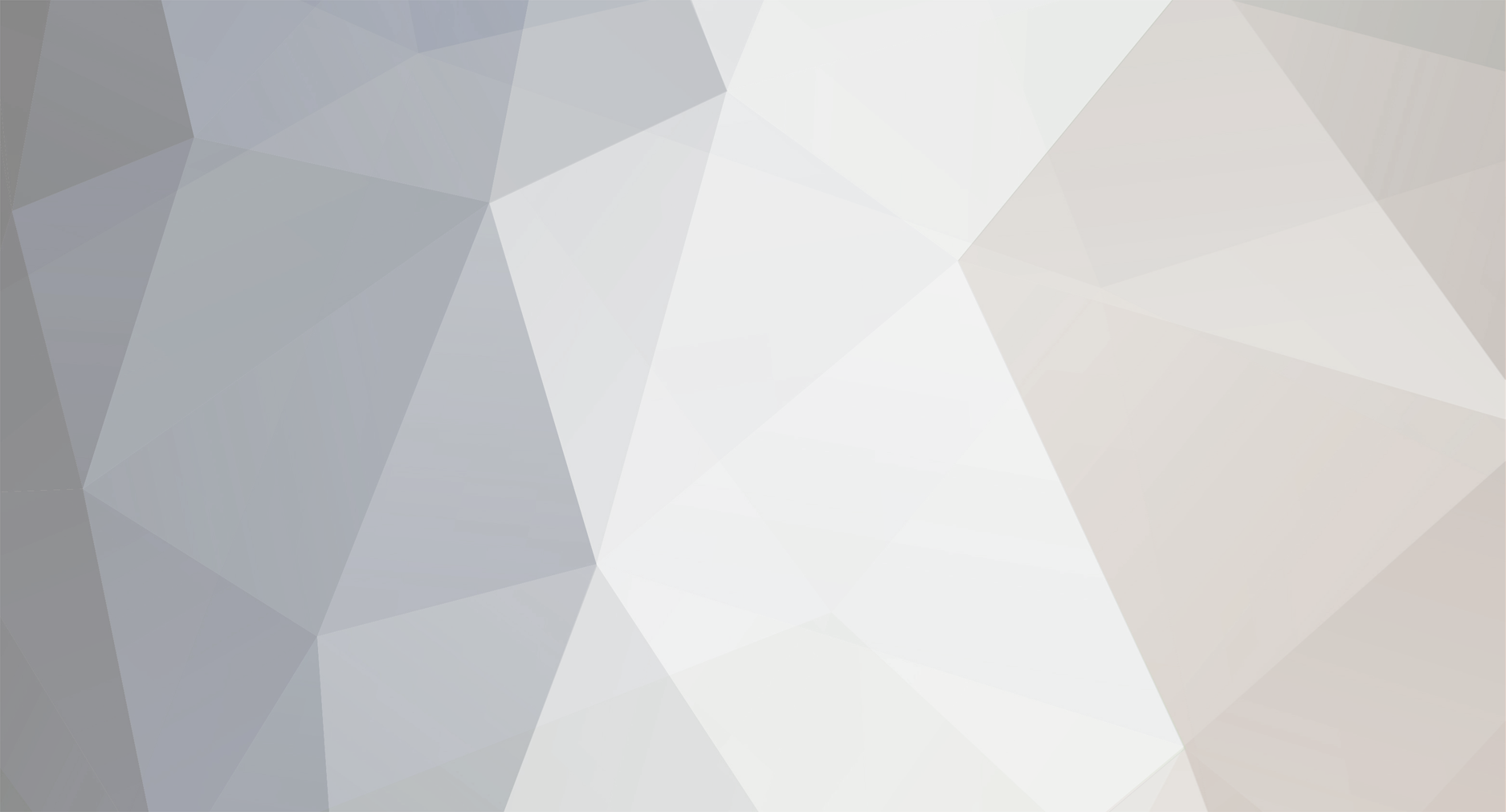
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
So, use the code I gave you but only use the $label or only use the $price or use both. Personally, I dump the hole thing into an array using the name as the key and the price as the value. Then you can loop over that array as needed $temp_array = array(); foreach(explode(',', $forfait) as $item) { list($price, $label) = explode('|', $item); $temp_array[$label] = $price; } //Now use a foreach() loop on $temp_array as needed
-
Looks like your connection to the DB is failing. If it worked before I see no reason it would not work with the modification I provided.
-
If you are going to use regular expression, I already gave you a single line solution that works: function wordCount($string) { return preg_match_all("#\b[\w]+\b#", $string, $matches); } Or, if you don't want to use a function $words = preg_match_all("#\b[\w]+\b#", $string, $matches); As I stated above this counts anything that is a-z, A-Z, 0-9 and underscore as possible words. If you want to expand the list of characters (or instead use a black list) that is easy to modify as well.
-
Really? Using THAT explode should give you: 158 Group Session,95 Group half session,45 Private Course,90 zumba course This will format the data as you request and you can reformat the output to be a select list option, but I don't know what you would make the value foreach(explode(',', $forfait) as $item) { list($price, $label) = explode('|', $item); echo "{$label} ({$price}$)<br>\n"; }
-
The bottom line is that salting will prevent anyone from brute forcing the password if they do not know what the salting is. Which is also why the salt should be unique per user. If the system is public, a cracker would almost more than likely create their own password in the system. Then if they were to get ahold of the database (and not the logic for salting) they could run some trial and error on the hash of their own password to try and figure out the salt. If they figure out the salt for their password using values only in the database, then they can determine the salts for all the users. So, it's not a bad idea to have a little salt and pepper (pun intended) whereby you use a variable value from each user (e.g. timestamp of when they signed up) AND a static (or semi-static) value that is determined in the code. One of the early examples in this thread was to use modulus on a value to determine the hashing method. Although not strictly a salt, it adds a level of variability that someone with access to only the database would not easily know. But, you could just as easily use something such as a math function against the timestamp or anything so that the hacker could not easily determine the salt from just the database. I'll sometimes use strrev() on the password in addition to the salt. If a hacker gets both the database and the logic then all bets are off. They can, with sufficient time and resources brute force any of the hashes. Which is why string-lengthening is important.
-
Did you validate that the query is returning results as Maq suggested? If the query can legitimately have no results then you need to define $data as an empty array as I provided in the code I posted above.
-
Well, it all depends on what you consider a word. Looking at what you have it appears that you want any group of characters that are separated by any number of spaces or hyphens to be considered words. You could use string functions or possibly regular expressions. But, as you saw with the string functions you can't (directly) account for when there are any number of multiple spaces. So, one solution would be to use a loop that continues indefinitely as long as there are any consecutive spaces. function wordCount($string) { //Replace hyphens with spaces $string = str_replace('-', '', $string); //As long as there are double spaces - replace with single spaces while(strpos($string, ' ')!==false) { $string = str_replace(' ', ' ', $string); } return count(explode(' ', trim($string))); } For some reason I think there has to be a built in function that would be more appropriate, but I can't think of it at the moment. Also, what about other "non printable" characters? Or, what if there are punctuation characters by themselves? If you want only alpha-numeric characters to be used as possible words, then a regex solution is probably better. function wordCount($string) { return preg_match_all("#\b[\w]+\b#", $string, $matches); } In the above, the characters a-z, A-Z, 0-9 and the underscore can make up words.
-
Ah, I didn't catch that. He's got an "or die" clause on the query - so it isn't failing. Just isn't' returning a result set - which may be valid. I'd suggest defining the array beforehand so that at least an empty array is returned when there are no results. But, on second inspection I see he is adding the results to "$data1", but is returning "$data" ! public function get_new_flows($level) { $last_flow_id = $_POST['id']; $query = "SELECT F.flow_id, F.user_id_fk, F.flow, F.time, U.name, U.facebook_id FROM flows F JOIN users U ON F.user_id_fk = U.user_id WHERE F.flow_id > '$last_flow_id' AND F.level = '$level' ORDER BY F.flow_id DESC " $result = mysql_query($query) or die(mysql_error()); $data = array(); //Define array while($row = mysql_fetch_array($result)) { $data[] = $row; } return $data; }
-
You must be defining $flowsarray somewhere before you are are including load_flows.php (which is why it does not create an error) and you must NOT be defining $newflowsarray before including load_new_flows.php - or it is not defined as an array (which is why it is failing). I'm guessing there is something in one of the other included files that is defining $flowsarray
-
Did you read my first response and the follow up code I provided? I pointed out the error and I provided some code that would solve your problem. As for the missing line of code, no it does not explain what the block of code was that I indicated. You have a succession of if() statemetns that in turn define other variables - but you never use those variables in your output. That is why I stated that I do not understand what that block is for. To be more specific you are, depending on the value of $fuel, defining the variables: $fuel1_total, $fuel2_total, $fuel3_total, $fuel4_total, $fuel5_total, $fuel6_total, $fuel2_pollution, $fuel3_pollution, $fuel6_pollution. But, those variables are not used at all in the output. If you need those values elsewhere in your code you are going to need to save them to an array instead of redefining the save variable over and over in the while() loop.
-
I think I see the problem. Check your variable names, specifically the "case" of the letters. foreach ( $myarray as $lineitem ) { echo($lineitem->getName()."~"); echo($lineItem->getOId()."~"); echo($lineItem->getProduct()."~"); echo($lineItem->getPIP()."~"); echo($lineItem->getQuantity()."~"); The foreach loop, and the first usage, uses $lineitem, but the other usages of the variable have $lineItem
-
<?php $db_hostname = "localhost"; $db_name = "dbname"; $db_username = "joeblow"; $db_password = "password"; $cxn = mysql_connect($db_hostname,$db_username,$db_password) or die ("Could not connect: " . mysql_error()); mysql_select_db($db_name); $sql = "SELECT output, fuel, numunits, capabilityfactor, pollution FROM db1 GROUP BY fuel ORDER BY sum(output) DESC"; $result = mysql_query($sql, $cxn) or die ("sorry, try again"); //Generate the output into a variable $output = ''; while ( $row = mysql_fetch_assoc($result)) { extract($row); $capfactor = number_format($capabilityfactor, 2) * 100; $waste = number_format($pollution); //Not sure what this block is for since none of these variables are used if ($fuel == "fuel1") $fuel1_total = $output; if ($fuel == "fuel2") {$fuel2_total = $output; $fuel2_pollution = $pollution;} if ($fuel == "fuel3") {$fuel3_total = $output; $fuel3_pollution = $pollution;} if ($fuel == "fuel4") $fuel4_total = $output; if ($fuel == "fuel5") $fuel5_total = $output; if ($fuel == "fuel6") {$fuel6_total = $output; $fuel6_pollution = $pollution;} $output .= " <tr>\n"; $output .= " <td>{$fue}</td>\n"; $output .= " <td>{$numunits}</td>\n"; $output .= " <td>{$foutpu}</td>\n"; $output .= " <td>{$capfactor}</td>\n"; $output .= " <td>{$waste}</td>\n"; $output .= " </tr>\n"; } ?> </head> <table border="1"> <tr> <th>Fuel type</th> <th>Number of units</th> <th>Output</th> <th>Capability factor</th> <th>Pollution, in tons<sub>2</sub> emitted</th> </tr> <?php echo $output; ?> </table> <body> </body> </html>
-
Your while loop is simply redefining variables on each iteration. You don't DO anything with those variables until AFTER all the rows from the result set have been processed. Thus, you are only displaying the last record since the data from the first rows have been overwritten.
-
Um no, you should always write clean, organized code. Otherwise trying to fix problems can be much more work than it needs to be and you end up spending much more time "cleaning up" your code than it would have taken to be more methodical in your original coding. Or, even worse, you will end up with "hidden" bugs that will come back to haunt you later. Anyway, your problem is pretty obvious while ($loop <= $num_queries - 1) { $query = $store[$loop]; <== Query is defined in the loop if (!mysql_query($query)){ // <== Each Query is run here within the loop die('Error: ' . mysql_error()); } mysql_query($query); // <== LAST query from the loop above is run here again Besides you should NEVER run queries in loops. Gather all the data for your INSERTS and run one INSERT query.
-
You should NEVER run queries in loops. It is horribly inefficient. In this case it probably wouldn't be an issue, but it is poor practice and, besides, there is a much, much easier solution. Your logic above is flawed in that you are trying to run array_diff() on ONE record id instead of all the ids returned from the query. You would need to run through ALL the records THEN use array_diff(). But, as I said above, this is all completely unnecessary since you can run your deletes with ONE query using the IN clause. And, you should do all your INSERTS with one query as well. Here is a complete rewrite that is much more efficient (and safer from SQL injection). Not tested: if (isset($_POST['submit'])) { require ('db.php'); $plant_id = intval($_POST['plant_id']); //Create an array of values that is DB safe //array_map applies intval() to each element and array_filter() removes empties or 0 elements $edible_ids = array_filter(array_map('intval', $_POST['option2'])); if(!count($edible_ids)) { echo "No valid IDs passed."; } else { //Create array of INSERT values $insertAry = array(); foreach($edible_ids as $edible_id) { $insertAry[] = "({$plant_id}, {$edible_id})"; } //Create ONE insert query //Use INSERT IGNORE instead of ON DUPLICATE $query = "INSERT IGNORE INTO plant_edible_link (plant_id, edible_id) VALUES " . implode(', ', $insertAry); $result = mysql_query($query) or die(mysql_error()); //Create ONE query to DELETE $query = "DELETE FROM plant_edible_link WHERE plant_id = {$plant_id} AND edible_id NOT IN (" . implode(', ', $edible_ids) . ")"; $result = mysql_query($query) or die(mysql_error()); } }
-
Good catch! I didn't see that before. Also, I would highly suggest changing the logic for your validations and the resulting error handling. Your validations always check for a "valid" result, then proceed to the next (embedded) validation. This makes it VERY difficult to debug your code. One, because of all the embedded statements and Two, because it is hard to match up the error message to the validation. Instead, I find it easier to check for the invalid condition so I can put the error message where the validation takes place. For example: if (!$getuser) { $errormsg = "You must enter your username to register."; } elseif (!$getemail) { $errrosmg = "You must enter your email to register."; } elseiff (!$getpass) { $errormsg = "You must enter your password to register."; } //Continue all validations else { //All validations passed, do something } However, there are several problems with the WAY you are doing your validations, but one thing at a time.
-
Well, here is where you define $dbpass $dbpass = $row['password']; So, I'm guessing 'password' is either blank or doesn't exist int eh result set. Your code is very sloppy and will result in these kind of problems. For example, here is where you do the insert mysql_query("INSERT INTO users VALUES ('', '$getuser', '$password', '$getemail', '0', '$code', '$date')"); First of all, you should always create your queries as string variables so you can echo them to the page for debugging purposes. Second, you are not specifying the fields for the values and are instead relying upon the order of the fields. That is what I mean by sloppy. If you ever need to make changes to your db structure in the future you may have many different pages to go update. Instead you should specify the fields and then the values. Then you wouldn't need to pass an empty value for fields that you don't have a value for like you are doing with the first field. Anyway, do a print_r($row) to see what is returned from the query.
-
The Option name cannot be retrieve after calling $POST
Psycho replied to shebbycs's topic in PHP Coding Help
You are passing the value from the field "ipdi" print "<option value='$getips3[ipid]'>$getips3[iP]</option>"; But, then you are using that value to try and delete a record where the field 'IP' is equal to that value $unban="Delete from ipbans where IP='$ipselects'"; So, you would not get the results you want even if you didn't get a blank page. -
Yeah, there probably is a way to "simply" add the functionality you were asking for. The problem is that your current logic is terrible and I (nor probably anyone else) can figure out what to put where. If your logic is so complex that YOU don't understand where to implement a simple if() statement, then the logic is flawed and/or overcomplicated. Again, you don't need to rewrite everything from scratch. Just identify the blocks of code that perform specific functions and put them in a logical order. I already gave you an example to follow.
-
You really need to take a look at a tutorial for JOINS because this is as simple as it gets. SELECT * FROM cart JOIN products USING (Prod) WHERE cartID = $cartID
-
Well, yeah. You start $i at 0, then decrease the value by 1 on each iteration. So, on the first loop $i = 11 (December) on the next loop it equals 10 (November), etc. So, logically if you want to start at January you need to start with 0 and go to 11. The order of the case statements has no bearing on what you are trying to do. If you didn't use the "break" on each case statement, the order of the case statements has an impact - but you don't want to do that for your problem. So, you need to start with 0. That should be pretty easy to change, right? Just use $i = 0; Then, since you want to go UP to 11, you need to change the line that decrements $i to instead increment it by one. That's simple too $i++; Now, the only problem is that you need to change the while() loop condition. Currently it is while($i >= 0). But since we are starting at 0 and incrementing, that condition would never return false, so the loop would continue forever. So, if we start from 0 and go up, when would you want the loop to exit? You could write the while condition to be while $i is less than or equal to 11 or just use while $i is less than 12. I'll let you fix the actual code. Just look at the link I provided previously for the proper format.
-
You're not getting any errors, because the script is never completing. You have a loop that will continue to run as long as $i is greater than or equal to 0. $i starts with a value of 11 and you do have a line of code to decrement $i. But, look where you put that line! It ONLY gets executed for case 3:. Therefore, the line to decrement $i never gets executed and $i retains its value of 11 and the loop will run continuously. You need to put the $i--; where it will get executed on each iteration of the loop - i.e. after the switch block of code which ends with the first "}".
-
Your comparison operator in the while condition is incorrect. "=>" is a type of assignment operator. You want to see of the value is "greater than or equal". You'll find the correct comparison operator here: http://php.net/manual/en/language.operators.comparison.php Also, your switch() is using a colon (":") to indicate the start of the case statements for the switch which is the alternative syntax for control structures (http://www.php.net/manual/en/control-structures.alternative-syntax.php), but you never close it. Look at the examples for the switch statement. Most use the curly braces for enclosing the case statements (which is the primary method for defining control structures). So, you can use curly braces or look at the example that uses the colon at the beginning and look for the correct way to terminate the block. http://php.net/manual/en/control-structures.switch.php
-
Is there a way to not print Headers and Footers?
Psycho replied to Matt Ridge's topic in PHP Coding Help
Right, if you are talking about the headers/footers that the browser inserts the answer is no since PHP has no ability to control what the browser does with the rendered page. However, if you are talking about headers/footers that YOU are putting on your web page and you don't want them displayed if the user prints the page. Then you can use the "@media" control within CSS to define different styles based upon whether the page is viewed in a browser or printed. Just set the display property to none within the "print" media type for those elements you do not want displayed in a printed page. -
Have you looked at the manual for the for() and while() loop controls? There are detailed descriptions and example scripts. Again, you need to at least show an attempt before we will help you with homework.