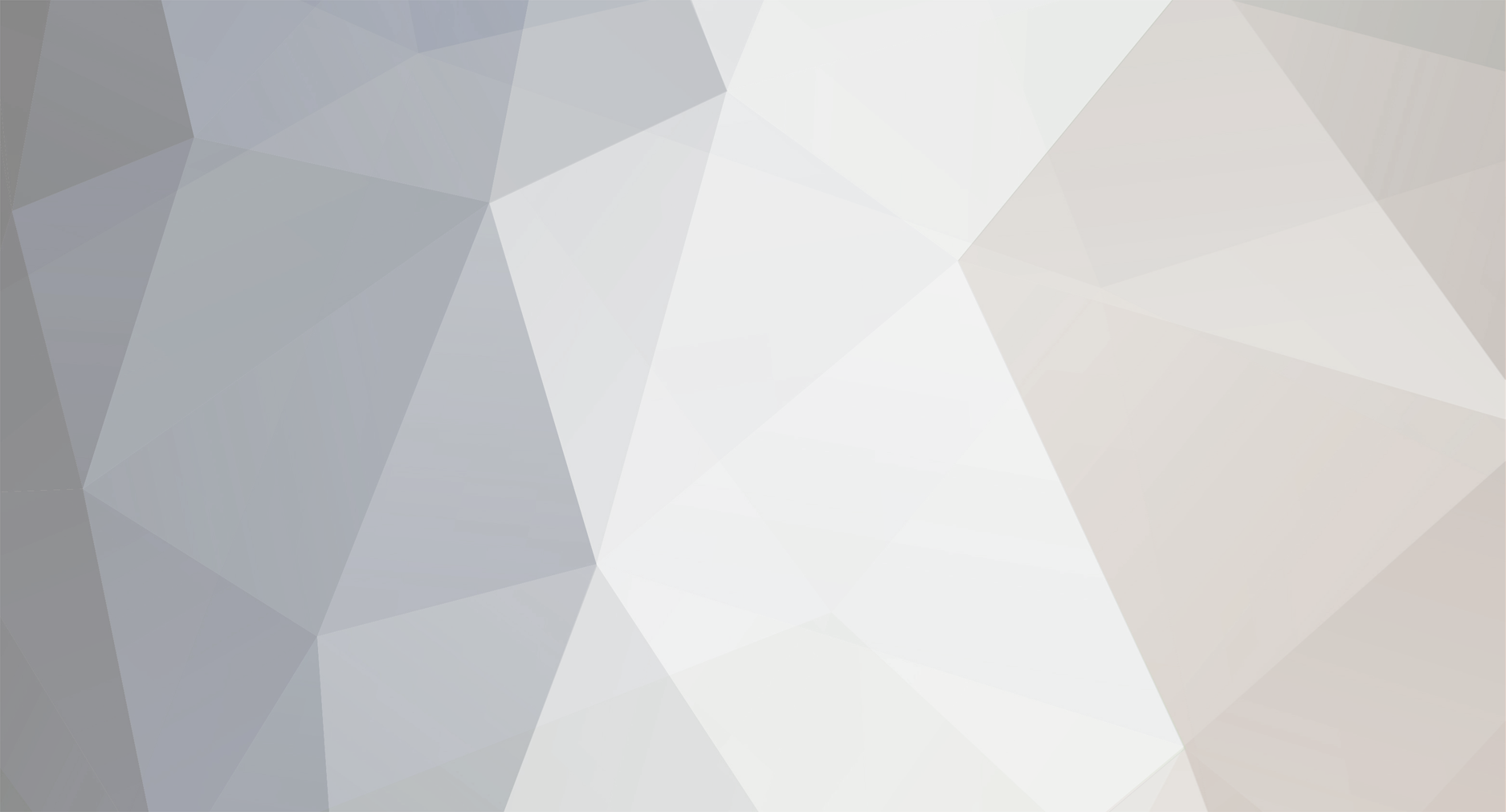
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Let me rephrase your question as it really doesn't make sense. You have one record int he database, but it is not displaying and you are wanting to know if you can remove the condition <?php if ($totalRows_rsStaff > 0) { // Show if recordset not empty ?> Yes you can, but it won't fix your problem. $totalRows_rsStaff is apparently not being set correctly. I'm not sure if you need that value or not. If so, you need to fix THAT problem. You also have another problem with your usage of a do..while loop. The do...while loop will execute at least one time, then it checks the condition in the while() statement to see if it should run again. The way you have it, the variables for staff name and staff id are not set the first time the block is executed so you would always have a blank row at the top. Here is a better implementation IMHO: <?php if (mysql_num_rows($rsStaff)>0) { // Show if recordset not empty ?> <table border="0" cellpadding="0" cellspacing="0" id="tblrepeat"> <tr> <th>Staff Name</th> <th>Remove</th> <th>Edit</th> </tr> <?php while ($row_rsStaff = mysql_fetch_assoc($rsStaff)) { ?> <tr> <td><?php echo $row_rsStaff['staffname']; ?></td> <td><a href="staff_delete.php?id=<?php echo $row_rsStaff['staffid']; ?>">Remove</a></td> <td><a href="staff_edit.php?id=<?php echo $row_rsStaff['staffid']; ?>">Edit</a></td> </tr> <?php } ?> </table> <br /> <?php } // Show if recordset not empty ?>
-
Multidimensional arrays, looping through them
Psycho replied to Pikachu2000's topic in PHP Coding Help
Yeah, I can't think of any good reasons for the previous for loop. At first I thought it would make sense if there were indexes that were non-numerical that you didn't want to process. but, if that was the case, then you wouldn't be using the count() as the total to iterrate through. The only other possibility is that you wanted a variable to use to use as a counter to possibly display a number next to each item. But, again, using foreach() would be a better option with using a variable that you increment on each loop. -
Multidimensional arrays, looping through them
Psycho replied to Pikachu2000's topic in PHP Coding Help
foreach($clients as $client) { print_r($client); } -
How to add multiple values in combobox from database in PHP?
Psycho replied to angel1987's topic in PHP Coding Help
Put this in the logic of your page: $office = //If there was a previously selected value set it here $query = "SELECT id, name FROM offices ORDER BY name"; $result = mysql_query($query); $officeOptions = ''; while($record = mysql_fetch_assoc($result)) { $selected = ($office==$record['id']) ? ' selected="selected"' : ''; $officeOptions .= "<option value=\"{$record['id']}\">{$record['name']}</option>\n"; } Then in the HTML/content you would do this <select name="office"> <?php echo $officeOptions; ?> </select> -
OK, the problem is in how you are constructing your pages. Not so much a logic problem. It is usually easier to build a framework for your page and then do all th elogic up front to determine the content for each section and THEN output the html. Here is a simple example using the above code: <?php //Set the selected category $selected_cat = (isset($_GET["cat_id"])) ? $_GET["cat_id"] : false; //show categories first $get_cats_sql = "SELECT cat_id, cat_title FROM category ORDER BY cat_id"; $get_cats_res = mysqli_query($mysqli, $get_cats_sql) or die(mysqli_error($mysqli)); if (mysqli_num_rows($get_cats_res) < 1) { $categoryList = "<p><em>Sorry, no categories to browse.</em></p>"; } else { //Display the categories while ($cats = mysqli_fetch_array($get_cats_res)) { $categoryList .= "<a href=\"{$_SERVER['PHP_SELF']}?cat_id={$cats['cat_id']}\">{$cats['cat_title']} <br /></a>\n"; if ($cats['cat_id']==$selected_cat) { //get items $get_items_sql = "SELECT id, photo, title, price FROM product WHERE cat_id = '{$selected_cat}' ORDER BY date"; $get_items_res = mysqli_query($mysqli, $get_items_sql) or die(mysqli_error($mysqli)); if (mysqli_num_rows($get_items_res) < 1) { $content = "<p><em>Sorry, no items in this category.</em></p>\n"; } else { $content .= "<ul>\n"; while ($items = mysqli_fetch_array($get_items_res)) { $item_url = "items3.php?id={$items['id']}"; $item_title = stripslashes($items['title']); $item_price = $items['price']; $item_photo = $items['photo']; $content .= "<li>"; $content .= "<a href=\"{$item_url}\">{$item_title}</a> (\${$item_price})"; $content .= "</li>\n"; } $content .= "</ul>\n"; } } } } ?> <html> <body> <div id="categories" style="width:200px;float:left;"> <?php echo $categoryList; ?> </div> <div id="content" style="width:600px;"> <?php echo $content; ?> </div> </body> </html>
-
Yes, it is absolutely possible. HOW you do it will depend on exactly what you need to do and the complexity involved. For example you might be able to accomplish what you need by running a simple query in PHPMYADMIN. Or you might need to run a query for all the current records and run them through a PHP processing script first. Here is a very basic example: The table badges requires a single record associated with each user. The feild user_id is used in both tables to associate them. INSERT INTO badges (user_id, badge_value) VALUES (SELECT user_id, 'default' FROM users u LEFT JOIN badges b ON u.user_id = b.user_id WHERE b.user_id IS NULL ) That willinsert a new record with the user ID and a default value of "default" for every user that does not currently have an associated record in the badges table.
-
<?php $input = '<p class="team_intro"><img src="john_smith.jpg" /><span class="leadername">John Smith</span> - a great funny witty super guy. </p><p>Rest of the text blabh blablahvlvlvlvdfjerfh etc... </p>'; //Get the first paragraph and put into $match array var preg_match('#<p[^>]*>(.*?)</p>#', $input, $match); //Remove images from the result $first_para = preg_replace('#<img[^>]*>#', '', $match[1]); echo $first_para; //Output: <span class="leadername">John Smith</span> - a great funny witty super guy. ?>
-
There were some inefficiencies in that code. Try this <?php //Set the selected category $selected_cat = (isset($_GET["cat_id"])) ? $_GET["cat_id"] : false; //show categories first $get_cats_sql = "SELECT cat_id, cat_title FROM category ORDER BY cat_id"; $get_cats_res = mysqli_query($mysqli, $get_cats_sql) or die(mysqli_error($mysqli)); if (mysqli_num_rows($get_cats_res) < 1) { $display_block = "<p><em>Sorry, no categories to browse.</em></p>"; } else { //Display the categories while ($cats = mysqli_fetch_array($get_cats_res)) { $display_block .= "<a href=\"{$_SERVER['PHP_SELF']}?cat_id={$cats['cat_id']}\">{$cats['cat_title']} <br /></a>\n"; if ($cats['cat_id']==$selected_cat) { //get items $get_items_sql = "SELECT id, photo, title, price FROM product WHERE cat_id = '{$selected_cat}' ORDER BY date"; $get_items_res = mysqli_query($mysqli, $get_items_sql) or die(mysqli_error($mysqli)); if (mysqli_num_rows($get_items_res) < 1) { $display_block = "<p><em>Sorry, no items in this category.</em></p>\n"; } else { $display_block .= "<ul>\n"; while ($items = mysqli_fetch_array($get_items_res)) { $item_url = "items3.php?id={$items['id']}"; $item_title = stripslashes($items['title']); $item_price = $items['price']; $item_photo = $items['photo']; $display_block .= "<li>"; $display_block .= "<a href=\"{$item_url}\">{$item_title}</a> (\${$item_price})"; $display_block .= "</li>\n"; } $display_block .= "</ul>\n"; } } } } ?>
-
Have you actually checked the raw HTML data? You have some problems with the HTML and that may prevent the data from being displayed in the browser. Namely, the items are in an unordered list and when you generate the links there is no opening <LI> tag, but there is a closing </LI> tag.
-
I hate checkboxes; please help me process them.
Psycho replied to bluegray's topic in PHP Coding Help
That is just a notice which would not be displayed in a production environment since you should not have error reporting set to show such messages. But, it's good to resolve those types of issues nonetheless. Basically, the error is due to the fact that you are trying to reference a variable that does not exist. Since none of the checkboxes are checked. So, you simply need to utilize an isset() in an if statement to only run the code for processign the checkboxes if, well, it's set. if(isset($_POST['keys'])) { //Code to process the checkbox data } Or, instead of having to break out that functionality entirely, you can just add a single line to ensure the value is set if no checkboxes are checked. just set it to an empty array. if(!isset($_POST['keys'])) { $_POST['keys'] = array(); } //Code to process checkboxes continues here -
1. Did you look at the sample output? How were the color assignments NOT random? Did you even bother reading the code to understand what is happening? I added plenty of comments so that anyone analyzing the code could understand how the colors were being randomized. But, I guess I have to hold your hand. So, after creating an array of 1 to 100 in sequential order, the next step will shuffle() the array to put all the values in a random order - but the index of the values will be from 0 to 99. So, half of the random vaues are in indexes 0 to 49 and the other half of random values are in indexes 50 to 99. Using this line: color = ($index<50) ? 'W' : 'B'; The values in the first 50 indexes are given a color of "W", while the last 50 are given "B". BUt, they are in a random order, so the color is evenly distributed - randomly - across all the values. 2. It's not that hard, but I can see where you might have some difficulty. I could just as easily implement in the code I provided above, but decided to change the approach just for my personal interest. So, here is revised code with LOTS of comments. Be sure to look at the last line and either keep or remove that line depending upon whether you want to allow for duplicate results. I also added some debugging lines so you can see the gernerated array of possibilities. Lastly, the two variables at the top allow you to change how many possibilities and how many generated numbers are created without touching the rest of the code. <?php //Number of possible numbers $maxPossible = 100; //Number of generated attempts $maxAttempts = 10; //Create separate arrays for W and B (1/2 of $maxPossible each) $white = array_fill(1, ceil($maxPossible/2), 'W'); $black = array_fill(1, floor($maxPossible/2), 'B'); //Create combined array first half 'W' and 2nd 1/2 half 'B' $colors = array_merge($white, $black); //Randomize the array (i.e. indexes 0 to n are randomly assigned W or B) shuffle($colors); //Append the colors to an array value of 0 at the 0 index //indexes 1 to $maxPossible have randomly assinged W or B $possibilities = array_merge(array(0), $colors); //The followng lines are for illustrative purposes only //Remove after you see how the array is generated echo "<pre>"; print_r($possibilities); echo "</pre>"; ///////////////////// for($attempt=0; $attempt<$maxAttempts; $attempt++) { //Generate a random number $generatedNumber = array_rand($possibilities); //Determine the results if($generatedNumber==0) { //Value was 0 $result = '-> (value was zero)'; } else { //Value was not 0, determine color and box $color = $possibilities[$generatedNumber]; $box = ceil($generatedNumber/($maxPossible/4)); $result = "-> it displays {$color}{$generatedNumber} - Box{$box}"; } //Output the results echo "Generated number: {$generatedNumber} {$result}<br />\n"; //Remove generated number from possibilities (if you don't want to allow diplicates) unset($possibleNumbers[$index]); } ?>
-
Huh? You can determine that easier with PHP. Take a look at the variables accessible via the array $_SERVER in PHP. http://php.net/manual/en/reserved.variables.server.php I think you would want to use $_SERVER['PHP_SELF'], $_SERVER['QUERY_STRING'] or $_SERVER['REQUEST_URI']
-
@Zach, Did you even bother reading the post? If so, you would have seen that 1) the issue the OP had was already solved and the discussion was only continuing to explain a concept with regex patterns and 2) the pattern you supplied would NOT solve the OPs problem because it is "greedy".
-
Pretty simple I think: <?php //genrate array of number 1 to 100 $numbers = range(1, 100); //Randomize the array shuffle($numbers); //generate 10 random numbers and output the results for($rand=0; $rand<10; $rand++) { $index = array_rand($numbers); $number = $numbers[$index]; //Remove value from array to prevent duplicates unset($numbers[$index]); $color = ($index<50) ? 'W' : 'B'; $box = "Box" . ceil($number/25); echo "Generated number: {$number} -> it displays {$color}{$number} - {$box}<br />\n"; } ?> Example output: Generated number: 14 -> it displays W14 - Box1 Generated number: 4 -> it displays W4 - Box1 Generated number: 72 -> it displays B72 - Box3 Generated number: 73 -> it displays W73 - Box3 Generated number: 43 -> it displays W43 - Box2 Generated number: 20 -> it displays B20 - Box1 Generated number: 54 -> it displays W54 - Box3 Generated number: 99 -> it displays B99 - Box4 Generated number: 90 -> it displays W90 - Box4 Generated number: 88 -> it displays B88 - Box4
-
Converting date entered into html form to php timestamp
Psycho replied to bobby317's topic in PHP Coding Help
//Option 1 $timestamp = strtotime("{$_POST['year']}-{$_POST['month']}-{$_POST['day']}"); //Option 2 $timestamp = mktime(0, 0, 0, $_POST['month'], $_POST['day'], $_POST['year']); http://php.net/manual/en/function.strtotime.php http://us.php.net/manual/en/function.mktime.php -
$timestamp = '20100614062921'; echo date('F jS, Y h:i:s', strtotime($timestamp)); //Output: June 14th, 2010 06:29:21
-
You are not looking at the ENTIRE pattern. If this is your pattern !name=\"(.*?)\"! Then you are telling the system to find text that begins with name=", then has 0 or more characters, and then ends with ". You are thinking too much like a human because you are assuming that it shouldn't match no characters. But, that is a perfectly valid match. What if you wanted to know the value of EVERY "name" parameter - even the ones that are empty? If (.*?) didn't do that, what would.
-
I already told you what to look for. You need to verify the actual data in the database. Then validate the data that is returned from the query. And, you haven't verified what the HTML actually contains.
-
What does the actual HTML code look like? If the value of $theme doesn't EXACTLY equal '1' or '2' then it won't work. You should double check the values in the DB and the values returned from the query. Although you could also make it a little more elegant to always get a value even if ther eis a problem form the query results: <?php //connect to database mysql_connect("$mysql_host","$mysql_user","$mysql_password"); mysql_select_db("$mysql_database"); $query = "SELECT theme FROM themes WHERE ip='$ip'"; $result = mysql_query($query); $theme = result($result, 0); $link = ($theme=='2') ? "style2.css" : ""style.css; ?> <link rel="stylesheet" type="text/css" href="<?php echo $link; ?>" />
-
how do i get a variable stored on another page?
Psycho replied to Fenhopi's topic in PHP Coding Help
Um, no. That is the whole point of storing variables in sessions - so you can track user variables across the session for the user. When a new page request is made from the server the page doesn't have any knowledge of what pages were processed previously or the variables that were calculated in them. And, what is so difficult about using session_start() and calling the variable you want? -
Well, as you stated * matches 0 or more occurances. The plus symbol matches one or more occurances - so there must be at least one match of the pattern for a result to be returned. Does that make sense?
-
how do i get a variable stored on another page?
Psycho replied to Fenhopi's topic in PHP Coding Help
You are not making any sense. I'm guessing you have multiple pictures in the DB for each user? And you want to display the current user's picture to them? Then you will need to use either cookies or session variables after the user has logged in so you "know" who they are on subsequent page requests. -
Well, you will need to identify the user in some manner. If this is a system where the user logs in then you will want to store a record in the database that includes the user ID and last submission time. Then whenever a submission is made you will need to check that table to see if enough time has elapsed since the last submission time to allow the new submissions. If this is not a system with known users that log in (i.e. anyone can access the page) then you have some decisions to make. Because it all depends on how fool-proof this needs to be. You can use a cookie to store the last submission time, assuming the user has cookies enabled. This allows the submission to be tracked on a machine by machine basis. But, if you are worried about malicious user's they can disable cookies and/or delete them on each submission. If you don't want to use cookies, you could store a last submission in the database by IP address. BUt, that has drawbacks as well. Users can change/spoof their IP address and it will also cause problems for users that are behind a NAT and are using the same external IP address.
-
how do i get a variable stored on another page?
Psycho replied to Fenhopi's topic in PHP Coding Help
If the 2nd page is accessed directly after the first, such as with a link or a redirect. You can append the variable to the URL (e.g. somepage.php?foo=bar). But, if the pages are not accessed sequentially and/or the value needs to persist over time, then you need to save the value somewhere. The most common solution would be a database. But, if you don't have access to a database and your need is limited, you can simply write the value to a flat-file on your server. Then read it on the pages where you need to access it. Tutorial for file writing and reading: http://www.tizag.com/phpT/filewrite.php -
OK, I understand this problem very well, but find that wikipedia entry confusing. Quite simply, after you have processed the POST/GET data, simply redirect the user to a "success" page (e.g. Thank you for your submission") using "header('Location: success.php');". When the user is redirected to that page, all POST/GET data is lost. So, if the user refreshes the page they will just get a refresh of that page, not the processing page. I hope that didn't make it more confusing.