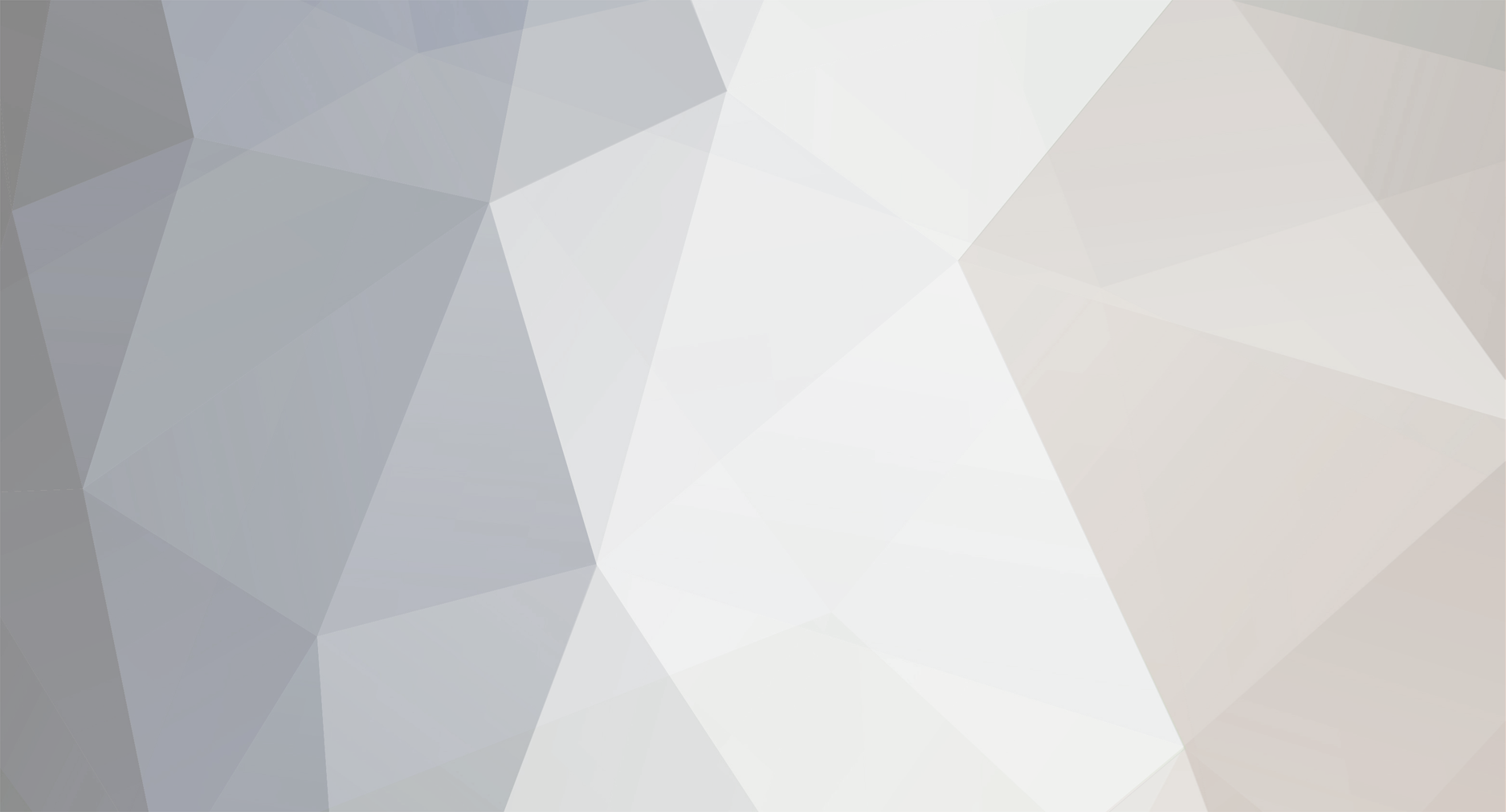
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
I would suggest preg_replace() instead. $new_text = preg_replace('/<img.*?>/', '', $old_text);
-
Your "question" is way too long and convoluted. The quality of the answers are directly proportional to the quality of the questions asked. All you needed to do was to boil the question down to the specific problem.
-
Yeah, there are some problems with the opening and closing brackets. Your opening script tag should be using "type" instead of "language". Here are some validations that should work for you below: The alpha check allows letters, the space, the hyphen and the apostrophe (since names can contain those characters). The phone check allows numbers, parenthesis, space, and the hyphen The email check only allows properly formatted email addresses. You can get more restrictive on these validations (e.g. phone numbers must be 7 or 10 characters), but I included validations based upon your post. <script type="text/javascript"> function validateForm(form) { var fe = form.elements; //validate owner id if (!requiredCheck(fe['dbOwnerId'], 'Owner')) return false; //validate first name if (!requiredCheck(fe['dbFname'], 'First Name')) return false; if (!validAlpha(fe['dbFname'], 'First Name')) return false; //validate last name if (!requiredCheck(fe['dbLname'], 'Last Name')) return false; if (!validAlpha(fe['dbLname'], 'Last Name')) return false; //validate email address if (!requiredCheck(fe['dbemail'], 'Email address')) return false; if (!validEmail(fe['dbemail'], 'Email address')) return false; //validate main phone if (!requiredCheck(fe['dbmainphone'], 'Main Phone')) return false; if (!validPhone(fe['dbmainphone'], 'Main Phone')) return false; //validate other phone if (!validPhone(fe['dbotherphone'], 'Other Phone')) return false; //validate address if (!requiredCheck(fe['dbaddress'], 'Address')) return false; //validate address 1 if (!requiredCheck(fe['dbcity'], 'City')) return false; if (!validAlpha(fe['dbcity'], 'City')) return false; //validate post code if (!requiredCheck(fe['dbpostcode'], 'Post Code')) return false; //validate password if (!requiredCheck(fe['dbpassword'], 'Password')) return false; return true; } function requiredCheck(fieldObj, fieldName) { if (!fieldObj.value) { alert(fieldName+' is required.'); fieldObj.focus(); return false; } return true; } function validEmail(fieldObj, fieldName) { validEmailRegEx = /^[\w\+-]+([\.]?[\w\+-])*@[a-zA-Z0-9]{2,}([\.-]?[a-zA-Z0-9]{2,})*\.[a-zA-Z]{2,4}$/ if (!fieldObj.value.match(validEmailRegEx)) { alert(fieldName+' is not in the proper format.'); fieldObj.focus(); return false; } return true; } function validAlpha(fieldObj, fieldName) { validAlphaRegEx = /^[a-zA-Z '-]*$/ if (!fieldObj.value.match(validAlphaRegEx)) { alert(fieldName+' contains invalid characters.'); fieldObj.focus(); return false; } return true; } function validPhone(fieldObj, fieldName) { validNumberRegEx = /^[\d \-()]*$/ if (!fieldObj.value.match(validNumberRegEx)) { alert(fieldName+' contains invalid characters.'); fieldObj.focus(); return false; } return true; } </script>
-
It's not that there are no records to display. Using die in that fashion means there was a critical error when trying to run the query. But, you can (and should) take an approach that exits the script gracefully. Note: You should create your queries as variables so you can echo them to the page when there is an error: Example: <?php $query = "Select count(*) from carshare"; $result = mysql_query($query); if (!$result) { echo "The query:<br>$query<br><br>"; echo "failed with the following error:<br>".mysql_error(); } else { //display the results } //Continue with rest of page ?>
-
I agree with Naez, but to explain why you have this problem... If a record set has 10 records then mysql_numrows() will return 10, obviously. But, the records go from 0 to 9! I wouldn't suggest using the code you have above, but the correct thing to do would be this: $num=mysql_numrows($result) - 1;
-
How many possible values do you have for $_GET['a']? Instead of stripping out values that shouldn't be in the result I ONLY accept the ones I know should be accepted. <?php switch ($_GET['a']) { case 'page1': case 'page2': case 'page3': case 'page4': case 'page5': include "$a.php"; break; default: include 'home.php'; break; } ?>
-
Or use preg_replace: <?php $date='yyyymmdd'; echo preg_replace('/(.{4})(.{2})(.{2})/', '$1/$2/$3', $date); //output: yyyy/mm/dd ?> You can change the order of the parts my modifying the second parameter
-
This is what the manual states the key being for use in a SQL statement
-
You are asking for help with creating a JavaScript function, correct? JavaScript is run within client-side code. What you have posted above is server-side code. It is very difficult to try and write JS code against server-side code. The server-side code should be run to produce the output that will be delivered to the browser (e.g. HTML) so that the JS can be created. You can then implement the JS code into your server-side code. I can't run the code above as I do not have the appropriate database set up. All you need to do is run the page above through your browser and post the output (i.e. the page source).
-
A couple things. I wouldn't suggest using htmlentities when inserting data into the database. I woud use it when displaying the data. the problem is that htmlentities converts characters to their HTML character code, so the ampersand character '&' becomes '&'. Now you will have problems with character lengths. If a db field only takes 10 characters and the input field accepts 10 characters an input by the user of 'some&else' will result in a string that is 13 characters long. Also, there is no need to do anything character escaping with the password. Just use MD5($_POST["password"]). The results will always be safe for insertion.
-
what is the difference between include and require_once?
Psycho replied to frijole's topic in PHP Coding Help
There's a manual?! -
I did find one small bug with the code I posted. When you press "Alt-Q" an alert box comes up. So, if you release the Alt key before cancelling the alert dialog the script thinks that Alt is still down. but, if you are not utilizing an alert at that time it shouldn't be an issue.
-
I don't think that will work. I took an existing script I have and modified it to determine if Alt-Q was being pressed (note: only workws within the text field). you can modify to suite your needs. Basically it just creates a global variable to detemine if the alt key is up or down. Then whenever the "q" is pressed check if Alt is also down. <!DOCTYPE HTML PUBLIC "-//W3C//DTD HTML 4.01//EN"> <html> <head> <title>test</title> <script language="javascript"> //Set global variable var altKey = false; function init() { var oinputs = document.getElementsByTagName('input'); for (var i = 0; i < oinputs.length; i++) { oinputs[i].onkeydown = function (e) { e = e || event; switch (e.keyCode) { case 18: // alt // same for the enter button altKey = true; break; case 81: // Q // same for the enter button if (altKey) { alert('Alt-q'); } break; default: // the rest of the keys break; } } oinputs[i].onkeyup = function (e) { e = e || event; if (e.keyCode == 18) { altKey = false; } } } } </script> </head> <body onload="init()"> <input type="text" name="1"/> </body> </html>
-
Could you post the code from the OUTPUT - it is very difficult to code javascript from within the PHP code.
-
OK, let me paraphrase so we all understand. You process the input file and create the (open,maxim,minim,close and a total volume) values for each symbol for each minute. Is that correct? Can you identify what the values are in the input file? the data & time are pretty obvious, not sure of the rest.
-
Well, I've taken a cursory look at the code and you have a lot of nested loops. Since there are not helpful comments in the code I can't determine if those are absolutely necessary or if there is a better approach. I'm not going to read the code line by line to try and figure out what each line is trying to accomplish. Can you explain how you get from the input to the output? You could also put in some debuggin to see how much time each process is taking. Create a start time at the beginning and then echo the (current time - start time) at key points. That will pinpoint the problem areas. It may not make much difference in performance, but I notice you are setting variables according to the number of items in an array and then doing a for loop using that value. I'd suggest using a foreach loop instead. EDIT: Sorry, you do have comments, they just aren't in my language. They probably are useful to you which is the most important.
-
Well, do you want to remove the first two digits or keep the last two digits? those are two different things - unless we make the assumption that the value will always be four characters long. This will return the last two charcters from a value: $last_two = substr($original_value, -2);
-
Well, i think you still need to go back to your function. I would create a function that takes an optional parameter. If the parameter is present the function would return only the values necessary from the query. If no parameter is present it would return the whole array. here is an example: Let's say the array that would normally be returned from the function looked like this array ( 'IndexA' => 'ValueA' 'IndexB' => 'ValueB' 'IndexC' => 'ValueC' 'IndexD' => 'ValueD' } Ok, in "some" situations you just want an individual value, in others you need the whole thing. So, the function could look like this: <?php function getValues($return_index=false) { //Code to generate $values_array goes here if ($return_index!=false && isset($values_array[$return_index])) { return $values_array[$return_index]; } else { return $values_array; } } ?> Now if you call $myObject = getValues(); you get the whole array back just as you do now. But you can also just have a single value returned (and use within an echo) by calling the function like this echo getValues('IndexC'); However, as stated previously I would go one step further and make it so the query is only selecting the data requested.
-
"maybe have different tables for each team?" I only meant that creating a table for RED "members" vs a table for BLUE "members" would be a poor choice. If the "teams" entities need additional data then having a separate table to describe team specific information would be the logical choice. Cray is correct that you should not repeat team specific data on a member by member basis.
-
So, the values are in the textbox and are separated by a single space? <?php $values = explode(' ', $_POST['fieldname']); foreach ($values as $value) { echo "Value: $value<br>"; } ?>
-
I don't think you can get it to work in that manner. You could change the function to return only the value you want to print to the page.
-
<?php $query = mysql_query("SELECT * FROM potdp WHERE position = '0'") or die("Error: ".mysql_error()); while($smffetch=mysql_fetch_array($query)) { //Create the code here for displaying each record echo $smffetch["id"] . ': '. $smffetch["date"] . '<br>'; } ?>
-
Just create a column for "team" in the member table and put the appropriate value for each member. Lets say you use 'r' and 'b' to identify the team assignment. You can then get all red members using the query SELECT * FROM members WHERE team='r' Create a separate table is not just a "waste of a table" it is bad practice.
-
On your index page use a switch() to determine the 'game', then I would suggest using an external file (as appropriate) to determine the sub-page. And then add'l external files for each page (assuming they don't share a lot of common code). This keeps the different bits of logic separate and prevents a page from becomming so long that it is difficut to troubleshoot or modify. Of course you can keep that logic in-line on the main page as well. <?php switch ($_GET['game']) { case 'dod': //Include the dod index file which will determine the page to display include ('dod_index.php'); break; case 'css': //Include the css index file which will determine the page to display include ('css_index.php'); break; default: //Include the main page - i.e. no 'game' page selected include ('main.php'); break; } ?> The 'dod_index.php' page could look very similar: <?php switch ($_GET['page']) { case 'contacts': //Include the contacts file to display the page content include ('dod_contacts.php'); break; case 'matches': //Include the matches file to display the page content include ('css_matches.php'); break; case 'roster': //Include the roster file to display the page content include ('css_roster.php'); break; default: //No 'page' was selected - display the home page for css include ('css_main.php'); break; } ?> However, if the 'contacts' page will have the same functionality for each game type, then I would create one file to display contacts and use the $game variable to make the page pull the ciorrect content.
-
Yes, there are tests to see if JavaScript is enabled. One way is to have JS code to set a cookie, then check in PHP if it is set. You can then use that to determine different logic to follow based upon JS being enabled or not. For example, if you have "nested" select boxes where the value of one determines the values in the other (e.g. state and city), then you would have to allow the user to select the state and submit the value to get the list of cities as opposed to using AJAX to populate the list on the fly. Each implementation will require a different approach.