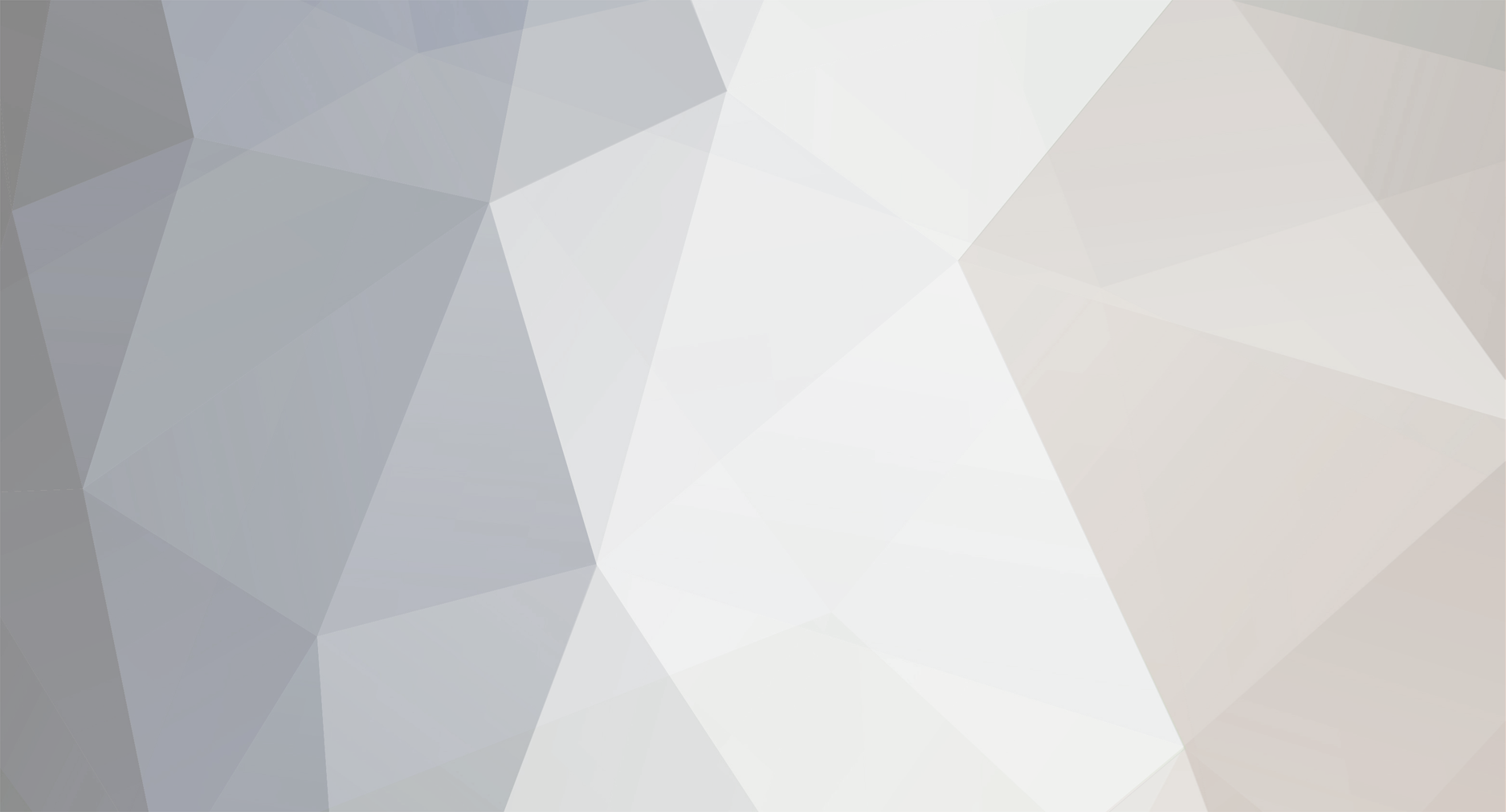
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
UPDATE orderTable SET custUsername = '$username', custPassword = '$password' WHERE orderNum = '$custOrderNum'
-
I'll try to explain again. You are currently selecting data from the data, then when you process that data you are using PHP code to calculate the distance, correct? Since the distance is not included in the database you cannot sort the data using that in the query - UNLESS you can move the distance calculation into the query. You can, possibly, create a dynamic field in the query that is a calculation of the distance. Here is a query that would return three values: fielda, fieldb and a dynamically generated field "sumAandB" that is the sum of fielda and fieldb SELECT fielda, fieldb, (fielda + fieldb) AS sumAandB Now, if the appropriate math functions are not available in MySQL for you to do the distance calculation in MySQL, then you will need to pull the records from the database first, run them through a process in PHP to calculate the distance but do not display them. Instead just store the records into an array along with the calculated distance value. Then you would sort the array using the distance value. The problem you have here is that you want to do pagination. So, if you wanted the records on page 2 with the sort order by distance you have no way in the query to determine which records those are without knowing the distance ahead of time. So, you would have to query ALL the records, calculate the distance as you add them to an array and then extract the relevant records from the array for the current page. I can also think of a third option if you can't do the calculations within the query. I assume that the start point will not change (or at least won't change as the user is paging through the records). So, one possibility would be to pull all the records the first time the user selects/changes the start location, go through a process to determine the distance, the save the record ID and the distance to a temporary table. Then you could do a JOIN on the main table and the temp table and be able to sort by distance. I've not worked with temporary tables, so you would have to do some research if that is the route you take. I was going to take a crack at modifying your code, but it's pretty unorganized and I just wasn't willing to invest the time.
-
Impossible to help with what you have provided. You are using the function "calc_postcode_seperation" which is not in the script you have posted. You will have to duplicate the logic for calculating the distance using MySQL math functions to define a dynamic field (e.g.'distance'). Then you can sort the results in MySQL using that field. Alternatively, you can dump the results into an array and while doing that calculate the distance and add the field for each record into the array. Once you have completed that, you could then sort the array by that field. Also, why are you using htmlentities() on the values before using them for a db query? EDIT: I just noticed that this is a pagination script. So, you really want to do this in the MySQL query. If you have to go the array route you would have to get ALL the records from the database, calculate the distance, then extract the current appropriate records for the current page. If you can't do it in MySQL and you don't have a lot of records it may be feasible, but is definitely a "hack" solution.
-
Run PHP if form submit() is ran by onclick on div.
Psycho replied to 00stuff's topic in PHP Coding Help
That should submit the form. I think the problem is how you are checking if the form was submitted on the processing page where you use if(isset($_POST['submit'])) { There is no field named 'submit' on your form - at least I don't see one. But, you do have a field named 'field1'. Use that field name to check if the form was posted if(isset($_POST['field1'])) { Although there are better methods of checking form submission. -
@Mike D. This is slightly off topic, but I would highly suggest that you consider taking a more modularized approach to your coding. It makes creating/debugging/maintaining/fixing so much easier. Having a file with 1400+ lines of code makes this more complicated than it needs to be. That is primarily why I chose not to respond further in this thread - it was going to take me more time to debug the issue due to the complicated structure than was worthwhile for me. As a quick example of one easy change you could make, I see that there is a switch() statement used to decide what the page is supposed to do. You should make this script your "controller" script to determine what actions to perform and then have separate scripts to perform those action. In other words, use the switch() statement in this script but then instead of having a bunch of code under each case statement put that code into a sepaate file and include() it under the case statement. Here is a very brief example of how I would make that main switch statement look. Also, you have a "default" for the switch statement that doesn't do anything. I typically set the default action for my pages to some benign action - such as a "display" type action to make sure the page never "fails". But, there is something to be said for having the page display empty if there is an unexpected action request as it could be a visual indicator that you forgot to handle a particular action. Lastly, you are using the variable $query as the value for the switch. I would suggest a different variable name ($action comes to mind) since $query is typically used as a variable to hold DB queries. Basically, give your variables meaningful names that someone can identify what it likely contains without reading all the code. In fact, I even go so far in some cases as to add a descriptor to my variables to identify the type of data it contains. E.g.: $userListAry vs. $userListStr Anyway here is the example switch($query) { case "players_online": include('_show_players_online.php'); break; case "cms": include('_show_cms.php'); break; case "opt": include('_show_opt.php'); break; case "deletecharacter": include('_show_deletecharacter.php'); break; case "highscores": default: include('_show_highscores.php') break; }
-
If you can't use fgetcsv(), then I would revert to using file(). I have to go, but this is mostly working. FOr some reason the trim() isn't being applied to the values, which will cause problems with the sorting and display. Plus, the sorting usign ksort() will likely not be case insensitive. Might need to use a different approach <?php $file = "phonelist.txt"; $linesAry = file($file); //Dump data into an array with "lname fname" as the key $resultsAry = array(); foreach ($linesAry as $line) { $dataAry = explode(',', $line); array_walk($dataAry, 'trim'); $resultsAry["{$dataAry[1]} {$dataAry[0]}"] = $dataAry; } //Sort the results by the key ksort($resultsAry); //Process the sorted results into HTML $htmlOutput = ''; foreach($resultsAry as $data) { //Parse the values list($lname, $fname, $addr, $city, $state, $zip, $acode, $phone) = $data; $fullName = htmlentities("{$fname} {$lname}"); $fullPhone = htmlentities("{$acode}-{$phone}"); //Gnerate HTML $htmlOutput .= "<tr>\n"; $htmlOutput .= "<td>{$fullName}</td>\n"; $htmlOutput .= "<td>{$fullPhone}</td>\n"; $htmlOutput .= "</tr>\n"; } ?> <table border='1' width='100%'> <tr><th>Name</th><th>Phone Number</th></tr> <?php echo $htmlOutput; ?> <table>
-
What version of PHP are you running? Attach the file to the post and I can work on it. I hate trying to resolve issues relating to data when I can't even see the data. If the file as data you don't want exposed, then use a search and replace to replace 0-9 with some other characters. That should remove enough information to make it non-identifiable.
-
@TeNDoLLA, My apologies. I see you DID use just '/' for the domain parameter. All I "saw" was the ".website.com" parameter.
-
Give this a try, not tested <?php $file = "phonelist.txt"; if (($handle = fopen($file, "r")) !== FALSE) { //Dump data into an array with "lname fname" as the key $resultsAry = array(); while (($line = fgetcsv($file, 1000, ",")) !== FALSE) { $resultsAry["{$line[1]} {$line[0]}"] = $line; } fclose($handle); //Sort the results by the key ksort($resultsAry); //Process the sorted results into HTML $htmlOutput = ''; foreach($resultsAry as $data) { //Parse the values list($lname, $fname, $addr, $city, $state, $zip, $acode, $phone) = $data; $fullName = htmlentities("{$fname} {$lname}"); $fullPhone = htmlentities("{$acode}-{$phone}"); //Gnerate HTML $htmlOutput .= "<tr>\n"; $htmlOutput .= "<td>{$fullName}</td>\n"; $htmlOutput .= "<td>{$fullPhone}</td>\n"; $htmlOutput .= "</tr>\n"; } } else { $htmlOutput .= "<tr><td colspan='2'>Unable to read data file</td></tr>\n"; } ?> <table border='1' width='100%'> <tr><th>Name</th><th>Phone Number</th></tr> <?php echo $htmlOutput; ?> <table>
-
Instead use '/', per the manual:
-
You need to change the style properties of the page to remove margins. Find a tutorial on CSS/Style Sheets and give it a read. But, to just remove the page margins, add the following style section inside the head tags of your page. <html> <head> <style> body { margin: 0px; } </style> </head> ...
-
There really isn't much to the logic. To create a "total" of all the records in the result set, just create a variable(s) to hold the total before you start processing the records. Then, when you process the records, increase the value of that variable(s) based upon the value of the current record. I have added comments to the code and also revised it further to be more structures. I put ALL the processing code at the head of the page and in the HTML I only echo one variable for the results. By structuring your code in this way it gives you a lot more flexibility. For instance you can have one script that gets/processes the data then have different "output" files based on the situation. Also, I may have had some typos, but the {} were by design. You can put variables within strings that are defined with double quotes. But, in some instances (such as referring to an array value) you need to enclose the variable in {} to have it interpreted correctly. Lastly, instead of checking the date separately against the begin and end dates separately, look into using the BETWEEN operator for the query. EDIT: OK, another thing. Don't use the string values "true" and "false" as your database values. use a tinyint type field and store 0 (false) or 1 (true). Those values are logically handled as Boolean true/false. Then you never need to compare the value to a string. <?php include("db_connect.php"); include("functions.php"); $_GET=sanitize($_GET); $query = "SELECT COUNT(consultant.flow_id) AS flow_id_count, consultant.flow_id, full_name, client_index, sales_manager_index, role, street, city, consultant.state, zip, start_date, SUM(gross_margin) AS gross_margin_sum FROM contract JOIN consultant ON contract.flow_id=consultant.flow_id WHERE contract.deleted='false' AND consultant.deleted='false' AND start_date>=str_to_date('{$_GET['start_date_begin']}', '%m/%d/%Y') AND start_date<=str_to_date('{$_GET['start_date_end']}', '%m/%d/%Y') AND status='active' GROUP BY full_name"; $result = mysql_query($query); //Create vars to store the totals for flow_id count and gross_margin $total_count = 0; $total_margin = 0; //Process the results into HTML output code $htmlOutput = ''; //Var to hold the html code while($row = mysql_fetch_array($result)) { //Define the record date for display $recordDate = date("m/d/Y", strtotime($row['start_date'])); //Process the record into HTML code and store in output var $htmlOutput .= "<tr>\n"; $htmlOutput .= "<td><a target='_blank' href='http://www.ajasa.com/cams/us_form.php?flow_id={$row['flow_id']}'>{$row['full_name']}</a></td>\n"; $htmlOutput .= "<td>{$row['flow_id_count']}</td>\n"; $htmlOutput .= "<td>{$row['client_index']}</td>\n"; $htmlOutput .= "<td>{$row['sales_manager_index']}</td>\n"; $htmlOutput .= "<td>{$row['role']}</td>\n"; $htmlOutput .= "<td>{$row['street']}</td>\n"; $htmlOutput .= "<td>{$row['city']}</td>\n"; $htmlOutput .= "<td>{$row['zip'}</td>\n"; $htmlOutput .= "<td>{$row['state']}</td>\n"; $htmlOutput .= "<td>{$recordDate}</td>\n"; $htmlOutput .= "<td>}$row['gross_margin_sum']}</td>\n"; $htmlOutput .= "</tr>\n"; //Increate the totals based upon current record values $total_count += $row['flow_id_count']; $total_margin += }$row['gross_margin_sum']; } //Create html output for the final, summary line $htmlOutput .= "<tr><td></td><td>{$total_count}</td><td colspan=\"8\"></td><td>{$total_margin}</td></tr>\n"; ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Hire Report</title> <link rel='stylesheet' type='text/css' href='styles/page.css' /> <script type='text/javascript' src='javascript/sortable.js'></script> </head> <body> <table class='sortable' id='sortabletable' rules=cols> <thead> <tr> <th>Name</th> <th>Total Hires</th> <th>Client</th> <th>Sales Manager</th> <th>Role</th> <th>Street</th> <th>City</th> <th>Zipcode</th> <th>State</th> <th>Start Date</th> <th>Total Gross Margin</th> </tr> </thead> <tbody> <?php echo $htmlOutput; ?> </tbody> </table> </body> </html>
-
Take a look at this thread: http://www.phpfreaks.com/forums/index.php?topic=339003.msg1598048#msg1598048
-
Looking at the manual, a ROLLUP will provide a null value for all fields - except those that are GROUP BY modifiers. Not, sure why state is in the summation record. Personally, I would do the summation logic in PHP (it would be less work). Otherwise you have to use if/else logic on all the values that shouldn't be displayed for the summation data. For example, I suspect you are still creating the hyperlink for that last line, but the text of the hyperlink is empty so you don't see the link. That's not a good idea. I would do the following: <?php include("db_connect.php"); include("functions.php"); $_GET=sanitize($_GET); $query = "SELECT COUNT(consultant.flow_id) AS flow_id_count, consultant.flow_id, full_name, client_index, sales_manager_index, role, street, city, consultant.state, zip, start_date, SUM(gross_margin) AS gross_margin_sum FROM contract, consultant WHERE contract.deleted='false' AND " . "consultant.deleted='false' AND contract.flow_id=consultant.flow_id AND start_date>=str_to_date('" . $_GET['start_date_begin'] . "', '%m/%d/%Y') AND start_date<=str_to_date('" . $_GET['start_date_end'] . "', '%m/%d/%Y') AND status='active' GROUP BY full_name"; ?> <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=utf-8" /> <title>Hire Report</title> <link rel='stylesheet' type='text/css' href='styles/page.css' /> <script type='text/javascript' src='javascript/sortable.js'></script> </head> <body> <table class='sortable' id='sortabletable' rules=cols> <thead><tr><th>Name</th> <th>Total Hires</th> <th>Client</th> <th>Sales Manager</th> <th>Role</th> <th>Street</th> <th>City</th> <th>Zipcode</th> <th>State</th> <th>Start Date</th> <th>Total Gross Margin</th></tr> <tbody></thead> <? //output the search results $total_count = 0; $total_margin = 0; $result = mysql_query($query); while($row = mysql_fetch_array($result)) { $recordDate = date("m/d/Y", strtotime($row['start_date'])); echo "<tr>\n"; echo "<td><a target='_blank' href='http://www.ajasa.com/cams/us_form.php?flow_id={$row['flow_id']}'>{$row['full_name']}</a></td>\n"; echo "<td>{$row['flow_id_count']}</td>\n"; echo "<td>{$row['client_index']}</td>\n"; echo "<td>{$row['sales_manager_index']}</td>\n"; echo "<td>{$row['role']}</td>\n"; echo "<td>{$row['street']}</td>\n"; echo "<td>{$row['city']}</td>\n"; echo "<td>{$row['zip'}</td>\n"; echo "<td>{$row['state']}</td>\n"; echo "<td>{$recordDate}</td>\n"; echo "<td>}$row['gross_margin_sum']}</td>\n"; echo "</tr>\n"; $total_count += $row['flow_id_count']; $total_margin += }$row['gross_margin_sum']; } echo "<tr><td></td><td>{$total_count}</td><td colspan=\"8\"></td><td>{$total_margin}</td></tr>\n"; ?>
-
Based upon the code you provided I don't see how you are even getting that last line that provides a summation of the values in the query. Did you leave some code out? EDIT: nm, I see you are using WITH ROLLUP. I don't use that and instead do the summary calculations in PHP. Let me take a look at what gets returned with that.
-
In my opinion I would determine the solution based upon the following question: Are there additional methods you want to execute against the results? If yes, then creating new objects from the results would make sense. If no, then I see no reason to create new objects out of the results.
-
As PFMaBiSmAd explained, the conditional blocks are not executed unless the condition is true. That means the include files would never be read into memory, except for the ones where the condition is true. But, I think you are really over thinking this. unless you are building a site that will have massive traffic from day one, build it to be logical and functional. The additional time for the parser to read all the code in one page would be inconsequential. But, as I stated above you should break the code out into separate pages just for the fact that it would be more logical and be easier to manage. The fact that the code won't need to be read (unless needed) is an added benefit. You should always take performance into consideration, but don't go overboard. You can spend 10x the effort to squeeze every ounce of performance out of the application and only get 5% increase in performance. That's not to say there aren't some things you should absolutely do and/or not due. The biggest performance issue I see in most scripts posted to this site is where the scripts run queries within loops. You should never do that.
-
There should be no significant performance if those lines are not actually being executed. There is always a cost as you add more complexity, but unless the files grew to many megabytes in size you should never see a difference. But, on the other hand I would suggest breaking out the code just for the sake of maintainability and flexibility. Have your five forms submit to the same page, but break the processing code for each form into separate file and use a switch to determine which processing code to include. if (isset($form1)){ include('form1processing.php'); } if isset($form2)){ include('form2processing.php'); } // Etc. That makes your files much more manageable IMHO. I abhor files with tons of code. It makes any modifications very difficult.
-
I have found the tutorials on the Tizag site very easy to follow. The tutorials start out with the basics and each one builds upon the previous ones. http://www.tizag.com/phpT/
-
It is not clear from your query where the comments are stored - or even if they are included in that query. I would think that the comments would be stored in a separate table with a reference back to the post. Are the posts and comments all stored in the wall_feeds table? If so, please provide the structure.
-
You can't dynamically update the content in a web page with just PHP. You will need to implement AJAX (client-side java script with server-side PHP) to dynamically query the server and get the updated info, then use the javascript to populate the new data on the page.
-
Finally, someone that thinks like me. I've never understood any reason to use mysql_fetch_array() when mysql_fetch_assoc() has all the data you need, and nothing else, with all of it nicely associated with keys that *should* have intuitive names.
-
Yeah, I knew that, don't know what I was thinking. I see that the problem is he is referring to the result field incorrectly. I don't know if there is a default field name given with a group by aggregate function. So, you are right he could use the 0 index or give the field an alias int he select query, which is whay I usually do. mysql_connect("connect.php"); $query = "SELECT SUM(Games) as gamesSum FROM stats"; $result = mysql_query($query) or die(mysql_error()); // Print out result $row = mysql_fetch_array($result)) echo "Total Games: $row['gamesSum']";
-
Everything has a cost. How many notifications will you have each day and how many concurrent requests will you have? Even if your answer to both of those is A LOT, you are still better off only selecting the records you want to display and then creating an automated process to delete records once a week or some other infrequent time period as is needed. You then get only the records you want displayed and you don't incur the unnecessary overhead of having to constantly delete records throughout the day. Doesn't that make more sense?
-
I'll give you a hint. The MySQL SUM() function is listed in the manual under http://dev.mysql.com/doc/refman/5.0/en/group-by-functions.html Check this tutorial on using SUM() http://www.tizag.com/mysqlTutorial/mysqlsum.php