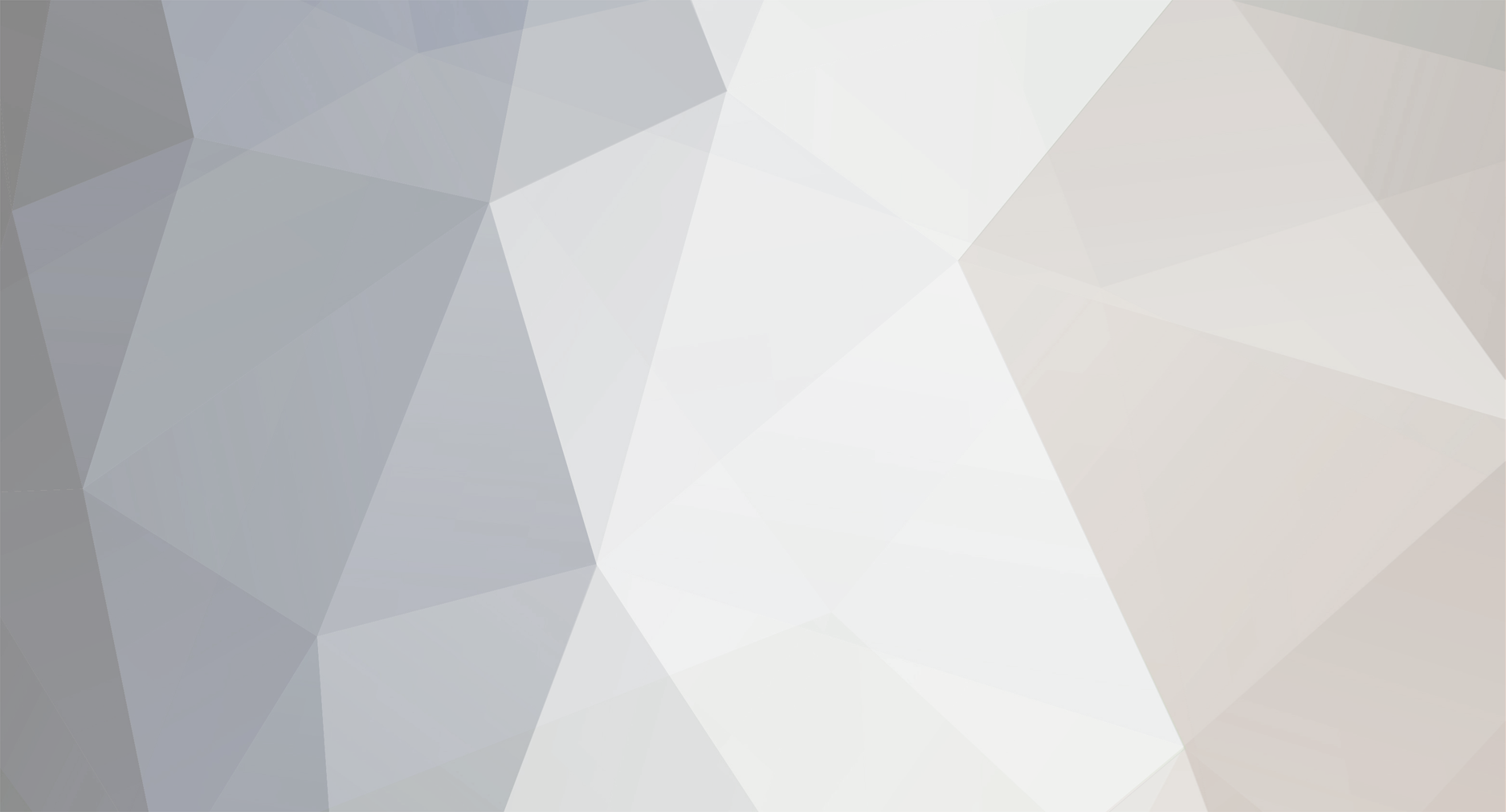
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Decide what solution you want to implement and, well, implement it. Are you wanting someone to write code for you? I can understand that you might not know what possibilities exist in order- which prevents you from being able to search for resources. But once you know what they are you can make a decision based upon the pros/cons of the possibilities and then search for additional resources. Then come back and ask questions if needed. YOU need to decide what you are going to do and implement accordingly. There is no bulletproof solution and some decisions need to be made. Neither I nor someone else can make those decisions for you.
-
No need to build separate pages for your "mobile" pages. The CSS standard has the ability to define different styles based upon the "media type". So, you could define a page to display differently based upon whether it is being displayed on a PC, a mobile device or when printed. For example, I will routinely define the styles for navigation sections to be "display:none" when the media type is print. No need to display the nav section in a printed page since the user is interested in the content. But, apparently not all mobile devices/browsers correctly define their media type, so you can go the route of maintaining separate pages. More info here: http://webdesign.about.com/od/mobile/a/detect-mobile-devices.htm
-
Splitting a string on (only) the last instance of a needle
Psycho replied to stubarny's topic in PHP Coding Help
Well, after looking back at the requirements I see that the "string" processes I built and the one you provided, as well as the regex function, would not work. The requirements are that the string being searched for is the last occurrence which starts with needle one AND ends with needle 2. The above string processes don't even look for needle 2. I didn't even test with sample data that includes needle 1 after the actual text being sought. Both my functions, and yours, would fail. You could still create a process using only string functions, but it would be more involved and probably include loops. Working from the back, you would have to find an instance of needle 1 that precedes an instance of needle 2 AND ensure it doesn't include a double quote between those two positions. However, I was able to make one simple change to the regex to find the target value with certainty function findTarget($subject) { $pattern = '#</span></a> <a href="([^"]+)" rel="nofollow"><span class=pn><span class=np>Next »</span></span></a></div>#'; $result = preg_match_all($pattern, $subject, $matches); if(!$result) { return false; } return array_pop($matches[1]); } Yes, it could be done with just string functions, but would be more complicated than the above. When it is all said and done, I don't think that .000004 seconds on a single operation is worth getting worked up about. If you are so inclined to try and build an operation of just string functions that meets the requirements, here is an input that would find the wrong text with the previous functions. The previous functions would find the text "THIS_IS_A_TRAP" instead of the correct text "CORRECT_TARGET". $subject = '</span></a> <a href="NOT_THIS_ONE" rel="nofollow"><span class=pn><span class=np>Next »</span></span></a></div></span></a> <a href="NOT_THIS_ONE_EITHER" rel="nofollow"><span class=pn><span class=np>Next »</span></span></a></div></span></a> <a href="CORRECT_TARGET" rel="nofollow"><span class=pn><span class=np>Next »</span></span></a></div>'; $subject .= '<a href="somesite.htm"><span>Hyperlink Text</span></a> <a href="THIS_IS_A_TRAP">other</a>'; -
Splitting a string on (only) the last instance of a needle
Psycho replied to stubarny's topic in PHP Coding Help
I am not saying regex is a substitute for string functions. but in this case it would take several string functions to get the value. First you have to find the position of the last instance of needle 2, then find the instance of the end of needle 1. Lastly, you would get the value by using substr() using the position of needle 1 (+ the length of needle 1) as the start position, then you have to calculate the start of needle 2 minus the start position. Seems like an awful lot of work for something I can do in one line - even if it isn't the most efficient function. In fact, just to see what the performance hit would be I built two solutions, one using Regex and the other with string functions. The string solution was about 2x as fast as the regex solution. But, we are talking very, very miniscule amounts of time. For 10,000 iterations the string function was averaging around .04 seconds of a second while the regex solution took about .08 seconds. So, the performance benefit is definitely there for string functions. But, the string solution took my much longer to put together and involved several steps. More steps mean more potential for bugs and regressions. -
Splitting a string on (only) the last instance of a needle
Psycho replied to stubarny's topic in PHP Coding Help
Or, learn how to program like the big boys and use regular expresssions $pattern = '#</span></a> <a href="([^"]+)" rel="nofollow"><span class=pn><span class=np>Next »</span></span></a></div>$#'; $output = preg_match($pattern, $subject, $matches); $lastMatchedValue = $matches[1]; -
1. Create a table to store the activity in. You'll need columns for at least 'ip' and 'page'. NOTE: You can have many users with the same IP if they are behind a router using NAT. So, you can't be sure that each IP is a unique user or not. Anyway, on each page load you will want to insert and/or update records in the table with the ip of the user and the page they requested. So, you could also use a session or cookie value to try and identify separate users on the same IP. Each has some benefits and drawbacks. Cookies can be deleted by the user or the user might not even accept cookies. But, on the other hand, cookies can be set to persist over time so they will be available over different sessions allowing you to track users consistently. Sessions cannot be modified by the user, but they also expire when the browser is closed. So a user who access your site, closes the browser and re-accesses the site will show two different users. If think you will ONLY ever want the current page of the user, then you would use an "INSERT ON DUPLICATE KEY UPDATE" query. That way if there is already an entry for that IP the previous entry will be overwritten by the new one. However, if you think that the historical data will ever be needed, then always do an insert. You can get the users most recent activity by applying the correct ORDERing and GROUPing logic in the query.
-
Well, if those are the ONLY values you want to check, then what you have will suffice. You could use modulus to check for each 5th instance, but then you need to add minimum and maximum validations. So the number of validations is not that different if($num>=1 && $num<=21 && $num%5==1) { Even though that only requires 3 checks as opposed to your original of 5, I would prefer your original code for the sake of readability. I can easily see what numbers the validation allows looking at your original code, whereas for the one using modulus it take some mental calculations. But, if you have a much wider minimum/maximum values then modulus would make sense.
-
preg_match is always returning true, problem with UTF-8?
Psycho replied to SuperBlue's topic in PHP Coding Help
Yes, that will work but, typically, if you are wanting to ensure that all characters are within a defined set a more efficient expression is to look for any characters NOT in the defined set. Just put the carat ("^") inside the character class to indicate any character not in the class. But, in this logic a true means disallowed characters were found, so you want to check for false result if (!preg_match("/[^a-zA-Z0-9]/", $_POST['title'])) { echo $_POST['title'];exit(); } -
preg_match is always returning true, problem with UTF-8?
Psycho replied to SuperBlue's topic in PHP Coding Help
The regular expression will return true if there is AT LEAST ONE alphanumeric character. All the other characters can be anything. So, "A!@#$%^&*()" will return true because of the letter "A". -
Well, if you would have followed directions you wouldn't have had that problem. The regex I supplied was defined using double quotes around the string for a reason - so you wouldn't need to escape the single quotes inside the string.
-
Near the top of the script you attempt to connect to the database before you even check if it exists $select_db = mysql_select_db($db); Then later - after you have created the database if it did not exist - you reuse the variable $select_db to detemine if you show the connection error - instead of reattempting to connect now that the database exists. After you create the databse you need to then attempt to connect to it again.
-
I don't get you. If there are only two records in the array what do you expect to happen after the two records have been processed? The code I provided with process each record one at a time and do the following: 1. If the value of 'amt' is >= the attack, then the attack value id deducted from that record's 'amt' and the script ends. 2. If the value of 'amt' is less than the attack, then that amount is deducted from the attack and the 'amt' for that record is set to 0. The loop then repeats so the remaining attack can be processed against the next record(s) If you want something different you need to provide more details. FYI: There was a small bug in what I provided. Here is the correct line: foreach($array as &$value)
-
Not that hard really $attack = 300; foreach($array &$value) { if($value['amt']>=$attack) { //There is enough in this record to subtract all of the attack //Remove the attack amount and exit loop $value['amt'] -= $attack; break; //exit the foreach loop } else { //Remove the amount for this record from the attack and set amount to 0 //Repeat loop for next record $attack-=$value['amt']; $value['amt'] = 0; } }
-
That line is not the problem. You have at least a couple of "}" that do not belong. Keep your code formatted/indented as per the logical structure of the code (as I provided earlier) so you don't make these mistakes.
-
elseif(isset($_COOKIE['geolocation'])) { $lang = $_COOKIE['geolocation']["countryCode"]; }
-
You were already given the solution by requinix: Use single quotes $replace = '{{$1||$2||$3}}'; or escape the dollar sign $replace = "{{\$1||\$2||\$3}}";
-
It's right there in the array under the key "countryCode". Use that value in your code to determine the language to use based upon country code. Also, you should change your switch() to convert the value to lowercase because "GR" != "gr" switch (strtolower($lang)) {
-
Forum Rules: Well, YOU need to determine what the trigger or triggers will be. You can't just redirect them without some sort of specific rule - otherwise the redirect will be caught in a loop. Do you only want to redirect if the language isn't set in the session value in the cookie value, what? If you have a means of allowing the user to select their language you don't want to override their selection based upon their location. So, you need to come up with the logic that makes sense for your implementation, then we can provide some guidance on code. But, for the sake of argument, you could simply add another ifelse() if(isSet($_GET['lang'])) { $lang = $_GET['lang']; // register the session and set the cookie $_SESSION['lang'] = $lang; setcookie("lang", $lang, time() + (3600 * 24 * 30)); } else if(isSet($_SESSION['lang'])) { $lang = $_SESSION['lang']; } else if(isSet($_COOKIE['lang'])) { $lang = $_COOKIE['lang']; } elseif(isset($_COOKIE['geolocation'])) { $lang = $_COOKIE['geolocation']; } else { $lang = 'en'; }
-
Doesn't the common.php file already handle that? What problems/errors are you facing?
-
Display latest 10 results on search page before searching
Psycho replied to bruisr's topic in PHP Coding Help
No, you would ORDER the results by their date and then use LIMIT to get only 10. At least if you want the last 10 records you should have a date. Otherwise you could use the ID value - but that is not the proper method. Just replace 'date' with 'id in the provided query below. The following fixes a lot of problems with your code that I'm too lazy to go into right now. But, let me know if you have any questions <?php //Define count of records to show by default $recentResultLimit = 10; require_once('dbconnection.php'); mysqli_select_db($conn, $dbname); //Create and run query if (isset($_POST['search'])) { $searchterm = mysql_real_escape_string(trim($_POST['search'])); $query = "SELECT * FROM locations WHERE loc_name LIKE '%{$searchterm}%' AND loc_approved != '0'"; } else { $query = "SELECT * FROM locations WHERE loc_approved != '0' ORDER BY date DESC LIMIT {$recentResultLimit}"; } $result = mysqli_query($conn, $query) or die(mysql_error($query)); //Generate message and output $message = ''; if(isset($_POST['search'])) { $resultCount = mysqli_num_rows($result); if($resultCount==0) { $message = "Sorry, but we can not find an entry to match your query: <strong>{$searchterm}</strong>"; } else { $message = "Your search for <strong>{$searchterm}</strong> returned {$resultCount} results."; } } else { $message = "Here are the {$recentResultLimit} most recent results."; } $output = ''; while($row = mysqli_fetch_assoc($result)) { $rentals = ($row['loc_gear'] == 1) ? 'Yes' : 'No'; $output .= "<tr>\n"; $output .= "<td><a href='loc_details.php?loc_id={$row['loc_id']}'>{$row['loc_name']}</a></td>\n"; $output .= "<td>{$row['loc_city']}</td>\n"; $output .= "<td>{$row['loc_state']}</td>\n"; $output .= "<td>{$row['loc_depth']}</td>\n"; $output .= "<td>\${$row['loc_fee']}</td>\n"; $output .= "<td>$rentals</td>\n"; $output .= "<td><a href='http://maps.google.com/maps?q={$row['loc_lat']},{$row['loc_lon']}' target='_blank'>View Map</a></td>\n"; $output .= "</tr>\n"; } mysqli_free_result($result); ?> <h1>Search for Scuba Dive Locations</h1> <form id="searchform" name="searchform" method="post" action="index.php"> <input type="text" name="search" id="search_bar" /> <input type="submit" name="submit" id="submit" value="Submit" /> </form> <span style="color: #333;">Search for the name of the location, ie: Devils Den or USS Oriskany.</span><br /> <table style="font-size: 14px;" border="0" width="650"> <tr> <td colspan="7"><p><?php echo $message; ?></p></td> </tr> <tr> <th>Location Name</th> <th>City</th> <th>State</th> <th>Depth (ft)</th> <th>Fees</th> <th>Gear Rentals</th> <th>Map</th> </tr> <?php echo $output; ?> </table> -
How to sum, emit and store result from a calculation
Psycho replied to u0867587's topic in PHP Coding Help
The problem was with this line: $sessionsAry[] .= array('SessionId'=>$row['SessionId'],'Mark'=>$row['Mark']); Should have used "=" instead of ".=" which means to concatenate strings. I made some other changes during the debugging process, so I'm not sure that was the only problem. Here is the updated code which I have tested with some mock data function outputModule($moduleID, $moduleName, $sessionData) { if(!count($sessionData)) { return false; } $markTotal = 0; $sessionsHTML = ''; foreach($sessionData as $session) { $sessionsHTML .= "<p><strong>Session:</strong> {$session['SessionId']} {$session['Mark']}</p>\n"; $markTotal += $session['Mark']; } $moduleHTML = "<p><br><strong>Module:</strong> {$moduleID} - {$moduleName} {$markTotal}</p>\n"; return $moduleHTML . $sessionsHTML; } $output = ""; $studentId = false; $courseId = false; $moduleId = false; while ($row = mysql_fetch_array($result)) { $moduletotal += $row['Mark']; $modulemark = (int)($moduletotal); if($studentId != $row['StudentUsername']) { //Student has changed $studentId = $row['StudentUsername']; $output .= "<p><strong>Student:</strong> {$row['StudentForename']} {$row['StudentSurname']} ({$row['StudentUsername']})\n"; } if($courseId != $row['CourseId']) { //Course has changed $courseId = $row['CourseId']; $output .= "<br><strong>Course:</strong> {$row['CourseId']} - {$row['CourseName']} <br><strong>Year:</strong> {$row['Year']}</p>\n"; } if($moduleId != $row['ModuleId']) { //Module has changed if(isset($sessionsAry)) //Don't run function for first record { //Get output for last module and sessions $output .= outputModule($moduleId, $moduleName, $sessionsAry); } //Reset sessions data array and Set values for new module $sessionsAry = array(); $moduleId = $row['ModuleId']; $moduleName = $row['ModuleName']; } //Add session data to array for current module $sessionsAry[] = array('SessionId'=>$row['SessionId'], 'Mark'=>$row['Mark']); } //Get output for last module $output .= outputModule($moduleId, $moduleName, $sessionsAry); //Display the output echo $output; -
How to sum, emit and store result from a calculation
Psycho replied to u0867587's topic in PHP Coding Help
The code I provided should do exactly as you say you want. There must be a bug, I can't debug the code since I don't have your database. The function outputModule() should only be called when there is a change in the module and that function should output the module (1 time) as well as all of the sessions associated with that module. -
Well, I would think that the src attribute of the image is not pointing to a valid image. Have you checked the rendered the HTML source and verified the value of the image attribute? Is it valid? EDIT: FYI you are providing a 'relative' path to the image. You may be running this from a URL that is not correct for that relative path.
-
As an aside, the code you have to build your queries is a bit overcomplicated. For example, this $in = '('; $or = ''; foreach ($prefix as $value){ $in .= "$or number LIKE $value%"; $or = ' OR'; } $in .= ')'; Could just be this: foreach ($prefix as &$value) { $value = "number LIKE $value%"; } $in = '(' . implode(' OR ', $prefix) . ')'; In fact, you use the same process several times, so I would make a function with appropriate parameters and just call it each time.