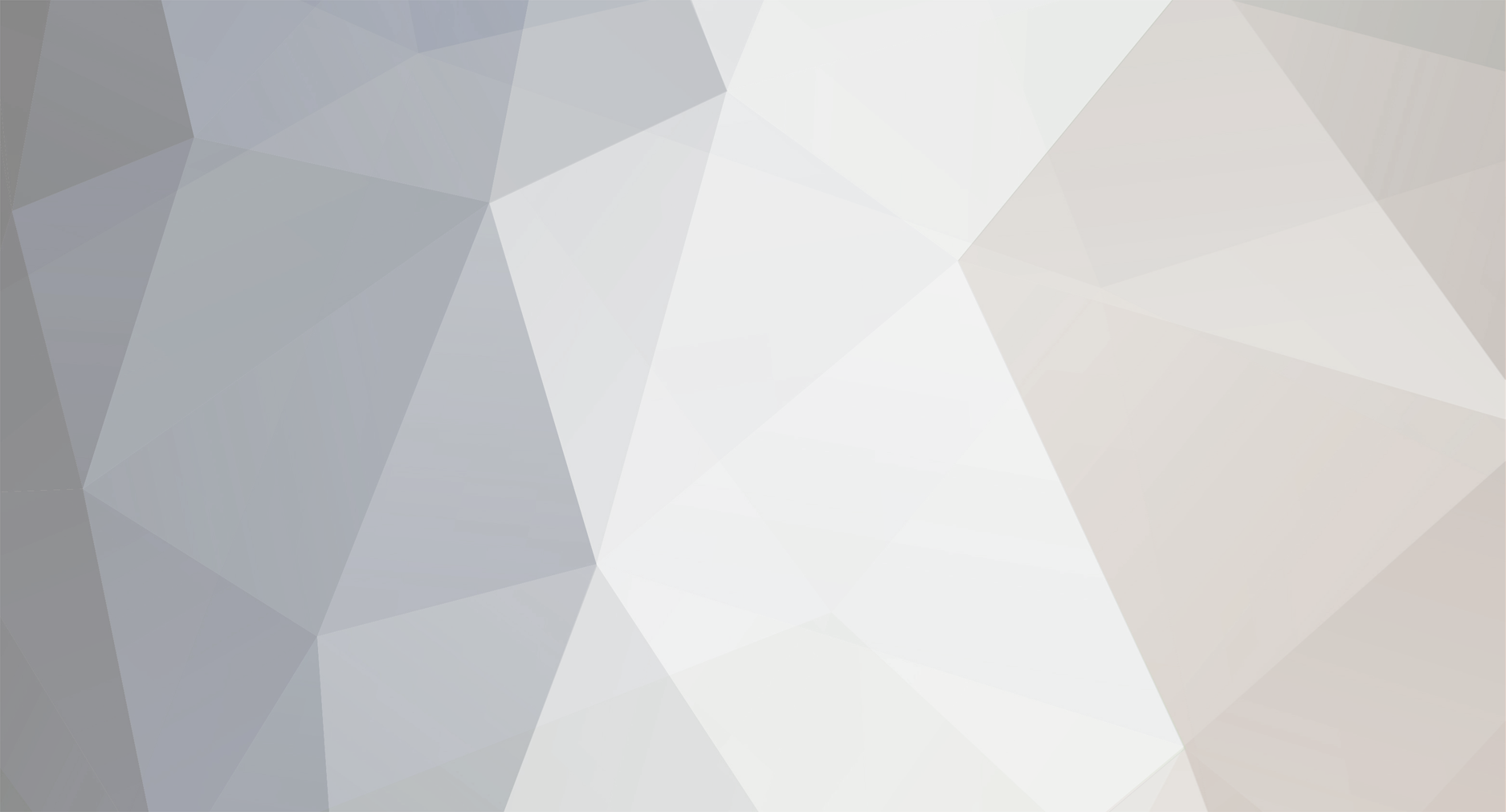
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Not likely. It's probably because of daylight savings time which changes on March 11 (at least for my location). Whenever calculating days you can avoid the problem by normalizing yoru date/times to noon (or some other time of day that won't be affected by DST). EDIT: The DST problem would only be an issue if 'expiredate' is in the future. Why are you doing the subtraction in reverse and then taking the absolute value?
-
Here is a script I created a long time ago that will resize an image to a specified dimension. It also takes an optional parameter to determine whetther you want the image cropped. If this parameter is set to yes, then the image will be resized (proportionally) so that one dimension is the same as the target and the other dimension will be larger than the target with the overlap cropped off. So, if you have an image that is 100 x 150 and you want it resized to 60 x 60, the result will be exactly 60 x 60 with 50% of the 150 dimension cropped off. As I said, I wrote this a long time ago and haven't used it in a long time. So, I give no warranty //This function resizes and, optionally, crops an image and returns an image resource. //If cropping is selected, the image will be resized and cropped such that it exactly fits the dimensions. ###UPADTE TO ALLOW DIFFERENT INPUT IMAGE TYPES ###UPADTE TO ALLOW DIFFERENT OUTPUT IMAGE TYPES function resizeImage($filename, $dst_w, $dst_h, $crop=false) { //Format new dimensions as integers $dst_w = intval($dst_w); $dst_h = intval($dst_h); //Get dimensions of original image $src_info = @getimagesize($filename); //Return false if any invalid input if($dst_w<1 || $dst_h<1 || !$src_info) { return false; } //Define additional variables needed list($src_w, $src_h) = $src_info; $dst_x = 0; $dst_y = 0; $src_x = 0; $src_y = 0; //Get ratios of source and destination $src_ratio = $src_w / $src_h; $dst_ratio = $dst_w / $dst_h; if($crop!==true) { //Calculate resize dimentions if ($src_ratio < $dst_ratio) { $dst_w = round($dst_h / $src_ratio); } else { $dst_h = round($dst_w / $src_ratio); } } else { //Calculate crop dimentions if ($src_ratio < $dst_ratio) { //Determine Resize ratio on width $ratio = $dst_w / $src_w; //Detemine cropping dimensions for height $crop = round((($src_h * $ratio) - $dst_h) / $ratio); $src_y = round($crop/2); $src_h = $src_h - $crop; } else { //Detemine Resize ratio on height $ratio = $dst_h / $src_h; //Detemine cropping dimensions for width $crop = round((($src_w * $ratio) - $dst_w) / $ratio); $src_x = round($crop/2); $src_w = $src_w - $crop; } } //Create new image $dst_image = imagecreatetruecolor($dst_w, $dst_h); $src_image = imagecreatefromjpeg($filename); imagecopyresampled($dst_image, $src_image, $dst_x, $dst_y, $src_x, $src_y, $dst_w, $dst_h, $src_w, $src_h); return $dst_image; }
-
This is rough, but it works. It only requires the first part of the input sting with each word and its type identifier. $neg_adv = array('not', 'never'); $input = "It/PRP was/VBD not/RB okay/JJ or/CC funny/JJ and/CC I/NN will/MD never/RB buy/VB from/IN them/PRP ever/RB again/RB (It was not okay or funny and I will never buy from them ever again)"; $output = array(); foreach(explode(' ', $input) as $part) { if(strpos($part, '/')) { list($word, $type) = explode('/', $part); if($type!='RB' || !in_array($word, $neg_adv)) { if($type=='JJ' || $type=='RB' || $type=='VB' || $type=='VBN') { $output[] = 'not-'.$word; } else { $output[] = $word; } } } } echo implode(' ', $output);
-
Well, as you just said you can't use the IP address since that would change. So, you can never really know if the person using an account is the same person or not. If you try to verify if the person logging into an account is the same person or not you are going to cause a lot of difficulty for your users and you. The least painful implementation would be to ensure you don't have the same login being used concurrently. But, since you never know if a user will log out vs. just closing the browser window you would have to implement a time-limit. For example, when someone completes the login you would store their IP in a table. Then on each page load you would save a "last_activity" timestamp. Then when a user attempts to log in you would see if there was a last_activity for the user at a different IP address within the lat 15 minutes (?) or whatever time period you want. You could then prevent the new user from logging in until the first session has expired or you could allow the new user to log in and terminate the previous users session. Basically there are any number of avenues you can take here.
-
When a user first starts the game, set the start time like so $_SESSION['Yahtzee']['start_time'] = time(); Then when the user finishes the game, calculate the total time like so $total_time = time() - $_SESSION['Yahtzee']['start_time'] = time(); Then do whatever you want with that value
-
So, you want to update three fields FOR THE SAME RECORD? You are making it too hard. $query = "UPDATE announcements SET title = {$title}, content = {$content}, lastmodified = NOW() WHERE id = '{$id}'"; mysql_query($query);
-
How do I compare 2 random numbers to entries in a table
Psycho replied to apw's topic in PHP Coding Help
I'm with Kicken on this. From what I understand you want to generate two random numbers and then try to find a matching record in your table. It would make more sense to simply select a random entry from your table. Then use the values from that record. -
Well then, it sucks to be you. Did you even try to figure out what was wrong? Did you run the query through PHPMyAdmin first to see what that produced before trying to implement it into your code. I did mistakenly alter the numeric search by changing the "> 0" to "= 0". That one should be using the greater than operator and the symbol search should be using the equal operator. but, in either case you would not get 0 results - unless you legitimately had no matching records. I tested the process above and it worked as I expected. I created sample data as follows: A - Alpha 1 x - Alpha 2 0 - Numeric 1 9 - Numeric 2 $ - Symbol 1 @ - Symbol 2 . - Symbol 3 When I used WHERE LEFT(UPPER(title), 1) = 'A' and WHERE LEFT(UPPER(title), 1) = 'X' I received the results of 'A - Alpha 1' and 'x - Alpha 2', respectively. When I used WHERE INSTR('012345679', LEFT(title, 1)) > 0 I received the results of '0 - Numeric 1' & '9 - Numeric 2'. When I used WHERE INSTR('ABCDEFGHIJKLMNOPQRSTUVWXYZ012345679', LEFT(UPPER(title), 1)) = 0, the results were: '$ - Symbol 1', '@ - Symbol 2', & '. - Symbol 3'. Test your queries in your database management APP before you try and implement in your code. Then, once you implement, if you do not get the results you expect 1) validate that the query you are running is the one you expect, 2) check if the query is returning errors, i.e. mysql_error(), 3) If no errors, see if the query is returning results, i.e. mysql_num_rows(). If the query is completing w/o errors and mysql_num_rows() is >t 0 and you are getting a blank page then there is most likely a problem in the PHP code for outputting the results.
-
"Kill off other queries"? Not sure what you mean by that. You can erroneously create code that prevents other code from running properly, but that is not a limitation of the code. That is simply a bug that needs to be fixed.
-
When you say symbol, don't you really mean anything that is NOT a letter or a number? And, what was your idea? I would just use the same logic applied for numbers, but use a negative check. if ($letter=='0-9') { //Number search $where = " WHERE INSTR('012345679', LEFT(title, 1)) = 0"; } elseif($letter=='SYMBOL') //Change to the value you use for symbol { //Symbol search $where = " WHERE INSTR('ABCDEFGHIJKLMNOPQRSTUVWXYZ012345679', LEFT(UPPER(title), 1)) = 0"; } else { //Letter search $where = " WHERE LEFT(UPPER(title), 1) = '$letter'"; }
-
Remove the comma before the "FROM". I also prefer to format my queries in multiple lines to make them easier to read/analyze. $query = "SELECT Car_Make, Car_Model, Image_Van FROM products WHERE Car_Make= '$strMake' AND Car_Model = '$strModel'";
-
OK, so let me restate what I think I understand, then I'll provide some sample code: You aren't building a timeclock system. Instead you have a door entry system that is bio-metrically controlled and records when a user unlocks the door with a timestamp. You are wanting to generate some type of report to show when a user is inside or outside the office. One question would be whether user's have to use the scanner to leave the office. If yes, is that record recorded any differently (e.g. an id of the scanner used) than the ones used to enter? If you can identify whether the user is using the enter scanner vs the exit scanner that is useful information. However, even if you know which scanner a person used you cannot know whether the user is inside or outside the office. It isn't going to verify that the person walked through the door. And, if you can't differentiate which scanner the person used it is even more dangerous to assume whether the user entered or left. Take the situation of someone unlocking the door then suddenly realizing they left something in their car. It's not a matter of whether something like that will happen it is a matter of when. If you rely upon these reports to determine when someone entered/left you are going to have situations that may cause a lot of headaches. But, based upon the input data I believe you have and what you want to accomplish, this is what I would suggest: In the table that you have now, add a column called "processed" and make it a tiny Int type. Set the default value to 0. Then, create a process that will process new records. To process the records, create a new table like we discussed above with one record to hold both an in and out time. In order to prevent problems with incomplete records (i.e. no Out time) you should only run this on records for the previous day. Then the actual process would work something like this: 1. Select all records from the original table prior to the current day where the processed value is 0 sorted by UserID and Date. 2. Create a loop to run through the results taking two records at a time for each user using the first as the In time and the second as the Out time. Calculate the time difference. Then create a new record it he new table with the in/out times and the time period. 3. At the end of the processing, update the records in the original table (prior to current day where processed is 0) to have a processed value of 1. Also, you would need to handle those situation where there is an uneven number of entries for a user (such as would occur in the situation I described above). I would probably create a record with an In time and no value for out or period. Then have some sort of exception report for someone to review. Also note that this would not work very well if you have people that are working past midnight. In that case it would be very difficult to make any determinations on In/Out. You can then use the data in the new table to run your reports.
-
Yes, I understand what you want. I was explaining one way the database records *should* be stored. The way you have them stored now it going to do nothing but cause problems. 1. If a punch is missed or a record doesn't get created properly your times are going to be messed up and you will have no easy way to identify the problem and/or fix it. 2. Calculating the time periods is going to require a VERY inefficient process of querying all the records and processing them one by one. I'm not going to try and provide a solution for a process that is so flawed to begin with. At the very least you should store an additional piece of data for each record to indicate if it is a punch out or in. That would solve problem #1, but it's still going to require an overly repetitive process to do the calculations.
-
The '.' and '..' are special links within the file system that allow you to traverse up the directory structure. You can simply add some if() conditions in your logic to skip them (see example #2 in the manual). Or, alternatively, you can use glob() - which is my preference when reading files/folders. It simply returns an array and never includes those two special links. As for the order - it is not random. It is in alphanumeric order. Windows has logic to do a "natual" sort how a human would order text with trailing numbers. PHP has a couple functions to do this type of sorting on an array. So, either use readdir() to dump the contents into an array first or used glob(). Then use either natsort() [case sensitive] or natcasesort() [case insensitive] to sort the array before outputting it.
-
Really not enough information on what you currently have or your specific requirements to provide a good solution. Most time tracking systems (that I've worked with) create/update records in pairs. And, each pair is given a "type": work time, break time, lunch, etc. So, if the user clocks in at 9:00 a new record will be created such as date | start | stop | type 20120209 | 09:00 | | work Then, when the user clocks out for a break at 9:10 the system will set the stop time for the first event and and create a new event with the start time date | start | stop | type 20120209 | 09:00 | 09:10 | work 20120209 | 09:10 | | break That process will continue until the user clock-out for the day. Then you just set the stop time for the last record and do not create a new record. You can then add up all the hours for the day, week, etc for each type (work, break, lunch etc.)
-
EDIT: removing my post.
-
I know it wasn't a complete copy/paste but still - my expectation is that the person receiving the code goes through it to understand what it is doing and can debug minor errors. The fact that you got errors stating "supplied argument is not a valid MySQL result resource" should have told you that the query failed. But, you seem to be willing to learn. So, I'll provide a couple suggestions on how you could have found/fixed these errors yourself. 1. I always create my queries as string variables instead of defining them in the mysql_query() function so I can echo them to the page when an error occurs. Of course, you should have a switch in your logic to show a generic error when in a production environment. 2. On the problem you had with no results being displayed you could have checked that there were results returned from the query. Example code: //Put this in a config file that is loaded for any page define('DEBUG_MODE', true); $query = "SELECT somethign FROM table_name WHERE foo='$bar'"; $result = mysql_query($query); if(!$result) { //Query failed if(DEBUG_MODE !== true) { echo "There seems to be a problem. Please try again later'" } else { echo "The query failed. Query: {$query}<br>Error: " . mysql_error(); } } else { //The query passed. Check if there were results if(!mysql_num_rows($result)) { echo "There were no matching records."; } else { //Output the result while($row = mysql_fetch_assoc($result)) { //Echo the formatted data } } }
-
Or, perhaps the OP should have looked further and adjusted the provided code to meet his needs. The code I provided was just an example and should never have been simply copy/pasted into the script.
-
Seriously, you're going to dump that entire script (with all those line breaks) and expect what from us? You can either store the image as a flat file (i.e. jpg, gif, png) and store the path in the DB or you can actually store the image data in the DB. The first method is the easiest IMO. So, just store the image path for each record in the field "Image_Van". Then, when you query the records use that value to create an image tag using that value as the src parameter value of the image. $query = "SELECT make, model, Image_Van FROM table_name WHERE make='$make' AND mode='$maode'"; $result = mysql_query($query); while($row = mysql_fetch_assoc($result)) { echo "{$row['make']}, {$row['model']} <img src='{$row['Image_Van']}'><br>\n"; }
-
Here is a basic idea of the process I use. Maybe it will help: //Create array to store validation errors $errors = array(); //Perform validations if(empty($name)) { $errors[] = "Name is required"; } if(empty($email)) { $errors[] = "Email is required"; } if(empty($password)) { $errors[] = "Password is required"; } elseif(strlen($password)<8 || strlen($password)>16) { $errors[] = "Password must be between 8 and 16 characters"; } elseif($password != $passwordMatch) { $errors[] = "Passwords do not match"; } //Check if there were errors if(!count($errors)) { //There were no errors, process the results (i.e. insert/update db records) } else { //There were errors, show them echo "The following errors occurred:<br>\n"; foreach($errors as $err) { echo " - {$err}<br>\n"; } }
-
Yeah, that's not going to work, well not without getting very creative. Once you submit the form the page will refresh and wait for the output from the server. You would have to use AJAX to avoid that. But, there are many different solutions to your problem. But, first you need to find out what is causing your script to take so long to run. I'm guessing it is the multiple emails you are sending. But, you should add some debugging code to take timings throughout the script and echo the results to the page. Then you can make informative decisions on what you should/need to do. Don't try to work around performance problems instead of addressing them! You really should not make a user wait to create/update a record because of notifications or something similar. As I stated you could send ONE email to all recipients to avoid that. Alternatively you could have the process simply add records to a notification table with the message and the email addresses. Then use a cron job to send the notifications on a regular basis. That way the notification process is completely separated from the create/update process.
-
If you are doing a process on 10K - 100K records you need to make sure these processes are extremely efficient. I really think you need to reassess what you are doing. Having all the records on one page is just plain dumb in my opinion. No one is going to read through 10,000 or 100,000 results. You are simply wasting resources by trying to show them all on one page. If you were to have multiple people trying to call that page simultaneously you are going to run into performance problems.
-
Well, pagination is really what you "should" be using. A person cannot realistically work with 100,000 records on a single page. They would have to scroll up and down to see/work with the records anyway. Pagination gives you (and the user) a lot more flexibility than showing the records on one page (sorting, filtering, etc.). For example, if the user wants to sort the records, doing that with pagination is simple and efficient. Trying to re-query all 100,000 records to resort them is a complete waste of resources. But, since you asked the question, I will provide an answer. You can dynamically load records by using AJAX. But, you would NOT want to only load one record at a time. Running database queries is an expensive operation and running 100,000 queries will bring your server to a crawl. Instead you should target larger subsets of data that can be grabbed efficiently (I'd start with 100, maybe even 500). You can run tests to find the optimal count. So, when the page loads you would have some JavaScript code that will make a call to a PHP script that will query 100 records, process it, and return the formatted data back to the JavaScript which will append the content into the display. Then the JavaScript will make the same call again to get the next 100 records. The process will continue until the PHP script returns to the JavaScript that there are no more records. But, there are some problems with this approach. The one that comes to mind is that if records are added/removed during this process it could cause records to not get added to the display or be duplicated. For example, if you get the first 100 records, then someone adds a record that would have been in position 50, the next call to get records 101-200 will have the record that was at position 100 will now be at position 101 and would be duplicated.
-
I'm not understanding what you expect that to do.
-
I'm not following you. If you don't know what your variables are doing you need to figure that out first. I routinely use an $errors array and do exactly as I described above. Do you define $errors as an empty array at the beginning of your code? If not, then that logic won't work. In other words, what do you get when using that condition? Do you get an error or does $errors have a count at that point? Did you test it (i.e. echo the count() of $errors)?