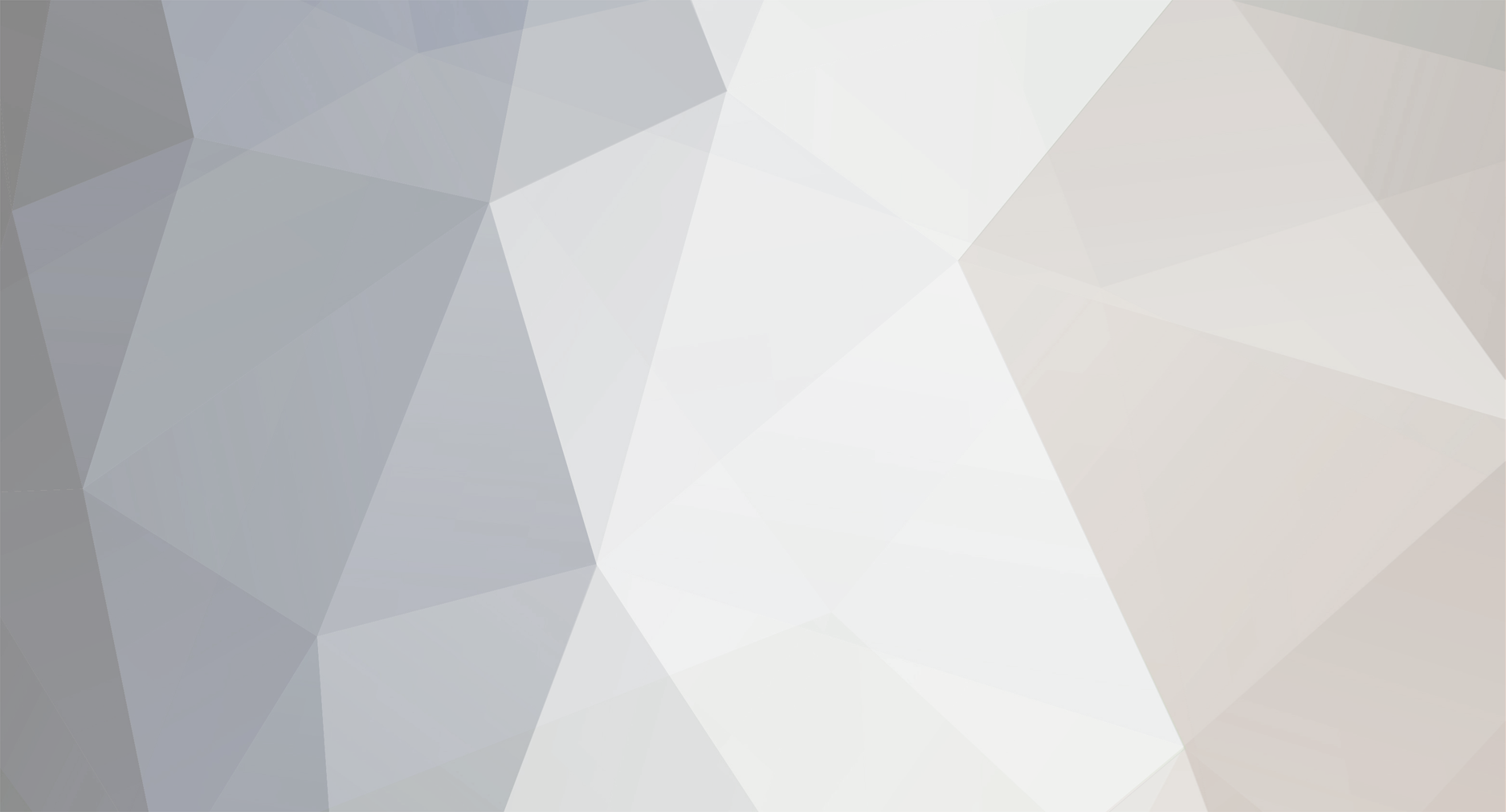
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Outputting data from MySQL Table to columns in PHP
Psycho replied to Travis959's topic in PHP Coding Help
Alrighty, I was bored today and this was kinda fun. The below script should produce the results you are looking for. I have hard-coded the dates and company names at the top of the script but you can hook in whatever means you are using to determine those values (a form POST I'm guessing). Also, I made one change to the output from what you presented above. I added field4 to the field5 for the headings for each sub-table output. It just made more sense to me. but, you can easily remove that if needed I have tested the code against the db you provided and it appears to be working correctly. But, I have not done a LOT of testing. So, you will want to give it a thorough going over. <?php mysql_connect('localhost', 'root', '') or die(mysql_error()); mysql_select_db('tab_test') or die(mysql_error()); $startyear = '2011'; $startmonth = '02'; $endyear = '2012'; $endmonth = '05'; $companyname = 'IMPORTADORA MADURO'; //Function to output parent (or summation) sub-table function createSeriesTable($dateCodes, $dataArray, $parentCode, $childCode, $colspan, $summation=false) { //Vars to calculate totals $unitsTotal = 0; $salesTotal = 0; //Vars to hold HTML content $unitsRow = ''; $salesRow = ''; $avgRow = ''; foreach($dateCodes as $dateCode) { $units = isset($dataArray[$dateCode]['units']) ? $dataArray[$dateCode]['units'] : 0; $sales = isset($dataArray[$dateCode]['sales']) ? $dataArray[$dateCode]['sales'] : 0; $avg = ($units!=0) ? $sales/$units : 0; $unitsTotal += $units; $salesTotal += $sales; $unitsRow .= "<td>{$units}</td>"; $salesRow .= "<td>" . number_format($sales, 2) . "</td>"; $avgRow .= "<td>" . number_format($avg, 2) . "</td>"; } $avgTotal = ($unitsTotal!=0) ?($salesTotal/$unitsTotal) : 0; if($summation) { $title = "Line Total for {$parentCode} : {$childCode}"; $label_class = 'summation_label'; $data_class = 'summationTable'; } else { $title = "{$parentCode} : {$childCode}"; $label_class = 'data_label'; $data_class = 'childTable'; } $tableHTML = "<tr class='{$label_class}'><th colspan='{$colspan}'>{$title}</th></tr>\n"; $tableHTML .= "<tr class='{$data_class}'><th>Units</td>{$unitsRow}<td>{$unitsTotal}</td></tr>\n"; $tableHTML .= "<tr class='{$data_class}'><th>Sales</td>{$salesRow}<td>" . number_format($salesTotal, 2) . "</td></tr>\n"; $tableHTML .= "<tr class='{$data_class}'><th>Average Price</td>{$avgRow}<td>" . number_format($avg, 2) . "</td></tr>\n"; if($summation) { $tableHTML .= "<tr><td colspan='{$colspan}'> </td></tr>\n"; } return $tableHTML; } //Create array of month/year codes and header HTML code $monthCount = 0; $datestamp = 0; $enddatestamp = mktime(12, 0, 0, $endmonth, 1, $endyear); $dateCodes = array(); $dateHeadersHTML = ''; while($datestamp < $enddatestamp) { $datestamp = mktime(12, 0, 0, $startmonth+$monthCount, 1, $startyear); $year = date('Y', $datestamp); $month = date('m', $datestamp); $monthName = date('M', $datestamp); $dateCodes[] = "{$year}-{$month}"; $dateHeadersHTML .= "<th>{$monthName}<br>{$year}</th>\n"; $monthCount++; } $table_colpsan = count($dateCodes) + 2; //Determine the number of months in the report $report_month_count = ($endyear*12+$endmonth) - ($startyear*12+$startmonth) + 1; $report_column_count = $report_month_count+2; $query = "SELECT field3 AS family, field4 AS parent_code, field5 as child_code, month, year, field10 AS units, field9 AS sales FROM slssum2 WHERE company = '$companyname' AND CONCAT(year,month) BETWEEN '$startyear$startmonth' AND '$endyear$endmonth' AND field3 != 0 AND field4 != '995' ORDER BY field3, field4, field5, year, month"; $result = mysql_query($query) or die(mysql_error()); //Create flag vars to detect changes $last_family = ''; $last_parent_code = ''; $last_child_code = ''; while($row = mysql_fetch_assoc($result)) { if($last_child_code != $row['child_code']) { //Display table for last child record set if($last_child_code != '') { $output .= createSeriesTable($dateCodes, $childData, $last_parent_code, $last_child_code, $table_colpsan); } //Create/reset temp array to hold data to be output $childData = array(); $last_child_code = $row['child_code']; } if($last_parent_code != $row['parent_code']) { //Display summation table for last parent if($last_parent_code != '') { $output .= createSeriesTable($dateCodes, $parentData, $last_family, $last_parent_code, $table_colpsan, true); } //Create/reset temp array to hold data to be summed $parentData = array(); $last_parent_code = $row['parent_code']; } if($last_family != $row['family']) { //Create Output for family heading $output .= "<tr><th colspan='{$table_colpsan}' class='familyHead'>FAMILY {$row['family']}</th></tr>"; $last_family = $row['family']; //Create summation table for last parent //$output .= createSeriesTable($dateCodes, $parentData, $row['parent_code'], $table_colpsan, $row['family']); } //Set child/parent data using the current record $month = "{$row['year']}-{$row['month']}"; $childData[$month] = array('units' => $row['units'], 'sales' => $row['sales']); $parentData[$month] = array('units'=>($parentData[$month]['units']+$row['units']), 'sales'=>($parentData[$month]['sales']+$row['sales']) ); } //Display last child record set and summation record set $output .= createSeriesTable($dateCodes, $childData, $last_parent_code, $last_child_code, $table_colpsan); $output .= createSeriesTable($dateCodes, $parentData, $last_family, $last_parent_code, $table_colpsan, true); ?> <html> <head> <style> .dateHead th { color:green; text-align:center; font-weight:normal; } .familyHead { text-align:left;background-color:#33ffbb; padding: 5px; } .summation_label th { text-align:left; padding: 5px; font-weight: bold; } .data_label th { text-align:left; padding: 5px; font-weight: normal; } .childTable th { text-align:left; background-color:#cecece; font-weight:normal; padding:5px; } .childTable td { background-color:#efefef; text-align:right; padding:5px;} .summationTable th { text-align:left; background-color:#9999ff; font-weight:normal; padding:5px; } .summationTable td { background-color:#ccccff; text-align:right; padding:5px;} </style> </head> <body> <table border='0'> <tr><td><?php echo "{$startmonth}/{$startyear} to {$endmonth}/{$endyear}</td></tr>"; ?></td></tr> <tr class='dateHead'><td></td><?php echo $dateHeadersHTML; ?><th>Total</th></tr> <tr><td colspan='<?php echo $table_colpsan; ?>'><hr></td></tr> <?php echo $output; ?> </table> <pre> <?php echo $query; ?> </pre> </body> </html> -
Outputting data from MySQL Table to columns in PHP
Psycho replied to Travis959's topic in PHP Coding Help
The names in the input file do not need to dictate the names of the fields in the database. When the import is done is when you should 'translate' the data to logically named fields. Can you provide an export of the table with some sample data? That would make this much easier. EDIT: A couple questions. Are the values for fiel9 & field10 already summed? For example, in the image above where you have 50441 for January you have values of 15 and 157.5 are those the values from a single DB entry or are there multiple records for 50441 in that minth that need to be summed? Also, is there ALWAYS a value for every month for each field5? -
Outputting data from MySQL Table to columns in PHP
Psycho replied to Travis959's topic in PHP Coding Help
Did you try the code that PFMaBiSmAd provided? Also, knowing what a field means "in your head" is freakin ludicrous. Give your fields descriptive names. It will make your life much easier. -
I'm not understanding the problem. If the variable is not set, then set it. I have no idea what that value is supposed to be or why it is not set in your code. YOU have put that variable in your code - so why are you not setting it? But, even if the value is not set, there should still be a posted value of a null string. I don't think your problem is the hidden variable - it is the fact that you can only click one button - so the other one is not part of the POST data. Instead of trying to work around a problem that shouldn't even exist, why don't you fix your processing code to NOT store the values in an array with no way to logically know what value is what? If you are going to store these values in an array, at least do it with named indexes. This is all you need to store the POST values in an array. If any POST values are missing a value of false will be stored for that index. //Populate array based on POST data $array = array(); $array['likes'] = (isset($_POST['likes'])) ? $_POST['likes'] : false; $array['dislikes'] = (isset($_POST['dislikes'])) ? $_POST['dislikes'] : false; $array['hidden_con_id'] = (isset($_POST['hidden_con_id'])) ? $_POST['hidden_con_id'] : false; $array['dnuser_id'] = (isset($_POST['dnuser_id'])) ? $_POST['dnuser_id'] : false;
-
Display the result of large select_form with many conditions
Psycho replied to Adib's topic in PHP Coding Help
You should definitely start considering to learn how to write modular code. I.e. write code that can be re-purposed when you have to do the same thing multiple times. It would also be a good idea to separate the logic (PHP) from the display (HTML) instead of intermixing the two. Here is one possibility for creating the select options in a more logical format. I would create all the fields as an array to make the processing easier. <?php //Function to generate select options from a query function querySelectOptions($query) { //Create default option $optionsHTML = "<option value=''>-----</option>\n"; //Run query $result = mysql_query $query); if(!$result) { return $optionsHTML; } //Process results while($row = mysql_fetch_array($result)) { $optionsHTML .= "<option value='{$row[0]}'>{$row[1]}</option>\n"; } return $optionsHTML; } //Create locatie options $locatieOptions = querySelectOptions("SELECT ID, locatie FROM locatie"); //Create prijsVan options $prijsVanOptions = querySelectOptions("SELECT ID, prijs FROM prijs"); //Create prijsTot options $prijsTotOptions = querySelectOptions("SELECT ID, prijs FROM prijs"); //Create ligging options $liggingOptions = querySelectOptions("SELECT ID, ligging FROM ligging"); //Create oppervlakte options $oppervlakteOptions = querySelectOptions("SELECT ID, oppervlakte from oppervlakte"); //Create kamers options $kamersOptions = querySelectOptions("SELECT ID, kamers from kamers"); //Create woning options $woningOptions = querySelectOptions("SELECT ID, type from woning"); ?> <select name="condition[locatie]" id="locatie"> <?php echo $locatieOptions; ?> </select> <select name="condition[prijsVan]" id="prijsVan"> <?php echo $locatieOptions; ?> </select> <select name="condition[prijsTot]" id="prijsTot"> <?php echo $prijsTotOptions; ?> </select> <select name="condition[ligging]" id="ligging"> <?php echo $liggingOptions; ?> </select> <select name="condition[oppervlakte]" id="oppervlakte"> <?php echo $oppervlakteOptions; ?> </select> <select name="condition[kamers]" id="kamers"> <?php echo $kamersOptions; ?> </select> <select name="condition[woning]" id="woning"> <?php echo $woningOptions; ?> </select> Then the processing code function processConditions($postConditions) { $conditionsAry = array(); foreach($postConditions as $field => $value) { $value = trim($value); if(!empty($value)) { $value = mysql_real_escape_string($value); $conditionsAry[] = "`{$field}` = '{$value}'" } } return $conditionsAry; } $eigenschappen = $_POST['eigenschappen']; //Process the conditions that were posted $conditionsArray = processConditions($_POST['conditions']); // Error: indien geen keuze gemaakt show deze error if(count($conditionsArray)==0) { echo "<center><font color='#666'> " . postError() . "</font></center>\n"; } else { //Execute query $query .= " AND " . implode(' AND ', processConditions($_POST['conditions'])) $query .= " ORDER BY prijs.ID"; $result = mysql_query($query) or die(mysql_error()); while($row = mysql_fetch_array($result)){ echo $row['image_id'] ; echo $row['locatie'] ; echo $row['prijs']; echo $row['type']; echo $row['kamers']; echo $row['oppervlakte'] . ' m²'; echo $row['ligging']; echo $row['eigenschappen']; } } mysql_close($con); None of this was tested, but is just an example of how you could make that code more efficient/modular -
You just answered your own question. $last_brand = ''; while($row = mysql_fetch_assoc($result)) { //Check if this brand is the same as the last if($last_brand != $row['brand']) { $rank = 0; $last_brand = $row['brand']; } $rank++; //Display results }
-
Yeah, your question is way to generalized to really provide a response. I interpret "Scope" as referring to "variable scope" which, if you built your function correctly, should not be an issues at all. But, since you didn't provide any code there is no way to say. The only reason scope would be an issue is if you were relying upon the use of global in order to reference variables. And, THAT, would be the wrong way to do it.
-
Don't you keep track of orders already? Shouldn't be anything additional you would need to do.
-
I would suggest a separate table to store any changes YOU make to stock. this would typically only include when you add new product, but their might be occasions when you would remove stock (spoilage, theft, etc.). Then you take the total from that table for a particular product and subtract the total ordered for that product in your orders table.
-
I'm not sure what more to explain without actually writing code for you. Simply set a flag (in the example I provided using a session variable) to determine if you want the scripts to run in "test" mode or not. There is nothing to "look up". It is up to YOU to determine what the application will do differently in test mode. As per your request, the test mode needs to allow you to pick your numbers AND set the winning numbers. So, the form will need to allow for additional input fields for the winning numbers //Normal form goes here //For user to enter their picks if($_SESSION['test']) { //Additional form goes here for you to enter the winning numbers when in test mode } Then on the processing page, if you are in test mode you need to use the winning numbers passed from the form instead of the current logic for determining winning numbers if($_SESSION['test']) { //Set winning numbers based upon additional form fields } else { //Set winning numbers based on current logic }
-
I would create a variable to indicate whether you are in 'test' mode or not. Since you appear to have multiple pages for this logic I'd probably save this as a session or cookie value. So, on the page where you would want to start the testing process (the form for the user to enter thier picks) do something like this: session_start(); $_SESSION['test'] = 1; //Set to 0 when NOT in test mode On your form script check to see if you are in test mode or not. If yes, then include addition fields in the form to enter the 'winning' numbers. On the processing script check to see if you are in test mode or not. If yes, then bypass the code to dynamically determine the winning numbers and instead use the numbers sent in the post data
-
The "purpose" of the switch for determining to use the key as the value has nothing to do with the process for determining the selected option. The purpose of using the key of the array or not is due to the fact that some select lists will have the same value as the label. I.e. <select> <option value="Red">Red</option> <option value="Green">Green</option> <option value="Blue">Blue</option> </select> While other select lists will have different values than the labels <select> <option value="1">Red</option> <option value="2">Green</option> <option value="3">Blue</option> </select> The latter is very common when dealing with assignments to DB records. So, the function I provided was an example of a function that one could use to meet the needs of both types of selects lists. $colors = (1 => 'Red', 2 => 'Green', 3 => 'Blue'); //Create select list with same data for the value and label echo dropdown('colors', $colors, true); //Create select list with IDs as values and description as label echo dropdown('colors', $colors, false); Again, the purpose of this option had nothing to do with the functionality for determining the selected value. It was about giving greater flexibility to the function for creating select lists. The ONLY line I provided for determining the selected value was this $selected = ($value===$selected_value) ? " selected='selected'": ''; And, I guess you still haven't found the flaw in the code you provided.
-
You don't need to dump the DB results into an array, simple run the same code in the while() loop when extracting the results. But, the problem with your code above is that you are redefining the array on each iteration of the while() loop instead of appending the results! The code should look something like this (replace 'res' and 'number' with the appropriate DB field names) $sql = mysql_query("SELECT * FROM `my_table` ")or die(mysql_error()); $lastRes = 1; while ($row=mysql_fetch_assoc($sql)) { if($row['res'] == 0 && $lastRes !== 0) { //This line is only executed if 'res'=0 and the last 'res' was not 0 $res11 = $row['number']; echo "A New '0' was encountered. res11 now equals {$res11}<br>\n"; } $lastRes = $row['res']; )
-
For the purposes of whether the option will be selected or not - that is exactly what that function does. I was simply providing some additional functionality into the function so it is more flexible and can be re-purposed as needed. By the way, there is a bug in the last script you provided. It would only be an issue based on specific values existing in the list of options and may not be experienced for the OPs implementation. But, the bug is there nonetheless.
-
I doubt this is a server issue. I can't think of a situation where an array variable is going to be interpreted as a non-array variable, produce the error, but then yet process the variable as an array. Just makes no sense. You say the line with the error is this foreach(array_keys($_SESSION['cart']) as $i) { ... Do you know the specific scenarios where the error occurs? Can you run through that script in the production environment and produce the error? You should probably take another look at the 'flow' for your code. How can users access that script? Will they ALWAYS have $_SESSION['cart'] defined and as an array? Where is that value first defined? I'm guessing that users are accessing that page in a manner that was not considered during development/testing.
-
Personally, I prefer the define a $selected variable. Just looks cleaner IMHO. Also, in many cases the actual 'value' of a select list options will be different than the 'label'. So, I create my functions with a parameter on whether to use the keys of the array or not. If so, the keys are used as the value and the values as the labels May seem backwards, but makes sense logically. Values are routinely numeric IDs in the database so using the keys of the array makes sense. Lastly, it is poor implementation to have your functions actually echo content. Instead the function should return the content to be displayed. Anyway, here is a possible solution for you. function dropdown($id, $optionsAry, $use_keys=false, $selected_value=false) { $selectHTML = "<select name='{$id}' id='{$id}' class='select'>\n"; $selectHTML .= "<option value=''>Select one...</option>\n"; foreach($optionsAry as $value => $label) { if(!$use_keys) { $value = $label; } $selected = ($value===$selected_value) ? " selected='selected'": ''; $selectHTML .= "<option value='{$value}'{$selected}>{$label}</option>\n"; } $selectHTML .= "</select>\n"; return $selectHTML; }
-
The answer is - it depends. A better approach is to identify these errors and code for them. But, from a practical standpoint, yes you can create a script that will restart if errors are encountered. But, you have to be careful about a possible error that may not be transient (i.e. an error that does not go away). If that happens you would be caught in an infinite loop which could have serious consequences on the rest of your application with regard to performance. For the sake of argument, you could create a while loop that contains the process you need run. Have the loop continue as long as the process does not complete and include a time-limit to prevent infinite loops. Just be sure you do extensive testing before actually implementing anything. Here is an example $complete = false; $timeLimit = time() + 120; //2 minutes while(!$complete && time()<$timeLimit) { //As you run each process that can generate an error, // suppress error messages but check for the errors $contents = @file_get_content('[url=http://somedomain.com/somedatafile.txt%27%29;]http://somedomain.com/somedatafile.txt');[/url] //If result was false restart at condition if(!$contents) { continue; } //If script completes w/o errors set $complete to true if(!$errors) { $complete = true; } }
-
The code I provided was only mock code based upon the requirements as I understood it. You did not provide enough information to provide a full-fledged solution or to even validate it was the correct solution for you. having said that . . . With the code I provided, you don't need to skip. The if() condition in that loop would only update $res11 if the 'res' value is 0 AND the last value was not 0. But, you don't want to 'skip' the 1's because you need to update $lastRes with the 1. I'm really not understanding you here. I'm guessing English is not your native language so please try to be specific. Can you provide an example of the data you are using and "how" the output is not what you expect/want? EDIT: I did find one bug which would prevent the very first record from being processed correctly - if the first record's res value was 0. But, all other scenarios will work as I intended. The problem was that I set the initial value of $lastRes to an empty string and when I did the comparison $lastRes != 0 It was resulting in true, because using a non type specific comparison and empty string is logically equal to 0 (or false). This can be fixed by making the comparison type specific or setting th einitial value to something like a negative 1. Here is the slightly modified code. I replaced the while() loop with a foreach() loop so I could test the process on an array using values similar to what you had in your first post. I have also included the output of the test. //Array of value to test against $testArray = array( array('res' => 0, 'number' => 1), //Starting 0 value array('res' => 0, 'number' => 2), array('res' => 0, 'number' => 3), array('res' => 1, 'number' => 4), array('res' => 1, 'number' => 5), array('res' => 1, 'number' => 6), array('res' => 1, 'number' => 7), array('res' => 1, 'number' => , array('res' => 0, 'number' => 9), //Starting 0 value array('res' => 0, 'number' => 10), array('res' => 1, 'number' => 11), array('res' => 1, 'number' => 12), array('res' => 1, 'number' => 13), array('res' => 1, 'number' => 14), array('res' => 1, 'number' => 15), array('res' => 0, 'number' => 16) //Starting 0 value ); $lastRes = 1; //while($row = mysql_fetch_assoc($result)) foreach($testArray as $row) { if($row['res'] == 0 && $lastRes !== 0) { //This line is only executed if 'res'=0 and the last 'res' was not 0 $res11 = $row['number']; echo "A New '0' was encountered. res11 now equals {$res11}<br>\n"; } $lastRes = $row['res']; } Output A New '0' was encountered. res11 now equals 1 A New '0' was encountered. res11 now equals 9 A New '0' was encountered. res11 now equals 16
-
Two infinite while loops, not sure how to connect them?
Psycho replied to phpchick's topic in PHP Coding Help
Are you only updating the file with the current price or are you keeping a running log of the price? If you are only updating the file with the current price then I think you are taking the entirely wrong approach. You don't need any script running all the time. Instead, change the page that the users access to dynamically get the price. If that process is time consuming, then you can implement a process in that page to get the value from a cache. It would first check the age of the cache and if it is older than a certain time limit then get a current value and update the cache. It makes no sense to constantly update the file every 10 seconds if the page is not being accessed every few seconds. If you are wanting a running log, the you should probably be updating a database with the values and the "HTML" page should be a script to get the values from the database. -
I really don't know what you are talking about. I see plenty of HTML code in what you posted above. Are you wanting to view the HTML page that is rendered from that PHP code? If so, you need to access the file via a web server that has PHP enabled. Also, that script requires a database to be able to create the content. So you might want to set up a web and DB server on your local machine. There are packages such as XAMPP and WAMP that allow you to do this. The setup is pretty strait forward to get the webserver and dbserver running. But, then you need to set up a database with the appropriate tables, fields and data for the script to run. So, if you downloaded this file from your server, you should be able to copy the database from your server and then recreate it on your machine. None of this is 'hard' but if you've never done any of this before you will have a bit of a challenge figuring it all out.
-
I'm a little confused by your 'logic', but I think what you are trying to say is that you want to set a value each time the result zero is encountered IF the previous result was not 0. $lastRes = ''; while($row = mysql_fetch_assoc($result)) { if($row['res'] == 0 && $lastRes != 0) { //This line is only executed if 'res'=0 and the last 'res' was not 0 $res11 = $row['number']; } $lastRes = $row['res']; }
-
First of all I would do the validation completely different. But, even with the process you were trying to implement - the actual format is very poorly done. First off, always indent your code to give it a visually logical structure. Makes editing/debugging much, much easier. You should not have all those nested statements. You should have been using elseif() statements. So, instead of if(condition1) { //Do something } else { if(condition2) { //Do something } else { if(condition3) { //Do something } } } You should instead use elseif() statements like this if(condition1) { //Do something } elseif(condition2) { //Do something } elseif (condition3) { //Do something } Plus, instead of putting all the headers() in each condition block, I would just set the error code. Here is your code in a more logical format: $error = false; if ($_POST['email1'] !== $_POST['email2']) { $error = "email_no_matchname"; } elseif ($password1 !== $password2) { $error = "password_no_match"; } elseif ($_POST['firstname'] == NULL) { $error = "firstname_null"; } elseif ($_POST['lastname'] == NULL) { $error = "lastname_null"; } elseif ($_POST['phonea'] == NULL) { $error = "phonea_null"; } elseif ($_POST['phoneb'] == NULL) { $error = "phoneb_null"; } elseif ($_POST['username'] == NULL) { $error = "username_null"; } elseif ($_POST['email1'] == NULL) { $error = "email_null"; } elseif (strlen($_POST['password1']) <= 6) { $error = "password_length"; } elseif (strlen($_POST['phoneb']) <= 5) { $error = "phone_length"; } elseif (!is_numeric($_POST['phoneb'])) { $error = "phonea_numeric"; } elseif (!is_numeric($_POST['phoneb'])) { $error = "phoneb_numeric"; } elseif (strlen($_POST['username']) <= 4) { $error = "username_length"; } elseif (preg_match('/[^a*()-z0@£"%&-9.#$-]/i', $_POST['password1'])) { $error = "pwd_inv_cha"; } elseif (preg_match('/[^a*()-z0@£"%&-9.#$-]/i', $_POST['password1'])) { $error = "usn_inv_cha"; } elseif(!eregi("^[_a-z0-9-]+(\.[_a-z0-9-]+)*@[a-z0-9-]+(\.[a-z0-9-]+)*(\.[a-z]{2,3})$", $_POST['email1'])) { $error = "email_validation"; } if($error != false) { header ("Location: /reg.php?error={$error}"); } } But anyway, the problem I have with your validation logic is that you simply stop validating after the first error. Personally, I hate when a site does that. I submit a form and it tells me that item 'A' is not correct. I fix that and resubmit only for the form to tell me the item 'B' is incorrect. Why didn't it tell me that items 'A' and 'B' were incorrect the first time I submitted the form? I typically have the validation logic for a form in the same script that produces the form. So, I submit forms back to themselves. I run through ALL the validations and store any errors in an array. If the array is empty at the end of the validations then no errors are present and I include the form processing logic. If the array is not empty then I display ALL the errors and redisplay the form with the fields populated with the submitted values. EDIT: You also need to rethink how you are doing your validations. Checking if a POST field is NULL is not appropriate. You need to first trim() the value and then check if the value of that is empty()
-
Using something like this is a very bad idea. if($adid == "") { $idquery = mysql_query("SELECT MAX(adid) FROM ads"); $idrow = mysql_fetch_row($idquery); $adid = $idrow[0] +1; } 1) It is inefficient. DB queries are expensive transactions. Running two queries just to insert a record is not wise. 2) MySQL will do a much better job of managing IDs than you or I can 3) You leave yourself open for creating database inconsistencies that will corrupt your database. In the unlikely event that two records are submitted at the same time, you could end up with records that have duplicate IDs. As stated previously, change the field to be an auto-increment primary key field and set the start value to be higher than the current highest ID. Then do not include the ID field in the insert clauses and let MySQL create it for you. Besides, if you did need to get the number that is one more than the current max value (which you should still not do in this instance) a more appropriate solution would be if($adid == "") { $query = "SELECT MAX(adid)+1 FROM ads"; $result = mysql_query($query); $adid = mysql_result($result, 0); }