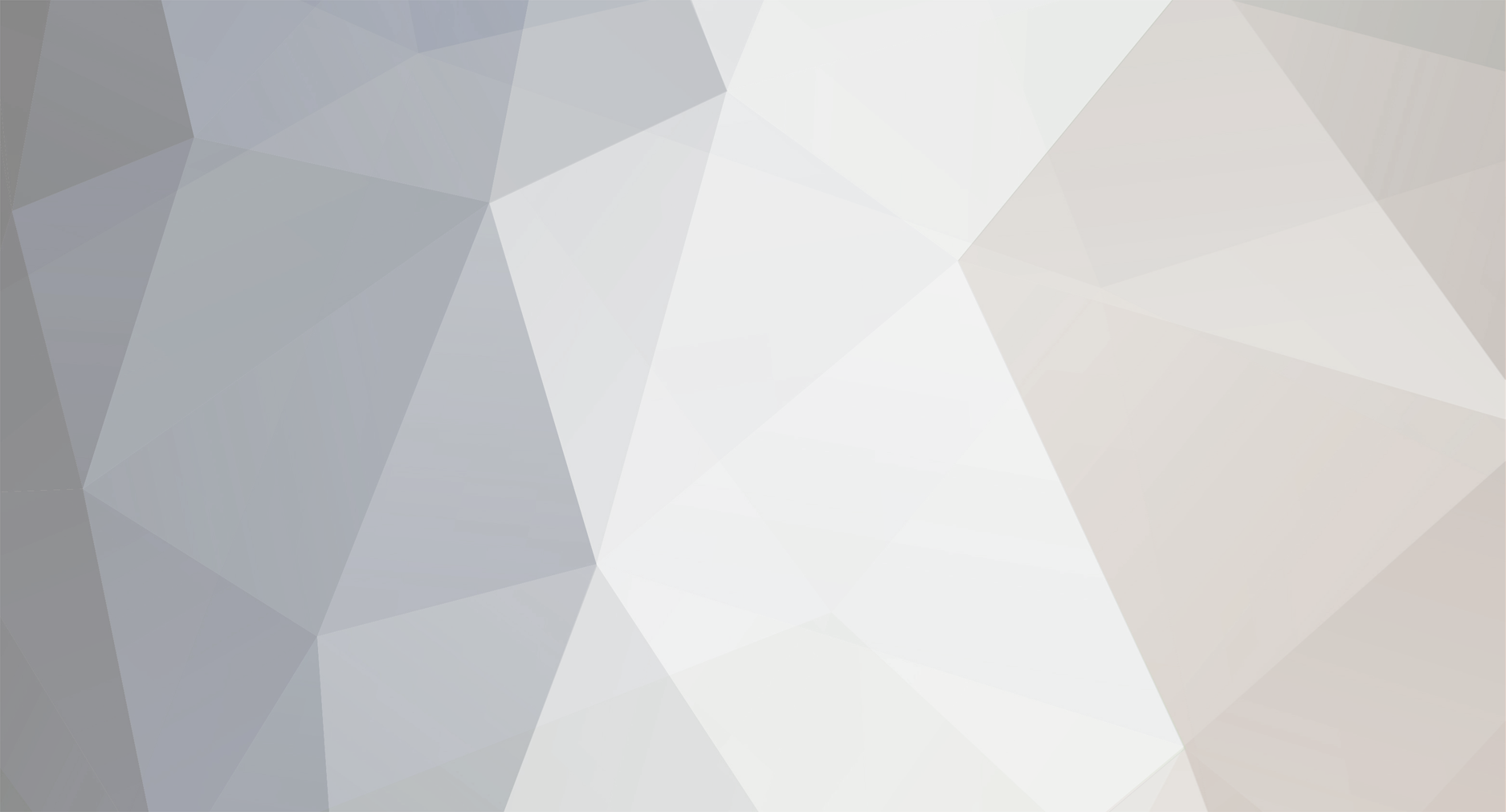
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Yeah, your post doesn't make sense. You state you want to show the listing made by a user on their profile page and you show the URL for that page which includes an ID parameter on the URL string for the user ID. Then you go on to show an input field used on the add listing page for 'submitted by". But, it is an input field, which means the user can type in any value. Shoudln't this be a hidden field using the user ID of the person submitting the listing (or get it dynamically on the processing page)? Then your page to display listings is apparently trying to find listing by the user submitted value for "submitted by". I assume then that you expect the uessr to enter the name of the person who submitted the values. If it was the user's page, then you would run a page to get the listings using the User ID. This looks more like a search of listings by a user - which has nothing to do with your original request.
-
MoFish, You problem doesn't make sense. When you define an array value such as this array('staff_last_login'=>'NOW()') The quotes are used as delimiters to define where the value starts and ends. They are not part of the value. So, if you use that array value in generating your query, there will be no quotes. $data = array('staff_last_login'=>'NOW()'); $query = "UPDATE staff SET staff_last_login={$data['staff_last_login']} WHERE staff_id='1'"; echo $query; //Output: UPDATE staff SET staff_last_login=NOW() WHERE staff_id='1'
-
How to merge several product lines into one
Psycho replied to dennis-fedco's topic in PHP Coding Help
My biggest question would be how is the data set up currently? Are each line in separate tables? Are those tables similarly constructed (i.e. same columns, at least for the same parameters)? If they are in the same tables or the tables are similar enough you can probably use what you have and create new procedures for performing the searches. But, even if the table structures are very dissimilar, you could run multiple "queries" on each product line in a single query to get the results from all lines using a UNION. A complete rewrite will be a huge task, but you would get better, cleaner code (assuming you do it right ). I would ask myself these questions. Aside from the new request are there other non-minor problems with the current functionality? How much time is spent currently maintaining the application? If there are problems for the users how much time or pain are these issues? If it's not broke don't fix it. Ugly inefficient code that works - still works. So, you have to weight the effort of the initial rewrite and the effort to clean up bugs that get missed during that process compared to the current pain to the users and the time you are investing to keep the current application running. -
elseif conditional expression evaluates to TRUE when it should not?
Psycho replied to terungwa's topic in PHP Coding Help
A couple other things: 1. You should trim() the string. 2. You don't need to use a string of if/else statements since if any are true they will do a 'return' and exit the function 3. You can use a switch() to check the return value 4. Be careful of using '0' as a return value when many values can be returned because 0 can also be interpreted as the Boolean false. 5. Think about the order in which you perform the checks since a value can actually meet multiple error conditions. I would do min/max lengths before checking the format. Here are some changes in the logic that I think work better function CheckFullname($string, $minlen, $maxlen) { /* define error codes 100 = wrong pattern match 101 = string is too long 102 = string is too short 0 = empty input */ //Trim the string $string = trim($string); //Check for empty value if(!strlen($string)) { return 0; } //Check minimum length if(strlen($string) < $minlen) { return 102; } //Check maximum length if (strlen($string) > $maxlen) { return 101; } //Check fullname format if (!preg_match('/^(?:[\p{L}\p{Mn}\p{Pd}\'\x{2019}]+\s[\p{L}\p{Mn}\p{Pd}\'\x{2019}]+\s?)+$/u', $string)) { return 100; } // No errors detected return true; } // this function executed below is returning error code 100. $fullName = "John Doe"; $nameMinimum = 4; $nameMaximum = 40; $nameError = false; switch(Checkfullname($fullName, $nameMinimum, $nameMaximum)) { case 0: $nameError = 'Your name is required.'; break; case 100: $nameError = 'Please provide your full name without any funny characters.'; break; case 101: $nameError = 'Your name should be at most 40 characters long.'; break; case 102: $nameError = 'Your name should be at least 4 characters long.'; break; } if($nameError) { echo $nameError; } else { $fullname = safe($string); } -
elseif conditional expression evaluates to TRUE when it should not?
Psycho replied to terungwa's topic in PHP Coding Help
EDIT: Also your usage of the function is redundant: // this function executed below is returning error code 100. $string = "John Doe"; if(Checkfullname($string, 40, 4)== 0) { echo 'Your name is required.'; } elseif(Checkfullname($string, 40, 4) == 100) { echo 'Please provide your full name without any funny characters.'; } elseif(Checkfullname($string, 40, 4)== 101) { . . . Call the function ONE TIME. Then perform whatever actions on that return value. As you have it now you could introduce a bug by accidnetally changing one of the parameters in a function call on one line and not another. -
Prevent database connection as a parameter to all function
Psycho replied to byenary's topic in PHP Coding Help
You could use a singleton factory. Take a look at the first response here: http://stackoverflow.com/questions/130878/global-or-singleton-for-database-connection -
Hundreds of variables? I doubt you really need all of those and it could be streamlines with better code. Otherwise, you should use array variables. E.g. $vars['a'], $vars['b'], etc. Then, at the very least, you could run a loop to zero all of them out or have a template array to set the initial state. But, as requinix stated, it would be nice to see the code as we can probably find a better solution.
-
FYI: If you are doing an ON DUPLICATE KEY UPDATE, there is a way to reference the INSERT values in the UPDATE portion of the query instead of having to reuse the variables. I find this a better approach. If you have a typo in the variable name you may not catch it. But, if you mistype a field reference it would produce an error regardless if whether the INSERT or UPDATE is run. Also, I would highly advise creating your queries as string variables so you can echo them to the page for debugging purposes. Plus, using tabs and spacing to format the query in a readable format helps save a lot of time. E.g. $query = "INSERT IGNORE INTO mxit (ip, time, user_agent, contact, userid, id, login, nick, location, profile) VALUES ('$ip', '$post_time', '$mxitua', '$mxitcont', '$mxituid', '$mxitid', '$mxitlogin', '$mxitnick', '$mxitloc', '$mxitprof') ON DUPLICATE KEY UPDATE ip = VALUES(ip), user_agent = VALUES(user_agent), contact = VALUES(contact), login = VALUES(login), nick = VALUES(nick), location = VALUES(location), profile = VALUES(profile)"; $result = mysqli_query($con, $query) or die(mysqli_error($con));
-
Also, some email clients will disable links based on various rules - typically to prevent the user from clicking malicious links. Have you tried reading the email is different email clients?
-
Yeah, but that would have probably required a JOIN!
-
my check boxes are not being sent in the email
Psycho replied to hammahead's topic in PHP Coding Help
$body .= 'Selected Projects: ' . $selectedProjects; $fields{"contact-comments"} = "Message"; $body = "We have received the following information:\n\n"; foreach($fields as $a => $b){ $body .= sprintf("%20s: %s\n",$b,$_REQUEST[$a]); } Look closely at the first and last lines above. -
As to your code, you do not change the value of the checkbox. And, in this case the value really isn't important. The checkbox should use the same value and you should check/uncheck it based upon the saved data. Also, you should save the value as a numeric equivalent of a Boolean, i.e. 0 (false) or 1 (true). visible: <input type="checkbox" name="inp_vis" value="visible" <?php echo if ($row['vis']) "checked='checked'"; ?>>
-
PHP Fields Logic & Math (input & Select fields)
Psycho replied to studgate's topic in PHP Coding Help
I think I understand the select fields to be just that - HTML select fields. However, I too am perplexed by what is meant by an "unlimited text field with an 'add more' option". Never heard of such a thing. And, if the field is "unlimited" what is the purpose of an "add more" option? In any event, it sounds like you want a JavaScript solution, not PHP. If so, this is in the wrong forum. -
You wrote that incorrectly. It should be "I distract easily and . . . squirrell!"
-
I've been 'scolded' in the past for not looking up from my screen when people come to my cube to talk with me. I can listen to what they are saying and carry on a conversation while working. But, apparently they "feel" I'm not giving the attention they deserve because my eyes are not trained on them.
-
If you are going to build logic to 'calculate' the process date, then you don't need to save it in the database and instead use that calculation when needed. But, I could see where some things may be easier to accomplish if you have that value saved. So, I will not tell you to do it one way or the other, but I will show you how to get the date in question. The logic could look something like this: SELECT -- If the Day of received date is <= 9 IF(DAY(received_date) <= 9, -- Then Use 9th of current month DATE_FORMAT(received_date, '%Y-%m-09'), -- Else use 9th of next month DATE_FORMAT(DATE_ADD(received_date, INTERVAL 1 MONTH), '%Y-%m-09') ) as processed_date Basically we test the "day" of the received date. If it is less than 9 (1-9) then we determine the processed date tot he 9th of the given month. Otherwise we determine it as the 9th of the next month. An update query to set a value in the new column might look like this UPDATE `table` SET processed_date = -- If the Day of received date is <= 9 IF(DAY(received_date) <= 9, -- Then Use 9th of current month DATE_FORMAT(received_date, '%Y-%m-09'), -- Else use 9th of next month DATE_FORMAT(DATE_ADD(received_date, INTERVAL 1 MONTH), '%Y-%m-09') )
-
I don't think this is a networking issue. You have to already be connected to the network in question in order to implement what you want. There is no way for a web page to connect a computer to a different network. You need to have your router redirect users to a page where you will run the logic to determine if the user is "authorized" or not. This is what you would see in a public Wifi. Sometimes you just need to accept some Terms of user and sometimes you need some sort of authentication (e.g. room number and name). I assume the page used for that simply passes back a confirmation to the router in some manner. So, you can probably do this with just PHP, but you would need to know how to configure the router to use your custom page and what data your page would receive and need to pass back to the router. Not to mention, the router would have to support that functionality in the first place. Apparently this functionality is referred to as "Captive Portal" Here are a few links that may help: http://www.bleepingcomputer.com/forums/t/506654/help-wifi-custom-login-page/ http://www.wi-fiplanet.com/tutorials/print.php/3730746 http://www.pcworld.com/article/2031443/how-to-set-up-public-wi-fi-at-your-business.html
-
I'm pretty well versed in networking technology, but I'm no expert. I think you are misunderstanding what is happening. A web page can't "connect" you or redirect you to a wifi network. You have to already be connected to the wifi network to access the login page. This is what is likely happening: You connect to the Wifi network. The router identifies that you are a new 'user' on the network and puts your IP in a restricted access mode. Any request you make over the network is redirected to the login page. Once you complete the login/confirmation process the router will allow your requests to go to the intended destination (e.g. Google). So what you are asking is possible, but comes with several caveats. I know my router allows me to create guest access and will direct users to a login page. But, that page is controlled by the logic in the router's firmware. I.e. I don't control the look and feel of the page and it only checks for a global password that is set through the router's firmware. What I think you need is the ability to have the router redirect the user to a custom page you create so you can authenticate users via parameters you choose (e.g. verify the users identity from a separate database). My router my have that capability, but I don't know and don't care to investigate. If you are using an off-the-shelf router you will want to hit up the manufacturer's site to see if this capability exists.
-
"How" are the files being accessed through the player? Are the files being accessed directly such as mysite.com/mp3s/somefile.mp3, or are they being accessed through an intermediary page such as mysite.com/playmp3.php?id=22? If it is the latter then you can just add code to the page that fetches the mp3 to also update metrics around those requests. E.g. how many requests, when, by who, etc. Basically you can track anything that you want to track as long as you have that data. I.e. you can track who is playing certain files if you have a login system (or you could at least track by IP, although it is a poor approximation of an individual). If the files are not being accessed through an intermediary page, you have some options: 1. You can create an intermediary page and use that instead of allowing them to access the files directly. This is a good idea anyway. 2. You could implement AJAX. But, this seems like a lot of complexity for what you are wanting to accomplish 3. There are server-side logs. Depending on how (and with whom) you are hosting your site, your level of access to those logs may differ. My host gives me access to my logs through a web interface for manual inspection. but, I also have direct read access to the files. So, I could build my own code to parse that data if I wanted. Although this would likely only include IP address and files accessed.
-
Most likely your query is failing. The error is telling you that the method fetchColumn() is trying to be executed against a non-object. In that line of code there is "$odb->query()" being used to execute a query and then "->fetchColumn(0)" is attempting to be executed against that result. If the query fails there is nothing for fetchColumn() to execute against. You have no error handling in that code. IMO, you should never assume queries will pass. You should always include error handling to at least provide the user some feedback. Although you would never want to dump raw error messages to the user in a production environment, just do something such as "a problem occurred, please try again later". But, you can either check for those errors and log them or echo them out in a non-prod environment. I also don't think that running "code" within HTML is a good idea, makes maintainability more difficult. <?php $result = $odb->query("SELECT `Tab` FROM `SiteConfig` LIMIT 1"); if(!$result) { $title = "Unable to retrieve title"; //Add additional error handling to get the real error info } else { $title = $result->fetchColumn(0); } ?> <title><?php echo $title; ?></title>
-
Unable to collate all array values outside for each loop.
Psycho replied to gsingh85's topic in PHP Coding Help
If the first word is unique in all values, I would just convert the array to use those as the index for each item to keep the association tightly coupled: $colors = array("red hello","green this","blue lovely","yellow morning"); $newColors = array(); foreach($colors as $towns) { list($id, $value) = explode(' ', $towns); $newColors[$id] = $value; } print_r($newColors); Output Array ( [red] => hello [green] => this [blue] => lovely [yellow] => morning ) -
No. There should be zero dependency on how you GET the data and how you DISPLAY the data. That is why I built the display function to take an array as a parameter instead of passing the DB Result object. If you pass the object, then the display functionality would be dependent on how you are storing and getting the data. I.e. if you change to PDO then the process of using that data in the function would have to change. You want to separate logic to reduce dependencies. You should reduce the process of getting the DB results and putting into array into a single function/method. Then when you change the manner in which you store or retrieve data you only need to modify that function/method.
-
Well, that was helpful. If you aren't even going to put forth the effort, I don't know why I bother, When I am feeling generous I do test code before posting. But, in this situation it requires a specific DB setup and I am not going to go to that trouble just to test some code to work out some minor errors. I did all of that on-the-fly and I stated as much. Did you look at the HTML source? WHat has changed?
-
$recCount = 0; //Create entity output $output .= "<tr>\n"; foreach($entityData as $data) { $countyCount++; //Open new column, if needed if($recCount%$recPerCol==1) { $output .= "<td valign='top' width='{$colWidth}%'>\n"; } The code is incrementing the variable $countyCount and that condition is expecting $recCount. Change $countyCount++; to $recCount++;
-
@Richard Grant, he already stated he doesn't care about the users with access copying the audio - only that he wants to limit who can access. A simple password protected access model should work fine. @EricBeaudoin, I don't know squat about Joomla. S, if this is a specific Joomla issue I can't help you. But, it may not be a Joomla issue. there are a couple of things to verify first. You say that it works with the first value but not the second. Is this with the .htaccess restriction in place? I have to assume not. The $host parameter would seem to indicate the value of $file is the web address from the user's browser to the file rather than the file system location on the server. If that is the case, that may be part of the problem. You are putting the psp.php file in that same directory - which you are trying to limit access to. Put that file in another location that is not restricted. There are a lot of different things going on here with PHP, file locations, JavaScript, etc. You need to take a systematic approach to finding the problem. I "assume" that the original value is the "web" location to the file rather than the file system location from the server (based on the fact that the first parameter is $host). But, one should never assume. So, echo $file to the page to verify exactly what it contains when it is working. You should be able to copy/paste the value into your browser address to directly access the file (once you remove the .htaccess restriction). Once you have verified that is indeed what the value contains, change it to instead point to the psp.php file and echo it to the page. Then, copy/paste that value into your browser to ensure the file is being called correctly (change it to just echo a statement). Once you have verified, that $file is pointing to the correct script and the script is executing, change the script to "load/read" the mp3 files. Test the functionality by directly calling the script. Once that is all working, then call the file through the above code and see if it works. If so, put the .htaccess restriction back on the folder and test again.