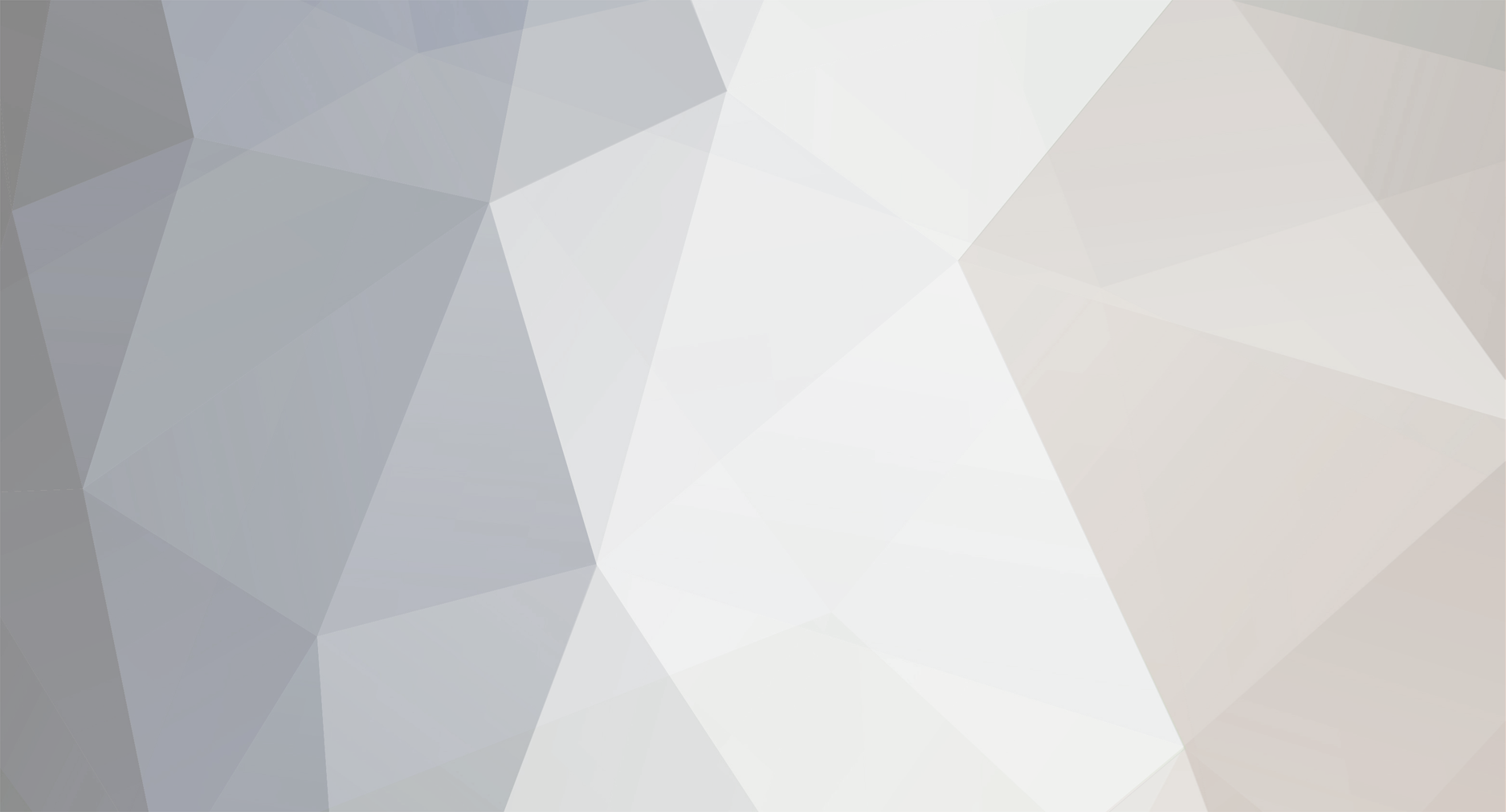
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Array_multisort with a variable amount of arrays
Psycho replied to longphant's topic in PHP Coding Help
Not pretty, but it works: //Class for custom sorting class customSort { private $sortArray = array(); function __construct($sortArray) { $this->sortArray = $sortArray; } function doSort__($a,$b) { foreach($this->sortArray as $key) { if ($a[$key] != $b[$key]) { return (($a[$key] > $b[$key]) ? 1 : -1); } } return 0; } } ## USAGE ## //Define array sof keys to sort by - in order $sortKeys = array('color', 'age', 'brand'); //Perform the sorting usort($inputArray, array(new customSort($sortKeys), "doSort__")); //Original array is now sorted -
Inserting multiple form checkbox results to MySQL database
Psycho replied to flipper828's topic in PHP Coding Help
Only checkboxes that are checked are sent in the form data. So, you cannot reference the unchecked checkboxes and expect there to be a value. However, it is pretty easy to write your code to work regardless. But, your code is full of problems. E.g. $check_list[] = $_POST['check_list[]']; The proper way to reference those checkbox fields is with $_POST['check_list'] (without the []). I would like to provide more help, but I'm not really understanding what you are really doing here and I suspect your approach is totally wrong. From what I see, you have a form that, when posted, creates a new record with two values: the first is 'regid' and the second is the array of the checked values. That really makes no sense to me. How do you differentiate one record vs. another. Plus, I don't see any lines of code in what you posted that would cause the errors you displayed. Are you sure that is the correct code that is processing the form? Anyway, based upon what you have posted, here are my assumptions and recommendations: It looks like this is a form for a person to sign-up for classes. So, first off you need to identify which person the form is being submitted for and include that in the data being stored for the record(s). You should create a table to store the available webinars and those records should have a primary id, available time, and webinar name. You would use that table to generate the form. Then, in that form the checkboxes would be created as arrays with the primary ID of each webinar as the value of the checkbox. <input type="checkbox" name="check_list[]" value="1" > Wednesday February 29, 2012 4:30 PM EST <input type="checkbox" name="check_list[]" value="2">Friday March 2, 2012 9:00 AM EST Then, when the user submits the form you would store 1 record for each checkbox selected. The table should have a user_id (or some way to identify the person) and a column for the webinar id as well as any additional information you need on the selections (e.g. timestamp?). -
It worked for me. As I said, there is still some cleanup needed - such as better error handling. Echo the entire query to the page. That should make it easier to debug. Also, I assume you needed to make same minor changes to the queries I provided. Please post the lines that create the query as well. You did make the 'code' field the primary field, right?
-
Well, you can write it different ways, but the underlying process will be the same. You need to query the DB for existing records. I will say, however, that having an if/else statement that returns 1 or 0 is superfluous. Simply return the condition. Here is how I would write that: //returns TRUE if email exists, else FALSE function verifmail($email) { db_connect(); $email = mysql_real_escape_string($email); $query = "SELECT email FROM users WHERE email='$email' LIMIT 1"; $result = mysql_query($query); return (mysql_num_rows($result)==1); }
-
Well, I am going to suggest a complete rewrite. The DB structure is wrong. You should have individual records for each currency type - not a field for each currency. That type of structure does not scale. I.e. If currencies are added/removed you should not have to change your DB structure. Your table should only need three fields: code, name, rate, ratedate. CREATE TABLE `exchange` ( `code` varchar(4) collate latin1_general_ci NOT NULL, `name` varchar(50) collate latin1_general_ci NOT NULL, `rate` float NOT NULL, `ratedate` timestamp NOT NULL default CURRENT_TIMESTAMP on update CURRENT_TIMESTAMP, PRIMARY KEY (`code`) ) Note the use of an auto-update timestamp field - so you don't have to set the time when creating/updating records, it will be done automatically. Here is the code to insert/update the database records. Note, this will work even if rates are added/removed from the feed. //Load XML file $XML=simplexml_load_file("[url=http://www.ecb.europa.eu/stats/eurofxref/eurofxref-daily.xml]http://www.ecb.europa.eu/stats/eurofxref/eurofxref-daily.xml[/url]"); //Process data into values for DB $insertValsAry = array(); foreach($XML->Cube->Cube->Cube as $rate) { $code = mysql_real_escape_string(trim($rate['currency'])); $rate = floatval($rate['rate']); $insertValsAry[] = "('$code', '$rate')"; } //Create and run INSERT/UPDATE query $insertVals = implode(', ', $insertValsAry); $query = "INSERT INTO exchange (`code`, `rate`) VALUES $insertVals ON DUPLICATE KEY UPDATE `rate` = VALUES(`rate`)"; $result = mysql_query($query) or die(mysql_error()); NOTE: The data feed does not include the 'name' of the currency, so you would have to add that value for each record one time. You could create a page to do that, but since it would be so rare, you should just do it directly in the DB. And, here is the page to allow the user to get the exchange rate value $fromCode = isset($_POST['selectCurrencyTo']) ? strtoupper($_POST['selectCurrencyTo']) : ''; $fromAmount = isset($_POST['from_amount']) ? floatval($_POST['from_amount']) : 0; $output = ''; //Create/run query to get list of available rate codes $query = "SELECT `code`, `name`, `rate` FROM `exchange` ORDER BY `name`"; $result = mysql_query($query) or die(mysql_error()); $rateOptions = ''; while($row = mysql_fetch_assoc($result)) { if($row['code'] != $fromCode) { $selected = ''; } else { $selected = ' selected="selected"'; $toAmount = round($row['rate'] * $fromAmount, 2); $output = "{$fromAmount} € = {$toAmount} {$row['code']}"; } $rateOptions .= "<option value=\"{$row['code']}\" {$selected}>{$row['name']} ({$row['code']})</option>\n"; } ?> <html> <head></head> <body> <form id="form1" name="form1" method="post" action="<?php htmlentities($_SERVER['PHP_SELF']); ?>"> <input name="from_amount" type="text" class="CP_ExchangeFormFields" id="CurrencyAmount" size="6" value="<?php echo $fromAmount; ?>" />€ <span style="padding:5px;">to</span> <select name="selectCurrencyTo" class="CP_loginFormFields" id="selectCurrencyTo"> <option value="" >Select currency</option> <?php echo $rateOptions; ?> </select> <input type="submit" name="button" id="button" value="convert" /> <br><br> <?php echo $output; ?> </form> </body> </html> There is still alot of clean up I would do on this though. I'll leave that to you.
-
Because storing data in that way takes away your ability to easily do queries based upon many different scenarios. For example, if you wanted a list of all the products a user was watching, it would require two queries instead of one. And, if you wanted a list of all the users that were watching a certain product it would be even more complicated. Don't go adding arrays to database fields because you don't know how to properly use a database. Using an intermediary table, this would give you a list of all the products watched by a user SELECT products.* FROM products RIGHT JOIN watched_products USING (product_id) WHERE watched_products.user_id = '$userID'
-
Yes, absolutely No offense, but you must not understand how relational databases work. Tables of records will routinely have a "primary" key which is the unique id for that record. Then in other tables where you have records that must be "related" to those record a "foreign" key will be used. The foreign key will be the primary ID value from the other table.
-
Not working "how"? what is the input and the output you feel is wrong. That condition is very "loose" meaning that as long as the variable is set and not an empty string it will result in true. Your textareas have a line break in them so it will always result in true unless the user removes the value. You should probably trim the value and then check if it is empty() if(isset($_POST['savebtn'])) { $left = trim($_POST['left']); $right = trim($_POST['right']); if(!empty($left) && !empty($right)) { echo "there is text"; }else{ echo "this is no text"; } }
-
Define css-class to be used based on the time?
Psycho replied to Luggruff's topic in PHP Coding Help
The following will give you the time in a decimal format of hours. You can use this in your comparisons $decTime = date('G') + (date('i')/60); So, 2AM will be 2.00, 2:15AM will be 2.25, etc. -
Define css-class to be used based on the time?
Psycho replied to Luggruff's topic in PHP Coding Help
PHP does not recognize 20:00 as a value as you have it. Date/times can be represented as a timestamp (which is an integer that represents the number of seconds since Jan 1, 1970) or as a string, e.g. "20:00". But, string values cannot be compared using < or > like you want. You will need to implement some actual logic to do the check for you. EDIT: Your current comparisons look to be off. The first one checks for times before 8PM or after 1AM. But, the second is checking for times after 8PM or before 2PM. -
$foo = 'b'; $foo .= 'a'; //Concatenate 'a' to current value $foo .= 'r'; //Concatenate 'r' to current value echo $foo; //Output: bar $foo = 'b'; $foo = 'a'; //Replace current value with 'a' $foo = 'r'; //Replace current value with 'r' echo $foo; //Output: r
-
Just a simple table with two columns for the user and product foreign keys should suffice.
-
If you would like further help from me, please post the entire script as well as a description of the database tables/fields.
-
What you are doing does not make sense. You can do as I stated without changing your current database. Whether you decide to put this new information in the database or a flat-file is not important. But, you need to create the data so you can associate the currently code with the currency name. If you have a table with the currency name, add another column for the currency code. Then use THAT for the value you pass. I just checked the feeds available for the European Central Bank and they are all in the format http://www.ecb.europa.eu/rss/fxref-[CURRENCY_CODE].html So, why you are not using the currency codes is beyond me.
-
You need to have the data that associates the currency "id" (e.g. 'AUD', 'BRL', etc) with the currency name is some for. This can be done in the database or in an array. Then you don't have to have 30+ lines of code to create your select list. Then you should use the ID as the value of the select list instead of $row_currency_RS[<ID VALUE>] Then when the user POSTS the data you can get the $row_currency_RS[<ID VALUE>] value from the ID passed AND you can get the name using that ID.
-
Sort it "how"? There are several functions for sorting arrays, the simplest of which is . . . drum-roll please . . . sort()
-
There have been several discussions on this (on this forum and other). There are also other methods that you did not include: heredoc and nowdoc methods. All of them are valid methods for creating output. The bottom line is that the performance difference of either method is so negligible that it shouldn't be a consideration for the method you use. The main thing is to find a process that works and be consistent.
-
This topic has been moved to MySQL Help. http://www.phpfreaks.com/forums/index.php?topic=354151.0
-
Your query is malformed. Right after the WHERE clause you have an "AND". Run your query through PHPMyAdmin to get it right - then create it in the code. Also, it does look like the fields referenced in the WHERE and ORDER BY clauses have an improper field name. I assume "t_joinpgmid" should be "t.joinpgmid" and "t_id" should be "t.id". I would also suggest using JOINS instead of joining the data in the WHERE clause SELECT * FROM p_transaction AS t JOIN p_joinpgm AS j ON t.t_joinpgmid = j.joinpgm_id JOIN p_merchant as m ON j.joinpgm_merchantid = m.merchant_id WHERE t.joinpgmid = '$joinid' ORDER BY t.id desc And, lastly, list out the fields you need in the SELECT clause rather than using '*'. It's inefficient to be querying data that you won't use
-
max() This will return the highest value in the array. But, you are referring to the field incorrectly if (isset($_POST['participantqty']) && max($_POST['participantqty']) > 0){ You would likely need to do some additional validations based upon what you expect the values to be. If you don't want the '0' values int eh array you might consider running it through array_filter() first.
-
Sure you can. I tested the query above before I posted and I just ran a very simply query using SELECT * FROM table_name GROUP BY field_name which worked fine as well. My results showed that when using GROUP BY on such a query the first record for the duplicate values in the GROUP BY clause are returned. So, the query I posted above creates a temporary table that orders the values such that the records returned are the ones the OP is after. I did state But, it does work. I would be interested in knowing a more proper method to get the requested data. EDIT: From the MySQL manual (emphasis added)
-
It might be more productive to show the code which is causing the error. You are getting an error that the resource is not a valid image. Based upon what you have stated I think the problem is that you have copied the temp image and changed the name in the process but you are using a different value when trying to reference the image for some other purposes. So, I don't think the problem is due to how you are changing the name, but that you aren't using the new name in every place that you need to.
-
I want all values, but MySQL is only returning one
Psycho replied to satre's topic in PHP Coding Help
And the reason you get an array with the same value twice is explained in the manual for mysql_fetch_array() Personally, I always use mysql_fetch_assoc() which only returns an array of associative (i.e. named) indices - which is the same as using mysql_fetch_array() with the MYSQL_ASSOC flag. I've never seen the need to return two sets of the same values or to refer to values by their indices. Either one can easily cause code to break of fields are added/removed. There are some situations when you would need the other data - but those should be the exception, not the norm. -
DO NOT RUN QUERIES IN LOOPS. It is terribly inefficient. Anyway, LittleGuy's solution in the other thread was adequate. But, I think your problem is this: If you have dates - store them as Dates. Otherwise, you will not be able to use the date functions within MySQL. You need to store the dates in one of the date/datetime formats supported by MySQL. Then just change the format of the date to YYYY-MM-DD when inserting the record. Also, use the field names when you retrieve the values instead of the index. Otherwise, if you add/remove a field your code will break Then just use a query with a JOIN. Here is what I would do. //Add any additional field you want from the products //or reservations table in the SELECT clause $query = "SELECT p.prodid, p.prodname FROM products AS p JOIN reservations AS r USING (`prodid`) WHERE `resdate` = '$resdate'"; $result = mysql_query($query) or die (mysql_error()); while ($row = mysql_fetch_assoc($resultavail)) { echo "{$row['prodid']} - {$row['prodname']}<br>\n"; } If you don't know how to use JOINs go find some tutorials. Trying to teach that in a forum post is not realistic.
-
Good point. But, without knowing more about the actual data and the context it was only a guess. The time periods for the activities might be dependent on the activities (e.g. dinner would always be a nighttime activity) or they may be dependent upon the location (e.g. scuba-diving may be offered as a daytime or nighttime activity based on location). You really have to do an analysis of your data and have a working knowledge on how data structures work. Else you are going to paint yourself into a corner.