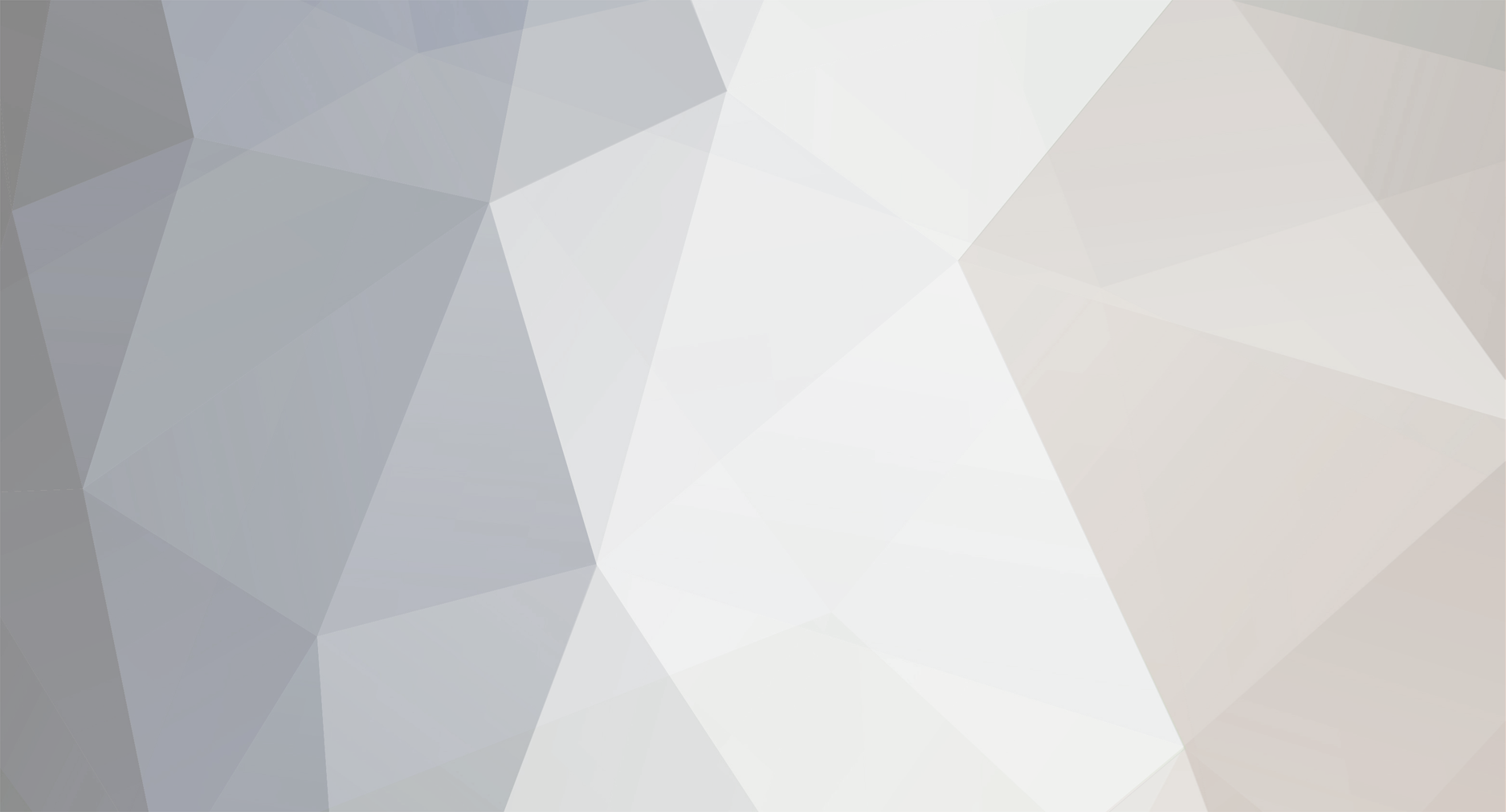
Psycho
Moderators-
Posts
12,146 -
Joined
-
Last visited
-
Days Won
127
Everything posted by Psycho
-
@benanamen: This is bad advise. You should never use hidden fields to pass 'critical' data to be relied upon. All data passed via GET, POST, COOKIE, etc. should assumed to be malicious, tainted, etc. It is a trivial task to manipulate data in a hidden field. Hidden fields have their purpose but, in this case, there is no good reason to use it since you would have to compare the POST value to what is in the DB on each submission anyway. So, the logical solution is to just rely upon the DB value anyway.
-
This makes no sense $_POST['grand_total'] = $_SESSION['grand_total']; What is the purpose of setting the value of a POST value? I will assume that you have code that was hard-coded to use the value sent via POST and instead of refactoring that code you decided to just overwrite the POST value using the SESSION value. I would highly recommend refactoring the code to correctly use the appropriate value. If you have code that is referencing POST values when you aren't actually using them it will lead to confusion later on. Secondly, why use SESSION at all? You should have a DB record for the order and you can get the current/correct value on any page load that you need it. Session data can be hijacked. I'm not an expert on all the vector points of attack and someone would need to review more of your code to know if you are at risk. But, using the data stored in the DB is far safer.
-
There is a freelance forum if you want to find someone to do work for you at a price
-
+1. I "mostly" follow those standards with only a couple exceptions. But, as Jacques1 states, consistency is key. But, I would definitely try to somewhat follow a common standard. Even if you are the only one that will work on the code it would be worthwhile when requesting help on this forum. And, as others have stated USE COMMENTS.
-
This forum is for people to get help with code they have written. Copy/pasting a ton of code and asking us to try and decipher it is a lot to ask from people providing support for free. Can you show the specific code for retrieving/displaying the content on the players_13 page?
-
Skinning a cat (Or how many ways to output "Hello World!")
Psycho replied to benanamen's topic in Miscellaneous
You'll have to wait for this one. Shuffle really isn't 'random' - it took 245,760 iterations to get the value (every time) on my machine. $letters = array('H','e','l','l','o',',',' ','W','o','r','l','d','!'); $string = ''; while($string != 'Hello, World!') { shuffle($letters); $string = implode('', $letters); } echo $string; -
Skinning a cat (Or how many ways to output "Hello World!")
Psycho replied to benanamen's topic in Miscellaneous
This code grabs the text from this forum post. Sort of a recursive implementation. $url = 'http://forums.phpfreaks.com/topic/298555-skinning-a-cat-or-how-many-ways-to-output-hello-world/'; $content = file_get_contents($url); $output = preg_match("#Or how many ways to output ([^\)]*)#", $content, $match); echo str_replace('"', '', $match[1]); -
Skinning a cat (Or how many ways to output "Hello World!")
Psycho replied to benanamen's topic in Miscellaneous
foreach(array(72,101,108,108,111,44,32,87,111,114,108,100,33) as $l) { echo chr($l); } -
Well, if we're just showing different ways to skin a cat: $fun = array( 'First Word' => 'Programming', 'Second Word' => 'in', 'Third Word' => 'PHP', 'Fourth Word' => 'is', 'Fifth Word' => 'fun!', 'Programming in PHP is fun!' ); $fullSentence = array_pop($fun); foreach($fun as $key => $val) { echo "$key, $val<br />"; } echo $fullSentence;
-
read a cvs file and only displaying some data
Psycho replied to manhattes's topic in PHP Coding Help
How you do this depends on how you will use it. You are pulling financial data from Yahoo. If you were to have a LOT of requests, Yahoo could block you. How often is the data updated? It may make sense to parse the data and store it for repeated requests for some amount of time then, after that time expires, fetch new data. But, that's really a different questions. Also, do you ONLY need the date with a specific date? If so, add the logic to the code that reads the data. So, to your code. If the resources is really a CSV file, then the current process is flawed. You don't need to split using \n or on the commas. In fact, that can break if there are other line breaks or commas in the data. PHP has functions for reading csv files which you should use: http://php.net/manual/en/function.fgetcsv.php When reading each line, you will want to test the date field to see if it matches the ones you are looking for. Without knowing the format of that field it is impossible to provide any sample code. Run your code above and change the for loop to this and show us the output for($i=0;$i<count($lines);$i++) { echo "<pre>".print_r($lines[0], true)."</pre>"; } -
update the data in the database from the HTML form
Psycho replied to sigmahokies's topic in PHP Coding Help
sigmahokies, You need to understand that just because something works does not mean it is correct. I can store a user's password in plain text in the database and it will work, but is absolutely wrong. Yes you "can" pass all of the data and it will work. But, it is not correct and will eventually cause you problems either in this project or another one some time later if you do the same thing. You should treat ALL user supplied data as "dirty". It could be that something is corrupted unintentionally or, worse, it malicious data intended to do harm. For your page above, it should only accept the ID for the process of setting up the form for edit. The code should do a SELECT query to get the current data instead of relying upon data submitted by the user via $_GET. Here is a quick rewrite of your code in a more logical format. This is not what I would consider complete, but is more complete than the current code and shows an example of a logical flow. There may be some minor errors in syntax as I did not test it. <?php $Garydb = mysqli_connect("XXXXX","XXXXX","XXXXX") or die("Could not connect MySQL Database"); mysqli_select_db($Garydb, "XXXXX") or die("Could not find a Database"); $response = ''; if(isset($_GET['id'])) { //User passed the ID of a record to be updated //Get current values to populate form field $id = intval($_GET['id']); $query = "SELECT First_name, Last_name, Locations, birthdate, Email FROM WHERE ID = {$id}"; $result = mysqli_query($Garydb, $query); if(!mysqli_num_rows($result)) { //No record matching the passed ID $response = 'Error: No record matching requested id.'; } else { //Define variables for form fields from current DB result $row = mysqli_fetch_assoc($result); $first_name = $row['first_name']; $last_name = $row['last_name']; $locations = $row['locations']; $birthdate = $row['birthdate']; $email = $row['email']; } } elseif ($_SERVER['REQUEST_METHOD']!='POST') { //User posted a form if data to be updated for a record $id = intval($_POST['id']); $first_name = trim($_POST['first_name']); $last_name = trim($_POST['last_name']); $locations = trim($_POST['locations']); $birthdate = trim($_POST['birthdate']); $email = trim($_POST['email']); if ($id && $first_name && $last_name && $locations && $birthdate && $email) { //All the posted value are not empty/zero //Should really have better validation logic $update = "UPDATE Members SET FirstName = '$first_name', LastName = '$last_name', Locations = '$locations', birthdate = '$birthdate', Email = '$email' WHERE ID = $id"; if(!mysqli_query($Garydb, $query) || ) { //Query failed $response = 'Error: Unable to update record.'; } elseif(!mysqli_affected_rows($link)) { //No records were updated. Record may have been deleted or ID manipulated $response = 'Error: No records updated.'; } else { $response = 'Record was updated.'; } } else { //Not all fields have values or non-zero $response = 'Error: Missing data required for update.'; } } else { //No POST or GET data submitted $response = 'Error: No data received.'; } ?> <!doctype html> <html> <head> <title>Update Members info</title> </head> <body> <?php //If there was a response, show it. Else show form. if($response) { echo "<div style=\"color:red;\">{response}</div>"; //Could add a link to go back to a listing page or somewhere appropriate } else { ?> <form action="insert.php" method="POST"> <table> <tr> <td>Identify Number:</td> <td><?php echo $id ?><input type="hidden" name="id" value="<?php echo $id; ?>"</td> </tr> <tr> <td>First Name:</td> <td><input type="text" name="first_name" value="<?php echo $first_name; ?>"></td> </tr> <tr> <td>Last Name:</td> <td><input type="text" name="last_name" value="<?php echo $last_name; ?>"></td> </tr> <tr> <td>Locations:</td> <td><input type="text" name="locations" value="<?php echo $locations; ?>"></td> </tr> <tr> <td>birthdate:</td> <td><input type="text" name="birthdate" value="<?php echo $birthdate; ?>"></td> </tr> <tr> <td>Email:</td> <td><input type="text" name="email" value="<?php echo $email; ?>"></td> </tr> <tr> <td colspan="2"><button type="submit">Update</button></td> </tr> </table> </form> <?php } ?> </body> </html> -
update the data in the database from the HTML form
Psycho replied to sigmahokies's topic in PHP Coding Help
Your UPDATE query has a WHERE clause as follows: WHERE ID='$id' The value of $id is defined here: $id = $_GET['id9']; However, there is no 'id9' field in your form. You provided a name for a TD element <td name="id9"><?php echo $edit ?></td> A TD element is not a form field and will not be included in the data passed with the form submission. You should use a hidden input field - although I usually use a text field at first so I can ensure the field is getting populated. There is a lot wrong with what you are doing. I hope this is just a learning exercise. -
Fatal error: Call to undefined function: filter_input()
Psycho replied to keaauBoy's topic in PHP Coding Help
According to this post, for what appears to be the same issue, the OP found that his host supported PHP5 but parsed php files using PHP4 by default. He solved the issue by naming his files with a .php5 extension. I know some hosts also allow you to set the default parsing method. -
Filtering data using filter_var_array before storing it into a DB
Psycho replied to ajoo's topic in Applications
The data you are receiving it url encoded. So, you should decode it first. Then parse the data into the specific values. You can then save the individual values into appropriate database fields. Rough example: $stringFromJava = "4%2C3%2C4%2C3%20%2C4%2C3%2C4%2C3%20%2C2%2C3%2C2%2C3%20%2C3%2C3%2C3%2C3%20%2C3%2C3%2C3%2C3%20"; $stringFromJava = urldecode($stringFromJava); $outputAry = array(); $groups = explode(' ', $stringFromJava); foreach($groups as $group) { $valuesAry = array(); $values = explode(',', $group); foreach($values as $value) { //If value is not numeric, skip it if(!is_numeric($value)) { continue; } //Add value to group values array $valuesAry[] = $value; } //Add validation logic for the group if(count($valuesAry) != 4) { continue; } $outputAry[] = $valuesAry; } echo "<pre>" . print_r($outputAry, TRUE) . "<pre>"; Output: Array ( [0] => Array ( [0] => 4 [1] => 3 [2] => 4 [3] => 3 ) [1] => Array ( [0] => 4 [1] => 3 [2] => 4 [3] => 3 ) [2] => Array ( [0] => 2 [1] => 3 [2] => 2 [3] => 3 ) [3] => Array ( [0] => 3 [1] => 3 [2] => 3 [3] => 3 ) [4] => Array ( [0] => 3 [1] => 3 [2] => 3 [3] => 3 ) ) -
Filtering data using filter_var_array before storing it into a DB
Psycho replied to ajoo's topic in Applications
Sounds like you have a string and not an array - what is the DB field type that you are storing this in. Would seem this needs to be sanitized as a string. But, . . . If you are storing data as comma separated values into a DB, then you are doing it wrong. Without knowing what the data represents, it is impossible to provide concrete advice. But, I would think this should be stored in a single separate table. I will assume each "group" of numbers is a record and each value in the group correlates to different values. So, you might have a table that has fields such as this: id: primary key for the 'array' records rec_id: a foreign key reference to the records for which this data is associated with val_1: the first value val_2: the second value val_3: the third value val_4: the fourth value Obviously, the fields shoudl be given more descriptive names. But, that format allows you to associate one or more "records" (e.g. 1,2,3,4) to some other entity. -
Because he doesn't know the full scope and purpose of your database. I agree with Jacques1 in that you should not delete rows from one table just to populate the same data into another table. There are some legitimate reasons why that might be appropriate, but I'm confident that is not the case here. If you need the data for historical purposes, just have a column in the table for a "deleted" flag. Then, YOU need to analyze your table. Are there columns that need to be unique (other than an internal primary key)? If so, those constraints could be moved to the business logic. But, assuming there are no unique fields other than the primary key it is much simpler. Then you would just need to make one last decision. It is assumed that most/all current queries should not return/operate on these "deleted" rows. depending on how many queries you have already built and/or the complexity you expect there will be you could 1) Modify the queries to exclude/include the deleted records as needed or 2) Create a VIEW with the records already filtered based on the deleted field and user that in the applicable queries.
-
dynamically populate dropdown list based on MySQL response
Psycho replied to SF23103's topic in Javascript Help
Create a function and call it within the ajax response code. Here is some untested code: In the ajax response code, something like: updateSeatsAvail($('input[name="seatch"]'), response.seats); function updateSeatsAvail(selObj, seats) { selObj.empty(); //Remove current seat options for(var seat=0; seat<=seats; seat++) { selObj.append($("<option></option>").attr("value", seat).text(seat)); } } -
Let me state it simply. You cannot send/set headers if any output has been made to the browser. The includes echo/print statements as well as any content not within PHP tags. In the code you just posted there is such content: $_X=base64_decode($_X);$_X=strtr($_X,'SgPO9YZWFKmqyfxcjLJRzuM5vNts1b.{B4nC]i/2Dl0EheA [d8=Qp>VXo H}6GIw7ka3TrU<','=R9odmplAEPyk8gv[53xrMezqZHi7YhW<DsG{>CcX}1N/afj6]JtuS .BUnwVKLQO20ITF4b');$_R=str_replace('__FILE__',"'".$_F."'",$_X);eval($_R);$_R=0;$_X=0; ?> <<== this would be output to the browser of at least two line breaks characters! <?php /** * * @ Universal Decoder PHP 5.2 Let me also try to again explain WHY this is the case. Your browser uses the headers to determine how to handle the content that it will be sent. It may be an HTML page, a download, a PDF, etc. The browser will do different things for each of those situations. If the browser receives headers that it will be sent an HTML page, but is instead sent a PDF file you will see a lot of "junk" displayed in the browser. That is why the headers must all be sent before any content is sent. As soon as any content is detected by the browser (even a space or line break) it has to commit to how it will process that content.
-
Well, let's start with the form creation. 1. Do NOT use PHP short tags for code blocks. 2. Do NOT use the mysql_ library - it has been deprecated for many years. Either use mysqli_ or, better yet, PDO 3. You create a loop to store the DB results in an array and then immediately iterate over the array to use those values. Why not just create the output when reading the DB results? There are reasons why separating the logic to get the data vs. using the data makes sense. But, in this instance is it a waste of code 4. There is no need to create the variable $c to use as the index in the array. You can simply append a new value to an array without defining the index and the next numeric index will be used automatically 5. The checkbox inputs have duplicate IDs. This is invalid code. They can have the same name - but not the same ID 6. The variable $career is getting written AFTER the input field is output. So, each input has the value for the previous checkbox. 7. The value of the checkboxes should be the ID - not the name You state "Then my form have an action=" " but is conected to 'function save'". What does that mean. You can't connect a PHP function to a form. The form is submitted and the PHP code determines what to do with it. A function would not get executed automatically. There's no way to tell if that function is even getting called based on what you've provided. But, I am assuming it is not getting called. The reason is the function expects certain parameters to be passed, but you are then defining the parameters within the function. If those parameters are not passed the function would fail and an error would be displayed on the page. Try this for the form <p><label>career:</label></p> <table border="0"> <td> <p> <?php $sql = "SELECT * FROM career ORDER BY id_career asc"; $res = mysql_query($sql); while($row = mysql_fetch_assoc($res)) { $id = $row['id_career']; $label = $row['career']; echo "<input type=\"checkbox\" name=\"career[$id]\" id="career[$id]" value=\"$id\">$label<br>\n"; } ?> </p> </td> </table> On the page that receives the form you will need to show the code that calls the function.
-
The message is as it says. Content has already been sent to the browser (e.g. echo/print or anything not within PHP tags). Look at file admin_controller.php (post the first 20 lines if you want feedback on it). The headers for a page request must be sent before the content. The headers contain information for the browser on how to handle the page. After content for the page starts, the browser is already committed to how it will handle the page (either with the headers that were already sent or using defaults). So, you need to go through any logic that may determine any header output without outputting any content. You can store potential content within a variable for output later.
-
How to access subdirectory without typing it to url?
Psycho replied to Andy_Kemp's topic in Apache HTTP Server
You can also change the 'root' folder for your site. Not knowing what you are using for hosting, I can't give you specific instructions. But, if you are with a host, they should have the ability to configure your domain. Just point the domain to the frontend folder (I assume that none of the files in the backend folder need to be directly accessible via a browser). If you do this, then frontend/ will be the root for your domain and no one would be able to directly access files in backend/ -
Just show the output of this: var_dump($cities); We want to verify that there is a value that starts with 'E' (not 'e') and that the value doesn't start with a space or some other non-printable character.
-
I was just going to suggest the same thing. The SELECT query and the loop are completely unnecessary for what is being accomplished here.