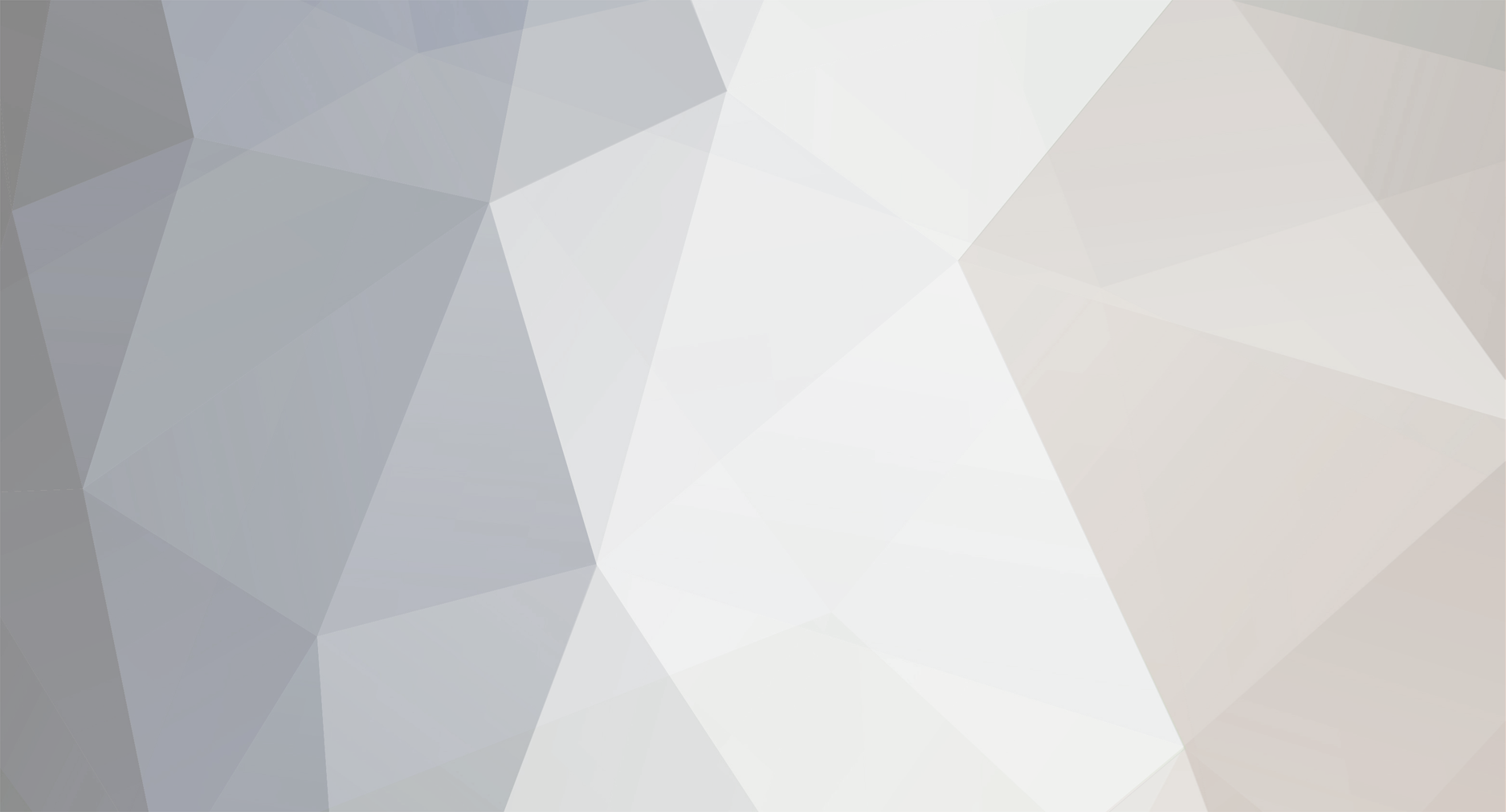
Psycho
Moderators-
Posts
12,164 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
The reason why the values are getting set to 0 is simple - looking at your previous code (and comments) and the current code should tell you why As your comment in the prior post shows you are retrieving the results as an array. But, you are trying to bind the value as if it is an object. But, you are doing this the wrong way. There is no need for a select query. Just the update query. Also, you are using prepared queried all wrong. You prepare the query one time, then execute it multiple times while changing the bound data.
-
Login script. Join table "user" to table "admin"
Psycho replied to Andy_Kemp's topic in PHP Coding Help
Not, to be rude but, yes, I think you are missing something. I am not talking about treating the "super admin" as an anonymous user by any means. Based on one of the OP's previous responses he intends to have "roles" that can be customized to have different permissions granted or not. The Roles table would still have a record for the Super Admin role (id = 1) and the same tables the OP described above would still exists to describe the permissions available for all the other role types (but not the super admin). Upon login, the system would store the role id in a session variable and upon attempting to access any functionality that requires a specific permission a service would be called to check if the user is assigned to a role with rights to that functionality. That service could look like this: //Very generalized code function checkPermision($roleID, $permissionID) { //Always return true for super-admin if($roleID==1) { return true; } //Else, query the DB to determine if the user's role has rights to the permission //Will return 1 if role has the permission, else 0 $query = "SELECT COUNT(*) FROM role_permission WHERE role_id = $roleID AND permission_id = '$permissionID'"; $result = mysqli_query($link, $query); $mysqli->query($query) $row = $result->fetch_row()) //Retuyrn 1/0 (i.e. true/false) return $row[0]; } The reason to do this is it ensure that the super admin does not lose permission because the role based permissions are accidentally deleted, corrupted, whatever. Imagine the scenario where only the super admin has permissions to edit roles and somehow that permission got deleted for the super admin. There would be no way to resolve the issue through the application. It would require someone with knowledge of the application and access to the DB to create a query to manually add/edit the necessary records. This could be catastrophic if it were to occur when the application is mission critical. Sure, you can build the application to prevent such things, but those pesky business rules to mandate very narrow logic such as that are the ones that get tested the least. And - bugs happen. By always returning true for the Super Admin when checking permissions you do not need to worry about any permission being removed either intentionally or by accident. -
Login script. Join table "user" to table "admin"
Psycho replied to Andy_Kemp's topic in PHP Coding Help
One suggestion: I assume the super_admin role would have full permissions and should never have limited permissions. So, you don't need to have any records in the role_permissions table for the super_admin. Instead, if the role is super_admin just assume all permissions are available. The reason for this is if any records from the role_permissions table were inadvertently deleted the super_admin could lose the ability to do things that no one has the rights to do. This basically ensure the system doesn't come to a halt. You would likely have a service to check a permission for a user based on their role. That service would simply return true is the user is the super_admin instead of checking the role assignments. -
Is there any way to grow my server's grabbing script?
Psycho replied to Rayhan420's topic in Apache HTTP Server
There are literally hundreds, if not thousands, of way to "optimize" code. It all depends on what your code is specifically doing. If you are running queries in loops - don't. If you are using RegEx try and find string function alternatives, etc. etc. etc. It is a waste of time for us to try and suggest optimizations that may be appropriate for your need without seeing any code. -
Also, you would not put quotes around the values within the query string and the values should be URL encoded (e.g. a space would be %20 www.mypage.com?name=John%20Smith
-
I've not tested it, but I would think having different form names would work too.
-
I have no clue about Laravel. What I posted was just an SQL query.
-
Looks like you are using PDO, not MySQLi_. PDO has the rowcount function. But, the manual states it may not work for SELECT queries on all databases. So, try it beforehand. You can always run a COUNT() query or loop through the results to get the count. http://php.net/manual/en/pdostatement.rowcount.php
-
I had to make some assumptions on the field names in the users table: SELECT DISTINCT users.username FROM users JOIN expectation_profile ON users.profile_id = expectation_profile.profile_id WHERE users.profile_id <> $currentUserID AND expectation_profile.expectation_id IN ( SELECT expectation_id FROM expectation_profile WHERE profile_id = $currentUserID )
-
Per the manual, fopen() should return an error of E_WARNING level. Even though you removed the @, I suspect the error reporting is set to a level where warnings are not displayed. Turn on full error reporting and see what is reported.
-
Searching a particular Column Instantly across all rows
Psycho replied to natasha_sharma's topic in MySQL Help
PHPMyAdmin is a standalone "administrative" application - it is not meant to be used to build functionality. It is only meant for developer use. If you wanted to hack the PhpMyAdmin code, yes, I guess you could do that. If you are wanting to build some sort of user facing feature, then your question should be about using the MySQL database. What does AJAX have to do with this? The back end processes needed are still the same. AJAX would only prevent a page refresh. Which is not to say there is no value to AJAX, it's just not the most important thing in getting what you state you are after. Again, PhpMyAdmin is used to administrate the MySQL DB. You can manually create/run queries in PhpMyAdmin if you want, but it is not for user-facing features, therefore it is not feature rich in such functionality since it would be expected that someone that can write a query would be using it. That is the responsibility of the developer. You didn't answer my last question, do you have the experience to create such queries? I'm not trying to be rude, but it sounds as if you do not. So, you can either learn to do so, find someone else to do it for you, or look for some pre-build script to do it for you. This forum is for people to get help with code they have written (or at least those that make an attempt). As for finding some pre-built script, it will almost certainly require the person implementing it to do some configuration to make it work right for the table being used - which would require some knowledge of how to use a database. Plus, if you don't understand the script you could inadvertently implement a feature with gaping security holes. -
[Moving to MySQL Forum] There is no indexing 'method', but there are best practices. There are many different considerations when determining what to index and I am by no means a DBA. I would suggest reading up on the subject. There are also ways to analyze queries to determine where indexes may be needed. Here are a few resources that may be of help. http://dev.mysql.com/doc/refman/5.7/en/mysql-indexes.html https://dev.mysql.com/doc/refman/5.7/en/using-explain.html http://www.databasejournal.com/features/mysql/article.php/3840606/Maximizing-Query-Performance-through-Column-Indexing-in-MySQL.htm http://www.slideshare.net/matsunobu/more-mastering-the-art-of-indexing
-
Searching a particular Column Instantly across all rows
Psycho replied to natasha_sharma's topic in MySQL Help
What, exactly, are you trying to accomplish? If you are doing this in PHPMyAdmin, then I assume you are just doing this to verify/correct some data. If this needs to be a user facing feature, then you need to build a page to allow a user to perform a search with relevant inputs and a query that will consume those inputs. But, even if you are just needing this data in PHPMyAdmin, the solution is the same - you need to build the relevant query and run it. Do you have any experience with constructing SQL Queries? E.g. SELECT * FROM table_name WHERE field_name = 'some_value' -
Use two <select> inputs with the relevant hours and minutes as <options>. Then on the page that receives the data, concatenate the two values with a colon.
-
To add to cyberRobot's response. In addition to checking if $_GET['varname'] exists, you would likely have a similar error when you try to reference $isTtex later in the code if it does not get defined, so I would suggest the following: $isTtex = (isset($_GET['varname'])) ? $_GET['varname'] : 'some_default_value';
-
Great point. This is something that most sites overlook, even some large technology companies. In fact, some sites exacerbate the problem by implementing a service call to specifically check for a duplicate email address. A bot can easily harvest "good" email addresses using these sites for spamming or other nefarious purposes. There is an excellent training video that covered this and other security issues that are commonplace that I would recommend (Requires a license or sign up for a free trial.): https://www.pluralsight.com/courses/play-by-play-website-security-review-troy-hunt-lars-klint. Lots of other great resources on that site. The video covers a lot issues related to business rules, not just coding issues. Programmers need to understand "why" certain things should not be done that a customer or product manager may be requesting to advise them as to the problems their request would create.
-
Splitting a string based on the last instance of a character
Psycho replied to sn00pers's topic in PHP Coding Help
I only lost the last part because I used pop() instead of the correct nomenclature of array_pop(). Other than that, the code retrieves both parts $source = "2001:db8:a0b:12f0::1:25"; $split_chr = ':'; $parts = explode($split_chr, $source); $last = array_pop($parts); $first = implode(':', $parts); echo "First: {$first}<br>\n"; echo "Last: {$last}<br>\n"; -
Splitting a string based on the last instance of a character
Psycho replied to sn00pers's topic in PHP Coding Help
If you're going to use explode, then might as well use pop() to remove the last element and implode() the remaining elements back together $split_chr = ':'; $parts = explode($split_chr, $source); $last = pop($parts); $first = implode(':', $parts); -
I agree with the rest of your last post, but as for this part, it is not necessarily true. My organization hosts applications that deal with data which has very stringent legal requirements for data access. We have a deployment organization that is responsible for the maintenance of the environments and the deployment of new releases. Even in that organization there are only a handful of people that have access to the databases and secured files in those environments. Developers do not have access to the production environments and there are very detailed processes and auditing for them to request data when troubleshooting issues. Even though a developer would know how to decrypt a file, they wouldn't have access to it. This is by no means fool-proof, a developer could work with a deployment engineer to get the data out of normal processes or a deployment engineer could try to get the source code or just brute force the encryption. My point is that securing sensitive files from developers is a valid (and sometimes legal) requirement.
-
Warning: mysql_real_escape_string() expects parameter 1 to be string,
Psycho replied to jarvis's topic in Applications
According to the manual for the update method, the second parameter should be an array of name/value pairs. Instead you are passing a name/array. 'meta_value' is supposed to be a field name and $meta_value is assumed to be a value for that field. You are passing an array for for $meta_value. You can't store an array as the value for a field. Not knowing exactly what you are trying to achieve, I can't provide the correct solution. I'm thinking you should either be storing the data in fields named 'address', 'lat', and 'long' using this $wpdb->update( $wpdb->postmeta, $meta_value, array( "meta_key" => 'location', "post_id" => $post_id ) ); Or, you may need to convert the array to a string. Definitely not advised to store data in this manner, but since you are using word-press, I'm not sure what your options are $value = implode(", ", ); $wpdb->update( $wpdb->postmeta, array('meta_value' => $value), array( "meta_key" => 'location', "post_id" => $post_id ) ); -
There are many options, but the best approach depends on many factors from what control you have to the business needs. Here are a few options: 1. Store the files on a drive location that is encrypted and which the developers do not have direct access. This requires that you have control of the server/environment. You could just store them in a location that the developers don't have access to, but not encrypted, depending on the significance of the data. 2. Create a process such that when files are uploaded they are encrypted before stored on the drive. The encryption key can be unique and random for each file to increase the entropy. So, no one would be able to 'open' a file without decrypting it first. If someone had the actual flat-files, they would still need the encryption key. Access to the database should be strictly controlled as well. 3. Store the files in the database - and encrypt them. Note, that no "encryption" method is 100%. Given enough time and processing power anything can be cracked. So, if you were to just encrypt the flat files, if someone could access those files, they could copy them off to try and brute force decrypt them.
-
Combining the previous two suggestions <?php $dbh = new PDO("mysql:host=$hostname;dbname=$db", $username, $password); $query = 'SELECT username, COUNT(*) as number FROM ballot GROUP BY username ORDER BY username'; $output = ""; foreach($dbh->query($query) as $row) { $output .= "<tr>\n"; $output .= "<td>" . htmlspecialchars($row['username']) . "</td>\n"; $output .= "<td> . htmlspecialchars($row['number']) . "</td>\n"; $output .= "</tr>\n"; } ?> <html> <head></head> <body> <table> <?php echo $output; ?> </table> </body> </html>
-
Because, that was how the language was built.
-
Ok, that is something else entirely. You are not looking for ONE record that matches those two conditions. You are looking for a record which has TWO associated records that match each of those conditions. Barand might have a better suggestion, but I would JOIN the dependent records twice: once with the first conditions and again with the second conditions. But, looking at your query, I don't see how that can logically be done. You are JOINing the 's' table (a subquery) independently from the 'sv' table (specific_values). I don't see the specific relation ship between the values and specific_ids.