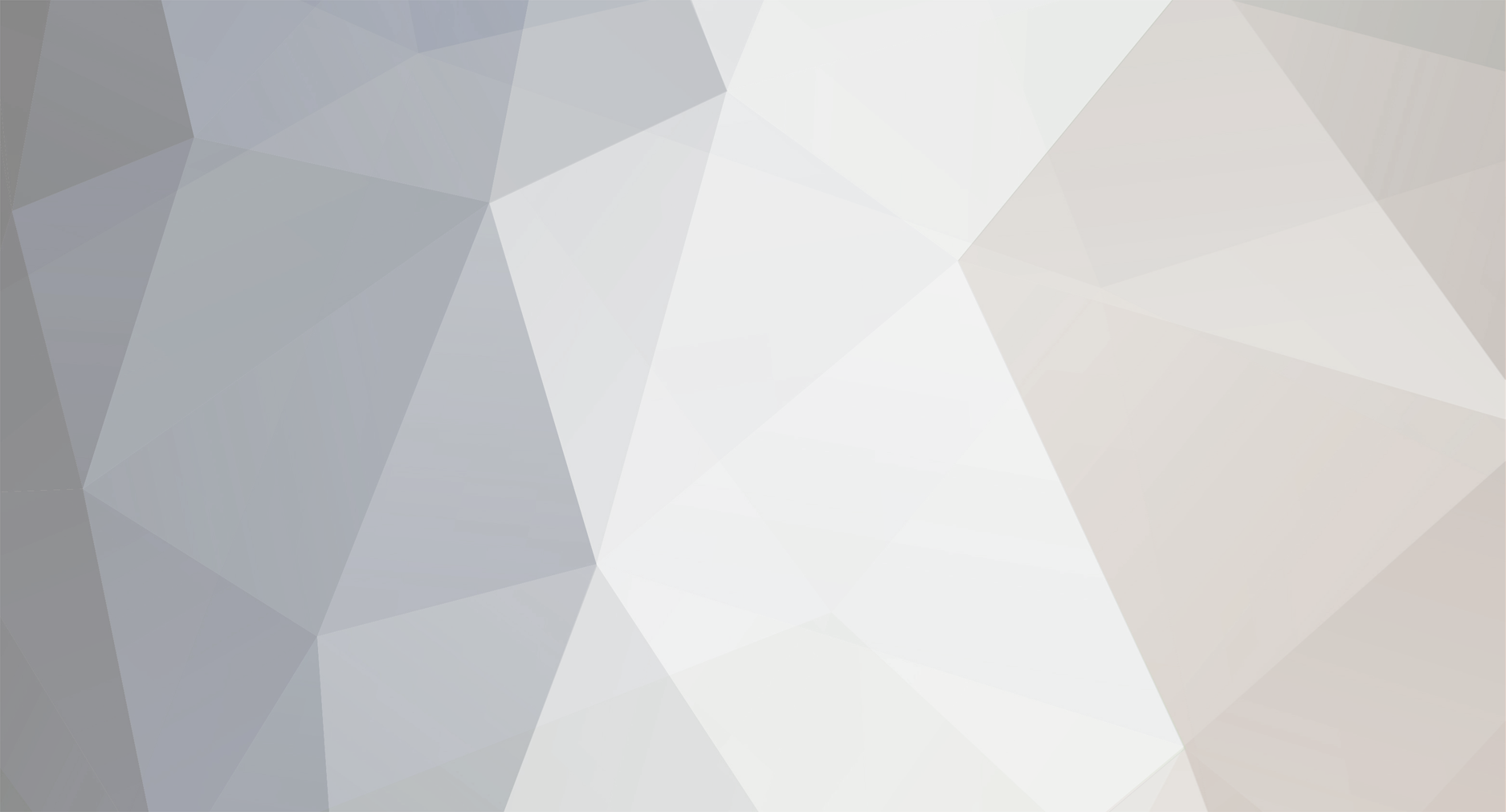
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Well, first off you need to modify your table structures. The reason you use an ID in your tables is so that you can reference that unique piece of data to tie information together. So, your associative table (Credits) only needs to use IDs. Also, there is no need to use an ID on that table. Films table: filmID, filmTitle, filmGenre, FilmLength Credits table: filmID, creditJob, personID Person table: personID, personName, eMail, photo, bio Now let's say you are going to query the data for your film page. you are going to want to query for the credits info on that page as well. While doing so you will want to get the person ID as well to use in the links. Sample code: <?php $query = "SELECT * FROM films JOIN credits ON films.id = credits.filmID JOIN persons ON credits.personID = persons.personID WHERE filmID = 5"; $result = mysql_query($query) or die (mysql_error()); $first = true; while ($film = mysql_fetch_assoc($result)) { if ($first) { $first = false; echo "Title: " . $film['title'] . "<br>"; echo "Genre: " . $film['genre'] . "<br>"; echo "Length: " . $film['length'] . "<br>"; echo "Credist:<br>"; } echo "< a href=\"showperson.php?id=".$film['personID']."\">".$film['personName']."</a><br>"; } ?>
-
AJAX
-
This is not a PHP problem. It is either HTML or CSS. Check the actual output for possible unwanted spaces. Also, check the classes used.
-
Well, I technically misspoke there. The "page" doesn't have to refresh, but the database changes would. AJAX allows you to update part of a page without refreshing the page. How long it takes will depend on many factors. How did you expect JS to update the page with new records without getting new data?
-
AJAX is the key. However there is no way to have the page automatically refresh when a record is updated. Your only option would be to set a javascript timer on the page to check for new data every n seconds.
-
[SOLVED] strikethrough text to normal text (onclick)
Psycho replied to technotool's topic in Javascript Help
Well, you are still not giving enough specifics. I will assume that the values are predetermined and not user entered. You can use the basic script I had above, buit instead of using text fields, just use SPAN tags around each value. Then when the page is submitted iterrate through each SPAN and populate hidden fields with the text that does not have the strike-through property. I would just use a single hidden field for each typ of variable and enter the text comma separated. You can easily parse the values within the PHP code using explode(). Here is another example. <html> <head> <script type="text/javascript"> function strikeText(fieldObj) { var style = (fieldObj.style.textDecoration!='line-through')?'line-through':'none'; fieldObj.style.textDecoration = style; return true; } function updateFormValues() { var n = 1; var places = new Array(); while (document.getElementById('place'+n)) { fieldObj = document.getElementById('place'+n); if (fieldObj.style.textDecoration!='line-through') { places[places.length] = fieldObj.innerHTML; } n++; } document.getElementById('places').value = places.join(); var n = 1; var orders = new Array(); while (document.getElementById('ord'+n)) { fieldObj = document.getElementById('ord'+n); if (fieldObj.style.textDecoration!='line-through') { orders[orders.length] = fieldObj.innerHTML; } n++; } document.getElementById('orders').value = orders.join(); return true; } </script> </head> <body> The woman went to the: <span id="place1" onclick="strikeText(this);">hairstylist</span>, <span id="place2" onclick="strikeText(this);">supermarket</span>, <span id="place3" onclick="strikeText(this);">restaurant</span>. <br><br> The woman ordered: <span id="ord1" onclick="strikeText(this);">salad</span>, <span id="ord2" onclick="strikeText(this);">wine</span>, <span id="ord3" onclick="strikeText(this);">fruit</span>, <span id="ord4" onclick="strikeText(this);">coffee</span>, <span id="ord5" onclick="strikeText(this);">milk</span>, <span id="ord6" onclick="strikeText(this);">tums</span>, <span id="ord7" onclick="strikeText(this);">juice</span>, <span id="ord8" onclick="strikeText(this);">eggs</span>, <span id="ord9" onclick="strikeText(this);">bacon</span>, <span id="ord10" onclick="strikeText(this);">tea</span> <br><br> <b>Assume these are hidden fields. They are populated on submission of the form.<br>You can 'split' them using PHP in the processing page.</b><br> <form name="test" onsubmit="return updateFormValues();" method="POST"> Place: <input type="text" name="places" id="places" value="" size="80"> <br> Orders: <input type="text" name="orders" id="orders" value="" size="80"> <br> <button type="submit">Submit</button> </form> </body> </html> Change the "return true" to a "return false" so you can easily see what is going on when you try to submit the page. -
[SOLVED] strikethrough text to normal text (onclick)
Psycho replied to technotool's topic in Javascript Help
I thought I understood your post at first, but then the aprt that states "If the text is normal when I hit submit it should send it to the php_processing.php file" throws a wrench into things. Are these input fields? If so, what is initiating the onclick event, a button? If he onsubmit is triggered and tehre are fields that have the strike-through property do you want to prevent submission of the form or only "blank out" the fields that have the strikethrough property? Here's a quick example for one interpretation of your request <html> <head> <script type="text/javascript"> function strikeText(fieldID) { fieldObj = document.getElementById(fieldID); var style = (fieldObj.style.textDecoration!='line-through')?'line-through':'none'; fieldObj.style.textDecoration = style; return; } function parseStrikeText(formFields) { for (var i=0; i<formFields.length; i++) { if (formFields[i].style.textDecoration=='line-through') { formFields[i].value=''; } } return true; } </script> </head> <body> <form name="test" action="php_processing.php" onsubmit="return parseStrikeText(this.elements);" method="POST"> Field 1: <input type="text" name="field1" id="field1" value="Field 1 Value"> <button onclick="strikeText('field1')">Strike-through</button> <br> Field 2: <input type="text" name="field1" id="field2" value="Field 1 Value"> <button onclick="strikeText('field2')">Strike-through</button> <br> <button type="submit">Submit</button> </form> </body> </html> -
I didn't supply "corrected" code. I added comments to show why it is not working as you intended. Here is a better way to do this: <?php if (isset($_GET['sort']) && $_GET['sort'] == "newest") { $query = "SELECT id, image, date, date2, name, descr, type, gen, cond, owner FROM ponycol WHERE owner='$g' LIMIT 50 ORDER BY id DESC"; $res = mysql_query($query)or die( mysql_error() ); while( $row = mysql_fetch_row($res) ){ echo "<a href='mlpcollection.php?id=$row[0]'>$row[4]</a><br>"; } } ?>
-
Two things. 1) A better way to limit your results to 50 is to use "LIMIT 50" in your query! Then ther is no need to synthetically limit the results in the PHP code. 2) If you break down the relevent code it shoud be obvious why it is not working as you intend <?php //$offset is not already set so it will be set to 0 if(!$offset) $offset=0; //$recent is set to 0 $recent = 0; $res = mysql_query("SELECT id, image, date, date2, name, descr, type, gen, cond, owner FROM ponycol WHERE owner='$g' ORDER BY id DESC")or die( mysql_error() ); while( $row = mysql_fetch_row($res) ){ //$recent and $offeset never get modified (in the loop) so this will always be true if( $recent >= $offset && $recent < ($offset + 50 )){ echo "<a href='mlpcollection.php?id=$row[0]'>$row[4]</a><br>"; } } //This is outside the loop $recent = $recent + 1;
-
It would only be a security risk if the name isn't properly handled in every place it is used: Queries, HTML display, etc. As long as it is properly escaped for each particular situation, there is no risk.
-
You are using the same variable for two different things <?php while($row1 = mysql_fetch_assoc($res1)){ //Set the variable to the current value $s_names = $row1['service_name']; //Prints the variable print $s_names; //Resets the variable to this new value - but it will get overwritten //on the next pass in the loop on the first line above $s_names .= "<option value='$s_names'>". $s_names . "</option>"; } ?> Try this: <?php while($row1 = mysql_fetch_assoc($res1)){ $s_names .= "<option value=\"" . $row1['service_name'] . "\">". $row1['service_name'] . "</option>"; } print $s_names; ?>
-
It is ALL user input. It makes no difference if it is a text field, a select list, a radio group, etc. Or even data sent on the URL (i.e. $_GET values). A user can create an "offline" form and post it to your page. Never, ever trust any data submitted from a user. You don't state if $ID is getting set properly. Since you don't state that that echo is not displaying the value I will assume it is getting set properly. Also, I don't see where you are running the query. Do you have any error handling when running the query? Try this: <?php $ID = $_GET['id']; echo "Submitted id [{$ID}]<br>"; $query = "SELECT * FROM phoneList WHERE ID= '$ID' "; $result = mysql_query($query) or die (mysql_error()."<br>Query: $query"); $user = mysql_fetch_assoc($result); echo "<br>My firstname is " . $user['Firstname']; echo "<br>My lastname is " . $user['Lastname']; ?> If this does not work, what output do you get?
-
SQL injection is where someone enters code into an input field and then when the page is posted (if the processing page does not prevent it) the code will be run in the query. For example if you had an authorization script such as this: SELECT * FROM users WHERE uname = '$username' and pword = '$password' Now, let's assume the user entered the values "bob" and "a' or 1 = 1". That would result in this query being run SELECT * FROM users WHERE uname = 'bob' and pword = 'a' or 1 = 1 Since 1 always equals 1 it will select ALL the records in the database. Then the code would most likely grab the first erecord (assuming there would have been only 1 match) and authenticate against that user. In most cases the first user account is an admin. By using mysql_real_escape_strings() the text is "escaped" so it will not be interpreted. In the example above, instead of the password being interpreted as code it would be trated as a literal string. I.e. the query would look for a password of "a' or 1 = 1"
-
OK, a few comments first. 1. The third line where you set $ID makes no sense. ID is not one of the values posted and you don't use it anyway. 2. You need to validate/clean ALL values from user input - especially when runing a query on it. Else, someone could use SQL injectyion to do nasty things to your data. 3. You have some invalid HTML that is being built. You first build a tablerow with a 3 column cell, then the first data row has two single cells and then a third with just one single cell??? 4. Perhaps the reason the ID is not getting displayed is due to the 'case' of the variable name. What is the exact name of your id field in the database: Id, id, ID? To see what data is actually being returned try adding "print_r($row)" within your while loop. Here is some modified code to fix issues 1-3. I can't do much about #4 without knowing exactly what your db structure is. <?php # GRAB THE VARIABLES FROM THE FORM $Lastname = mysql_real_escape_string($_POST['Lastname']); $Firstname = mysql_real_escape_string($_POST['Firstname']); //this should select based on form selections $query = "SELECT * FROM phoneList WHERE ID > 0"; if (strlen($Firstname) > 0) { $query .= " AND Firstname='$Firstname'"; } if (strlen($Lastname) > 0) { $query .= " AND Lastname='$Lastname'"; } $result = mysql_query($query) or die ("There was an error:<br />".mysql_error()."<br />Query: $query"); if(!mysql_num_rows($result)) //if none!!! { echo "There were no rows returned from the database"; } else //otherwise draw the table { //put info on new lines echo "<table cellspacing=\"15\">\n"; while($row = mysql_fetch_assoc($result)) { extract($row); echo "<tr><td>"; echo "$Firstname $Lastname<br />"; echo "$Email<br />"; echo "$Phone<br />"; echo "<a href=\"showdetails.php?id=$id\">View Details</a>"; echo "</td></tr>\n"; } } echo "</table>\n"; ?>
-
That code is pretty strit forward. There is an element ont he page with the name 'hiddenvalue' (I'm assuming it is a hidden input field). That code is setting the value of that field to the src attribute value of the element with the name 'main'. Assuming these are the two elements <nput type="hidden" name="hiddenvalue"> <img name="main" src="main_image.jpg"> Then the field 'hiddenvalue' will have the value of 'main_image.jpg'
-
I see. So what are you using these for? If you are creating scripts to do something on your PC there are much better scripting languages for that purpose than JS. Even so, you could still start the initial js file using an HTML page.
-
Passing username is not a good idea IMHO. Because username may contain many different characters that don't work and play well when included in a URL. Since he is create a list page of "known" records, the error handling would only kickin if 1) a record was deleted after the user generated the list page and before they clicked the link or 2) the user manually modifies the URL to include an invalid id. Both of which would rarely happen. Also, the OP only stated that the records had a firstname and lastname. This could be a database for a mailing list or contacts. I did not make the assumption that there is even a username. In any case, the whole point of having a unique record ID is to use it for instances such as this. For example, look at the URL of this page and you will see "topic,196946". That is the ID for the record of this topic. You wouldn't want to pass the title of the topic as the parameter. If anything, I would agree that the error handling could be modified to produce differenet, targeted messages. E.g.; No user ID submitted, unable to find user record, etc.
-
It works for me. Here are the three test files I created: HTML File: <html> <head> <script type="text/javascript" src="library.js"></script> </head> <body> Testing dynamically loaded JS </body> </html> Librabry JS File function include(file) { var script = document.createElement('script'); script.src = file; script.type = 'text/javascript'; script.defer = true; document.getElementsByTagName('head').item(0).appendChild(script); } include('include1.js'); Dynamically included JS file alert('Loaded JS 1');
-
OK, let's say you have a page called "showdetails.php" that will act as you display page. On your listing page when you create the links, create the links something like this: <a href="showdetails.php?id=5">Bob</a> Where 5 is the ID for the person Bob. Then on the "showdetails.php" page you will access the ID through $_GET['id'] and then just query for that users data from the database and display it however you want. Example $userID = (int) $_GET['id']; $query = "SELECT * FROM users WHERE id = $userID"; $result = mysql_query($query) or die (mysql_error()."<br />Query: $query"); if (mysql_num_rows($result)==0) { echo "No user found for id '$userID'"; } else { $user = mysql_fetch_assoc($result); echo "Name: {$user['name']}<br />"; echo "Address: {$user['address']}<br />"; //etc... }
-
I'm sorry, did you mean to say "It's now working"? If not, then what is happening? Show us the code you currently have.
-
Not to be rude, but it would take you about 2 minutes to verify that for yourself. As I stated in my first post you just need to use the DOM to dynamically "add" a script tag. That's what the author of that code states it does. Since it is entirely JS you can add it in any javascript you want: in the head, inline or in an external file.
-
Um, use a correct integer value? Seriously though, you will need to post some code for us to be of any help. Most likely your query is screwed up. You should create your query as a variable so you can echo it to the page for debugging purposes.
-
If I may, abetter alternative is to use "return someFunction()" within the onsubmit trigger. That way the validation script only returns true/false. This allows you to make your code more transportable. You aslo don't state whether the value must be numeric OR if it can only be digits (e.g. 1.2 is a numeric value, but is not all numbers). You aslo don't state if the value can be empty. I also prefer to have a validatin script that then calls sub-functions for each type of validation. That way you can easily run the same validation on multiple values without rewriting a lot of code. <form name="foo" onsubmit="return checkForm();"> <input type="text" size="8" name="num" /> <input type="submit" /> </form> <script language="javascript" type="text/javascript"> function isNum(value) { //Validate that it is not NULL and is all number characters return (value && !value.match(/[^0-9]/)); //Validate that it is not NULL and is a numeric value // return (value && !isNaN(value)); } function checkForm(value) { //Check each field var value = document.foo.num.value; if (!isNum(value)) { alert('value must be a number'); document.foo.num.focus(); document.foo.num.select(); return false; } //Add additional validations as needed //Return false if any fail //If all validations pass return true return true; } </script>
-
Actually, having JS in a page DOES prompt the message However, that message only appears for JavaScript content when you are running that file directly on your computer. If you are accessing that webpage through an actual web server you will not get that alert (for Javascript content). So, there should be no need to include a work-around as your users will never see it. Besides, I know of no workaround for that anyway.
-
Sounds like a job for DOM. I had a project once where I needed to swap out "included" js code on-the-fly and did something like this: <script type="text/javascript" src="library.js" id="JS_include"></script> function changeJS(includeFile) { document.getElementById(JS_include).src = includeFile; } You should be able to "add" javascript includes through the DOM and set their src attribute, but my DOM skills are not the best. EDIT: I was correct. Here is a page with the instructions for doing just that: http://www.chapter31.com/2006/12/07/including-js-files-from-within-js-files/ Here is the JS code from that page //this function includes all necessary js files for the application function include(file) { var script = document.createElement('script'); script.src = file; script.type = 'text/javascript'; script.defer = true; document.getElementsByTagName('head').item(0).appendChild(script); } /* include any js files here */ include('js/myFile1.js'); include('js/myFile2.js');