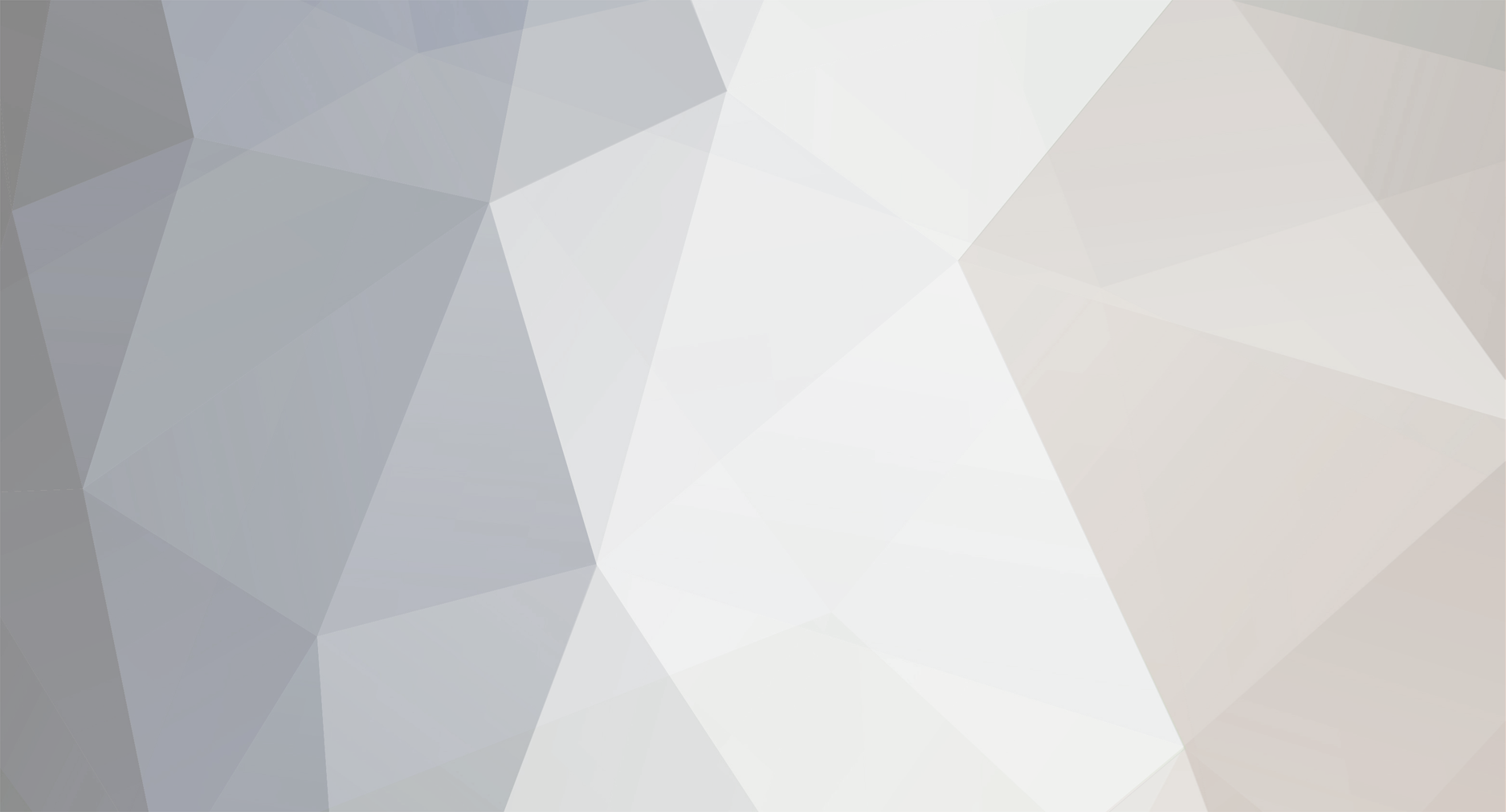
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
That's because $row will never be empty. If there are no records that code never goes inside the first IF loop. Also there is some duplicity in that code.: Try this: <?php $q = mysql_query($sql) or die("Error running query:".mysql_error()); if($row = mysql_fetch_array($q)) { echo "<img src='http://www.runningprofiles.com/members/images/thumbs/". $pid . "." . $row['ext'] . "'"; } else { echo "<img src='http://www.runningprofiles.com/members/images/noimage.jpg>"; } ?> Edit: Since you do this if($q && mysql_num_rows($q) > 0) { This is kind of irrelevant if(!empty($row)) {
-
Sure, put a variable on the URL of the link and check it on the receiving page: LINK: <a href="somepage.php?switch=true">Click Me</a> PROCESSING PAGE: <?php if ($_GET['switch']=='true') { //Link was clicked } else { //Link was NOT clicked } ?> This is not a 100% guarantee, since the user can type the url and the varialbe as well, but would be OK for most usage.
-
Really? that makes no sense, because your loop is continually adding to the $keys and $values arrays. The way you had it before would first process all the values from the first array - then it would process all the values from the first & second arrays. You really haven't provided enough information to help you.
-
~~ Updating Arrays stroed in sessions and retrieving them? ~~
Psycho replied to Ryanz's topic in PHP Coding Help
Sorry, but I'm not going to invest a ton of time doing all this for you. this forum is for people who want help with their code. You can incorporate as many questions as you want, just create the code to pull and display the question and answers. I only put one question in repeated multiple times to concentrate on the functionality you asked for. Good luck. -
Because your: $data .= str_replace($keys, $values, $loop_source); is inside the loop. Move it after the loops.
-
Absolutley NOT! Never use variables on the query string to determine logged in/admin status. The user can simple change the query to give themselves access they should not have.
-
Based upon the array at the end of your post the problem is that you are trying to reference those inner arrays with numerical values using the $i variable. There is NO $array[0] value so your foreach loop is failing. You need to reference those inner arrays using their proper index values: <?php // Loop within the values array foreach ($array as $subarray) { // Now we go through the keys foreach ($subarray as $key => $value) { $keys[] = '{X_'.$key.'}'; $values[] = $value; } // Assign values within the loop source and add to data variable $data .= str_replace($keys, $values, $loop_source); } ?>
-
Why use onblur? If a user enters a field and makes no changes, why would you need to revalidate it? Granted, there could be some situations where onblur might be useful, such as if the validation parameters are changing in real-time. But, those situations are few and far between. I still stand by using onchange for form validations.
-
How do I stop a contact form being processed if a condition applies?
Psycho replied to john8m's topic in PHP Coding Help
<?php if ($_POST['hidden_field']!='') { //do not process form } else { //process form } ?> -
onkeyup would be a poor choice for performing form validations. it would be too intensive and be difficult to prevent false-positive. Ex: there is an existing field called 'name' and the user tries to create a new field called 'name_suffix'. As soon as the user enteres 'name' it would generate an error and most likely prevent the user from contuniuing. onchange would be a much better choice. Yes you can do this with AJAX, but you also still need to implement the validation server-side once the form is submitted in case the user does not have JS enabled. I would aslo agree that generating an array of the values to be validated for uniqueness instead of sending an individual request each time the user changes the value.
-
You can do whatever you want with it. Of course if you are wantingthe textual values of 'one, two, three, four' you will need to add some more functionality to transpose the numbers to their textual representations.
-
You would have more luck creating a PDF. Would still be a good deal of work, but it would definitely be easier than a word doc.
-
array_sum() with multidimensional $_SESSION arrays
Psycho replied to vassili's topic in PHP Coding Help
You can't store an array as a session value directly. Try serialize() and unserialize(). -
Something like this: <?php $lines = file("file.txt"); $no = 1; foreach ($lines as $line) { $data[$key] = explode("|", $line); $value = $no . ". " . trim($data[$key][1]); print $value; $no = ($no==5) ? 1 : $no+1 ; } ?>
-
Here is a tutorial with the code to convert images from one type to another: http://www.phpit.net/article/image-manipulation-php-gd-part1/4/
-
Just create the static pages as html and give them a php extension. Then both you and the client are happy. It will add an insignificant additional load on the server because it will be processing those 'static' pages when it doesn't need to. However, I would typically create even a brocure type site in all PHP because there will be common elements between pages: header, navigation, etc. It is much easier to just create these elements as PHP include files than to recreate on the static pages.
-
Determining if all JPEG's in directory have the same aspect ratio?
Psycho replied to cgm225's topic in PHP Coding Help
Those would NOT have the same aspect ratio. 4:3 os not the same as 3:4. Are you planning to create thumbnails that are at a 90 degree rotaion from the original? You state that if they do not have the sme ratioi you want to create thumbnails that are cropped. I would suggest that you create a "max" thumbnail height & width and make the thumbnails such that they fit within that dimension. The problem with cropping the images is that they then lose some information about the original - not just the missing pixels, but the dimension as a visual que. For example let's say the max thumbnail size is 100 X 100. You have three images: image A is 200 X 400, image B which is 300 X 200, and image C which is 400 X 400. The result would be thumbnails with the following sizes (that maintain their original aspect ratio, but 'fit' within the same box): Image A: 50 X 100 Image B: 100 X 67 Image C: 100 X 100 -
~~ Updating Arrays stroed in sessions and retrieving them? ~~
Psycho replied to Ryanz's topic in PHP Coding Help
I only created a simple true/false response to test the functionality. The true/false should NOT be the value of the answers. Instead you should be creating the questions and possible answers dynamically. Then you should be checking the response form the user and determining if the response was true or false and taking appropriate action. -
persoanlly I use a single script/page to check the user's login/rights and call that at the beginning of every applicable page. You use an login.php for example, set an variable or session, include it at every page that needs an valid login. ? does it slow down the response. How would you accomplish this then ? It all depends on how secure you want the applicatin to be. For instance, if you have a system where an administrator can disable access to a user and that change needs to take place "immediateky" then you need to check the user's credentials on every page load. However, if those changes only need to be checked at the start of each session, then you can refer to the session value. In any case, the method of checking for logged in or rights just needs to be written once and included on every page. That is the reason it should be a separate file. That way you can modify that method for the entire site in one fell swoop.
-
~~ Updating Arrays stroed in sessions and retrieving them? ~~
Psycho replied to Ryanz's topic in PHP Coding Help
By putting the next page value ont he query string, you would be making it more difficult to control the order of the questions since the user can edit that value. Take a look at this: <?php session_start(); $page_count = 10; $page = $_GET['page']; if (!isset($_POST['answer'])) { //Create an array with the values from 1 to $page_count $pages = range(1, $page_count); //Randomize the array shuffle($pages); //Save the array to a session variable $_SESSION['pages'] = serialize($pages); echo "You are on the start page. There are $page_count random questions to answer.<br><br>"; echo "<form method=\"POST\" action=\"{$_SERVER['PHP_SELF']}\">\n"; echo "<button type=\"submit\" name=\"answer\" value=\"start\">Start</button> the test\n"; echo "<form>\n"; } else { //retrieve the pages array from the session $pages = unserialize($_SESSION['pages']); if ($_POST['answer']=='False') { echo "You got the last question <b>wrong</b>. Try again."; $this_page = $_POST['current_page']; } else { $last_page = (count($pages)<1)?true:false; //Get the first from the random list $this_page = array_shift ($pages); //Save the new pages array to the cookie $_SESSION['pages'] = serialize($pages); if ($_POST['answer']=='Start') { echo "This is your first question. Let's start the test."; } else { echo "You got the last question <b>correct</b>."; } } if ($last_page) { echo "<br><br>You have completed the test."; } else { echo "<h2>You are on question $this_page.</h2>"; echo "<form method=\"POST\" action=\"{$_SERVER['PHP_SELF']}\">Question goes here:<br>\n"; echo "<input type=\"hidden\" name=\"current_page\" value=\"{$this_page}\">\n"; echo "<button type=\"submit\" name=\"answer\" value=\"True\">True</button> \n"; echo "<button type=\"submit\" name=\"answer\" value=\"False\">False</button> \n"; echo "<form>\n"; echo "<br><br><br><br><div style=\"margin-left:30px;background-color:yellow;width:400px;padding:20px;\">"; echo "<b>This section for illustrative purposes only:</b><br><br>"; echo "Pages <span style=\"background-color:#696969;\">used</span>/remaining:<br><br>"; //This section is for debugging illustrative purposes only for ($i=1; $i<=$page_count ; $i++) { if (in_array($i, $pages)) { $bgcolor = "#ffffff;"; } else { $bgcolor = "#696969;"; } echo "<span style=\"background-color:$bgcolor;padding:3px;padding-left:6px;padding-right:6px;border:1px solid #000000;\">$i</span> "; } echo "<br></div>"; } echo "<br><br>Go <a href=\"$_SERVER[php_SELF]\">home</a> and start over."; } ?> -
~~ Updating Arrays stroed in sessions and retrieving them? ~~
Psycho replied to Ryanz's topic in PHP Coding Help
And what was wrong with the solution I provided you in this thread: http://www.phpfreaks.com/forums/index.php/topic,181018.0.html It did exactly what you wanted except it used cookies instead of session. Just change the cookies to sessions and you're golden. Did you even try the code I provided? If so, what does it not do that you need it to? Below is the same code again with session data instead of cookies as well as some add'l changes for clarity. note that most of that code is just there for illustrative purposes to show what is happening. The code allows you to set a finite number of questions for the user and all of those questions will be presented in a random order. The user MUST proceed from one question to the next and cannot manually change the order. <?php session_start(); $page_count = 10; $page = $_GET['page']; if ($page!='Next') { //Create an array with the values from 1 to $page_count $pages = range(1, $page_count); //Randomize the array shuffle($pages); //Save the array to a session variable $_SESSION['pages'] = serialize($pages); echo "You are on the start page. There are $page_count random questions to answer.<br><br>"; echo " Click <a href=\"$_SERVER[php_SELF]?page=Next\">here</a> to start."; } else { //retrieve the pages array from the session $pages = unserialize($_SESSION['pages']); //Get the first from the random list $this_page = array_shift ($pages); //Determine if there are additional pages left $last_page = (count($pages)>0)?false:true; //Save the new pages array to the cookie $_SESSION['pages'] = serialize($pages); echo "<h2>You are on question $this_page.</h2>"; echo "Pages <span style=\"background-color:#696969;\">used</span>/remaining:<br><br>"; //This section is for debugging illustrative purposes only for ($i=1; $i<=$page_count ; $i++) { if (in_array($i, $pages)) { $bgcolor = "#ffffff;"; } else { $bgcolor = "#696969;"; } echo "<span style=\"background-color:$bgcolor;padding:3px;padding-left:6px;padding-right:6px;border:1px solid #000000;\">$i</span> "; } //Display messages based upon whether this is the last page or not. if ($last_page) { echo "<br><br>This is the last question."; } else { echo "<br><br>Go to <a href=\"$_SERVER[php_SELF]?page=Next\">next</a> question."; } echo "<br><br>Go <a href=\"$_SERVER[php_SELF]\">home</a> and start over."; } ?> -
persoanlly I use a single script/page to check the user's login/rights and call that at the beginning of every applicable page.
-
JavaScript cannot access the user's file system.
-
You would want to do that before updating so you know the extension of the old file to be deleted. However, instead of going to all of this trouble I would suggest converting all the images to one file type. Then you don'yt need the database for this at all. Here are a few possibilities: http://www.phpit.net/article/image-manipulation-php-gd-part1/4/ http://www.phpclasses.org/browse/file/8221.html http://phpclasses.ca/browse/package/2073.html
-
First off you should place your query into a variable instead of directly into the mysql_query() command. Makes it MUCH easier to debug later on. In this case I would just use an ON DUPLICATE KEY UPDATE in the query. You can just change your query as follows: $query = "INSERT INTO user_images (user_id, ext) VALUES ('$id', '$file_ext') ON DUPLICATE KEY UPDATE ext = '$file_ext'"; mysql_query($query) or die(mysql_error());