (sell, use or otherwise reduce inventory). But, if your example above is not really accurate and you are only looking for total units generated since a certain time you could simply set the time that production started and dynamically calculate the total whenever you need to. For example, if you set the start of production using a timestamp you could calculate the total production at any time using the following process: //This value would be set/saved when production was supposed to have started //Retrieve it whenever you need to know the current total production $production_start; //Set the time period (in seconds) that production takes $production_cycle = 30 * 60; //30 minutes //Set the units per production period $units_per_cycle = 750; //calculate the total production of units at the current time $total_units_produced = floor((time()-$production_start)/$production_cycle) * $units_per_cycle; If cron/scheduled tasks are not an option and you need to do inventory as well, you *could* use the method above but it wouldn't be idea. You could either update the inventory with new production amounts by checking when the last update was (on a page load) and seeing if any new production was done. If so, update the inventory with the newly produced quantities. OR, don't add to the inventory. Instead always use the calculated value and only store deductions from inventory. The whole downside to this is if you want to change production levels or other criteria change. It becomes a headache trying to keep it all in line.
-
Ah, yes, line 55 should be while ($row = mysql_fetch_assoc($result)) // <== use $result, not $query If you look above, I create the query as a string variable ($query) then run it and assign the results to $result //Create and run query for records on current page $query = "SELECT bookname, bookauthor, bookpub, bookisbn FROM booktable ORDER BY ".mysql_real_escape_string($_SESSION['order'])." ASC LIMIT $limit_start, $records_per_page" $result = mysql_query($query) or die mysql_error(); This is a VERY good process to follow instead of writing your queries directly in the mysql_query() function like this: $result = mysql_query("SELECT * FROM table") Many times when you have errors running queries it is due to some dynamic data in the query and it is difficult to debug without seeing the query with those dynamic values. So, using the method I provided above you could echo the $query variable to the page if you need to debug an issue.
-
You should start with the pagination and then add the sorting ability. The issue you need to overcome is that for each page load you need to determine both the page and the sort order. How you do that is up to you. Typically for the links to change pages you will have an href with a parameter added for the page to load. But, if that page loads and you don't know the sort order, well it fails. You could add the sort order to each link as well. Or, alternatively, you could add it to a session value. I would use the latter. Your code was kind of messy, so here is a quick rewrite. I put into what I feel is a more logical structure and changed some variable names to be more descriptive. Since I don't have your DB this is not tested, so there will likely be some typos. But, I think the comments should help explain what the code is supposed to be doing and you can fix them <?php session_start(); //Config variables $records_per_page = 10; //If user set the sort order, save to session var - else set default if($_POST['order']) { $_SESSION['order'] = trim($_POST['order']); } elseif(!isset($_SESSION['order'])) { $_SESSION['order'] = 'bookname'; } //Determine the total records and pages $query = "SELECT COUNT(bookname) FROM booktable"; $result = mysql_query($query) or die mysql_error(); $total_records = mysql_result($result, 0); $total_pages = ceil($total_records / $records_per_page); //Set the page to load $page = (isset($_GET['page'])) ? (int) $_GET['page'] : 1; if($page<1 || $page>$total_pages) { $page = 1; } //Create limit var for query $limit_start = ($page-1) * $records_per_page; //Create and run query for records on current page $query = "SELECT bookname, bookauthor, bookpub, bookisbn FROM booktable ORDER BY ".mysql_real_escape_string($_SESSION['order'])." ASC LIMIT $limit_start, $records_per_page" $result = mysql_query($query) or die mysql_error(); //Prepare output $recordsHTML = ''; while ($row = mysql_fetch_assoc($get)) { $urlParams = "bookname={$row['bookname']}&bookauthor={$row['bookauthor']}&bookpub={$row['bookpub']}&bookisbn={$row['bookisbn']}"; $recordsHTML .= "<tr>\n"; $recordsHTML .= " <td><a href='addtolist.php?{$urlParams}'>Add to basket</a></td>\n"; $recordsHTML .= " <td>$bookname</td>\n"; $recordsHTML .= " <td>$bookauthor</td>\n"; $recordsHTML .= " <td>$bookpub</td>\n"; $recordsHTML .= " <td>$bookisbn</td>\n"; $recordsHTML .= "</tr>\n"; } //Create pagination links $navLinks = ''; //Prev page if($page > 1) { $prevPage = $page - 1; $navLinks .= "<a href='products.php?page=$prevPage'>Prev</a> "; } else { $navLinks .= "Prev "; } //Individual pages for($p=1; $p<=$total_pages; $p++) { $pageNo = ($p == $page) ? "<b>{$p}</b>" : $p; $navLinks .= "<a class='pagin' href='products.php?page=$p'>$pageNo</a> "; } //next page if($page < $total_pages) { $nextPage = $page + 1; $navLinks .= "<a href='products.php?page=$nextPage'>Next</a>"; } else { $navLinks .= "Next"; } //$record_count = mysql_num_rows($get); //if (isset($_GET['showerror'])) //$errorcode = $_GET['showerror']; //else //$errorcode = 0; ?> <html> <head></head> <body> wont include all the html rubbish and the ordering menu! <div id="mid"> <table> <tr> <th></th> <th>Book Title</th> <th>Book Author</th> <th>Book Publisher</th> <th>Book ISBN</th> <th></th> </tr> <?php echo $recordsHTML; ?> </table> <?php echo $navLinks; ?> </body> </html> EDIT: you didn't include the part of the code with the select list for determining the sort order. I would update that code to auto-populate with the currently selected sort order.
-
Sounds like a JavaScript problem. To debug those I suggest generating a complete/working HTML page. Save it as a flat file. Then, work on the JavaScript code there to get it working. Once you have it working then you can determine what changes are needed in the PHP script. Trying to fix JavaScript problems in a PHP script can be very difficult.
-
OK, I lied. I found this interesting and wanted to give it a shot. This is pretty sloppy, but it works with the testing I did. I'll leave it to you to parse it down as needed and clean it up function getTextPart($text, $maxCount=800) { preg_match_all('#([^<]*)|(<[^>]*>)|([^<]*)#', $text, $matches); $output = ''; $letterCount = 0; $maxLength = false; foreach($matches[0] as $line) { if(empty($line)) { continue; } if($line[0]=="<") { $output .= $line; } elseif(!$maxLength) { $space = 0; foreach(explode(' ', $line) as $word) { if(!$maxLength && (strlen($word) + $letterCount + $space) <= $maxCount) { if($space) { $output .= ' '; } $output .= $word; $letterCount += strlen($word) + $space; $space = 1; } else { $maxLength = true; } } } } return $output; } echo getTextPart($input);
-
Since the tags aren't displayed I wouldn't consider them part of the "first 800" characters. So, I'd build a process to strip out all the characters after the first 800 that aren't tags. So, you might have some empty tags towards the end but since there would be no content between them they wouldn't do anything to the display. It's a little late, otherwise I might tinker with this.
-
If people are buying things you should not rely upon data submitted by the user to use as the price. you should simply have the user pass the product(s) that they are buying then you would determine the price in the code using the values you set (most likely in the DB). The logic you are wanting to use would not prevent someone from passing a 1 when the cost might be 1000.
-
I really don't know and just don't have the time to get into it today. Besides, a new thread would be best since I don't want to give you a sub-optimal solution. Best that someone with experience with JSON help you.
-
Why are you asking me? Do whatever you wish (within the rules of the forum). I doubt I will take the time to go through your code line-by-line.
-
But that's no true. A legitimate GIF could have... <?php phpinfo(); ?> That is moot as long as you are renaming the file so it would not be executed as a PHP file. You shouldn't care what anyone puts as comments inside a file. As stated COUNTLESS times, you have already been given the information needed to make sure that you are receiving valid images and what to do to safeguard them from being executed.
-
I've never worked with JSON encode. But, now that you know you are getting the correct data, you should probably create a new thread about formatting the output into the format you need. I would provide an example of the data you have and the format you need it in.
-
Pass it a file with a gif extension which is NOT a gif file. If you are already validating that the file is an actual image (not just a file with an image extension) then most likely you would never get to this code with an invalid image (which is why it has been stated previously that this code would not add any additional security). And, since I assume you are pulling the $imageType from the file you should be confident that the type is correct. For example, if you were to rename a jpeg image to have a .gif extension, it would pass the validation that it really is an image and when it gets to this section it should "know" that the image is really a gif image and process it accordingly - instead of failing. So, a couple thoughts: 1. Just to validate this code as a backup to the code you already have in place to validate that it is an image file, bypass the image validation that comes before this code. Then pass the script an invalid image file with an image extension. This code should produce the error condition. 2. Pass the script a valid image file that uses the wrong extension (e.g. a jpeg image with a gif extension). That image should be processed without error. but, that is just a guess because I don't know what prior validations you have.
-
The "nefarious" code you keep referring to would make the file an invalid image - i.e. it would not display as an image. So, if getimagesize() returns valid values then it doesn't have "nefarious" code. But, as stated already, if you have a need to convert the images, then by all means do so. But, if you are doing what you say you are, then you are adding any more security prevention to the process. The only thing that may be happening by recreating the images is that you would probably be removing any meta information attached to the image. That wouldn't be a security risk for your application, but there could be anything listed in those tags. But, this is all moot. You've stated that you need to re-size these images anyway. So, the question of whether you "should" recreate the images or not is not a question since that will be happening when you re-size them anyway. Whether you use a common image type or not is a personal preference. Personally, I would use a common image type with the user id as the name of the image.
-
How to use real time instead of a tick based system
Psycho replied to kaosjon's topic in PHP Coding Help
Well, using your example as a starting point, all you would need is a timestamp field in the DB for the "completed_ts". So, when a user selects to build a house you would set the timestamp to 10 minutes in the future. Now, whenever someone is doing something that requires you to provide a list of completed houses you would simply add a condition to the query to only show the ones where the "completed_ts" is >= NOW() So, to restate this another way. Instead of trying to UPDATE records as they change status after a certain time, simply save values for the records that will determine when they change state. You can then SELECT the records based upon those that make sense based upon context. For example, other users may not be able to see houses that are in the process of being built. but, users can see those that they are building. -
Looks like some code that is ready for you to test. Determine the success/error conditions you want to test for and run the script with those conditions to verify the results. Although I would probable add a default case to the switch statement to set $origImage = FALSE;. Then if the image type isn't found the error condition will run correctly.
-
And you were given instructions on how to prevent those security risks by renaming the file such that is doesn't have any php 'embedded' extensions and/or validating that it really is an image. The "malicious" images you are worried about are not really images. They are simply a script that is renamed to have the extension of an image (typically with an embedded 'php' extension). So, the process to convert an image will do the same thing as getimagesize() would do. It will work when it is a valid image and it will fail when it is not. Are these profile type images? If so, then you will want to do some processing of the images to put them into an acceptable size. And, while doing that you can convert to a standard format. Otherwise, you might have someone upload an image like this for their profile image. As already stated, there is no security need to convert the images to a standard format. If you need to maintain the original size of the image it would be more efficient to use the getimagesize() method to filter our potentially malicious uploads.
-
if($origImage===false) { //Could not create the new image //Add error messaging and or handling as you see fit } else { //Creation of new image was successful //Continue with additional processes to run against image identifier }