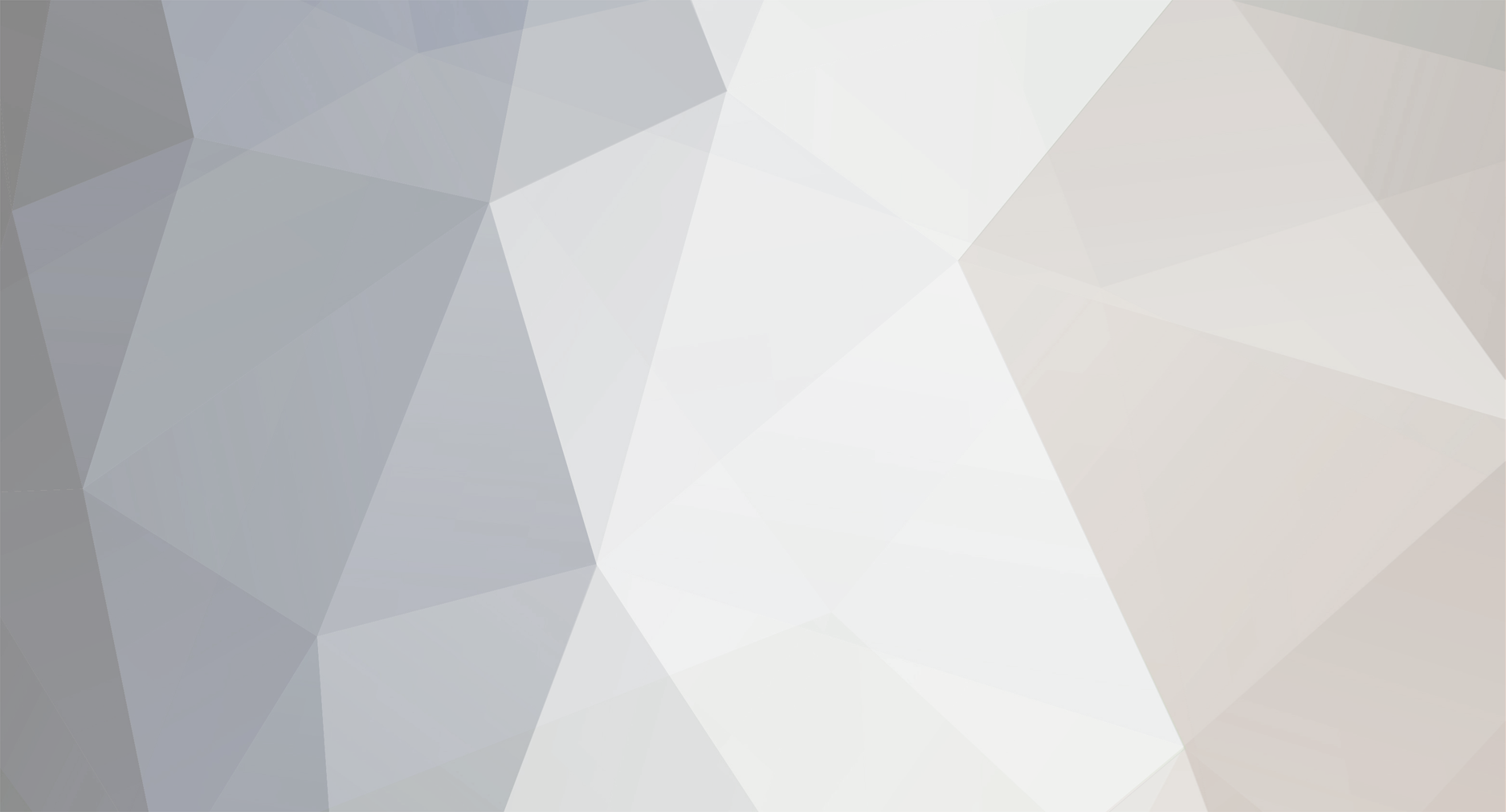
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
For a kill/death ratio (KDR) the 'death' is the divisor and is the only value that cannot be zero. However, if the 'kill' value is zero, then the KDR will be zero - which can be used in the calculation. So, there is no need to add special handling for the kill value. The problem you have is if the 'death' value is zero. In that instance there really is no KDR that can be calculated. As you get smaller and smaller values for death as they approach zero, the KDR will approach infinity. I have seen games that simply report no KRD when the user had no deaths or report a KDR based upon one death. I think the latter 'looks' better. But, this is a judgement call because when there are no deaths there is no real KDR value. So, to use a default of 1 death when the deaths are zero becomes a very trivial solution. if ($row['death'] == 0) { $row['death'] = 1; } $kdr = $row['kill'] / $row['death']; The only other - legitimate - options are to show no KDR (e.g. '--') or use something such as an infinity symbol (e.g. '∞'. That would look pretty 1337. But, you'd probably only want to use that if they had at least one kill. if ($row['death'] == 0) { if ($row['kill'] == 0) { $kdr = 0; } else { $kdr = '∞'; } } else { $kdr = $row['kill'] / $row['death']; }
-
You can change the field to be an auto-increment field without causing problems. Just determine the highest ID in use and set teh start counter above that.
-
http://php.net/manual/en/function.str-replace.php Although, you should not be doing this if your intent is to prevent SQL Injection.
-
PHP MYSQL Putting users into database using HTML form??
Psycho replied to huggies12345's topic in PHP Coding Help
Your errors are due to these lines $name = $_POST['name']; $regnum = $_POST['regnum']; You are getting an error because you have a higher error reporting level than the guy did in the video - which is a good thing. If you copied the code from the tutorial, then that tutorial is full of bad practices. 1. Always prevent against referencing variables that might not be set. 2. Don't reference variables from POST/GET unless you need them. In the code you provided you tried to define $name and $regnum before you even knew whether you would use them. That's a waste. <?php if(!isset($_POST['submit2'])) { //Form was not submitted $message = "Enter A Value"; } else { //Form was submitted $name = (isset($_POST['name'])) ? trim($_POST['name']) : false; $regnum = (isset($_POST['regnum'])) ? trim($_POST['regnum']) : false; if(empty($name) || empty($regnum)) { //Required fields were not set or were empty $message = "<span style='color:#ff0000'>Name and registration number are required</span>"; } else { //Required fields were present. Connect to database $host="localhost"; $username="root"; $password=""; $database="lab2"; mysql_connect("$host", "$username", "$password") or die(mysql_error()); mysql_select_db("$database") or die(mysql_error()); //Sanitize values and create/run query $nameSql = mysql_real_escape_string($name); $regnumSql = mysql_real_escape_string($regnum); $query = "INSERT INTO lab2('name', 'regnum') VALUES('$nameSql', '$regnumSql')"; $result = mysql_query($query); if(!$result) { $message = "<span style='color:#ff0000'>There was a problem adding the result</span>"; //Next line for debugging purposes only. $message .= "<br>Query: {$query}<br>Error: " . mysql_error(); } else { $message = "User Added To Database"; } } } ?> <html> <body> <?php echo $message; ?> <br> <form action="" method="post"> <p> Name: <input type="text" name="name"/> </p> <p> Regnum: <input type="text" name="regnum"/> </p> <p> <input type="submit" value="Add To Database" name = "submit2" /> </p> </form> </body> </html> -
No offense taken. I just gave you an outline of some of the things that need to be done. I don't have any sample code for this at my disposal, so the only way for me to provide anything would be to build it myself. There are many ways to accomplish this via PHP and MySQL and I'm sure there are some best practices. But, I think the most important decision is to normalize the times via PHP or MySQL. I would probably go with PHP since you can then re-purpose the code to work with another database if needed. PHP has a lot of built-in function for manipulating the timezone. I'd suggest reading through that section of the manual: http://www.php.net/manual/en/book.datetime.php
-
This forum is for people to get help with code they have written. If you want someone to write code for you, post your needs in the freelance forum and someone might be willing to do it for $$$. Have you even thought through what you would need to do and made an attempt? (I say this rhetorically because it is obvious you haven't). I'll provide some rough guidelines for you to build your solution. Then if you get stuck, post back with what you have and what isn't working. 1. Get the user timezone offset. You are already getting that value in the JavaScript code above. 2. Store the "current" datetime in the database using the database's timezone (Use NOW() or a field that automatically inserts the timestamp) or normalize everything to GMT see CONVERT_TZ(). Also, store the user's offset (i.e. +0800) 3. When you need to display a date time to the user, extract the datetime value from the database and convert to the user's timezone. You can do this in the query using CONVERT_TZ() or you can do it in the PHP code using strtotime() or another datetime conversion method.
-
Do not store the users date/time based upon their timezone. Instead, store all date/times based upon the server and also save a value for the user's offset. Then when you pull date/times for display use PHP to modify the value based upon the user's offset.
-
1. Do sanitize user input as appropriate before using in a Query 2. Do NOT use '*' in your SELECT queries unless you really need all the fields. 3. Do NOT use multiple queries when you can achieve the same thing with one. I.e. learn to do JOINs 4. DO create your queries as string variables so you can echo the query to the page for debugging purposes I would also advise not using spaces in table or field names. require_once('Connections/myConnect.php'); $detnum = mysql_real_escape_string(trim($_GET['Details'])); $query = "SELECT s1.* FROM `By Agency Store _` as s1 LEFT JOIN `Agency Stores - Table 1` as s2 ON s1.store_store = s2.F3 WHERE s2.F11 = {$detnum}"; $salesinfo = mysql_query($query); if(mysql_num_rows($salesinfo)) { //No matching records } else { //Do somethign with the results }
-
Did you even run the query I provided before trying to modify it? It was ALREADY ordering the results as you needed. All you did was complicate the query. The ORDER BY in the query I provided was being interpreted against the UNION results, not the individual tables results. To be honest, I didn't realize it worked that way until I tried it out.
-
So then in that case you'd use the sanitation script? I hate to sound dense, but when people say don't use some sanitation for some situations, but to use it in others, and not really say which is the best for which, I tend to get confused. Blogs, don't use htmlentities/htmlspecialchars, but at the same time use mysql_real_escape_string with the script a few pages back? Or no? This is what I mean by a standard. There has to be a way to sanitize script depending on what you are doing... THERE IS NO STANDARD BECAUSE THE APPROPRIATE METHOD OF SANITIZING A VALUE IS COMPLETELY DEPENDENT UPON HOW THE VALUE WILL BE USED. That is the reason you were advised not to use that sanitation script previously posted. Many people do create sanitation scripts such as that, but they are being short-sighted and possibly (probably) have bugs they aren't even aware of. You need to know "how" your variables are being used, "what" values could pose a problem and "implement" the necessary validation and sanitation processes to prevent those problems. This just doesn't apply to storing values in the database or displaying value in the webpage. It can apply to many different scenarios. For example, if you are using a value as the denominator in a division you need to ensure the value is not 0. I will state it again - there is no one size fits all solution. You need to understand the type of application you are building and have a grasp of the language you are working with. Then you need to use critical thinking skills to identify the potential problems and then use the correct process to guard against them. There are "best practices" that you need to learn, such as using mysql_real_escape_string() for any "string" values that you will use in a DB query. As an analogy, think about making dinner. There are no absolutes, but there are best practices. Do you always use a 3qt pot over high heat? No. Sometimes you need a bigger pot or maybe you need a pan. Hell, sometimes you don't even cook the meal. Programming is no different.
-
But you've already made it where it couldn't be more that 15 for initial input correct? The sanitation would add two more slashes to it, the final line as far as I read it is less than or equal to 17... so that would mean that it couldn't be beyond 17, no matter how many slashes are there if the value is greater than 17 it wouldn't accept it... Or am I reading that wrong? Are you for real? I'm starting to suspect that you are answering the way you are just to see how far you can take this thread. User inputs data into a field that should only be 15 characters long. The PHP code will accept that value and then VALIDATE that the value does not exceed 15 characters. If so, then it rejects the user input. If the value passes validation (and all other validations pass for the submission), then the value will be sanitized before being used in a query (the sanitation is dependent upon the storage method). In this cae we would use mysql_real_escape_string(). That function will, among other things, precede quote marks with a backslash. The backslash doesn't get added to the stored value in the database. It only ensures that the quote mark is interpreted as a quote match character instead of being interpreted as a control character for defining the query. So, a value of 15 quote marks would be 30 characters if you were to try and validate the length after sanitation. But, since that sanitized value won't actually be stored, it would be incorrect to use it for that validation. User Value | Sanitized Value | Stored Value --------------------------------------------- abcd abcd abcd abc ' 123 abc \' 123 abc ' 123 1'2'3'4'5'6 1\'2\'3\'4\'5\'6 1'2'3'4'5'6 '''''' \'\'\'\'\'\' ''''''
-
Here are a revised table structures and UNION query that works how you need it CREATE TABLE `calls` ( `id` int(10) NOT NULL auto_increment, `number` varchar(255) NOT NULL, `duration` int( NOT NULL, `call_date` datetime NOT NULL, `type` int(1) NOT NULL, PRIMARY KEY (`id`) ) ENGINE=MyISAM AUTO_INCREMENT=180 DEFAULT CHARSET=latin1 AUTO_INCREMENT=180 ; CREATE TABLE `messages` ( `id` int(9) NOT NULL auto_increment COMMENT 'internal id of the message', `user_id` int(5) default NULL COMMENT 'The UserID within this DB that that the message belongs to ', `thread_number` varchar(255) NOT NULL COMMENT 'The phonenumber of the thread that the message is part of', `message` text NOT NULL COMMENT 'The Message', `message_type` int(1) NOT NULL COMMENT '1: Send BY the other person, 2: Sent by YOU, the user', `message_date` datetime NOT NULL COMMENT 'The Date/Time in Epoch Format.. much easier for comparison', PRIMARY KEY (`id`) ) ENGINE=MyISAM AUTO_INCREMENT=16652 DEFAULT CHARSET=latin1 AUTO_INCREMENT=16652 ; SELECT message AS message_message, message_type, 0 AS call_duration, 0 as call_type, message_date AS record_date FROM messages WHERE thread_number = '8028816610' UNION SELECT '' as message_message, 0 as message_type, duration as call_duration, type as call_type, call_date AS record_date FROM calls WHERE number = '8028816610' ORDER BY record_date
-
Well, you should really never use '*' in your SELECT queries unless you really need everything. In this case you should definitely NOT use '*' when you have a UNION. If you ever need to update the columns for one of the two tables this query would break. Anyway, for UNION to work you must be SELECTING the same number columns and the columns (in the order given) must be of the same general type. So, if field 1 of the first SELECT is a varchar, then field 1 in the second SELECT should also be a varchar. You look to be violating the second condition. The first field in the first query is 'message' (which I would assume is a varchar or text type), whereas the first field in the second query is duration, which I would assume is a numeric type. Also, I don't know why you are creating the two queries as sub-queries. I don't know if that is also causing problems, but it is unnecessary. Plus, since you want the data ordered between tables (not independently in each table) the where clause needs to be made outside the union. Lastly, your field types are illogical. For one date field you are using a BigInt and for another you are using a varchar. Use one of the MySQL date type fields.
-
@Matt: You have a lot of enthusiasm and you are actually trying to learn (which is more than most people who visit these forums). But, the problem is that we cannot answer your questions in a way that you can understand because you apparently don't understand some of the basics yet. That's not meant to be mean, it's just an observation. We've tried to answer some of the questions in generalizations, but you are wanting specific details. But, if we gave you the details you wouldn't understand those either. Your last question is one of the most basic things, but you don't understand it. You can redefine a variable based on the current value of the variable $var = 1; //Var = 1 $var = $var +2 //Var = (1) + 2 = 3 $var = $var * 3 //Var = (3) * 3 = 9 $var = $var - 4 //Var = (9) - 4 = 5 echo $var; //Output: 5 As stated before "sanitizing" means many things: 1. Validating that the value is appropriate for what it is being submitted for. If you expect the value to be an integer, make sure it is an integer. If it should be a data, make sure it is a date 2. Escaping the value to prevent errors. This can be using mysql_real_escape_string() for data before it is used in a query or it could be running it through htmlentities() before echoing it to an html page. The appropriate Validation and Escaping are completely dependent upon what the particular value is and should be and how you are going to use it. I have seen many people use an all-in-one sanitize process - which is the wrong thing to do. Although you could create such a process that takes two parameters (the value and the type) and then performs the necessary processes for the type of value. YOU need to analyze the data you are capturing and determine what processes need to be done based upon how YOU plan to use that data. If you were to ask how to validate the input for a monetary field before saving to the database we could give specific code examples. But, there is no ONE answer that fits every situation.
-
It is apparent from the code you just posted that you are storing the images in a comma separated list in a single field. Just as you shouldn't be doing that for feature IDs, you shouldn't be doing that for images either. The code I posted was based upon your table structure being 'correct'. As for the code you posted based upon your current table structure, the solution is pretty obvious. You have the 'name', 'product' and 'features' values before you run the foreach loop, so why don't you echo them before the loop? That is why you are getting that data duplicated for each image. But you already have the images in a comma separated list. So why would you split the images based upon the comma and then run a foreach loop to echo them out comma separated? You should really fix your DB design. But, this should work with your current structure $query = "SELECT listings.name, listings.product, listings.features, listimages.imagepath FROM listings JOIN listimages ON listings.id = listimages.listingid ORDER BY listings.id DESC"; $result = mysql_query($query) or die(mysql_error()); echo "name,product,features,photo<br>"; // keeps getting the next row until there are no more to get $flag = ''; while ($row = mysql_fetch_assoc($result)) { echo "{$row['name']},{$row['product']},{$row['features']},\"{$row['imagepath']}\""; }
-
Complete a foreach array WITHOUT a implode function
Psycho replied to panthers_uni's topic in PHP Coding Help
Use foreach() to iterate over each element in the array echo '<p><b>This is what you are getting on your Tombstone: '; foreach($_POST['toppings'] as $topping) { echo " - $topping<br>\n"; } echo '</p></b>'; -
Um, let's not, since that won't work. A field that a users does not fill in a value for is still set - it just happens to have a value of an empty string. And, you should always use trim() on user input. Otherwise, a value of only spaces will appear as having a non-empty value. $first = trim($_POST['First']); $middle = trim($_POST['Middle']); $last = trim($_POST['Last']); $personal = trim($_POST['Persona1']); if(!empty($middle)) { $middle = $middle.'.'; } echo "{$first}.{$middle}{$last}.{$personal}.<br>\n"; Although you should really think about using htmlentities() on the values to prevent any values that might be interpreted as HTML code from screwing up the page.
-
I do not have your database and you didn't even provide all the necessary field names, so I make assumptions on a lot of things. I provide code as a guide on how you can implement it and leave it to you to properly implement it. I've looked at the code again and don't see any error that would cause the problems you state. This leads me to believe that either 1) You have duplicate image records for the listings or 2) the JOIN statement is using the wrong criteria and all the images records are being associated with all the listings. You should check your database for duplicates and you could also run the query in PHP admin to view the results to see if they look proper.
-
I'm not really understanding your criteria of I *think* you are wanting to output all the messages and calls in a single, date ordered list. If so, then you can do that in your query with a UNION clause. Or you could put all the records into a single array and then sort the arrya by the date element of the records. I think it would be helpful for you to show two or three real-world records for the elements and how you want the output for those records.
-
I'm not sure what response you are wanting with regard to the code you posted. But, sanitizing could mean different things to different people. I would call it the process of escaping or transforming ANY data to prevent errors. Those errors can be significant security issues or relatively benign display issues. You should ALWAYS sanitize user input before storing/using it. The methods you use will depend upon how you are using/storing the data. If you are storing the data in a MySQL database, for example, you would want to run mysql_real_escape_string() on string data, you would need to validate and format dates appropriately, and the same goes for numeric input - validate that the data is numeric and of the right type: int float, length, etc. The same goes for outputting data either to the browser or as a URL. For the former you might want to use htmlspecialcharacters() or htmlentities, for the latter you would likely use urlencode(). There could be any number of other scenarios, such as if you are outputting data to an xml or csv file. The bottom line is that you should really think about how your data is used. Where is it coming from, what do you *expect* it to be, what values could cause problems and how you can protect against those problems. Never assume that user data is safe. Even if you have a select list of known values, don't assume that the value submitted by the user is one of those values - it can easily be spoofed. Validate that the value is in the list of values you expect.
-
Right, and that class then calls another class "addComparison()". Which could lead to another class and/or will reference properties that are defined elsewhere. Someone would likely need to review the entire class to see if it is even possible to use the current code to do a search against multiple columns or if the class would need to be modified. The description for this forum is (emphasis added): You should really contact the author of the class you are using.
-
There's no way for us to answer your question from the code provided. You are apparently using a custom class to drive a lot of your functionality. addComparisonFromEdit() is a method within that class. I have no idea what that function does, what it returns or what variations in parameters it can accept. If you wrote that class then you should know that info. If you did not write the class then contact the author for help.
-
function listingImagesOutput($listingName, $imageList) { return "$listingName " . implode(', ', $imageList) . "<br>\n"; } $query = "SELECT l.listing_name, li.imagespath FROM Listings AS l JOIN listimages AS li ON l.listingid = li.listingid ORDER BY l.listingid"; $result = mysql_query($query); $current_listing = false; while($row = mysql_fetch_assoc($result)) { if($current_listing != $row['listing_name']) { if($current_listing != false) { //Output listing and images echo listingImagesOutput($current_listing, $imagesAry); } //Create temp array for images of this listing $imagesAry = array(); //Set flag to check for change in listing $current_listing = $row['listing_name']; } //Add image path to temp array for current listing $imagesAry[] = $row['imagespath']; } //Output last listing and images echo listingImagesOutput($current_listing, $imagesAry);
-
Cant get past IF statement to connect to database
Psycho replied to nander's topic in PHP Coding Help
You have no values being passed in the $_GET array. Therefore the condition in you if() statement is resulting in true which causes the page to redirect. -
No, of course you can. You can work around the problem with a bunch of hacks or you can make the necesasry changes to fix it the right way. It would not be difficult to migrate the existing data to an appropriate structure. Just create the new table as indicated above. Then create a simple script to run through all the records one time to do the migration. But, you would also need to modify any scripts you currently have to add, edit, delete and read the data. Step 1: Create the new table with columns for the listing id and the feature id. Step 2: Create a script to get the listing id and the CSV features list from the current listings table. Process the recorsd and run an insert query to create all the new records in the new table. Step 3: Rewrite your scripts to work with the new, correct design Step 4: delete the features column in the listings table. Here is a sample script to complete step 2 //Script to run ONE TIME to populate new records $query = "SELECT id, features FROM listings"; $result = mysql_result($query) or die(mysql_error()); //Process results into insert value $insertValues = array(); while($row = mysql_fetch_assoc($result)) { $listing_id = $row['id']; $features_ary = split(',', $row['features']); foreach($features_ary as $feature_id) { $insertValues[] = "($listing_id, $feature_id)" } } //Create and run INSERT query for all the records $query = "INSERT INTO listing_features (`listing_id`, `feature_id`) VALUES " . implode(", ", $insertValues); $result = mysql_result($query) or die(mysql_error());