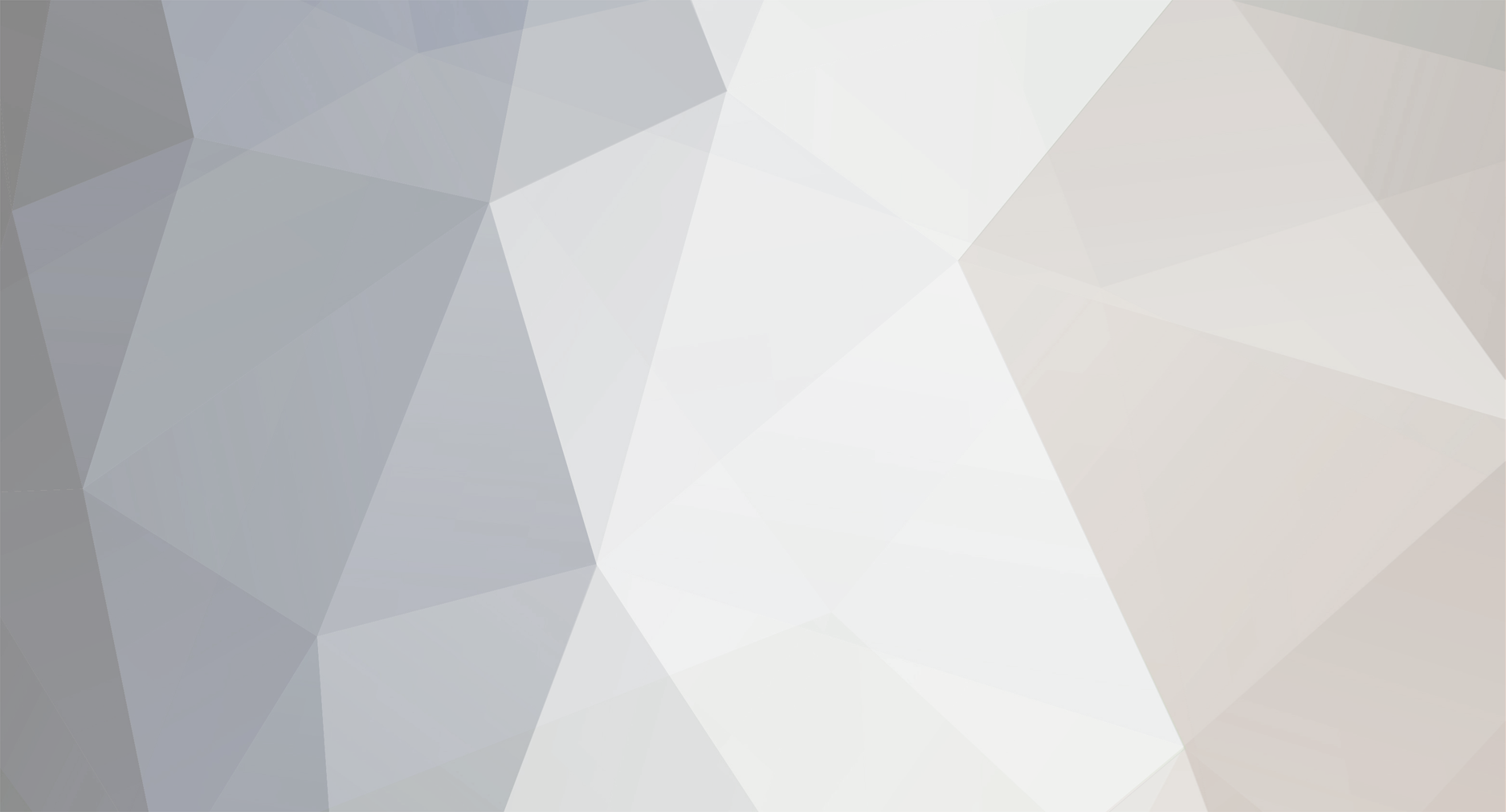
Psycho
Moderators-
Posts
12,159 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
Hmm... the style property for direction:rtl appeared to foul up the Next/Prev links. The double arrows had a different page number than the word. Very odd. I even checked the HTML output and it made no sense. I had to take it out. Anyway, that script is much, much more complex than it needs to be. I have rewritten it to be much more elegant. All the configuration variables are at the top. There is now one for direction. Change it from a 1 to a -1 to make it go from right to left. <?php //Configuration variables $tbl_name="class"; //your table name $targetpage = "result.php"; //your file name (the name of this file) $limit = 1; //how many items to show per page $adjacents = 2; // How many adjacent pages should be shown on each side? $beginend = 2; //Number of pages to always show at beginning & end $direction = -1; //Direction: 1 = forward, -1 = reverse $next_text = "Next »"; $prev_text = "« Prev"; //Default query $default_query = "SELECT [FIELD_LIST] as num FROM class WHERE $category_sql AND $type_sql AND $area_sql AND $rooms_sql AND $preco_between AND $day_sql"; //Get total number of records $query .= str_replace("[FIELD_LIST]", "COUNT(*) as num", $default_query); $result = mysql_query($query) $total_records = mysql_fetch_assoc($result); $total_records = $result['num']; // Determine total pages $total_pages = ceil($total_records/$limit); //total pages = total pages / items per page, rounded up. //Determine current page $page = (int) $_GET['page']; if ($page==0 || $page>$total_pages) { $page = 1; //if no page is given or > total pages, default to 1. } // Get data for current page $limit_start = ($page - 1) * $limit; //first item to display on this page $query .= str_replace("[FIELD_LIST]", "*", $default_query) . " LIMIT $limit_start, $limit"; $result = mysql_query($query); //Now we apply our rules and draw the pagination object. //We're actually saving the code to a variable in case we want to draw it more than once. function createPageLink($baseLink, $linkText, $pageNum=false) { if($pageNum) { $link = "<a href=\"$baseLink&page=$pageNum\">{$linkText}</a>\n"; } else { $link = "<span class=\"disabled\">{$linkText}</span>\n"; } return $link; } $pagination_link="{$targetpage}?category={$category}&type={$type}&area={$area}&rooms={$rooms}&preco1={$preco1}&preco2={$preco2}$day={$day}"; if($total_pages > 1) { //pages $loopStart = ($direction==1) ? 1 : $total_pages; for($counter=$loopStart; $counter>0 && $counter<=$total_pages; $counter=$counter+$direction) { //Add ellipses at beginning if needed if ( ($counter > $beginend) && ($counter < ($page-$adjacents)) ) { $page_links .= createPageLink('', "...\n", false); $counter = ($direction==1) ? ($page-$adjacents) : $beginend; } //Add ellipses at end if needed if ( ($counter > ($page+$adjacents)) && ($counter < ($total_pages-$beginend+1)) ) { $page_links .= createPageLink('', "...\n", false); $counter = ($direction==1) ? ($total_pages-$beginend+1) : ($page+$adjacents); } //Add page link $page_links .= createPageLink($pagination_link, $counter, (($counter!=$page)?$counter:false)); } //previous/next buttons $prev_bttn = createPageLink($pagination_link, $prev_text, (($page>1)?($page-1):false)); $next_bttn = createPageLink($pagination_link, $next_text, (($page<$total_pages)?$page+1:false)); //Create pagination div $pagination.= "<div class=\"pagination\" style=\"direction:; float:right;\">\n"; //Add prev/next buttons and page links if($direction==1) { $pagination .= $prev_bttn . $page_links . $next_bttn; } else { $pagination = $next_bttn . $page_links . $prev_bttn; } //End pagination div $pagination.= "</div>\n"; } echo $pagination; ?>
-
Really? That would produce an error after the first iteration of the loop. :-\
-
Just tested out the code I posted previously and there are two minor errors: #1: change the IF statement to this: if(isset($_POST) && count($_POST)>0) These lines had a typo in the function name: $name = "'" . mysql_real_escape_string($name) . "'"; $value = "'" . mysql_real_escape_string($value) . "'"; Other than that the script *should* work. EDIT: One thing the above script does not do is validate the values with respect to the database field constraints. So, if those fields have a limit of x number of characters, expect numbers, etc. then you should be validating them. Also, since these are configuration settings I would assume each config value has specific validation that is separate from the database constraints. So, a 'domain name' would have different constraints as opposed to an "admin email" setting. I would suggest creating a function with a switch statement to validate all the particular values and call it within the loop that parses the POST data.
-
Give this a try (not tested so there may be some syntax errors): <?php $response = ''; if(isset($_POST)) { //Changes were submitted //Put post data in another array for cleaning/parsing $configValues = array(); foreach($_POST as $name => $value) { $name = "'" . mysql_real_esacape_string($name) . "'"; $value = "'" . mysql_real_esacape_string($value) . "'"; $configValues[$name] = $value; } //Create/run update query $caseConditions = ''; foreach ($configValues as $name => $value) { $caseConditions .= " WHEN {$name} THEN {$value}\n"; } $query = "UPDATE `configTable` SET `config_value` = CASE `config_name` {$caseConditions} END WHERE `config_name` IN (" . implode(', ', array_keys($configValues)) . ")"; $result = mysql_query($query) or die (mysql_error()); $response = 'Config values were updated'; } //Get the current values $query = "SELECT `config_name`, `config_value` FROM `configTable`"; $result = mysql_query($query) or die (mysql_error()); //Process results into form fields $configFields = ''; while($record = mysql_fetch_assoc($result)) { $configFields .= "{$record['config_name']}: "; $configFields .= "<input type=\"text\" name=\"{$record['config_name']}\" value=\"{$record['config_value']}\" /><br />\n"; } ?> <html> <head></head> <body> <div style="color:red;"><?php echo $response; ?></div> <form name="updateConfigs" action="" method="POST"> <?php echo $configFields; ?> <br /> <button type="submit">Update Config Values</button> </form> </body> </html>
-
That will not work. When file_get_contents() calls an external php file the web server will parse that php file and return the processed results (i.e. the rendered html code), it will not return the PHP code.
-
include() is used to include PHP code - not html. Use file_get_contents() to get the html code of the html code and echo it out.
-
// Images Directory imagesDir = "<?php echo $baseurl; ?>/wysiwyg/icons/";
-
The problem is with the $n_american array. You are apparently wanting each "country" index to have a value of the country arrays created above. But, you are encasing the country array variables withing single quotes. variables are not interpreted when enclosed within single quotes. So, the value of the 'Mexico' index is the literal string of "$mexico" not the array of Mexican territories. But, I'm not sure it would work the way you want using doub;e quotes either. Just use this: $n_america = array( 'Mexico' => $mexico 'United States' => $us, 'Canada' => $canada );
-
Yeah, not only did you fail to read the previous posts where a solution was provided (#3 & #7) your solution is flawed - for reasons stated in the previous posts.
-
Nice, I did not know this Yeah, I'm a bit of a perfectionist. Checking for divisible by 4 will work for any date from 1901 to 2099. So, if we are working with birth dates of people that are currently living they would have to be 110 years old for the rule to fail - pretty unlikely. But, since developers will commonly pick up existing code to reuse for other purposes and/or the OP could be dealing with a geaneology project it's better safe than sorry to do it exactly right.
-
Yes, that function works for any year that you pass to it and returns a true or false based upon whether that year is a leap year. Nope, leap year is not determined by whether it is divisable by 4. There is more to it than that. If the year is divisable by 4 AND it is not divisible by 100 (UNLESS it is also divisible by 400) then it is a leap year: ($year%4==0 && ($year%100!=0 || $year%400==0)) After some more though on this I was a littel curious and decided to test what the performance difference was between determining leap year via the date() function vs. determining the leap year through mathmatical calculations. Although we are talking about milliseconds, the following functin is almost 100 times faster thant he function I posted above: function isLeap($year) { return ($year%4==0 && ($year%100!=0 || $year%400==0)); }
-
No, the date() function requires a timestamp - if you don't supply one then it assumes the current date/time. Here is a small function that you can pass a year value to to determine if it is a leap year (also included a loop for testing purposes): <?php function isLeap($year) { return (date('L', mktime(0, 0, 0, 1, 1, $year))==1); } //For testing for($year=1899; $year<2020; $year++) { If (isLeap($year)) { echo "$year : LEAP YEAR<br />\n"; } else { echo "$year : Not leap year<br />\n"; } } ?>
-
The error is exactly what I posted. That is all of the information that I get, then a white blank screen. I can post the entire array.txt file as it sits when I get back to work (about 15-20 minutes). mjdamato, I tried to get the serialize to work but I don't have the time to wait, you mentioned this code and it worked. If it is so inefficient, why did you suggest it? As freakstyle stated you should be getting more information with the error - such as a LINE NUMBER which would tell us where the error was occuring. I said YOUR code was inefficient, not mine. If you had provided more information previously I would have been willing to help. There is no reason to read through the entire text file to create an array and THEN go through each record in the array. You can do all the work in one loop - not two. Secondly doing multiple queries in loops puts a HUGE overhead on the server. You could easily combine the values for the multiple records and insert them with one query. Lastly, your loop to insert the records as an artificial limit of 3664. If the data has less values then that you will get an error and if there are more values you will not process them all. Since you are using an array, you should be using foreach(). Even if you expect the array to always contain 3664 records you shoudl still use foreach() and just do a check of the count() on the array for validation.
-
I'm not going to take the time to implement a right-click menu. But this should get you started. I used PHP to create the table to cut down on the code to write. If you need the raw output let me know and I can post it. The solution below only requires theuser to click once in a row to select the cells - not twice as you were asking. My implementation is more intuitive. <?php $table = "<table class=\"selector\" border=\"1\">\n"; for ($rows=0; $rows<10; $rows++) { $table .= " <tr>\n "; for ($cols=0; $cols<10; $cols++) { $table .= " <td id=\"{$rows}-{$cols}\" onclick=\"selectCells(this);\"> </td>\n"; } $table .= " <td>"; $table .= "<select name=\"{$rows}-color\" id=\"{$rows}-color\" disabled=\"disabled\" onchange=\"setColor(this)\">"; $table .= "<option value=\"\">--Select Color--</option>"; $table .= "<option value=\"#ff0000\">Red</option>"; $table .= "<option value=\"#00ff00\">Green</option>"; $table .= "<option value=\"#0000ff\">Blue</option>"; $table .= "</select>\n"; $table .= "</td>\n"; $table .= "\n </tr>\n"; } $table .= "</table>\n"; ?> <html> <head> <style> .selector td { height:20px; width:20px; } </style> <script type="text/javascript"> function selectCells(selectedCell) { var selectedRow = selectedCell.id.substr(0, selectedCell.id.indexOf('-')); var selectedCol = selectedCell.id.substr(selectedCell.id.indexOf('-')+1); //Unset all previous selections for (var row=0; row<10; row++) { document.getElementById(row+'-color').disabled = (row!=selectedRow); document.getElementById(row+'-color').disabled = (row!=selectedRow); if (row==selectedRow) { document.getElementById(row+'-color').selectedIndex = 0; } for(var col=0; col<10; col++) { var cellObj = document.getElementById(row+'-'+col); if (cellObj.selected==true) { cellObj.selected = false; cellObj.style.backgroundColor = '#ffffff'; } } } for(var col=0; col<10; col++) { var cellObj = document.getElementById(selectedRow+'-'+col); cellObj.style.backgroundColor = (col<=selectedCol) ? '#0066ff' : '#ffffff'; cellObj.selected = (col<=selectedCol) ? true : false; } } function setColor(selectObj) { var selectedRow = selectObj.id.substr(0, selectObj.id.indexOf('-')); var selectedColor = selectObj.value; if (selectedColor=='') { return false; } for (var col=0; col<10; col++) { var cellObj = document.getElementById(selectedRow+'-'+col); if(cellObj.selected) { cellObj.style.backgroundColor = selectedColor; cellObj.selected = false; } } } </script> </head> <body> <?php echo $table; ?> </body> </html>
-
LOL, That's my code from your other post today. What is the specific line that is producing the error? In any event that is some VERY inefficient code. First of all you are creating a loop with an artificial limit instead of using the actual count of the array and you only need to do one query to insert all the values.
-
I'm really not following what you want. Are you wanting a new window to open for each error? If so, just change the window name to an empty string. var dwindow = window.open('debug.php','',',toolbar=1,resizable=1,scrollbars=yes');
-
You have nothing in that script that would give you any errors if something was not right. I suspect either the POST value is not set or there is a database error. Try this: <?php include("config.php"); $db_link = mysql_connect($db_server, $db_uname, $db_pass) or die ("unable to connect to database server"); mysql_select_db($db_name, $db_link) or die ("unable to select to database"); if (!isset($_POST['user'])) { echo "The post value for 'user' is not set."; } else { $user = mysql_real_escape_string(trim($_POST['user'])); $date = mysql_real_escape_string(trim($_POST['date'])); $time = mysql_real_escape_string(trim($_POST['time'])); $device = mysql_real_escape_string(trim($_POST['device'])); $info = mysql_real_escape_string(trim($_POST['info'])); $query = "INSERT INTO `alanz`.`logs` (`user`, `date`, `time`, `device`, `info`) VALUES ('{$user}', '{$date}', '{$time}', '{$device}', '{$info}')" mysql_query ($query, $db_link) or die ("Query:<br />{$query}<br />Error:<br />" . mysql_error()); } ?>
-
This code will read the text file and convert it into an array variable. <?php $textData = file('array_data.txt'); $dataArray = array(); $tempArray = array(); $array_start = false; foreach ($textData as $line) { if ($array_start && preg_match("/\[([^\]]*)\] => (.*)/", $line, $keyValue)!= 0) { $tempArray[$keyValue[1]] = $keyValue[2]; } if(substr($line, 0, 1)=='(') { $array_start = true; } if(substr($line, 0, 1)==')') { $array_start = false; $dataArray[] = $tempArray; $tempArray = array(); } } print_r($dataArray); ?>
-
I don't think those have anything to do with what the OP is asking for now. I believe the OP is now asking how to logically break a word at a syllable, i.e. hyphenate it. Example (lines 1 & 3): Toyota has for years blocked access to data stored in de- vices similar to airline "black boxes" that could explain crashes blamed on sudden unintended acceleration, accord- ing to an Associated Press review of lawsuits nationwide and interviews with auto crash experts. @OP: If you are creating the PDF file via the PHP PostScript functions there are a couple that may help: ps_show_boxed() and/or ps_hyphenate(). however, if you are creating the PDF through other means, e.g. writing raw postscript and distilling this may be more difficult as you will not "know" ahead of time where the word will need to break. @Dennis1986, I like how you updated your original post to include information about using substr() after I posted a solution using it.
-
I used some PHP code to build the select lists to cut down on the HTML code needed. But, the javascript function below will ensure the "day" select list has the correct number of days according to the month/year the user has selected: <?php $monthOptions = ''; $dayOptions = ''; $yearOptions = ''; for($month=1; $month<=12; $month++) { $monthName = date("M", mktime(0, 0, 0, $month)); $monthOptions .= "<option value=\"{$month}\">{$monthName}</option>\n"; } for($day=1; $day<=31; $day++) { $dayOptions .= "<option value=\"{$day}\">{$day}</option>\n"; } for($year=1900; $year<=2010; $year++) { $yearOptions .= "<option value=\"{$year}\">{$year}</option>\n"; } ?> <html> <head> <script type="text/javascript"> function updateDays() { //Create variables needed var monthSel = document.getElementById('month'); var daySel = document.getElementById('day'); var yearSel = document.getElementById('year'); var monthVal = monthSel.value; var yearVal = yearSel.value; //Determine the number of days in the month/year var daysInMonth = 31; if (monthVal==2) { daysInMonth = (yearVal%4==0 && (yearVal%100!=0 || yearVal%400==0)) ? 29 : 28; } else if (monthVal==4 || monthVal==6 || monthVal==9 || monthVal==11) { daysInMonth = 30; } //Add/remove options from days select list as needed if(daySel.options.length > daysInMonth) { //Remove excess days, if needed daySel.options.length = daysInMonth; } while (daySel.options.length != daysInMonth) { //Add additional days, if needed daySel.options[daySel.length] = new Option(daySel.length+1, daySel.length+1, false); } return; } </script> </head> <body> Birthdate:<br /> <select name="month" id="month" onchange="updateDays();"> <?php echo $monthOptions; ?> </select> <select name="day" id="day"> <?php echo $dayOptions; ?> </select> <select name="year" id="year" onchange="updateDays();"> <?php echo $yearOptions; ?> </select> </body> </html>
-
Unless you want the user to submit the form after selecting the month (or use AJAX) - neither of which makes sense - then you should be using JavaScript for this. Moving post to correct forum.
-
Use substr() $limit = 10; $firstpart = substr($string, 0, $limit); //The first 10 characters $secondpart = substr($string, $limit); //The remaining characters
-
If you want this to happen automatically and not by some user action, then why don't you just build the table with 20 cells to begin with? JavaScript has it's uses, but if you want 20 cells by default, JavaScript is not the way to go.
-
I don't use dreamweaver, so I can't help you with any functionality implemented through that or even with how to implement your own code. But, I do have a solution. Raio groups can be an odd imlpementation to understand. A radio "group" is a collection of radio buttons. The group itself does not have a value - each of the radio buttons have thier own values. So, you need to iterrate through all of the radio options to find which one is checked to get the selected value of the group. Another problem is that if there is only one button in the group it is not treated as a group. This is typically not an issue, but I try to build my code such that it will work in any situation. Anyway, here is a function that takes all of the above into consideration and returns the selected value of a radio group (or returns false if none are selected) function radioGroupValue(groupObj) { //Check if there is only one option (i.e. not an array) if (!groupObj.length) { //Only one option in group return (groupObj.checked) ? groupObj.value : false; } //Multiple options, iterate through each option for (var i=0; i<groupObj.length; i++) { //Check if option is checked if (groupObj[i].checked) { //Return value of the checked radio button return groupObj[i].value; } } //No option was selected return false; } You need to pass an object reference to the function. So, here is an example of how you could add up all the selected options from multiple radio groups: function calcTotal() { var formElements = document.forms['formName'].elements; var total = 0; total += radioGroupValue(formElements['group1Name']); total += radioGroupValue(formElements['group2Name']); total += radioGroupValue(formElements['group3Name']); total += radioGroupValue(formElements['group4Name']); alert("The total is " + total); return; }
-
Is there a way to unzip zip files using bzip2 or zlib?
Psycho replied to OsvaldoM's topic in PHP Coding Help
If you are using PHP5 and zip support is configured on the server you can use the ZipArchive class. Specifically the extractto method: http://www.php.net/manual/en/function.ziparchive-extractto.php