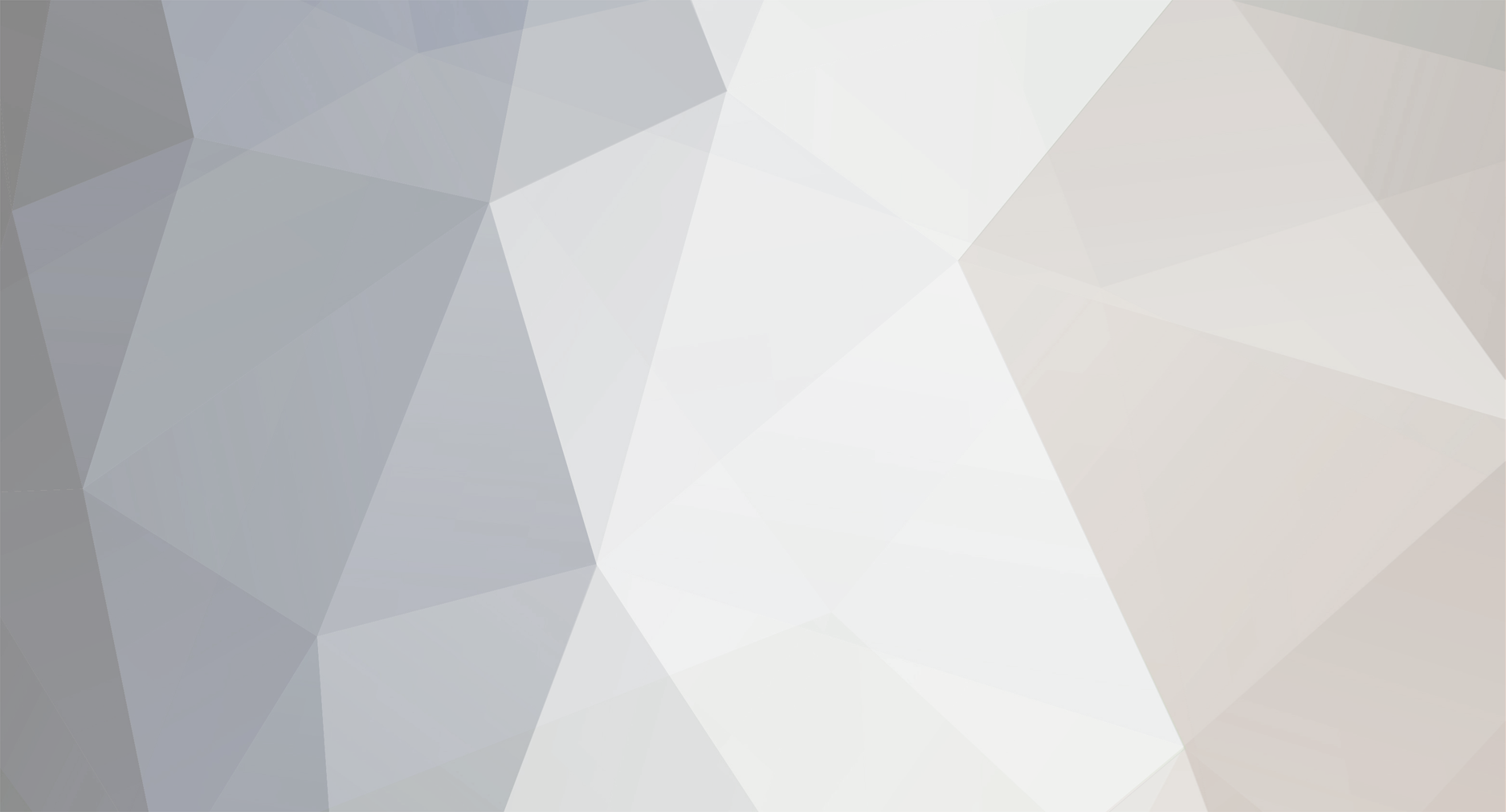
Psycho
Moderators-
Posts
12,159 -
Joined
-
Last visited
-
Days Won
130
Everything posted by Psycho
-
Note that this only works if they put the href content in double quotes. They could use single quotes or no quotes. I just didn't have the time to code for all three scenarios. <?php $url = "http://www.somesite.com"; $allowedDomains = array ( 'rapidshare', 'megaupload' ); $page = file_get_contents($url); preg_match_all("/<a.*?href="([^"]*).*?>.*?</a>/is", $page, $matches); echo "Links at $URL<br /> "; echo "<ul> "; foreach ($matches[1] as $match) { $validDomain = false; foreach ($allowedDomains as $domain) { if (strpos($match, $domain)) { $validDomain = true; break; } } if ($validDomain) { echo "<li>$match</li>"; } } echo "</ul> "; ?>
-
mod_rewrite is what you are looking for. http://articles.sitepoint.com/article/guide-url-rewriting Note: I can't speak to the quality of that tutorial, it was just the first one when I did a google search.
-
use ceil($l/3)*3 I knew there had to be an easier method and was even trying to use ceil() but couldn't figure out a way to make it work. Good one.
-
I had the same problem and discovered a solution - using modulus twice!. str_pad($l + (3-($l%3)%3))
-
sorting array descending/ascending order confusion
Psycho replied to silverglade's topic in PHP Coding Help
Yes, but the function will relate them by the index when you use it in this manner. By doing a multisort on the two arrays the function is associating $staff["givenname"][0] with $staff["surname"][0]. Read the manual for this function. Specifically look at examples #1 and #2 http://us3.php.net/manual/en/function.array-multisort.php -
Right, exactly as I pointed out. I really hope you are not using the original code with that additional WHERE clause added. I wrote a lot of code and was unable to test it, so I expect there may have been a few errors, but the logic is sound and is the right way to do this. I suggest you start with one table (the orders table) to get the information you need from that table. Then add just one table using a JOIN to get the information you need from that table, etc.
-
FIRST: You should really read a tutorial on how to do JOINS in your queries. You should be able to get all the information you need in ONE query. Your current process is terribly inefficient. SECOND: The WHERE caluse in your first query doesn't include a specific record, so it is getting ALL records. Then after you run your query you iterate through every record redefining $description on each iteration. But, you don't use $description in your loop, so what happens is that when the loop finished you are left with the description for the LAST record. I think you probably want to be specifying a specific record within the WHERE clause using an order ID or something similar. You appear to have this same problem on all of your queries. Give this a try. I had to make a lot of changes so I can't guarantee it will work - especially since I don't have your database to test against. Also, I took out a lot of the variables you were SELECTing in the query because I didn't see that you were actually using them. <?php $query = "SELECT adtype.description as adDesc, adsize.description as adSizeDesc, seller.name as student, design.description as designDesc, orders.designsugg as designsugg, orders.adfor as adfor FROM orders JOIN adtype ON orders.adtype = adtype.typeid JOIN adsize ON orders.adsize = adsize.sizeid JOIN seller ON orders.student = seller.sellerid JOIN design ON orders.design = design.designid WHERE orders.orderno = $orderno"; $result = @mysql_query($sql,$connection) or die(mysql_error()); $output .= "Ad Type: {$result['adDesc']}<br /><br /> "; $output .= "Ad Size/Pricing Information: {$result['adSizeDesc']}<br /><br /> "; $output .= "Sales Credit: {$result['student']}<br /><br /> "; $output .= "Design: {$result['designDesc']}<br /><br /> "; $output .= "Design Information: {$result['designsugg']}<br /><br /> "; $output .= "Ad Recipient: {$result['adfor']} "; print $output; ?>
-
sorting array descending/ascending order confusion
Psycho replied to silverglade's topic in PHP Coding Help
Your code is sorting by 'surname' first and then by 'givenname'. So, it will be sorted by lastname, firstname is ascending order: a comes before b, b before c, etc. Your results should be: - Champy, Many - Gilmore, James - Gilmore, Jason - Grisold, Gary -
If not using a database, you could use the same logic that mattal999 posted above but use a flat file to keep track of whether the script had been run that day (e.g. change the content of the file to the current date). Although, I would change the order of operations to update the trigger after the processes you want to run once have completed. Alternatively, you could create two files - one that is accessed by the users wich just displays the information and another which performs the scripting you want run once per day. Then use a cron job to schedule that script to run each day at a specified time.
-
exclude fields being checked for empty when using getElementByTagName
Psycho replied to laPistola's topic in Javascript Help
I would suggest that the proper method would be to explicitly state what fields should be validated instead of using a blacklist. But if you must... I would create an array of the fields that do not need to be validated. Then just check the field name against the array to determine if you should validate or not. I found the array prototype below on this page: http://snippets.dzone.com/posts/show/3631 So, I take no responsibility as to it's capability. Also, you cannot validate and input by using innerHTML. You have to use the value property. Plus, if you do a "return" before your alert, the alert will never fire. Give this a try (not tested) //Prototyp[e function for searching an array Array.prototype.find = function(searchStr) { var returnArray = false; for (i=0; i<this.length; i++) { if (typeof(searchStr) == 'function') { if (searchStr.test(this[i])) { if (!returnArray) { returnArray = [] } returnArray.push(i); } } else { if (this[i]===searchStr) { if (!returnArray) { returnArray = [] } returnArray.push(i); } } } return returnArray; } //Create an array of non required fields var nonRequiredFields = new Array ('field-1', 'field-3', 'field-10'); function checkF() { //reference to input fields object var allInputs = document.getElementsByTagName('input'); //Iterrate through all the input fields for (var i=0; i<allInputs.length; i++) { //Check that field is NOT in non required fields if (!nonRequiredFields.find(allInputs[i].name)) { //Check if field is empty if(!allInputs[i].value) { alert("Required field(s) are empty, please make sure all required fields are entered.") return false; } } } //All required fields are enbtered return true; } -
Speaking for myself, I like problems where you have to think outside the box. And I like the challenge of trying to solve a problem with an elegant, concise solution. I'm no RegEx guru, but I can stumble my way through when I have to. This RegEx is not very complicated though. preg_replace() simply finds text that matches the expression of the first parameter and replaces it with the text in the second parameter (with the third parameter being the text to be operated on). The one part that is a little more "advanced" is the use of a back reference. Let me explain. Here is the RegEx pattern: "/(.{3})/" The period is a wildcard character that matches any character. The "{3}" states that I want to find three of those, i.e. 3 of any character. So, that will find all groupings of three characters. Then by putting parents around that I create a backreference to the matches. I can then use that backreference in the replacement expression. The backreferences are referenced using "\1" for the first backreference, and \2 for the second, etc. I only had one backreference so, I just use "\1" in my replacement expression which is "\1 ". So basically the full line finds all three character parts and replaces then with their original value plus a space.
-
My signature line states Not to be rude, but this is a help forum. I would hope that the people receiving help can solve simple syntax errors. Anyway, the code below is fixed and tested: <?php //Used for testing $find = array ( array('rank'=>1), array('rank'=>, array('rank'=>4), array('rank'=>, array('rank'=>7), array('rank'=>5), array('rank'=>1), array('rank'=>4), array('rank'=>1), array('rank'=>5) ); //Create an array of the valid options $all_ranks = range(1, 10); //Create new array and fill with used ranks $used_ranks = array(); foreach ($find as $record) { if (!in_array($record['rank'], $used_ranks)) { $used_ranks[] = $record['rank']; } } //Get the diffrence of $all_ranks and $used_ranks (i.e. the unused ranks) $unused_ranks = array_diff($all_ranks, $used_ranks); //Create the options for select list $unused_rank_options = ''; foreach ($unused_ranks as $rank) { $unused_rank_options .= " <option value=\"{$rank}\">{$rank}</option>\n"; } ?> Rank: <select name="rank"> <?php echo $unused_rank_options; ?> </select> Output: Rank: <select name="rank"> <option value="2">2</option> <option value="3">3</option> <option value="6">6</option> <option value="9">9</option> <option value="10">10</option> </select>
-
I'll throw my hat into the ring function padByOct($value) { $parts = explode('.', $value); $pre = str_pad($parts[0], (strlen($parts[0])+((3-strlen($parts[0])%3)%3)), '0', STR_PAD_LEFT); $post = str_pad($parts[1], (strlen($parts[1])+((3-strlen($parts[1])%3)%3)), '0', STR_PAD_RIGHT); return preg_replace("/(.{3})/", "\1 ", $pre).'.'.preg_replace("/(.{3})/", " \1", $post); } $str = '1011101.1011'; echo padByOct($str); //Output: 001 011 101 . 101 100
-
What you are asking is about several different pieces of functionality, all of which would require different implementations based upon your needs. There are many ways to implement multiple languages and it is up to you to determine the best approach to what you need to accomplish. Here are the things I would consider when implementing such a functionality. How to determine what language to show the user. You can use session data, cookie data or a combination of both. You can also leverage the user's system to try and dynamically predetermine what language might be the right one for them. Here is a small script I have used to determine the user's language and set a variable to be used later in the script. It works by first checking if the user has selected a new language. If not then checking to see if they have a saved language in session data. If not then checking to see if they have a saved value in cookie data. If not, then go with the default. <?php //Create array of available languages // NOTE: Could programatically create this list based upon available language files or DB //Use ISO two letter abbreviations. $languageListAry = array ('en', 'sp', 'fr'); //Set default to first language in list $language = $languageListAry[0]; //Check if request was made to change the language via query string if (isset($_GET['language']) && in_array($_GET['language'], $languageListAry)) { $language = $_GET['language']; setcookie('language', $language, time()+3600*24*365); //Expires in 1 year $_SESSION['language'] = $language; //Check if there is a saved language value in the COOKIE } else if (isset($_COOKIE['language']) && in_array($_COOKIE['language'], $languageListAry)) { $language = $_COOKIE['language']; $_SESSION['language'] = $language; //Check if there is a saved language value in the SESSION (In case cookies are disabled) } else if (isset($_SESSION['language']) && in_array($_SESSION['language'], $languageListAry)) { $language = $_SESSION['language']; } ?> You will have to determine how you will change the content based upon the selected language and how you will store/retrieve that data. One way I have done this is by using placeholders in my content (e.g. "[WELCOME_MESSAGE]") and then build my pages into a variable instead of directly echo'ing the content. Once the entire page is built, I will replace all the placeholders with the appropriate text based upon the language. There are also ways to do this with output buffering. Depending upon how much content there is I might also categorize the replacement text according to the pages. Many times the content on a particular page will be somewhat dynamic (e.g. error messages), but I will have all the possible text for each page categorized. This is especially helpful when the text is in a database as you can have columns for the page, the placeholder name, and the actual content. You would just need to pull the content for the current page instead of all the text. The other option is to have different language files for each page with the appropriate references to each placeholder. Then just pull the appropriate language file and make the replacements.
-
well, this is an implementation of COMET, the idea is to send alerts to the client without a request http://en.wikipedia.org/wiki/Comet_%28programming%29 the user request the main page, and the conection is open, at the time the table is update, this is displayed on the user's browser without a request by the user(real-time alarms) You should not put an infinite loop on the server code. "Comet" typically uses AJAX code. If you are using AJAX, then you should have JavaScript code ont he client side that performs a request to the server on set intervals and brings down any new content. Having infinite loops on the server is going to bring your server to a crawl with just a small number of users. And, to be honest, I'm not even sure how having an infinite loop on the server would even work. How do you populate the new data back down to the client in that model without the client making an AJAX request?
-
This is NOT a PHP issue, this is basic HTML. The fact that you are using PHP to create the hyperlink is irrelevant to the issue. [Moved post to HTML forum] You cannot put a hyperlink around a table element. It seems to work in IE (except the hand icon doesn't appear on mouseover), but IE tends to be very forgiving of coding errors. By your use of FONT tags (which have been deprecated for years) I think you need a refresher on HTML coding. Assuming you are using PHP to echo the hyperlink, try this: echo "<tr>\n"; echo "<td width=\"330px\" height=\"103px\" background=\"{$image}\" valign=\"middle\""; echo " style=\"border:1px solid #99731E;color:#FF6600;font-family:Arial, Sans-serif;font-size:16pt;font-weight:bold;text-align:center;\">\n"; echo "<a href=\"http://www.u-stack.com/display.php?id={$id}\" style=\"text-decoration: none\">{$newname}</a>\n"; echo "</td>\n"; echo "</tr>\n"; Or, better yet, create a class for the td style properties.
-
I suppose the Web server is seeing the requests fromthe same browser as a single instance. Since you have an infinite loop running it doesn't have any available resources for that instance. But, it may be seeing the use of different browsers as different instances. A better question is why in the world would you want to have an infinite loop running? There is most definitely a beeter way to accomplish what you are trying to do.
-
I believe the 'used' ranks are sub elements in the multi-dimensional array $find. So, you would need to iterrate through all of those. <?php //Create an array of the valid options $all_ranks = range(1, 10); //Create new array and fill with used ranks $used_ranks = array(); foreach ($find as $record) { if (!in_array($record['rank']), $used_ranks) { $used_ranks[] = $record['rank']; } } //Get the diffrence of $all_ranks and $used_ranks (i.e. the unused ranks) $unused_ranks = array_diff($all_ranks, $used_ranks); //Create the options for select list $unused_rank_options = ''; foreach ($unused_ranks as $rank) { $unused_rank_options .= "<option value="{$rank}">{$rank}</option> " } ?> Rank: <select name="rank"> <?php echo $unused_rank_options; ?> </select>
-
<?php function showSelectedRecords($dataAry, $selectionAry) { foreach ($selectionAry as $dataIdx) { echo "<tr><td colspan=\"2\"> </td></tr>\n"; echo "<tr>\n"; echo "<td align=left valign=top width=180>"; echo "<a href=\"{$dataAry[$dataIdx]['url']}\"><img src=\"{$dataAry[$dataIdx]['image']}\" border=\"0\"></a>"; echo "</td>\n"; echo "<td valign=\"top\" style=\"padding-left:10; font-size:14;font-weight:bold;\">"; echo "{$dataAry[$dataIdx]['name']}<br /><br />{$dataAry[$dataIdx]['amenities']}<br />"; echo "<p align=\"justify\">{$dataAry[$dataIdx]['abstract']}</p>"; echo "</td>\n"; echo "</tr>\n"; echo "<tr>\n"; echo "<td colspan=\"2\"><br /><hr size=\"1\" color=\"c4940f\"></td></tr>\n"; } } @include($document . "destination-data.php"); //Create an array of the items to display //from the $offroad array $selected = array(1,3,4,7,9); showSelectedRecords($offroad, $selected); ?>
-
When using double quotes to delimit text and variables within the quotes will be interpreted as the value of those vairables. however, in single quoted test the variable is not interpreted: $foo = 'bar'; echo 'I'm going to the $foo'; //Output I'm going to the $foo echo "I'm going to the $foo"; //Output I'm going to the bar This works fine as long as there is a space after the variable to be interpreted (or some characters). But, if you need other text to display right after the variable, the PHP parser will not know where the variable name ends. Suppose you have a variable which holds a numeric value for gigabytes of RAM and you want to display it like this: "4GB" This would not work $ram = '6'; echo "The PC has $ramGB of ram."; //Output: The PC has of ram The parser will think the variable is $ramGB. So, you can further delimit variable in double quotes by putting them in curly braces $ram = '6'; echo "The PC has {$ram}GB of ram."; //Output: The PC has 6GB of ram Therefore, I always use curly braces around my variables in double quotes as a standard practice. It also helps to make the variables stand out when reviewing code.
-
There is no mistery here. I have not readall the code, but there are two things you need to do: 1. Enclose HTML tag parameters in quotes. Wrong: echo '<a href=' . $value . '>Link</a> If the $value has a space in it the result will be <a href=some value.htm>Link</a> Right: echo "<a href=\"{$value}\"'>Link</a> Also, for any vlaue being put into a URL string you should use urlencode to convert any problematic characters for URL parsing: $value = urlencode($value); echo "<a href=\"{$value}\"'>Link</a>
-
[SOLVED] PHP Multi-Language code help
Psycho replied to worldcomingtoanend's topic in PHP Coding Help
That is precisely why I asked to see the URLs for an English vs German page. If there is not going to be some programatic way of determining the corresponding page from one language to another they you will have to build a list of every page and it's corresponding page in the other language. Much easier to just build the site in a format that it can be done programatically. Also, with only two languages to support, having two copies of the site is easy enough to manage. But, another method of accomplishing this - which would be a must if supporting several lanaguages - would be to build all your pages with placeholders for the text and have separate pages that hold the appropriate text for each placeholder based upon the laguage. Then when the page loads it will detemine the correct language, load the appropriate language and then replace the placeholders with the actual text. This way you have only 1 page and would just need to manage the language files. -
Without seeing the "real" data it is difficult to determine the problem. If the line has a space or other "hidden" character you can't do a comparison with "See". Also, '\t' will not produce a tab character when delimited within single quotes - it will only produce the actual characters of '\t'. You need to use double quotes. The code - as written - takes a chunk of data and does a split on the tab character. But, you do not state that there are tabs in the data only that "See" is on some lines by themselves and other lines have two pieces of data which appear to be separated by multiple spaces. It would be helpful to know EXACTLY how those two pieces of data are separated. Are the separated by a tab, by a fixed number of spaces or are they fixed-width? The answer will detemine the best solution. Use file() to read the file into an associative array with each line a separate element in the array http://us2.php.net/manual/en/function.file.php This example uses preg_split to split the data line on one or more spaces, but depending on how theY really are delimited this is probably not the best solution. For testing purposes you will want to echo PRE tags before and after calling this function so the HTML output will look like the file created. Otherwise the presentation of the HTML output will not show line breaks. function openInFile ($file) { $lines = file($file); foreach ($lines as $line) { $line = trim($line); if ($line == 'See') { echo "rtv\n"; } else { $dataAry = preg_split("/[\s]+/", $line); echo "{$dataAry[0]}\t{$dataAry[1]}\n"; } } }
-
While Garethp is correct that that loop will never run, I would not suggest using his "fix" unless you want an infinite loop. You must use two equal signs to see if two values are equal. using a single equal sign will only assign the value to the variable. However, it looks like you are using the $Loops variable as a switch with 1 indicating that the loop should continue and any other value that it shoudl exit. unless you have some other use for the value assigned to that variable, you should just use true/false instead.
-
[SOLVED] PHP Multi-Language code help
Psycho replied to worldcomingtoanend's topic in PHP Coding Help
Change the last line in that code to echo the $new_page value. Then see if the value makes sense. I did some basic testing and it worked fine for me. In fact I went back just now tested it both in IE & FF in my XAMPP environment (on Windowz) and then tested again in both IE & FF on my Linux host. No problems. Here is the full code from my test files: changelanguage.php placed at root of site folder: http://www.mysite.com/changelanguage.php) <?php //Get the URL of the referrer page $last_page = $_SERVER['HTTP_REFERER']; //Set the value for the new language - default is german if (isset($_GET['lang']) && ($_GET['lang']=='en' || $_GET['lang']=='de')) { $new_lang = $_GET['lang']; } else { $new_lang = 'de'; } //Set the value of the old language $old_lang = ($new_lang=='en') ? 'de' : 'en'; //Replace the language with the new language in the referrer URL $new_page = str_replace("/{$old_lang}/", "/{$new_lang}/", $last_page); //Redirect to new page header('Location: '.$new_page); ?> English test file: Placed at http://www.mysite.com/en/test.htm <html> <body> <h1>English</h1> <a href="http://www.mysite.com/changelanguage.php?lang=de">Change to German</a><br> <a href="http://www.mysite.com/changelanguage.php?lang=en">Change to English</a> </body> </html> German test file: Placed at http://www.mysite.com/de/test.htm <html> <body> <h1>German</h1> <a href="http://www.mysite.com/changelanguage.php?lang=de">Change to German</a><br> <a href="http://www.mysite.com/changelanguage.php?lang=en">Change to English</a> </body> </html>