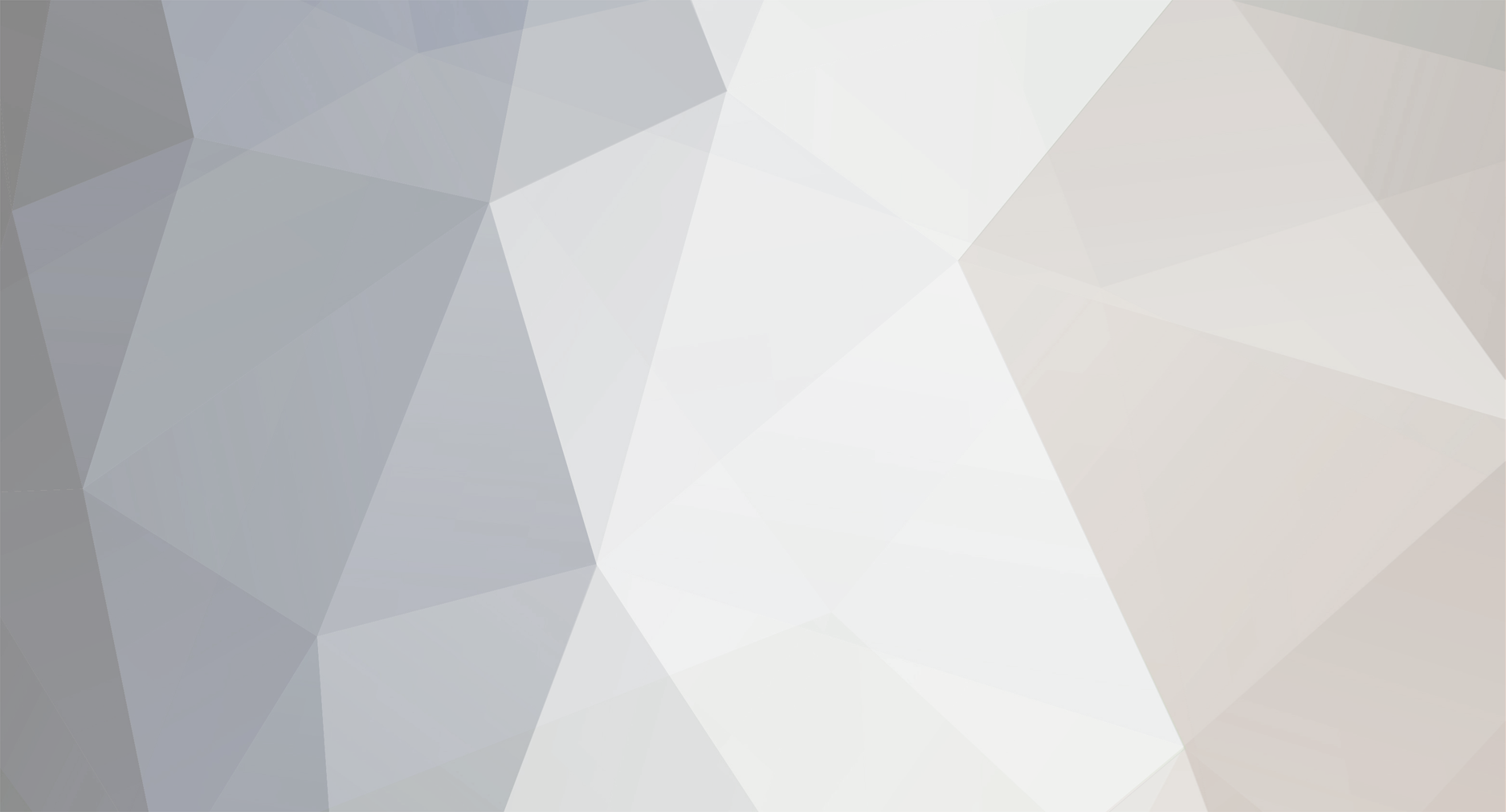
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Well, you can't use a space in a URL so your proposal is out of the question (you have to use %20 to represent a space). I'm also curious - are you really expecting user's to type that information into a URL (Me being a bad speller would probably type "Mathew" instead of "Matthew" and get no results)? Are you not going to provide some sort of user interface for the user to get the data they want?
-
Here is some mock code based upon a script I have and the XML schema you provided. It is definitely not a complete script but hopefully it is enough for you to get started: <?php //Load the XML file $xml_data = simplexml_load_file('xmldata\data.xml'); $data_output = array(); //Iterrate through each product foreach ($xml_data->product as $product_data) { $this_record = array(); //Read and VALIDATE each field (add your own validation) $this_record['result'] = (string)$product_data->result; $this_record['responsedatetime'] = (string)$product_data->responsedatetime; $this_record['stockcode'] = (string)$product_data->stockcode; $this_record['description'] = (string)$product_data->description; //Etc for each needed field //Add any necessary validation for each //field so you don't INSERT a bad record //Assuming all validation passed for the current record $data_output[] = "('" . implode("', '", $this_record) . "')"; } //Use the $data_output array to create a single query to insert all records $values = implode(", ", $data_output); $query = "INSERT INTO products (result, responsedatetime, stockcode, description) VALUES " . $values; $result = mysql_query($query) or die (mysql_error()); ?>
-
There is no one-size-fits-all script that I know of. Mostly because each situation is different. Please provide some more details of the data that will exist in the XML file and how you want it parsed and inserted intot he database and we can provide more assistance.
-
This is NOT a PHP problem. this is an HTML and/or CSS problem. I would suggest building your forms in ONLY HTML/CSS. Once they look correct, then incorporate the PHP code needed for the variability and functionality needed. however, the easiest solution to your problem would be to use a table. (although many would suggest that CS is the proper solution). I also see no need for each field label to state "Enter your...". That's kind of implied by the fact that you have a form, is it not? Here's a quick rewrite: <form method="post" action="register1submit.php" > <table> <tr><td>First name:</td><td><input type="text" name="first" /></td></tr> <tr><td>Last name:</td><td><input type="text" name="last" /></td></tr> <tr><td>Last six digits of your Student ID:</td><td><input type="text" name="id" maxlength="6" /></td></tr> <tr><td>Password (minimum 6 characters):</td><td><input type="password" name="pswd1" /></td></tr> <tr><td>Re-enter password:</td><td><input type="password" name="pswd2" /></td></tr> <tr> <td>BRANCH</td> <td> <select name="branch"> <option value=""></option> <option value="CS">Computer Science</option> <option value="IT">Information and Technology</option> <option value="EC">Electronics and Communication</option> <option value="EI">Electronics and Instrumentation</option> </select> </td> </tr> <tr> <td>Semester</td> <td> <select name="semester"> <option value=""></option> <option value="1">1st</option> <option value="2">2nd</option> <option value="3">3rd</option> <option value="4">4th</option> <option value="5">5th</option> <option value="6">6th</option> <option value="7">7th</option> <option value="8">8th</option> </select> </td> </tr> <tr> <td colspan="2"> <font style=" color:#FFFFFF; font-family:Comic Sans MS, Georgia; font-size: 18px">PERSONAL DETAILS</font> </td> </tr> <tr><td>Father's Name:</td><td><input type="text" name="fname" /></td></tr> <tr><td>Mother's Name:</td><td><input type="text" name="mname" /></td></tr> <tr><td>Address Line 1:</td><td><input type="text" name="add1" /></td></tr> <tr><td>Address Line 2:</td><td><input type="text" name="add2" /></td></tr> <tr> <td>City</td> <td> <select name="city"> <option value="Anuppur">Anuppur</option> <option value="Ashoknagar">Ashoknagar</option> <option value="Balaghat">Balaghat</option> <option value="Barwani">Barwani</option> <option value="Betul">Betul</option> <option value="Bhind">Bhind</option> <option value="Bhopal">Bhopal</option> <option value="Burhanpur">Burhanpur</option> <option value="Chhatarpur">Chhatarpur</option> <option value="Chhindwara">Chhindwara</option> <option value="Damoh">Damoh</option> <option value="Datia">Datia</option> <option value="Dhar">Dhar</option> <option value="Dindori">Dindori</option> <option value="Guna">Guna</option> <option value="Gwalior">Gwalior</option> <option value="Harda">Harda</option> <option value="Hoshangabad">Hoshangabad</option> <option value="Indore">Indore</option> <option value="Jabalpur">Jabalpur</option> <option value="Jhabua">Jhabua</option> <option value="Katni">Katni</option> <option value="Khandwa">Khandwa</option> <option value="Khargone">Khargone</option> <option value="Mandsaur">Mandsaur</option> <option value="Morena">Morena</option> <option value="Narsinghpur">Narsinghpur</option> <option value="Neemuch">Neemuch</option> <option value="Panna">Panna</option> <option value="Raisen">Raisen</option> <option value="Rajgarh">Rajgarh</option> <option value="Rewa">Rewa</option> <option value="Sehore">Sehore</option> <option value="Seoni">Seoni</option> <option value="Shahdol">Shahdol</option> <option value="Sheopur">Sheopur</option> <option value="Shivpuri">Shivpuri</option> <option value="Sidhi">Sidhi</option> <option value="Singrauli">Singrauli</option> <option value="Tikamgarh">Tikamgarh</option> <option value="Umaria">Umaria</option> <option value="Vidisha">Vidisha</option> </select> </td> </tr> <tr><td>State:</td><td><input type="text" name="state" /></td></tr> <tr><td>Pin Code:</td><td><input type="text" name="pin" /></td></tr> <tr><td>Contact Number:</td><td><input type="text" name="no" /></td></tr> <tr><td>Email address:</td><td><input type="text" name="email"></td></tr> <tr><td align="center" colspan="2"><input type="submit" name="submit" value="submit"></td></tr> </table>
-
Sorry, I thought that first bit of code was from your previous post. Well, you just need to trace what is going on. The first thing I would do would be to put this code right before that IF statement: echo "<pre>"; print_r($_POST); echo "<pre>"; die(); Do the values make sense to what you expect? Is 'username' one of the values? Take a look at that output and then determine what to check next. Is there any chance the very first page the user is presented to log in is NOT rmslogin.php?
-
Is it really that difficult to understand? In rmsloginverify.php you have these lines: $sql="SELECT * FROM user WHERE username='$username' and password='$userpassword'"; $result=mysql_query($sql); if (mysql_num_rows($result)==0) { $_SESSION['invalid']='invalid'; header("Location:rmslogin.php"); } So if the username & password combination doesn't exist in the table a seesion variable is set and the user is redirected to rmslogin.php In the rmslogin.php page you have these lines: if (isset($_SESSION['invalid'])) { Print '<h2>The username or password you entered was incorrect Please try again</h2>'; } Which detects if that session value has been set (thereby indicating a failed login) and displays an appropriate error message. By the way, don't double post.
-
Well, I'm not sure how may verses there are in the Bible, but using a text file for this purposes seems very inefficient. I would suggest using a database. You could create a script that would read the file one time and create all the records needed. That one-time process would be similar to reading the file each and every time. But, getting the results from a database would be much faster. In any case I would suggest using file() which reads the text file as an array with each line of the text file as an array element. You can them loop through the array and explode each line using the tab character (I assume that is the delimeter) and search for the appropriate values. You will also need to have a lookup list to translate the book name to the book number. For example <?php $books = array ( '010' = 'Genesis', '020' = 'Exodus', /// etc. ); ?> (not tested) <?php //Lookup values $bk_lu = $_GET['book']; $ch_lu = $_GET['chapter']; $vs_lu = $_GET['verse']; $lang = $_GET['ver']; //Read file into array $verses = file($lang . '.txt'); foreach ($verses as $verse_data) { list($bk_no, $ch_no, $vs_no, $text) = $verse_data; if ($books[$bk_no]==$book_lu && $ch_no==$ch_lu && $vs_no==$vs_lu) { echo trim($text); break; } } ?>
-
Sorry, been out for a bit. OK, the problem was that I mixed up PHP and JavaScript variable conventions. I used $password (withthe dollar sign) in one instance of the javascript variables. In addition to that fix, I added the additional check for the proper domain on the email address. <script type="text/javascript"> function validate() { var email = document.getElementById("userEmail"); if (!validEmail(email.value)) { alert("Please Insert a Valid Email Address"); email.focus(); return false; } var firstname = document.getElementById("userFName"); if (!firstname.value) { alert("Please Insert a Valid First Name"); firstname.focus(); return false; } var surname = document.getElementById("userSName"); if (!surname.value) { window.alert("Please Insert a Valid Surname"); surname.focus(); return false; } var password = document.getElementById("userPassword"); if (!password.value || password.value.length<6) //Use minimum length here { alert("Please Insert a Valid Password of 6 characters or more."); password.focus(); return false; } var passwordconfirm = document.getElementById("userConfirmPassword"); if (password.value != passwordconfirm.value) { alert("Passwords do not match!"); password.focus(); return false; } return true; } function validEmail(emailStr) { validEmailRegEx = /^[\w\+-]+([\.]?[\w\+-])*@hope.ac.uk$/ return emailStr.match(validEmailRegEx); } </script>
-
That sounds about right. Although I don't understand your comment " I will have to mess about with swfobject so it places the flash in a div in another frame". Weren't you already placing the flash object in another frame? If you are running the AJAX request from one frame and using it to populate the Flash in another, you may need to get a little creative. A quick liik at the SWFObject documentation shows that you indicate the DIV to place the content in using a line like this: so.write("flashcontent"); So, you can't indicate a div in another frame. What you cn do is have teh AJAX function in one frame and then use the return data and call a custom function in the other frame which will then run all the necessary code "locally" within that frame. FRAME 1 - Make AJAX call and get return data - Call function in FRAME 2 passing the return data as a parameter FRAME 2 - Function1 takes the AJAX return data and parses it into the individual elements (Flash object and parameters for the SWFObject) - Function 1 writes the Flash object to a div - Function 1 calls Function 2 (in the same frame) and passes the SWFObject parameters - Function 2 will run the code for SWFObject Example for function 2 (assuming the flash file, heihgt & width are the only variable elements) function setSWFObject(swfFile, width, height) { var fo = new FlashObject(swfFile, "movie", width, height, "7", ""); fo.addParam("allowScriptAccess", "sameDomain"); fo.addParam("quality", "high"); fo.addParam("FlashVars", "playlistfile=config/PlayData.php?id=198&configurationfile=config/configuration.xml"); fo.addParam("wmode", "transparent"); fo.write("flashPlayer"); } EDIT: You can cll a function in another frame, but I haven't done that in a while and don't remember the correct method of making the call.
-
Well, there's a couple of problems. 1) The isEmpty funtion is written inside the formValidator function. While this will work, it is inefficient as the function is recreated each time formValidator is called. 2) When you do call isEmpty() with an IF statement you have no action - so nothing that the isEmpty function returns is returned to the FORM object (i.e. the form will submit anyway. 3) The isEmpty function returns true is the field is empty - so even if that return value was returned to the form, the form would submit if validation failed and not submit if it passed. Try this: <script type="text/javascript"> function formValidator(){ // Make quick references to our fields var field1 = document.getElementById('field1'); var field2 = document.getElementById('field2'); //Check each field and return false if invalid if (isEmpty(field1, 'Please enter')) return false; if (isEmpty(field2, 'Please enter 1')) return false; return true; } function isEmpty(elem, helperMsg){ if(elem.value.length == 0){ elem.focus(); alert(helperMsg); return true; } return false; } </script>
-
No confusion, I understood the alert was just an example. The process I described above is still valid. Create a function on the page to run the swfovject script, but create it to accept parameters for whatever data will be variable. Then in the data that is created from the AJAX request add those parameters using a delimeter. Then the JS that handles the AJAX return value can parse the return value and call the function with those parameters. In fat, I would create a single function that would take the return value and 1) parse the data, 2) Populate the div and 3) Run the swfovject code.
-
Ah, but leap year doesn't occur every four years. It occurs every four years EXCEPT on any year that is divisible by 100 (unless it is also divisible by 400). 2000 = Leap year 2100 = Not a leap year 2200 = Not a leap year 2300 = Not a leap year 2400 = Leap year Anyway, here is some code for ya <html> <head> <script type="text/javascript"> var monthDays = [31, 28, 31, 30, 31, 30, 31, 31, 30, 31, 30, 31]; function changeDays() { var year = parseInt(document.getElementById('year').value); var month = parseInt(document.getElementById('month').value); var day = document.getElementById('day'); //Determine number of days in the month if (month==2 && (year%4==0 && (year%100!=0 || year%400==0))) { //Feb in a leap year var daysInMonth = 29; } else { var daysInMonth = monthDays[month-1]; } //Add days (if needed) while (daysInMonth>day.length) { day.options[day.length] = new Option((day.length+1), (day.length+1)); } //remove days (if needed) day.length = daysInMonth; return; } </script> </head> <body onload="changeDays();"> Month: <select id="month" name="month" onchange="changeDays()"> <option value="1">1</option> <option value="2">2</option> <option value="3">3</option> <option value="4">4</option> <option value="5">5</option> <option value="6">6</option> <option value="7">7</option> <option value="8">8</option> <option value="9">9</option> <option value="10">10</option> <option value="11">11</option> <option value="12">12</option> </select> Day: <select id="day" name="day"></select> Year: <select id="year" name="year" onchange="changeDays()"> <option value="2000">2000</option> <option value="2001">2001</option> <option value="2002">2002</option> <option value="2003">2003</option> <option value="2004">2004</option> <option value="2005">2005</option> <option value="2006">2006</option> <option value="2007">2007</option> <option value="2008">2008</option> <option value="2009">2009</option> <option value="2010">2010</option> </select><br> </body> </html>
-
I don't think that is a good process. Since you are populating the text via JavaScript, just run that alert (or function or whatever) within the code that receives/populates the data. One trick would be to use a delimeter to separate the text from the javascript you want to act upon. Example: <b>Hello World|hi</b> Then just use the "|" to split the data. Use the first part to populate the div and the second part to use in the alert (or for whatever purpose you need)
-
[SOLVED] Programatically setting a javascript event with an argument
Psycho replied to Goose's topic in Javascript Help
I don't know that you can do that. But, I can think of two solutions that are similar (except where you set the "random" text). Both solutions involve creating a custom attribute for the object and setting the value of that attribute to the "random text". Then in the function showTooltip you don't need to pass it the text - you can access the appropriate text with the attribute, such as "this.getAttribute('tooltip')". Option 1 - Set the attribute when creating the event handlers ... obj.onmouseover = showTooltip('Some random text.'); obj.onmouseout = hideTooltip; obj.tooltip='Some random text.'; ... Option2 - set the attribute in the field itself <div tooltip="Some random text">This is the object</div> -
Or even better create the query as a variable so you can echo it out to the page if it fails! $query = "INSERT INTO tmp_test VALUES ('{$car->color}','{$car->year}','{$car->price}','{$car->model}','{$car->company}');"; $res = mysql_query($query, $hd) or die ("Unable to run cars insert query:<br />$query<br />Error:<br />".mysql_error());
-
As Barand stated, the fact that you are not normalising your data is the issue. When there are many-to-one associations you shoud have a secondary table. I.e. there shoudl be a "ReqItem" table with the records haing an id tying them to the record in the "quests" table. However, assuming you still want to do things the wrong way, you could at least create a loop to do the queries for you. <?php for ($i=1; $i<=6; $i++) { if ($i <=4) { $query = "UPDATE quests SET ReqItemId{$i}='$last' where ReqItemId{$i}='$items[entry]'" mysql_query($query) or die (mysql_error()."<br />on query:<br >$query"); $query = "UPDATE quests SET RewItemId{$i}='$last' where RewItemId{$i}='$items[entry]'" mysql_query($query) or die (mysql_error()."<br />on query:<br >$query"); } $query = "UPDATE quests SET RewChoiceItemId{$i}='$last' where RewChoiceItemId{$i}='$items[entry]'" mysql_query($query) or die (mysql_error()."<br />on query:<br >$query"); } ?>
-
I could probably do this in several fewer lines using a one line regular expression, but my brain can't process regEx this late at night. I think this is a little more elegant. MORE IMPORTANTLY: The code in the previous two posts will not calculate correctly for abourt 1/2 the dates (8/22/2001 being one of them due to daylight savings going back for the fall equinox). because the date you used to create the original timestamp has no time it is assumed to be midnight. With 8/22/2001 if you add 24 hours x 180 days it will only take you to 10PM on the 179th day. I added four hours as a buffer to account for that. var dateStr = "08/22/2001"; var millis = Date.parse(dateStr); var newDate = new Date(); //Create new date object +180 days & // +4 hours so daylight savings won't mess it up newDate.setTime(millis+(180*24*60*60*1000)+(4*60*60*1000)); //Create string objects of month & day with 0 appended //Then take jsut the last two characters var month = String('0'+(newDate.getMonth()+1)); month = month.substr((month.length-2), 2); var day = String('0'+newDate.getDate()); day = day.substr((day.length-2), 2); var year = String(newDate.getFullYear()); //Create new date string var newDateStr = month+'/'+day+'/'+year; alert(newDateStr);
-
[SOLVED] Java Dropdown Population Code Change
Psycho replied to mikemessiah's topic in Javascript Help
Made some major changes for your situation. Should be working now. I would suggest pullin all the Javascript code for the select boxes into an external file to make editing easier later on <html> <head> <script type="text/javascript"> function getProvinceList(country) { provinceList = ['-- Any --']; switch (country) { case 'Namibia': provinceList = [ 'Any','Academia / Pioneers Park / Hochland','Auasblick / Olympia','Bethanie','Caprivi Region','Gobabis','Henties Bay', 'Karibib','Keetmanshoop','lein Windhoek / Eros / Avis / Ludwigsdorf','Kleine Kuppe / Cimbabasia / Prosperitas / Rocky Crest', 'Langstrand / Dolfynstrand','Luderitz','Luxury hill / Suiderhof / Windhoek West','Maltahohe','Hardap Region','Monte Christo', 'Okahandja / Okahandja District','Omaruru','Ondangwa','Oshakati','Otjiwarongo','Otjiwarongo District','Rundu','Swakopmund', 'Tsumeb','Walvis Bay','Windhoek Central','Windhoek District']; break; case 'South Africa': provinceList = ['Northern Cape','Western Cape','Eastern Cape','Free State','North West','Gauteng','KZN','Mpumalanga','Limpopo']; break; } return provinceList; } function getCityList(country, province) { cityList = ['-- Any --']; switch (country) { case 'South Africa': switch (province) { case 'Northern Cape': cityList = [ 'Any','Tswala','Kuruman','Augrabies Falls','Upington','Kakamas','Pofadder','Kimberly','Alexander Bay', 'Okiep','Springbok','Niewoudtville','Garies','Calvinia','Hopetown','De Aar','Colesburg','Hanover']; break; case 'Western Cape': cityList = [ 'Cape Flats','Cape Town City Bowl and Atlantic Seaboard','Cape Town Northern Suburbs','Garden Route', 'Great Karoo','Milnerton','Tableview and Blaauwberg','Overberg','Peninsula (Simons Town to Hout Bay)', 'Somerset West and Boland','Southern Suburbs (Tokai to Pinelands)','West Coast']; break; case 'Eastern Cape': cityList = [ 'East Cape Interior','East London, Eastern Karoo','EC Jeffreys Bay to Tsitsikamma','EC Wild Coast', 'Grahamstown and Surrounds','Port Alfred and Coastal Towns','Port Elizabeth']; break; case 'Free State': cityList = [ 'Bethlehem / Harrismith / Clarence & Region','Bloemfontein & Central Freestate', 'Ficksburg / Clocolan / Rosendal & Region','Vaalriver / Parys & Region', 'Vaaltriangle / Vaalpark / Sasolburg','Welkom / Goudveld & Region']; break; case 'North West': cityList = [ 'Carltonville','Derby','Fochville','Groot Marico','Hartbeesfontein','Klerksdorp','Koster','Lichtenburg', 'Mafikeng','Makwassie','Northam','Orkney','Potchefstroom','Rustenburg','Sannieshof','Schweizer Reyneke', 'Stilfontein','Swartruggens','Ventersdorp','Vredefort','Vryburg','Wolmaranstad','Zeerust']; break; case 'Gauteng': cityList = [ 'Alberton Southern suburbs','Benoni','Boksburg','Brakpan','Centurion','Edenvale','Germiston', 'Johannesburg CBD and Bruma','Kempton Park','Midrand','Nigel & Heidelberg','Northcliff and Melville (West)', 'Northern Pretoria','Pretoria Central and Old East','Pretoria East','Randburg and Ferndale (North West)', 'Roodepoort (Far West) and Krugersdorp','Rosebank and Parktown (Central)','Sandton and Bryanston (North)', 'Soweto','Springs','Sunninghill','Lonehill and Fourways','Vereeniging','Vanderbijlpark and Vaal Dam','Wynburg (East)']; break; case 'KZN': cityList = [ 'Dolphin Coast','Durban Central North and CBD','Durban Central South','Durban North','Durban South', 'Durban Upper Highway','Durban West / Highway','KZN Midlands','KZN North Coast','KZN South Coast','Richards Bay']; break; case 'Mpumalanga': cityList = [ 'Barberton', 'Belfast', 'Bethal', 'Burgersfort', 'Carolina / Badplaas', 'Delmas', 'Dullstroom', 'Ermelo / Chrissiesmeer', 'Graskop', 'Groblersdal', 'Hazyview', 'Hendrina', 'Kinross', 'Komatipoort', 'Lydenburg', 'Machadodorp', 'Malelane / Hectorspruit', 'Marloth Park', 'Middelburg', 'Nelspruit', 'Ohrigstad', 'PietRetief / Volksrust / Wakkerstroom', 'Rietkruil', 'Sabie', 'Secunda', 'Standerton', 'Sundra', 'Trichardt', 'Waterval Boven / Onder', 'White River', 'Witbank']; break; case 'Limpopo': cityList = [ 'Bela-Bela (Warmbaths) / Rooiberg, Hoedspruit','Makhado (Louis Trichardt) / Musina, Modimole (Nylstroom)', 'Mokopane (Potgietersrus), Mookgophong (Naboomspruit)','Phalaborwa','Polokwane (Pietersburg)', 'Thabazimbi / Lephalale (Ellisras)','Tzaneen / Duiwelskloof / Gravelotte','Vaalwater','Welgelegen']; break; case 'Mpumalanga': cityList = []; break; } break; } return cityList; } function getTownList(country, province, city) { townList = ['-- Any --']; switch (country) { case 'South Africa': switch (province) { case 'KZN': switch (city) { case 'Durban Central South': townList = ['Town1','Town2','Town3']; break; case 'Durban North': townList = ['Town1','Town2','Town3']; break; case 'Durban South': townList = ['Town1','Town2','Town3']; break; case 'Durban Upper Highway': townList = ['Town1','Town2','Town3']; break; case 'Durban West / Highway': townList = ['Town1','Town2','Town3']; break; case 'KZN Midlands': townList = ['Town1','Town2','Town3']; break; case 'KZN North Coast': townList = ['Town1','Town2','Town3']; break; case 'KZN South Coast': townList = ['Town1','Town2','Town3']; break; case 'Richards Bay': townList = ['Town1','Town2','Town3']; break; } break; } break; } return townList; } function setProvince(){ cntrySel = document.getElementById('country'); prvncSel = document.getElementById('province'); prvncList = getProvinceList(cntrySel.value); changeSelect(prvncSel, prvncList); setCities(); } function setCities(){ cntrySel = document.getElementById('country'); prvncSel = document.getElementById('province'); citySel = document.getElementById('city'); cityList = getCityList(cntrySel.value, prvncSel.value); changeSelect(citySel, cityList); } function setTowns(){ cntrySel = document.getElementById('country'); prvncSel = document.getElementById('province'); citySel = document.getElementById('city'); townSel = document.getElementById('town'); townList = getTownList(cntrySel.value, prvncSel.value, citySel.value); changeSelect(townSel, townList); } //**************************************************************************// // FUNCTION changeSelect(fieldObj, valuesAry, [optTextAry], [selectedVal]) // //**************************************************************************// function changeSelect(fieldObj, valuesAry, optTextAry, selectedValue) { //Clear the select list fieldObj.options.length = 0; //Set the option text to the values if not passed optTextAry = (optTextAry)?optTextAry:valuesAry; //Itterate through the list and create the options for (i in valuesAry) { selectFlag = (selectedValue && selectedValue==valuesAry[i])?true:false; fieldObj.options[fieldObj.length] = new Option(optTextAry[i], valuesAry[i], false, selectFlag); } } </script> </head> <body onLoad="setProvince();"> <form name="test" method="POST" action="processingpage.php"> <label>Country:</label> <select name="country" id="country" onChange="setProvince();"> <option value="Italy">Italy</option> <option value="Namibia">Namibia</option> <option value="South Africa" selected>South Africa</option> </select> <br> <label>Province:</label> <select name="province" id="province" onChange="setCities();"> <option value="" selected>-- Any --</option> </select> <br> <label>City:</label> <select name="city" id="city" onChange="setTowns();"> <option value="" selected>-- Any --</option> </select> <br> <label>Town:</label> <select name="town" id="town" > <option value="" selected>-- Any --</option> </select> </form> </body> </html> -
Here, this uses sessions and the code is much cleaner. <?php session_start(); $choices = array('rock', 'paper', 'scissors'); $result1 = array('Win', 'Loss', 'Tie'); $result2 = array('beats', 'loses to', 'ties'); if (in_array($_POST['selection'],array_keys($choices))) { $player = $_POST['selection']; $computer = array_rand(array_keys($choices)); //Determine the results if ($player == $computer) { //Tie $result = 2; $_SESSION['ties']++; } else if ($player==($computer+1) || ($player==0 && $computer==2)) { //Player wins $result = 0; $_SESSION['wins']++; } else { //Player loses $result = 1; $_SESSION['losses']++; } } ?> <html> <head> <title>RPS</title> </head> <body> <div align="center"><h2>Rock - Paper - Scissors in PHP</h2></div> <hr> <form action="<?php echo $_SERVER[php_SELF]; ?>" method="post" enctype="multipart/form-data"> Select one object:<br> <input type="radio" name="selection" value="0"> Rock<br> <input type="radio" name="selection" value="1"> Paper<br> <input type="radio" name="selection" value="2"> Scissors<br> <br><input type="submit" value="Play"> </form> <hr> <?php if ($result1) { //Display the results ?> <p><b>Result: <?php echo $result1[$result]; ?></b></p> <table border="1" summary=""> <tr> <td> <?php echo ucfirst($choices[$player]); ?><br> <img src="<?php echo $choices[$player]; ?>.gif" border="0"> </td> <td><?php echo $result2[$result]; ?></td> <td> <?php echo ucfirst($choices[$computer]); ?><br> <img src="<?php $choices[$computer]; ?>.gif" border="0"> </td> </tr> </table> <hr> <?php echo (int)$_SESSION['wins']; ?> wins <br> <?php echo (int)$_SESSION['losses']; ?> losses <br> <?php echo (int)$_SESSION['ties']; ?> ties <br> <hr> <?php } ?>
-
Well, either pull all the values from the DB and populate JS arrays or use AJAX. This is the Javascript forum, so I provided a response using Javascript.
-
I've never used that class/pprogram. It could be that it has the capability - maybe. Check the documentation. No, MS Word will not "detect" the table of contents. All the elements in Word have embedded coding that makes it all work. Word will be able to detect any raw text as anything othr than raw text. However, that does give me an idea. If you create a proper HTML file and use header tags (H1, H2, etc) then you can open that HTML file in word and those headers will be interpreted correctly. You could then put a page break at the very top and then use Word to insert a TOC on that first page. You might also want to go into the page numbering properties to start the page numbers after the TOC page. By the way, I see from yur first example that you are creating test case docs. What type of software do you work with?
-
Take a look at my example code in reply #3 in this post: http://www.phpfreaks.com/forums/index.php/topic,192055.new.html#new
-
That's what I get for making a change right before posting. Thanks for the catch.
-
[SOLVED] Java Dropdown Population Code Change
Psycho replied to mikemessiah's topic in Javascript Help
You don't need to escape the parentheses at all the problem was that you had a couple of misplaced commas in your array declarations. Here is the corrected code // Province lists var province = new Array(); province['Italy'] = new Array(); province['Namibia'] = new Array( 'Any','Academia / Pioneers Park / Hochland','Auasblick / Olympia','Bethanie','Caprivi Region','Gobabis','Henties Bay','Karibib','Keetmanshoop','lein Windhoek / Eros / Avis / Ludwigsdorf','Kleine Kuppe / Cimbabasia / Prosperitas / Rocky Crest','Langstrand / Dolfynstrand','Luderitz','Luxury hill / Suiderhof / Windhoek West','Maltahohe','Hardap Region','Monte Christo','Okahandja / Okahandja District','Omaruru','Ondangwa','Oshakati','Otjiwarongo','Otjiwarongo District','Rundu','Swakopmund','Tsumeb','Walvis Bay','Windhoek Central','Windhoek District'); province['South Africa'] = new Array('Northern Cape','Western Cape','Eastern Cape','Free State','North West','Gauteng','KZN','Mpumalanga','Limpopo'); // City lists var cities = new Array(); cities['Namibia'] = new Array(); cities['Namibia']['Any'] = new Array('Any'); cities['South Africa'] = new Array(); cities['South Africa']['Northern Cape'] = new Array( 'Any','Tswala','Kuruman','Augrabies Falls','Upington','Kakamas','Pofadder','Kimberly','Alexander Bay', 'Okiep','Springbok','Niewoudtville','Garies','Calvinia','Hopetown','De Aar','Colesburg','Hanover'); cities['South Africa']['Western Cape'] = new Array( 'Cape Flats','Cape Town City Bowl and Atlantic Seaboard','Cape Town Northern Suburbs','Garden Route', 'Great Karoo','Milnerton','Tableview and Blaauwberg','Overberg','Peninsula (Simons Town to Hout Bay)', 'Somerset West and Boland','Southern Suburbs (Tokai to Pinelands)','West Coast'); cities['South Africa']['Eastern Cape'] = new Array( 'East Cape Interior','East London, Eastern Karoo','EC Jeffreys Bay to Tsitsikamma','EC Wild Coast', 'Grahamstown and Surrounds','Port Alfred and Coastal Towns','Port Elizabeth'); //cities['South Africa']['Free State'] = new Array( 'Bethlehem / Harrismith / Clarence & Region','Bloemfontein & Central Freestate', 'Ficksburg / Clocolan / Rosendal & Region','Vaalriver / Parys & Region', 'Vaaltriangle / Vaalpark / Sasolburg','Welkom / Goudveld & Region'); cities['South Africa']['North West'] = new Array( 'Carltonville','Derby','Fochville','Groot Marico','Hartbeesfontein','Klerksdorp','Koster','Lichtenburg', 'Mafikeng','Makwassie','Northam','Orkney','Potchefstroom','Rustenburg','Sannieshof','Schweizer Reyneke', 'Stilfontein','Swartruggens','Ventersdorp','Vredefort','Vryburg','Wolmaranstad','Zeerust'); cities['South Africa']['Gauteng'] = new Array( 'Alberton Southern suburbs','Benoni','Boksburg','Brakpan','Centurion','Edenvale','Germiston', 'Johannesburg CBD and Bruma','Kempton Park','Midrand','Nigel & Heidelberg','Northcliff and Melville (West)', 'Northern Pretoria','Pretoria Central and Old East','Pretoria East','Randburg and Ferndale (North West)', 'Roodepoort (Far West) and Krugersdorp','Rosebank and Parktown (Central)','Sandton and Bryanston (North)', 'Soweto','Springs','Sunninghill','Lonehill and Fourways','Vereeniging','Vanderbijlpark and Vaal Dam','Wynburg (East)'); cities['South Africa']['KZN'] = new Array( 'Dolphin Coast','Durban Central North and CBD','Durban Central South','Durban North','Durban South', 'Durban Upper Highway','Durban West / Highway','KZN Midlands','KZN North Coast','KZN South Coast','Richards Bay'); cities['South Africa']['Mpumalanga'] = new Array( 'Barberton', 'Belfast', 'Bethal', 'Burgersfort', 'Carolina / Badplaas', 'Delmas', 'Dullstroom', 'Ermelo / Chrissiesmeer', 'Graskop', 'Groblersdal', 'Hazyview', 'Hendrina', 'Kinross', 'Komatipoort', 'Lydenburg', 'Machadodorp', 'Malelane / Hectorspruit', 'Marloth Park', 'Middelburg', 'Nelspruit', 'Ohrigstad', 'PietRetief / Volksrust / Wakkerstroom', 'Rietkruil', 'Sabie', 'Secunda', 'Standerton', 'Sundra', 'Trichardt', 'Waterval Boven / Onder', 'White River', 'Witbank'); cities['South Africa']['Limpopo'] = new Array( 'Bela-Bela (Warmbaths) / Rooiberg, Hoedspruit','Makhado (Louis Trichardt) / Musina, Modimole (Nylstroom)', 'Mokopane (Potgietersrus), Mookgophong (Naboomspruit)','Phalaborwa','Polokwane (Pietersburg)', 'Thabazimbi / Lephalale (Ellisras)','Tzaneen / Duiwelskloof / Gravelotte','Vaalwater','Welgelegen'); // Towns lists var towns = new Array(); towns['KZN'] = new Array(); towns['KZN']['Dolphin Coast'] = new Array( 'Ballito','Blythedale / Princes Grant','Chakas Rock / Salt Rock','La Mercy','Sheffield Beach','Stanger', 'Tongaat / Verulam','Umdloti','Westbrook','Zinkwazi Beach'); towns['KZN']['Durban Central North and CBD'] = new Array( 'Beachfront','Berea','CBD','Morningside','Overport / Puntan\'s Hill','Point Waterfront', 'Sherwood / Sydenham / West Riding / Asherville'); towns['KZN']['Durban Central South'] = new Array('000000'); towns['KZN']['Durban North'] = new Array('000000'); towns['KZN']['Durban South'] = new Array('000000'); towns['KZN']['Durban Upper Highway'] = new Array('000000'); towns['KZN']['Durban West / Highway'] = new Array('000000'); towns['KZN']['KZN Midlands'] = new Array('000000'); towns['KZN']['KZN North Coast'] = new Array('000000'); towns['KZN']['KZN South Coast'] = new Array('000000'); towns['KZN']['Richards Bay'] = new Array('000000');