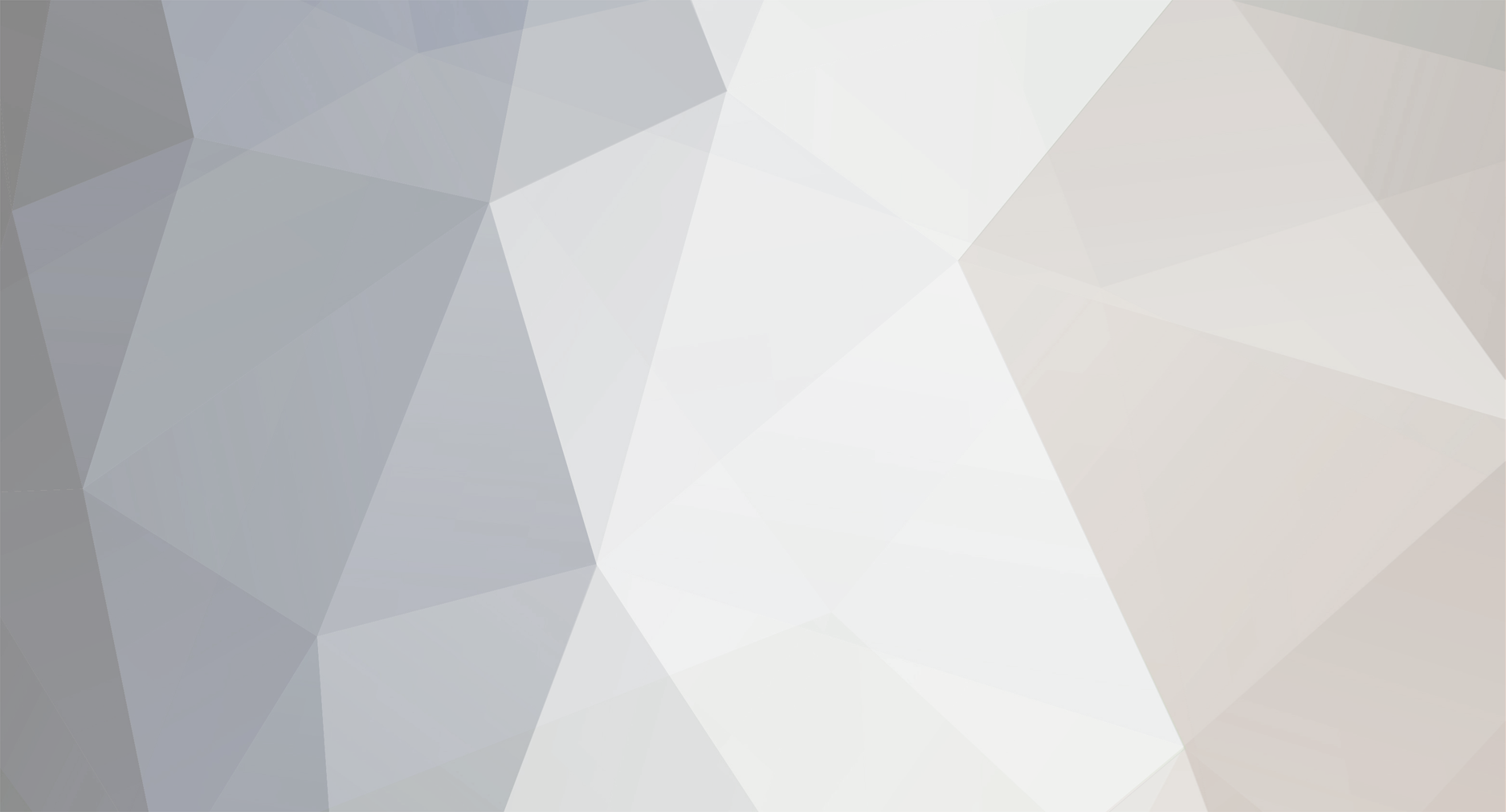
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
OK, that's better. In addition to your particular problem I would not use bold tags. Instead, you should set an appropriate class, add another class or set the style of the element. I also think it's a good idea to separate your logic from the presentation. So, I would create the content for the links at the top of my script or a separate file entirely. Then echo the links into the body of my output document. Anyway, give this a go $nav_links = ''; foreach ($menu as $elem) { $style = ($elem['system_name'] == $this->system_name) ? ' style="font-weight:bold;"' : ''; if ($elem['system_name'] == 'home-content') { $url = ROOT . '/'; } elseif($elem['system_name'] == 'apply') { $url = 'http://www.anothersite.com'; } else { $url = ROOT . '/' . $elem['system_name'] . '.html'; } $nav_links .= sprintf('<li class="%s"><a href="%s"%s>%s</a></li>', $elem['li_class'], $url, $style, $elem['title']); } //Echo this in the output document echo $nav_links; ?>
-
It's not clear what to give you. You obviously know you need to put an if statement in. But, what the condition should be is up to you. You haven't provided any details about what values determine the change or what that change would be. I'm guessing that the variable to check may be: $elem['title'] So, if($elem['title'] == 'userpage') { //Do normal process } else { //Do something else }
-
Going with one table to store all this information is a bad idea. Making multiple columns in a table to store the same type of data will make the code much more difficult to create and the solution would not be scalable. Although I'm not totally understanding your data structure, here is an idea: Table: places This table would have data such as : place_id, place_name, location, etc. Anything that is a one-to-one correspondence Table: Activities This table would store different types of activities that can be performed at the palaces. The activities are not specific to the places. Field would be something like: activity_id, activity_description, time_of_day (morning, afternoon, evening). Table: place_activities This table would be used to associate activities to the places. Each record would have two columns to associate each activity to each place. So, if place #1 is Orlando Florida and activity 15 is Disney World and activity 22 is Universal Studios you would have two records such as place_id | activity_id 1 15 1 22 If you go with one table your queries are going to become very complex.
-
I wouldn't see how that code would cause a problem. The date/time functions should be working off of the server. But, that could cause a slight problem around the beginning/end of the month. For example, if the server is 12 hours ahead of the user, on the last day of the month that month would not be available for that user after noon on that day. Other than that, I don't know why you would have a problem. Have you tested it? Try changing the timezone on your PC to see if there are any problems.
-
I'm not sure I understand what you are talking about. You really need to define your requests with a little more clarity. If you are talking about the process of creating the links that is pretty simple. Just set the value in the URL for each link you create. Since you provided no code I can't give you anything specific. But, I would create a function. Also, I see you have two similar values in the URL "sortorder" and 'sortby'. But, the 'sortby' value is apparently an order ID, so I would call that something such as 'filterby' Here is an example function searchURL($pageName, $sortBy, $fromDate, $toDate, $sortOrder) { $url = "{$pageName}?sortby={$sortBy}&fromdate={$fromDate}&todate={$toDate}&sortorder={$sortOrder}"; return $url; }
-
There will be no $URL['orders_id'], there will be a variable as $_GET['sortby'] with the value associated. So, you simply need to use the value of $_GET['sortby'] to determine what field to sort on. Of course you want to sanitize and validate the value before using it in a query Sample code //Create list of approved field the query can be ordered by $approvedSortField = array('orders_id', 'from_date', 'todate'); //Create ORDER BY caluse for query if(in_array($_GET['orders_id'], $approvedSortField)) { $orderByClause = " ORDER BY {$_GET['orders_id']} "; } else { $orderByClause = ''; } //Add order by clause to query $query = "SELECT * FROM table_name {$orderByClause}";
-
That is absolutely NOT how you should do this. You need to learn how to do JOINs in your queries. The real benefit of a relational database is to be able to pull related records in one call. I'll give a very generic example. Let's say you have two tables: Authors and Book. The authors table has columns for the author_id (primary) and name. The Books table has two columns: book_id (primary), author_id (foreign key) and title. You can then run one query to get a list of the authors and their books SELECT a.name, b.title FROM authors AS a JOIN books USING (author_id) ORDER BY a.name, b.title
-
OK, this "should" work, but I leave it to you to test. Also, not sure if this is the most efficient method. This basically puts the records in the correct order before doing the GROUP BY $sql="SELECT * FROM (SELECT price, dep, des, airline, flighttype, baggage, season, cabin, id FROM faresheet WHERE cabin = '{$q}' AND des = 'DEL' ORDER BY price ASC) as temp GROUP BY airline";
-
The GROUP BY clause should just use 'price' not 'MIN(price)'. Not sure if that will fix your problem, but give it a shot. EDIT: Never mind, that won't work. May need a subquery. I'll see if I can find a solution if no one else has an answer.
-
This topic has been moved to Announcements. http://www.phpfreaks.com/forums/index.php?topic=353968.0
-
There have been numerous posts on this forum in the past for similar things. But, if you are planning to do this with anything more than a small subset of characters and character count it is not realistic. The permutations will expand exponentially. Here are a few related posts: http://www.phpfreaks.com/forums/index.php?topic=346630.0 http://www.phpfreaks.com/forums/index.php?topic=298656.0 http://www.phpfreaks.com/forums/index.php?topic=135633.0
-
Note: the manual even contains a user submitted comment regarding this issue http://www.php.net/manual/en/function.strtotime.php#107331
-
Hmm, may be a version issue with PHP. I just tested it and got incorrect results. I tested the original code using a "seed" date of Jan 31 and I tested the actual result of the timestamp created in the loop and it went as follows: Jan 1, March 2, then March 31. It completely skipped Feb and had two results for March. When I tested it by "normalizing" the date to the 1st of the month it worked correctly. //Create a seed timestamp for 1st of current month $start_month = strtotime(date('M 1, Y')); // start a for loop to increment an integer // $i will start at 0 and end at 11 (so 12 increments) for($i = 0; $i <= 11; $i++) { // $timestamp will contain the UNIX timestamp returned by strtotime // by default, strtotime works off the current time // so in this case we are adding X months to the current month // the amount of months is determined by $i which will be // incremented with each pass of the for loop, starting with 0 $timestamp = strtotime('+' . $i . ' month', $start_month); // here we are just getting the different formats of the date // from the UNIX timestamp that we got above $month_text = date('F', $timestamp); $month_num = date('n', $timestamp); $year = date('Y', $timestamp); // and finally, we append a formatted string to $option $option .= sprintf("<option value='%d-%d'>%s</option>\n", $year, $month_num, $month_text); }
-
Again, I would first test it to see if my understanding is correct (like I said I'm having problems right now, so I can't). But, if the problem does exist, you just need to set an initial timestamp where adding '+1 month' will get you the results you want. But, I think I was mistaken about setting to the 1st, the 15th might be a better option. I'll see about uploading files to a different environment and testing this and I'll respond back on whether any changes are needed.
-
I think there might be a problem with that code. If it was Jan 31st, and you did strtotime('+1 month') it will skip February. I'm having problems with my dev environment right now, But, I'm pretty sure I've seen this behavior before. You could set the optional parameter for strtotime() to be the 1st of the current month.
-
Need some help with creating a treeview from an array please
Psycho replied to gibbo1715's topic in PHP Coding Help
Your question is about an array that you have (which I guess the code above is creating) and outputting it in a specific format. You need to describe the format of the array and how you want the output generated. The code you have above really doesn't make sense to what I understand you have/want. The code above will simply create a multidimensional array with each sub-array consisting of two values "area" and "title". You state "id love to be able to do is use my area field as the parent and my title field as the child", but they are not a parent/child of each other in the current format of your array. They are siblings. Further the asort() wouldn't do anything (if I understand the code correctly) since it will only sort the values of the first level of the array, which are arrays. It wouldn't sort by the values of the sub-arrays. If you have multiple records with the same "area" then you need to make the area the index in the parent array with the "title" as the values in sub-arrays. But, I am really just guessing here because I really have no idea what your data looks like since you only provided code for building the array. You really need to show the input data you are working with and an example of the output you want generated. But, here is a *guess* at what I *think* you may want. //Put results into a multi-dimensional array with //the area as the key and the titles as values $data_array = array(); foreach ($view->result as $result) { //Hey look, descriptive variable names! $area = $view->render_field('field_decision_type_value', $row); $title = $view->render_field('title', $row); $data_array[$area][] = $title; } //Sort the array by the 'area' key values ksort($data_array); //Output the results foreach($data_array as $area => $titles) { //Display area header w/ title count $title_count = count($titles); echo "<b>{$area} ({$title_count})</b>\n"; //Sort the titles asort($titles); //Display the titles for the current area echo "<ol>\n"; foreach($titles as $title) { echo "<li>{$title}</li>\n"; } echo "</ol><br>\n"; } -
You can't put a PHP block inside another PHP block. You already put an opening tag for PHP processing right here <? //change this to your email. (Although you should NOT be using short tags!) So, when the PHP parser get here $message="<?php include("another.php"); ?>" It's going to be confused because the PHP parser was already initiated. However, you *could* do this: $message = include("another.php"); Although that can work I doubt it will work for you with your current file. An include file can be configured to "return" a value, but it is not very common so I doubt you configured it in that way. You would need to build up the content in that file as a variable, and then at the end do a return $email_content;
-
From the manual for getimagesize() under Return Values See the related function exif_imagetype() which lists the numeric number associated with each type (3 == PNG image) Again from the manual for getimagesize(), this time under Errors/Exceptions Although your problem may be a folder issue - the function can and will return errors if you don't pass it an image.
-
storing current date and time in datetime format
Psycho replied to php_begins's topic in PHP Coding Help
You're looking at it the wrong way. Don't try and SET the value before you run the query - just use NOW() IN the query and the database will insert the correct time. So, just pass the string of 'NOW()' - but if your insert method does any parsing of the values this might not work $qcData = Array( "date_time" => 'NOW()', "note" => $note ); $qcNotes = new App_Model_DbTable_QCNotes(); $qcNotes->insert($qcData); However, there is a MUCH, MUCH easier solution. Just set up your datetime field in the database to automatically use the current timestamp as the default value. Then you do not need to pass/set the value at all - it will be done automatically -
I think your dates need to be enclosed in quotes
-
The problem is not with Chrome - it is with the code. It just seems that FF is correcting the error - something you should not be relying on. I didn't check all of your code, but I found this one line that would cause problems <option label='People's Democratic Republic of Yemen' value='YD'>People's Democratic Republic of Yemen</option> You use single quotes to enclose the value for the label parameter. But, that value ALSO has a single quote mark in it. You could do several things: 1. Use double quotes (as long as no values use double quotes) 2. Use addslashes() 3. Use htmlspecialchars()
-
You should index it if you plan on using it for JOINs gizmola's response was based upon the fact that you have a WHERE clause that checks if game_id is a value between two date values - when you should be checking if the "date" is between those two values. Give this a try $query = "SELECT COUNT(g.game_id) FROM scheduled_umps AS u JOIN games AS g USING(game_id) WHERE u.ump_id = '$_SESSION[ump_id]' AND g.date BETWEEN $four_months_ago AND $four_months_from_now GROUP BY u.ump_id";
-
Try austDay.setMinutes(austDay.getMinutes()+20); Or austDay = new Date(austDay.getFullYear(), austDay.getMonth(), austDay.getDay(), austDay.getHours(), austDay.getMinutes()+20);
-
Yeah, there were spaces that were replaced with line-breaks. I assumed that was a copy/paste error.
-
Forget what I said about normalizing the times to 12:00:00, that is a way to overcome a problem with DST, but a different problem than yours. You can simply round the result. function daysDiff($startDateTS, $endDateTS) { $days = round(($startDateTS - $endDateTS) / (60 * 60 * 24)); return $days; } $days = daysDiff(strtotime($info['expiredate']), time());