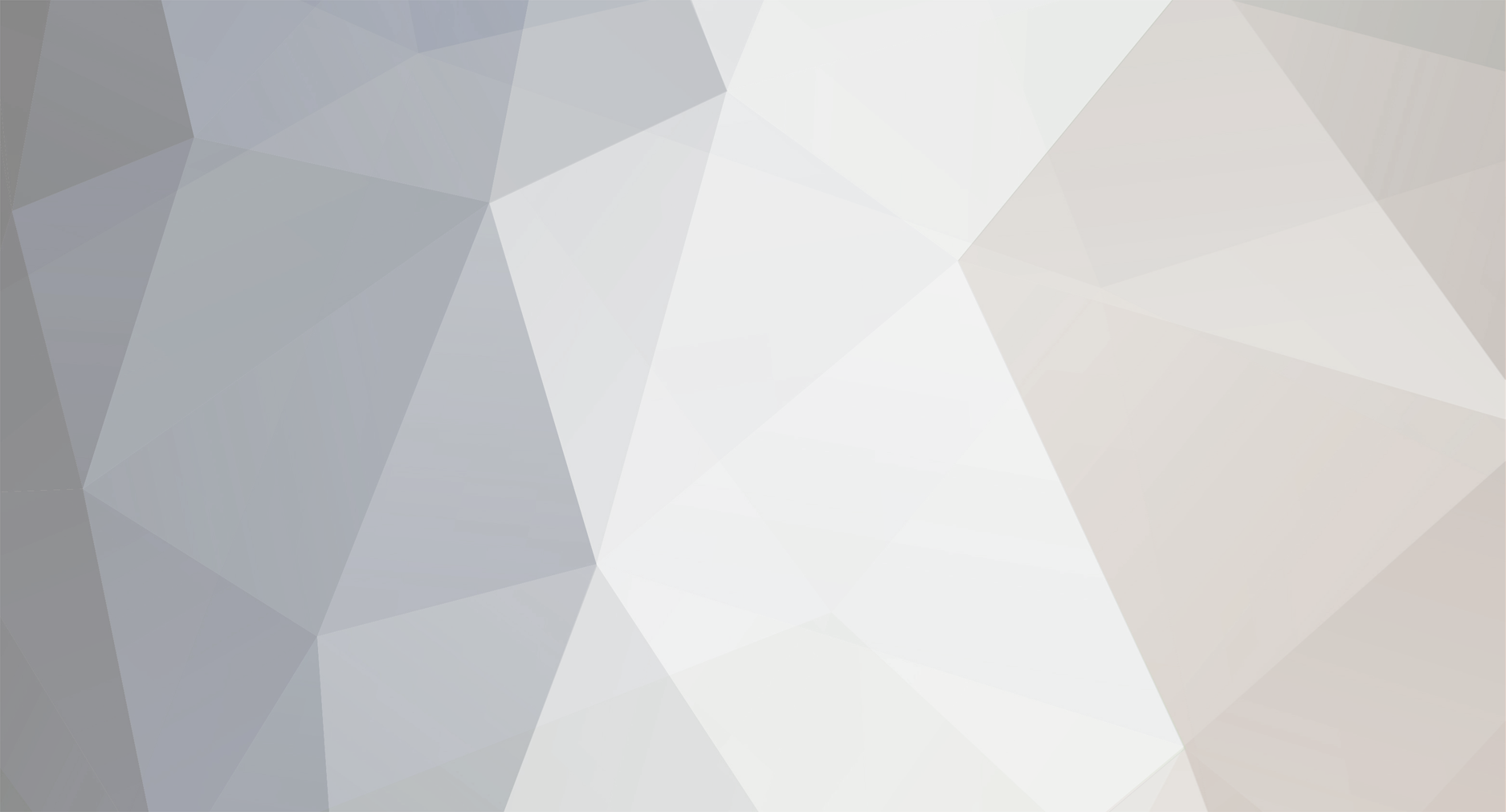
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
EDIT: Requinix beat me to it, but I'll leave this anyway as I think there is add'l information that might be of value. You can't JOIN a single csv list in one table with individual IDs in another. MySQL is 'trying' to compare the two values in your JOIN statement. I'm guessing the features ID is of a numeric type (i.e. an int) whereas the listing.features value is likely a varchar. Therefore, MySQL is doing type conversion to do the comparison. I'm guessing it is converting the listing.features value to an int and only the first value is left. You are going about this completely wrong. You should never store multiple values in a single field. It defeats the whole purpose of a relational database. You have a many-to-one relationship between the listings and feature. So, you need a third table to store the relationships. The "listings" table should store a unique id (listing_id) along with the basic information of the listing The "features" table should store a unique id (feature_id) along with the basic information of the feature Then you would have a third table 'listing_features' to associate a listing to multiple features. You would have a single record for each feature for a listing. It would only need two columns 'listing_id' and 'feature_id'.
-
OK< I have rewritten what you had. Here are some things to note: 1. You should pre-process the post data to trim the values. Otherwise a value of nothing but spaces would pass validation. 2. No need to use a $valid variable. Just check the count() of the $error_msgs array. 3. I would only run the DB check to see if the user had exceeded the daily limit if all the other validations passed. DB transactions are costly and should only be done if necessary. 4. You have two separate fields for date and time. You could use a timestamp field that is automatically populated when the record is created. Then you don't need to include it in the INSERT statement - it just happens automatically. If you do this, then you would use the follwing int he query to get the posts for the last 24 hours AND `date` >= DATE_SUB(NOW(), INTERVAL 1 DAY) 5. If you stick with separate fields for date and time, then you would use AND `date` = CURDATE() There might be some minor errors, but give this a try $allowed_posts_per_day = 3 if(isset($_POST['subComposeBlog'])) { //Preprocess post vars $category = (isset($_POST['Category'])) ? trim($_POST['Category']) : ''; $title = (isset($_POST['blogTitle'])) ? trim($_POST['blogTitle']) : ''; $body = (isset($_POST['blogBody'])) ? trim($_POST['blogBody']) : ''; //Create error array $error_msgs = array(); if(empty($category) || $category=='null') { $error_msgs[] = "Whoops! Please select a category for this blog."; } if(empty($title)) { $error_msgs[] = "Whoops! Cannot submit a blog without a title, how are you going to attract people's attention to read your masterpiece?"; } if(empty($body)) { $error_msgs[] = "Whoops! Cannot submit a blog without a body,that would not be a blog now would it?"; } if(count($error_msgs) == 0) { //Get number of posts by user in last 24 hours $query = "SELECT COUNT(`id`) FROM `cysticBlogs` WHERE `Author` = '{$auth}' AND `date` = CURDATE() AND `status` = 'active'"; $result = mysql_query($query, $connection) or die(mysql_error()); $post_count = mysql_result($result, 0); if($post_count > $allowed_posts_per_day) { $error_msgs[] = "Whoops! You can only write three blogs per day due to spam"; } } if(count($error_msgs) > 0) { //There were errors echo "The following errors occured:\n"; echo "<ul>\n"; foreach($error_msgs as $error) { echo "<li>$error</li>\n"; } echo "</ul>\n"; } else { //No errors, insert post $query = "INSERT INTO `cysticBlogs` (`blogTitle`, `blogBody`, `date`, `time`, `Author`, `Category`) VALUES ('" . mysql_real_escape_string($title) ."', '" . mysql_real_escape_string($body) ."', '" . date("Y-m-d") ."', '" . date("G:i:s") ."', '" . $auth->id ."', '" . mysql_real_escape_string($category). "'"; mysql_query($query, $connection) or die (mysql_error()); header("Location: BlogsSecurity.php"); } } EDIT: I just realized that you REALLY need to change how you are storing your dates. If you are using PHP to generate the dates, then you can't rely upon MySQL to run any queries using dates since the PHP server and MySQL server can have different date/time settings. Use MySQL to set and work with the dates.
-
Yeah, the problem is likely due to the fact that NOW() returns a timestamp (down to the second). You could try and manipulate the value of NOW() and your 'date' field to only be a month-day-year value, but there is an easier solution. Do your count based upon the 'date' being >= the date_sub() of NOW() - 1 day. Not sure of the exact syntax. Let me check. EDIT: IN addition to that your code to test the results isn't doing anything.
-
Cant get past IF statement to connect to database
Psycho replied to nander's topic in PHP Coding Help
Oh, sorry, forgot the 2nd parameter for print_r(). Use this: echo "Get Vars:<pre>" . print_r($_GET, true) . "</pre>"; -
Cant get past IF statement to connect to database
Psycho replied to nander's topic in PHP Coding Help
You should also put quotes around the array index. if (!isset($_GET['topic_id'])) { If you are still not getting past that if() statement, then put this before the if statement for debugging purposes to see what, if any, values are present in the global $_GET array echo "Get Vars:<pre>" . print_r($_GET) . "</pre>"; exit(); -
Hmm, I hate to state something contrary to your instructor, but that's a very poor 'solution' in my book. You should almost never 'mask' errors. You can simply use the isset() function to determine if a variable is set before trying to reference it. Look at the first section of code I posted. I didn't use if($_POST['op']) or if(@$_POST['op']), I used isset() to determine if the variable was set. The logic would use isset() to determine what value to use then set a value for $op. So, in the rest of the script you can use $op and not have to worry about suppressing errors. By suppressing errors you are going to create more headaches for yourself. You might have a situation, for example, where you are 'certain' that all your variables have values and something doesn't work. You are not going to get any errors that would have identified the problem so you will need to go through a tedious debugging process that may take a couple minutes or even hours depending on the situation. But, that's just my opinion. You can make your own choice.
-
How are we supposed to know? What is the value of $link? Did you verify it has the value you expect? Does the variable contain a value that is consistent with the database field (i.e. are you trying to store text into a numeric field)? Although, that last problem would likely create an error rather than inserting an empty field. Also, there is no need to include the 'id' in the insert query if it is an auto-increment field. Try the following $link = $m[1]; $ssql = "INSERT INTO `site` (`link`) VALUES ('$link');"; $result = mysql_query($ssql); //Debugging code echo "The value of the link: '{$link}'<br>\n"; echo "Insert Query: {$ssql}<br>\n"; if(!$result) { echo "Query failed: " . mysql_error();
-
I think you are making your conditions overly complex. For example, what is all of this: if( @$_POST && @$_POST['submitted'] && ( !@$_GET[ 'id' ] ) ) Why are you using the at symbol in there? Anyway, you need to simplify your logic. I suspect that code is the core logic for any editing you want to do for new articles: add, edit or delete - correct? Then you should only be performing one type of operation when the page is called. So, if you can detect that a delete operation is being requested, then you don't need to check that an edit request wasn't submitted. For each type of operation you should only need to check one value. I'll assume that deletes are done by clicking a link and that updates and adds are done via a form POST. I would always pass a variable to determine the operation to be performed (let's say 'op'). So, my delete link might look something like this <a href="somefile.php?op=del&id=12">Delete this news article</a> Then for my form(s) for update and add operations I would create a hidden field with the same name ('op') and would use 'add' or 'edit' as the value. Also, the edit form would require a hidden field for the 'id'. Then the page that handles all the operations can simply check the value of 'op' to determine what logic to perform if(isset($_POST['op'])) { $op = $_POST['op']; } elseif(isset($_GET['op'])) { $op = $_GET['op']; } else { $op = false; } if($op=='del') { //Perform delete operations } elseif($op=='edit') { //Perform Edit operations }elseif($op=='add') { //Perform Add operations } else { //No valid operation passed. Display error or display news list }
-
I think you need to rethink your table structure. If you have multiple members that are picking the same player in a given week, you should not store the score for that player in multiple records - only one. I would use a table (say picks) that stores each members picks of players with a column to identify the week of the pick. So you would store all their picks for the season. Then you would have a table to store the score for each player each week. You can then determine a members score for the week by making the appropriate JOINs. You could also easily pull up a form (or forms) to enter the players' scores based upon which ones were picked that week. Having multiple records for each member associated with a player and storing the players score in all of those is poor design. Here is what I would go with: Members member_id, member_name Players player_id, player_position, player_name Picks week_no, member_id, player_id Scores: week_no, player_id, score I really, really suggest you consider changing your design. But, if you don't want to you can easily add the score for each player to multiple records. Just run a SELECT query for the players that are picked that week in your temp table and do a group by the players. You should then have a result set of all the players picked that week. Take those results and build a form to enter all the players' scores. Player 1 <input type='text' name='score[15]' > Player 2 <input type='text' name='score[19]' > The '15' and '19' represent the ID's of the players which you will use in the update script. When the form is posted the update script will cycle through the $_POST['score'] array and get the player id and the player score for that week. I always advise against running queries in loops but I am too lazy to look up the right syntax for this right now and it would only be used for an admin to run once a week. So, just run an update query such as foreach($_POST['score'] as $playerID => $playerScore) { $query = "UPDATE table SET score = $playerScore WHERE player_id = $playerID"; mysql_query($query); } If you are storing the previous weeks data in the same table then you would only want to update those records for the player where there is currently no score, so you could add an addition parameter to the WHERE clause UPDATE table SET score = $playerScore WHERE player_id = $playerID" AND score = ''
-
PHP wouldn't introduce that (at least not to my knowledge). What are the exact contents of the URL when you get to that page? I have to assume the "?undefined" is in there. If this data is submitted from a form it could be caused by JavaScript on the form.
-
Which is the Most Efficient Way to Generate an Unique Random Number?
Psycho replied to Glese's topic in PHP Coding Help
OK, let's back up a second. You want some random number to use for the title of the contribution. Whether you do that or not, you should still use an auto-incrementing field for the records primary id. Using that, you can have the primary ID field as a relatively smaller int type than you would need for a random value. Which should result in better performance. So, as Pikachu2000 stated, it really doesn't matter if you do get a duplicate. The chances, as you already said, would be very small. No one is going to see some randomly generated title for a contribution and say "Hey I've seen that number used on a contribution from four months ago". But, since this value really would have no functional purpose, you have many options. You could do an MD5() or SHA() hash of the timestamp + userID, for example. -
No one has black listed you. I just looked at your other posts and they are, in my opinion, too general and vague which is probably why no one has responded. For example your post "Random Numbers" has been read over 100 times. In the post you ask for someone to explain how a quick pick option could be implemented and then post all your code. It is unclear from that post if you are asking about how to implement the option for a user to select a quick pick, how to actually implement logic to generate the quick pick, or what. When a question is vague like that and the user is unable or unwilling to specify the specific code section that applies to their question, many users are hesitant to respond. The posts can go on for pages because the helper is constantly having to ask the OP for clarification. And, in many cases, the OP is unable to implement anything without serious hand holding. In other words they become a lot of work. We all do this for free because we enjoy helping people. But, when some users sense that trying to respond to a certain post will become tedious and unfulfilling they simply choose not to respond.
-
I'm not sure I follow what you are needing. Part of the problem is that you are referring to "player" in several instances and it is not clear in each one if you are referring to the football players or the users. For example: If you are referring to the football player, I'm not sure what rows you are talking about. I would assume there would only be one row for each player each week. And, if you are referring to the users, then I am even more confused. There is no need to save records for each user's score each week. You already have the users tied to the football players, so you only need to store one record for each football player's score (each week) which is associated with the football player. You can then dynamically determine a user's score. I would think you need the following tables: USERS: A table to store basic information about the users PLAYERS: A table to store basic information about the football players USER_PICKS: A table to store each user's picks PLAYER_SCORES: A table that will hold one record for each player each week with the player's score. Then you can determine any user's weekly or total score with a single query. For example, the following is an example to get a user's total score for all of their selected players. SELECT SUM(score) FROM PLAYER_SCORES JOIN USER_PICKS ON USER_PICKS.playerID = PLAYER_SCORES.playerID JOIN USERS ON USERS.userID = USER_PICKS.userID WHERE USERS.userID = $userID GROUP BY USERS.userID
-
Which is the Most Efficient Way to Generate an Unique Random Number?
Psycho replied to Glese's topic in PHP Coding Help
Which is not random, but why do you need it to be random? Using an auto-increment column is the best way to ensure each record has a unique primary key. You should have a really good reason for needing a random unique number. The problem is that the more records you have, the larger you need to make the pool of possible numbers to ensure you don't get caught in a loop looking for an available value and the complexity will grow. -
I don't know why you would have two different functions with the same name. Perhaps one isn't even used? You could either: 1. Use the function I provided and call it wherever you are getting the price. echo customFormat($this->getPriceHtml($_product)); 2. Or you could modify the getPriceHtml() functions to implement the same logic But, there is a lot going on in those functions that I don't have any knowledge of and I don't have the time or the inclination to deconstruct the magento framework to figure out how to implement it in the function without breaking something else.
-
And, why aren't you using a foreach() loop?
-
You know, I was trying to be helpful and reach out with an olive branch after all the negativity in this thread and you just come right back with an attitude. The reason I didn't look through your code initially is that you simply posted too much without giving any context, no helpful comments, etc. As, I stated you shouldn't have to guess where the problem is if your code was written in a logical formal. Having poor logic doesn't necessarily mean something wont work. It could mean that the approach is so overcomplicated and superfluous that trying to fix a bug or add a feature is more complicated than it needs to be (such as this was). I'll admit some were a little overbearing in their response, but you have to take some responsibility for your own responses. Also, as I pointed out in the last code you posted you are completely duplicating the logic to determine whether the user won anything based upon whether they submitted their own numbers or a quick pick. That is the type of flawed logic I was referring to. Whether the user submitted their numbers or used a quick pick has no bearing on what and how they win. By duplicating the logic you are making it more complicated than it needs to be. You should determine the user's numbers (quick pick or submitted) - then determine their winnings.
-
Hmm, never used GROUP_CONCAT, I'll have to keep that in mind. However, you may need more than just the name. For example, listing the user names with each being a link to the user's profile page would be a common feature. In this case you would only need a simple JOIN. (Also, don't use * in your select queries unless you really need all the data - otherwise you are wasting resources). And, give yourself a fighting chance to debug errors by creating your queries as variables so you can echo to the page if needed. Anyway, the key to the logic is in the output process to 'detect' when there is a change in the parent record (in this case the photo) so you don't duplicate those values. This is only a little trickier since you want a count of users. The logic could look something like this [not tested]: function displayPhotoAndUsers($photo, $users) { $userCount = 0; $userOutput = ''; foreach($users as $user) { $userCount++; $userOutput .= "<li><a href='show_profile.php?id={$user['userID']}'>{$user['username']}</a></li>\n"; } $output = "<h1>{$photo}</h1>\n"; if(!$userCount) { $output .= "No users are using this photo.<br>\n"; } else { $output .= "There are {$userCount} users who are using this photo:\n"; $output .= "<ul>{$userOutput}</ul>\n"; } return $output; } $query = "SELECT p.photo, u.username, u.userID AS usercount FROM gallery AS g LEFT JOIN users AS u ON g.img_id=u.photo ORDER BY date_uploaded ASC"; $result = mysql_query($query); $photo = ''; while($row = mysql_fetch_assoc($result)) { if($photo != $row['photo']) { //This photo is different from last if($photo!='') //Skip 1st since there was no preceeding data { //Display data for last photo echo displayPhotoAndUsers($photo, $userData); } //Reset/create $photo var and temp user array $photo = $row['photo']; $userData = array(); } //Add user data for this photo if($row['username']!='') //Don't include nulls (i.e. no users have that photo) { $userData[$row['userID']] = $row['username']; } } //Display the last photo echo displayPhotoAndUsers($photo, $userData);
-
I think you are over-complicating this. I think I understand that you have some prices that can be fractions of a cent. But, it is not clear as ManiacDan has stated previously because your examples don't make sense. You state that .15 is less than one cent, but I interpret that as 15 cents. I agree with him that you shoudl display everything in dollars. So, $0.015 would represent 1.5 cents. So, in order to determine if you need two decimals or three just check of the value rounded to 2 decimals is equal to the original value. This seems to work for both strings and numeric values: function customFormat($amount) { $decimals = ($amount==round($amount, 2)) ? 2 : 3; return number_format($amount, $decimals); } //Unit Test $numbers = array(1.015, 1.1, 1.00, 1.10, 2, .005, '1.015', '1.1', '1.100', '1.10', '2', '.005'); foreach($numbers as $number) { echo "{$number} : " . customFormat($number) . "<br>\n"; } Results Orig. : Formated 1.015 : 1.015 1.1 : 1.10 1 : 1.00 1.1 : 1.10 2 : 2.00 0.005 : 0.005 1.015 : 1.015 1.1 : 1.10 1.100 : 1.10 1.10 : 1.10 2 : 2.00 .005 : 0.005
-
Where is this data coming from? If the data is in a database (where it should probably be) then you can get the results you need with an appropriately crafted query rather than looping through all the records like that.
-
I don't work with DOM elements in PHP. I would think there would be a way to get the count of elements in the object but couldn't find a way. If so, that would be the best option as you could simply start at whatever element you wanted. but, this will work. Simply skip the first n elements. /*** loop over the table rows ***/ $startRow = 48; // 49th row $rowNo = 0; foreach ($rows as $row) { if($rowNo>=$startRow) { /*** get each column by tag name ***/ $cols = $row->getElementsByTagName('a'); /*** echo the values ***/ echo $cols->item(0)->nodeValue.'<br />'; } $rowNo++; }
-
Do some research on mod rewrite
-
As I just stated we couldn't give you that kind of advice because we couldn't understand the logic. I only had a very vague idea of what you were trying to accomplish and I'm not going to take hours of my time reading your code line by line trying to interpret what you really meant to accomplish in order to try and provide that type of help. If you would have provided clear comments in your code to explain the logic that would have helped too. No one could tell you anything specific about what was wrong because it was just too haphazard. If you have a bad foundation where you want to build a home do you go ahead and build anyway or do you put in a new foundation? The same applies for programming. If you have bad logic to begin with and then try to fix something by "tinkering" with it you are way beyond anyone to help you with the code you have. You were given advice, which was to simply pull out a sheet of paper and map out some appropriate logic. I never said all of your code was crap and you needed to start everything over. Only that you needed a more logical approach. Your response was that you were just going to tinker with it. No, it isn't. The very high level flow I provided showed that you should determine the user's numbers (either user defined or quick pick - THEN you should perform the logic to determine the results of the user's picks. The code you last posted duplicated the logic for determining whether the user won or not. Go back and look at the high-level logic I provided previously. Again, you have some good code in there. You just need to take a step back, determine the correct flow for the logic and then reuse the code you have or implement new code where necessary.
-
The two conflicting examples you showed above are probably the reason that, accordingly to you, "this problem is not solved". There are just too many nuances in the English (and other) languages that this is an exercise in futility. You could probably implement some rudimentary logic to pose suggestions on "like" sounding tags, but anything further than that and you would be probably implementing limitations that will create more problems than they are solving. For example, what if you tried to limit plural entries as in the suggestion above. How do you know that "flowers" is supposed to be the plural of the word "flower" and not something else? One scenario that comes to mind is when "Jennifer Flowers" was all over the news back when Bill Clinton was running for president. What if there were a lot of articles relating to Jennifer Flowers and users attempted to enter tags for her last name? Yes, that is a manufactured scenario, but there would be many like that. At least that is my two cents.
-
I did tell you what was wrong with your code. It had no discernible logic - which was why no one could simply state "insert some code here". You had no idea what to do where because the logic was terrible. I even provided a sample high-level logic that would at least provide a jumping off point. As I stated previously, no one was stating you should just throw out all your current code. Most of it could be reused - you just needed to put it in a more logical order. Aside from rewriting it for you I don't know what you expect.