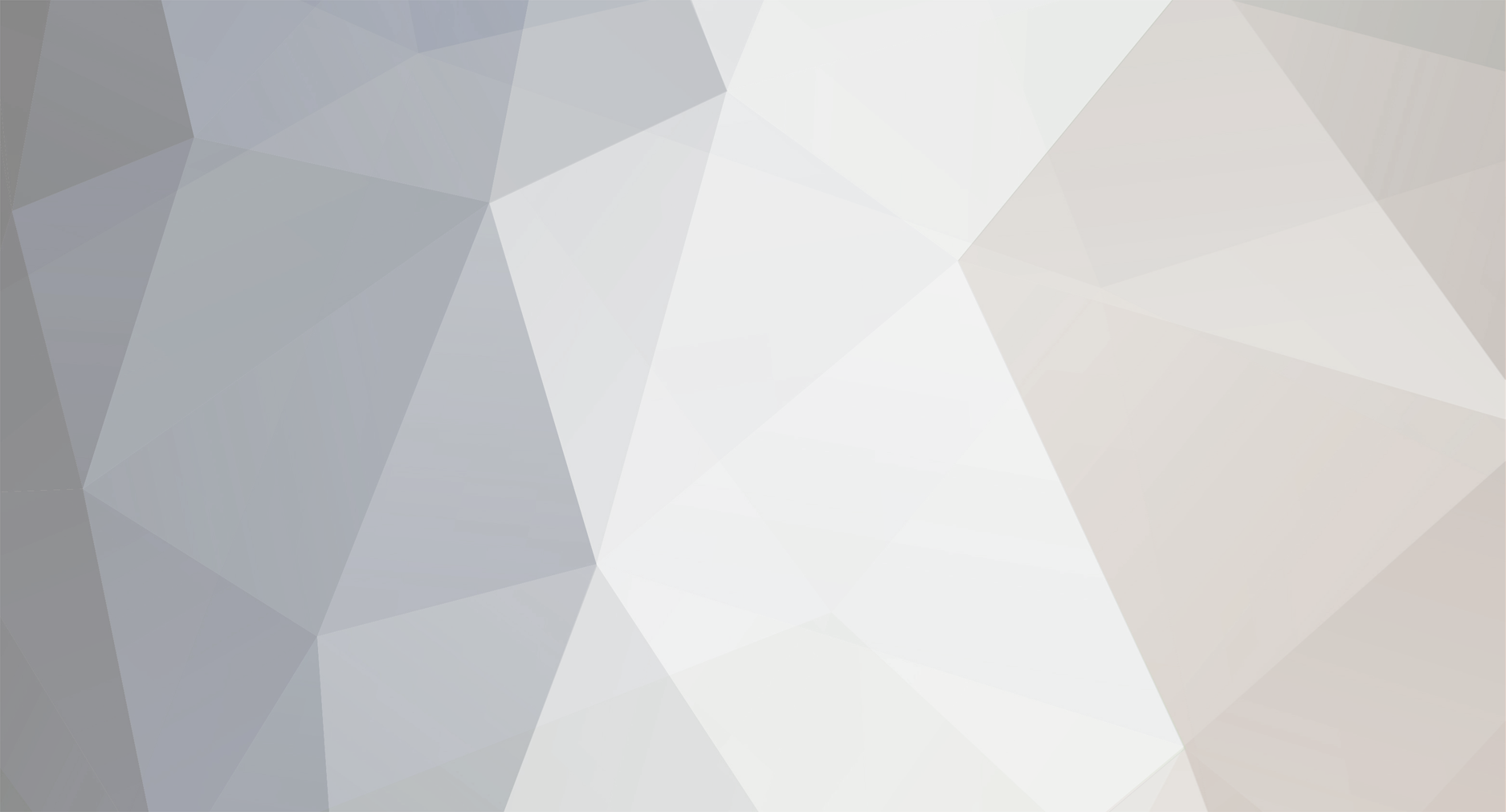
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Or, use the ternary operator function toggleDelete(obj, el) { document.getElementById(el).style.backgroundColor = (obj.checked) ? 'red' : 'transparent'; }
-
As I already stated you can pass a unique value via GET/POST vars, but those can be easily determined and replicated by a user since the values would have to be determine in the source of the HTML page.
-
I can't say for sure, but I think the answer is no. When an ajax call is made it is coming from the user's browser anyway. You could add POST/GET parameters to the AJAX request to specify the calling page, but the user could easily replicate that if they wanted. One thought though is to use a session variable as a flag. I typically have a default script that is included at the top of every page that is user accessible which sets database connection info, default variables, etc. Assuming you are doing the same thing, just set a session variable on every page load to identify the page being viewed. Then when the AJAX call is made, the server AJAX script could check that session variable to see if it is the home page. If not, have the script return false or an empty string.
-
using a calendar script, how to prevent old date selected
Psycho replied to jasonc's topic in Javascript Help
You *can* prevent the popup calendar from allowing the user to input previous dates as well. But, I would definitely use MrAdams solution as well. By the way, the code you are using has an error in the logic to determine the number of days in February: if (m == 1 || m == 3 || m == 5 || m == 7 || m == 8 || m == 10 || m == 12) { days = 31; } else if (m == 4 || m == 6 || m == 9 || m == 11) { days = 30; } else { days = (y % 4 == 0) ? 29 : 28; } That formula for determining days in february is not correct. It should be this days = (y%4==0 && (y%100!=0 || y%400==0)) ? 29 : 28; To disable the prior year or month links when they would take you to a period before the current date replace the ds_template_main_above() function with this: function ds_template_main_above(t) { return '<table cellpadding="3" cellspacing="1" class="ds_tbl">' + '<tr>' //Prior Year link + ((new Date(ds_c_year-1, ds_c_month, 1)<ds_i_date) ? '<td class="ds_head" style="color: #cecece"><<</td>' : '<td class="ds_head" style="cursor: pointer" onclick="ds_py();"><<</td>') //Prior Month link + ((new Date(ds_c_year, ds_c_month-1, 1)<ds_i_date) ? '<td class="ds_head" style="color: #cecece"><</td>' : '<td class="ds_head" style="cursor: pointer" onclick="ds_pm();"><</td>') + '<td class="ds_head" style="cursor: pointer" onclick="ds_hi();" colspan="3">[Close]</td>' + '<td class="ds_head" style="cursor: pointer" onclick="ds_nm();">></td>' + '<td class="ds_head" style="cursor: pointer" onclick="ds_ny();">>></td>' + '</tr>' + '<tr>' + '<td colspan="7" class="ds_head">' + t + '</td>' + '</tr>' + '<tr>'; } Then to prevent a day within the currently displayed month from being selected when it is before the current day, change the ds_template_day() function to this: function ds_template_day(d, m, y) { if (new Date(y, m-1, d)>=ds_i_date) { return '<td class="ds_cell" onclick="ds_onclick('+d+','+m +','+y+');">' + d + '</td>'; } else { return '<td class="ds_cell" style="cursor:auto;color: #cecece">' + d + '</td>'; } } -
Here is a breakdown of the pattern Crayon Violent supplied: ~^([a-g]|h([a-e]))~i ~ = delimiter ^ = match must start at the beginning of string [a-g] = letter must be 'a' through 'g' (because of the ^ the match has to be at the beginning of the string) | = OR h([a-e]) = letter must be an 'h' followed by 'a' through 'e' (because of the ^ the match has to be at the beginning of the string) ~ = delimiter i = matching will be case insensitive
-
Well, if I am understanding your code correctly, the code that selects the records has a lot more complexity in determining the where clause: $sql = "SELECT * FROM behan WHERE 1=1"; //get the values from the form //NOTE: You should do way more valdation on the values before you attempt to process anything if ((!empty($_SESSION['be']))&&($_SESSION['be'] != 'alle')) { $sql .= " and be like '". mysql_real_escape_string($_SESSION['be'])."%' "; } if ((!empty($_SESSION['omraede']))&&($_SESSION['omraede'] != 'alle')) { $sql .= " and omraede like '". mysql_real_escape_string($_SESSION['omraede'])."%' "; } if ((!empty($_SESSION['pro']))&&($_SESSION['pro'] != 'alle')) { $sql .= " and pro = '". mysql_real_escape_string($_SESSION['pro'])."' "; } // ADD ORDER BY $sql .= " GROUP BY na order by total_value DESC $limstring "; The query to get all the records will need the same logic, otherwise you are basing the total records on a different subset than the query you are using to get the page records. I don't have the time to do a complete rewrite, but just create one process to generate the where clause and then use that for both queries. Here is an example: //Parse input for where clause $whereParams = array(); //get the values from the form //NOTE: You should do way more valdation on the values before you attempt to process anything if ((!empty($_SESSION['be']))&&($_SESSION['be'] != 'alle')) { $beSearch = mysql_real_escape_string(trim($_SESSION['be'])); $whereParams[] = "`be` LIKE '{$beSearch}%'"; } if ((!empty($_SESSION['omraede']))&&($_SESSION['omraede'] != 'alle')) { $omraedeSearch = mysql_real_escape_string(trim($_SESSION['omraede'])); $whereParams[] = "`omraede` LIKE '{$omraedeSearch}%'"; } if ((!empty($_SESSION['pro']))&&($_SESSION['pro'] != 'alle')) { $proSearch = mysql_real_escape_string(trim($_SESSION['pro'])); $whereParams[] = "`pro` = '{proSearch}'"; } //Create where clause $WHERE_CLAUSE = (count($whereParams)>0) ? " WHERE " . implode(' AND ', $whereParams) : ''; //Run queries to get total count and records for current page $getcountQry = "SELECT COUNT(*) FROM `behan` {$WHERE_CLAUSE} GROUP BY `na`"; $recordsQry = "SELECT * FROM `behan` {$WHERE_CLAUSE} GROUP BY na ORDER BY total_value DESC {$limstring}";
-
As I said, use the same WHERE clause conditions to get your total record count. Then determine the total pages on that.
-
Either: 1) The IP does not match (they can change you know) OR 2) The query is failing. Try the following: <?php date_default_timezone_set('America/Los_Angeles'); $timestamp = date('H:m:s m.d'); $admin = '24.68.214.97'; $visitor_ip = $_SERVER['REMOTE_ADDR']; $host = "mysql"; // Host name $username = "15557_test"; // Mysql username $password = "**********"; // Mysql password $db_name = "15557_test"; // Database name $tbl = "announce"; // Table name mysql_connect($host, $username, $password)or die("cannot connect to server"); mysql_select_db("$db_name")or die("cannot select DB"); $body = $_POST["body"]; if ($visitor_ip == $admin) { $query = "INSERT INTO {$tbl} (`body`, `date`) VALUES ('{$body}', '{$timestamp}')"; mysql_query($query) ordie("Query: {$query}<br />Error:".mysql_error()); } else { die("no"); } ?> <meta http-equiv="REFRESH" content="0;url=http://testchan.dcfilms.org/board-b.php">
-
Ok, I'm not going to read through ALL of that code. It is appreciated if you post just the relevant code. But, I think the problem is that you are using a count of ALL the records to determine the number of pages to display. But, the records are filtered according to what the user has selected. You need to determine the total i]relevant[/i] records based on the filter (i.e. the same WHERE clause used on the select query to get the current page records without the LIMIT clause). So, right now you are getting page links as if you had no filtering criteria.
-
It looks like from the above that the "best" value is the smallest value. You can just use min() instead of all those if/else statements. Anyway, you don't need all that code to sort the array, just a simple function along with usort(). I don't see any qXs in the input array or your code, so I will assume it is for the minimum of the q1s, q2s, q3s. This should be all you need: function customSort($a, $b) { //Primary sort on minimum value of q1, q2 & q3, if different $minA = min($a['q1'], $a['q2'], $a['q3']); $minB = min($b['q1'], $b['q2'], $b['q3']); if($minA != $minB) { return ($minA > $minB) ? 1 : -1; } //Secondary sort on minimum value of q1s, q2s & q3s $minA = min($a['q1s'], $a['q2s'], $a['q3s']); $minB = min($b['q1s'], $b['q2s'], $b['q3s']); if($minA != $minB) { return ($minA > $minB) ? 1 : -1; } //Both minimums are equal return 0; } usort($indexH[$var], 'customSort'); ?> Note: I always mix up the 1 and -1 for how they should sort. So reverse those values where is does not sort as needed.
-
NEED HELP w displaying multiple files with similar filenames!
Psycho replied to prototype18's topic in PHP Coding Help
As long as you can access the "default" file I see no reason you wouldn't be able to access the other files in the same directory. You say you don't think it is working but have provided no details as to why you believe it is not working. Give an example of a default file name and the other files in the same directory that should match. I tested the logic above so I know it works. Using a folder with the following files: convert.file_size.php convert.images.php convert.php create mp3 playlist.php I used the following code: $path = 'file:temp/convert.php'; $filename = substr($path, 5); $basename = substr($filename, 0, strrpos($filename, '.')); $ext = strrchr($filename, '.'); $allfiles = glob("{$basename}.*.php"); And get the following output: Array ( [0] => temp/convert.file_size.php [1] => temp/convert.images.php ) -
NEED HELP w displaying multiple files with similar filenames!
Psycho replied to prototype18's topic in PHP Coding Help
It appears that the file name is part of a string, specifically: file_exists(substr($path, 5) By the way, instead of using that same function multiple times, just assign that to a variable so the PHP parser doesn't need to run that function multiple times. Not knowing everything about your particular need, this is just a shot in the dark: //Check display value and print row if($display) { //Check if file exists if(substr($path, 0, 5) == "file:") { $filename = substr($path, 5); if(file_exists($filename)) { $basename = substr($filename, 0, strrpos($filename, '.')); $ext = strtolower(substr(strrchr($filename, '.'))); $files = glob("{$basename}.*.pdf"); foreach($files as $file) { if($ext == ".pdf") { $column4 = "{$file} <a href=\"pdf.php?src={$file}\">VIEW</a><br />\n"; } if($ext == ".jpg") { $column4 = "{$file} <a href=\"jpg.php?src={$file}\">VIEW</a><br />\n"; } } } else { $column4 = "Not Available"; } } } -
As I already stated. In the form 1 script all you are doing is creating variables which are not used on that page. As soon as that script completes execution those variables are lost. You should create hidden fields in form 1 with those values so they will be sent to form 2.
-
NEED HELP w displaying multiple files with similar filenames!
Psycho replied to prototype18's topic in PHP Coding Help
Assuming all the files will be pdf's and are in the same directory, here is all you need. $files = glob(substr($basefile, 0, -4)."*.pdf"); $files will be an array of all the files that match the $basefile with the same name in the format you specified above. NOTE: That example is case sensitive. So, if the primary name of the file has a different case than the other files or if some files use "pdf" while others use "PDF" you will not get all the matches. You can check the manual for glob() in the "User Contributed Notes" to see examples on how to make the matching case-insensitive. http://us3.php.net/manual/en/function.glob.php -
Can you explain the purpose of this array? Creating an array of all the days in the years seems wholly unnecessay given all the avaialble data/time features built into PHP where you can get what you need dynamically.
-
Perhaps you could explain the actual problem you are having? It looks like the first form is receiving data via POST and you are using it to create variables - but you aren't doing anything with the data. But, there is a form created on that page. I *guess* that the form on the first page is POSTING to form 2. Form 2 is apparently checking for GET data, but no GET values were defined/set in the previous form. Try taking the values in FORM 1 and setting them as hidden fields as you did in form 2. Then those values will be available on form 2 from the POST values.
-
How to create category of output like chapters
Psycho replied to jeshrian's topic in PHP Coding Help
I'm not going to go through and rewrite all of your code for you, if that is what you are needing. You should be able to do that yourself or you should be posting in the freelance forum. But, I will provide some guidance on a *possible* solution. I say possible because I really don't understand what your project is so I am basing my comments on a general solution. I am a little confused by the code above. The first code block is saving data to the table 'tbl_coursecategory', and the second is pulling data from a table called 'tbl_lecture', so I have no idea what the two scripts have to do with each other. Ok, assuming you want content associated with a chapter/category, if you simply need chapters/categories listed as numerical entities (i.e. 1, 2, 3,...) AND you won't need to reorder the chapters/categories, you could simply add a field to the content table to specify the chapter/category. You can then pull the content from the table using an ORDER BY on that field. However, if you need the chapters/categories to have descriptive names and/or you may need to reorder them, you could create another table (e.g. chapters). That table could have fields such as chpt_id, chpt_name and chpt_order. The ID would just be a unique, auto-increment field, the name is obvious, and the order field is to specify the order in which the chapters are displayed. Then in your content table you would add a new field for a foreign key to specify the chapter ID. Then when you pull the records you would do a JOIN from the content table to the chapter table so you can order the content records by the chapter. Rough example: SELECT *FROM content_tableJOIN chapters ON content_table.chpt_id = chapters.chpt_idORDER BY chapters.chpt_order ASC -
My first javascript function (pllease don't laugh ;)
Psycho replied to fortnox007's topic in Javascript Help
There's one other error Should be -
The problem was you didn't give an example of what you wanted the output to be. The above explains the changes for src. But, what is this supposed to mean: alt="name of site insted of default" Where does the name of the site come from??? Also, you stated you wanted the images to have a width attribute. But in your example above you ahve removed the style attributes and appended (incorrectly) a "?w=400". So, is the 400 supposed to be appended to the URL (correctly with an ampersand) or do you need the attribute set in the img tag? The code below will replace the source as specified above (without the w=400). //Replace src attribute$text = preg_replace("#(<img[^>]+src=[\"|'])([^\"|']+)#", '${1}http://www.mysite.com/phpthumb.php?${2}', $text);//Remove style attribute (if exist)$text = preg_replace("#(<img[^>]+?)(style=[\"|'][^\"|']+[\"|'])#", '${1}', $text);//Add custom style attribute$text = preg_replace("#(<img )([^>]+)#", '${1}style="width:400px;" ${2}', $text);
-
Hmm... I always thought that execution on the current page stopped when a header redirect was encountered. I always used exit() only for "completeness" of the code. I did some testing and setting a session variable after the redirect seems to work. But, any variable setting or output statements (e.g. echo) after a redirect have no effect on the output to the user. Good to know. Could do some interesting things with that.
-
Yeah, you're going to have to make a decision. Are you going to require the user to use specific patterns as you showed in your example? If so, you will need to build a list of those patters to compare against. Or you can just accept one pattern and ensure the form gives an example of the pattern that is required. However, I think a better approach is to not require a pattern, but to just identify if the digits can be used to make up a phone number. Here are the rules I would follow (this assumes you require the area code): 1. Remove all non-digit characters from the input. 2. Is the remaining length 10 characters (it is valid) 3. Is the remaining length 11 characters and the first character is a '1' (it is valid) If the input is valid then I would format it to MY preferenced format. Example: $stripped_input = preg_replace('#[^\d]#', '', $input); if(strlen($stripped_input)==10 || (strlen($stripped_input)==11 && $stripped_input[0]=='1')) { $format = (strlen($stripped_input)==10) if (strlen($stripped_input)==10) ? : { $formatted_input = preg_replace('#(\d\d\d)(\d\d\d)(\d\d\d\d)#', '$1-$2-$3', $stripped_input); } else { $formatted_input = preg_replace('#(\d)(\d\d\d)(\d\d\d)(\d\d\d\d)#', '$1-$2-$3-$4', $stripped_input); } echo "Valid: {$formatted_input}"; } else { echo "Invalid: {$input}"; }
-
//Replace src attribute $text = preg_replace("#(<img[^>]+src=[\"|'])([^\"|']+)#", '${1}http://www.mysite.com/phpthumb.php', $text); //Remove style attribute (if exist) $text = preg_replace("#(<img[^>]+?)(style=[\"|'][^\"|']+[\"|'])#", '${1}', $text); //Add custom style attribute $text = preg_replace("#(<img )([^>]+)#", '${1}style="width:400px;" ${2}', $text);
-
The following will replace the src attribute in all image tags with "http://www.mysite.com/phpthumb.php". I think you actually want more than that, but you're going to have to do a better job of explaining/showing examples if you need more. $output = preg_replace("#(<img [^>]+src=\")([^\"]+)#", '${1}http://www.mysite.com/phpthumb.php', $input);
-
OK, I read this post twice and then took a look at the previous post. In the previous post the very first response stated: Looking at the post above, where exactly do you show the after of what you want? I have no idea how to interpret this statement:
-
Seriously, you can't find a PHP script that will allow you to resize images? Either 1) You didn't look or 2) you lack the basic abilities to find information that is easily available. http://lmgtfy.com/?q=php+resize+image