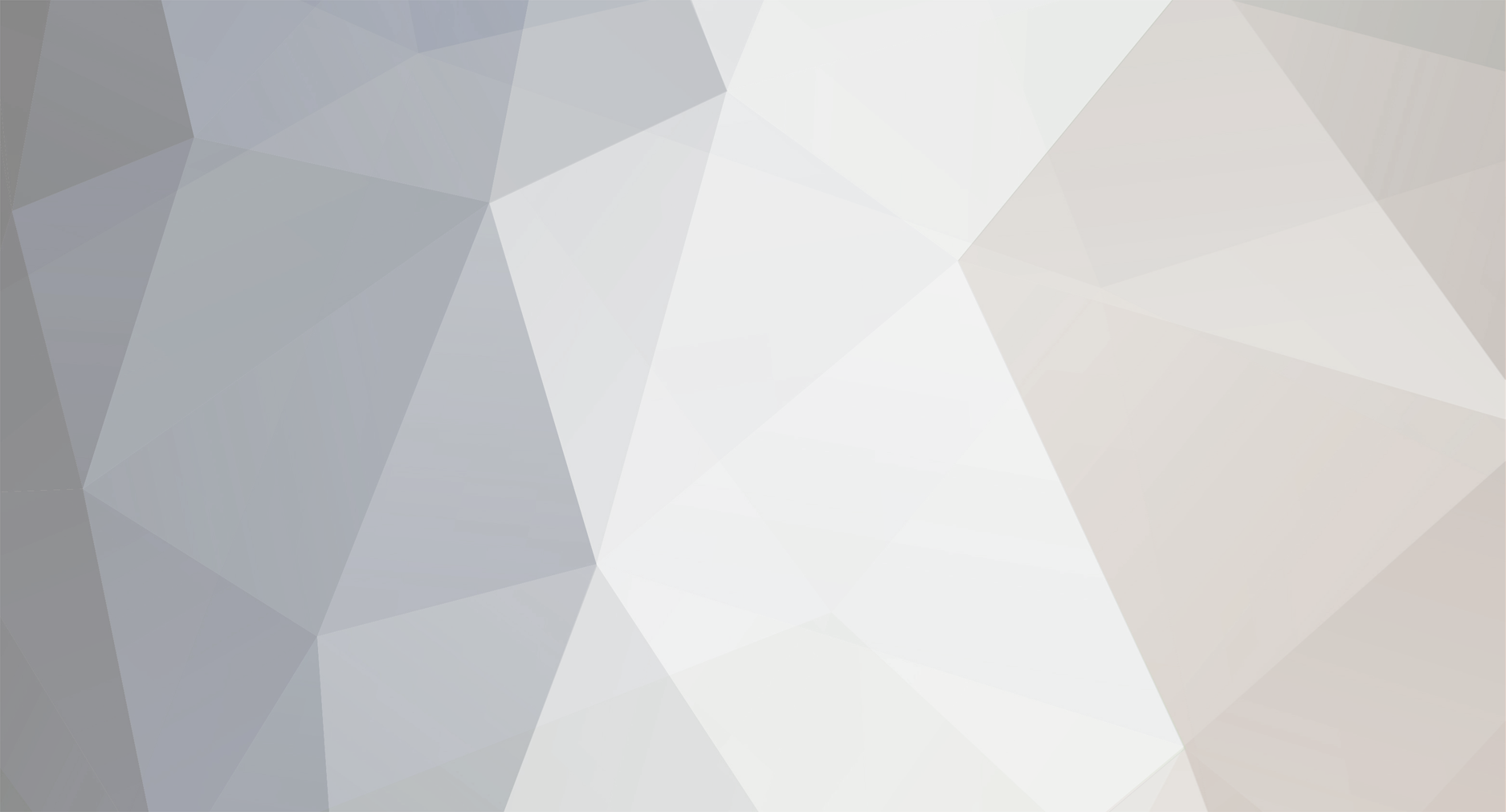
php_joe
-
Posts
175 -
Joined
-
Last visited
Never
Posts posted by php_joe
-
-
Yes, that's better.
Thank you.
-
This may be a stupidly ignorant question, but is there a simple way of switching two members in an array without disturbing the rest of the array?
For example:
<? // Begin with: $array = array('one', 'two', 'three', 'four'); // End with: $newarray = array('one', 'three', 'two', 'four'); ?>
I guess I could do something like this:
<? // Begin with: $array = array('one', 'two', 'three', 'four'); foreach($array as $key => $value) { if($key == 1) $newarray[$key] = $array[$key+1]; else if($key == 2) $newarray[$key] = $array[$key-1]; else $newarray[$key] = $value; } ?>
-
Can't you use fgets()?
http://www.php.net/manual/en/function.fgets.php
<?php $handle = fopen("file.txt", "r"); $firstline = fgets($handle); echo $firstline; ?>
Thank you!
This looks like what I'm looking for.
-
To take it one step further you could strip out any non-numerical characters in case there was a typo:
<?php $letters = preg_split('//', $var, -1, PREG_SPLIT_NO_EMPTY); foreach($letters as $key => $letter) if(!is_numeric($letter) && $letter != '.') unset($letters[$key); if($letters) $checked_var = implode("", $letters); if (is_numeric($checked_var)){ echo "The value is numeric."; } else { echo "ERROR: The value you entered is not numeric!"; } ?>
-
Exactly!
I've got a rather large file full of information. The first page that you see has a search form on it that allows the viewer to search for information within each column, and it uses the first line of the document for the search topics. At the moment I'm using file() and then just $line[0] to get the info but I'd like to have the page load faster if it's possible so I was looking for a way to avoid loading the entire document when it's not needed.
-
You can't do that with the file() function, neither can I think of one that you can - Although not a perfect solution, could you set the maxlen parameter of the file_get_contents() function? Or would that be too vague?
That's what I'll probably end up doing, I was just hoping for something a little cleaner.
-
is there a way to read a text document into an array, like with the file() function, but have it stop reading the file after just one or two lines?
Example: If I wanted to read just the first line of the following text?
Text:
Header1, Header2, Header3
Dog, Big, Loyal
Cat, Medium, Aloof
Mouse, Small, Scavenger
I know that fread can be stopped after a specified number by bytes has been read. is there a way to have it read until it hits a "\n"?
-
I'm not sure how it would work, but couldn't you use nl2br() to change the lines into <br /> and then use preg_match() to replace the <br /> between the <pre> and </pre> back into \n?
-
<?php ///////// To display GM date ///////// echo gmdate("M d Y H:i:s"); ///////// To convert a date to GM date ///////// echo gmdate("M d Y H:i:s", mktime($hour, $minute, $second, $month, $day, $year)); ?>
-
can't you also use border-collapse:separate;empty-cells:show; in your CSS to show empty cells?
-
Hi,
I want to position an image over a frame or iframe so that the contents of the frame/iframe can move up and down, back and forth, but the image doesn't.
Anyone know how I can I do this?
Thanks!
Joe
-
Ok, I got it working.
Thanks a lot!
<? $filename= "./image.jpg"; list($w, $h, $type, $attr) = getimagesize($filename); $src_im = imagecreatefromjpeg($filename); $src_x = '0'; // begin x $src_y = '0'; // begin y $src_w = '100'; // width $src_h = '100'; // height $dst_x = '0'; // destination x $dst_y = '0'; // destination y $dst_im = imagecreatetruecolor($src_w, $src_h); $white = imagecolorallocate($dst_im, 255, 255, 255); imagefill($dst_im, 0, 0, $white); imagecopy($dst_im, $src_im, $dst_x, $dst_y, $src_x, $src_y, $src_w, $src_h); header("Content-type: image/png"); imagepng($dst_im); imagedestroy($dst_im); ?>
-
I tried that. when I use the code that you posted I get:
Warning: getimagesize(Resource id #4): failed to open stream: No such file or directory
-
Hi,
I have an image on my site that I want to copy a chunk out of and save. Preferably I'd like a function that will just copy and save a section starting at an x/y location and ending at an x/y location in the image.
I have read the page on php.net for imagecopy() and I just can't seem to get it to work (I always have trouble with the image codes).
I keep getting this:
Warning: imagecopy(): supplied argument is not a valid Image resourcethis is what I have so far:
<? $img = "./arkijah.jpg"; list($w, $h, $type, $attr) = getimagesize($img); $temp = imagecreatetruecolor($w, $h); $white = imagecolorallocate( $temp, 255, 255, 255 ); $crop_top = '10'; $crop_left = '10'; imagecopy($temp, $img, 0, 0, $crop_top, $crop_left, $w, $h); imagefill( $temp, 0, 0, $white ); ?>
The only think I can think of is that $img is not a "truecolor" image, whatever that is. However, when I try to make it truecolor, I can't seem to get the height & width any more.
Any suggestions?
-
Hi,
I know how to use an external javascript file:
<script language="javascript" src="external_file.js"></script>
The thing is that some of the variables will be constantly changing (via php).
JavaScript doesn't seem to have anything equivilant to PHP's file() or file_get_contents() functions for local files, right? So I thought I could put the php on an external javascript code and have it loaded via a function.
Is there a way to load a script from an external file (located locally) each time a function is used?
Thanks,
Joe
-
yes, except for one or two cases, the maps would be much simpler than mazes.
I guess that one solution, that would prevent getting stuck in a corner, would be to:
- first check if a direct route is possible, if not:
- find a route along the edge
- count the steps
- check each possible route, giving up when the step-count exceeds that of the edge route
Or if a direct route is not possible you could attempt a direct route, walking around obsticles, and then use that route's step-count to search for a shorter route.
-
Thanks for the great posts guys, you've given me a lot to work with.
now if I can just manage to wrap my brain around it :-\ (why do I always pick such difficult projects???)
-
Please post back with details of what exactly you're trying to do.
I play a game called Dark Swords (see my sig), which I like and I'd like to make one that is similar (but not a copy, I'm not a thief).
The game is a 2-D game with a map layed out as a block-style grid. you can walk around by pressing the arrow keys on your keyboard or "auto-walk" to a distant location by double-clicking on that square on the map.
I think that I can figure out how do duplicate most of the game's functions using PHP and JavaScript, but I'm having trouble figuring out their auto-walk feature...
-
I'm looking at tom's pages now. I have no desire to re-invent so much as a screw.
This is for an RPG that I'm trying to build. It's a simple grid layout and I want the characters to have the ability to click on a point and walk there.
-
php_tom:
Eventually it will be getting pretty large.
I have considered using that method, and breaking the map down into areas. I could have the shortest route through each area preset, so the script would only have to find the shortest route out of the starting area and to the final point in the final area.
I was just hoping that there was a better way.
-
Ok, I have a database with x/y coordinates, like a grid.
I'm trying to write a script that will find the shortest path from one point to another on the grid.
For example, if I remove some of the paths to make it look like this:
Then the scrip might come up with something like this:
I'm just getting started on this, so any suggestions are appreciated
-
<?php echo '<select name="rate">'; $rate = 10; while ($rate <= 1000) { echo "<option value=\"$rate\">$rate</option>\n"; $rate = $rate + 10; } echo '</select><br />'; ?>
-
for large mailing lists read this:
http://pear.php.net/package/Mail,
http://pear.php.net/package/Mail_Queue
and for attachments read this:
-
Ok, I have this function for changing bbcode into html:
<? function bbcode($string){ $find = array( '/\[ol\](.*?)\[\/ol\]/is', '/\[ul\](.*?)\[\/ul\]/is', '/\[li\](.*?)\[\/li\]/is' ); $replace = array( '<ol>$1</ol>', '<ul>$1</ul>', '<li>$1</li>' ); $output = preg_replace($find, $replace, $string); $output = nl2br($output); return $output; } ?>
This works great if there are no line breaks between the [ol] & [/ol] tags.
What I'd like better is to be able to have a list between the [ol] and [/ol] tags:
[ol]one two three[/ol]
and have it change to:
<ol> <li>one</li> <li>two</li> <li>three</li> </ol>
witout having to use the [li] [/li] tags and without any br tags in the output list.
Any suggestions?
Do you copyright your web sites?
in PHP Coding Help
Posted
It's automatic under US law too, but it's always a good idea to put it into your footer. With the law documentation rules.