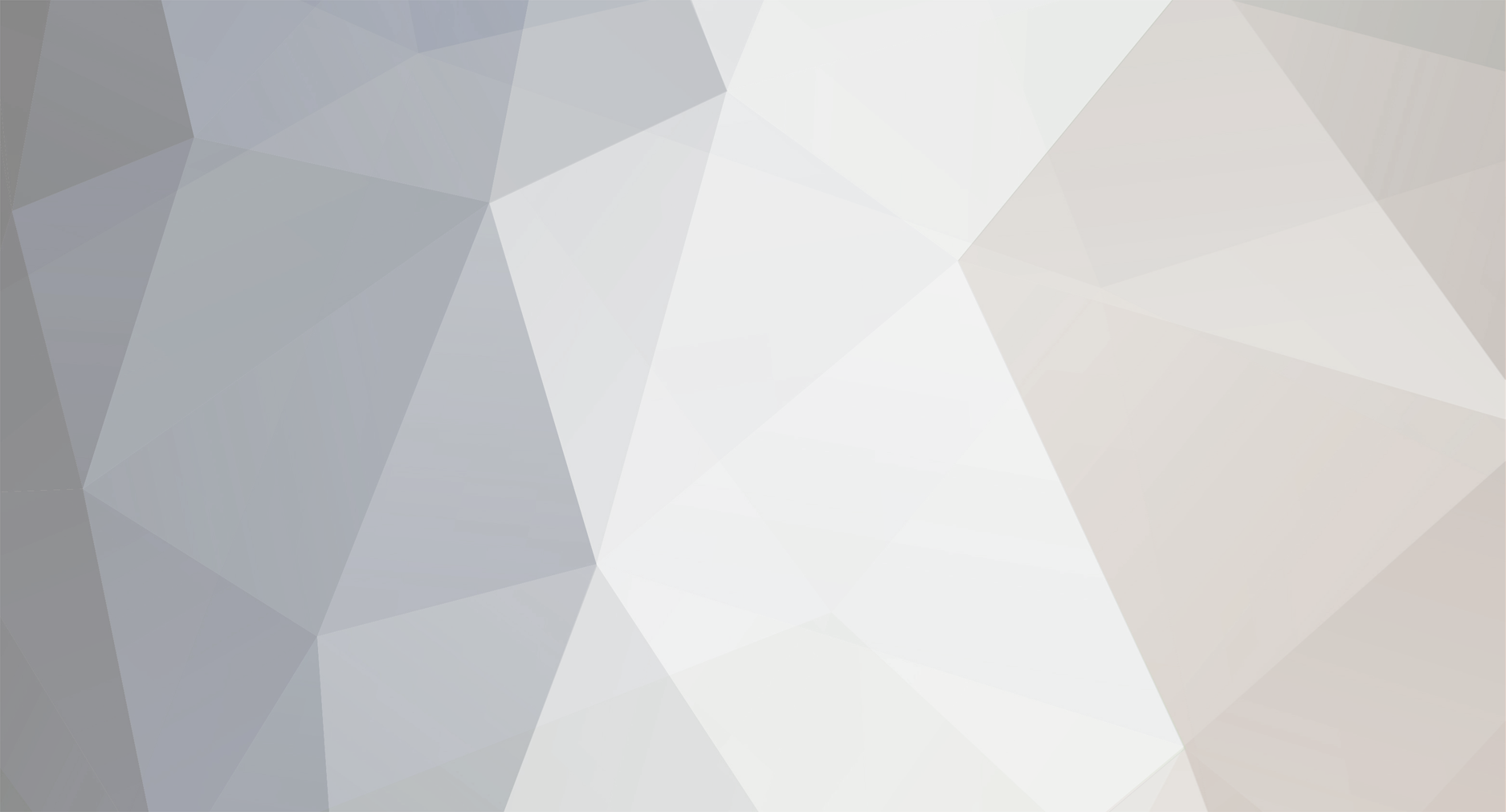
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Displaying information from 2 MySQL tables on the same page
Psycho replied to dinog's topic in PHP Coding Help
You are going to want to use ORDER BY EpisodeTable.EpisodeID, SongTable.SongID To first sort by Episodes and then sort by Songs -
Displaying information from 2 MySQL tables on the same page
Psycho replied to dinog's topic in PHP Coding Help
<?php include 'dbconfig.php'; include 'dbopen.php'; $query = "SELECT * FROM SongTable JOIN EpisodeTable ON EpisodeTable.EpisodeID = SongTable.EpisodeID ORDER BY EpisodeTable.EpisodeID"; $result = mysql_query($query2) or die(mysql_error()); $current_episode = ''; while ($record = mysql_fetch_assoc($result)) { //New episode record if ($record['EpisodeName'] != $current_episode) { //Close previous table (if exist) if ($current_episode != '') { print "</table><p>"; } //Create new episode table $current_episode = $record['EpisodeName']; print "<h2><a name=\"ep\">Episode: $current_episode</a></h2><p>"; print "<table width=\"95%\" border=\"1\" cellpadding=\"4px\" cellspacing=\"0\">"; print "<tr bgcolor=\"#d0d0d0\">"; print "<td width=\"20%\"><strong>Song Title</strong><td width=20%><strong>Artist</strong><td width=30%><strong>Album information</strong>"; } //Display the song record print "<tr><td>{$record['Title']}</td><td>{$record['Artist']}</td><td>{$record['Album']}</td></tr>"; } //Clost the last table print "</table><p>"; include 'dbclose.php'; ?> -
Well, I'm not sure what you are trying to accomplish with the htmlentities() command (you should be validating that the value is a whole number). But, your problem is this line: $page = '$page1'; It is setting $page to the string value of "$page1". In fact there is no reason for that line at all: $charset='UTF-8'; $page = htmlentities ($_GET['page'], ENT_NOQUOTES, $charset); if(!isset($page)){ $page = 1; } $max_results = 10; $from = (($page * $max_results) - $max_results); Although I still think you should be doing a different validation other than htmlentities().
-
If you want to the user to select the fields to be searched just create a form field(s) for selecting the fields: multi-select list, checkboxes, whatever. If multiple fields are being searched you would just need to use an OR clause in your query "field1 LIKE '%searchvalue%' or field2 LIKE '%searchvalue%'", etc. $words = preg_split('%[\s,]+%', $string); $first_fifty = implode(' ', array_slice($words, 0, 50)); $search = 'text to be highlighted'; $replace = '<span style="background-color:yellow;>'.$search.'</span>'; $output = str_replace($search, $replace, $input);
-
Again, no way to respond without knowing your table structures. I'm not a clairvoyant. Please list the fields in the tables you need to get data from, identifying the foreign keys as applicable. Also, your explanatin of the data you are after is vague at best. Please be VERY specific.
-
Um, you can still do it all in one script without knowing the id ahead of time. Just insert the data into the database and then use mysql_insert_id to get the just inserted record id and change the uploaded image's name.
-
There's no way for us to know if a JOIN is needed since we have no clue as to the structure of your database. Are you querying data from multiple tales? If so, then a JOIN would be needed. But if all the data is in one table then it would look something like this: SELECT count(*) FROM question WHERE CorrectAnswer = 'A' AND chapterid = 1
-
There are a few problems. 1. You are missing a closing "}". You have three opeinging curly brackets for the function, for the if statemetn and for the for loop, but there are only two closing curly brackets. 2. opening script tag is incorrect. It should be <script type="text/JavaScript"> 3. You have a double quote mark within the iChars variable, but the variable is enclosed in double quotes. So, you need to escape the one inside the quotes with a backslash: var iChars = "!@#$%^&*()+=-[]';,./{}|\":<>?"; Having said all that, however, this would be better done using a regular expression instead of looping through every character.
-
Then don't make them qty1, qty2, etc. And instead make them an array to begin with...sheesh. (Although if you used proper validation on your page they WOULD be qty1, qty2, etc.) Just name the fields "qty[]", "window[]", "width[]", etc. and the values will be returned as sub arrays.
-
This should give you a start. Although i would add a lot more verification of the data: <?php if ($_POST) { $fname = mysql_real_escape_string($_POST['first_name']); $lname = mysql_real_escape_string($_POST['last_name']); $addr = mysql_real_escape_string($_POST['address']); $city = mysql_real_escape_string($_POST['city']); $state = mysql_real_escape_string($_POST['zip']); $zip = mysql_real_escape_string($_POST['state']); $ph1 = mysql_real_escape_string($_POST['phone_1']); $ph2 = mysql_real_escape_string($_POST['phone_2']); $ph3 = mysql_real_escape_string($_POST['phone_3']); $email = mysql_real_escape_string($_POST['email']); $query = "INSERT INTO quotes (first_name, last_name, address, city, state, zip, phone_1, phone_2, phone_3 and email) VALUES ('$fname', '$lname', '$addr', '$city', '$state', '$zip', '$ph1', '$ph2', '$ph3', '$email')"; $result = mysql_query($query); $i = 1; while ($_POST['window'.$i]) { $qty = mysql_real_escape_string($_POST['qty'.$i]); $window = mysql_real_escape_string($_POST['window'.$i]); $width = mysql_real_escape_string($_POST['width'.$i]); $height = mysql_real_escape_string($_POST['height'.$i]); $room = mysql_real_escape_string($_POST['room'.$i]); $window = mysql_real_escape_string($_POST['window'.$i]); $i++; $query = "INSERT INTO quote_items (qty, window, width, height, room) VALUES ('$qty', '$window', '$width', '$height', '$room')"; $result = mysql_query($query); } } ?>
-
Um, no. You use a hash for a password, which is not really encryption. With a hash there is no way to deduce the original value from the hashed value. And before anyone pipes up saying there are plenty of sites that can get the value of an MD5 hash, those sites use a rainbow table of known hashed values. That is not unencrypting something. Hashing is good for passwords, because you never really need to know the password. When the user logs in you just take their entered value, hash it, and see if it matches the value in the database. When hashing a password it is a good idea to use a "salt" (this is additional information added tot he hashed value) or multiple hashes this makes it impossible for someone to 'crack' the password unless they have knowledge of your method. For example if username cannot be changed then you can use that as a salt" $hased_password = MD5(SHA($password.$username)); Encryption is used when yuo want to secure a value, but you must be able to retrieve the original value. This would be if you needed to store CC info so the user could make a purchase with their saved CC info, for instance.
-
The page at the link you provided works for me. The code I provided is only a rough framework. There is still a lot of work that needs to be done (Much more than I am willing to provide). But, realize that the page should now have 2 forms. The original form you had is only for adding the data to the table which is another form. That is the form that should get submitted for processing.
-
OK, here is something I did quick and dirty. It works but will need more functionality and validation to be finished: <!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN" "http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd"> <html xmlns="http://www.w3.org/1999/xhtml"> <head> <meta http-equiv="Content-Type" content="text/html; charset=iso-8859-1" /> <title>Untitled Document</title> <script type="text/javascript"> function valueField(fieldName, formObj) { var fieldObj = formObj[fieldName]; var fieldValue = fieldObj.value; fieldName = fieldName + '[]'; var inputTxt = '<input type="text" name="'+fieldName+'" value="'+fieldValue+'"'; inputTxt += ' readonly="readonly" style="background-color:#cecece" />'; inputObj = document.createElement(inputTxt); return inputObj; } function addRow(tableID) { // Get a reference to the form var formObj = document.getElementById('addItem'); // Get a reference to the table var tableRef = document.getElementById(tableID); // Insert a row in the table at row index 0 var newRow = tableRef.insertRow(tableRef.rows.length); // Insert the cells left to right var newCell = newRow.insertCell(0); newCell.appendChild(valueField('mfgr', formObj)); var newCell = newRow.insertCell(0); newCell.appendChild(valueField('location', formObj)); var newCell = newRow.insertCell(0); newCell.appendChild(valueField('height', formObj)); var newCell = newRow.insertCell(0); newCell.appendChild(valueField('width', formObj)); var newCell = newRow.insertCell(0); newCell.appendChild(valueField('wtype', formObj)); var newCell = newRow.insertCell(0); newCell.appendChild(valueField('qty', formObj)); //Clear form values formObj.mfgr.value = ''; formObj.location.value = ''; formObj.height.value = ''; formObj.width.value = ''; formObj.wtype.value = ''; formObj.qty.value = ''; document.getElementById('submitButton').disabled = false; } // Call addRow() with the ID of a table </script> </head> <body> <form name="valuesToSubmit"> <table id="itemList" border="1px"; style="width:800px;background-color:#cecece;"> <tr> <th>Qty.</th> <th>Window Type</th> <th>Width</th> <th>Height</th> <th>Room Location</th> <th>Manufacture 1</th> </tr> </table> <button type="submit" name="submit" id="submitButton" disabled="disabled">Submit the Values</button> </form> <form id="addItem"> <table cellpadding="5" cellspacing="0"> <tr> <th>#</th> <th>Qty.</th> <th>Window Type</th> <th>Width</th> <th>Height</th> <th>Room Location</th> <th>Manufacture 1</th> <th></th> </tr> <tr bgcolor="#ececec"> <td>1.</td> <td><input type="text" size="3" name="qty" value="" /></td> <td> <select name="wtype"> <option value="">...Choose</option> <option value="Window 1">A - Slider (left to right)</option> <option value="Window 2"> Slider (right to left)</option> <option value="Window 3">3 lite slider</option> <option value="Window 4">Picture Window</option> <option value="Round Top">Round Top</option> <option value="Single Hung">Single Hung</option> <option value="Garden Window">Garden Window</option> <option value="Bay Window">Bay Window</option> <option value="Casement">Casement</option> <option value="Awning">Awning</option> <option value="Sliding Glass Doors">Sliding Glass Doors</option> <option value="3 lite Sliding Patio Door">3 lite Sliding Patio Door</option> <option value="French Door">French Door</option> </select> </td> <td><input type="text" size="8" name="width" value="" /></td> <td><input type="text" size="8" name="height" value="" /></td> <td> <select name="location"> <option value="">...Choose</option> <option value="Location 1">Bedroom</option> <option value="Location 2">Living/Family Room</option> <option value="Location 3">Dinning Room</option> <option value="Location 4">Kitchen</option> <option value="Location 4">Utility Room</option> <option value="Location 4">Garage</option> </select> </td> <td> <select name="mfgr"> <option value="">...Choose</option> <option value="Manufacture 1">Cascade Windows</option> <option value="Manufacture 1">Certainteed Windows</option> <option value="Manufacture 2">Comfort Design Inc.</option> <option value="Manufacture 3">Empire Pacific Windows</option> <option value="Manufacture 4">LBL Windows</option> <option value="Manufacture 4">Marvin Windows</option> <option value="Manufacture 4">Milgard Windows</option> </select> </td> <td><button onclick="addRow('itemList');">Add Items</button></td> </tr> </table> </form> </body> </html>
-
OK, I just reread your post and you want the items added to a table each time they click the button and then submit everything. A little different from what I proposed, but it is still a javascript solution. Of course you could do it with AJAX as well.
-
I *think* he is wanting to add additional fields on the page without having to submit the page - i.e. add all the items at once and then submit the page. You will need JavaScript to do this. Here is a working page that demonstrates one way to accomplish that: <html> <head> <script> function addTiming(field) { thisFieldIdx = field.id.substr(6); newFieldIdx = thisFieldIdx*1 + 1; if (!document.getElementById('timing'+newFieldIdx)) { timingDiv = document.getElementById('timings'); timingDiv.innerHTML = timingDiv.innerHTML + 'Timing '+newFieldIdx+': <input type="text" name="timing'+newFieldIdx+'" id="timing'+newFieldIdx+'" onfocus="addTiming(this);"><br>'; } document.getElementById('timing'+thisFieldIdx).select(); document.getElementById('timing'+thisFieldIdx).focus(); return; } </script> </head> <body> <form name="formname"> <div id="timings"> Timing 1: <input type="text" name="timing1" id="timing1"><br> Timing 2: <input type="text" name="timing2" id="timing2"><br> Timing 3: <input type="text" name="timing3" id="timing3"><br> Timing 4: <input type="text" name="timing4" id="timing4" onfocus="addTiming(this);"><br> </form> </div> </body> </html>
-
That creates an empty array variable. It's just good practice to initialize your variables before you start using them.
-
The code I provided takes the email addresses from the query and puts them into a single string that is comma separated. You just need to use that value ($recipient_list) as the first parameter in the email() function: <?php include("../dbinfo.inc.php"); $cxn = mysqli_connect($host,$username,$password,$database) or die ("Couldn't connect to server."); $game="Chelsea V Liverpool"; ////// MANUALLY CHANGE THE VARIABLE $query = "SELECT DISTINCT user FROM history WHERE game='$game'"; ///////// SELECTS RELEVANT USERS $result = mysqli_query($cxn,$query) or die ("Couldn't execute query."); $recipients = new array(); while($row = mysqli_fetch_assoc($result)) { $recipients[] = $row['user']; } $recipient_list = implode(", ", $recipients); $tsubject = "subject"; $ttext = "text"; $sender = "sender@sender.com"; @mail($recipient_list, $tsubject, $ttext, "FROM: $sender"); /////////// SENDS EMAIL ?>
-
This will put all the emails in a single string, comma separated. <?php $recipients = new array(); while($row = mysqli_fetch_assoc($result)) { $recipients[] = $row['user']; } $recipient_list = implode(", ", $recipients); ?>
-
Well, there are a few different ways to attack this problem. unfortunately you're not in a position to try each to find the best fit. If you create your own code using the PDF Library within PHP the process may be very different from any previous programming you have done. With PDF creation you don't just "print" text to the page. You need to first create the page (size) set the font properties and then select the coordinates on the page (in points: 72dpi) that you want to place the text. Also, it will not wrap text for you. If you need text to break accross multiple lines then you need to break it apart in the code and place eah part on the page according to the appropriate coordinates. There are probably some free PHP classes that would make this easier, but I haev never used any so I can't recommend any. Lastly you could get a 3rd party utility that will convert HTML output to PDF. So, all you need to do is have a page that outputs the format in HTML format of how you want the PDF created. You don't need to display that page tot he user, just pass the URL to the app. A quick search found a free app: http://www.rustyparts.com/pdf.php But, I don't know about it's quality. In previous companies we have paid a quite a bit for robust applications to do this. here are a few examples: http://www.activepdf.com/ http://www.realobjects.com/PDFreactor.808.0.html?gclid=CNT_3Mud55ECFQJEPAodgiZPgA
-
<?php $query = "SELECT email FROM PHPAUCTIONXL_users WHERE nick='$user_nick'"; $result =mysql_query($query) or die (mysql_error()); $record = mysql_fetch_assoc($result); $email = $record['email']; ?>
-
Then you are not using the database how it should be. you already have the name of the category in the category table, so the product table should just hold the ID of the category - not the value. That is the whole point of using a relational database, you don't have to replicate data you can relate it to another table. If you decided you needed to rename a category you would just need to update a single record instead of possibly many, many records in the product table. I would highly suggest changing the category column in the product table to the category ID. However, assuming you don't, this shoudl work (although I supply this against my better judgement): SELECT * FROM ds_products JOIN ds_foodcats ON ds_products.foodcat = ds_foodcats.name ORDER BY ds_foodcats.sort_order Also, why are you renaming all of those fields? That's a lot of unnecessary code (unnecessary code = error prone code). Just use 'pWeight' in the result instead of 'weight'. Or if you really want to use 'weight' then change the field name to that in the table.
-
short way of writing if OR OR OR OR statement?
Psycho replied to wfcentral's topic in PHP Coding Help
I see three options: You could combine the options: <?php if ($row['label']=='Family Attraction' || $row['label']=='Museum') || $row['label']=='Arts' || $row['label']=='Landmark') { $color='ORANGE'; } if ($row['label']=='Italian' || $row['label']=='Greek' || $row['label']=='Steak' || $row['label']=='Seafood') { $color='RED'; } ?> You could use a switch which is better programatically, but not shorter <?php switch ($row['label']) { case 'Family Attraction': case 'Museum': case 'Arts': case 'Landmark': $color = 'ORANGE'; break; case 'Italian': case 'Greek': case 'Steak': case 'Seafood': $color = 'RED'; break; } ?> Or you could use an array: <?php $colors = array ( 'Family Attraction' => 'ORANGE', 'Museum' => 'ORANGE', // etc.. } $color = $colors[$row['label']]; ?> Which one I would use would depend upon how often the list changes, how big the list is, etc. But, without more information I would suggest using the switch() statement. -
Hmmm, when you say "One of the fields in that table is categories" does that mean there are multiple categories listed for a product? If so, you will want an associative table. if there is only one category per product it is very simple: SELECT * FROM products JOIN categories ON products.category_id = categories.id ORDER BY category.sort_order
-
Well, you didn't state what value in the query should match the $d_mtime values, so I will go with the field name 'value' <?php $split_m = explode(",",$d_mtime); $values = "'" . implode("','", $split_m) . "'"; $query = "SELECT * FROM ds_mealtimes WHERE rid = '$xxid' AND value IN ($values)"; $result = mysql_query($query) or die(mysql_error()); while($row = mysql_fetch_array($result, MYSQL_ASSOC)) { $d_name = $row['name']; print "<option value=$d_name>$d_name</option>"; } ?>
-
I would suggest generating a random GUID that gets populated into a hidden field on the page that POSTs the data. Then when iserting the High Score also isert the GUID. You can then check if the GUID exists before inserting a new high score.