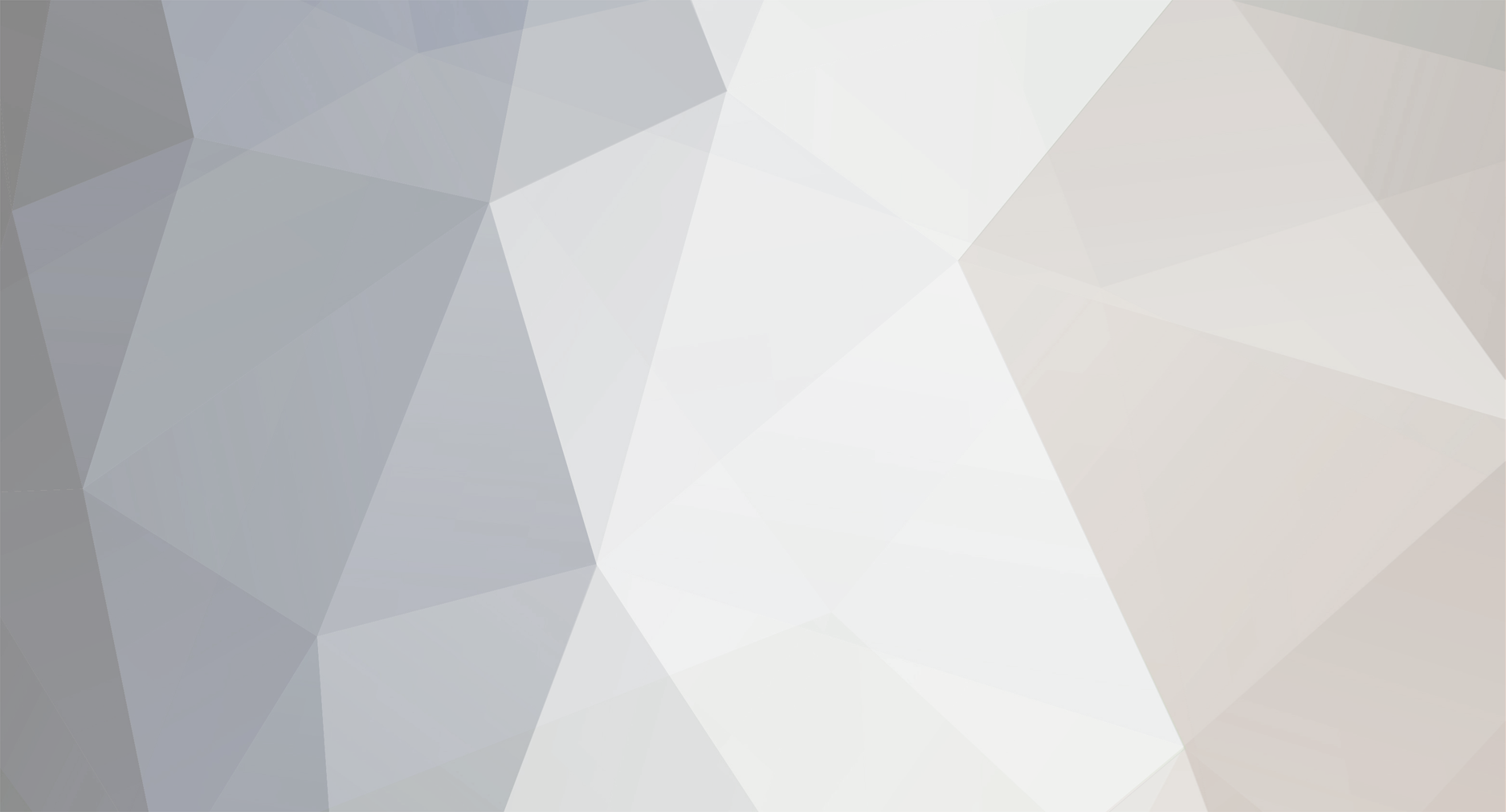
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
<?php //Set this variable to the value in the database //I hard code it here for example purposes $db_date = '2007'; //Create an array of all possible values //Or, if all possible values are stored in a list table from DB, get them there $dates = array('2006', '2007', '2008', '2009'); //Create the radio options $radio_opts = ""; foreach ($dates as $date) { $checked = ($date==$db_date) ? ' checked="checked"' : ''; $radio_opts .= "<input type=\"radio\" name=\"groupname\" value=\"{$date}\"{$checked}> {$date}<br />\n"; } //other code goes here as needed ?> //Form Select the date: <?php echo $radio_opts; ?>
-
You need to redo how you are creating the values. By concatentating the values into single string with a comma at the end, you are making this more difficult than it needs to be. If you explode the values like Mark suggests you will alwaus have an empty value at the end of the array. Create your values in an array to begin with and then just use array_rand() to get a random value out of that array $rr = array(); if(getramdoms(2) != 0) { $rr[] = 1; } if(getramdoms(44) != 0) { $rr[] = 2; } if(getramdoms(99) != 0) { $rr[] = 3; } if(getramdoms(132) != 0) { $rr[] = 4; } $randomnumber = array_rand($rr);
-
And how to you expect to get the values into an array if you don't get them from the database? Why don't we address the real problem of why you are unable to get the values from the database rather than trying to make a workaround when one isn't needed. Let's start with some information on the errors encountered when it "bugs out". Without going into great detail in reading your scripts above it looks like you have a block of code that echo's out the shopping cart contents to the page. Then it appears you have a function for sending the email. But, I don't see how you are passing the requisite vallues to the function or how the function obtains those values (i.e. the cart details). Are you wanting to have the email sent automatically when the page to display the cart is generated or is there some user initiated event that would send the email?
-
Try this: mainArray[type[3]];
-
$new_string = substr($old_string, -4);
-
Those are not multi-select lists. It also works fine for me in FF, so I'm not sure what problem you are having. In any event, I think that code can be improved. 1. There is no need to use JavaScript to populte the main category list since it is not dynamic - just populate it initially. 2. The scripts are too dependant on the data. All the IF statements are looking for specific values. It is better, in my opinion, to separate the data from the logic. The scriopt below is much more flexible in my opinion. You can add additional categories and subcategories without updaing the logic, just add additional arrays for the data. <html> <head> <script> var subcategories = new Array(); subcategories['Fruits'] = new Array( ['Mango', 'Mango'], ['Banana', 'Banana'], ['Orange', 'Orange'] ); subcategories['Games'] = new Array( ['Cricket', 'Cricket'], ['Football', 'Football'], ['Polo', 'Polo'] ); subcategories['Scripts'] = new Array( ['PHP', 'PHP'], ['ASP', 'ASP'], ['Perl', 'Perl'] ); function updateSubCat(categoryObj, subCatSelectID) { var selectedVal = categoryObj.options[categoryObj.selectedIndex].value; var subCatSelectObj = document.getElementById(subCatSelectID); if (subcategories[selectedVal]) { optionList = subcategories[selectedVal]; subCatSelectObj.disabled = false; } else { optionList = [['Select a Category','']]; subCatSelectObj.disabled = true; } //Empty the list & repopulate subCatSelectObj.options.length = 0; addOptionsList(subCatSelectObj, optionList) return; } function addOptionsList(selectObj, optionList) { optLength = optionList.length; for (var i=0; i<optLength; i++) { var optn = document.createElement("option"); optn.text = optionList[i][0]; optn.value = optionList[i][1]; selectObj.options.add(optn); } } </script> </head> <body> <script type="text/javascript" src="templates/khepri/js/list.js"></script> </head> <body id="minwidth-body"> blahblah, lots of other code... <form name="drop_list" action="yourpage.php" method="post" > <select name="Category" onChange="updateSubCat(this, 'SubCat');" > <option value="">Category</option> <option value="Fruits">Fruits</option> <option value="Games">Games</option> <option value="Scripts">Scripts</option> </select> <select id="SubCat" name="SubCat" disabled="disabled"> <option value="">Select a Category</option> </select> </form> </body> </html>
-
What about setTimeout()? He really needs a loop that executes every three seconds as opposed to pausing the execution. <html> <head> <script type="text/javascript"> function countToTen(num) { var ouputObj = document.getElementById('output'); var newLine = num + '=sani<br />'; ouputObj.innerHTML = ouputObj.innerHTML + newLine; if (num<10) { num++; setTimeout('countToTen('+num+')', 3000); } } </script> </head> <body onload=countToTen(0);> <div id="output"></div> </body> </html>
-
Just take the results and dump them into a multidimensional array, then sort the array however you wish. THEN iterrate through the array to echo your results. Also, the arrays you are using for the sectors doesn't make sense. Is there any reason you just dont have one array for those in a format like this: $sectors = array ( 'sector1code' => 'Sector 1', 'sector2code' => 'Sector 2', 'sector3code' => 'Sector 3', 'sector4code' => 'Sector 4' ); Then you don't need all those IF statements. Instead you would have one line to set the $id value like this: $id = $sectors[$key]; Anyway, here is what you can do (although I would suggest a better array for the sector information): <?php $dn = "cn=Unit"; $filter = "(&(objectclass=*)(|(|(|(id=$sector[$key])(id=$sector2[$key])(id=$sector3[$key])(id=$sector4[$key])))))"; $justthese = array('cn','id'); $sr = ldap_search($ds, $dn, $filter, $justthese); $info = ldap_get_entries($ds, $sr); $ldap_results = array(); for ($n=0; $n<$info['count']; $n++) { $user = $info[$n]['cn'][0]; $id = $info[$n]['id'][0]; if ($sector==$sector[$key]) $dist="Sector "; if ($sector==$sector2[$key]) $dist="Sector 2 "; if ($sector==$sector3[$key]) $dist="Sector 3"; if ($sector==$sector4[$key]) $dist="Sector 4"; $ldap_results[] = array ( 'user' => $user, 'sector' => $$sector ); } function sortLDAPResults($a, $b) { if ($a['sector']==$b['sector']) { return 0; } return ($a < $b) ? -1 : 1; } //Sort the resutls usort($ldap_results, 'sortLDAPResults'); //Echo the results foreach ($ldap_results as $ldap_record) { echo " {$ldap_record['sector']} - {$ldap_record['user']}<br>"; } ?>
-
This is actually a very simple problem to solve, which only takes 4 steps 1. Get a cup of coffee or red bull (well, get a lot actually) 2. Using the URL add the letter 'a' as the parameter (or, idealy, start at the lowest character code) 3. Submit the URL and see if a page appears with the expected output 4. Increment the character used (or add another character if att he last character code) then repeat from step 2 (about 1 billion times)
-
Drop Down menu Value should stay selected when selected
Psycho replied to southpawnigeria's topic in PHP Coding Help
Of course there is a reason, but I'm not understanding you. Are you saying that the "Select Company Name" is not included in the select list? If so, I don't see how since it is hard coded - unless there is some JavaScript which manipulates that list. The other possible problem that you are trying to describe may be that the 1st company from the database is not included in the select list. If that is the problem, I suspect you implement the code I provided incorrectly and are running a mysql_fetch of some sort before the loop starts - which would "use up" the first record. In any case a more detailed explanation of the problem and a link to the page or the current code would be helpful. -
Just query all the records first. You can then use mysql_num_rows() to get the count. Also, any reason you are using $query2, $result2. Unless you are still using the previous query and result you are just creating more variables in memory I would also suggest separating the logic from the output. I assumed that there was a unique ID for the property records as well. PHP Logic <?php $query = "SELECT propertyID, PropertyName FROM property WHERE Owner_ID = '$ownerid'"; $result = mysql_query($query); $propertyCount = mysql_num_rows($result); $propertyOptions = ''; while ($row = mysql_fetch_assoc($result) { $propertyOptions = "<option value="{$row['propertyID']}">{$row['propertyname']}</option> "; } ?> Output Total Properties Owned: <?php echo $propertyCount; ?> <br /><br /> Select a property: <select name="property"> <?php echo $propertyOptions; ?> </select>
-
If you "know" PHP then you should know that you do not need to give the array fields a numerical index - PHP will do that for you. Your script should work just fine - although it could use some edits to make it more efficient and secure. Note sure what this is supposed to do if($v) { If you are checking to see if that field has a non empty string value there are better ways to check that. That test would be problematic if the user accidentally entered spaces into that field. Also, you are doing absolutely no validation of the input on the server-side. You should never rely on javascript for validation. It is a nice feature to add for the user, but all validation must be done on the server-side and only optionally added to the client side. //Create an array to hold all the records until the INSERT $records = array(); foreach($_POST['option'] as $key => $option) { //Remove leading/trailing spaces $option = trim($option); $description = trim($_POST['desc'][$key]); //Only process if both fields have a value //Add error handling if you wish if only one has a value if(!empty($option) && !empty($description)) { $_SESSION['option'][$key] = $option; $_SESSION['desc'][$key] = $description; $option_SQL = mysql_real_escape_string(); $description_SQL = mysql_real_escape_string(); $customerID_SQL = mysql_real_escape_string(); //Add new record to records array so only 1 query needs to be run $records[] = "('{$option_SQL}', '{$description_SQL}', '{$customerID_SQL}')" } } //Check if there are any records to add if (count($records)>0) { //Create one query will ALL the records to add $query = "INSERT INTO customer_options (firstName, lastName, customerID) values" . implode(",\n", $records); $result = mysql_query($query) or die (mysql_error()); }
-
I'm not understanding what you want. What exactly isn't working. You start off by saying the script works well and then go into some other details that I'm not making sense of. For example, you state that when the user clicks "OK" that the form is submitted. THat would not be possible with the code you have posted - unless there is a JavaScript error somewhere on the page. The code you posted would redirect the page to another page and not submit the form. You script should be called onsubmit() of the form and would look something like this: Form Tag <form name="formName" onsubmit="return confirmation();" method="POST" action="somepage.php"> function confirmation() { var answer = confirm("Click ok to accept terms and submit form\r\nOtherwise click Cancel to return back to website") if (answer){ return true; } else{ window.location = "index.htm"; return false; } }
-
Return false even if something else wants to return true
Psycho replied to ecopetition's topic in PHP Coding Help
I see a couple possible solutions. 1. Utilize array_merge_recursive() on both arrays and create a new array with the second array overwriting the first array values. But, because of the format of your arrays this can't be done. The values will only overwrite if you are not using numerically based indexes. Your inner most values do not have numerically based indexes, but your outer ones do. So, array_merge_recursive() will create new keys to merge the data. Make your arrays like this and you can use array_merge_recursive() and be done with it. [view_hidden_posts] => Array ( [perm_name_id] => 4 [perm_name] => view_hidden_pages [perm_group] => 3 [perm_id] => 4 [perm_user] => 4 [forum_id] => 2 [perm_value] => 1 ) 2. Iterrate through all key/value pairs in array 2 and overwrite the values in array one. This will only work if the structure for the two arrays is exactly the same. This will need to be a recursive function. Ok, this function seems to work. It will overwrite the original array with new values, so if you need to maintain the original values make a copy before running it. function overwriteArray(&$originalAry, $newAry) { foreach ($newAry as $key => $value) { if (is_array($value)) { overwriteArray($originalAry[$key], $newAry[$key]); } else { $originalAry[$key] = $value; } } } -
Try changing the field names to not have a numerical index. The PHP processing page will give them unique indexes as long as they are named as arrays. The probelm you are facing is that you cannot reference these arrays in Javascript the same way you would in PHP. So, name your fields like this: name="option[]" name="desc[]" Then give this a try (Google XOR operator for more info.) var df = document.form1; for(i=1; i<11; i++) { if (df['option[]'][i].value XOR df['desc[]'][i].value) { alert("Please fill out all required information."); df['option[]'][i].focus(); return false; } }
-
Aslo, you need to modify your INSERT query. You reference the POST values with the field names NOT enclosed in quotes. This, may cause the interpreter to assume you are referencing a static variable as the index instead of the field name: $sql="INSERT INTO articles (title, description, link) VALUES ('{$_POST['title']}', '{$_POST['description']}', '{$_POST['link']}')";
-
This should be all that you need. May need to check that field names are correct for your environment. It basically does a select all names on the 'types' table and then appends a new field to each record ('checked' with a value of 1 or 0) based upon whether there is a match in the 'bugs2' table with the type value matching the name. $query = "SELECT name, IF (name IN (SELECT type FROM bugs2 WHERE id='{$_GET['id']}'),1,0) as checked FROM types ORDER BY name" $result = mysql_query($query) or die(mysql_error()); $checkboxes = ''; while ($checkData = mysql_fetch_assoc($result)) { $checked = ($checkData['checked']==1) ? ' checked="checked"' : ''; $checkboxes .= "<input type="checkbox" name="categories[]" value="{$checkData['name']}"{$checked} />$checkData['name']<br /> "; } echo $checkboxes; EDIT: kickstart's method should work too. Just different ways to accomplish the same thing. I suspect his may be more efficient, but don't know for sure.
-
You are making this way more difficult than it needs to be. Simply do ONE query to get all the optins and add a parameter using JOIN to determine which ones should be checked. Give me a moment and I'll post some code based upon what you currently have. However, based on what you have the 'type' field in the 'bugs2' table would need to match up with the 'name' field in the 'types' table? Is that correct?
-
Drop Down menu Value should stay selected when selected
Psycho replied to southpawnigeria's topic in PHP Coding Help
I suggest separating your logic from your HTML. Makes things much easier, IMO. Also, I would revise the variable naming. The select list is currently named "clients", but it is a list to select ONE client, so it should logically be called "client". That way on the processing page you are referncing "$_POST['client']" which makes more sense since you are only expecting one client. This would go in the head or logic file <?php $client_options = ''; while ($client_row = mysql_fetch_assoc($clients)) { $selected = ($client_row['clientsid'] == $_POST['clients']) ? ' selected="selected"': ''; $client_options .= " <option value=\"{$client_row['clientsid']}\"{$selected}>".ucfirst($client_row['name'])."</option>\n"; } ?> This goes in the bottom of the document or in the output script <select name="clients" class="DEPENDS ON form BEING 2" id="clients"> <option value="">Select Company Name:</option> <?php echo $client_options; ?> </select> -
[SOLVED] Question about sorting multidimensional arrays
Psycho replied to isbhenrylu's topic in PHP Coding Help
If you look at the comparisons and the return values, it should be self explanatory. If the comparison is equal then it returns a 0 - i.e. the values will not be sorted. If a 1 or -1 are returned then the values will be sorted: 1 will sort the first before the second and -1 will do the opposite. Which you return will depend on what direction you want the values sorted. You can also use the function to sort on multiple columns. Let's say you have a multidimensional array in this format: [0] => ( [firstname] => 'John', [lastname] => 'Smith'. [age] => 25 ) Now if you want to sort by age descending, last name ascending, then by first name ascending your function would look like this: sortByAgeName($a, $b) { if ($a['age'] != $b['age']) { return ($a[age] > $b[age]) ? 1 : -1; } else if ($a['lastname'] != $b['lastname']) { return ($a['lastname'] < $b['lastname']) ? 1 : -1; } else if($a['firstname'] != $b['firstname']) { return ($a['lastname'] < $b['lastname']) ? 1 : -1; } return 0; } -
Check what other programs you are running which may be interfering. I know that if I have Skype running Apache will do the same thing - immediately start and then stop.
-
Your question really doesn't make any sense. Declaring a variable and assigning a value are two different things. Are you saying you want to randomly assign a value to those variables from the arrays? If that is the case then a simple function to randomly return a value from an array is all you need. function getRandFromArray(array) { return array[Math.floor(Math.random()*array.length)]; } Then, to assign a random value from the 'dow' array to another variable just do this var randDOW = getRandFromArray(dow); Also, since your arrays are sequentially based starting at 0, a more efficient way to create them would be like so: var dow = new Array('f', 's'); var cdate = new Array ( 'a', 'b', 'c', 'd', 'e', 'f', 'g', 'h', 'i', 'j', 'k', 'l', 'm', 'o', 'p' ); var ctime = new Array('a', 'b', 'c', 'd', 'e', 'f'); var city = new Array( '0', '1', '2', '3', '4', '5', '6', '7', '8', '9', '10', '11', '12', '13', '14', '15', '16', '17', '18', '19', '20', '21', '22', '23', '24', '25', '26', '27', '28', '29', '30', '31', '32', '33', '34', '35', '36' );
-
OK, you need to use nl2br() on your text when echo'ing it to the page. When HTML code is rendered the browser does not interpret a new line character. But, your data is saved with the line breaks. So, just run it through nl2br() and it will convert new line characters into BR tags.
-
Where's your code? Are you saying that the text entered contains spaces and line breaks? If not, then your only resolution is to process any text and dynamically break it when there are "words" which are excessively long. Otherwise, there is no way to "break" it across lines. however, if the entered text does contain spaces and line breaks, something is removing it.
-
Not sure what you are trying to do with the USING() clause, but your usage is incorrect. That clause is supposed to specify multiple columsn - separated by commas - which exist in both tables. Records will be matched where the values of those columns are equal.