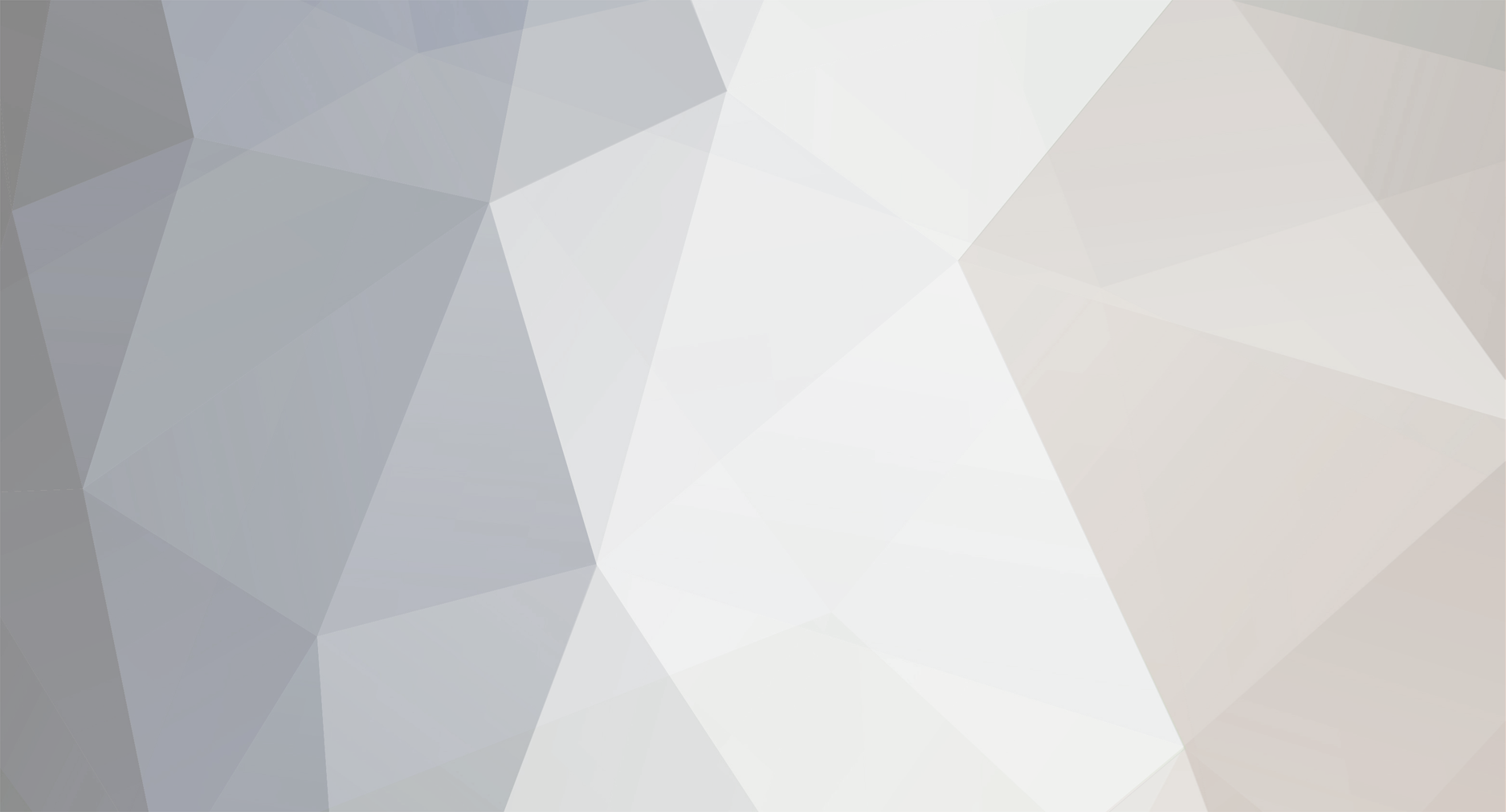
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
$datalist = mysql_fetch_array($select); $num=mysql_num_rows($select); //$datalist = htmlspecialchars($datalist); foreach($datalist as $data){ if (intval($data) >= 80) { echo "<td>$grade[0]</td>"; $data is an ARRAY. You can't get the integer of an arryay. Although there is a lot more wrong with that code.
-
Correct usage (int) $someVar; Example: $foo = 1.23; $bar = (int) $foo; echo $bar; //Output 1
-
You can't populate different colored text into a form field. It all has to be the same color. You will need to use a DIV (or something comparable). I didn't even look at your function as it wasn't making sense. Here are a couple of functions that takes two words (the target and the guess) and returns a string that is formatted to display in the format you asked. you can figure out how to implement it. <html> <head> <script type="text/javascript"> function compareGuess(targetID, guessID) { var target = document.getElementById(targetID).value; var guess = document.getElementById(guessID).value; document.getElementById('result').innerHTML = guessResult(target, guess); return; } function guessResult(target, guess) { var letterLen = Math.min(target.length, guess.length); var guessAry = guess.split(''); var targetAry = target.split(''); //Find all letter/place matches for(var i=0; i<letterLen; i++) { if(guessAry[i].toLowerCase()==targetAry[i].toLowerCase()) { guessAry[i] = '<span style="color:green;">' + guessAry[i] + '</span>'; targetAry[i] = ''; } } //Letters left in target that were not exact matches var remainder = targetAry.join('').toLowerCase(); //Find remaining letter only matches for(var i=0; i<guess.length; i++) { if(guessAry[i].length==1 && remainder.indexOf(guessAry[i].toLowerCase())!=-1) { position = remainder.indexOf(guessAry[i]); guessAry[i] = '<span style="color:blue;">' + guessAry[i] + '</span>'; remainder = remainder.substr(0, position) + remainder.substr(position+1); } } return guessAry.join(''); } </script> </head> <body> Target Word: <input type="text" id="target" /><br /> Guess: <input type="text" id="guess" /><br /> <button onclick="compareGuess('target', 'guess');" />Test</button><br /> <div id="result" style="letter-spacing:6px"></div><br /> </body> </html> EDIT: fixed one bug for handling case insensitive handling.
-
Your regular expression is looking for "quote", not "QUOTE". You need to make it case insensitive. Also, using "(.*)" is not efficient. Also, since you also need to replace the ending tag you should just do a search/replace for the name and message. Try this $message = preg_replace('/^\[quote=([^\]]*)\]([^\[]*)\[\/quote\]/i', "<div class='nm'>$1</div>\n$2", $message);
-
display first letter of string followed by _ _ _
Psycho replied to husslela03's topic in Javascript Help
Damn, salathe beat me to it. Ah well, here is a working example: <html> <head> <script type="text/javascript"> function displayPattern(theWord) { var pattern = theWord.replace(/(?!^)\w/g, "_") document.getElementById('pattern').innerHTML = pattern; return; } </script> </head> <body> Select a phrase:<br /> <select onchange="displayPattern(this.options[this.selectedIndex].value)"> <option value="">-- Select One --</option> <option value="The First Option">The First Option</option> <option value="Another Option">Another Option</option> <option value="Last Option">Last Option</option> </select> <br /><br /><br /> Pattern:<br /> <div id="pattern" style="letter-spacing:6px"></div> </body> </html> -
Here is a function I have used previously to "fit" an image to a fixed size. It will size the image to fit the available space in both dimensions and the dimension with excess information is cropped. Should work for you. //This function resizes and crops an image to completely FIT into a specified size function fitImageToSize($filename, $width_new, $height_new) { $src_x = 0; $src_y = 0; //Get dimensions of original image list($width_old, $height_old) = getimagesize($filename); //Resize & crop image based on smallest ratio if ( ($width_old/$height_old) < ($width_new/$height_new) ) { //Determine Resize ratio on width $ratio = $width_new / $width_old; //Detemine cropping dimensions for height $crop = $height_old - ($height_new/$ratio) ; $height_old = $height_old - $crop; $src_y = floor($crop/2); } else { //Detemine Resize ratio on height $ratio = $height_new / $height_old; //Detemine cropping dimensions for width $crop = $width_old - ($width_new/$ratio); $width_old = $width_old - $crop; $src_x = floor($crop/2); } $image_old = imagecreatefromjpeg($filename); $image_new = imagecreatetruecolor($width_new, $height_new); imagecopyresampled($image_new, $image_old, 0, 0, $src_x, $src_y, $width_new, $height_new, $width_old, $height_old); return $image_new; }
-
I don't see what the purpose is. If the user selects a value in the select list - use that value. I see no need to populate a text field. But, maybe there is some reason you are not sharing. A few problems. 1. "var input_user = document.getElementById('input');", the id of the text field is "sample" not "input" 2. "input_user.src", field objects do not have a src attribute. You want to set the "value" 3. input_user.src = ' + membername + '. If that worked, it would set the value to literally ' + membername + '. The quote marks tell the JS parser that it is a literal string. 4. You pass the select field OBJECT to the function and then attempt to set the value of the text field to that object. You need to get the VALUE of the select object In the code below, the function is modified as well as the onchange trigger event in the select field. <html> <head> <script type="text/javascript"> function changeEditMember(selObj, textID) { document.getElementById(textID).value = selObj.options[selObj.selectedIndex].value; return; } </script> </head> <body> <select style="width: 200px; vertical-align: top; height: 143px;" id="background" name="members" size="7" onchange="changeEditMember(this, 'sample')"> <option value="option1">option1</option> <option value="option2">option2</option> <option value="option3">option3</option> </select> <br /> Text field: <input type="text" id="sample"><br /> </body> </html>
-
OK, I figured out what I was missing. I was joining the two tables and only associating the records where the two values were the same, but the query needs to have an exclusion so records aren't joined on themsleves. That would be done by stating the ID cannot be the same. So this query should do what you need: SELECT d1.* FROM db_main d1 JOIN db_main d2 WHERE d1.color = d2.color AND d1.name = d2.name AND d1.id <> d2.id That will return every record where there is another record with the same color and name. EDIT: My appologies, I totally misread what you were asking for. I thought you were looking for existing duplicates already in the DB, not if a new record would be a duplicate.
-
What you need to do is JOIN the table on itself and compare each record to the others. Here is what you want (as I understand your requirements) SELECT d1.* FROM db_main d1 JOIN db_main d2 WHERE d1.color = d2.color AND d1.name = d2.name EDIT: Wait, I think that might not be right. I'm on a call and will review once I can.
-
As I first stated, if you are only wanting to show the records where there is missing data, then you should build your query to only pull those records. How that is done will depend on what an "empty" field means. So, I did not provide any guidance on that. Or, if you want to be inefficient and just pull all the records and change the code above to not display those records: while ($record = mysql_fetch_assoc($result)) { $emptyFields = array(); foreach($record as $field => $value) { $value = trim($value); if(empty($value)) { $emptyFields[] = "<li>$field</li>"; } } if (count($emptyFields)>0) { echo "<b>{$record['company']}</b>\n"; echo "<ul>" . implode('', $emptyFields) . "<\ul>\n"; } }
-
Ah, I see. Change mysql_fetch_array() to mysql_fetch_assoc(). I always use mysql_fetch_assoc(). I forgot that mysql_fetch_array() gets two copies of every value, one with the index of the field name and one with a numeric index.
-
So, don't include that field in your db query. I assume you are using '*' instead of only specifying the fields you want/need. Or, if you still want to take the lazy method, then add an exception clause the the processing code. EDIT: Wait, that makes no sense. The code only displays field NAMES where there is no value. There's nothing in the code I provided that would display the ID - which is a field VALUE. You must have misimplemented the code - or you have a column name of '1' in your table.
-
What are the values of $vkey and $meta? I'd use this: $link_b = "<a href=\"view.php?vkey={$vkey}&title={$meta}\">{$meta}</a>";
-
If you are wanting to modify a URL that is not related to the URL the user is currently viewing (i.e. user entered value), then this will work: preg_replace("/www\./", '', $url); It has one flaw. If the url contains 'www.' anywhere in the url it will be removed. Ex: "subdomainwww.domain.com" would become "subdomaindomain.com". I did that so it would work for values such as "http://www.domain.com". But, I think the likelyhood of such an occurance would be very remote. However, if the values will always start from the subdomain (i.e. not 'http://') then this would be better: preg_replace("/^www\./", '', $url);
-
If you ONLY want to display records with missing information, then the first step is to create the db query such that it only returns records with missing data. How that query looks will depend upon whether the "missing" data is an empty string, a null value, contains only white-space characters, or a combination of the three. You mean column. dotMoe's example is a start, but it has a flaw. If the first field is empty it will only show that one as missing data. So, any other fields missing data would not display. This code will work without having to explicitly code for each field. Of course, it uses the db field names and not "friendly" names. But, that may be acceptable in your situation. while ($record = mysql_fetch_array($result)) { $emptyFields = array(); foreach($record as $field => $value) { $value = trim($value); if(empty($value)) { $emptyFields[] = "<li>$field</li>"; } } echo "<b>{$record['company']}</b>\n"; echo "<ul>" . implode('', $emptyFields) . "<\ul>\n"; }
-
Here is a very simple example: <?php //Only validate if form was submitted if(isset($_POST)) { //Form was submitted, validate data $errors = false; $fname = trim($_POST['fname']); $lname = trim($_POST['lname']); $phone = trim($_POST['phone']); //Validate data submitted if(empty($lname) || empty($phone)) { $errors = "Last name and phone are required."; } //Validation passed, include processing page if(!$errors) { //Form 1 validation failed, redisplay the form include('processForm.php'); exit(); } } ?> <html> <body> <div style="color:#ff0000;"> <?php echo $errors; ?> </div><br /> Please enter your details.<br /> <form name="test" method="POST"> First Name: <input type="text" name="fname" value="<?php echo $_POST['fname']; ?>" /><br /> <b>Last Name:</b> <input type="text" name="lname" value="<?php echo $_POST['lname']; ?>" /><br /> <b>Phone:</b> <input type="text" name="phone" value="<?php echo $_POST['phone']; ?>" /><br /> <button type="submit">Submit</button> </form> * Required fields in bold<br /> </form> </body> </html>
-
A better process, in my opinion, is to always have form scripts POST to themsleves. If validation fails, it is a trivial matter to redisplay the form with the values the user entered. If validation passes, then include() the page that actually processes the data and displays the confirmation page to the user.
-
sort and delete duplicates in a multidimensional array?
Psycho replied to jjfletch's topic in PHP Coding Help
I think usort() is a much better option here. array_multisort requires you to create separate arrays for each "field" in the multidimensional array. Seems like a lot of overhead to me. The following code works to do as you asked, but I'll leave it to you to make it work inside your class. The following assumes the array is in the variable $locations function sortLocations($a, $b) { if($a['zip']!=$b['zip']) { return ($a['zip']>$b['zip']) ? 1 : -1; } if($a['distance']!=$b['distance']) { return ($a['distance']>$b['distance']) ? 1 : -1; } return 0; } function dedupeLocations(&$locationAry) { $zipCodes = array(); foreach($locationAry as $index => $location) { if(!in_array($location['zip'], $zipCodes)) { $zipCodes[] = $location['zip']; } else { unset($locationAry[$index]); } } return; } usort($locations, 'sortLocations'); dedupeLocations($locations); -
No, that is not what you did. You didn't use the code I provided. In your original code you declared the query string INSIDE the mysql_query() function. In the code I provided I broke that up to where the query string is assigned to a variable and THEN that query string is run within the mysql_query() function. I always advise against defining the query string within the function as it makes debugging much more difficult - this post is a prime example of that. Follow the code I first provided so you can echo the query to the page.
-
http://us3.php.net/get_magic_quotes_gpc I already responded to that page. As stated, every single one of the links in the description show a warning that magic quotes should not be used.
-
The only problem with that is if one of the array values is itself an array. The first link I provided has an example of how to handle multidimensional arrays.
-
Probably should be, but if you click on any of the three links in the description for that function the first thing you will see is the same messages I posted above.
-
Care to provide a link. Here is just one direct quote from php.net regarding magic quotes: http://www.php.net/manual/en/security.magicquotes.whynot.php http://php.net/manual/en/security.magicquotes.disabling.php
-
Two things: 1. The semicolon cannot be inside the parameter value 2. The border property does not apply to a TR, only to a TABLE or TD
-
What does the code you posted have to do with your question? I see no form in that code - where is that code? When I typed in "form file upload" into Google, the second result was "PHP Tutorial - File Upload": http://www.tizag.com/phpT/fileupload.php Wait, a tutorial on doing file uploads with PHP, sounds interesting. Maybe you should give that a read. EDIT: I just completely rewrote this entire post. I had a lot of condescending and belittling statements. Although I think you may be worthy of such treatment I am trying to refrain from such commentary.