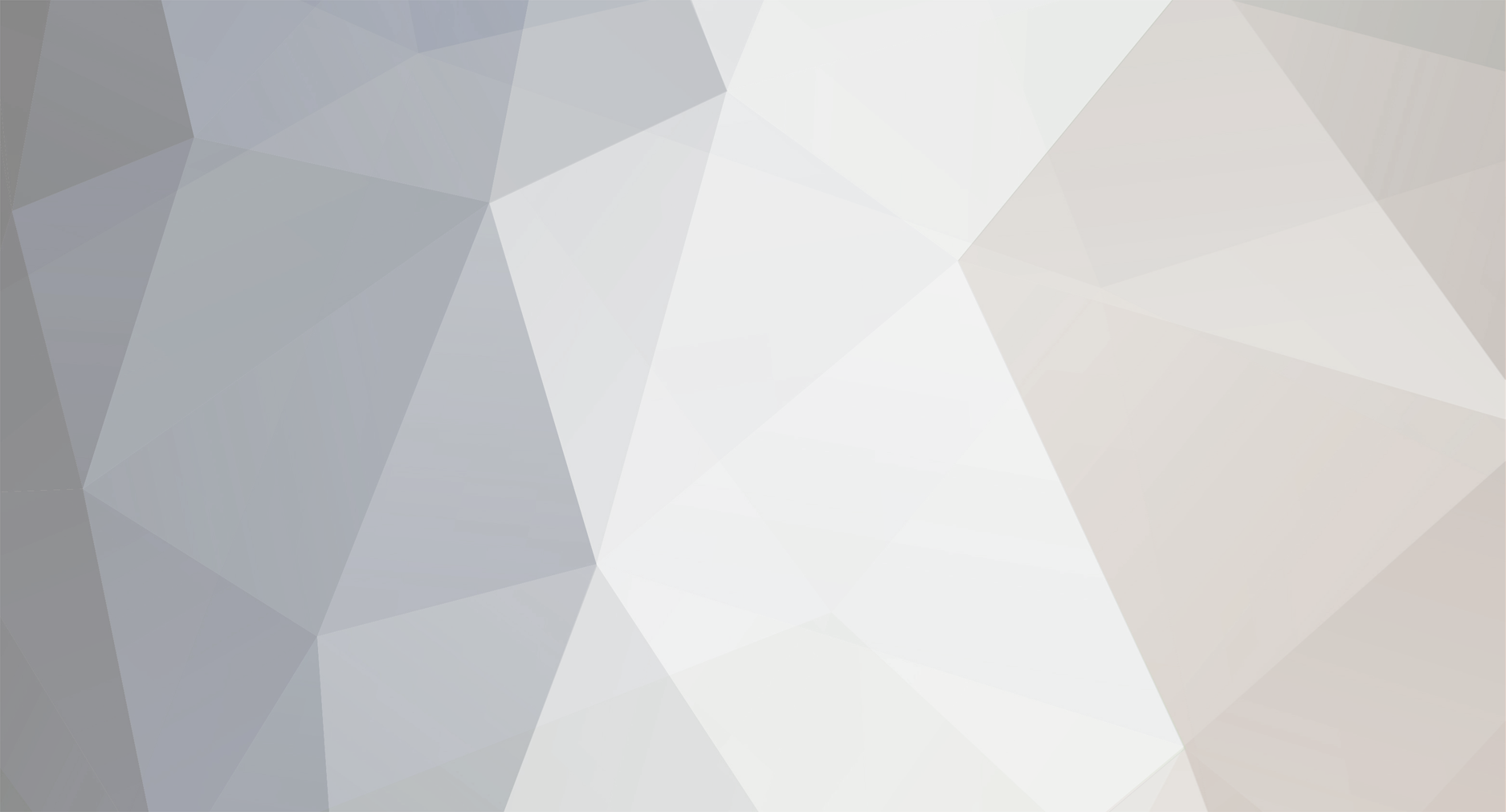
Psycho
Moderators-
Posts
12,157 -
Joined
-
Last visited
-
Days Won
129
Everything posted by Psycho
-
Creating a varied number of dropdown lists dynamically
Psycho replied to cbassett03's topic in HTML Help
The answer is - it depends. It's difficult to really provide a "best" solution without knowing all the particulars. I see three possible options: 1. Create ALL the drop-downs ahead of time and just us JS to hide/display as needed. 2. Dynamically create each of the drop-downs dynamically using just client-side code. I'd probably save all the data for all the levels in a multi-dimensional array. 3. Create the drop-downs dynamically using AJAX. Unless you have a small number of drop-downs and levels, I'd go with #2 or #3. Having all the drop-downs created ahead of time would make it hard to process the data because you have to check the first field to determine the next field to process. If you dynamically create the options you can create them in a logical format to make processing easier. As for #2 or #3, #2 would be better performance. But, if you have a ton of data then it might be more efficient to go with #2. -
I see a many problems. But, I'm not really going to go through line by line since it would take me quire a while. You are trying to get the IDs to update using a hidden field, but that makes no sense since the user cannot modify those fields (directly). foreach($_POST["id"] AS $key => $val) { $id = $val; But, the id field is not an array, so you should get an error on the foreach() loop. Plus, you should not run multiple queries to make the updates. Instead you should do an UPDATE using an IN() listing all the IDs to update. On another note, you need to code more efficiently. For example you have this: if ($row2[groupmember]=="No") { echo "<input type=\"hidden\" name=\"id\" value=\"$row2[id]\" /><tr><td>$row2[deckname]</td><td>$category[$cat]</td><td><input name=\"reme[$row2[id]]\" value=\"1\" type=\"radio\">Yes</td><td><input name=\"reme[$row2[id]]\" value=\"0\" type=\"radio\">No</td></tr>\n"; } else { echo "<input type=\"hidden\" name=\"id\" value=\"$row2[id]\" /><tr><td>$row2[set2]: $row2[deckname]</td><td>$category[$cat]</td><td><input name=\"reme[$row2[id]]\" value=\"1\" type=\"radio\">Yes</td><td><input name=\"reme[$row2[id]]\" value=\"0\" type=\"radio\">No</td></tr>"; } The if and else are basically the same with a minor change. You should instead do this $label = ($row2['groupmember']=="No") ? $row2['deckname'] : "{$row2['set2']}: {$row2['deckname']}"; echo "<tr>"; echo "<td>{$label}<input type=\"hidden\" name=\"id\" value=\"{$row2['id']}\" /></td>"; echo "<td>{$category[$cat]}</td>"; echo "<td><input name=\"reme[{$row2['id']}]\" value=\"1\" type=\"radio\">Yes</td>"; echo "<td><input name=\"reme[{$row2['id']}]\" value=\"0\" type=\"radio\">No</td></tr>\n"; echo "</tr>\n"; echo "</table>\n"; Note, I broke up the echo to improve readability and I moved the hidden input into a TD because it creates invalid code to put elements in-between table elements.
-
<?php //Insert code here to dynamically set the page title $pageTitle = "Today is " . date('m-d-Y'); ?> <html> <head> <title><?php echo $pageTitle; ?></title> </head> <body>
-
You have two options. You can either have the date formatted within your query - so you don't have to do anything with the value once returned. Or, you can format it within PHP. There are benefits and drawbacks to both and the determination would be based on many factors (e.g. do the date/times need to be timezone aware for the user). But, in either case, you can format the date in any manner you wish. strtotime() is only needed to convert the date string to a timestamp - it is the date() function that determines what the format it: http://php.net/manual/en/function.date.php For the format "08 July 2013 12:24:04", you would use "d F Y H:i:s" - this assumes you want the hour n 24 hour format. date("d F Y H:i:s", strtotime($result['cdate']))
-
I was referring to this in the last code you submitted: $user_query = mysql_query("SELECT `user_id`, `name`, `email` FROM `users` WHERE `user_id`=".$_SESSION['user_id'].""); The query is written directly in the mysql_query() call, so if there were errors you can't echo the query to verify it. There isn't even any error handling on the query execution anyway. And, even though session values should be fairly safe to use w/o validation, you should always validate/escape values that could cause errors. In this case, you could be using prepared statements (MySQLi or PDO) or you can format the value to be in integer before running the query. You shoudl definitely look at using MySQLi or PDO for database handling, but there will be a learning curve. I missed removing that comment in my response. I really isn't good practice, but passwords are unique. What I was getting at is you shouldn't typically do a empty() check on POST values directly to verify if the user made an entry into a field. The reason is because spaces are not considered "empty". So, if you have a name field that is required, you would not want the user to use spaces for a name. Therefore, you should first trim() the values and then test them. However, passwords are different. In fact, you should almost always trim() user entered data. But, passwords are a little different. Unlike trimming the value for a user's name, if you were to trim a password, it is really changing what the password is. Now, it could be argued that as long as you are always trimming the password upon creation and when the user logs in that it would always work - and that is true. But, removing anything from a password could decrease the complexity of the password. For example, let's say the user made their password "__password__", with two leading and trailing spaces. If you were to trim the value, then their password would just be "password" making them much more open to being compromised.
-
I already gave you some tips and you didn't want to implement those (e.g. creating your query as a string variable so you can echo to the page for debugging). But, regardless, here are some other tips. 1. Give your variables meaningful names. Your function getuser has the variable $getUser. That doesn't give you any indication what the value really is. It should be $userID (or something like that). 2. Don't assume your queries will always work. Add error handling as shown previously. You will save yourself hours of hair pulling when you do hit a snag. 3. In your function getuser() you have a while() loop to process the DB result. First, you don't verify if any records were even returned. Plus, do you expect to have more than 1 record returned? No need for a while loop if there will only be one record. This is a waste of code: While ($user_row = mysql_fetch_assoc($user_query)){ $user[] = array( 'userid' => $user_row['user_id'], 'user_name' => $user_row['name'], 'user_email' => $user_row['email'] ); } return $user; Just do this (after implementing error handling for failed query and no records returned): $user = mysql_fetch_assoc($user_query); return $user; 4. This seems to have no purpose since use should only ever be one person. Doesn't do anything anyway. //// loop through array and return values foreach ($user as $individual){ } 5. OK, now I see why you have the above $email = $individual['user_email']; $user_id = $individual['userid']; . . . and what is the problem with just referencing $user['email'] and $user['user_id']? All you are doing is creating a lot of duplicative data in different variables. 6. This is not a good validation if(!empty($_POST['password'])){ A value of a space will OK, I'm not going to go through any more as it will be too time consuming. So, I will leave you with this. Take your time when determining the process flow in your logic. Get out a piece of paper and determine what data you need and when. Figure out any branching logic (e.g. if/else) and where it makes sense to do it - and ensuring you don't have any dead ends. Then, write your code. In your validation you do some checking of the password from the POST data and put a value into a variable. But then later you do another check on the POST value - you should instead check the value you set earlier.
-
That is nothing like what you originally posted. Are you hashing the password before you do this? if($password !== null) If so, that will always return true since the hash of an empty string is NOT an empty string. But, I already provided updated code that will check for errors. Are you using that? Are you getting errors? We can't tell you what is wrong if you don't provide the information needed.
-
Always create your queries as a variable first so you can echo them to the page for debugging purposes. In this case I think one of two things is happening. Either the query is failing because it has an error - and you are not checking for errors. In fact, why are you stating "successful update" before you even make the update?! Or, there is no record that matches the value of $_SESSION['user_id'] - it may not even be set. Also, do NOT use mysql_real_escape_string() on a value that is going to be hashed! The hashing will prevent any sql injection and it will lead to problems later. Looking closer at your code it is obvious it is failing. You are trying to include the hashed password in the query, but the md5() is not being run because it is inside a string. Plus, you need a comma between each field. Try this <?php $email = mysql_real_escape_string($user_email); $password = md5($password); ## Note: md5() is not suitable for password hashing $query = "UPDATE users SET email = '{$email}', password = '{$password}' WHERE `user_id` = {$_SESSION['user_id']}"; $result = mysql_query($query); if(!$result) { //Query failed echo "Query failed!<br>Query: {$query}<br>Error: " . mysql_error(); } elseif(!mysql_affected_rows()) { //No record was updated echo "No records updated!<br>Query: {$query}"; } else { //Record was updated echo 'successful update'; echo $user_email; echo $password; $_SESSION['user']['email'] = $_POST['email']; echo $_SESSION['user']['email']; } ?> Edit: Note that the two error conditions above are only for debugging purposes. You should use generic messages for users in real use and not provide any actual errors from the system.
-
MySQL Select x FROM y WHERE z="a", b="c", d="e";
Psycho replied to SteelyDan's topic in PHP Coding Help
I was going to state something similar to Zane. Instead of having unique fields to search just by buildname or just by weapon, etc. you could instead have a single field for the user to enter their search values and compare against all the relevant columns. However, this is a business decision. It may actually result in a better, more user friendly experience. But, it could also result in some false positives. If the user needs explicit filtering (e.g. buildname is exactly a specific value and weapon is a specific value) then you probably need to stick with what you have. However, as to why you weren't getting any results, doing an equal comparison to an asterisk is NOT a wildcard. It will look for matches that are exactly an asterisk. The asterisk is only a wild card when doing a LIKE comparison. Also, if you go with your previous logic, instead of doing a comparison of the wildcard - just remove the comparison completely. -
how to know if the chosen date range is exactly 1 month
Psycho replied to sasori's topic in PHP Coding Help
If the user is limited to only selecting a month, then why have two date pickers? Or, are you saying the user can select a range "up to" one month, but not more? If the user can only select a single month, then only give them a start date and programatically determine the month period for them. If the user can select a range "up to" one month, then you can determine if they exceeded a month using strtotime() with the "+1 month" modifier (you would use the same thing if you go with the single date picker). A few comments: 1) Although you definitely want to add validation server-side, you should do the same client side. Your date picker should have the ability to limit the available dates. So you can update t he second datepicker when a user makes a selection from the first. You would also need to handle when a user changes the first date picker to update the second. 2) You need to determine what to do about selections at the end of the month. For example, if a user selects January 31st and the first date, what is the max for the second date? Feb 28/29th? You will likely need to add specific logic to handle these situations.- 2 replies
-
- jquery
- datepicker
-
(and 2 more)
Tagged with:
-
Multiple values in drop down submitted as two fields?
Psycho replied to BSlover's topic in PHP Coding Help
Well, you should begin by not doing this. You should only pass the ID. Doing it the way you propose can cause data consistency issues. You can always determine the meta data (e.g. the name) by using the ID. Not knowing the specifics of how your contact form is used, I can't give you a qualified solution. If your contact form is sending an email and you want the toy name in the content of that email, then the page that receives the value from the form should do a query to look up the toy name. However, if the contact form is used to create a record/message in your database where there will be an associated "toy" then you should store the toy ID as a value associated with that record. Then when a user views that record you could dynamically retrieve the toy name. To expand on why you should not do this, here is just one example: Let's say the contact for is for a user to send an email to a support rep about a particular toy. Plus, let's further assume that toy names will not change often (if at all). Then the only way the ID and Name could be incorrectly passed would be: 1) If there is a code error that incorrectly sets the value for the select options (which could easily happen if the names have certain characters and aren't correctly handled). 2. The user "hacks" the form to send different data than what you intended. Never trust data that a user submits (POST, GET, COOKIE, etc.). Don't assume that because you created a select list that the user necessarily submitted a value from that list. It is very easy to submit any values you wish, regardless of what is in the form,. -
I have to disagree with that. That makes it difficult to gracefully handle errors and can result in invalid output. Also, it makes it so execution stops on the first error encountered instead of telling the user all the errors that need to be resolved. A better approach, IMHO, is to perform all the necessary validations and use a flag or some other process to make a determination as to whether or not to process the results or to show the errors. Also, no need to run the query to check if the username is a duplicate if it doesn't meet the format test. There are other problems as well. For example, you can't send content to the page and then do a header(). Also, you are not escaping your input for use in queries. And, the password is not being hashed. I didn't fix all the problems below. <?php include 'config.php'; //Preprocess input $username = isset($_POST['username']) ? trim($_POST['username']) : ''; $password = isset($_POST['password']) ? $_POST['password']: ''; $email = isset($_POST['email']) ? trim($_POST['email']) : ''; $ip = $_SERVER['REMOTE_ADDR']; //Create array to hold the errors $errors = array(); if(strlen($username < 4)) { $errors[] = "Error 5215: Username contains less than 4 characters."; } else { $usernameSqlSafe = mysql_real_escape_string($username); $sql = "SELECT username FROM users WHERE username = '$usernameSqlSafe'"; $res = mysql_query($sql); if(mysql_num_rows($res)) { $errors[] = "Error 21663: Username already exists in database."; } } if(strlen($password < 4 )) { $errors[] = "Error 5215-1: Password contains less than 4 characters."; } if(strlen($email < 4)) { $errors[] = "Error 5215-2: Email contains less than 4 characters. "; } if(count($errors) { echo "The following error(s) occured:<br><ul>\n"; foreach($errors as $err) { echo "<li>{$err}<li>\n"; } } else { $passwordSqlSafe = mysql_real_escape_string($username); $emailSqlSafe = mysql_real_escape_string($email); $sql = "INSERT INTO users (username, password, email) VALUES ('$usernameSqlSafe', '$passwordSqlSafe', '$emailSqlSafe')" mysql_query($sql); header("refresh:5;url=login.html"); echo "Success! Redirecting...."; } ?>
-
Try the query I gave you in PHPMyAdmin or whatever DB management app you are using. Then go from there.
-
$query = "SELECT cid, pid, cname, IF(pid=0, cid, pid) as sortID FROM categories ORDER BY sortID, pid"; $result = $DB['DB_Database']->query($query); while ($row = $DB['DB_Database']->fetch_assoc($result)) { if($row['pid'] == 0) { //Insert code for displaying parent category } else { //Insert code for displaying child cateory } }
-
PHP Show a Text box for each row and update all with 1 submit button?
Psycho replied to Stalingrad's topic in PHP Coding Help
This is totally off the cuff and not tested. I don't have your database and I'm not going to take the time to create one. Here is how you can create the form with inputs for all the items in one form. Note that the id of each record is used as the index of the field name <?php if($action == "stock") { $sql = "SELECT uitemid, price, name, image FROM uitems JOIN items ON uitems.theitemid = items.itemid WHERE username='$suserid' AND location='2' GROUP BY theitemid"; $result = mysql_query($sql); $formHTML = ''; while($row = mysql_fetch_assoc($result)) { $formHTML .= "{$row['name']}<br><img src=/images/items/{$row['image']}><br>\n"; $formHTML .= "<input type=\"text\" name=\"prices[{$row['uitemid']}]\" value=\"{$row['price']}\" /><br><br>"; } ?> <a href=?action=edit>Edit Shop</a> | <a href=?action=view&user=$suserid>View Shop</a> | <a href=?action=stock>View Stock</a> | <a href=?action=quick>Quick Stock</a> <br><br> <font size=5>Stock Shop</font> <br><br> <form action="<?php echo "$PHP_SELF"; ?>" method="POST"> <?php echo $formHTML; ?> </form> Here is how you could process the form to update the values in the DB <?php if(isset($_POST['prices'])) { //Process user input $updateValues = ''; $updateIDs = array(); foreach($_POST['prices'] as $uitemid => $price) { $uitemid = intval($uitemid); if(!$uitemid) { continue; } $updateIDs[] = $uitemid; $price = round(floatval($price), 2); $updateValues .= "WHEN {$uitemid} THEN {$price} \n"; } $uitemidList = implode(',', array_keys()); //Create ONE query to update all the values $sql = "UPDATE uitems SET price = CASE uitemid {$updateValues} WHERE username='$suserid' AND location='2'"; $result = mysql_query($sql); } ?> -
I'm guessing the problem is this: $image.getWidth() and $image.getHeight() As far as I know, that is not a valid way to get the dimensions of an image identifier (if you can get them at all from an identifier). Try using getimagesize() on the file ($CACHE_FILE_PATH), then use the returned values in the function. list($originalWidth, $originalHeight) = getimagesize($CACHE_FILE_PATH); $new_image = imagecreatetruecolor($width, $height); imagecopyresampled($new_image, $image, 0, 0, 0, 0, $width, $height, $originalWidth, $originalHeight);
-
Since you want the list of countries to be concatenated with a comma, you could just implode() the countries instead of doing a second foreach() loop. foreach($continents as $continent => $countries) { $countriesList = implode(', ', $countries); echo "In {$continent} there are: {$countriesList}.<br>\n"; }
-
Admin? The "Admin" is responsible for administrating the forums - not for answering your questions. Everyone here is a volunteer and we respond out of our own willingness to do so. You are not entitled to a response and being insistent is a sure way to encourage people NOT to respond. You were already given an explanation of what causes the header error you initially reported. Your last report stated you are getting "errors or warning" without clarifying which you are getting, whether they are the same header() error as before, or a different one. But, assuming you are getting the same type of header error - we already told you what causes that. You cannot output any content before sending any header commands. The error should state what line number where this is happening. You need to find that line and rework your logic so that line can be executed before you output any content. I already gave you a method of doing that. Put all of your logic at the top of the page. Do NOT use any echo statements or have any content outside of PHP tags. For any output that you need that code to create - have it stored in a variable. Then, after all of the logic of the page has completed, then start your output and use the variables to echo the variable content to the page. As for why you may not get errors in one environment and do in another, consider this: echo "Some string"; if(!isset($_COOKIE['foo'])) { header("Location somepage.php"); } else { //Do something else } If you had already set that cookie while testing in your dev environment you would not trigger the header() command. But, when you move the code to another environment, that cookie would not be set for that environment and the header() command would be attempted - and produce the error. This would mean 1) You did not take the instructions provided previously to fix all the related errors - only the ones you were seeing at the time, and 2) you are not testing your code appropriately. You need to test every "branch" of your code. Anywhere there is an if/else, switch(), or any logic that would do something different in different situations, you need to simulate the conditions to test all of those branches.
-
You are getting that error because your error reporting is set to a high threshold, which it should be for developing purposes. But, they would be lower in production. When you try to reference a variable that doesn't exist - it is an undefined variable or, in this case, you may be referencing the index of an array where the array exists but the index does not. For example: if($_SESSION['msg']['reg-err']) If that session value has not been set that if() statement would generate that error. TI don't know what the intent was for that condition. If the intent was to check if the variable was set, then you should use if(isset($_SESSION['msg']['reg-err'])) But if the intent was to check if the value was not false, then you should first check if the variable is set, then check if it is not false. if(isset($_SESSION['msg']['reg-err']) and $_SESSION['msg']['reg-err'] != false) In a production environment set to a lower error reporting threshold, that code would probably work just fine, but it is poor coding practice. But, looking at that tutorial, I would suggest finding a better one.
-
SELECT *, DATEDIFF(DATE_ADD(date_calib, INTERVAL calib_freq day), NOW()) AS days_till_calib FROM calibrated_items This will give you ALL the records fom the database along with the number of days until calibration is needed. A negative number will mean it has past the date EDIT: To explain this. We first take the last calibration date and the calibration frequency and use DATE_ADD() to calculate the next date that calibration is due. Then we take that date and NOW() and use the DATEDIFF() function to calculate the number of days between now and the next calibration date. EDIT #2: I just realized there is a simpler solution. Calculate the number of days that have passed since the last calibration (using DATEDIFF() ), then just subtract that from the frequency. SELECT *, (calib_freq - DATEDIFF(NOW(), date_calib)) AS days_till_calib FROM calibrated_items
-
htmlspecialchars() is NOT an appropriate manner to prevent sql injection and will actually prevent searching on some values. //Set searchtest from POST if exists, else set to empty string $searchText = isset($_POST['searchText']) ? trim($_POST['searchText']) : ''; //Create WHERE clause $WHERE = ''; if(!empty($searchText)) { $searchText = mysql_real_escape_string($searchText); $WHERE = "WHERE FirstName LIKE '%{$searchText}%"; } // query with simple search criteria $sql = "SELECT * FROM persons {$WHERE} ORDER by RAND() LIMIT $offset, $per_page"; $query = mysql_query($sql);