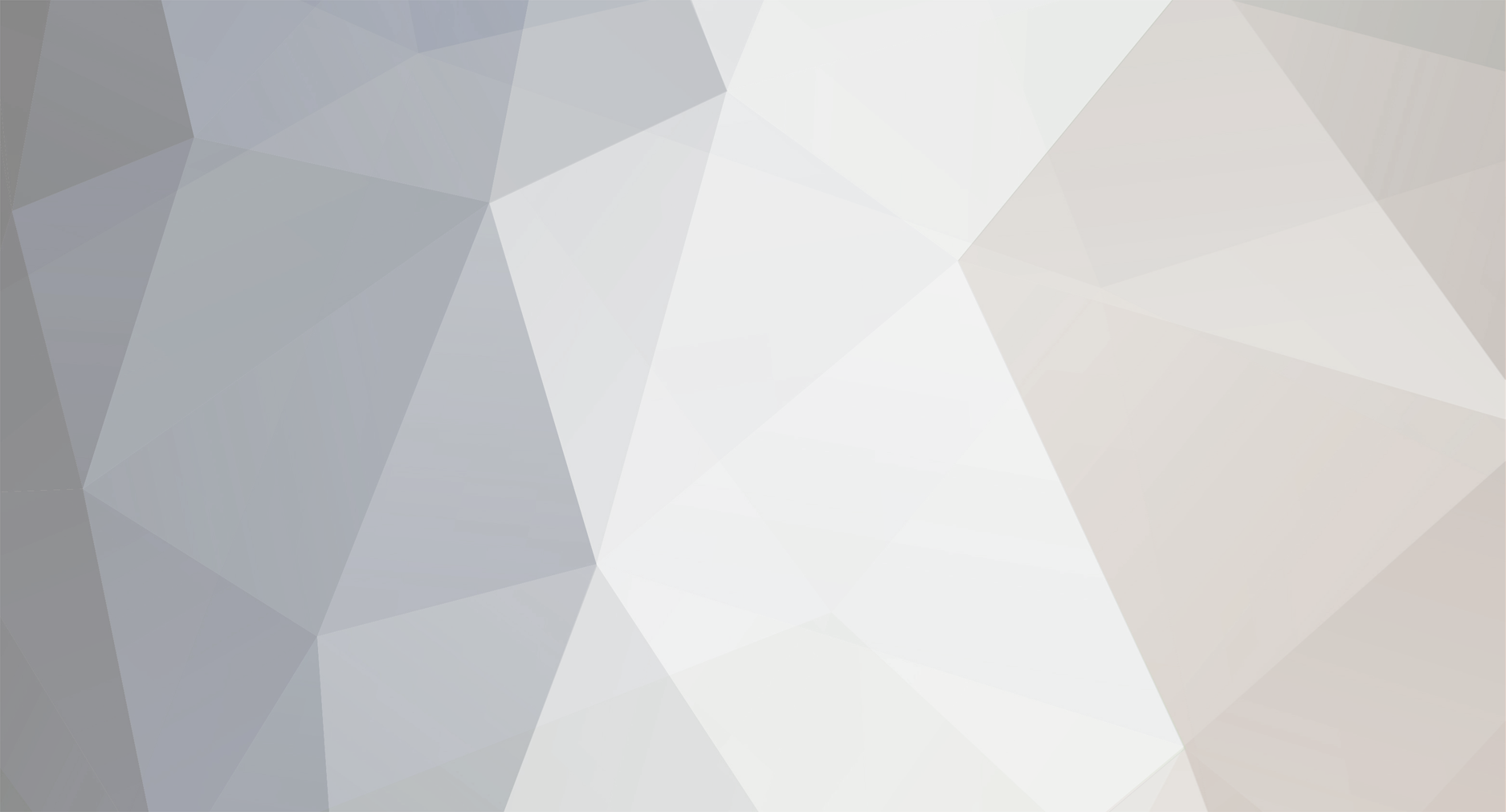
Psycho
Moderators-
Posts
12,159 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
I would simply associate each product with all four levels of groupings ass applicable. So, let's assume you have four tables for each level: Section 1 (e.g. Furniture) Section 2 (e.g. Living Room) Section 3 (e.g. Sofas) Section 4 (e.g. Sleepers) Every lower section will have a parent ID field that relates it to the next higher level (i.e. Sofas will be associated with Living Room). So, you don't have to have child records for each possible parent record. Then, for your products, have four separate fields to identify the relationship between the product and all four levels of sections. If you have a product that is related to records at sections 1 & 2, then the fields for sections 3 & 4 will remain NULL. So, if you need to find all products related to Furniture, just query all products where the ID in the section1 field is the same as the ID for furniture. Now, if you need to relate products to multiple values int eh same section it gets more complicated, But, you didn't state thar requirement.
-
Then you just need to have a table with the questions and build a form that displays the question, has a hidden input for the question ID and a text box for the response. Veery straight forward.
-
You really should start another thread as what you are asking now has nothing to do with the original title. But, I'll provide answers to your last post and you can start a new thread if there is additional help needed. 1. How you should "store" the questions depends on many factors. If the questions are all mutiple choice with say four possible answers, just create a record for each question with fields for 1) the question, 2-5) The answers, and 6) The correct answer. Of course you could always put the correct answer in position 1 and just randomize how the answers are displayed. It all depends on how YOU want to work it. Then just build a page that gets the question record from the database and then builds the form, displaying the question and the possible answers as radio button options. Of course, if the questions are not simply multiple choice then it might get more difficult. I can't really provide any more help as I have no idea what format your questions/answers need to be in. If it gets too complex you can just store the entire form in the database. For the time remaining, use a session value to store the start time when the users starts the test. Then on each page load you would calculate the time remaining and display it. Pretty simple concept. Example Code: <?php //Setting start time when user starts test $_SESSION['start_time'] = time(); ?> Form page code: <?php if(isset($_POST['question_id'])) { //Determine time left $time_left = 3600 - (time() - $_SESSION['start_time']); //See if thre is time left if ($time_left<1) { $output = "The time to take this test has expired."; } else { //Create display for the time left $minutes = floor($time_left/60); $seconds = printf("[%02s]\n", ($time_left-($minutes*60)));; $output = "Time remaining: {$minutes}:{$seconds}<br /><br />\n"; $question_id = $_POST['question_id']; $answer_id = $_POST['answer_id']; //Create code to store the user's response in the DB //Get the next question $question_id++; $query = "SELECT question, answer1, answer2, answer3, answer4 FROM questions WHERE id = {$question_id}"; $result = mysql_query($query); $question = mysql_fetch_assoc($result); //create the form $output = "<form action=\"\" method="POST">\n"; $output .= "<b>{$question['question']}</b>\n"; $output .= "<input type=\"hidden\" name=\"question_id\" value=\"$question_id\"><br /><br />\n"; //Add questions in a random order foreach(shuffle(range(1,4)) as $answer_id) { $answer = $question['answer'.$answer_id]; $output .= "<input type=\"radio\" name=\"answer_id\" value=\"$answer_id\">\n"; $output .= "{$answer}<br />\n"; } //Close out the form $output .= "<button type=\"submit\">Answer</button>\n"; $output .= "</form>\n"; } } ?> <html> <head></head> <body> <?php echo $output; ?> </body> </html> This is by no means complete code. There is no validation and it doesn't test to see if the last question has been reached. There is still much to do. I haven't even tested it, but the logic is sound.
-
You would just display a form for each question. I would create a table for the test and have a different record for each question. Then create code to pull the "current" question and display the form. When user submits the form, record the results to the database and then display the next question.
-
You are apparently doing queries within a foreach loop - vary bad idea. You can get all your values in a single query. Show the foreach and queries used and I can provide more details on that. But, as to the specific problem you are asking about, I think you are overcomplicating the process by appending arrays. Try this: while($x = mysql_fetch_array($q)) { //Do some stuff $string = 'some random string generated above'; $inserted_id = mysql_insert_id(); $array[$inserted_id] = $string; //This is the end of the foreach $increment++; } } Edit: teamatomic beat me to it, but I call foul since I was unearthing other problems not shown.
-
???. That is a description of a round robin tournament. The OP needs a single elimination tournament. @onthespot, You are asking for A LOT. You are asking for the logic, how to display it, etc., etc. You need to figure out all the pieces of functionality you want, then start coding each. If you have a problem with something specific, then post here on that specific problem. But, I'll give some advise. You don't need to "advance" someone to the next round. Using a database you would just need to assign each player/team a position (i.e. 1 to 16). Then just store the results of each matchup (i.e. winner_id and loser_id). You could then use the data of who played who and who the winners were to determine display the results and determine the current round and the remaining competitors. You don't even need to record the "rounds" played since 1 would play 16 ONLY in round 1, 1 would play 2 or 15 ONLY in round 2, etc. Displaying the results would just require you to determine how you want it displayed and then just walking though the process logically.
-
Delete all files in a folder except one with a certain name
Psycho replied to jamiet757's topic in PHP Coding Help
I've read your post a few times and it makes less sense each time I read it. The title states you want to delete all fiels in a folder except one with a certain name. Then the first paragraph you talk about deleting "declined" photos but provide no explanation of how that is determined. Then in the second paragraph you talk about deleting only files older than 7 days. And, in the last paragraph you state you need to delete files based on their names. What - EXACTLY - are you trying to accomplish? -
Typically, an exam like this is implemented with a single question presented at a time. Of course you can have a single form with all the questions presented, but there is no way to "save" a partially completed test if the user was to accidentally close the browser (it does happen). Well, there is a way to do timed saves using AJAX, but IMHO, that is only making this more complicated than it needs to be. So, if you make it one long form, then the user will need to submit in order for any data to be saved. And, at that point, the whole quiz would be complete anyway I guess. As for an "auto-logout" mechanism you need to be more specific. I was only referring to a function for the user to manually log out which, as I stated above, is kind of pointless in most situations as people don't use it. It seems you are really talking about enforcing the 60 minute time period. For enforcing the time period, you already have the information you need if you are saving the login timestamp. If the person submits a form after 60 minutes of the login, then you reject the submission. This is another reason why having one question submitted at a time would be advantageous as the user could save questions during the time period. With an all-or-nothing approach, if the user exceeds the 60 minutes before submitting the long form, then the user would not get any credit for questions they answered within the time period. In any event, you will want to give the user some visual cue as to the time remaining. JavaScript would be a good method since you could use it to provide a countdown. However, what if the user has JS disabled? If using a long form I would have the page state a static time of when the 60 minutes will end (i.e. "You must submit your answers by 10:22am"). If using a multi-part form, then you could print the time remaining one page load for each question. What it comes down to is you need to develop a process for the workflow of your application. This is before writing any code. Sit down and map out the processes on paper and ensure you have captured all the use cases and exception scenarios. Then write your code.
-
Whoops! That query should have had a less than instead of a greater than on the last line. But, I think you got the idea.
-
There is no "logging out" per se. Yes, you could create a Log Out button, but the only time that really makes sense, IMHO, is a financial/shopping site where another user could potentially launch back into a site with sensitive information. 99% of the time users simply close the browser window which destroys the session. (OK there is no such statistic, I just base that on my personal experience and what I typically see other users do). But, the solution is still simple enough. As the users progresses through the test you will need to store their answers to the database. Then if the user logs back in within the 60 minute time period you will be able to determine that. After the user logs in, just query the database to see if there are stored results. If so, you could either take them to the first unanswered question or start them from the beginning and display their previous answers as they step thorough the questions. Personally, I would give the user the option to do either.
-
OK, that would have been helpful previously. So, I would assume that if the user, for some reason, closes their browser and then attempts to log in within 60 minutes of the initial login that the user should be able to get back in, correct? Then, a small change to the sample code I provided should work. However, you would need to determine what should happen if a user has a subsequent log in within the 60 minutes. Are you storing thier answers or are they going to have to start over. Also, if the user completes the test do you still want them to be able to log in within the 60 minute time period? If not, you may want to add a "login_allowed" flag as offered previously. Example code //Escape user input $username = mysql_real_escape_string($_POST['username']); $password = mysql_real_escape_string($_POST['password']); //Update record with current time IF the account has never logged in before $query = "UPDATE `logins` SET `login_timestamp` = NOW() WHERE `username` = '$username' AND `password` = '$password' AND login_timestamp = ''"; $result = mysql_query($query); //Check if query ran succesfully if(!$result) { //Query failed, add error handling $response = "Query failed"; } else { //Set flag $error = false; if(mysql_affected_rows()!=1) { //Record doesn't exist OR credentials have been previously used //Run query to see when the initial login was $query = "SELECT `login_timestamp` FROM `logins` WHERE `username` = '$username' AND `password` = '$password'"; $result = mysql_query($query); if (mysql_num_rows($result)!=1) { //username/password doesn't exist $error = "That username/password is not valid."; } else { //Get record and check first login time $record = mysql_fetch_assoc($result); if ($record['login_timestamp']<strtotime("-60 minutes")) { //username/password was used more than 60 minutes ago $error = "That username/password has expired"; } } } //Check if error occured if ($error == false) { //Credentials have not been previously used OR user //logging back in within 60 minutes of initial log in // //Log user in and restore date } }
-
Example code $username = mysql_real_escape_string($_POST['username']); $password = mysql_real_escape_string($_POST['password']); $query = "UPDATE `logins` SET `login_timestamp` = NOW() WHERE `username` = '$username' AND `password` = '$password' AND login_timestamp = ''"; $result = mysql_query($query); if(!$result) { //Query failed, add error handling $response = "Query failed"; } else if(mysql_affected_rows()!=1) { //Record doesn't exist OR credentials have been previously used //Can either provide a single error/warning condition, OR can //provide different messages for the two conditions as follows: $query = "SELECT `login_timestamp` FROM `logins` WHERE `username` = '$username' AND `password` = '$password'"; $result = mysql_query($query); if (mysql_num_rows($result)==1) { $record = mysql_fetch_assoc($result); $response = "That username/password was used on {$record['login_timestamp']}."; } else { $response = "That username/password is not valid."; } } else { //Credentials have not been previously used, log user in }
-
But, you are "assuming" the code should be cleaned up. There may be reasons to retain the data for historical purposes. Likewise, if you don't need the data for historical purposes, then just delete the record when the user logs in and no further clean-up is needed. One other consideration is if the system should provide a warning (e.g. "That username/password has already been used"). In that situation, it "might" make sense to purge records after some time, but still you don't have to. Just use the logon timestamp to determine if the user should be told those credentials have already been used or if you just want to tell them the credentials are not valid.
-
You shouldn't need to know "which" user it is coming from. User's should be using different login credentials anyhow. Otherwise a user can just go to a different computer and log in. The OPS requirement was So, the first time a login credential is used, simply set a value to show that it was used and log the person in. The OP already stated he was already maintaining the session. I think you are overcomplicating the requirement. Heck, you could even just delete the record with the login credentials after the credentials are used.
-
You would do the same thing to look at acrchived news - just set an appropriate WHERE clause for the time period you need. You didn't state what the format of the field is in the database, but if it is datetime or a timestamp (and not simply a date field) then you need to ensure the timestamps you create for the queries are at midnight of the start of the search day or midnight at the end of the search day as appropriate. For example if trying to find all the articles in Dec. you would want the start timestamp to be at 00:00:00 on Dec 1st. (i.e. midnight between Nov. 30th and Dec 1st.) and the end timestamp would be at 24:00:00 on Dec. 31st (i.e. midnight between Dec 31st. and Jan. 1st.). Then just create your query like so: SELECT * FROM `tablenews` WHERE `Date` > $startTimestamp AND `Date` > $endTimestamp
-
Just set the login time when the user logs in the first time as schilly suggested. Then when a user attempts to log in you can ascertain that the account has already been used to log in previously. Then once the user's session ends they will not be able to get back in using the previous credentials.
-
Show us the query that is currently used to pull the records for the scrolling panel. Also, what "type" of field is the date field? Is it a date, datetime, or timestamp field? You will just need to modify that query to add a where clause to filter the results for records that are newer than 1 month (or whatever period you want). Example: SELECT * FROM `table` WHERE `Date` > $timestampOneMonthBack
-
If the problem only occurs when including the layout file then it stands to reason that the problem has something to do with the code there.
-
Let me pull out my crystall ball How are we expected to help you without seeing any code? You could just include a blank file and then put parts of the original file back in a part at the time until you experience the same problem so you can pinpoint the problem code, other than that I have no idea.
-
You should get in the habit of creating your query as a string variable. Then when there is a problem you can echo the query to the page or log it somewhere so you can debug the error. It is not always apparent what the error is when looking at how the query is built. It is much more apparent when looking at the final code of the query. Like this: $query = "INSERT INTO `orders` (`order_status`, `productcode`, `dispatch_date`, `customer_id`) VALUES ('Ordered', '$item', '$date', '$email')"; $result = mysql_query($query) or trigger_error("Query:<br />$query<br />Error:<br />".mysql_error());
-
To be honest I had started some code, but I had more questions than answers and I really didn't want to invest the time to formulate all the questions. If you want to provide detailed specifics then I might be able to provide more help. Specifically you need to provide details regarding the relationship, if any, between the data. The screenshot you provided is helpful, but the the image doesn't make it obvious what table the data for each column is coming from. For example,the first column is called BUS#. I would think that comes from the jobs table since that is where all the data is related to, but you also have a bus_info table. To make matters worse, your sample code in your second post shows data in a different order than your image and there are more fields than the image. Lastly, I can't tell if there is any relationship between sub-data of the columns. For example, in the image record 805 has three values under sides and three under advertiser. Is the first side value related to the first advertiser value? If so, how is that represented in the database. Also, in record 811, there appear to be three values under the installer column, but they are not separated into three cells as they are for other records. Was that intentional? If so, what is the logic to determine that?
-
Here's my input: It *may* work. Im not going to really review the code as using foreach() and while() loops to run multiple queries is just a bad idea. Running multiple queries like that will have a huge overhead on your server and can cause pages to timeout when loading. Why would you need to run the query against the "extraq" table for each $_REQUEST value when the value is not used in the query and you are not updating the data in that table within the loops? The data will be the same on each query, right? Not to mention you are using "*" to get allfields from the query, but only using one field. Plus, you are running this query on user input that is not validated! This is a perfect situation where someone could create havoc in your database using sql injection. if (isset($_REQUEST)) { //Get records from extraq table and put into array //Query only needs to be run once $query = "SELECT `id` FROM `extraq` WHERE `programid`='$programid'"; $result = mysql_query($schq); $extraq_IDs = array(); while($row=mysql_fetch_assoc($result)) { $extraq_IDs[] = $row['id'] } //Get keys for the request values $requestKeys = array_keys($_REQUEST); //Compute the values to be inserted $insertIDs = array_intersect($requestKeys, $extraq_IDs); //Create insert query $valuesAry = array; foreach($insertIDs as $id) { $valuesAry[] = "('$id', '" . mysql_real_escape_string($_REQUEST[$id]) . "', '$subid')"; } $query = "INSERT INTO $extraqopt (extraqid, value, subid) VALUES " . implode(', ', $valuesAry); $result = mysql_query($query); }
-
OK, your first post threw me off. What I understand now is that there is ONE poll and the table in question stores one record for each user who responds to the poll. If that is correct, then the database structure is still terrible and will make getting the data harder than it needs to be, but anyway... I absolutely abhore looping queries, as they are terribly inefficient, but I can't think of a better solution at the moment because of the design. I wrote all of this "freehand" so if you find syntax errors go ahead and fix them <?php //Gather results into array $results = array(); //Query for each question for ($qIdx=0; $qIdx<15; $qIdx++) { $results[$qIdx][1] = 0; $results[$qIdx][2] = 0; $results[$qIdx][3] = 0; $qField = "q" . ($qIdx+1); $query = "SELECT {$qField}, COUNT({$qField}) as count FROM `questionnaire` GROUP BY {$qField}"; $result = mysql_query($query); while($row = mysql_fetch_assoc($result) { $results[$qIdx][$result[$qField]] = $result["count"]; } } //Create array of the questions $questions = array( 'Do you like water?', 'Do you like soda?', // ..etc. ); //Create the output $output = ''; foreach ($questions as $idx => $question) { $output .= "<tr>\n"; $output .= "<td>{$question}</td>\n"; $output .= "<td>{$results[$idx][1]}</td>\n"; $output .= "<td>{$results[$idx][2]}</td>\n"; $output .= "<td>{$results[$idx][3]}</td>\n"; $output .= "</tr>\n"; } ?> <table> <tr> <th> </th> <th>yes</th> <th>no</th> <th>maybe</th> </tr> <?php echo $output; ?> </table>
-
Typically you would validate each field individually and give the user specific details about what fields need to be changed, but if you want to do it all in one go, then use the correct method for concatenating string - which is a period: if (!validCharacters($forname.$surname.$city))
-
Yes it is possible, but because you are using JQuery you "may" not be able to do it as easily. Typically a fade uses a settimeout() loop that repeats until the process is done. Simply interject an additional condition to check to determine whether the loop should repeat. For example, have a variable called runFadeOut and have the default value set to true. The loop would check that the fade is not complete AND that that variable equals true. If both are ture then the loop is repeated via settimeout(). So, if a new action takes place that you want to stop the current fadeout, that process would first set runFadeOut to fase to stop any current fade outs. But, as stated above you are using built in functions from JQuery, so standard JavaScript will not help. Perhaps someone with JQuery knowledge knows of a workaround.