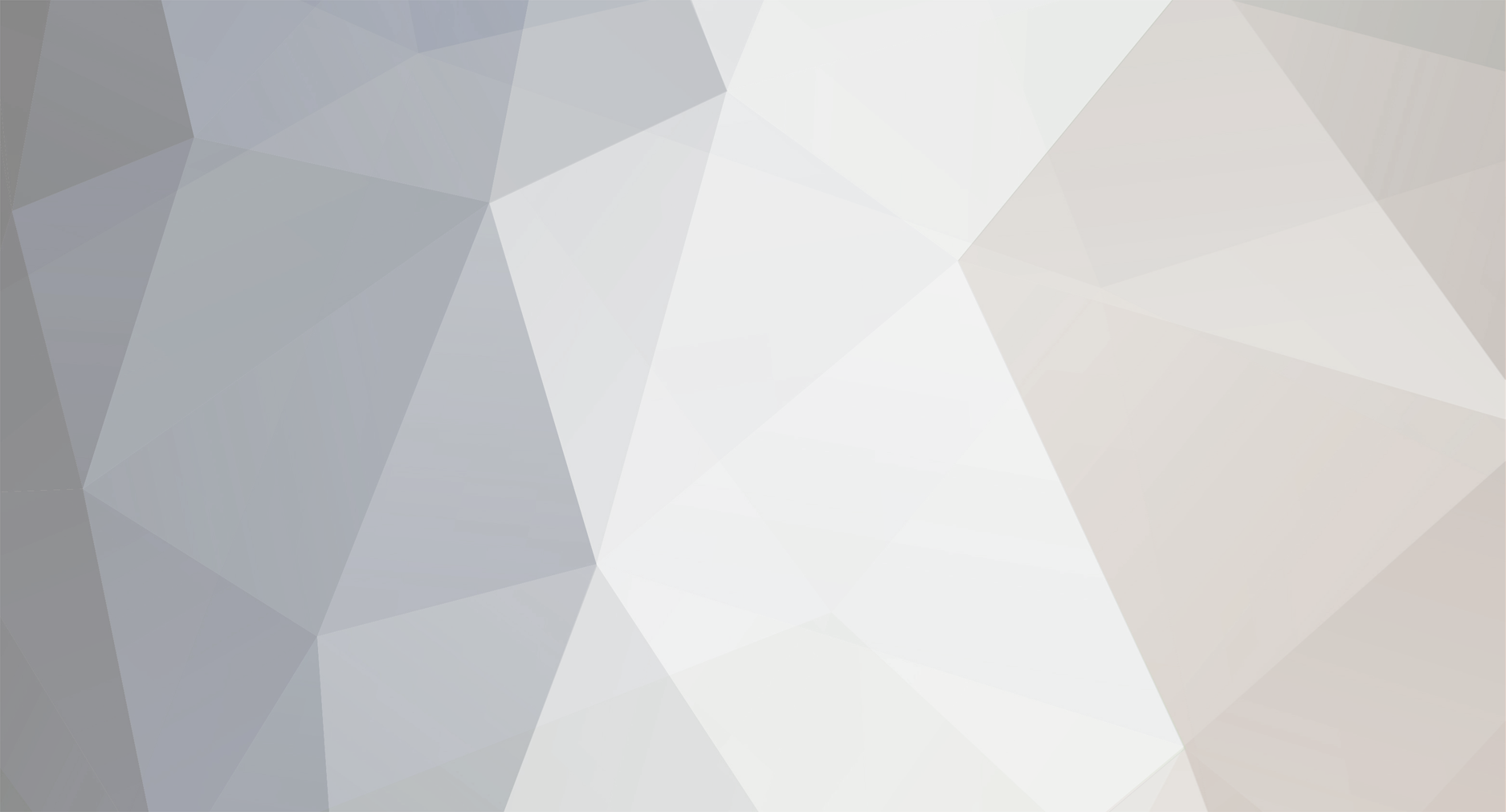
Psycho
Moderators-
Posts
12,159 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
What I understand of your request is that you are displaying an image that belongs to a specific category and when the user selects next you want the next image - in that category - to be displayed. I'm not going to wade through your code to try and understand it, but you would be better off having an explicit field for the sequence of the images instead of relying upon the image IDs. It would make this functionality much easier and the database format would be better off as well. Anyway, here are a couple of solutions: 1. Using your current design you could do two additional queries when displaying an image: one to get the prev photo id and one to get the next photo id. Example: $cid = mysql_real_escape_string($cid); $pid = mysql_real_escape_string($pid); //Query to get the next photo $query = "SELECT id FROM art_photos WHERE photo_category='{$cid}' AND id > '{$pid}' LIMIT 1"; //Query to get the prevphoto $query = "SELECT id FROM art_photos WHERE photo_category='{$cid}' AND id < '{$pid}' LIMIT 1"; You could then append the appropriate image IDs to the prev/next links 2. Alternatively, the next/prev link could simply pass two variables a) the current photo ID and b) either 'next' or 'prev'. Using those two variables you could first determine the category of the image that was viewed and then query for the next or prev image n that category. This would be a more efficient solution. Since you would not need to run the two queries identified in #1 each time an image is displayed. However, if you would not be able to determine if the prev/next links should be disabled in this process if the image being viewed is the first/last.
-
If these records have any importance I can't see how creating an automated process to print and then delete the records is, in any way, a good idea. The printer can't commnicate back to your script to verify if the record was actually printed or readable. Would it be feasible to create a script to create a printable versioin of all the currently unprinted records so a user can print those records - then verify that all the records were printed?
-
Did you TRY that code??? If you want it to be literally 1 image then you will need to use image manipulation code to create a physical 1 image. That code will create a grid with all the images in a 3 x 8 table and, yes, it will correctly use the default image when there are less than 24. Just set the table properties so there is no cellpadding or cellspacing and all the images will be "smashed" together to appear as a single image.
-
<?php $max_cols = 8; $max_rows = 3; $total_images = $max_cols * $max_rows; $query = "SELECT Avatar FROM users WHERE Activated = '1' ORDER BY RAND() LIMIT {$total_images}"; $result = mysql_query($query); if(!$result) { echo "Error retrieving the images."; } else { echo "<table>\n"; for($img_count=0; $img_count<$total_images; $img_count++) { if(($img_count%$max_cols)==0) { echo " <tr>\n"; } if($img = mysql_fetch_array($result)) { echo " <td><a href=\"#\"><img src=\"../resize_image.php?file={$img['Avatar']}&size=75\" title=\"\" border=\"0\" /></a></td>\n"; } else { echo " <td><img src=\"resize_image.php?file=profile/photos/default/default.jpg&size=75\" title=\"\" border=\"0\" /></td>\n"; } if(($img_count%$max_cols)==($max_cols-1)) { echo " </tr>\n"; } } echo "<table>\n"; } ?>
-
Why are you entering "<p>"? Is the purpose of this data to display code to the user? If so, just use <XMP> tags so you don't have to enter in the codes for those characters. Example: <b>Here is the code</b> <xmp> <b>Bold Text</b> <div>A Div</div> </xmp> Output: Here is the code <b>Bold Text</b> <div>A Div</div>
-
As he ^^ said Remove the lines I have commented here .alertBoxStyle { cursor: default; filter: alpha(opacity=90); background-color: #E4E4E4; position: absolute; top: 200px; left: 200px; width: 100px; /* height: 50px; */ visibility:hidden; z-index: 999; border-style: groove; border-width: 5px; border-color: #FFFFFF; text-align: center; } and here aWidth = (thisText * 5) + 80; //aHeight = 100; if (aWidth < 150){ aWidth = 200; } if (aWidth > 350){ aWidth = 350; } //if (thisText > 60){ aHeight = 110; } //if (thisText > 120){ aHeight = 130; } //if (thisText > 180){ aHeight = 150; } //if (thisText > 240){ aHeight = 170; } //if (thisText > 300){ aHeight = 190; } //if (thisText > 360){ aHeight = 210; } //if (thisText > 420){ aHeight = 230; } //if (thisText > 490){ aHeight = 250; } //if (thisText > 550){ aHeight = 270; } //if (thisText > 610){ aHeight = 290; } alertBox.width = aWidth; //alertBox.height = aHeight; alertBox.left = (document.body.clientWidth - aWidth)/2; alertBox.top = (document.body.clientHeight - aHeight)/2;
-
<?php //define the input file $file = "somefile.txt"; //Read the file into an array $line = file($file); //Process the file array into a multi-dimensional array $data = array(); foreach($lines as $line) { $data = explode("\t", $line); } //Function to sort the array by index 1 funtion sortTheArray($a, $b) { if($a[1]==$b[1]) { return 0; } return ($a[1] > $b[1]) ? 1 : -1; } //Sort the array usort($data, "sortTheArray"); //Create output //======================== //Detemine columns $columns = count($data[0]); //Open table echo "<table>\n"; //Column headers echo " <tr>\n"; for($col=0; $col<$columns; $col++) { echo " <th>Field {$col}</th>\n"; } echo " <tr>\n"; //output records foreach($data as $record) { echo " <tr>\n"; foreach($record as $field) { echo " <td>{$field}</td>\n"; } echo " <tr>\n"; } //Close table echo "</table>\n"; ?>
-
Finding INTERSECTION of time - Between 2 or more times! GOING NUTS!
Psycho replied to stangn99's topic in PHP Coding Help
As cags stated: You are storing the values as a time format and the query is then comparing a time to a datetimestamp. I would suggest using datetimestamps for the start/end times in the database. Then you do not need the date field for the record since you can ascertain that from the start/end values. -
Finding INTERSECTION of time - Between 2 or more times! GOING NUTS!
Psycho replied to stangn99's topic in PHP Coding Help
Echo the query to the page and echo the results of the query to the page. Then analyze the data. -
Finding INTERSECTION of time - Between 2 or more times! GOING NUTS!
Psycho replied to stangn99's topic in PHP Coding Help
Here is the correct logic SELECT * FROM bookingdata WHERE meetingroom = '$meetingroom' AND bookTimeFrom < '$to' AND bookTimeTo > '$from' It first checks if the any existing meeting has a start time that is before the new meeting's end time (if they start after an existing meeting ends there is no conflict). Then IF an existing meeting does start before the new meeting it checks if that existing meeting ends after the new meetings begin time. -
Finding INTERSECTION of time - Between 2 or more times! GOING NUTS!
Psycho replied to stangn99's topic in PHP Coding Help
Yeah, that won't work. Example: Existing entry of 8:00AM to 11:00AM New Entry: 9:00AM and 10:00AM. Neither of the existing begin/end times are between the new begin/end times. I recall this same type of question being asked a long time ago on these forums. I'll see if I can dig it up. -
You should store dates as, well, dates. If your program needs to display dates in a specific format then you format it after you extract it from the database. You are creating a problem where there shouldn't be one to begin with.
-
YOU need to check for that error condition BEFORE you do the insert. $query = "SELECT username FROM table WHERE username='$username'"; $result = mysql_query($query); if(mysql_num_rows($result)>0) { echo "The username '{$username}' is already in use."; } else { $query = "INSERT INTO table (username) VALUES ('{$username}')"; $result = mysql_query($query); }
-
The parameters for the password make the possibilities much more limited than it should be. I would suggest making the length variable and adding upper case letters and special characters to the possible combinations. Also, most password generators will ensure that there is a mix of different character type (1 lower and/or upper case, 1 number, 1 special). Here is what I would do to create a random length and add more variablility in the characters. I see you left out some characters (i.e. 1(one) and l (lowercase L)) as is common. So, I also left out "O" (upper case Oh). mt_srand((double)microtime() * 1000000); //Variable length $length = mt_rand(7,11); //Available characters $characters = 'abcdefghjkmnpqrstwxyzABCDEFGHIJKLMNPQRSTUVWXYZ234 56789!@$%^&+'; $password = ''; for($i=0; $i<$length; $i++) { $password .= substr($characters, mt_rand(0, strlen($characters)-1, 1); }
-
Inserting multiple form entries into one table column
Psycho replied to bobicles2's topic in PHP Coding Help
Good catch, but not quite. The query results are being assigned to $result, not $error. So it should be if(!$result) As far as I know there's no law against kissing minors. -
The function I provided is only used to determine the correct language to display to the user. I included the list of parameters and what they are for. Plus, the comments should be enough to understand what it does. Below is the sample code I have as an example of how to use it. But, again, it only determines which language to show to the user. It is then up to you to decide on the functionality you will use to disply the appropriate content based upon the language selected. You will need some functionaliy on the site for the user to select/chnage their language. This should pass a GET or POST var whcih is used by the function. <?php //Example usage // //Create list of available languages $languages = array ('Spanish'=>'sp', 'French'=>'fr', 'English'=>'en'); //Get the language to show the user $currentLanguage = getLanguage($languages, $_GET['lang'], 'en'); //Create vars for illustrative purposes $sessionLang = (isset($_SESSION['language'])) ? $_SESSION['language'] : 'Not set' ; $cookieLang = (isset($_COOKIE['language'])) ? $_COOKIE['language'] : 'Not set' ; ?> <html> <body> Current language: <?php echo array_search($currentLanguage, $languages) . " ($currentLanguage)"; ?> <br /><br /> $_GET['lang']: <?php echo $_GET['lang']; ?><br /> Session language: <?php echo $sessionLang; ?><br /> Cookie language: <?php echo $cookieLang; ?><br /><br /> Change Language <?php foreach ($languages as $name => $value) { echo "<a href=\"?lang={$value}\">{$name}</a> "; } ?> </body> </html>
-
Here's my two cents: From your description I am thinking this is a primarily static site that you want as multi-language. Go ahead and use "designer" mode or whatever you are comfortable with to create the pages initially. Then, replace each phrase word with a snippet of PHP with a single echo statement. That way the file is still in a human "readable" format. Example: ABC Company<br /> <p><?php echo $companyDescription; ?></p> <br /> <p><?php echo $contactLabel; ?><br /> <?php echo $phoneLable; ?>: 1-800-123-4567<br /> <?php echo $AddressLable; ?>: 123 Main St., Anywhere, ST 12345<br /> </p> You will then need a script to determine the user's language and you could call it with an include at the top of every page. Here is a script I have used before: // Function: getLanguage($languageList [, $selectedLang] [, $defaultLang]) // // Parameters: // - $languageList: (Required) An array of all available languages for the user // - $selectedLang: (Optional) The language the user has selected (GET or POST) // - $defaultLang: (Optional) the default language to use if unable to determine // user's language from seleted or saved values. function getLanguage($languageList, $selectedLang=null, $defaultLang=null) { //Set the default value (or first option in $languageList) $userLanguage = (!in_array($defaultLang, $languageList)) ? $languageList[0] : $defaultLang; //Detemine selected/saved user language if (!is_null($selectedLang) && in_array($selectedLang, $languageList)) { //Request was made to change the language $userLanguage = $selectedLang; setcookie('language', $userLanguage, time()+3600*24*365); //Expires in 1 year $_SESSION['language'] = $userLanguage; } else if (isset($_SESSION['language']) && in_array($_SESSION['language'], $languageList)) { //There is a saved language value in the SESSION data $userLanguage = $_SESSION['language']; } else if (isset($_COOKIE['language']) && in_array($_COOKIE['language'], $languageListAry)) { //There is a saved language value in the COOKIE data $userLanguage = $_COOKIE['language']; $_SESSION['language'] = $userLanguage; } //return the user's language return $userLanguage; } Once the user's langauge has been determined you can get the appropriate content to create the variables to populate the page. As Permiso stated thsi can be done from a database or a flat-file
-
Here is the JavaScript version: function validEmail(emailStr) { //Return true/false for valid/invalid email formatTest = /^[\w!#$%&\'*+\-\/=?^`{|}~]+(\.[\w!#$%&\'*+\-\/=?^`{|}~]+)*@[a-z\d]([a-z\d-]{0,62}[a-z\d])?(\.[a-z\d]([a-z\d-]{0,62}[a-z\d])?)*\.[a-z]{2,6}$/i lengthTest = /^(.{1,64})@(.{4,255})$/ return (formatTest.test(emailStr) && lengthTest.test(emailStr)); }
-
Here is a simple three line function I have been improving upon for some time which captures just about every validation of an email format that I can find: function is_email($email) { $formatTest = '/^[\w!#$%&\'*+\-\/=?^`{|}~]+(\.[\w!#$%&\'*+\-\/=?^`{|}~]+)*@[a-z\d]([a-z\d-]{0,62}[a-z\d])?(\.[a-z\d]([a-z\d-]{0,62}[a-z\d])?)*\.[a-z]{2,6}$/i'; $lengthTest = '/^(.{1,64})@(.{4,255})$/'; return (preg_match($formatTest, $email) && preg_match($lengthTest, $email)); } // NOTES: // // Format test // - Username: // - Can contain the following characters: // - Uppercase and lowercase English letters (a-z, A-Z) // - Digits 0 to 9 // - Characters _ ! # $ % & ' * + - / = ? ^ ` { | } ~ // - May contain '.' (periods), but cannot begin or end with a period // and they may not appear in succession (i.e. 2 or more in a row) // - Must be between 1 and 64 characters // - Domain: // - Can contain the following characters: 'a-z', 'A-Z', '0-9', '-' (hyphen), and '.' (period). // - There may be subdomains, separated by a period (.), but the combined domain may not // begin with a period and they not appear in succession (i.e. 2 or more in a row) // - Domain/Subdomain name parts may not begin or end with a hyphen // - Domain/Subdomain name parts must be between 1-64 characters // - TLD accepts: 'a-z' & 'A-Z' // // Note: the domain and tld parts must be between 4 and 256 characters total // // Length test // - Username: 1 to 64 characters // - Domain: 4 to 255 character //===================================================== // Function: is_email ( string $email ) // // Description: Finds whether the given string variable // is a properly formatted email. // // Parameters: $email the string being evaluated // // Return Values: Returns TRUE if $email is valid email // format, FALSE otherwise. //===================================================== Note: this does not allow for quoted string format email addresses (which allows pretty much ANY character in the username) but I have never seen one in real life. If anyone can find an error or flaw in this, please let me know.
-
Inserting multiple form entries into one table column
Psycho replied to bobicles2's topic in PHP Coding Help
Unless you are a girl, no thanks. A "thank you" will suffice. What is the error? -
Inserting multiple form entries into one table column
Psycho replied to bobicles2's topic in PHP Coding Help
Sorry, put the db connection info before you use that. <?php $con = mysql_connect("localhost","teamrend_rwowen","291Aug89"); if (!$con) { die('Could not connect: ' . mysql_error()); } mysql_select_db("teamrend_rwowen", $con); $date = (int) $_POST['Year'] . '-' . (int) $_POST['Month'] . '-' . (int) $_POST['Day']; $event = mysql_real_escape_string($_POST['Event']); $genre = mysql_real_escape_string($_POST['Genre']); $price = mysql_real_escape_string($_POST['Price']); $venue = mysql_real_escape_string($_POST['Venue']); $tickets = mysql_real_escape_string($_POST['Tickets']); $sql="INSERT INTO Events (Event, Genre, Date, Price, Venue, Tickets) VALUES ('$event','$genre','$date','$price','$venue','$tickets')"; $result = mysql_query($sql, $con); if ($result) { echo "Error :" . mysql_error(); } else { echo "Thank You, Your Event has now been added to our Records"; } mysql_close($con); ?> -
No. Those are the parameters that "most" email servers would allow. Technically, I think any character is allowed in the username portion of the email address - even a space. But, many/most email servers are not configured to support it. Yes, it is an elaborate validation, but many other validation scripts create false positives (flagging email addresses as invalid when they are valid). The perfect example is the plus sign. That is a perfectly legitimate character for the username, however many validation scripts do not allow it. That is unfortunate since gmail has a fantastic feature the utilizes that character. Example: if I have the gmail address of username@gmail.com, then I can also use username+freaks@gmail.com and it will still go to the same mailbox. Basically you can add any characters after the plus sign up to a certain number. This is a great feature because you can set up a different email address when you sign up on different sites. You can then use it to categorize yur email and identify what sites are reselling your email address.
-
Wait, I posted my JavaScript validation (because this is the JS forum), but I see you are doing the validation in PHP. So, not sure what you are really wanting, but here is the same funciton in a PHP version: function is_email($email) { $formatTest = '/^[\w!#$%&\'*+\-\/=?^`{|}~]+(\.[\w!#$%&\'*+\-\/=?^`{|}~]+)*@[a-z\d]([a-z\d-]*[a-z\d])?(\.[a-z\d]([a-z\d-]*[a-z\d])?)*\.[a-z]{2,6}$/i'; $lengthTest = '/^(.{1,64})@(.{4,255})$/'; return (preg_match($formatTest, $email) && preg_match($lengthTest, $email)); }
-
You have way more logic than needed to validate the email format. Here is a function with just three lines of code that does all of what you have in more than a dozen lines: function validEmail(emailStr) { //Return true/false for valid/invalid email formatTest = /^[\w!#$%&\'*+\-\/=?^`{|}~]+(\.[\w!#$%&\'*+\-\/=?^`{|}~]+)*@[a-z\d]([a-z\d-]*[a-z\d])?(\.[a-z\d]([a-z\d-]*[a-z\d])?)*\.[a-z]{2,6}$/i lengthTest = /^(.{1,64})@(.{4,255})$/ return (formatTest.test(emailStr) && lengthTest.test(emailStr)); } That function will validate that the email meets the following criteria: The username: [*]Can contain the following characters: Uppercase and lowercase English letters (a-z, A-Z) Digits 0 to 9 Characters: _ ! # $ % & ' * + - / = ? ^ ` { | } ~ [*]May contain '.' (periods), but cannot begin or end with a period and they may not appear in succession (i.e. 2 or more in a row) [*]Must be between 1 and 64 characters The domain name: [*]Can contain the following characters: 'a-z', 'A-Z', '0-9', and '-' (hyphen). [*]There may be subdomains, separated by a period (.), but the combined domain may not begin with a period and they not appear in succession (i.e. 2 or more in a row) [*]Hostname parts may not begin or end with a hyphen [*]The 'combined' domain name must be followed by a period and the TLD (top level domain). The TLD (Top Level Domain): [*]Can contain the following characters: 'a-z', and 'A-Z'. [*]The TLD must consist of 2-6 alpha characters in either upper or lower case. (6 characters are needed to support .museum). Note: the domain and tld parts must be between 4 and 256 characters total
-
Inserting multiple form entries into one table column
Psycho replied to bobicles2's topic in PHP Coding Help
You are really asking for someone to corrupt your database. You must always validate/cleanse data from the user before using it in a query. Your error is a PHP error and has nothing to do with the query. However, what is interesting is that the error message states the problem is on line 23 and there are not 23 lines in the code you posted for the page. But, I assume the problem is you don't have a semi-colon at the end of the last line. Try this, which is more secure: <?php $date = (int) $_POST['Year'] . '-' . (int) $_POST['Month'] . '-' . (int) $_POST['Day']; $event = mysql_real_escape_string($_POST['Event']); $genre = mysql_real_escape_string($_POST['Genre']); $price = mysql_real_escape_string($_POST['Price']); $venue = mysql_real_escape_string($_POST['Venue']); $tickets = mysql_real_escape_string($_POST['Tickets']); $con = mysql_connect("localhost","teamrend_rwowen","291Aug89"); if (!$con) { die('Could not connect: ' . mysql_error()); } mysql_select_db("teamrend_rwowen", $con); $sql="INSERT INTO Events (Event, Genre, Date, Price, Venue, Tickets) VALUES ('$event','$genre','$date','$price','$venue','$tickets')"; $result = mysql_query($sql, $con); if ($result) { echo "Error :" . mysql_error(); } else { echo "Thank You, Your Event has now been added to our Records"; } mysql_close($con); ?>